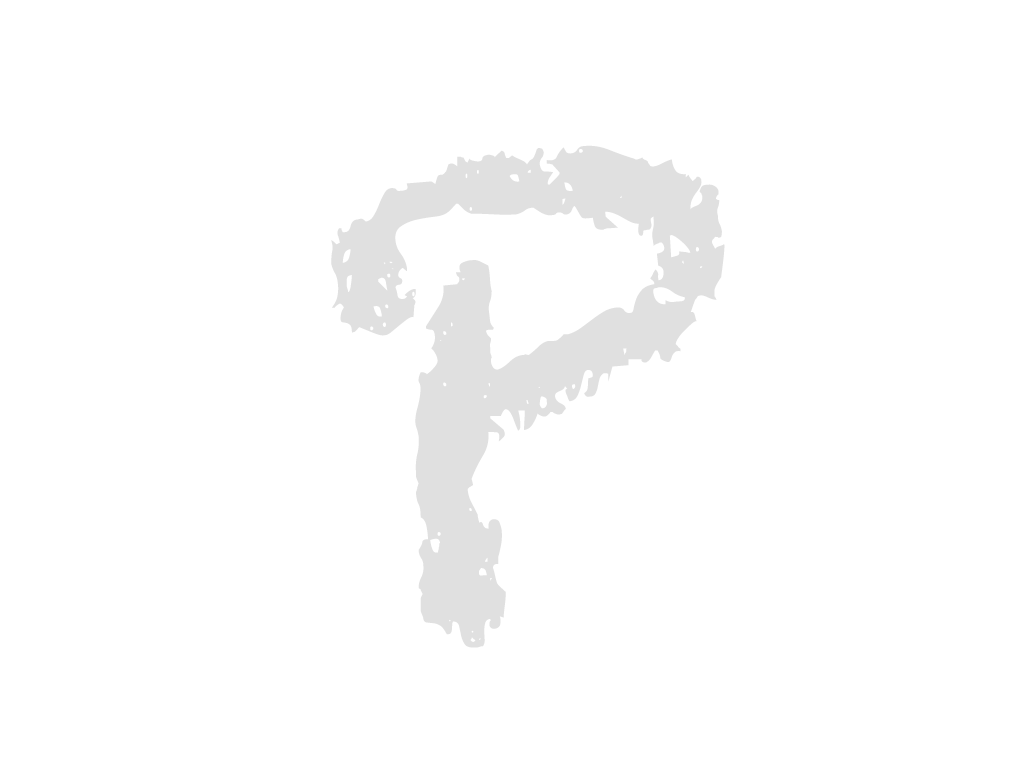
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="title-box flex justify-between mb40">
<p class="title">교재</p>
</div>
<div class="content-t">
<div class="flex" style="gap: 2.5%;" :style="{flexWrap: 'wrap'}">
<div class="textbook" v-for="textbookItem in textbookList" :key="textbookItem.book_id" style="width: 22.5%; margin-bottom: 30px;">
<div class="box" style="gap: 10px;" @click="goToPage('TextBookDetail', textbookItem.book_id)">
</div>
<div class="text">
<p class="title1" style="color: #fff;">{{ textbookItem.book_nm }}</p>
<div class="btnGroup mt15 flex align-center justify-end" style="gap: 10px;">
<button @click="editBook(textbookItem)">수정</button>
<p>|</p>
<button @click="deleteBook(textbookItem.book_id)">삭제</button>
</div>
</div>
</div>
<div class="textbook-add" style="width: 22.5%; margin-bottom: 30px;">
<button @click="buttonSearch">
<img src="../../../resources/img/btn32_98t_normal.png" alt="">
</button>
</div>
<div v-show="searchOpen" class="popup-wrap">
<div class="popup-box">
<div class="flex justify-between mb30">
<p class="popup-title">교재 이름</p>
<button type="button" class="popup-close-btn" @click="closeBtn">
<svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon>
</button>
</div>
<div class="search-wrap mb30">
<input type="text" class="data-wrap" v-model="newBookName" placeholder="교재 이름을 입력하세요">
<button type="button" title="교재 검색" @click="insertBook">
<img src="../../../resources/img/look_t.png" alt="">
</button>
</div>
<div class="flex justify-center">
<button type="button" title="취소" class="new-btn mr10" @click="closeBtn">
취소
</button>
<button type="button" title="생성" class="new-btn" @click="editMode ? updateBook() : insertBook()">
{{ editMode ? '수정' : '생성' }}
</button>
</div>
</div>
</div>
</div>
</div>
</template>
<script>
import SvgIcon from '@jamescoyle/vue-icon';
import { mdiWindowClose } from '@mdi/js';
import axios from 'axios';
export default {
data() {
return {
mdiWindowClose: mdiWindowClose,
showModal: false,
searchOpen: false,
textbookList: [],
newBookName: '',
editMode: false,
editBookId: null,
}
},
methods: {
goToPage(page, book_id) {
this.$router.push({ name: page, query: { book_id: book_id } });
},
buttonSearch() {
this.searchOpen = true;
},
closeBtn() {
this.searchOpen = false;
this.editMode = false;
this.editBookId = null;
this.newBookName = '';
},
editBook(book) {
this.newBookName = book.book_nm;
this.editMode = true;
this.editBookId = book.book_id;
this.searchOpen = true;
},
bookList() {
axios({
url: "/book/findAll.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
}
})
.then(response => {
this.textbookList = response.data;
})
.catch(error => {
console.error('Error fetching books:', error);
});
},
insertBook() {
const newBook = {
book_nm: this.newBookName
};
axios.post('/book/insert.json', newBook)
.then(response => {
this.bookList();
this.closeBtn();
})
.catch(error => {
console.error('Error creating book:', error);
});
},
updateBook() {
const updatedBook = {
book_id: this.editBookId,
book_nm: this.newBookName
};
axios({
url: "/book/update.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: updatedBook,
})
.then(response => {
this.bookList();
this.closeBtn();
})
.catch(error => {
console.error('Error updating book:', error);
});
},
deleteBook(book_id) {
if (confirm('삭제하시겠습니까?')) {
axios({
url: "/book/delete.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
book_id: book_id
},
})
.then(response => {
this.bookList();
})
.catch(error => {
console.error('Error deleting book:', error);
});
}
},
},
components: {
SvgIcon
},
mounted() {
console.log('Main2 mounted');
this.bookList();
}
}
</script>
<style>
.content-t {
flex-wrap: wrap;
height: 90%;
overflow-y: scroll;
}
.flex {
display: flex;
flex-wrap: wrap;
}
.textbook, .textbook-add {
margin-bottom: 30px;
}
</style>