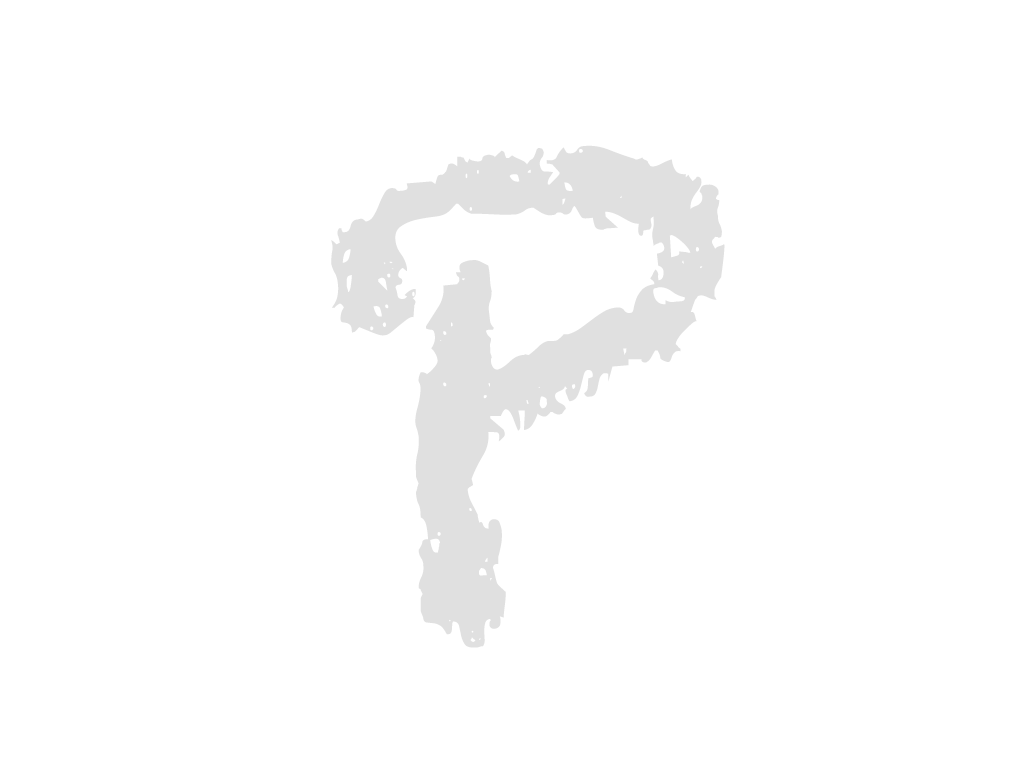
File name
Commit message
Commit date
2023-04-18
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React from "react";
import Table from "../../component/Table.jsx";
import Button from "../../component/Button.jsx";
import { useNavigate } from "react-router";
import AgencySearch from "../../component/AgencySearch.jsx";
import ContentTitle from "../../component/ContentTitle.jsx";
import Modal from "../../component/Modal.jsx";
import SubTitle from "../../component/SubTitle.jsx";
import RestoreFromTrashIcon from '@mui/icons-material/RestoreFromTrash';
import CorporateFareIcon from '@mui/icons-material/CorporateFare';
export default function SeniorSelect() {
const navigate = useNavigate();
const [modalOpen, setModalOpen] = React.useState(false);
const openModal = () => {
setModalOpen(true);
};
const closeModal = () => {
setModalOpen(false);
};
//게시판
const thead = [
"No",
"기관명",
"담당 부서 연락처(담당자)",
"관리 장비 개수",
"돌봄 대상자 인원",
"주소",
"사용자관리",
"기관통계보기",
"삭제"
];
thead.map(() => {
// console.log(elem);
// console.log(index);
console.log(thead[0]);
});
const key = [
"No",
"name",
"phone",
"equip",
"senior",
"address",
"worker",
"statistics",
"delete"
];
const content = [
{
No: (
<p><span><CorporateFareIcon sx={{fontSize:20 }} />{thead[0]}</span> 1</p>
),
name: (
<p><span>{thead[1]}</span> A복지관</p>
),
phone: (
<p><span>{thead[2]}</span> 053-811-1234(김대리)</p>
),
equip: (
<p><span>{thead[3]}</span> 80대</p>
),
senior: (
<p><span>{thead[4]}</span> 75명</p>
),
address: (
<p><span>{thead[5]}</span> 경상북도 군위군 삼국유사면</p>
),
worker: (
<p><span>{thead[6]}</span><Button
className={"btn-small gray-btn"}
btnName={"상세보기"}
onClick={() => {
navigate("/ProtectorSelect");
}}
/></p>
),
statistics: (
<Button
className={"btn-small gray-btn"}
btnName={"통계보기"}
onClick={() => {
navigate("/MedicineStatistics");
}}
/>
),
delete: (
<RestoreFromTrashIcon sx={{ width: "30px", height: "30px", }} />
),
},
];
//select option 동적 생성
// const optionList = () => {
// let year = [];
// let month = [];
// let days = [];
// let currentDate = new Date();
// let currentYear = currentDate.getFullYear();
// for (let i = 1910; i < currentYear.length; i++) {
// year.push(i);
// }
// for (let j = 1; i < 13; j++) {
// month.push(j);
// }
// for (let k = 1; i < 32; k++) {
// days.push(k);
// }
// return year, month, days;
// };
// React.useEffect(() => {
// optionList();
// }, []);
return (
<main>
<Modal open={modalOpen} close={closeModal} header="기관 등록">
<div className="board-wrap">
<table className="flex70 margin-bottom agency-insert">
<tbody className="">
<tr>
<th>기관명</th>
<td colSpan={5}>
<input type="text" name="" id="" />
</td>
</tr>
<tr>
<th>담당 부서 연락처</th>
<td>
<input type="text" name="" id="" />
</td>
<th>담당자</th>
<td>
<input type="text" name="" id="" />
</td>
</tr>
<tr>
<th>주소</th>
<td colSpan={5}>
<input type="text" name="" id="" />
</td>
</tr>
</tbody>
</table>
</div>
</Modal>
<div className="content-wrap">
<ContentTitle contentTitle={"기관 조회"} />
<div>
<AgencySearch />
<div className="board-wrap">
<div className="flex margin-bottom">
<div className="total flex">
<p className="total-equip">총 장비 개수 : 500대</p>
<p className="total-senior">전체 대상자(사용자)인원 : 500명</p>
</div>
<div className="btn-wrap flex-end margin-bottom ">
<Button
className={"btn-small gray-btn"}
btnName={"등록"}
onClick={openModal}
/>
</div>
</div >
<Table
className={"senior-table"}
head={thead}
contents={content}
contentKey={key}
/>
</div>
</div>
</div>
</main>
);
}