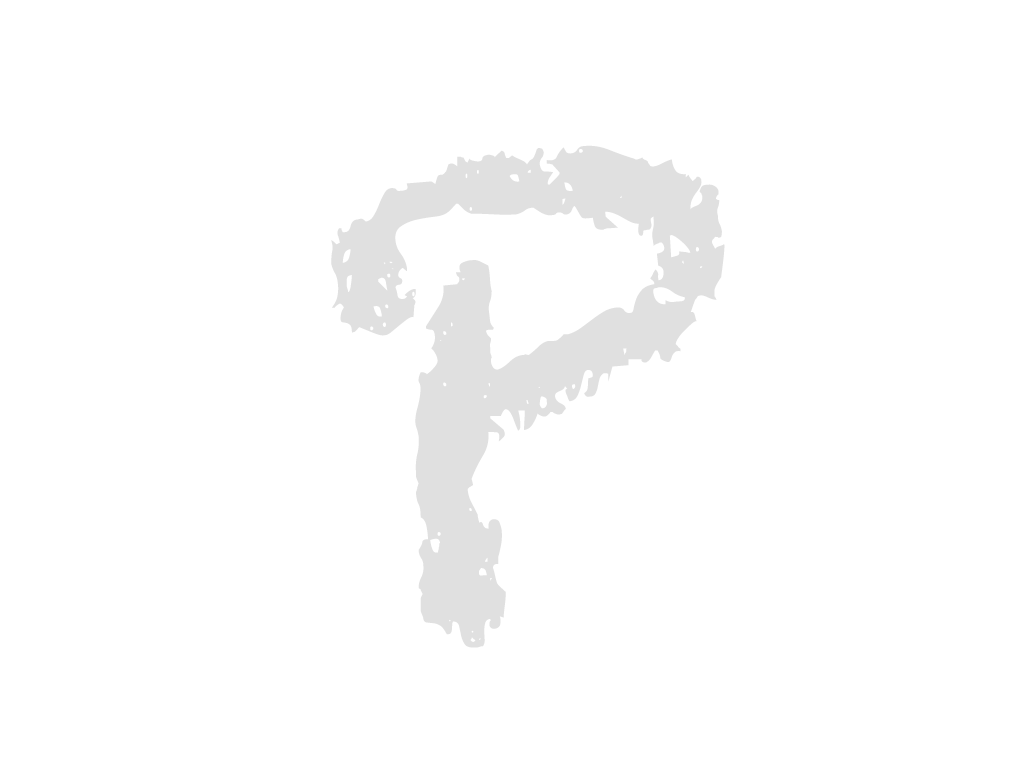
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="title-box flex justify-between mb40">
<p class="title">게시판</p>
<select name="" id="" v-model="selectedClass" @click="fetchStudents">
<option value="" disabled selected>반을 선택하세요</option>
<option v-for="sClass in classesList" :key="sClass.sclsId" :value="sClass.sclsId">
{{ sClass.sclsNm }}
</option>
</select>
</div>
<div class="flex justify-end mb20">
<div class="search-wrap ">
<select name="" id="" class="mr10 data-wrap">
<option value="">전체</option>
</select>
<input type="text" placeholder="검색하세요.">
<button type="button" title="위원회 검색">
<img src="../../../resources/img/look_t.png" alt="">
</button>
</div>
<button type="button" title="글쓰기" class="new-btn ml10" @click="buttonSearch">
학생 추가
</button>
</div>
<div class="table-wrap">
<table>
<thead>
<td>No.</td>
<td>학년</td>
<td>반</td>
<td>이름</td>
</thead>
<tbody>
<!-- StudentDetail로 갈때 stdId? userId? -->
<tr v-for="(student, index) in students" :key="index" class="student"
@click="goToPage('StudentDetail')">
<td>{{ index + 1 + (currentPage - 1) * pageSize }}</td>
<td>{{ student.grd_no }}</td>
<td>{{ student.scls_nm }}</td>
<td>{{ student.user_nm }}</td>
</tr>
</tbody>
</table>
<article class="table-pagination flex justify-center align-center mb20 mt30" style="gap: 10px;">
<button><img src="../../../resources/img/btn27_90t_normal.png" alt=""></button>
<button v-for="page in totalPages" :key="page" @click="changePage(page)"
:class="{ 'selected-btn': currentPage === page }">
{{ page }}
</button>
<button><img src="../../../resources/img/btn28_90t_normal.png" alt=""></button>
</article>
<div class="flex justify-end ">
</div>
</div>
<div v-show="searchOpen" class="popup-wrap">
<div class="popup-box ">
<div class="flex justify-between mb30">
<p class="popup-title">학생 검색</p>
<button type="button" class="popup-close-btn" @click="closeBtn">
<svg-icon type="mdi" :path="mdiWindowClose" class="close-btn"></svg-icon>
</button>
</div>
<div class="search-wrap mb30">
<input type="text" class="data-wrap" placeholder="">
<button type="button" >
<img src="../../../resources/img/look_t.png" alt="">
</button>
</div>
<div class="table-wrap">
<table>
<thead>
<td>No.</td>
<td>학년</td>
<td>반</td>
<td>번호</td>
<td>이름</td>
<td>선택</td>
</thead>
<tbody>
<tr>
<td></td>
<td></td>
<td></td>
<td></td>
<td></td>
</tr>
<td><input type="checkbox" class="ui-checkbox"></td>
</tbody>
</table>
<article class="table-pagination flex justify-center align-center mb20 mt30" style="gap: 10px;">
<button><img src="../../../resources/img/btn27_90t_normal.png" alt=""></button>
<button v-for="page in totalPages" :key="page" @click="changePage(page)"
:class="{ 'selected-btn': currentPage === page }">
{{ page }}
</button>
<button><img src="../../../resources/img/btn28_90t_normal.png" alt=""></button>
</article>
<div class="flex justify-end ">
</div>
</div>
<div class="flex justify-end ">
<button type="button" title="" class="new-btn mr10">
취소
</button>
<button type="button" title="" class="new-btn">
등록
</button>
</div>
</div>
</div>
</template>
<script>
import SvgIcon from '@jamescoyle/vue-icon';
import { mdiMagnify, mdiWindowClose } from '@mdi/js';
import axios from 'axios';
export default {
data() {
return {
classesList: [],
mdiMagnify: mdiMagnify,
mdiWindowClose: mdiWindowClose,
showModal: false,
searchOpen: false,
selectedClass: "",
students: [],
currentPage: 1,
pageSize: 10,
totalStudents: 0,
}
},
methods: {
selectClass(){
axios({
url: "/classes/selectClass.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
userId: "2"
},
})
.then((res) => {
if (res.data.status === "success") {
console.log("classesList - response(조회) : ", res.data.data);
this.classesList = res.data.data;
} else {
console.log("조회에 실패했습니다: ", res.data);
alert("조회에 실패했습니다.")
}
}).catch((err) => {
console.log("classesList - error(조회) : ", err);
alert("조회에 오류가 발생했습니다.");
});
},
fetchStudents() {
const idx = (this.currentPage - 1) * this.pageSize;
axios({
url: "/userclass/find.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
"pageSize": this.pageSize,
"startIndex": idx,
"sclsId": this.selectedClass
},
})
.then(response => {
console.log("<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<>>>>>>>>>>>>>>>>>>>>>>>>>>>>",response.data.students);
this.students = response.data.students;
this.totalStudents = response.data.totalStudent;
})
.catch(error => {
console.error("fetchStudents - error: ", error);
alert("조회 중 오류가 발생했습니다.");
});
},
changePage(page) {
if (page > 0 && page <= this.totalPages) {
this.currentPage = page;
this.fetchStudents();
}
},
goToPage(page) {
this.$router.push({ name: page });
},
showConfirm(type) {
let message = '';
if (type === 'cancel') {
message = '삭제하시겠습니까?';
} else if (type === 'reset') {
message = '초기화하시겠습니까?';
} else if (type === 'save') {
message = '등록하시겠습니까?';
}
if (confirm(message)) {
this.goBack();
}
},
closeModal() {
this.showModal = false;
},
buttonSearch() {
this.searchOpen = true;
},
closeBtn() {
this.searchOpen = false;
},
},
watch: {
},
computed: {
totalPages() {
console.log("totalPages@@@@@@@@@@@@@@@@@@@@@@@")
return Math.ceil(this.totalStudents / this.pageSize);
}
},
components: {
SvgIcon
},
mounted() {
console.log('Main2 mounted');
this.selectClass();
}
}
</script>