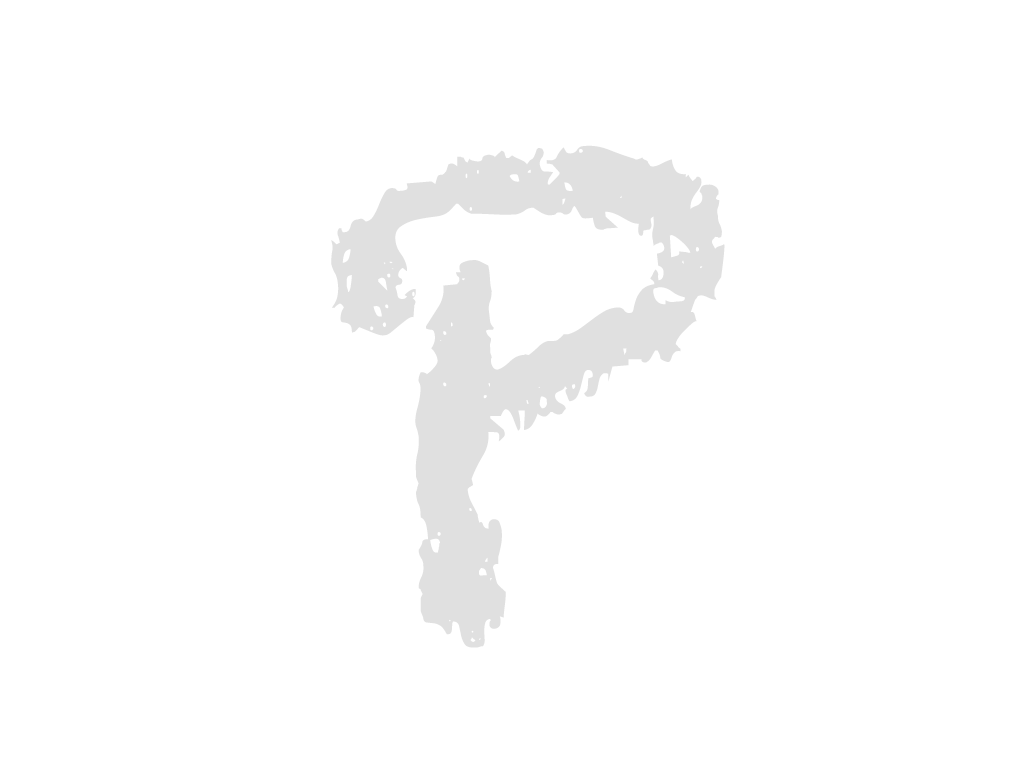
--- client/views/component/Modal_Guardian.jsx
+++ client/views/component/Modal_Guardian.jsx
... | ... | @@ -85,6 +85,80 @@ |
85 | 85 |
}); |
86 | 86 |
} |
87 | 87 |
|
88 |
+ // 연락처 정보 검색 |
|
89 |
+ const [isPhoneCheck, setIsPhoneCheck] = React.useState(false); |
|
90 |
+ // 연락처 정보 검색 결과 |
|
91 |
+ const [searchGuardian, setSearchGuardian] = React.useState({}); |
|
92 |
+ |
|
93 |
+ const insertGuardianValueChange = (targetKey, value) => { |
|
94 |
+ searchGuardian[targetKey] = value; |
|
95 |
+ setGuardian(searchGuardian); |
|
96 |
+ } |
|
97 |
+ |
|
98 |
+ const userPhoneCheck = () => { |
|
99 |
+ if (CommonUtil.isEmpty(searchGuardian['user_id']) == true) { |
|
100 |
+ guardianRef.current['gardian_phonenumber'].focus(); |
|
101 |
+ alert("연락처를 입력해 주세요."); |
|
102 |
+ return false; |
|
103 |
+ } |
|
104 |
+ |
|
105 |
+ fetch("/user/userSelectOne.json", { |
|
106 |
+ method: "POST", |
|
107 |
+ headers: { |
|
108 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
109 |
+ }, |
|
110 |
+ body: JSON.stringify(searchGuardian), |
|
111 |
+ }).then((response) => response.json()).then((data) => { |
|
112 |
+ console.log("정보 검색 결과 : ", data); |
|
113 |
+ if (CommonUtil.isEmpty(data) == true) { |
|
114 |
+ setIsPhoneCheck(false); |
|
115 |
+ guardianRef.current['gardian_phonenumber'].focus(); |
|
116 |
+ alert("존재하지 않는 보호자 연락처입니다."); |
|
117 |
+ } else { |
|
118 |
+ if (data['authority'] == 'ROLE_GUARDIAN') { |
|
119 |
+ setIsPhoneCheck(true); |
|
120 |
+ alert("보호자 정보 검색에 성공했습니다."); |
|
121 |
+ setSearchGuardian(data); |
|
122 |
+ } else { |
|
123 |
+ setIsPhoneCheck(false); |
|
124 |
+ guardianRef.current['gardian_phonenumber'].focus(); |
|
125 |
+ alert("보호자의 연락처가 아닙니다."); |
|
126 |
+ } |
|
127 |
+ } |
|
128 |
+ }).catch((error) => { |
|
129 |
+ console.log('userIdCheck() /user/userSelectOne.json error : ', error); |
|
130 |
+ }); |
|
131 |
+ } |
|
132 |
+ |
|
133 |
+ // 기존 보호자 등록 |
|
134 |
+ const guardianNewInsert = () => { |
|
135 |
+ searchGuardian['senior_id'] = seniorId; |
|
136 |
+ searchGuardian['guardian_id'] = searchGuardian['user_id']; |
|
137 |
+ |
|
138 |
+ if (CommonUtil.isEmpty(guardian['guardian_id']) && isPhoneCheck == false) { |
|
139 |
+ alert("연락처 검색을 해주세요."); |
|
140 |
+ return false; |
|
141 |
+ } |
|
142 |
+ |
|
143 |
+ fetch("/user/seniorGuardianInsert.json", { |
|
144 |
+ method: "POST", |
|
145 |
+ headers: { |
|
146 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
147 |
+ }, |
|
148 |
+ body: JSON.stringify(searchGuardian), |
|
149 |
+ }).then((response) => response.json()).then((data) => { |
|
150 |
+ console.log("시니어 등록 결과(건수) : ", data); |
|
151 |
+ if (data > 0) { |
|
152 |
+ alert("등록완료"); |
|
153 |
+ guardianManagementCallback(); |
|
154 |
+ } else { |
|
155 |
+ alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); |
|
156 |
+ } |
|
157 |
+ }).catch((error) => { |
|
158 |
+ console.log('guardianNewInsert() /user/seniorGuardianInsert.json error : ', error); |
|
159 |
+ }); |
|
160 |
+ } |
|
161 |
+ |
|
88 | 162 |
//보호자 등록 유효성 검사 |
89 | 163 |
const guardianInsertValidation = () => { |
90 | 164 |
if (CommonUtil.isEmpty(guardian['user_name']) == true) { |
... | ... | @@ -129,12 +203,12 @@ |
129 | 203 |
|
130 | 204 |
return true; |
131 | 205 |
} |
206 |
+ |
|
132 | 207 |
//보호자 등록 |
133 | 208 |
const guardianInsert = () => { |
134 | 209 |
if (guardianInsertValidation() == false) { |
135 | 210 |
return; |
136 | 211 |
} |
137 |
- |
|
138 | 212 |
fetch("/user/guardianInsert.json", { |
139 | 213 |
method: "POST", |
140 | 214 |
headers: { |
... | ... | @@ -184,10 +258,6 @@ |
184 | 258 |
if (confirm("보호자를 삭제하시겠습니까?") == false) { |
185 | 259 |
return; |
186 | 260 |
} |
187 |
- if (guardianInsertValidation() == false) { |
|
188 |
- return; |
|
189 |
- } |
|
190 |
- |
|
191 | 261 |
fetch("/user/guardianDelete.json", { |
192 | 262 |
method: "POST", |
193 | 263 |
headers: { |
... | ... | @@ -203,11 +273,9 @@ |
203 | 273 |
alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다."); |
204 | 274 |
} |
205 | 275 |
}).catch((error) => { |
206 |
- console.log('guardianInsert() /user/guardianInsert.json error : ', error); |
|
276 |
+ console.log('guardianDelete() /user/guardianDelete.json error : ', error); |
|
207 | 277 |
}); |
208 | 278 |
} |
209 |
- |
|
210 |
- |
|
211 | 279 |
|
212 | 280 |
//주소 검색 결과 객체 |
213 | 281 |
const [jusoList, setJusoList] = React.useState({ |
... | ... | @@ -320,169 +388,240 @@ |
320 | 388 |
} |
321 | 389 |
|
322 | 390 |
{CommonUtil.isEmpty(guardian['guardian_id']) && !isNewGuardian ? ( |
323 |
- <table className="margin-bottom2 senior-insert"> |
|
324 |
- <tr> |
|
325 |
- <th><span style={{ color: "red" }}>*</span>연락처 검색</th> |
|
326 |
- <td colSpan={3}> |
|
327 |
- <input type="number" maxLength="11" style={{ width: 'calc(100% - 160px)' }} |
|
328 |
- value={guardian['user_phonenumber']} |
|
329 |
- onChange={(e) => { guardianValueChange('user_phonenumber', e.target.value); setIsIdCheck(false); }} |
|
330 |
- ref={el => guardianRef.current['user_phonenumber'] = el} |
|
331 |
- /> |
|
332 |
- <button className={"red-btn btn-large"} onClick={userIdCheck}> |
|
333 |
- 정보검색 |
|
334 |
- </button> |
|
335 |
- </td> |
|
336 |
- </tr> |
|
337 |
- </table> |
|
338 |
- ) : null} |
|
391 |
+ <> |
|
392 |
+ <table className="margin-bottom2 senior-insert"> |
|
393 |
+ <tr> |
|
394 |
+ <th><span style={{ color: "red" }}>*</span>연락처 검색</th> |
|
395 |
+ <td colSpan={3}> |
|
396 |
+ <input type="number" maxLength="11" style={{ width: 'calc(100% - 160px)' }} |
|
397 |
+ value={searchGuardian['user_phonenumber']} |
|
398 |
+ onChange={(e) => { insertGuardianValueChange('user_id', e.target.value); setIsPhoneCheck(false); }} |
|
399 |
+ ref={el => guardianRef.current['gardian_phonenumber'] = el} |
|
400 |
+ /> |
|
401 |
+ <button className={"red-btn btn-large"} onClick={userPhoneCheck}> |
|
402 |
+ 정보검색 |
|
403 |
+ </button> |
|
404 |
+ </td> |
|
405 |
+ </tr> |
|
406 |
+ </table> |
|
339 | 407 |
|
340 |
- <table className="margin-bottom2 senior-insert"> |
|
341 |
- <tr> |
|
342 |
- <th><span style={{ color: "red" }}>*</span>이름</th> |
|
343 |
- <td> |
|
344 |
- <input type="text" |
|
345 |
- value={guardian['user_name']} |
|
346 |
- onChange={(e) => { guardianValueChange('user_name', e.target.value) }} |
|
347 |
- ref={el => guardianRef.current['user_name'] = el} |
|
348 |
- /> |
|
349 |
- </td> |
|
350 |
- <th><span style={{ color: "red" }}>*</span>성별</th> |
|
351 |
- <td className=" gender"> |
|
352 |
- <div className="flex-start"> |
|
353 |
- <input type="radio" id="user_gender_m" name="user_gender" value="남" |
|
354 |
- onChange={(e) => { e.target.checked ? guardianValueChange('user_gender', e.target.value) : null }} |
|
355 |
- ref={el => guardianRef.current['user_gender']['m'] = el} |
|
356 |
- /> |
|
357 |
- <label for="user_gender_m">남</label> |
|
358 |
- </div> |
|
359 |
- <div className="flex-start"> |
|
360 |
- <input type="radio" id="user_gender_f" name="user_gender" value="여" |
|
361 |
- onChange={(e) => { e.target.checked ? guardianValueChange('user_gender', e.target.value) : null }} |
|
362 |
- ref={el => guardianRef.current['user_gender']['f'] = el} |
|
363 |
- /> |
|
364 |
- <label for="user_gender_f">여</label> |
|
365 |
- </div> |
|
366 |
- </td> |
|
367 |
- </tr> |
|
368 |
- <tr> |
|
369 |
- <th><span style={{ color: "red" }}>*</span>생년월일</th> |
|
370 |
- <td> |
|
371 |
- <div className="flex"> |
|
372 |
- <input type='date' |
|
373 |
- value={guardian['user_birth']} |
|
374 |
- onChange={(e) => { guardianValueChange('user_birth', e.target.value) }} |
|
375 |
- ref={el => guardianRef.current['user_birth'] = el} |
|
376 |
- /> |
|
377 |
- </div> |
|
378 |
- </td> |
|
379 |
- </tr> |
|
380 |
- {isNewGuardian ? ( |
|
381 |
- <tr> |
|
382 |
- <th><span style={{ color: "red" }}>*</span>연락처</th> |
|
383 |
- <td colSpan={3}> |
|
384 |
- {CommonUtil.isEmpty(guardian['guardian_id']) ? |
|
385 |
- <> |
|
386 |
- <input type="number" maxLength="11" style={{ width: 'calc(100% - 160px)' }} |
|
387 |
- value={guardian['user_phonenumber']} |
|
388 |
- onChange={(e) => { guardianValueChange('user_phonenumber', e.target.value); setIsIdCheck(false); }} |
|
389 |
- ref={el => guardianRef.current['user_phonenumber'] = el} |
|
408 |
+ <table className="margin-bottom2 senior-insert"> |
|
409 |
+ <tr> |
|
410 |
+ <th><span style={{ color: "red" }}>*</span>이름</th> |
|
411 |
+ <td> |
|
412 |
+ <input type="text" |
|
413 |
+ value={searchGuardian['user_name']} |
|
414 |
+ disabled |
|
415 |
+ /> |
|
416 |
+ </td> |
|
417 |
+ <th><span style={{ color: "red" }}>*</span>성별</th> |
|
418 |
+ <td className=" gender"> |
|
419 |
+ <div className="flex-start"> |
|
420 |
+ <input type="radio" id="user_gender_m" name="user_gender" value="남" disabled checked={searchGuardian['user_gender'] == "남"} /> |
|
421 |
+ <label for="user_gender_m">남</label> |
|
422 |
+ </div> |
|
423 |
+ <div className="flex-start"> |
|
424 |
+ <input type="radio" id="user_gender_f" name="user_gender" value="여" disabled checked={searchGuardian['user_gender'] == "여"} /> |
|
425 |
+ <label for="user_gender_f">여</label> |
|
426 |
+ </div> |
|
427 |
+ </td> |
|
428 |
+ </tr> |
|
429 |
+ <tr> |
|
430 |
+ <th><span style={{ color: "red" }}>*</span>생년월일</th> |
|
431 |
+ <td> |
|
432 |
+ <div className="flex"> |
|
433 |
+ <input type='date' |
|
434 |
+ value={searchGuardian['user_birth']} |
|
435 |
+ disabled |
|
390 | 436 |
/> |
391 |
- <button className={"red-btn btn-large"} onClick={userIdCheck}> |
|
392 |
- 중복확인 |
|
393 |
- </button> |
|
394 |
- </> |
|
395 |
- : <input type="number" maxLength="11" disabled |
|
396 |
- value={guardian['user_phonenumber']} |
|
397 |
- />} |
|
398 |
- </td> |
|
399 |
- </tr> |
|
400 |
- ) : null} |
|
401 |
- <tr> |
|
402 |
- <th><span style={{ color: "red" }}>*</span>주소</th> |
|
403 |
- <td colSpan={3}> |
|
404 |
- <div> |
|
405 |
- <input type="text" style={{ width: 'calc(100% - 160px)' }} |
|
406 |
- value={guardian['user_address']} disabled={CommonUtil.isEmpty(guardian['zip_no']) == false} |
|
407 |
- onChange={(e) => { guardianValueChange('user_address', e.target.value) }} |
|
408 |
- onKeyUp={(e) => { e.key == 'Enter' ? jusoSearch() : null }} |
|
409 |
- ref={el => guardianRef.current['user_address'] = el} |
|
410 |
- /> |
|
411 |
- {CommonUtil.isEmpty(guardian['zip_no']) |
|
412 |
- ? <button className={"red-btn btn-large"} onClick={() => { jusoSearch() }}>주소검색</button> |
|
413 |
- : <button className={"gray-btn btn-large"} onClick={() => { guardianValueChange('zip_no', null) }}>다시검색</button> |
|
414 |
- } |
|
415 |
- </div> |
|
416 |
- {CommonUtil.isEmpty(jusoList.juso) == false && CommonUtil.isEmpty(guardian['zip_no']) ? |
|
417 |
- <div> |
|
418 |
- <ul className="list-box" style={{ width: '100%' }}> |
|
419 |
- {CommonUtil.isEmpty(jusoList.juso) == false ? jusoList.juso.map((item, idx) => { |
|
437 |
+ </div> |
|
438 |
+ </td> |
|
439 |
+ </tr> |
|
440 |
+ <tr> |
|
441 |
+ <th><span style={{ color: "red" }}>*</span>주소</th> |
|
442 |
+ <td colSpan={3}> |
|
443 |
+ <input type="text" |
|
444 |
+ value={searchGuardian['user_address']} |
|
445 |
+ disabled |
|
446 |
+ /> |
|
447 |
+ </td> |
|
448 |
+ </tr> |
|
449 |
+ |
|
450 |
+ <tr> |
|
451 |
+ <th><span style={{ color: "red" }}>*</span>대상자와의 관계</th> |
|
452 |
+ <td colSpan={3}> |
|
453 |
+ <select onChange={(e) => { insertGuardianValueChange('senior_relationship', e.target.value) }} |
|
454 |
+ ref={el => guardianRef.current['senior_relationship'] = el}> |
|
455 |
+ {relationshipList.map((relationship, idx) => { |
|
420 | 456 |
return ( |
421 |
- <li className={guardian['zip_no'] == item['zipNo'] ? 'active' : null} onClick={() => { |
|
422 |
- guardian['zip_no'] = item['zipNo']; guardian['adm_cd'] = item['admCd']; |
|
423 |
- guardian['rn_mgt_sn'] = item['rnMgtSn']; guardian['bd_mgt_sn'] = item['bdMgtSn']; |
|
424 |
- guardian['siNsi_nmm'] = item['siNm']; guardian['sgg_nm'] = item['sggNm']; |
|
425 |
- guardian['emd_nm'] = item['emdNm']; guardian['li_nm'] = item['liNm']; |
|
426 |
- guardian['rn'] = item['rn']; guardian['emd_no'] = item['emdNo']; |
|
427 |
- guardian['hemd_nm'] = item['hemdNm']; guardian['road_addr'] = item['roadAddr']; |
|
428 |
- guardian['buld_mnnm'] = item['buldMnnm']; guardian['buld_slno'] = item['buldSlno']; |
|
429 |
- guardian['user_address'] = item['roadAddr']; |
|
430 |
- |
|
431 |
- if (CommonUtil.isEmpty(item['udrtYn'])) { |
|
432 |
- guardian['udrt_yn'] = null; |
|
433 |
- } else { |
|
434 |
- if (item['udrtYn'] == 1 || item['udrtYn'] == true || item['udrtYn'] == '1' || item['udrtYn'].toLowerCase() == 'true') { |
|
435 |
- guardian['udrt_yn'] = true; |
|
436 |
- } else { |
|
437 |
- guardian['udrt_yn'] = false; |
|
438 |
- } |
|
439 |
- } |
|
440 |
- |
|
441 |
- setGuardian({ ...guardian }); coordSearch(); |
|
442 |
- }}> |
|
443 |
- <span style={{ fontWeight: 600 }}>[지번]</span> {item['jibunAddr']} |
|
444 |
- <br /> |
|
445 |
- <span style={{ fontWeight: 600 }}>[도로명]</span> {item['roadAddr']} |
|
446 |
- </li> |
|
457 |
+ <option key={idx}>{relationship}</option> |
|
447 | 458 |
) |
448 |
- }) : null} |
|
449 |
- </ul> |
|
450 |
- </div> |
|
451 |
- : null} |
|
452 |
- </td> |
|
453 |
- </tr> |
|
459 |
+ })} |
|
460 |
+ <option value="" selected={relationshipList.indexOf(guardian['senior_relationship']) == -1}>기타</option> |
|
461 |
+ </select> |
|
462 |
+ {relationshipList.indexOf(guardian['senior_relationship']) == -1 ? ( |
|
463 |
+ <input type="text" |
|
464 |
+ value={guardian['senior_relationship']} |
|
465 |
+ onChange={(e) => { insertGuardianValueChange('senior_relationship', e.target.value) }} |
|
466 |
+ ref={el => guardianRef.current['senior_relationship_etc'] = el} |
|
467 |
+ /> |
|
468 |
+ ) : null} |
|
469 |
+ </td> |
|
470 |
+ </tr> |
|
471 |
+ </table> |
|
454 | 472 |
|
455 |
- <tr> |
|
456 |
- <th><span style={{ color: "red" }}>*</span>대상자와의 관계</th> |
|
457 |
- <td colSpan={3}> |
|
458 |
- <select onChange={(e) => { guardianValueChange('senior_relationship', e.target.value) }} |
|
459 |
- ref={el => guardianRef.current['senior_relationship'] = el}> |
|
460 |
- {relationshipList.map((relationship, idx) => { |
|
461 |
- return ( |
|
462 |
- <option key={idx}>{relationship}</option> |
|
463 |
- ) |
|
464 |
- })} |
|
465 |
- <option value="" selected={relationshipList.indexOf(guardian['senior_relationship']) == -1}>기타</option> |
|
466 |
- </select> |
|
467 |
- {relationshipList.indexOf(guardian['senior_relationship']) == -1 ? ( |
|
468 |
- <input type="text" |
|
469 |
- value={guardian['senior_relationship']} |
|
470 |
- onChange={(e) => { guardianValueChange('senior_relationship', e.target.value) }} |
|
471 |
- ref={el => guardianRef.current['senior_relationship_etc'] = el} |
|
472 |
- /> |
|
473 |
- ) : null} |
|
474 |
- </td> |
|
475 |
- </tr> |
|
476 |
- </table> |
|
473 |
+ <div className="btn-wrap flex-center margin-bottom5"> |
|
474 |
+ <button className={"btn-small gray-btn"} onClick={guardianNewInsert}>기존추가</button> |
|
475 |
+ </div> |
|
476 |
+ </> |
|
477 |
+ ) : ( |
|
478 |
+ <> |
|
479 |
+ <table className="margin-bottom2 senior-insert"> |
|
480 |
+ <tr> |
|
481 |
+ <th><span style={{ color: "red" }}>*</span>이름</th> |
|
482 |
+ <td> |
|
483 |
+ <input type="text" |
|
484 |
+ value={guardian['user_name']} |
|
485 |
+ onChange={(e) => { guardianValueChange('user_name', e.target.value) }} |
|
486 |
+ ref={el => guardianRef.current['user_name'] = el} |
|
487 |
+ /> |
|
488 |
+ </td> |
|
489 |
+ <th><span style={{ color: "red" }}>*</span>성별</th> |
|
490 |
+ <td className=" gender"> |
|
491 |
+ <div className="flex-start"> |
|
492 |
+ <input type="radio" id="user_gender_m" name="user_gender" value="남" |
|
493 |
+ onChange={(e) => { e.target.checked ? guardianValueChange('user_gender', e.target.value) : null }} |
|
494 |
+ ref={el => guardianRef.current['user_gender']['m'] = el} |
|
495 |
+ /> |
|
496 |
+ <label for="user_gender_m">남</label> |
|
497 |
+ </div> |
|
498 |
+ <div className="flex-start"> |
|
499 |
+ <input type="radio" id="user_gender_f" name="user_gender" value="여" |
|
500 |
+ onChange={(e) => { e.target.checked ? guardianValueChange('user_gender', e.target.value) : null }} |
|
501 |
+ ref={el => guardianRef.current['user_gender']['f'] = el} |
|
502 |
+ /> |
|
503 |
+ <label for="user_gender_f">여</label> |
|
504 |
+ </div> |
|
505 |
+ </td> |
|
506 |
+ </tr> |
|
507 |
+ <tr> |
|
508 |
+ <th><span style={{ color: "red" }}>*</span>생년월일</th> |
|
509 |
+ <td> |
|
510 |
+ <div className="flex"> |
|
511 |
+ <input type='date' |
|
512 |
+ value={guardian['user_birth']} |
|
513 |
+ onChange={(e) => { guardianValueChange('user_birth', e.target.value) }} |
|
514 |
+ ref={el => guardianRef.current['user_birth'] = el} |
|
515 |
+ /> |
|
516 |
+ </div> |
|
517 |
+ </td> |
|
518 |
+ </tr> |
|
519 |
+ <tr> |
|
520 |
+ <th><span style={{ color: "red" }}>*</span>연락처</th> |
|
521 |
+ <td colSpan={3}> |
|
522 |
+ {CommonUtil.isEmpty(guardian['guardian_id']) ? |
|
523 |
+ <> |
|
524 |
+ <input type="number" maxLength="11" style={{ width: 'calc(100% - 160px)' }} |
|
525 |
+ value={guardian['user_phonenumber']} |
|
526 |
+ onChange={(e) => { guardianValueChange('user_phonenumber', e.target.value); setIsIdCheck(false); }} |
|
527 |
+ ref={el => guardianRef.current['user_phonenumber'] = el} |
|
528 |
+ /> |
|
529 |
+ <button className={"red-btn btn-large"} onClick={userIdCheck}> |
|
530 |
+ 중복확인 |
|
531 |
+ </button> |
|
532 |
+ </> |
|
533 |
+ : <input type="number" maxLength="11" disabled |
|
534 |
+ value={guardian['user_phonenumber']} |
|
535 |
+ />} |
|
536 |
+ </td> |
|
537 |
+ </tr> |
|
538 |
+ <tr> |
|
539 |
+ <th><span style={{ color: "red" }}>*</span>주소</th> |
|
540 |
+ <td colSpan={3}> |
|
541 |
+ <div> |
|
542 |
+ <input type="text" style={{ width: 'calc(100% - 160px)' }} |
|
543 |
+ value={guardian['user_address']} disabled={CommonUtil.isEmpty(guardian['zip_no']) == false} |
|
544 |
+ onChange={(e) => { guardianValueChange('user_address', e.target.value) }} |
|
545 |
+ onKeyUp={(e) => { e.key == 'Enter' ? jusoSearch() : null }} |
|
546 |
+ ref={el => guardianRef.current['user_address'] = el} |
|
547 |
+ /> |
|
548 |
+ {CommonUtil.isEmpty(guardian['zip_no']) |
|
549 |
+ ? <button className={"red-btn btn-large"} onClick={() => { jusoSearch() }}>주소검색</button> |
|
550 |
+ : <button className={"gray-btn btn-large"} onClick={() => { guardianValueChange('zip_no', null) }}>다시검색</button> |
|
551 |
+ } |
|
552 |
+ </div> |
|
553 |
+ {CommonUtil.isEmpty(jusoList.juso) == false && CommonUtil.isEmpty(guardian['zip_no']) ? |
|
554 |
+ <div> |
|
555 |
+ <ul className="list-box" style={{ width: '100%' }}> |
|
556 |
+ {CommonUtil.isEmpty(jusoList.juso) == false ? jusoList.juso.map((item, idx) => { |
|
557 |
+ return ( |
|
558 |
+ <li className={guardian['zip_no'] == item['zipNo'] ? 'active' : null} onClick={() => { |
|
559 |
+ guardian['zip_no'] = item['zipNo']; guardian['adm_cd'] = item['admCd']; |
|
560 |
+ guardian['rn_mgt_sn'] = item['rnMgtSn']; guardian['bd_mgt_sn'] = item['bdMgtSn']; |
|
561 |
+ guardian['siNsi_nmm'] = item['siNm']; guardian['sgg_nm'] = item['sggNm']; |
|
562 |
+ guardian['emd_nm'] = item['emdNm']; guardian['li_nm'] = item['liNm']; |
|
563 |
+ guardian['rn'] = item['rn']; guardian['emd_no'] = item['emdNo']; |
|
564 |
+ guardian['hemd_nm'] = item['hemdNm']; guardian['road_addr'] = item['roadAddr']; |
|
565 |
+ guardian['buld_mnnm'] = item['buldMnnm']; guardian['buld_slno'] = item['buldSlno']; |
|
566 |
+ guardian['user_address'] = item['roadAddr']; |
|
477 | 567 |
|
478 |
- <div className="btn-wrap flex-center margin-bottom5"> |
|
479 |
- {CommonUtil.isEmpty(guardian['guardian_id']) |
|
480 |
- ? <button className={"btn-small gray-btn"} onClick={guardianInsert}>추가</button> |
|
481 |
- : <> |
|
482 |
- <button className={"btn-small gray-btn"} onClick={guardianUpdate}>수정</button> |
|
483 |
- <button className={"btn-small red-btn"} onClick={guardianDelete}>삭제</button> |
|
484 |
- </>} |
|
485 |
- </div> |
|
568 |
+ if (CommonUtil.isEmpty(item['udrtYn'])) { |
|
569 |
+ guardian['udrt_yn'] = null; |
|
570 |
+ } else { |
|
571 |
+ if (item['udrtYn'] == 1 || item['udrtYn'] == true || item['udrtYn'] == '1' || item['udrtYn'].toLowerCase() == 'true') { |
|
572 |
+ guardian['udrt_yn'] = true; |
|
573 |
+ } else { |
|
574 |
+ guardian['udrt_yn'] = false; |
|
575 |
+ } |
|
576 |
+ } |
|
577 |
+ |
|
578 |
+ setGuardian({ ...guardian }); coordSearch(); |
|
579 |
+ }}> |
|
580 |
+ <span style={{ fontWeight: 600 }}>[지번]</span> {item['jibunAddr']} |
|
581 |
+ <br /> |
|
582 |
+ <span style={{ fontWeight: 600 }}>[도로명]</span> {item['roadAddr']} |
|
583 |
+ </li> |
|
584 |
+ ) |
|
585 |
+ }) : null} |
|
586 |
+ </ul> |
|
587 |
+ </div> |
|
588 |
+ : null} |
|
589 |
+ </td> |
|
590 |
+ </tr> |
|
591 |
+ |
|
592 |
+ <tr> |
|
593 |
+ <th><span style={{ color: "red" }}>*</span>대상자와의 관계</th> |
|
594 |
+ <td colSpan={3}> |
|
595 |
+ <select onChange={(e) => { guardianValueChange('senior_relationship', e.target.value) }} |
|
596 |
+ ref={el => guardianRef.current['senior_relationship'] = el}> |
|
597 |
+ {relationshipList.map((relationship, idx) => { |
|
598 |
+ return ( |
|
599 |
+ <option key={idx}>{relationship}</option> |
|
600 |
+ ) |
|
601 |
+ })} |
|
602 |
+ <option value="" selected={relationshipList.indexOf(guardian['senior_relationship']) == -1}>기타</option> |
|
603 |
+ </select> |
|
604 |
+ {relationshipList.indexOf(guardian['senior_relationship']) == -1 ? ( |
|
605 |
+ <input type="text" |
|
606 |
+ value={guardian['senior_relationship']} |
|
607 |
+ onChange={(e) => { guardianValueChange('senior_relationship', e.target.value) }} |
|
608 |
+ ref={el => guardianRef.current['senior_relationship_etc'] = el} |
|
609 |
+ /> |
|
610 |
+ ) : null} |
|
611 |
+ </td> |
|
612 |
+ </tr> |
|
613 |
+ </table> |
|
614 |
+ |
|
615 |
+ <div className="btn-wrap flex-center margin-bottom5"> |
|
616 |
+ {CommonUtil.isEmpty(guardian['guardian_id']) |
|
617 |
+ ? <button className={"btn-small gray-btn"} onClick={guardianInsert}>추가</button> |
|
618 |
+ : <> |
|
619 |
+ <button className={"btn-small gray-btn"} onClick={guardianUpdate}>수정</button> |
|
620 |
+ <button className={"btn-small red-btn"} onClick={guardianDelete}>삭제</button> |
|
621 |
+ </>} |
|
622 |
+ </div> |
|
623 |
+ </> |
|
624 |
+ )} |
|
486 | 625 |
</div> |
487 | 626 |
</div> |
488 | 627 |
) : null} |
--- client/views/pages/healthcare/Healthcare.jsx
+++ client/views/pages/healthcare/Healthcare.jsx
... | ... | @@ -185,6 +185,36 @@ |
185 | 185 |
}); |
186 | 186 |
}; |
187 | 187 |
|
188 |
+ const Temperature = ({ data }) => { |
|
189 |
+ let seniorTemperature = []; |
|
190 |
+ if (data['seniorTemperatureList'] && data['seniorTemperatureList'].length > 0) { |
|
191 |
+ data['seniorTemperatureList'].map((data, dataIdx) => { |
|
192 |
+ seniorTemperature.push(data['temperature']); |
|
193 |
+ }) |
|
194 |
+ if (data['seniorTemperatureList'].length < 12) { |
|
195 |
+ for (let i = 0; i < 12 - data['seniorTemperatureList'].length; i++) { |
|
196 |
+ seniorTemperature.push('-'); |
|
197 |
+ } |
|
198 |
+ } |
|
199 |
+ } |
|
200 |
+ |
|
201 |
+ console.log(">>", seniorTemperature) |
|
202 |
+ |
|
203 |
+ if (seniorTemperature.length > 0) { |
|
204 |
+ return ( |
|
205 |
+ seniorTemperature.map((item, idx) => { |
|
206 |
+ return ( |
|
207 |
+ <td>{item}</td> |
|
208 |
+ ) |
|
209 |
+ }) |
|
210 |
+ ) |
|
211 |
+ } else { |
|
212 |
+ return ( |
|
213 |
+ <td colSpan={12}>집계된 정보가 없습니다.</td> |
|
214 |
+ ) |
|
215 |
+ } |
|
216 |
+ } |
|
217 |
+ |
|
188 | 218 |
React.useEffect(() => { |
189 | 219 |
searching(); |
190 | 220 |
}, []); |
... | ... | @@ -203,13 +233,18 @@ |
203 | 233 |
<table className={"protector-user"}> |
204 | 234 |
<thead> |
205 | 235 |
<tr> |
206 |
- <th>No</th> |
|
207 |
- <th>소속기관명</th> |
|
208 |
- <th>이름</th> |
|
209 |
- <th>생년월일</th> |
|
210 |
- <th>성별</th> |
|
211 |
- <th>연락처</th> |
|
212 |
- <th colSpan={3}>최근복약률</th> |
|
236 |
+ <th rowSpan={2}>No</th> |
|
237 |
+ <th rowSpan={2}>소속기관명</th> |
|
238 |
+ <th rowSpan={2}>이름</th> |
|
239 |
+ <th rowSpan={2}>생년월일</th> |
|
240 |
+ <th rowSpan={2}>성별</th> |
|
241 |
+ <th rowSpan={2}>연락처</th> |
|
242 |
+ <th colSpan={3}>최근복약률(집계/전체)</th> |
|
243 |
+ </tr> |
|
244 |
+ <tr> |
|
245 |
+ <th>최근 하루 전</th> |
|
246 |
+ <th>최근 이틀 전</th> |
|
247 |
+ <th>최근 사흘 전</th> |
|
213 | 248 |
</tr> |
214 | 249 |
</thead> |
215 | 250 |
<tbody> |
... | ... | @@ -238,7 +273,7 @@ |
238 | 273 |
</td> |
239 | 274 |
) |
240 | 275 |
}) |
241 |
- : null} |
|
276 |
+ : <td colSpan={3}>집계된 정보가 없습니다.</td>} |
|
242 | 277 |
</tr> |
243 | 278 |
) |
244 | 279 |
})} |
... | ... | @@ -299,17 +334,7 @@ |
299 | 334 |
<td data-label="생년월일">{item['user_birth']}</td> |
300 | 335 |
<td data-label="성별">{item['user_gender']}</td> |
301 | 336 |
<td data-label="연락처">{item['user_phonenumber']}</td> |
302 |
- { |
|
303 |
- item['seniorTemperatureList'] && item['seniorTemperatureList'].length > 0 ? |
|
304 |
- item['seniorTemperatureList'].map((data, dataIdx) => { |
|
305 |
- return ( |
|
306 |
- <td data-label="최근온도"> |
|
307 |
- {data['temperature']} |
|
308 |
- {/* <small>({data['time']})</small> */} |
|
309 |
- </td> |
|
310 |
- ) |
|
311 |
- }) |
|
312 |
- : null} |
|
337 |
+ <Temperature data={item} /> |
|
313 | 338 |
</tr> |
314 | 339 |
) |
315 | 340 |
})} |
--- client/views/pages/healthcare/HealthcareSelectOne.jsx
+++ client/views/pages/healthcare/HealthcareSelectOne.jsx
... | ... | @@ -183,6 +183,25 @@ |
183 | 183 |
}); |
184 | 184 |
}; |
185 | 185 |
|
186 |
+ //특정 대상자의 일별 배터리 목록 |
|
187 |
+ const [seniorBatteryListByDay, setSeniorBatteryListByDay] = React.useState([]); |
|
188 |
+ //특정 대상자의 일별 배터리 목록 조회 |
|
189 |
+ const seniorBatterySelectListByDay = (seniorNum) => { |
|
190 |
+ fetch("/user/seniorBatterySelectListByDay.json", { |
|
191 |
+ method: "POST", |
|
192 |
+ headers: { |
|
193 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
194 |
+ }, |
|
195 |
+ body: JSON.stringify(seniorNum), |
|
196 |
+ }).then((response) => response.json()).then((data) => { |
|
197 |
+ console.log("seniorBatteryListByDay data : ", data); |
|
198 |
+ setSeniorBatteryListByDay(data); |
|
199 |
+ console.log("seniorBatteryListByDay : ", seniorBatteryListByDay); |
|
200 |
+ }).catch((error) => { |
|
201 |
+ console.log('seniorBatterySelectListByDay() /user/seniorBatterySelectListByDay.json error : ', error); |
|
202 |
+ }); |
|
203 |
+ }; |
|
204 |
+ |
|
186 | 205 |
//방문 기록 정보 |
187 | 206 |
const [visitRecordList, setVisitRecordList] = React.useState({}); |
188 | 207 |
const visitRecordInit = { |
... | ... | @@ -267,6 +286,7 @@ |
267 | 286 |
setVisitRecordInit(); |
268 | 287 |
seniorMedicationSelectList(senior); |
269 | 288 |
seniorTemperatureSelectListByDay(senior); |
289 |
+ seniorBatterySelectListByDay(senior); |
|
270 | 290 |
visitRecordSelectList(senior); |
271 | 291 |
closeModal(); |
272 | 292 |
alert("등록완료"); |
... | ... | @@ -428,6 +448,7 @@ |
428 | 448 |
seniorSelectOne(); |
429 | 449 |
seniorMedicationSelectList(senior); |
430 | 450 |
seniorTemperatureSelectListByDay(senior); |
451 |
+ seniorBatterySelectListByDay(senior); |
|
431 | 452 |
visitRecordSelectList(senior); |
432 | 453 |
}, []) |
433 | 454 |
|
... | ... | @@ -461,10 +482,18 @@ |
461 | 482 |
<tbody> |
462 | 483 |
<DateMedication /> |
463 | 484 |
<DateTemperature /> |
464 |
- <tr> |
|
465 |
- <th>배터리</th> |
|
466 |
- <td colSpan={5}>30%</td> |
|
467 |
- </tr> |
|
485 |
+ {seniorBatteryListByDay.map((item, idx) => { |
|
486 |
+ return ( |
|
487 |
+ item['battery_date'] == visitDate ? ( |
|
488 |
+ <tr> |
|
489 |
+ <th>배터리</th> |
|
490 |
+ <td colSpan={5}> |
|
491 |
+ {item['battery_power_data']} |
|
492 |
+ </td> |
|
493 |
+ </tr> |
|
494 |
+ ) : null |
|
495 |
+ ) |
|
496 |
+ })} |
|
468 | 497 |
</tbody> |
469 | 498 |
</table> |
470 | 499 |
|
--- client/views/pages/join/Join.jsx
+++ client/views/pages/join/Join.jsx
... | ... | @@ -432,7 +432,7 @@ |
432 | 432 |
|
433 | 433 |
<div className="btn-wrap"> |
434 | 434 |
<button className={"gray-btn btn-large"} onClick={() => {navigate(-1)}}>취소</button> |
435 |
- {user['authority'] == "ROLE_AGENCY" |
|
435 |
+ {user['authority'] == "ROLE_AGENCYADMIN" || user['authority'] == "ROLE_AGENCY" |
|
436 | 436 |
? <button className={"red-btn btn-large"} onClick={agentInsert}>등록</button> |
437 | 437 |
: <button className={"red-btn btn-large"} onClick={userInsert}>등록</button> |
438 | 438 |
} |
--- client/views/pages/senior_management/SeniorSelectOne.jsx
+++ client/views/pages/senior_management/SeniorSelectOne.jsx
... | ... | @@ -376,6 +376,7 @@ |
376 | 376 |
if (data > 0) { |
377 | 377 |
alert("담당자 매칭이 종료 되었습니다."); |
378 | 378 |
seniorSelectListByAgent(); |
379 |
+ agentSelectListBySenior(); |
|
379 | 380 |
} else { |
380 | 381 |
alert("담당자 매칭 종료에 실패하였습니다."); |
381 | 382 |
} |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?