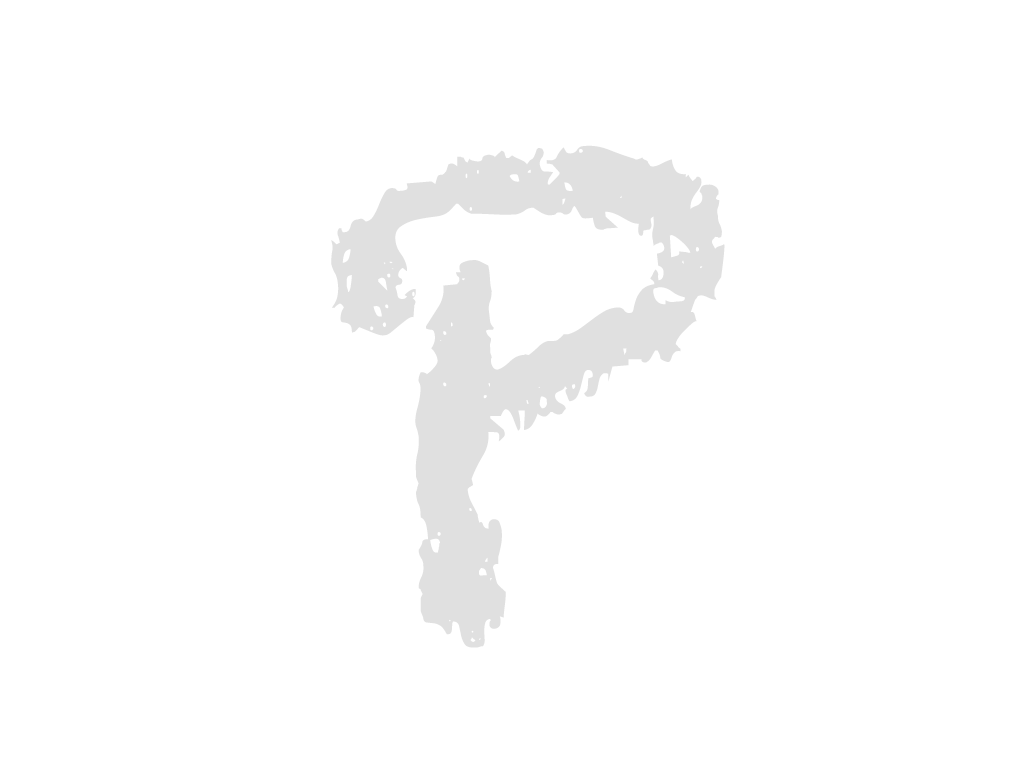
--- client/views/Login.vue
+++ client/views/Login.vue
... | ... | @@ -187,10 +187,7 @@ |
187 | 187 |
}).then(function (response) { |
188 | 188 |
|
189 | 189 |
const token = response.headers.get('Authorization'); |
190 |
- const Refreshtoken = response.headers.get('RefreshToken'); |
|
191 |
- |
|
192 | 190 |
store.dispatch('login', token); |
193 |
- localStorage.setItem("Refreshtoken", Refreshtoken); |
|
194 | 191 |
|
195 | 192 |
vm.branchPage(); |
196 | 193 |
}).catch(function (error) { |
... | ... | @@ -231,13 +228,13 @@ |
231 | 228 |
|
232 | 229 |
// 역할에 따라 페이지 이동 |
233 | 230 |
if (roles.includes("STUDENT")) { |
234 |
- vm.goToPage('Dashboard'); |
|
231 |
+ vm.goToPage('MyPlan'); |
|
235 | 232 |
} else if (roles.includes("TEACHER")) { |
236 | 233 |
vm.goToPage('Board'); |
237 | 234 |
} else if (roles.includes("PARENT")) { |
238 | 235 |
vm.goToPage('Main_p'); |
239 | 236 |
} else if (roles.includes("ADMIN")) { |
240 |
- vm.goToPage('Dashboard'); |
|
237 |
+ vm.goToPage('MyPlan'); |
|
241 | 238 |
} |
242 | 239 |
} else { |
243 | 240 |
console.error('Invalid token structure'); |
--- client/views/pages/AppRouter.js
+++ client/views/pages/AppRouter.js
... | ... | @@ -423,4 +423,52 @@ |
423 | 423 |
routes, |
424 | 424 |
}); |
425 | 425 |
|
426 |
+AppRouter.beforeEach((to, from, next) => { |
|
427 |
+ const token = localStorage.getItem('token'); |
|
428 |
+ |
|
429 |
+ if (!token && (to.name !== 'login' && to.name !== 'Join')) { |
|
430 |
+ // 토큰이 없고 로그인 페이지가 아닐 경우 로그인 페이지로 리다이렉트 |
|
431 |
+ next({ name: 'login' }); |
|
432 |
+ } else if (token) { |
|
433 |
+ // 토큰이 있을 경우 역할에 따른 접근 제어 |
|
434 |
+ const tokenParts = token.split('.'); |
|
435 |
+ const payload = tokenParts[1]; |
|
436 |
+ |
|
437 |
+ const base64 = payload.replace(/-/g, '+').replace(/_/g, '/'); |
|
438 |
+ |
|
439 |
+ const jsonPayload = decodeURIComponent( |
|
440 |
+ Array.prototype.map.call(atob(base64), c => { |
|
441 |
+ return '%' + ('00' + c.charCodeAt(0).toString(16)).slice(-2); |
|
442 |
+ }).join('') |
|
443 |
+ ); |
|
444 |
+ |
|
445 |
+ const userInfo = JSON.parse(jsonPayload); |
|
446 |
+ const teacherRoutes = routes[35].children.map(route => route.path); |
|
447 |
+ |
|
448 |
+ if (userInfo.author[0].authority === 'STUDENT') { |
|
449 |
+ if (teacherRoutes.includes(to.path) || to.name === 'Main_p') { |
|
450 |
+ next({ name: 'MyPlan' }); |
|
451 |
+ } else { |
|
452 |
+ next(); // 다른 페이지로 접근 허용 |
|
453 |
+ } |
|
454 |
+ } else if (userInfo.author[0].authority === 'TEACHER') { |
|
455 |
+ if (!teacherRoutes.includes(to.path)) { |
|
456 |
+ next({ name: 'Home' }); |
|
457 |
+ } else { |
|
458 |
+ next(); |
|
459 |
+ } |
|
460 |
+ } else if (userInfo.author[0].authority === 'PARENT') { |
|
461 |
+ if (to.name !== 'Main_p') { |
|
462 |
+ next({ name: 'Main_p' }); |
|
463 |
+ } else { |
|
464 |
+ next(); |
|
465 |
+ } |
|
466 |
+ } else { |
|
467 |
+ next(); |
|
468 |
+ } |
|
469 |
+ } else { |
|
470 |
+ next(); |
|
471 |
+ } |
|
472 |
+}); |
|
473 |
+ |
|
426 | 474 |
export default AppRouter; |
--- client/views/pages/main/Dashboard.vue
+++ client/views/pages/main/Dashboard.vue
... | ... | @@ -16,78 +16,40 @@ |
16 | 16 |
</div> |
17 | 17 |
<div class="race-box"> |
18 | 18 |
<div class="rabbit-start"> |
19 |
- <img |
|
20 |
- src="../../../resources/img/img09_s.png" |
|
21 |
- alt="" |
|
22 |
- :style="{ display: rabbitPos[0] ? 'block' : 'none' }" |
|
23 |
- /> |
|
19 |
+ <img src="../../../resources/img/img09_s.png" alt="" :style="{ display: rabbitPos[0] ? 'block' : 'none' }" /> |
|
24 | 20 |
</div> |
25 | 21 |
<div class="rcon flex justify-end mb5"> |
26 | 22 |
<div class="race-btn" @click="[storeLearningId(labeledItems[0])]"> |
27 | 23 |
<div class="rabbit-running"> |
28 |
- <img |
|
29 |
- src="../../../resources/img/img09_s.png" |
|
30 |
- alt="" |
|
31 |
- :style="{ display: rabbitPos[1] ? 'block' : 'none' }" |
|
32 |
- /> |
|
24 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
25 |
+ :style="{ display: rabbitPos[1] ? 'block' : 'none' }" /> |
|
33 | 26 |
</div> |
34 |
- <button |
|
35 |
- class="popTxt" |
|
36 |
- v-for="(item, index) in items" |
|
37 |
- :key="index" |
|
38 |
- @click="toggleImage(index)" |
|
39 |
- data-num="1" |
|
40 |
- > |
|
41 |
- <img |
|
42 |
- :src=" |
|
43 |
- labeledItems[0].label.length >= 4 |
|
44 |
- ? item.imgSrc3 |
|
45 |
- : item.imgSrc1 |
|
46 |
- " |
|
47 |
- :style="{ display: !rabbitCompl[1] ? 'block' : 'none' }" |
|
48 |
- /> |
|
49 |
- <img |
|
50 |
- :src=" |
|
51 |
- labeledItems[0].label.length >= 4 |
|
52 |
- ? item.imgSrc4 |
|
53 |
- : item.imgSrc2 |
|
54 |
- " |
|
55 |
- :style="{ display: rabbitCompl[1] ? 'block' : 'none' }" |
|
56 |
- /> |
|
27 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" data-num="1"> |
|
28 |
+ <img :src="labeledItems[0].label.length >= 4 |
|
29 |
+ ? item.imgSrc3 |
|
30 |
+ : item.imgSrc1 |
|
31 |
+ " :style="{ display: !rabbitCompl[1] ? 'block' : 'none' }" /> |
|
32 |
+ <img :src="labeledItems[0].label.length >= 4 |
|
33 |
+ ? item.imgSrc4 |
|
34 |
+ : item.imgSrc2 |
|
35 |
+ " :style="{ display: rabbitCompl[1] ? 'block' : 'none' }" /> |
|
57 | 36 |
</button> |
58 | 37 |
<p>{{ labeledItems[0].label }}</p> |
59 | 38 |
</div> |
60 | 39 |
<div class="race-btn" @click="[storeLearningId(labeledItems[1])]"> |
61 | 40 |
<div class="rabbit-running"> |
62 |
- <img |
|
63 |
- src="../../../resources/img/img09_s.png" |
|
64 |
- alt="" |
|
65 |
- :style="{ display: rabbitPos[2] ? 'block' : 'none' }" |
|
66 |
- /> |
|
41 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
42 |
+ :style="{ display: rabbitPos[2] ? 'block' : 'none' }" /> |
|
67 | 43 |
</div> |
68 |
- <button |
|
69 |
- class="popTxt" |
|
70 |
- v-for="(item, index) in items" |
|
71 |
- :key="index" |
|
72 |
- @click="toggleImage(index)" |
|
73 |
- data-num="2" |
|
74 |
- > |
|
75 |
- <img |
|
76 |
- :src=" |
|
77 |
- labeledItems[1].label.length >= 4 |
|
78 |
- ? item.imgSrc3 |
|
79 |
- : item.imgSrc1 |
|
80 |
- " |
|
81 |
- :style="{ display: !rabbitCompl[2] ? 'block' : 'none' }" |
|
82 |
- /> |
|
83 |
- <img |
|
84 |
- :src=" |
|
85 |
- labeledItems[1].label.length >= 4 |
|
86 |
- ? item.imgSrc4 |
|
87 |
- : item.imgSrc2 |
|
88 |
- " |
|
89 |
- :style="{ display: rabbitCompl[2] ? 'block' : 'none' }" |
|
90 |
- /> |
|
44 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" data-num="2"> |
|
45 |
+ <img :src="labeledItems[1].label.length >= 4 |
|
46 |
+ ? item.imgSrc3 |
|
47 |
+ : item.imgSrc1 |
|
48 |
+ " :style="{ display: !rabbitCompl[2] ? 'block' : 'none' }" /> |
|
49 |
+ <img :src="labeledItems[1].label.length >= 4 |
|
50 |
+ ? item.imgSrc4 |
|
51 |
+ : item.imgSrc2 |
|
52 |
+ " :style="{ display: rabbitCompl[2] ? 'block' : 'none' }" /> |
|
91 | 53 |
</button> |
92 | 54 |
<p>{{ labeledItems[1].label }}</p> |
93 | 55 |
</div> |
... | ... | @@ -95,350 +57,173 @@ |
95 | 57 |
<div class="lcon flex justify-between mb5"> |
96 | 58 |
<div class="race-btn" @click="[storeLearningId(labeledItems[6])]"> |
97 | 59 |
<div class="rabbit-running"> |
98 |
- <img |
|
99 |
- src="../../../resources/img/img09_s.png" |
|
100 |
- alt="" |
|
101 |
- :style="{ display: rabbitPos[7] ? 'block' : 'none' }" |
|
102 |
- /> |
|
60 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
61 |
+ :style="{ display: rabbitPos[7] ? 'block' : 'none' }" /> |
|
103 | 62 |
</div> |
104 |
- <button |
|
105 |
- class="popTxt" |
|
106 |
- v-for="(item, index) in items" |
|
107 |
- :key="index" |
|
108 |
- @click="toggleImage(index)" |
|
109 |
- data-num="7" |
|
110 |
- > |
|
111 |
- <img |
|
112 |
- :src=" |
|
113 |
- labeledItems[6].label.length >= 4 |
|
114 |
- ? item.imgSrc3 |
|
115 |
- : item.imgSrc1 |
|
116 |
- " |
|
117 |
- :style="{ display: !rabbitCompl[7] ? 'block' : 'none' }" |
|
118 |
- /> |
|
119 |
- <img |
|
120 |
- :src=" |
|
121 |
- labeledItems[6].label.length >= 4 |
|
122 |
- ? item.imgSrc4 |
|
123 |
- : item.imgSrc2 |
|
124 |
- " |
|
125 |
- :style="{ display: rabbitCompl[7] ? 'block' : 'none' }" |
|
126 |
- /> |
|
63 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" data-num="7"> |
|
64 |
+ <img :src="labeledItems[6].label.length >= 4 |
|
65 |
+ ? item.imgSrc3 |
|
66 |
+ : item.imgSrc1 |
|
67 |
+ " :style="{ display: !rabbitCompl[7] ? 'block' : 'none' }" /> |
|
68 |
+ <img :src="labeledItems[6].label.length >= 4 |
|
69 |
+ ? item.imgSrc4 |
|
70 |
+ : item.imgSrc2 |
|
71 |
+ " :style="{ display: rabbitCompl[7] ? 'block' : 'none' }" /> |
|
127 | 72 |
</button> |
128 | 73 |
<p>{{ labeledItems[6].label }}</p> |
129 | 74 |
</div> |
130 |
- <div |
|
131 |
- class="race-btn" |
|
132 |
- @click="[storeLearningId(labeledItems[5]),]" |
|
133 |
- > |
|
75 |
+ <div class="race-btn" @click="[storeLearningId(labeledItems[5]),]"> |
|
134 | 76 |
<div class="rabbit-running"> |
135 |
- <img |
|
136 |
- src="../../../resources/img/img09_s.png" |
|
137 |
- alt="" |
|
138 |
- :style="{ display: rabbitPos[6] ? 'block' : 'none' }" |
|
139 |
- /> |
|
77 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
78 |
+ :style="{ display: rabbitPos[6] ? 'block' : 'none' }" /> |
|
140 | 79 |
</div> |
141 |
- <button |
|
142 |
- class="popTxt" |
|
143 |
- v-for="(item, index) in items" |
|
144 |
- :key="index" |
|
145 |
- @click="toggleImage(index)" |
|
146 |
- data-num="6" |
|
147 |
- > |
|
148 |
- <img |
|
149 |
- :src=" |
|
150 |
- labeledItems[5].label.length >= 4 |
|
151 |
- ? item.imgSrc3 |
|
152 |
- : item.imgSrc1 |
|
153 |
- " |
|
154 |
- :style="{ display: !rabbitCompl[6] ? 'block' : 'none' }" |
|
155 |
- /> |
|
156 |
- <img |
|
157 |
- :src=" |
|
158 |
- labeledItems[5].label.length >= 4 |
|
159 |
- ? item.imgSrc4 |
|
160 |
- : item.imgSrc2 |
|
161 |
- " |
|
162 |
- :style="{ display: rabbitCompl[6] ? 'block' : 'none' }" |
|
163 |
- /> |
|
80 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" data-num="6"> |
|
81 |
+ <img :src="labeledItems[5].label.length >= 4 |
|
82 |
+ ? item.imgSrc3 |
|
83 |
+ : item.imgSrc1 |
|
84 |
+ " :style="{ display: !rabbitCompl[6] ? 'block' : 'none' }" /> |
|
85 |
+ <img :src="labeledItems[5].label.length >= 4 |
|
86 |
+ ? item.imgSrc4 |
|
87 |
+ : item.imgSrc2 |
|
88 |
+ " :style="{ display: rabbitCompl[6] ? 'block' : 'none' }" /> |
|
164 | 89 |
</button> |
165 | 90 |
<p>{{ labeledItems[5].label }}</p> |
166 | 91 |
</div> |
167 | 92 |
<div class="race-btn" @click="[storeLearningId(labeledItems[4])]"> |
168 | 93 |
<div class="rabbit-running"> |
169 |
- <img |
|
170 |
- src="../../../resources/img/img09_s.png" |
|
171 |
- alt="" |
|
172 |
- :style="{ display: rabbitPos[5] ? 'block' : 'none' }" |
|
173 |
- /> |
|
94 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
95 |
+ :style="{ display: rabbitPos[5] ? 'block' : 'none' }" /> |
|
174 | 96 |
</div> |
175 |
- <button |
|
176 |
- class="popTxt" |
|
177 |
- v-for="(item, index) in items" |
|
178 |
- :key="index" |
|
179 |
- @click="toggleImage(index)" |
|
180 |
- data-num="5" |
|
181 |
- > |
|
182 |
- <img |
|
183 |
- :src=" |
|
184 |
- labeledItems[4].label.length >= 4 |
|
185 |
- ? item.imgSrc3 |
|
186 |
- : item.imgSrc1 |
|
187 |
- " |
|
188 |
- :style="{ display: !rabbitCompl[5] ? 'block' : 'none' }" |
|
189 |
- /> |
|
190 |
- <img |
|
191 |
- :src=" |
|
192 |
- labeledItems[4].label.length >= 4 |
|
193 |
- ? item.imgSrc4 |
|
194 |
- : item.imgSrc2 |
|
195 |
- " |
|
196 |
- :style="{ display: rabbitCompl[5] ? 'block' : 'none' }" |
|
197 |
- /> |
|
97 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" data-num="5"> |
|
98 |
+ <img :src="labeledItems[4].label.length >= 4 |
|
99 |
+ ? item.imgSrc3 |
|
100 |
+ : item.imgSrc1 |
|
101 |
+ " :style="{ display: !rabbitCompl[5] ? 'block' : 'none' }" /> |
|
102 |
+ <img :src="labeledItems[4].label.length >= 4 |
|
103 |
+ ? item.imgSrc4 |
|
104 |
+ : item.imgSrc2 |
|
105 |
+ " :style="{ display: rabbitCompl[5] ? 'block' : 'none' }" /> |
|
198 | 106 |
</button> |
199 | 107 |
<p>{{ labeledItems[4].label }}</p> |
200 | 108 |
</div> |
201 | 109 |
<div class="race-btn" @click="[storeLearningId(labeledItems[3])]"> |
202 | 110 |
<div class="rabbit-running"> |
203 |
- <img |
|
204 |
- src="../../../resources/img/img09_s.png" |
|
205 |
- alt="" |
|
206 |
- :style="{ display: rabbitPos[4] ? 'block' : 'none' }" |
|
207 |
- /> |
|
111 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
112 |
+ :style="{ display: rabbitPos[4] ? 'block' : 'none' }" /> |
|
208 | 113 |
</div> |
209 |
- <button |
|
210 |
- class="popTxt" |
|
211 |
- v-for="(item, index) in items" |
|
212 |
- :key="index" |
|
213 |
- @click="toggleImage(index)" |
|
214 |
- data-num="4" |
|
215 |
- > |
|
216 |
- <img |
|
217 |
- :src=" |
|
218 |
- labeledItems[3].label.length >= 4 |
|
219 |
- ? item.imgSrc3 |
|
220 |
- : item.imgSrc1 |
|
221 |
- " |
|
222 |
- :style="{ display: !rabbitCompl[4] ? 'block' : 'none' }" |
|
223 |
- /> |
|
224 |
- <img |
|
225 |
- :src=" |
|
226 |
- labeledItems[3].label.length >= 4 |
|
227 |
- ? item.imgSrc4 |
|
228 |
- : item.imgSrc2 |
|
229 |
- " |
|
230 |
- :style="{ display: rabbitCompl[4] ? 'block' : 'none' }" |
|
231 |
- /> |
|
114 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" data-num="4"> |
|
115 |
+ <img :src="labeledItems[3].label.length >= 4 |
|
116 |
+ ? item.imgSrc3 |
|
117 |
+ : item.imgSrc1 |
|
118 |
+ " :style="{ display: !rabbitCompl[4] ? 'block' : 'none' }" /> |
|
119 |
+ <img :src="labeledItems[3].label.length >= 4 |
|
120 |
+ ? item.imgSrc4 |
|
121 |
+ : item.imgSrc2 |
|
122 |
+ " :style="{ display: rabbitCompl[4] ? 'block' : 'none' }" /> |
|
232 | 123 |
</button> |
233 | 124 |
<p>{{ labeledItems[3].label }}</p> |
234 | 125 |
</div> |
235 | 126 |
<div class="race-btn" @click="[storeLearningId(labeledItems[2])]"> |
236 | 127 |
<div class="rabbit-running"> |
237 |
- <img |
|
238 |
- src="../../../resources/img/img09_s.png" |
|
239 |
- alt="" |
|
240 |
- :style="{ display: rabbitPos[3] ? 'block' : 'none' }" |
|
241 |
- /> |
|
128 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
129 |
+ :style="{ display: rabbitPos[3] ? 'block' : 'none' }" /> |
|
242 | 130 |
</div> |
243 |
- <button |
|
244 |
- class="popTxt" |
|
245 |
- v-for="(item, index) in items" |
|
246 |
- :key="index" |
|
247 |
- @click="toggleImage(index)" |
|
248 |
- data-num="3" |
|
249 |
- > |
|
250 |
- <img |
|
251 |
- :src=" |
|
252 |
- labeledItems[2].label.length >= 4 |
|
253 |
- ? item.imgSrc3 |
|
254 |
- : item.imgSrc1 |
|
255 |
- " |
|
256 |
- :style="{ display: !rabbitCompl[3] ? 'block' : 'none' }" |
|
257 |
- /> |
|
258 |
- <img |
|
259 |
- :src=" |
|
260 |
- labeledItems[2].label.length >= 4 |
|
261 |
- ? item.imgSrc4 |
|
262 |
- : item.imgSrc2 |
|
263 |
- " |
|
264 |
- :style="{ display: rabbitCompl[3] ? 'block' : 'none' }" |
|
265 |
- /> |
|
131 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" data-num="3"> |
|
132 |
+ <img :src="labeledItems[2].label.length >= 4 |
|
133 |
+ ? item.imgSrc3 |
|
134 |
+ : item.imgSrc1 |
|
135 |
+ " :style="{ display: !rabbitCompl[3] ? 'block' : 'none' }" /> |
|
136 |
+ <img :src="labeledItems[2].label.length >= 4 |
|
137 |
+ ? item.imgSrc4 |
|
138 |
+ : item.imgSrc2 |
|
139 |
+ " :style="{ display: rabbitCompl[3] ? 'block' : 'none' }" /> |
|
266 | 140 |
</button> |
267 | 141 |
<p>{{ labeledItems[2].label }}</p> |
268 | 142 |
</div> |
269 | 143 |
</div> |
270 | 144 |
<div class="rcon flex"> |
271 |
- <div |
|
272 |
- class="race-btn" |
|
273 |
- @click=" |
|
274 |
- [ |
|
275 |
- goToPage('Chapter8'), |
|
276 |
- storeLearningId(labeledItems[7].learning_id), |
|
277 |
- ] |
|
278 |
- " |
|
279 |
- > |
|
145 |
+ <div class="race-btn" @click=" |
|
146 |
+ [storeLearningId(labeledItems[7]),] |
|
147 |
+ "> |
|
280 | 148 |
<div class="rabbit-running"> |
281 |
- <img |
|
282 |
- src="../../../resources/img/img09_s.png" |
|
283 |
- alt="" |
|
284 |
- :style="{ display: rabbitPos[8] ? 'block' : 'none' }" |
|
285 |
- /> |
|
149 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
150 |
+ :style="{ display: rabbitPos[8] ? 'block' : 'none' }" /> |
|
286 | 151 |
</div> |
287 |
- <button |
|
288 |
- class="popTxt" |
|
289 |
- v-for="(item, index) in items" |
|
290 |
- :key="index" |
|
291 |
- @click="toggleImage(index)" |
|
292 |
- data-num="8" |
|
293 |
- > |
|
294 |
- <img |
|
295 |
- :src=" |
|
296 |
- labeledItems[7].label.length >= 4 |
|
297 |
- ? item.imgSrc3 |
|
298 |
- : item.imgSrc1 |
|
299 |
- " |
|
300 |
- :style="{ display: !rabbitCompl[8] ? 'block' : 'none' }" |
|
301 |
- /> |
|
302 |
- <img |
|
303 |
- :src=" |
|
304 |
- labeledItems[7].label.length >= 4 |
|
305 |
- ? item.imgSrc4 |
|
306 |
- : item.imgSrc2 |
|
307 |
- " |
|
308 |
- :style="{ display: rabbitCompl[8] ? 'block' : 'none' }" |
|
309 |
- /> |
|
152 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" data-num="8"> |
|
153 |
+ <img :src="labeledItems[7].label.length >= 4 |
|
154 |
+ ? item.imgSrc3 |
|
155 |
+ : item.imgSrc1 |
|
156 |
+ " :style="{ display: !rabbitCompl[8] ? 'block' : 'none' }" /> |
|
157 |
+ <img :src="labeledItems[7].label.length >= 4 |
|
158 |
+ ? item.imgSrc4 |
|
159 |
+ : item.imgSrc2 |
|
160 |
+ " :style="{ display: rabbitCompl[8] ? 'block' : 'none' }" /> |
|
310 | 161 |
</button> |
311 | 162 |
<p class="long">{{ labeledItems[7].label }}</p> |
312 | 163 |
</div> |
313 | 164 |
<div class="race-btn" @click="[storeLearningId(labeledItems[8])]"> |
314 | 165 |
<div class="rabbit-running"> |
315 |
- <img |
|
316 |
- src="../../../resources/img/img09_s.png" |
|
317 |
- alt="" |
|
318 |
- :style="{ display: rabbitPos[9] ? 'block' : 'none' }" |
|
319 |
- /> |
|
166 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
167 |
+ :style="{ display: rabbitPos[9] ? 'block' : 'none' }" /> |
|
320 | 168 |
</div> |
321 |
- <button |
|
322 |
- class="popTxt" |
|
323 |
- v-for="(item, index) in items" |
|
324 |
- :key="index" |
|
325 |
- @click="toggleImage(index)" |
|
326 |
- data-num="9" |
|
327 |
- > |
|
328 |
- <img |
|
329 |
- :src=" |
|
330 |
- labeledItems[8].label.length >= 4 |
|
331 |
- ? item.imgSrc3 |
|
332 |
- : item.imgSrc1 |
|
333 |
- " |
|
334 |
- :style="{ display: !rabbitCompl[9] ? 'block' : 'none' }" |
|
335 |
- /> |
|
336 |
- <img |
|
337 |
- :src=" |
|
338 |
- labeledItems[8].label.length >= 4 |
|
339 |
- ? item.imgSrc4 |
|
340 |
- : item.imgSrc2 |
|
341 |
- " |
|
342 |
- :style="{ display: rabbitCompl[9] ? 'block' : 'none' }" |
|
343 |
- /> |
|
169 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" data-num="9"> |
|
170 |
+ <img :src="labeledItems[8].label.length >= 4 |
|
171 |
+ ? item.imgSrc3 |
|
172 |
+ : item.imgSrc1 |
|
173 |
+ " :style="{ display: !rabbitCompl[9] ? 'block' : 'none' }" /> |
|
174 |
+ <img :src="labeledItems[8].label.length >= 4 |
|
175 |
+ ? item.imgSrc4 |
|
176 |
+ : item.imgSrc2 |
|
177 |
+ " :style="{ display: rabbitCompl[9] ? 'block' : 'none' }" /> |
|
344 | 178 |
</button> |
345 | 179 |
<p>{{ labeledItems[8].label }}</p> |
346 | 180 |
</div> |
347 |
- <div |
|
348 |
- class="race-btn" |
|
349 |
- @click="[storeLearningId(labeledItems[9])]" |
|
350 |
- > |
|
181 |
+ <div class="race-btn" @click="[storeLearningId(labeledItems[9])]"> |
|
351 | 182 |
<div class="rabbit-running"> |
352 |
- <img |
|
353 |
- src="../../../resources/img/img09_s.png" |
|
354 |
- alt="" |
|
355 |
- :style="{ display: rabbitPos[10] ? 'block' : 'none' }" |
|
356 |
- /> |
|
183 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
184 |
+ :style="{ display: rabbitPos[10] ? 'block' : 'none' }" /> |
|
357 | 185 |
</div> |
358 |
- <button |
|
359 |
- class="popTxt" |
|
360 |
- v-for="(item, index) in items" |
|
361 |
- :key="index" |
|
362 |
- @click="toggleImage(index)" |
|
363 |
- data-num="10" |
|
364 |
- > |
|
365 |
- <img |
|
366 |
- :src=" |
|
367 |
- labeledItems[9].label.length >= 4 |
|
368 |
- ? item.imgSrc3 |
|
369 |
- : item.imgSrc1 |
|
370 |
- " |
|
371 |
- :style="{ display: !rabbitCompl[10] ? 'block' : 'none' }" |
|
372 |
- /> |
|
373 |
- <img |
|
374 |
- :src=" |
|
375 |
- labeledItems[9].label.length >= 4 |
|
376 |
- ? item.imgSrc4 |
|
377 |
- : item.imgSrc2 |
|
378 |
- " |
|
379 |
- :style="{ display: rabbitCompl[10] ? 'block' : 'none' }" |
|
380 |
- /> |
|
186 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" @click="toggleImage(index)" |
|
187 |
+ data-num="10"> |
|
188 |
+ <img :src="labeledItems[9].label.length >= 4 |
|
189 |
+ ? item.imgSrc3 |
|
190 |
+ : item.imgSrc1 |
|
191 |
+ " :style="{ display: !rabbitCompl[10] ? 'block' : 'none' }" /> |
|
192 |
+ <img :src="labeledItems[9].label.length >= 4 |
|
193 |
+ ? item.imgSrc4 |
|
194 |
+ : item.imgSrc2 |
|
195 |
+ " :style="{ display: rabbitCompl[10] ? 'block' : 'none' }" /> |
|
381 | 196 |
</button> |
382 | 197 |
<p>{{ labeledItems[9].label }}</p> |
383 | 198 |
</div> |
384 | 199 |
|
385 |
- <div |
|
386 |
- class="race-btn" |
|
387 |
- @click=" |
|
388 |
- [ |
|
389 |
- goToPage('Chapter2_8'), |
|
390 |
- storeLearningId(labeledItems[10].learning_id), |
|
391 |
- ] |
|
392 |
- " |
|
393 |
- > |
|
200 |
+ <div class="race-btn" @click=" |
|
201 |
+ [storeLearningId(labeledItems[10]),] |
|
202 |
+ "> |
|
394 | 203 |
<div class="rabbit-running"> |
395 |
- <img |
|
396 |
- src="../../../resources/img/img09_s.png" |
|
397 |
- alt="" |
|
398 |
- :style="{ display: rabbitPos[11] ? 'block' : 'none' }" |
|
399 |
- /> |
|
204 |
+ <img src="../../../resources/img/img09_s.png" alt="" |
|
205 |
+ :style="{ display: rabbitPos[11] ? 'block' : 'none' }" /> |
|
400 | 206 |
</div> |
401 |
- <button |
|
402 |
- class="popTxt" |
|
403 |
- v-for="(item, index) in items" |
|
404 |
- :key="index" |
|
405 |
- @click="toggleImageAndShowPopup(index, '11')" |
|
406 |
- data-num="11" |
|
407 |
- > |
|
408 |
- <img |
|
409 |
- :src=" |
|
410 |
- labeledItems[10].label.length >= 4 |
|
411 |
- ? item.imgSrc3 |
|
412 |
- : item.imgSrc1 |
|
413 |
- " |
|
414 |
- :style="{ display: !rabbitCompl[11] ? 'block' : 'none' }" |
|
415 |
- /> |
|
416 |
- <img |
|
417 |
- :src=" |
|
418 |
- labeledItems[10].label.length >= 4 |
|
419 |
- ? item.imgSrc4 |
|
420 |
- : item.imgSrc2 |
|
421 |
- " |
|
422 |
- :style="{ display: rabbitCompl[11] ? 'block' : 'none' }" |
|
423 |
- /> |
|
207 |
+ <button class="popTxt" v-for="(item, index) in items" :key="index" |
|
208 |
+ @click="toggleImageAndShowPopup(index, '11')" data-num="11"> |
|
209 |
+ <img :src="labeledItems[10].label.length >= 4 |
|
210 |
+ ? item.imgSrc3 |
|
211 |
+ : item.imgSrc1 |
|
212 |
+ " :style="{ display: !rabbitCompl[11] ? 'block' : 'none' }" /> |
|
213 |
+ <img :src="labeledItems[10].label.length >= 4 |
|
214 |
+ ? item.imgSrc4 |
|
215 |
+ : item.imgSrc2 |
|
216 |
+ " :style="{ display: rabbitCompl[11] ? 'block' : 'none' }" /> |
|
424 | 217 |
</button> |
425 | 218 |
<p class="long">{{ labeledItems[10].label }}</p> |
426 | 219 |
</div> |
427 | 220 |
</div> |
428 | 221 |
<div class="race-btn"> |
429 | 222 |
<div class="rabbit-running" style="display: flex"> |
430 |
- <img |
|
431 |
- class="rabbit-end" |
|
432 |
- src="../../../resources/img/img138_72s.png" |
|
433 |
- alt="" |
|
434 |
- :style="{ display: rabbitEnd ? 'block' : 'none' }" |
|
435 |
- /> |
|
436 |
- <img |
|
437 |
- class="fireworks-end" |
|
438 |
- src="../../../resources/img/fireworks.gif" |
|
439 |
- alt="" |
|
440 |
- :style="{ display: rabbitEnd ? 'block' : 'none' }" |
|
441 |
- /> |
|
223 |
+ <img class="rabbit-end" src="../../../resources/img/img138_72s.png" alt="" |
|
224 |
+ :style="{ display: rabbitEnd ? 'block' : 'none' }" /> |
|
225 |
+ <img class="fireworks-end" src="../../../resources/img/fireworks.gif" alt="" |
|
226 |
+ :style="{ display: rabbitEnd ? 'block' : 'none' }" /> |
|
442 | 227 |
</div> |
443 | 228 |
</div> |
444 | 229 |
</div> |
... | ... | @@ -446,17 +231,9 @@ |
446 | 231 |
<!-- 팝업 --> |
447 | 232 |
<div v-show="searchOpen2" class="popup-wrap"> |
448 | 233 |
<div class="popup-box"> |
449 |
- <button |
|
450 |
- type="button" |
|
451 |
- class="popup-close-btn" |
|
452 |
- style="position: absolute; top: 10px; right: 10px" |
|
453 |
- @click="closeModal" |
|
454 |
- > |
|
455 |
- <svg-icon |
|
456 |
- type="mdi" |
|
457 |
- :path="mdiWindowClose" |
|
458 |
- class="close-btn" |
|
459 |
- ></svg-icon> |
|
234 |
+ <button type="button" class="popup-close-btn" style="position: absolute; top: 10px; right: 10px" |
|
235 |
+ @click="closeModal"> |
|
236 |
+ <svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon> |
|
460 | 237 |
</button> |
461 | 238 |
|
462 | 239 |
<div class="mb30 text-ct"> |
... | ... | @@ -466,12 +243,7 @@ |
466 | 243 |
</p> |
467 | 244 |
</div> |
468 | 245 |
<div class="flex justify-center"> |
469 |
- <button |
|
470 |
- type="button" |
|
471 |
- title="사진촬영" |
|
472 |
- class="new-btn" |
|
473 |
- @click="openCameraModal" |
|
474 |
- > |
|
246 |
+ <button type="button" title="사진촬영" class="new-btn" @click="openCameraModal"> |
|
475 | 247 |
사진 촬영 |
476 | 248 |
</button> |
477 | 249 |
</div> |
... | ... | @@ -483,29 +255,15 @@ |
483 | 255 |
<div class="flex mb10 justify-between"> |
484 | 256 |
<p class="popup-title">사진 촬영</p> |
485 | 257 |
<button type="button" class="popup-close-btn" @click="closeModal"> |
486 |
- <svg-icon |
|
487 |
- type="mdi" |
|
488 |
- :path="mdiWindowClose" |
|
489 |
- class="close-btn" |
|
490 |
- ></svg-icon> |
|
258 |
+ <svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon> |
|
491 | 259 |
</button> |
492 | 260 |
</div> |
493 | 261 |
<div class="box"> |
494 | 262 |
<div style="width: 100%"> |
495 | 263 |
<div id="container" ref="container"> |
496 |
- <video |
|
497 |
- v-if="!photoTaken" |
|
498 |
- autoplay="true" |
|
499 |
- ref="modalVideoElement" |
|
500 |
- class="mirrored" |
|
501 |
- @canplay="onVideoLoaded" |
|
502 |
- style="position: absolute; height: 100%" |
|
503 |
- ></video> |
|
504 |
- <img |
|
505 |
- src="../../../resources/img/camera-rabbit.png" |
|
506 |
- class="camera-rabbit" |
|
507 |
- style="position: absolute" |
|
508 |
- /> |
|
264 |
+ <video v-if="!photoTaken" autoplay="true" ref="modalVideoElement" class="mirrored" |
|
265 |
+ @canplay="onVideoLoaded" style="position: absolute; height: 100%"></video> |
|
266 |
+ <img src="../../../resources/img/camera-rabbit.png" class="camera-rabbit" style="position: absolute" /> |
|
509 | 267 |
<canvas ref="canvas" style="pointer-events: none"></canvas> |
510 | 268 |
</div> |
511 | 269 |
</div> |
... | ... | @@ -518,17 +276,8 @@ |
518 | 276 |
</div> |
519 | 277 |
|
520 | 278 |
<div class="complete-wrap myphoto"> |
521 |
- <button |
|
522 |
- class="login-btn mt10" |
|
523 |
- type="submit" |
|
524 |
- style="width: 100%" |
|
525 |
- @click="finishSchedule" |
|
526 |
- > |
|
527 |
- <img |
|
528 |
- src="../../../resources/img/btn07_s.png" |
|
529 |
- alt="" |
|
530 |
- style="width: 100%; height: 100px" |
|
531 |
- /> |
|
279 |
+ <button class="login-btn mt10" type="submit" style="width: 100%" @click="finishSchedule"> |
|
280 |
+ <img src="../../../resources/img/btn07_s.png" alt="" style="width: 100%; height: 100px" /> |
|
532 | 281 |
<p>학습 종료하기</p> |
533 | 282 |
</button> |
534 | 283 |
<h2 class="mb40 mt10">이 단원을 끝낸 친구들</h2> |
... | ... | @@ -536,19 +285,9 @@ |
536 | 285 |
<article class="flex-column"> |
537 | 286 |
<div class="flex-row"> |
538 | 287 |
<div class="flex" style="gap: 5px; flex-wrap: wrap"> |
539 |
- <div |
|
540 |
- v-for="(image, index) in images" |
|
541 |
- :key="image.fileId" |
|
542 |
- @click="buttonSearch(image)" |
|
543 |
- class="photo" |
|
544 |
- style="margin-bottom: 5px" |
|
545 |
- > |
|
546 |
- <img |
|
547 |
- :src="image.url" |
|
548 |
- :alt="image.fileNm" |
|
549 |
- reloadable="true" |
|
550 |
- style="height: 100%" |
|
551 |
- /> |
|
288 |
+ <div v-for="(image, index) in images" :key="image.fileId" @click="buttonSearch(image)" class="photo" |
|
289 |
+ style="margin-bottom: 5px"> |
|
290 |
+ <img :src="image.url" :alt="image.fileNm" reloadable="true" style="height: 100%" /> |
|
552 | 291 |
</div> |
553 | 292 |
</div> |
554 | 293 |
</div> |
... | ... | @@ -557,51 +296,27 @@ |
557 | 296 |
<div class="popup-box" style="top: 50%"> |
558 | 297 |
<div class="flex mb10 justify-between"> |
559 | 298 |
<button type="button" class="popup-close-btn" @click="closeModal"> |
560 |
- <svg-icon |
|
561 |
- type="mdi" |
|
562 |
- :path="mdiWindowClose" |
|
563 |
- class="close-btn" |
|
564 |
- ></svg-icon> |
|
299 |
+ <svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon> |
|
565 | 300 |
</button> |
566 | 301 |
</div> |
567 | 302 |
<div class="box"> |
568 | 303 |
<div style="width: 910px; height: 680px"> |
569 |
- <img |
|
570 |
- :src="selectedImage.image" |
|
571 |
- alt="Image" |
|
572 |
- @error="onImageError" |
|
573 |
- reloadable="true" |
|
574 |
- /> |
|
304 |
+ <img :src="selectedImage.image" alt="Image" @error="onImageError" reloadable="true" /> |
|
575 | 305 |
</div> |
576 | 306 |
</div> |
577 | 307 |
<div class="flex justify-between mt20"> |
578 | 308 |
<div class="text flex"> |
579 | 309 |
<p class="title2 date ml30">{{ selectedImage.date }}</p> |
580 |
- <span class="title1 ml30" |
|
581 |
- >{{ selectedImage.unit }}을 마친 |
|
582 |
- <em class="yellow">{{ selectedImage.name }}</em |
|
583 |
- >친구</span |
|
584 |
- > |
|
310 |
+ <span class="title1 ml30">{{ selectedImage.unit }}을 마친 |
|
311 |
+ <em class="yellow">{{ selectedImage.name }}</em>친구</span> |
|
585 | 312 |
</div> |
586 | 313 |
<div class="title2 flex align-center" style="gap: 10px"> |
587 |
- <svg-icon |
|
588 |
- v-if="!isHeartFilled" |
|
589 |
- type="mdi" |
|
590 |
- :path="mdiHeartOutline" |
|
591 |
- @click=" |
|
592 |
- toggleHeart(selectedImage.heart, selectedImage.fileMngId) |
|
593 |
- " |
|
594 |
- style="color: #ffba08; cursor: pointer" |
|
595 |
- ></svg-icon> |
|
596 |
- <svg-icon |
|
597 |
- v-if="isHeartFilled" |
|
598 |
- type="mdi" |
|
599 |
- :path="mdiHeart" |
|
600 |
- @click=" |
|
601 |
- toggleHeart(selectedImage.heart, selectedImage.fileMngId) |
|
602 |
- " |
|
603 |
- style="color: #ffba08; cursor: pointer" |
|
604 |
- ></svg-icon> |
|
314 |
+ <svg-icon v-if="!isHeartFilled" type="mdi" :path="mdiHeartOutline" @click=" |
|
315 |
+ toggleHeart(selectedImage.heart, selectedImage.fileMngId) |
|
316 |
+ " style="color: #ffba08; cursor: pointer"></svg-icon> |
|
317 |
+ <svg-icon v-if="isHeartFilled" type="mdi" :path="mdiHeart" @click=" |
|
318 |
+ toggleHeart(selectedImage.heart, selectedImage.fileMngId) |
|
319 |
+ " style="color: #ffba08; cursor: pointer"></svg-icon> |
|
605 | 320 |
<p> |
606 | 321 |
<em class="yellow">{{ selectedImage.heart }}</em> |
607 | 322 |
</p> |
... | ... | @@ -660,7 +375,7 @@ |
660 | 375 |
schedules: [], |
661 | 376 |
nowSchedule: "", |
662 | 377 |
state: "", |
663 |
- rabbitPos: [1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], |
|
378 |
+ rabbitPos: [1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], |
|
664 | 379 |
rabbitCompl: [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], |
665 | 380 |
rabbitEnd: false, |
666 | 381 |
|
... | ... | @@ -930,6 +645,7 @@ |
930 | 645 |
this.titleUnitName = this.roadmapData[0].unit_nm; |
931 | 646 |
this.titleBookName = this.roadmapData[0].book_nm; |
932 | 647 |
this.labeledItems = this.processedRoadmap; |
648 |
+ console.log(this.roadmapData, this.labeledItems) |
|
933 | 649 |
} else { |
934 | 650 |
this.state = "noProblem"; |
935 | 651 |
} |
... | ... | @@ -961,12 +677,14 @@ |
961 | 677 |
this.goToPage(this.problemType); |
962 | 678 |
} else if (labeledItems.label.startsWith("단어장")) { |
963 | 679 |
this.handleWordBookContent(this.$store.getters.getLearningId); |
964 |
- } |
|
965 |
- |
|
966 |
- if (labeledItems.label.startsWith("지문")) { |
|
680 |
+ } else if (labeledItems.label.startsWith("지문")) { |
|
967 | 681 |
//console.log("지문 아이디 : ", labeledItems.learning_id); |
968 | 682 |
this.$store.dispatch("updateTextId", labeledItems.learning_id); |
969 | 683 |
this.fetchTextType(labeledItems.learning_id); |
684 |
+ } else if (labeledItems.label.startsWith("중간평가")) { |
|
685 |
+ this.fetchEvalData(labeledItems); |
|
686 |
+ } else if (labeledItems.label.startsWith("최종평가")) { |
|
687 |
+ this.fetchEvalData(labeledItems); |
|
970 | 688 |
} |
971 | 689 |
}, |
972 | 690 |
handleWordBookContent(item) { |
... | ... | @@ -1060,7 +778,39 @@ |
1060 | 778 |
console.log("지문 에러 : ", err); |
1061 | 779 |
}); |
1062 | 780 |
}, |
781 |
+ |
|
782 |
+ // 평가 정보를 통해서 관련 문제 가져오기 |
|
783 |
+ fetchEvalData(evaldata) { |
|
784 |
+ axios({ |
|
785 |
+ url: "/evalProblem/selectEvalProblem.json", |
|
786 |
+ method: "post", |
|
787 |
+ headers: { |
|
788 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
789 |
+ }, |
|
790 |
+ data: { |
|
791 |
+ evalId: evaldata.learning_id |
|
792 |
+ }, |
|
793 |
+ }) |
|
794 |
+ .then(response => { |
|
795 |
+ const payload = { |
|
796 |
+ learning_id: response.data, |
|
797 |
+ label: evaldata.label, |
|
798 |
+ seqNum: evaldata.seqNum |
|
799 |
+ }; |
|
800 |
+ |
|
801 |
+ // Vuex 뮤테이션 호출 |
|
802 |
+ this.$store.commit('setLearningData', payload); |
|
803 |
+ this.handleProblemDetail(response.data[0]); |
|
804 |
+ this.goToPage(this.problemType); |
|
805 |
+ }) |
|
806 |
+ .catch(error => { |
|
807 |
+ console.error("fetchData - error: ", error); |
|
808 |
+ return []; |
|
809 |
+ }); |
|
810 |
+ }, |
|
811 |
+ |
|
1063 | 812 |
handleProblemDetail(item) { |
813 |
+ |
|
1064 | 814 |
if (item.prblm_type_id === "prblm_type_001") { |
1065 | 815 |
this.problemType = "Chapter3"; |
1066 | 816 |
} else if (item.prblm_type_id === "prblm_type_002") { |
... | ... | @@ -1072,6 +822,7 @@ |
1072 | 822 |
} else if (item.prblm_type_id === "prblm_type_005") { |
1073 | 823 |
this.problemType = "Chapter3_3_1"; |
1074 | 824 |
} else if (item.prblm_type_id === "prblm_type_006") { |
825 |
+ console.log(item); |
|
1075 | 826 |
this.problemType = "Chapter3_4"; |
1076 | 827 |
} else if (item.prblm_type_id === "prblm_type_007") { |
1077 | 828 |
this.problemType = "Chapter3_5"; |
--- client/views/pages/teacher/ExamDetail.vue
+++ client/views/pages/teacher/ExamDetail.vue
... | ... | @@ -343,6 +343,7 @@ |
343 | 343 |
await this.updateSequence(); |
344 | 344 |
|
345 | 345 |
// 업데이트 후 평가 문제 목록 갱신 |
346 |
+ alert('문제 수정에 성공했습니다!'); |
|
346 | 347 |
this.fetchEvalQues(); |
347 | 348 |
} catch (error) { |
348 | 349 |
console.error('오류:', error); |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?