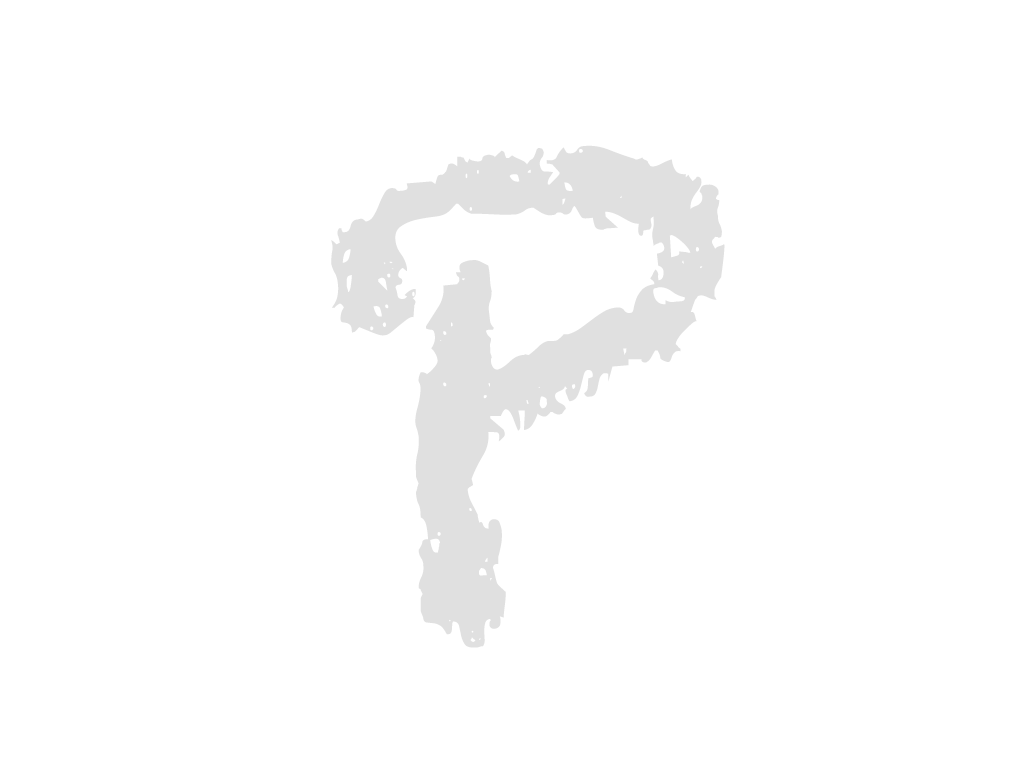
--- client/views/pages/teacher/TextBookDetail.vue
+++ client/views/pages/teacher/TextBookDetail.vue
... | ... | @@ -136,7 +136,7 @@ |
136 | 136 |
<button type="button" title="" class="new-btn" @click="goToPage('RoadMap')"> |
137 | 137 |
로드맵 |
138 | 138 |
</button> |
139 |
- <button type="button" title="" class="new-btn" @click="showConfirm('delete')"> |
|
139 |
+ <button type="button" title="" class="new-btn" @click="deleteUnit()"> |
|
140 | 140 |
삭제 |
141 | 141 |
</button> |
142 | 142 |
</div> |
... | ... | @@ -431,7 +431,35 @@ |
431 | 431 |
}); |
432 | 432 |
}, |
433 | 433 |
|
434 |
+ // 단원 삭제 |
|
435 |
+ deleteUnit(){ |
|
436 |
+ const result = confirm('해당 단원을 삭제 하시겠습니까?') |
|
437 |
+ if (result) { |
|
438 |
+ } else { |
|
439 |
+ alert("삭제를 취소했습니다") |
|
440 |
+ return; |
|
441 |
+ } |
|
434 | 442 |
|
443 |
+ axios({ |
|
444 |
+ url: "/unit/deleteUnit.json", |
|
445 |
+ method: "post", |
|
446 |
+ headers: { |
|
447 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
448 |
+ }, |
|
449 |
+ data: { |
|
450 |
+ "unitId": this.selectedUnitId |
|
451 |
+ }, |
|
452 |
+ }) |
|
453 |
+ .then(response => { |
|
454 |
+ alert("삭제가 완료됐습니다"); |
|
455 |
+ this.fetchBookDetails(); |
|
456 |
+ this.findUnit(); |
|
457 |
+ }) |
|
458 |
+ .catch(error => { |
|
459 |
+ console.error("fetchData - error: ", error); |
|
460 |
+ alert("삭제 중 오류가 발생했습니다."); |
|
461 |
+ }); |
|
462 |
+ } |
|
435 | 463 |
}, |
436 | 464 |
mounted() { |
437 | 465 |
console.log('Mounted with book_id:', this.$route.query.book_id); |
--- client/views/pages/teacher/VocaDetail.vue
+++ client/views/pages/teacher/VocaDetail.vue
... | ... | @@ -55,6 +55,10 @@ |
55 | 55 |
<div v-for="(word, index) in words" :key="index" class="flex align-center" style="gap: 10px;"> |
56 | 56 |
<input v-model="word.eng" type="text" class="data-wrap" placeholder="영어"> |
57 | 57 |
<input v-model="word.kor" type="text" class="data-wrap" placeholder="한글"> |
58 |
+ <div v-for="(data, index2) in word.file" :key="index2"> |
|
59 |
+ <input v-model="data.fileNm" type="text" disabled> |
|
60 |
+ |
|
61 |
+ </div> |
|
58 | 62 |
<button type="button" @click="removeWord(index)"> |
59 | 63 |
<img src="../../../resources/img/btn38_120t_normal.png" alt=""> |
60 | 64 |
</button> |
... | ... | @@ -87,6 +91,9 @@ |
87 | 91 |
목록 |
88 | 92 |
</button> |
89 | 93 |
<div class="flex"> |
94 |
+ <button type="button" title="삭제" class="new-btn mr10" @click="deleteVoca"> |
|
95 |
+ 삭제 |
|
96 |
+ </button> |
|
90 | 97 |
<button type="button" title="취소" class="new-btn mr10" @click="cancelAction"> |
91 | 98 |
취소 |
92 | 99 |
</button> |
... | ... | @@ -125,6 +132,8 @@ |
125 | 132 |
|
126 | 133 |
wordbooks: [], |
127 | 134 |
selectedWdBookId: null, |
135 |
+ |
|
136 |
+ selectedfiles: [], |
|
128 | 137 |
} |
129 | 138 |
}, |
130 | 139 |
methods: { |
... | ... | @@ -154,10 +163,21 @@ |
154 | 163 |
}, |
155 | 164 |
// |
156 | 165 |
|
157 |
- addWord() { // 단어 추가 |
|
166 |
+ async addWord() { // 단어 추가 |
|
158 | 167 |
if (this.newWord.eng && this.newWord.kor) { |
159 |
- const newWordWithFile = { ...this.newWord }; // 새 단어 객체 |
|
160 |
- this.words.push(newWordWithFile); |
|
168 |
+ const newWordWithFile = { |
|
169 |
+ ...this.newWord, |
|
170 |
+ file: [] // 파일 정보 저장을 위한 배열 추가 |
|
171 |
+ }; |
|
172 |
+ |
|
173 |
+ // 파일 매니지 ID가 있을 경우 파일 정보를 추가 |
|
174 |
+ if (newWordWithFile.fileMngId) { |
|
175 |
+ const fileInfo = await this.findFile(newWordWithFile.fileMngId); |
|
176 |
+ newWordWithFile.file = fileInfo; // 파일 정보 추가 |
|
177 |
+ } |
|
178 |
+ |
|
179 |
+ this.words.push(newWordWithFile); // 단어 추가 |
|
180 |
+ |
|
161 | 181 |
// 초기화 |
162 | 182 |
this.newWord.eng = ''; |
163 | 183 |
this.newWord.kor = ''; |
... | ... | @@ -177,6 +197,28 @@ |
177 | 197 |
|
178 | 198 |
cancelAction() { |
179 | 199 |
this.$router.go(-1); |
200 |
+ }, |
|
201 |
+ |
|
202 |
+ // 업로드한 파일 정보 가져오기 |
|
203 |
+ async findFile(fileMngId) { |
|
204 |
+ const vm = this; |
|
205 |
+ try { |
|
206 |
+ const response = await axios({ |
|
207 |
+ url: "/file/find.json", |
|
208 |
+ method: "post", |
|
209 |
+ headers: { |
|
210 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
211 |
+ }, |
|
212 |
+ data: { |
|
213 |
+ file_mng_id: fileMngId, |
|
214 |
+ }, |
|
215 |
+ }); |
|
216 |
+ console.log("fileInfo - response : ", response.data.list); |
|
217 |
+ return response.data.list; // 파일 정보를 반환 |
|
218 |
+ } catch (error) { |
|
219 |
+ console.log("result - error : ", error); |
|
220 |
+ return []; // 오류 발생 시 빈 배열 반환 |
|
221 |
+ } |
|
180 | 222 |
}, |
181 | 223 |
|
182 | 224 |
|
... | ... | @@ -259,14 +301,23 @@ |
259 | 301 |
wdBookId: vm.selectedWdBookId, |
260 | 302 |
}, |
261 | 303 |
}) |
262 |
- .then(function (response) { |
|
304 |
+ .then(async function (response) { |
|
263 | 305 |
console.log("dataList - response: ", response.data); |
264 | 306 |
vm.words = response.data.map(word => ({ |
265 | 307 |
eng: word.wdNm, |
266 | 308 |
kor: word.wdMnng, |
267 |
- fileMngId: word.fileMngId // 필요시 fileMngId도 포함 |
|
309 |
+ fileMngId: word.fileMngId, // 필요시 fileMngId도 포함 |
|
310 |
+ file: [] // 파일 정보를 저장할 배열 초기화 |
|
268 | 311 |
})); |
269 | 312 |
|
313 |
+ // 각 단어에 대해 파일 정보를 조회 |
|
314 |
+ for (const word of vm.words) { |
|
315 |
+ if (word.fileMngId) { |
|
316 |
+ word.file = await vm.findFile(word.fileMngId); // 파일 정보를 단어에 추가 |
|
317 |
+ } |
|
318 |
+ } |
|
319 |
+ |
|
320 |
+ console.log(vm.words); |
|
270 | 321 |
}) |
271 | 322 |
.catch(function (error) { |
272 | 323 |
console.log("dataList - error: ", error); |
... | ... | @@ -401,6 +452,36 @@ |
401 | 452 |
} |
402 | 453 |
}, |
403 | 454 |
|
455 |
+ // 단어장 삭제 |
|
456 |
+ deleteVoca() { |
|
457 |
+ const result = confirm('해당 단어장을 삭제 하시겠습니까?') |
|
458 |
+ if (result) { |
|
459 |
+ } else { |
|
460 |
+ alert("삭제를 취소했습니다") |
|
461 |
+ return; |
|
462 |
+ } |
|
463 |
+ |
|
464 |
+ axios({ |
|
465 |
+ url: "/wordbook/delete.json", |
|
466 |
+ method: "post", |
|
467 |
+ headers: { |
|
468 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
469 |
+ }, |
|
470 |
+ data: { |
|
471 |
+ "wdBookId": this.selectedWdBookId |
|
472 |
+ }, |
|
473 |
+ }) |
|
474 |
+ .then(response => { |
|
475 |
+ alert("삭제가 완료됐습니다"); |
|
476 |
+ this.goToPage('VocaList'); |
|
477 |
+ }) |
|
478 |
+ .catch(error => { |
|
479 |
+ console.error("fetchData - error: ", error); |
|
480 |
+ alert("삭제 중 오류가 발생했습니다."); |
|
481 |
+ }); |
|
482 |
+ }, |
|
483 |
+ |
|
484 |
+ |
|
404 | 485 |
// 단어장 정보 가져오기 |
405 | 486 |
wordBookFind() { |
406 | 487 |
const vm = this; |
--- client/views/pages/teacher/VocaInsert.vue
+++ client/views/pages/teacher/VocaInsert.vue
... | ... | @@ -49,15 +49,15 @@ |
49 | 49 |
<img src="../../../resources/img/btn39_120t_normal.png" alt=""> |
50 | 50 |
</button> |
51 | 51 |
</div> |
52 |
- |
|
53 | 52 |
<!-- 여기에 단어장에 소속될 단어들 태그 형태 리스트 --> |
54 | 53 |
<div v-for="(word, index) in words" :key="index" class="word-item flex align-center" style="gap: 10px;"> |
55 | 54 |
<span>{{ word.eng }} / {{ word.kor }}</span> |
55 |
+ <div v-for="(data, index2) in word.file" :key="index2"> |
|
56 |
+ / {{ data.fileNm }} |
|
57 |
+ </div> |
|
56 | 58 |
<button type="button" @click="removeWord(index)">삭제</button> |
57 | 59 |
</div> |
58 |
- |
|
59 | 60 |
</div> |
60 |
- |
|
61 | 61 |
</div> |
62 | 62 |
</div> |
63 | 63 |
<div class="flex justify-between mt50"> |
... | ... | @@ -215,10 +215,22 @@ |
215 | 215 |
}); |
216 | 216 |
}, |
217 | 217 |
|
218 |
- addWord() { // 단어 추가 |
|
218 |
+ |
|
219 |
+ async addWord() { // 단어 추가 |
|
219 | 220 |
if (this.newWord.eng && this.newWord.kor) { |
220 |
- const newWordWithFile = { ...this.newWord }; // 새 단어 객체 |
|
221 |
- this.words.push(newWordWithFile); |
|
221 |
+ const newWordWithFile = { |
|
222 |
+ ...this.newWord, |
|
223 |
+ file: [] // 파일 정보 저장을 위한 배열 추가 |
|
224 |
+ }; |
|
225 |
+ |
|
226 |
+ // 파일 매니지 ID가 있을 경우 파일 정보를 추가 |
|
227 |
+ if (newWordWithFile.fileMngId) { |
|
228 |
+ const fileInfo = await this.findFile(newWordWithFile.fileMngId); |
|
229 |
+ newWordWithFile.file = fileInfo; // 파일 정보 추가 |
|
230 |
+ } |
|
231 |
+ |
|
232 |
+ this.words.push(newWordWithFile); // 단어 추가 |
|
233 |
+ |
|
222 | 234 |
// 초기화 |
223 | 235 |
this.newWord.eng = ''; |
224 | 236 |
this.newWord.kor = ''; |
... | ... | @@ -227,7 +239,6 @@ |
227 | 239 |
console.log("단어 입력이 비어 있음"); |
228 | 240 |
} |
229 | 241 |
}, |
230 |
- |
|
231 | 242 |
removeWord(index) { // 단어 삭제 |
232 | 243 |
this.words.splice(index, 1); |
233 | 244 |
}, |
... | ... | @@ -276,7 +287,27 @@ |
276 | 287 |
throw new Error("파일 업로드에 실패했습니다."); |
277 | 288 |
} |
278 | 289 |
}, |
279 |
- |
|
290 |
+ // 업로드한 파일 정보 가져오기 |
|
291 |
+ async findFile(fileMngId) { |
|
292 |
+ const vm = this; |
|
293 |
+ try { |
|
294 |
+ const response = await axios({ |
|
295 |
+ url: "/file/find.json", |
|
296 |
+ method: "post", |
|
297 |
+ headers: { |
|
298 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
299 |
+ }, |
|
300 |
+ data: { |
|
301 |
+ file_mng_id: fileMngId, |
|
302 |
+ }, |
|
303 |
+ }); |
|
304 |
+ console.log("fileInfo - response : ", response.data.list); |
|
305 |
+ return response.data.list; // 파일 정보를 반환 |
|
306 |
+ } catch (error) { |
|
307 |
+ console.log("result - error : ", error); |
|
308 |
+ return []; // 오류 발생 시 빈 배열 반환 |
|
309 |
+ } |
|
310 |
+ }, |
|
280 | 311 |
|
281 | 312 |
async registerWordBook() { |
282 | 313 |
const vm = this; |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?