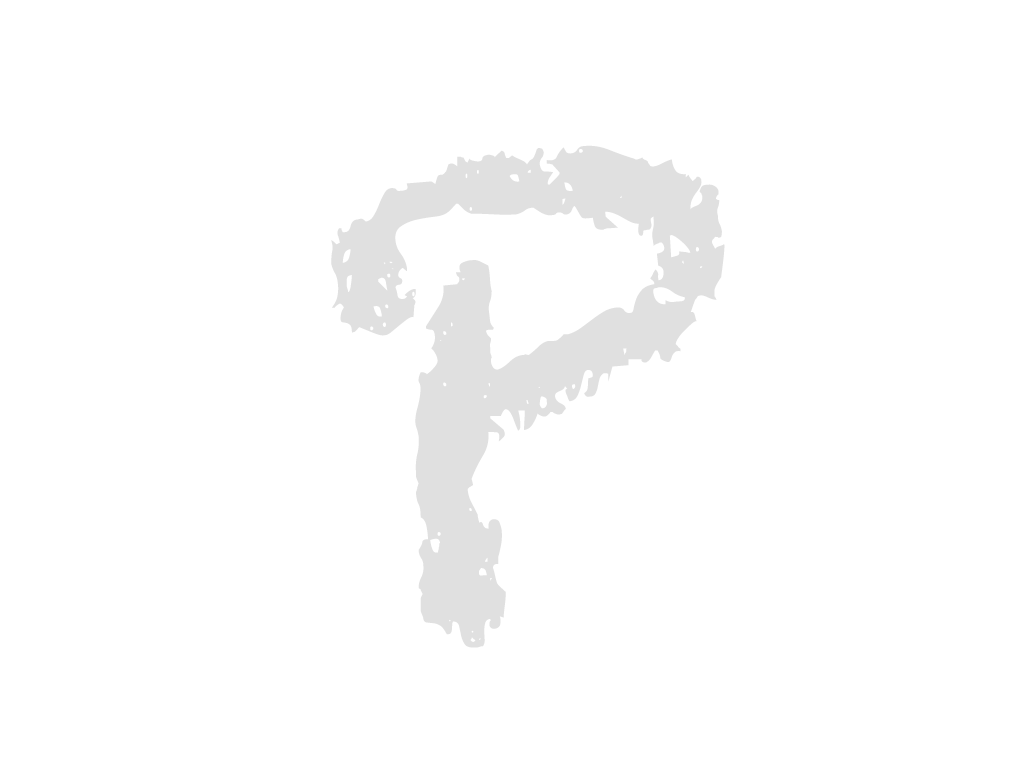
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="title-box flex justify-between mb40">
<p class="title">교재</p>
<select v-model="selectedClassId">
<option v-for="classItem in classList" :key="classItem.sclsId" :value="classItem.sclsId">
{{ classItem.sclsNm }}
</option>
</select>
</div>
<div class="content-t">
<div class="flex" style="gap: 2.5%;" :style="{flexWrap: 'wrap'}">
<div class="textbook" v-for="textbookItem in textbookList" :key="textbookItem.book_id" style="width: 22.5%; margin-bottom: 30px;">
<div class="box" style="gap: 10px;" @click="goToPage('C_TextBookDetail', textbookItem.book_id)">
</div>
<div class="text">
<p class="title1" style="color: #fff;">{{ textbookItem.book_nm }}</p>
<div class="btnGroup mt15 flex align-center justify-end" style="gap: 10px;">
<button @click="deleteBook(textbookItem.book_id)">삭제</button>
</div>
</div>
</div>
<div class="textbook-add" style="width: 22.5%; margin-bottom: 30px;">
<button @click="buttonSearch">
<img src="../../../resources/img/btn32_98t_normal.png" alt="">
</button>
</div>
</div>
</div>
<div v-show="searchOpen" class="popup-wrap">
<div class="popup-box">
<div class="flex justify-between mb30">
<p class="popup-title">교재 이름</p>
<button type="button" class="popup-close-btn" @click="closeBtn">
<svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon>
</button>
</div>
<div class="search-wrap mb30">
<input v-model="searchKeyword" type="text" placeholder="검색하세요." @keyup.enter="bookDataSearch"/>
<button type="button" @click="bookDataSearch()" title="교재 검색">
<img src="../../../resources/img/look_t.png" alt="" />
</button>
</div>
<div class="table-wrap">
<div v-if="searchResults.length">
<tbody>
<tr v-for="book in searchResults" :key="book.book_id">
<td>{{ book.book_nm }}<button type="button" title="등록" class="new-btn" @click="insertBook(book.book_id)" style="margin-left: 10px;">추가</button></td>
</tr>
</tbody>
</div>
<div v-else>
<p>검색 결과가 없습니다.</p>
</div>
</div>
</div>
</div>
</template>
<script>
import SvgIcon from '@jamescoyle/vue-icon';
import { mdiMagnify, mdiWindowClose } from '@mdi/js';
import axios from 'axios';
export default {
data () {
return {
mdiWindowClose: mdiWindowClose,
showModal: false,
searchOpen: false,
classList: [],
textbookList: [],
newBookName: '',
editMode: false,
editBookId: null,
selectedClassId: '',
searchOpen: false,
searchKeyword: '',
searchResults: [],
}
},
methods: {
goToPage(page, book_id) {
this.$router.push({ name: page, query: { book_id: book_id } });
},
buttonSearch() {
this.searchOpen = true;
},
closeBtn() {
this.searchOpen = false;
this.editMode = false;
this.editBookId = null;
this.newBookName = '';
},
editBook(book) {
this.newBookName = book.book_nm;
this.editMode = true;
this.editBookId = book.book_id;
this.searchOpen = true;
},
closeModal() {
this.showModal = false;
},
showConfirm(type) {
let message = '';
if (type === 'delete') {
message = '삭제하시겠습니까?';
} else if (type === 'reset') {
message = '초기화하시겠습니까?';
} else if (type === 'save') {
message = '등록하시겠습니까?';
}
if (confirm(message)) {
this.goBack();
}
},
bookDataSearch() {
const vm = this;
const searchPayload = {
keyword: vm.searchKeyword,
};
axios.post("/book/search.json", searchPayload)
.then(function (res) {
console.log("bookDataSearch - response : ", res.data);
vm.searchResults = res.data.result; // 검색 결과 저장
})
.catch(function (error) {
console.log("bookSearch - error : ", error);
alert("책이 존재하지 않습니다.");
});
},
stdClassesSelectList() {
const vm = this;
axios({
url: "/classes/selectClass.json",
method: "post",
headers:{
"Content-Type": "application/json; charset=UTF-8",
},
data: {
userId:"USID_000000000000001"
}
})
.then(function (response) {
console.log("classList - response : ", response.data);
vm.classList = response.data.data;
})
.catch(function (error) {
console.log("classList - error : ", error);
alert("학생 반 조회에 오류가 발생했습니다.");
});
},
bookList() {
console.log('Fetching books for class ID:', this.selectedClassId);
if (!this.selectedClassId) return;
const vm = this;
axios({
url: "/classBook/findAll.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
sclsId: this.selectedClassId
}
})
.then(response => {
console.log("bookList - response : ", response.data);
vm.textbookList = response.data.result;
})
.catch(error => {
console.error('Error fetching books:', error);
});
},
insertBook(bookId) {
const bookToAdd = [{
bookId: bookId,
sclsId: this.selectedClassId
}];
axios.post('/classBook/register.json', bookToAdd)
.then(response => {
console.log('Book added successfully:', response.data);
this.bookList();
})
.catch(error => {
console.error('Error adding book:', error);
});
},
deleteBook(bookId) {
if (confirm('삭제하시겠습니까?')) {
axios({
url: "/classBook/delete.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
bookId: bookId,
sclsId: this.selectedClassId
},
})
.then(response => {
this.bookList();
})
.catch(error => {
console.error('Error deleting book:', error);
});
}
},
},
watch: {
selectedClassId(newVal) {
if (newVal) {
this.bookList();
}
}
},
components: {
SvgIcon
},
mounted() {
console.log('Main2 mounted');
this.stdClassesSelectList();
}
}
</script>
<style scoped>
.content-t {
flex-wrap: wrap;
height: 90%;
overflow-y: scroll;
}
.flex {
display: flex;
flex-wrap: wrap;
}
.textbook, .textbook-add {
margin-bottom: 30px;
}
</style>