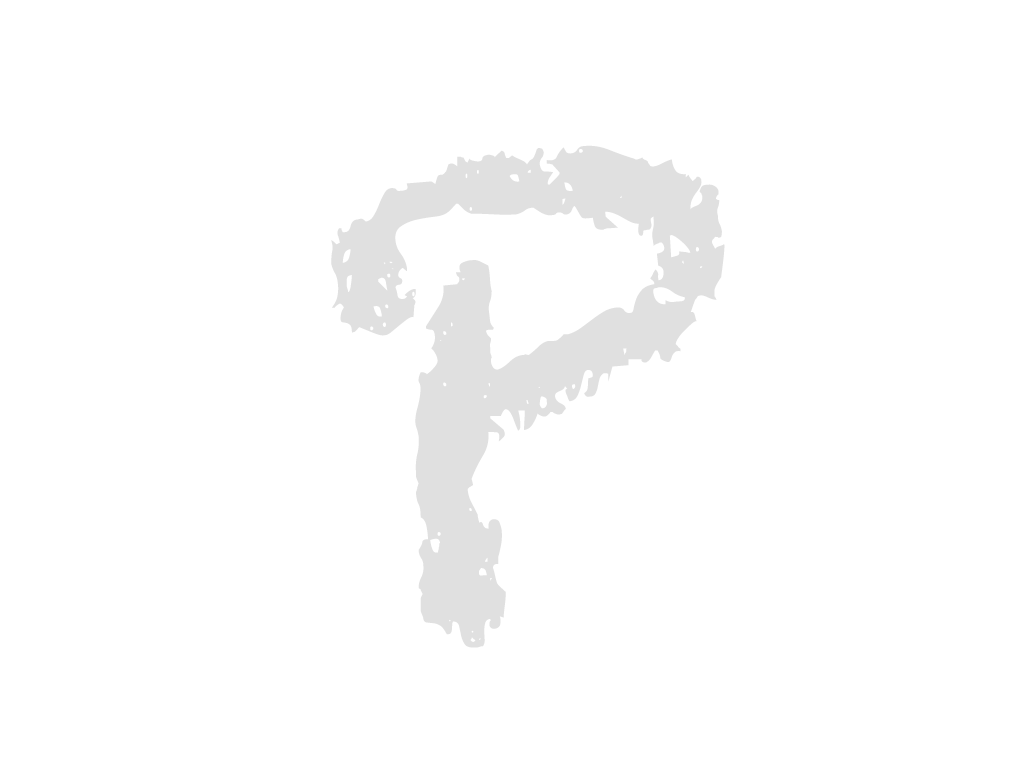
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
2024-11-19
2024-11-19
<template>
<div class="title-box flex justify-between mb40">
<p class="title">단어장 수정</p>
</div>
<div class="content-t">
<div class="board-wrap">
<div class="flex align-center mb20">
<div class="flex align-center">
<label for="" class="title2">교재</label>
<select name="" id="" v-model="selectedBookId" @change="fetchUnits" class="mr10">
<option value="" disabled>교재를 선택하세요</option>
<option v-for="book in books" :key="book.book_id" :value="book.book_id">
{{ book.book_nm }}
</option>
</select>
<select name="" id="" v-model="selectedUnitId" @change="fetchTexts" class="mr10">
<option value="" disabled>단원을 선택하세요</option>
<option v-for="unit in units" :key="unit.unitId" :value="unit.unitId">
{{ unit.unitName }}
</option>
</select>
<select v-model="selectedTextId" class="mr10 data-wrap">
<option value="" disabled>지문을 선택하세요</option>
<option v-for="text in texts" :key="text.textId" :value="text.textId">
{{ text.textTtl }}
</option>
</select>
</div>
</div>
<div class="flex align-center mb20">
<label for="" class="title2">단어장 타입</label>
<select v-model="selectedWdBookTypeId" class="mr10 data-wrap">
<option value="1">단어장 (일반)</option>
<option value="2">단어장 (스피킹)</option>
<option value="3">단어장 (숏폼)</option>
<option value="4">단어장 (카드 뒤집기)</option>
<option value="5">단어장 (카드 맞추기)</option>
</select>
</div>
<div class="flex align-center">
<label for="" class="title2">단어 목록</label>
<div class="flex-column" style="gap: 10px;">
<div class="flex align-center" style="gap: 10px;">
<input v-model="newWord.eng" type="text" class="data-wrap" placeholder="영어">
<input v-model="newWord.kor" type="text" class="data-wrap" placeholder="한글">
<input type="file" ref="fileInput" @change="handleFileUpload" multiple />
<button type="button" @click="addWord">
<img src="../../../resources/img/btn39_120t_normal.png" alt="">
</button>
</div>
<!-- 여기에 단어장에 소속될 단어들 태그 형태 리스트 -->
<div v-for="(word, index) in words" :key="index" class="flex align-center" style="gap: 10px;">
<input v-model="word.eng" type="text" class="data-wrap" placeholder="영어">
<input v-model="word.kor" type="text" class="data-wrap" placeholder="한글">
<div v-for="(data, index2) in word.file" :key="index2">
<input v-model="data.fileNm" type="text" disabled>
</div>
<button type="button" @click="removeWord(index)">
<img src="../../../resources/img/btn38_120t_normal.png" alt="">
</button>
</div>
</div>
<!--
<div class="flex align-center" style="gap: 10px;">
<input type="text" class="data-wrap" placeholder="영어">
<input type="text" class="data-wrap" placeholder="한글">
<button type="button" @click="addThesis">
<img src="../../../resources/img/btn39_120t_normal.png" alt="">
</button>
</div>
<div class="flex align-center " style="gap: 10px;" v-for="(thesis, index) in thesised" :key="thesis.id">
<input type="text" class="data-wrap" placeholder="영어">
<input type="text" class="data-wrap" placeholder="한글">
<button type="button" @click="removeThesis(thesis.id)">
<img src="../../../resources/img/btn38_120t_normal.png" alt="">
</button>
</div>
-->
</div>
</div>
<div class="flex justify-between mt50">
<button type="button" title="목록" class="new-btn" @click="goToPage('VocaList')">
목록
</button>
<div class="flex">
<button type="button" title="삭제" class="new-btn mr10" @click="deleteVoca">
삭제
</button>
<button type="button" title="취소" class="new-btn mr10" @click="cancelAction">
취소
</button>
<button type="button" title="수정" class="new-btn" @click="registerWordBook">
수정
</button>
</div>
</div>
</div>
</template>
<script>
import SvgIcon from '@jamescoyle/vue-icon';
import { mdiMagnify, mdiPlusCircleOutline, mdiWindowClose } from '@mdi/js';
import axios from "axios";
export default {
data() {
return {
thesised: [],
mdiPlusCircleOutline: mdiPlusCircleOutline,
mdiMagnify: mdiMagnify,
mdiWindowClose: mdiWindowClose,
selectedBookId: null,
selectedUnitId: null,
selectedTextId: null,
newWord: { eng: '', kor: '', fileMngId: null }, // 입력된 새 단어
words: [], // 단어 목록
books: [],
units: [],
texts: [],
wordbooks: [],
selectedWdBookId: null,
selectedfiles: [],
}
},
methods: {
async handleFileUpload(e) {
const files = e.target.files;
if (files.length > 0) {
const fileMngId = await this.uploadFiles(files);
this.newWord.fileMngId = fileMngId; // 파일 매니지 ID 저장
}
},
// 논문실적 버튼 추가
addThesis() {
// 고유 ID로 현재 시간의 타임스탬프를 사용
const uniqueId = Date.now();
this.thesised.push({
id: uniqueId, // 고유 ID 추가
});
},
removeThesis(thesisId) {
// ID를 기준으로 교육 정보 객체를 찾아서 삭제
const index = this.thesised.findIndex(thesis => thesis.id === thesisId);
if (index !== -1) {
this.thesised.splice(index, 1);
}
},
//
async addWord() { // 단어 추가
if (this.newWord.eng && this.newWord.kor) {
const newWordWithFile = {
...this.newWord,
file: [] // 파일 정보 저장을 위한 배열 추가
};
// 파일 매니지 ID가 있을 경우 파일 정보를 추가
if (newWordWithFile.fileMngId) {
const fileInfo = await this.findFile(newWordWithFile.fileMngId);
newWordWithFile.file = fileInfo; // 파일 정보 추가
}
this.words.push(newWordWithFile); // 단어 추가
// 초기화
this.newWord.eng = '';
this.newWord.kor = '';
this.newWord.fileMngId = null; // 파일 매니지 ID 초기화
} else {
console.log("단어 입력이 비어 있음");
}
},
removeWord(index) { // 단어 삭제
this.words.splice(index, 1);
},
goToPage(page) {
this.$router.push({ name: page });
},
cancelAction() {
this.$router.go(-1);
},
// 업로드한 파일 정보 가져오기
async findFile(fileMngId) {
const vm = this;
try {
const response = await axios({
url: "/file/find.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
file_mng_id: fileMngId,
},
});
console.log("fileInfo - response : ", response.data.list);
return response.data.list; // 파일 정보를 반환
} catch (error) {
console.log("result - error : ", error);
return []; // 오류 발생 시 빈 배열 반환
}
},
// 교재 정보 가져오기
fetchBooks() {
axios({
url: "/book/findAll.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
})
.then(response => {
console.log(response.data)
this.books = response.data;
this.selectedTextId = '';
})
.catch(error => {
console.error("fetchBooks - error: ", error);
alert("교재 목록을 불러오는 중 오류가 발생했습니다.");
});
},
// 단원 정보 가져오기
fetchUnits() {
if (!this.selectedBookId) return;
axios({
url: "/unit/unitList.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
"bookId": this.selectedBookId
},
})
.then(response => {
this.units = response.data;
this.selectedTextId = '';
this.fetchTexts();
})
.catch(error => {
console.error("fetchUnits - error: ", error);
alert("단원 목록을 불러오는 중 오류가 발생했습니다.");
});
},
// 지문 정보 가져오기
fetchTexts() {
axios({
url: "/text/textSearch.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
"unitId": this.selectedUnitId
},
})
.then(response => {
this.texts = response.data.list;
})
.catch(error => {
console.error("fetchTexts - error: ", error);
alert("지문 목록을 불러오는 중 오류가 발생했습니다.");
});
},
// 단어장의 단어 정보 조회 메서드
dataSelectList() {
const vm = this;
axios({
url: "/word/getWordsByBookId.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
wdBookId: vm.selectedWdBookId,
},
})
.then(async function (response) {
console.log("dataList - response: ", response.data);
vm.words = response.data.map(word => ({
eng: word.wdNm,
kor: word.wdMnng,
fileMngId: word.fileMngId, // 필요시 fileMngId도 포함
file: [] // 파일 정보를 저장할 배열 초기화
}));
// 각 단어에 대해 파일 정보를 조회
for (const word of vm.words) {
if (word.fileMngId) {
word.file = await vm.findFile(word.fileMngId); // 파일 정보를 단어에 추가
}
}
console.log(vm.words);
})
.catch(function (error) {
console.log("dataList - error: ", error);
alert("단어장 목록 조회에 오류가 발생했습니다.");
});
},
// 기존 단어 조회 메서드
fetchExistingWords(wdBookId) {
return axios.post('/word/getWordsByBookId.json', { wdBookId: wdBookId })
.then(response => {
console.log("기존 단어 ", response.data);
return response.data;
})
.catch(error => {
console.error('기존 단어 목록 가져오기 실패:', error);
return [];
});
},
// 파일 업로드 메서드
async uploadFiles(files) {
if (!files || files.length === 0) {
return null;
}
const formData = new FormData();
for (let i = 0; i < files.length; i++) {
formData.append("files", files[i]);
}
try {
const response = await axios.post("http://165.229.169.113:9080/file/upload.json", formData, {
headers: {
"Content-Type": "multipart/form-data",
},
});
return response.data.fileMngId; // 파일 매니지 ID 반환
} catch (error) {
console.error("파일 업로드 오류:", error);
throw new Error("파일 업로드에 실패했습니다.");
}
},
async registerWordBook() {
const vm = this;
try {
const response = await axios.post('/wordbook/update.json', {
wdBookTypeId: vm.selectedWdBookTypeId,
textId: vm.selectedTextId,
userId: vm.wordbooks.userId,
bookId: vm.selectedBookId,
unitId: vm.selectedUnitId,
wdBookId: vm.selectedWdBookId,
});
const wdBookId = vm.selectedWdBookId;
const existingWords = await vm.fetchExistingWords(wdBookId);
console.log('기존 단어 목록:', existingWords);
const existingWordMap = new Map(existingWords.map(word => [word.wdNm, word]));
const wordsToInsert = [];
const wordsToUpdate = [];
const wordsToDelete = [];
// 신규 단어 처리
vm.words.forEach(word => {
const existingWord = existingWordMap.get(word.eng);
if (existingWord) {
wordsToUpdate.push({
wdId: existingWord.wdId,
wdBookId: wdBookId,
wdNm: existingWord.wdNm,
wdMnng: word.kor,
fileMngId: word.fileMngId,
});
} else {
wordsToInsert.push({
wdBookId: wdBookId,
wdNm: word.eng,
wdMnng: word.kor,
fileMngId: word.fileMngId
});
}
});
// 삭제할 단어 처리
existingWords.forEach(word => {
if (!vm.words.find(newWord => newWord.eng === word.wdNm)) {
wordsToDelete.push(word);
}
});
console.log('신규 단어 목록:', wordsToInsert);
console.log('업데이트 단어 목록:', wordsToUpdate);
console.log('삭제할 단어 목록:', wordsToDelete);
// 단어 삽입
for (const word of wordsToInsert) {
const insertResponse = await axios.post('/word/insert.json', word);
console.log('삽입 응답:', insertResponse);
}
// 단어 업데이트
for (const word of wordsToUpdate) {
const updateResponse = await axios.post('/word/update.json', {
wdId: word.wdId,
wdBookId: wdBookId,
wdNm: word.wdNm,
wdMnng: word.wdMnng,
fileMngId: word.fileMngId,
});
console.log('업데이트 응답:', updateResponse);
}
// 단어 삭제
for (const word of wordsToDelete) {
const deleteResponse = await axios.post('/word/delete.json', {
wdBookId: wdBookId,
wdId: word.wdId
});
console.log('삭제 응답:', deleteResponse);
}
alert('단어장이 성공적으로 수정되었습니다.');
vm.goToPage('VocaList');
} catch (error) {
console.error('단어장 등록 중 오류 발생:', error);
alert('단어장 등록에 실패했습니다.');
}
},
// 단어장 삭제
deleteVoca() {
const result = confirm('해당 단어장을 삭제 하시겠습니까?')
if (result) {
} else {
alert("삭제를 취소했습니다")
return;
}
axios({
url: "/wordbook/delete.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
"wdBookId": this.selectedWdBookId
},
})
.then(response => {
alert("삭제가 완료됐습니다");
this.goToPage('VocaList');
})
.catch(error => {
console.error("fetchData - error: ", error);
alert("삭제 중 오류가 발생했습니다.");
});
},
// 단어장 정보 가져오기
wordBookFind() {
const vm = this;
axios({
url: "/wordbook/find.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
wdBookId: vm.selectedWdBookId,
},
})
.then(function (response) {
console.log("dataList - response: ", response.data);
vm.selectedWdBookTypeId = response.data.wdBookTypeId;
vm.selectedTextId = response.data.textId;
vm.wordbooks = response.data;
})
.catch(function (error) {
console.log("dataList - error: ", error);
alert("단어장 목록 조회에 오류가 발생했습니다.");
});
},
},
watch: {
},
computed: {
},
components: {
SvgIcon
},
mounted() {
this.selectedBookId = this.$route.query.selectedBookId || '';
this.selectedUnitId = this.$route.query.selectedUnitId || '';
this.selectedWdBookId = this.$route.query.wdBookId || '';
this.fetchBooks();
this.fetchUnits();
this.dataSelectList();
this.wordBookFind();
}
}
</script>