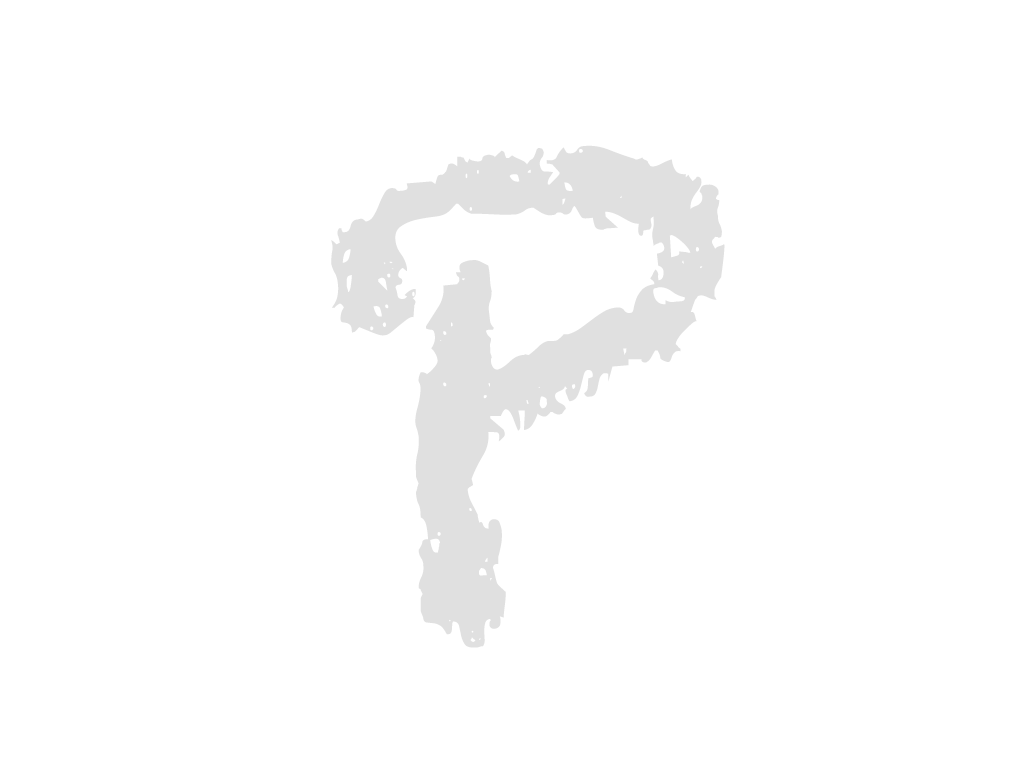
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="title-box flex justify-between mb40 myplan">
<p class="title">지문</p>
<select name="" id="" v-model="selectedBook" @change="fetchUnits">
<option value="" disabled selected>교재를 선택하세요</option>
<option v-for="book in books" :key="book.book_id" :value="book.book_id">
{{ book.book_nm }}
</option>
</select>
</div>
<div class="content-t">
<label for="" class="title2">단원</label>
<div class="unit-pagination flex mt10 mb20" style="gap: 10px;">
<button
v-for="(unit, index) in units"
:key="index"
:class="{ 'selected-btn': selectedUnit === unit.unitId }"
@click="selectUnit(unit.unitId)">
{{ unit.unitName }}
</button>
</div>
<div class="search-wrap flex justify-end mb20">
<select name="" id="" class="mr10 data-wrap" style="width: 15rem;" v-model="option">
<option value="" disabled>검색유형</option>
<option v-for="g in optionList" :key="g.value" :value="g.value">{{ g.text }}</option>
</select>
<input type="text" placeholder="검색하세요." v-model="keyword">
<button type="button" title="위원회 검색" @click="search">
<img src="../../../resources/img/look_t.png" alt="">
</button>
</div>
<div class="table-wrap">
<table>
<thead>
<tr>
<td>No.</td>
<td>제목</td>
<td>내용</td>
<td>작성자</td>
<td>등록일</td>
</tr>
</thead>
<tbody>
<tr v-for="(post, index) in posts" :key="index" class="post"
@click="goToPage('TextDetail', post.textId)">
<td>{{ index + 1 + (currentPage - 1) * pageSize }}</td>
<td>{{ post.textTtl.slice(0, 20) }}{{ post.textTtl.length > 20 ? '...' : '' }}</td>
<td>{{ post.textCnt.slice(0, 20) }}{{ post.textCnt.length > 20 ? '...' : '' }}</td>
<td>{{ post.userId }}</td>
<td>{{ post.regDt }}</td>
</tr>
</tbody>
</table>
<article class="table-pagination flex justify-center align-center mb20 mt30" style="gap: 10px;">
<button @click="changePage(currentPage - 1)" :disabled="currentPage === 1">
<img src="../../../resources/img/btn27_90t_normal.png" alt="Previous">
</button>
<button v-for="page in totalPages" :key="page" @click="changePage(page)"
:class="{ 'selected-btn': currentPage === page }">
{{ page }}
</button>
<button @click="changePage(currentPage + 1)" :disabled="currentPage === totalPages">
<img src="../../../resources/img/btn28_90t_normal.png" alt="Next">
</button>
</article>
<div class="flex justify-end">
<button type="button" title="등록" class="new-btn" @click="goToPage('TextInsert')">
등록
</button>
</div>
</div>
</div>
</template>
<script>
import axios from "axios";
import SvgIcon from '@jamescoyle/vue-icon';
import { mdiMagnify } from '@mdi/js';
export default {
data() {
return {
mdiMagnify: mdiMagnify,
posts: [],
currentPage: 1,
pageSize: 10,
totalPosts: 0,
option: "",
keyword: "",
books: [],
selectedBook: "",
optionList: [
{ text: "번호", value: "textId" },
{ text: "제목", value: "textTtl" },
{ text: "내용", value: "textCnt" },
{ text: "작성자", value: "userId" },
{ text: "등록일", value: "regDt" },
],
units: [],
selectedUnit: null,
searching: false,
};
},
methods: {
goToPage(page, textId) {
this.$router.push({ name: page, query: { textId, unit_id: this.selectedUnit, book_id: this.selectedBook } });
},
showConfirm(type) {
let message = '';
if (type === 'cancel') {
message = '삭제하시겠습니까?';
} else if (type === 'reset') {
message = '초기화하시겠습니까?';
} else if (type === 'save') {
message = '등록하시겠습니까?';
}
if (confirm(message)) {
this.goBack();
}
},
selectUnit(unitId) {
this.selectedUnit = unitId;
this.currentPage = 1;
this.fetchData();
},
search() {
if (!this.option) {
alert("검색유형을 선택해 주세요")
} else {
this.currentPage = 1;
this.searching = true;
this.fetchData();
}
},
fetchData() {
const idx = (this.currentPage - 1) * this.pageSize;
axios({
url: "/text/textSearch.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
"option": this.option,
"keyword": this.keyword,
"pageSize": this.pageSize,
"startIndex": idx,
"unitId": this.selectedUnit
},
})
.then(response => {
this.posts = response.data.list;
console.log(response.data.totalText);
if (!this.searching || this.keyword === "") {
this.totalPosts = response.data.totalText;
} else if (this.searching) {
this.totalPosts = response.data.resultCount;
}
this.posts.forEach(post => {
let regDt = post.regDt;
let date = new Date(regDt);
post.regDt = date.toISOString().split('T')[0];
});
})
.catch(error => {
console.error("fetchData - error: ", error);
alert("검색 중 오류가 발생했습니다.");
});
},
changePage(page) {
if (page > 0 && page <= this.totalPages) {
this.currentPage = page;
this.fetchData();
}
},
fetchBooks() {
axios({
url: "/book/findAll.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
})
.then(response => {
console.log(response.data)
this.books = response.data;
})
.catch(error => {
console.error("fetchBooks - error: ", error);
alert("교재 목록을 불러오는 중 오류가 발생했습니다.");
});
},
fetchUnits() {
if (!this.selectedBook) return;
axios({
url: "/unit/unitList.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
"bookId": this.selectedBook
},
})
.then(response => {
console.log(response.data)
this.units = response.data;
})
.catch(error => {
console.error("fetchUnits - error: ", error);
alert("단원 목록을 불러오는 중 오류가 발생했습니다.");
});
},
},
computed: {
totalPages() {
return Math.ceil(this.totalPosts / this.pageSize);
}
},
components: {
SvgIcon
},
mounted() {
this.selectedUnit = this.$route.query.unit_id || '';
this.selectedBook = this.$route.query.book_id || '';
this.fetchBooks(); // Fetch books when the component is mounted
this.fetchData(); // Fetch data for the default view
this.fetchUnits();
}
};
</script>