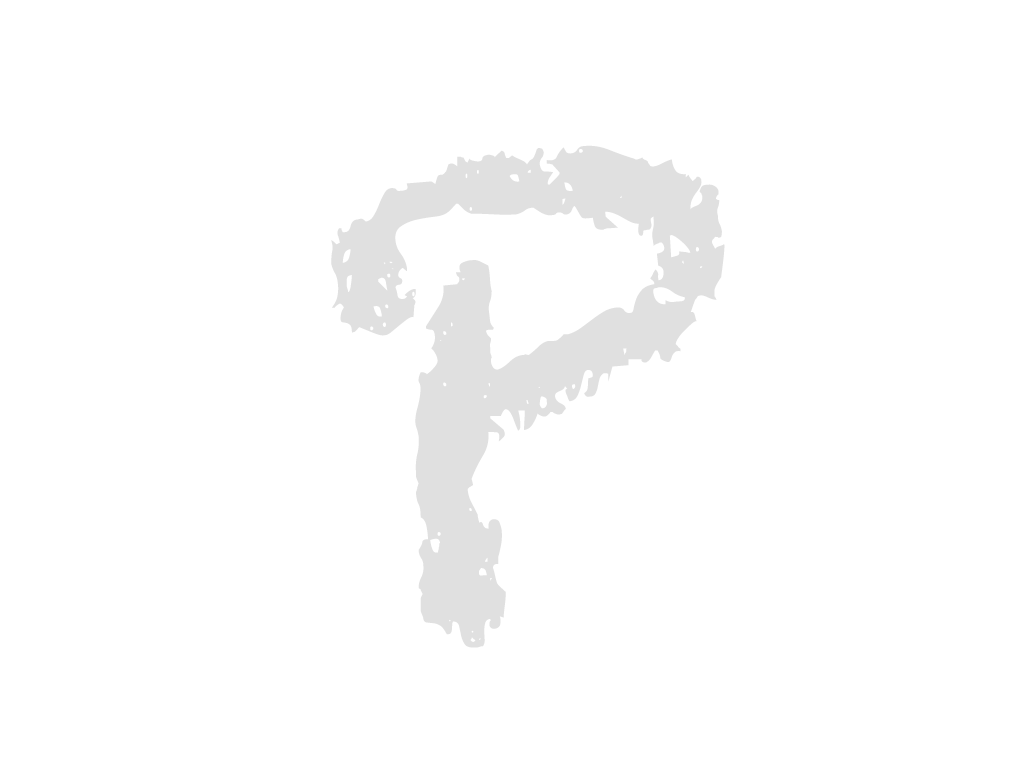
--- client/views/pages/teacher/C_TextBookDetail.vue
+++ client/views/pages/teacher/C_TextBookDetail.vue
... | ... | @@ -1,6 +1,6 @@ |
1 | 1 |
<template> |
2 | 2 |
<div class="title-box flex justify-between mb40"> |
3 |
- <p class="title">A교재</p> |
|
3 |
+ <p class="title">{{ bookDetails ? bookDetails.book_nm : 'Loading...' }}</p> |
|
4 | 4 |
<select name="" id=""> |
5 | 5 |
<option value="">A 반</option> |
6 | 6 |
</select> |
... | ... | @@ -66,7 +66,7 @@ |
66 | 66 |
import SvgIcon from '@jamescoyle/vue-icon'; |
67 | 67 |
import { mdiMagnify, mdilArrowRight } from '@mdi/js'; |
68 | 68 |
import ProgressBar from '../../component/ProgressBar.vue'; |
69 |
- |
|
69 |
+import axios from 'axios'; |
|
70 | 70 |
|
71 | 71 |
export default { |
72 | 72 |
data() { |
... | ... | @@ -74,7 +74,9 @@ |
74 | 74 |
mdiMagnify: mdiMagnify, |
75 | 75 |
mdilArrowRight: mdilArrowRight, |
76 | 76 |
timer: "00:00", |
77 |
- progress: 20 |
|
77 |
+ progress: 20, |
|
78 |
+ selectedBookId: this.$route.query.book_Id, |
|
79 |
+ bookDetails: null, |
|
78 | 80 |
} |
79 | 81 |
}, |
80 | 82 |
methods: { |
... | ... | @@ -100,6 +102,25 @@ |
100 | 102 |
this.goBack(); |
101 | 103 |
} |
102 | 104 |
}, |
105 |
+ fetchBookDetails() { |
|
106 |
+ axios({ |
|
107 |
+ url: "/book/find.json", |
|
108 |
+ method: "post", |
|
109 |
+ headers: { |
|
110 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
111 |
+ }, |
|
112 |
+ data: { |
|
113 |
+ book_id: this.$route.query.book_id |
|
114 |
+ } |
|
115 |
+ }) |
|
116 |
+ .then(response => { |
|
117 |
+ console.log('Book details:', response.data); |
|
118 |
+ this.bookDetails = response.data; |
|
119 |
+ }) |
|
120 |
+ .catch(error => { |
|
121 |
+ console.error('Error fetching book details:', error); |
|
122 |
+ }); |
|
123 |
+ } |
|
103 | 124 |
}, |
104 | 125 |
watch: { |
105 | 126 |
|
... | ... | @@ -112,7 +133,8 @@ |
112 | 133 |
ProgressBar |
113 | 134 |
}, |
114 | 135 |
mounted() { |
115 |
- console.log('Main2 mounted'); |
|
136 |
+ console.log('Mounted with book_id:', this.$route.query.book_id); |
|
137 |
+ this.fetchBookDetails(); |
|
116 | 138 |
} |
117 | 139 |
} |
118 | 140 |
</script> |
--- client/views/pages/teacher/C_Textbook.vue
+++ client/views/pages/teacher/C_Textbook.vue
... | ... | @@ -1,62 +1,67 @@ |
1 | 1 |
<template> |
2 | 2 |
<div class="title-box flex justify-between mb40"> |
3 | 3 |
<p class="title">교재</p> |
4 |
- <select name="" id=""> |
|
5 |
- <option value="">A반</option> |
|
4 |
+ <select v-model="selectedClassId"> |
|
5 |
+ <option v-for="classItem in classList" :key="classItem.sclsId" :value="classItem.sclsId"> |
|
6 |
+ {{ classItem.sclsNm }} |
|
7 |
+ </option> |
|
6 | 8 |
</select> |
7 |
- </div> |
|
8 |
- <div class="content-t"> |
|
9 |
- <div class=" flex " style="gap: 50px;"> |
|
10 |
- <div class="textbook"> |
|
11 |
- <div class="box " style="gap: 10px;" @click="goToPage('C_TextBookDetail')"> |
|
12 |
- </div> |
|
13 |
- <div class="text "> |
|
14 |
- <p class="title1" style="color: #fff;">A 교재</p> |
|
15 |
- <div class="btnGroup mt15 flex align-center justify-end" style="gap: 10px;"> |
|
16 |
- <button>수정</button><p>|</p> |
|
17 |
- <button @click="showConfirm('delete')">삭제</button> |
|
18 |
- </div> |
|
19 |
- </div> |
|
9 |
+ </div> |
|
10 |
+ <div class="content-t"> |
|
11 |
+ <div class="flex" style="gap: 2.5%;" :style="{flexWrap: 'wrap'}"> |
|
12 |
+ <div class="textbook" v-for="textbookItem in textbookList" :key="textbookItem.book_id" style="width: 22.5%; margin-bottom: 30px;"> |
|
13 |
+ <div class="box" style="gap: 10px;" @click="goToPage('C_TextBookDetail', textbookItem.book_id)"> |
|
20 | 14 |
</div> |
21 |
- |
|
22 |
- <div class="textbook-add"> |
|
23 |
- <button @click="buttonSearch"><img src="../../../resources/img/btn32_98t_normal.png" alt=""></button> |
|
24 |
- |
|
15 |
+ <div class="text"> |
|
16 |
+ <p class="title1" style="color: #fff;">{{ textbookItem.book_nm }}</p> |
|
17 |
+ <div class="btnGroup mt15 flex align-center justify-end" style="gap: 10px;"> |
|
18 |
+ <button @click="deleteBook(textbookItem.book_id)">삭제</button> |
|
19 |
+ </div> |
|
25 | 20 |
</div> |
26 | 21 |
</div> |
27 |
- </div> |
|
28 |
- <div v-show="searchOpen" class="popup-wrap"> |
|
29 |
- <div class="popup-box "> |
|
30 |
- <div class="flex justify-between mb30"> |
|
31 |
- <p class="popup-title">교재 이름</p> |
|
32 |
- <button type="button" class="popup-close-btn" @click="closeBtn"> |
|
33 |
- <svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon> |
|
34 | 22 |
|
35 |
- </button> |
|
36 |
- </div> |
|
37 |
- <div class="search-wrap mb30"> |
|
38 |
- <select v-model="selectedSearchOption" class="mr10 data-wrap"> |
|
39 |
- <option value="bbsTtl">제목</option> |
|
40 |
- <option value="bbsCnt">내용</option> |
|
41 |
- <option value="userNm">작성자</option> |
|
42 |
- <option value="bbsCls">카테고리</option> |
|
43 |
- </select> |
|
44 |
- </div> |
|
45 |
- <div class="flex justify-center "> |
|
46 |
- <button type="button" title="글쓰기" class="new-btn mr10"> |
|
47 |
- 취소 |
|
48 |
- </button> |
|
49 |
- <button type="button" title="글쓰기" class="new-btn"> |
|
50 |
- 등록 |
|
51 |
- </button> |
|
52 |
- </div> |
|
53 |
- </div> |
|
23 |
+ <div class="textbook-add" style="width: 22.5%; margin-bottom: 30px;"> |
|
24 |
+ <button @click="buttonSearch"> |
|
25 |
+ <img src="../../../resources/img/btn32_98t_normal.png" alt=""> |
|
26 |
+ </button> |
|
54 | 27 |
</div> |
28 |
+ </div> |
|
29 |
+ </div> |
|
30 |
+ <div v-show="searchOpen" class="popup-wrap"> |
|
31 |
+ <div class="popup-box"> |
|
32 |
+ <div class="flex justify-between mb30"> |
|
33 |
+ <p class="popup-title">교재 이름</p> |
|
34 |
+ <button type="button" class="popup-close-btn" @click="closeBtn"> |
|
35 |
+ <svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon> |
|
36 |
+ </button> |
|
37 |
+ </div> |
|
38 |
+ <div class="search-wrap mb30"> |
|
39 |
+ <input v-model="searchKeyword" type="text" placeholder="검색하세요." @keyup.enter="bookDataSearch"/> |
|
40 |
+ <button type="button" @click="bookDataSearch()" title="교재 검색"> |
|
41 |
+ <img src="../../../resources/img/look_t.png" alt="" /> |
|
42 |
+ </button> |
|
43 |
+ </div> |
|
44 |
+ <div class="table-wrap"> |
|
45 |
+ <div v-if="searchResults.length"> |
|
46 |
+ <tbody> |
|
47 |
+ <tr v-for="book in searchResults" :key="book.book_id"> |
|
48 |
+ <td>{{ book.book_nm }}<button type="button" title="등록" class="new-btn" @click="insertBook(book.book_id)" style="margin-left: 10px;">추가</button></td> |
|
49 |
+ </tr> |
|
50 |
+ </tbody> |
|
51 |
+ </div> |
|
52 |
+ <div v-else> |
|
53 |
+ <p>검색 결과가 없습니다.</p> |
|
54 |
+ </div> |
|
55 |
+</div> |
|
56 |
+ |
|
57 |
+ </div> |
|
58 |
+</div> |
|
55 | 59 |
</template> |
56 | 60 |
|
57 | 61 |
<script> |
58 | 62 |
import SvgIcon from '@jamescoyle/vue-icon'; |
59 | 63 |
import { mdiMagnify, mdiWindowClose } from '@mdi/js'; |
64 |
+import axios from 'axios'; |
|
60 | 65 |
|
61 | 66 |
export default { |
62 | 67 |
data () { |
... | ... | @@ -64,21 +69,38 @@ |
64 | 69 |
mdiWindowClose: mdiWindowClose, |
65 | 70 |
showModal: false, |
66 | 71 |
searchOpen: false, |
72 |
+ classList: [], |
|
73 |
+ textbookList: [], |
|
74 |
+ newBookName: '', |
|
75 |
+ editMode: false, |
|
76 |
+ editBookId: null, |
|
77 |
+ selectedClassId: '', |
|
78 |
+ searchOpen: false, |
|
79 |
+ searchKeyword: '', |
|
80 |
+ searchResults: [], |
|
67 | 81 |
} |
68 | 82 |
}, |
69 | 83 |
methods: { |
70 |
- goToPage(page) { |
|
71 |
- this.$router.push({ name: page }); |
|
72 |
- }, |
|
73 |
- closeModal() { |
|
74 |
- this.showModal = false; |
|
84 |
+ goToPage(page, book_id) { |
|
85 |
+ this.$router.push({ name: page, query: { book_id: book_id } }); |
|
75 | 86 |
}, |
76 | 87 |
buttonSearch() { |
77 | 88 |
this.searchOpen = true; |
78 | 89 |
}, |
79 | 90 |
closeBtn() { |
80 | 91 |
this.searchOpen = false; |
81 |
- |
|
92 |
+ this.editMode = false; |
|
93 |
+ this.editBookId = null; |
|
94 |
+ this.newBookName = ''; |
|
95 |
+ }, |
|
96 |
+ editBook(book) { |
|
97 |
+ this.newBookName = book.book_nm; |
|
98 |
+ this.editMode = true; |
|
99 |
+ this.editBookId = book.book_id; |
|
100 |
+ this.searchOpen = true; |
|
101 |
+ }, |
|
102 |
+ closeModal() { |
|
103 |
+ this.showModal = false; |
|
82 | 104 |
}, |
83 | 105 |
showConfirm(type) { |
84 | 106 |
let message = ''; |
... | ... | @@ -94,22 +116,131 @@ |
94 | 116 |
this.goBack(); |
95 | 117 |
} |
96 | 118 |
}, |
119 |
+ bookDataSearch() { |
|
120 |
+ const vm = this; |
|
121 |
+ const searchPayload = { |
|
122 |
+ keyword: vm.searchKeyword, |
|
123 |
+ }; |
|
124 |
+ axios.post("/book/search.json", searchPayload) |
|
125 |
+ .then(function (res) { |
|
126 |
+ console.log("bookDataSearch - response : ", res.data); |
|
127 |
+ vm.searchResults = res.data.result; // 검색 결과 저장 |
|
128 |
+ }) |
|
129 |
+ .catch(function (error) { |
|
130 |
+ console.log("bookSearch - error : ", error); |
|
131 |
+ alert("책이 존재하지 않습니다."); |
|
132 |
+ }); |
|
133 |
+ }, |
|
134 |
+ stdClassesSelectList() { |
|
135 |
+ const vm = this; |
|
136 |
+ axios({ |
|
137 |
+ url: "/classes/selectClass.json", |
|
138 |
+ method: "post", |
|
139 |
+ headers:{ |
|
140 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
141 |
+ }, |
|
142 |
+ data: { |
|
143 |
+ userId:"USID_000000000000001" |
|
144 |
+ } |
|
145 |
+ }) |
|
146 |
+ .then(function (response) { |
|
147 |
+ console.log("classList - response : ", response.data); |
|
148 |
+ vm.classList = response.data.data; |
|
149 |
+ }) |
|
150 |
+ .catch(function (error) { |
|
151 |
+ console.log("classList - error : ", error); |
|
152 |
+ alert("학생 반 조회에 오류가 발생했습니다."); |
|
153 |
+ }); |
|
154 |
+ }, |
|
155 |
+ bookList() { |
|
156 |
+ console.log('Fetching books for class ID:', this.selectedClassId); |
|
157 |
+ if (!this.selectedClassId) return; |
|
97 | 158 |
|
159 |
+ const vm = this; |
|
160 |
+ axios({ |
|
161 |
+ url: "/classBook/findAll.json", |
|
162 |
+ method: "post", |
|
163 |
+ headers: { |
|
164 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
165 |
+ }, |
|
166 |
+ data: { |
|
167 |
+ sclsId: this.selectedClassId |
|
168 |
+ } |
|
169 |
+ }) |
|
170 |
+ .then(response => { |
|
171 |
+ console.log("bookList - response : ", response.data); |
|
172 |
+ vm.textbookList = response.data.result; |
|
173 |
+ }) |
|
174 |
+ .catch(error => { |
|
175 |
+ console.error('Error fetching books:', error); |
|
176 |
+ }); |
|
177 |
+ }, |
|
178 |
+ insertBook(bookId) { |
|
179 |
+ const bookToAdd = [{ |
|
180 |
+ bookId: bookId, |
|
181 |
+ sclsId: this.selectedClassId |
|
182 |
+ }]; |
|
183 |
+ |
|
184 |
+ axios.post('/classBook/register.json', bookToAdd) |
|
185 |
+ .then(response => { |
|
186 |
+ console.log('Book added successfully:', response.data); |
|
187 |
+ this.bookList(); |
|
188 |
+ }) |
|
189 |
+ .catch(error => { |
|
190 |
+ console.error('Error adding book:', error); |
|
191 |
+ }); |
|
192 |
+ }, |
|
193 |
+ deleteBook(bookId) { |
|
194 |
+ if (confirm('삭제하시겠습니까?')) { |
|
195 |
+ axios({ |
|
196 |
+ url: "/classBook/delete.json", |
|
197 |
+ method: "post", |
|
198 |
+ headers: { |
|
199 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
200 |
+ }, |
|
201 |
+ data: { |
|
202 |
+ bookId: bookId, |
|
203 |
+ sclsId: this.selectedClassId |
|
204 |
+ }, |
|
205 |
+ }) |
|
206 |
+ .then(response => { |
|
207 |
+ this.bookList(); |
|
208 |
+ }) |
|
209 |
+ .catch(error => { |
|
210 |
+ console.error('Error deleting book:', error); |
|
211 |
+ }); |
|
212 |
+ } |
|
213 |
+ }, |
|
98 | 214 |
}, |
99 | 215 |
watch: { |
100 |
- |
|
101 |
- }, |
|
102 |
- computed: { |
|
103 |
- |
|
216 |
+ selectedClassId(newVal) { |
|
217 |
+ if (newVal) { |
|
218 |
+ this.bookList(); |
|
219 |
+ } |
|
220 |
+ } |
|
104 | 221 |
}, |
105 | 222 |
components: { |
106 | 223 |
SvgIcon |
107 | 224 |
}, |
108 | 225 |
mounted() { |
109 | 226 |
console.log('Main2 mounted'); |
227 |
+ this.stdClassesSelectList(); |
|
110 | 228 |
} |
111 | 229 |
} |
230 |
+ |
|
112 | 231 |
</script> |
232 |
+ |
|
113 | 233 |
<style scoped> |
114 |
-.textbook{width: 300px;} |
|
234 |
+.content-t { |
|
235 |
+ flex-wrap: wrap; |
|
236 |
+ height: 90%; |
|
237 |
+ overflow-y: scroll; |
|
238 |
+} |
|
239 |
+.flex { |
|
240 |
+ display: flex; |
|
241 |
+ flex-wrap: wrap; |
|
242 |
+} |
|
243 |
+.textbook, .textbook-add { |
|
244 |
+ margin-bottom: 30px; |
|
245 |
+} |
|
115 | 246 |
</style>(No newline at end of file) |
--- client/views/pages/teacher/TextBookDetail.vue
+++ client/views/pages/teacher/TextBookDetail.vue
... | ... | @@ -1,18 +1,13 @@ |
1 | 1 |
<template> |
2 | 2 |
<div class="title-box flex justify-between mb40"> |
3 |
- <p class="title">A교재</p> |
|
4 |
- <!-- <select name="" id=""> |
|
5 |
- <option value="">1단원</option> |
|
6 |
- </select> --> |
|
7 |
- </div> |
|
3 |
+ <p class="title">{{ bookDetails ? bookDetails.book_nm : 'Loading...' }}</p> |
|
4 |
+ </div> |
|
8 | 5 |
<label for="" class="title1">단원</label> |
9 | 6 |
<div class="unit-pagination flex mt10" style="gap: 10px;"> |
10 | 7 |
<button class="selected-btn">1</button> |
11 | 8 |
<button>2</button> |
12 | 9 |
<button>3</button> |
13 |
- <button ><svg-icon type="mdi" :path="mdiPlus" style=" padding-top: 6px; |
|
14 |
- width: 30px; |
|
15 |
- height: 30px;"></svg-icon></button> |
|
10 |
+ <button ><svg-icon type="mdi" :path="mdiPlus" style=" padding-top: 6px; width: 30px; height: 30px;"></svg-icon></button> |
|
16 | 11 |
</div> |
17 | 12 |
<div class="board-wrap mt30"> |
18 | 13 |
<div class="mb20 "> |
... | ... | @@ -177,57 +172,52 @@ |
177 | 172 |
|
178 | 173 |
<script> |
179 | 174 |
import SvgIcon from '@jamescoyle/vue-icon'; |
180 |
-import { mdiMagnify,mdiPlus } from '@mdi/js'; |
|
175 |
+import { mdiMagnify, mdiPlus } from '@mdi/js'; |
|
181 | 176 |
import { mdilArrowRight } from '@mdi/light-js'; |
182 | 177 |
import ProgressBar from '../../component/ProgressBar.vue'; |
183 |
- |
|
178 |
+import axios from 'axios'; |
|
184 | 179 |
|
185 | 180 |
export default { |
186 | 181 |
data() { |
187 | 182 |
return { |
188 |
- mdiPlus :mdiPlus , |
|
183 |
+ mdiPlus: mdiPlus, |
|
189 | 184 |
mdiMagnify: mdiMagnify, |
190 | 185 |
mdilArrowRight: mdilArrowRight, |
191 |
- timer: "00:00", |
|
192 |
- progress: 20 |
|
193 |
- } |
|
186 |
+ selectedBookId: this.$route.query.book_Id, |
|
187 |
+ bookDetails: null, |
|
188 |
+ }; |
|
194 | 189 |
}, |
195 | 190 |
methods: { |
196 | 191 |
goToPage(page) { |
197 | 192 |
this.$router.push({ name: page }); |
198 | 193 |
}, |
199 |
- increaseProgress() { |
|
200 |
- if (this.progress < 100) { |
|
201 |
- this.progress += 10; |
|
202 |
- } |
|
203 |
- }, |
|
204 |
- showConfirm(type) { |
|
205 |
- let message = ''; |
|
206 |
- if (type === 'delete') { |
|
207 |
- message = '삭제하시겠습니까?'; |
|
208 |
- } else if (type === 'reset') { |
|
209 |
- message = '초기화하시겠습니까?'; |
|
210 |
- } else if (type === 'save') { |
|
211 |
- message = '등록하시겠습니까?'; |
|
212 |
- } |
|
213 |
- |
|
214 |
- if (confirm(message)) { |
|
215 |
- this.goBack(); |
|
216 |
- } |
|
217 |
- }, |
|
194 |
+ fetchBookDetails() { |
|
195 |
+ axios({ |
|
196 |
+ url: "/book/find.json", |
|
197 |
+ method: "post", |
|
198 |
+ headers: { |
|
199 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
200 |
+ }, |
|
201 |
+ data: { |
|
202 |
+ book_id: this.$route.query.book_id |
|
203 |
+ } |
|
204 |
+ }) |
|
205 |
+ .then(response => { |
|
206 |
+ console.log('Book details:', response.data); |
|
207 |
+ this.bookDetails = response.data; |
|
208 |
+ }) |
|
209 |
+ .catch(error => { |
|
210 |
+ console.error('Error fetching book details:', error); |
|
211 |
+ }); |
|
212 |
+ } |
|
218 | 213 |
}, |
219 |
- watch: { |
|
220 |
- |
|
221 |
- }, |
|
222 |
- computed: { |
|
223 |
- |
|
214 |
+ mounted() { |
|
215 |
+ console.log('Mounted with book_id:', this.$route.query.book_id); |
|
216 |
+ this.fetchBookDetails(); |
|
224 | 217 |
}, |
225 | 218 |
components: { |
226 | 219 |
SvgIcon, |
227 | 220 |
ProgressBar |
228 |
- }, |
|
229 |
- mounted() { |
|
230 |
- console.log('Main2 mounted'); |
|
231 | 221 |
} |
232 | 222 |
} |
233 | 223 |
</script> |
--- client/views/pages/teacher/textbook.vue
+++ client/views/pages/teacher/textbook.vue
... | ... | @@ -1,61 +1,56 @@ |
1 | 1 |
<template> |
2 | 2 |
<div class="title-box flex justify-between mb40"> |
3 |
- <p class="title">교재</p> |
|
4 |
- <select name="" id=""> |
|
5 |
- <option value="">A반</option> |
|
6 |
- </select> |
|
3 |
+ <p class="title">교재</p> |
|
7 | 4 |
</div> |
8 | 5 |
<div class="content-t"> |
9 |
- <div class="flex" style="gap: 2.5%;" :style="{flexWrap: 'wrap'}"> |
|
10 |
- <div class="textbook" v-for="textbookItem in textbookList" :key="textbookItem.book_id" style="width: 22.5%; margin-bottom: 30px;"> |
|
11 |
- <div class="box" style="gap: 10px;" @click="goToPage('TextBookDetail', textbookItem.book_id)"> |
|
12 |
- </div> |
|
13 |
- <div class="text"> |
|
14 |
- <p class="title1" style="color: #fff;">{{ textbookItem.book_nm }}</p> |
|
15 |
- <div class="btnGroup mt15 flex align-center justify-end" style="gap: 10px;"> |
|
16 |
- <button @click="editBook(textbookItem)">수정</button> |
|
17 |
- <p>|</p> |
|
18 |
- <button @click="deleteBook(textbookItem.book_id)">삭제</button> |
|
19 |
- </div> |
|
20 |
- </div> |
|
6 |
+ <div class="flex" style="gap: 2.5%;" :style="{flexWrap: 'wrap'}"> |
|
7 |
+ <div class="textbook" v-for="textbookItem in textbookList" :key="textbookItem.book_id" style="width: 22.5%; margin-bottom: 30px;"> |
|
8 |
+ <div class="box" style="gap: 10px;" @click="goToPage('TextBookDetail', textbookItem.book_id)"> |
|
9 |
+ </div> |
|
10 |
+ <div class="text"> |
|
11 |
+ <p class="title1" style="color: #fff;">{{ textbookItem.book_nm }}</p> |
|
12 |
+ <div class="btnGroup mt15 flex align-center justify-end" style="gap: 10px;"> |
|
13 |
+ <button @click="editBook(textbookItem)">수정</button> |
|
14 |
+ <p>|</p> |
|
15 |
+ <button @click="deleteBook(textbookItem.book_id)">삭제</button> |
|
21 | 16 |
</div> |
22 |
- |
|
23 |
- <div class="textbook-add" style="width: 22.5%; margin-bottom: 30px;"> |
|
24 |
- <button @click="buttonSearch"> |
|
25 |
- <img src="../../../resources/img/btn32_98t_normal.png" alt=""> |
|
26 |
- </button> |
|
27 |
- </div> |
|
28 |
- |
|
29 |
- <div v-show="searchOpen" class="popup-wrap"> |
|
30 |
- <div class="popup-box"> |
|
31 |
- <div class="flex justify-between mb30"> |
|
32 |
- <p class="popup-title">교재 이름</p> |
|
33 |
- <button type="button" class="popup-close-btn" @click="closeBtn"> |
|
34 |
- <svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon> |
|
35 |
- </button> |
|
36 |
- </div> |
|
37 |
- <div class="search-wrap mb30"> |
|
38 |
- <input type="text" class="data-wrap" v-model="newBookName" placeholder="교재 이름을 입력하세요"> |
|
39 |
- <button type="button" title="교재 검색" @click="insertBook"> |
|
40 |
- <img src="../../../resources/img/look_t.png" alt=""> |
|
41 |
- </button> |
|
42 |
- </div> |
|
43 |
- <div class="flex justify-center"> |
|
44 |
- <button type="button" title="취소" class="new-btn mr10" @click="closeBtn"> |
|
45 |
- 취소 |
|
46 |
- </button> |
|
47 |
- <button type="button" title="생성" class="new-btn" @click="editMode ? updateBook() : insertBook()"> |
|
48 |
- {{ editMode ? '수정' : '생성' }} |
|
49 |
- </button> |
|
50 |
- </div> |
|
51 |
- </div> |
|
52 |
- </div> |
|
17 |
+ </div> |
|
53 | 18 |
</div> |
19 |
+ |
|
20 |
+ <div class="textbook-add" style="width: 22.5%; margin-bottom: 30px;"> |
|
21 |
+ <button @click="buttonSearch"> |
|
22 |
+ <img src="../../../resources/img/btn32_98t_normal.png" alt=""> |
|
23 |
+ </button> |
|
24 |
+ </div> |
|
25 |
+ |
|
26 |
+ <div v-show="searchOpen" class="popup-wrap"> |
|
27 |
+ <div class="popup-box"> |
|
28 |
+ <div class="flex justify-between mb30"> |
|
29 |
+ <p class="popup-title">교재 이름</p> |
|
30 |
+ <button type="button" class="popup-close-btn" @click="closeBtn"> |
|
31 |
+ <svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon> |
|
32 |
+ </button> |
|
33 |
+ </div> |
|
34 |
+ <div class="search-wrap mb30"> |
|
35 |
+ <input type="text" class="data-wrap" v-model="newBookName" placeholder="교재 이름을 입력하세요"> |
|
36 |
+ <button type="button" title="교재 검색" @click="insertBook"> |
|
37 |
+ <img src="../../../resources/img/look_t.png" alt=""> |
|
38 |
+ </button> |
|
39 |
+ </div> |
|
40 |
+ <div class="flex justify-center"> |
|
41 |
+ <button type="button" title="취소" class="new-btn mr10" @click="closeBtn"> |
|
42 |
+ 취소 |
|
43 |
+ </button> |
|
44 |
+ <button type="button" title="생성" class="new-btn" @click="editMode ? updateBook() : insertBook()"> |
|
45 |
+ {{ editMode ? '수정' : '생성' }} |
|
46 |
+ </button> |
|
47 |
+ </div> |
|
48 |
+ </div> |
|
49 |
+ </div> |
|
50 |
+ </div> |
|
54 | 51 |
</div> |
55 | 52 |
</template> |
56 |
- |
|
57 |
- |
|
58 |
- |
|
53 |
+ |
|
59 | 54 |
<script> |
60 | 55 |
import SvgIcon from '@jamescoyle/vue-icon'; |
61 | 56 |
import { mdiWindowClose } from '@mdi/js'; |
... | ... | @@ -75,7 +70,7 @@ |
75 | 70 |
}, |
76 | 71 |
methods: { |
77 | 72 |
goToPage(page, book_id) { |
78 |
- this.$router.push({ name: page, query: { book_id }}); |
|
73 |
+ this.$router.push({ name: page, query: { book_id: book_id } }); |
|
79 | 74 |
}, |
80 | 75 |
buttonSearch() { |
81 | 76 |
this.searchOpen = true; |
... | ... | @@ -160,10 +155,7 @@ |
160 | 155 |
console.error('Error deleting book:', error); |
161 | 156 |
}); |
162 | 157 |
} |
163 |
- } |
|
164 |
- }, |
|
165 |
- computed: { |
|
166 |
- |
|
158 |
+ }, |
|
167 | 159 |
}, |
168 | 160 |
components: { |
169 | 161 |
SvgIcon |
... | ... | @@ -188,4 +180,4 @@ |
188 | 180 |
.textbook, .textbook-add { |
189 | 181 |
margin-bottom: 30px; |
190 | 182 |
} |
191 |
-</style>(No newline at end of file) |
|
183 |
+</style> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?