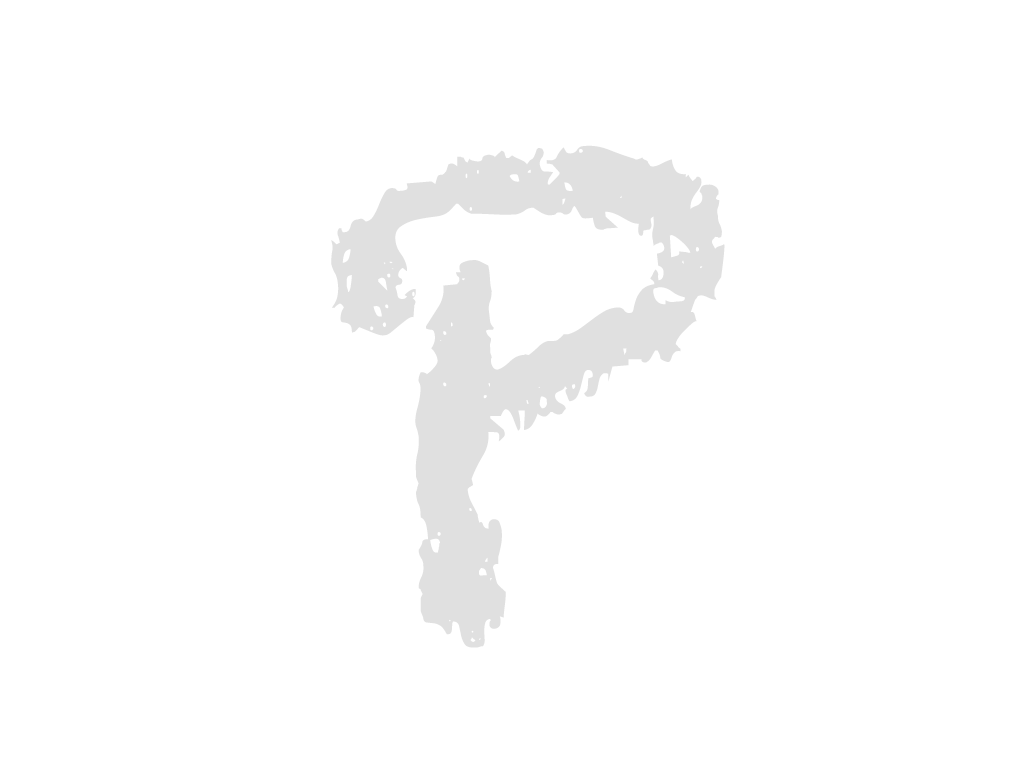
--- client/views/component/chart/Chart7.jsx
+++ client/views/component/chart/Chart7.jsx
... | ... | @@ -2,205 +2,162 @@ |
2 | 2 |
import * as am5 from "@amcharts/amcharts5"; |
3 | 3 |
import * as am5xy from "@amcharts/amcharts5/xy"; |
4 | 4 |
import am5themes_Animated from "@amcharts/amcharts5/themes/Animated"; |
5 |
+import CommonUtil from "../../../resources/js/CommonUtil"; |
|
5 | 6 |
|
6 |
-class Chart6 extends Component { |
|
7 |
- componentDidMount() { |
|
8 |
- am5.ready(function () { |
|
9 | 7 |
|
10 |
- // Create root element |
|
11 |
- // https://www.amcharts.com/docs/v5/getting-started/#Root_element |
|
12 |
- var root = am5.Root.new("chart7"); |
|
8 |
+export default function Chart7({ data }) { |
|
9 |
+ const createChart = () => { |
|
10 |
+ console.log('createChart7 data : ', data); |
|
13 | 11 |
|
14 |
- // Set themes |
|
15 |
- // https://www.amcharts.com/docs/v5/concepts/themes/ |
|
16 |
- root.setThemes([ |
|
17 |
- am5themes_Animated.new(root) |
|
18 |
- ]); |
|
12 |
+ /* Chart code */ |
|
19 | 13 |
|
20 |
- // Create chart |
|
21 |
- // https://www.amcharts.com/docs/v5/charts/xy-chart/ |
|
22 |
- var chart = root.container.children.push( |
|
23 |
- am5xy.XYChart.new(root, { |
|
24 |
- panX: false, |
|
25 |
- panY: false, |
|
26 |
- wheelX: "panX", |
|
27 |
- wheelY: "zoomX", |
|
28 |
- layout: root.verticalLayout, |
|
29 |
- arrangeTooltips: false |
|
14 |
+ // Create root element |
|
15 |
+ // https://www.amcharts.com/docs/v5/getting-started/#Root_element |
|
16 |
+ let root = am5.Root.new("Chart7"); |
|
17 |
+ root._logo.dispose(); |
|
18 |
+ // Set themes |
|
19 |
+ // https://www.amcharts.com/docs/v5/concepts/themes/ |
|
20 |
+ root.setThemes([ |
|
21 |
+ am5themes_Animated.new(root) |
|
22 |
+ ]); |
|
23 |
+ |
|
24 |
+ // Create chart |
|
25 |
+ // https://www.amcharts.com/docs/v5/charts/xy-chart/ |
|
26 |
+ let chart = root.container.children.push( |
|
27 |
+ am5xy.XYChart.new(root, { |
|
28 |
+ panX: false, |
|
29 |
+ panY: false, |
|
30 |
+ wheelX: "panX", |
|
31 |
+ wheelY: "zoomX", |
|
32 |
+ layout: root.verticalLayout, |
|
33 |
+ arrangeTooltips: false |
|
34 |
+ }) |
|
35 |
+ ); |
|
36 |
+ |
|
37 |
+ // Use only absolute numbers |
|
38 |
+ chart.getNumberFormatter().set("numberFormat", "#.#s"); |
|
39 |
+ |
|
40 |
+ // Add legend |
|
41 |
+ // https://www.amcharts.com/docs/v5/charts/xy-chart/legend-xy-series/ |
|
42 |
+ let legend = chart.children.push( |
|
43 |
+ am5.Legend.new(root, { |
|
44 |
+ centerX: am5.p50, |
|
45 |
+ x: am5.p50 |
|
46 |
+ }) |
|
47 |
+ ); |
|
48 |
+ |
|
49 |
+ // Create axes |
|
50 |
+ // https://www.amcharts.com/docs/v5/charts/xy-chart/axes/ |
|
51 |
+ let yAxis = chart.yAxes.push( |
|
52 |
+ am5xy.CategoryAxis.new(root, { |
|
53 |
+ categoryField: "age", |
|
54 |
+ renderer: am5xy.AxisRendererY.new(root, { |
|
55 |
+ inversed: true, |
|
56 |
+ cellStartLocation: 0.1, |
|
57 |
+ cellEndLocation: 0.9 |
|
30 | 58 |
}) |
31 |
- ); |
|
59 |
+ }) |
|
60 |
+ ); |
|
32 | 61 |
|
33 |
- // Use only absolute numbers |
|
34 |
- chart.getNumberFormatter().set("numberFormat", "#.#s"); |
|
62 |
+ yAxis.data.setAll(data); |
|
35 | 63 |
|
36 |
- // Add legend |
|
37 |
- // https://www.amcharts.com/docs/v5/charts/xy-chart/legend-xy-series/ |
|
38 |
- var legend = chart.children.push( |
|
39 |
- am5.Legend.new(root, { |
|
40 |
- centerX: am5.p50, |
|
41 |
- x: am5.p50 |
|
64 |
+ let xAxis = chart.xAxes.push( |
|
65 |
+ am5xy.ValueAxis.new(root, { |
|
66 |
+ renderer: am5xy.AxisRendererX.new(root, { |
|
67 |
+ strokeOpacity: 0.1 |
|
42 | 68 |
}) |
43 |
- ); |
|
69 |
+ }) |
|
70 |
+ ); |
|
44 | 71 |
|
45 |
- // Data |
|
46 |
- var data = [ |
|
47 |
- { |
|
48 |
- age: "85+", |
|
49 |
- male: -0.1, |
|
50 |
- female: 0.3 |
|
51 |
- }, |
|
52 |
- { |
|
53 |
- age: "80-54", |
|
54 |
- male: -0.2, |
|
55 |
- female: 0.3 |
|
56 |
- }, |
|
57 |
- { |
|
58 |
- age: "75-79", |
|
59 |
- male: -0.3, |
|
60 |
- female: 0.6 |
|
61 |
- }, |
|
62 |
- { |
|
63 |
- age: "70-74", |
|
64 |
- male: -0.5, |
|
65 |
- female: 0.8 |
|
66 |
- }, |
|
67 |
- { |
|
68 |
- age: "65-69", |
|
69 |
- male: -0.8, |
|
70 |
- female: 1.0 |
|
71 |
- }, |
|
72 |
- { |
|
73 |
- age: "60-64", |
|
74 |
- male: -1.1, |
|
75 |
- female: 1.3 |
|
76 |
- }, |
|
77 |
- { |
|
78 |
- age: "55-59", |
|
79 |
- male: -1.7, |
|
80 |
- female: 1.9 |
|
81 |
- }, |
|
82 |
- { |
|
83 |
- age: "50-54", |
|
84 |
- male: -2.2, |
|
85 |
- female: 2.5 |
|
86 |
- }, |
|
87 |
- ]; |
|
88 |
- |
|
89 |
- // Create axes |
|
90 |
- // https://www.amcharts.com/docs/v5/charts/xy-chart/axes/ |
|
91 |
- var yAxis = chart.yAxes.push( |
|
92 |
- am5xy.CategoryAxis.new(root, { |
|
93 |
- categoryField: "age", |
|
94 |
- renderer: am5xy.AxisRendererY.new(root, { |
|
95 |
- inversed: true, |
|
96 |
- cellStartLocation: 0.1, |
|
97 |
- cellEndLocation: 0.9 |
|
72 |
+ // Add series |
|
73 |
+ // https://www.amcharts.com/docs/v5/charts/xy-chart/series/ |
|
74 |
+ function createSeries(field, labelCenterX, pointerOrientation, rangeValue) { |
|
75 |
+ let series = chart.series.push( |
|
76 |
+ am5xy.ColumnSeries.new(root, { |
|
77 |
+ xAxis: xAxis, |
|
78 |
+ yAxis: yAxis, |
|
79 |
+ valueXField: field, |
|
80 |
+ categoryYField: "age", |
|
81 |
+ sequencedInterpolation: true, |
|
82 |
+ clustered: false, |
|
83 |
+ tooltip: am5.Tooltip.new(root, { |
|
84 |
+ pointerOrientation: pointerOrientation, |
|
85 |
+ labelText: "{categoryY}: {valueX}" |
|
98 | 86 |
}) |
99 | 87 |
}) |
100 | 88 |
); |
101 | 89 |
|
102 |
- yAxis.data.setAll(data); |
|
90 |
+ series.columns.template.setAll({ |
|
91 |
+ height: am5.p100, |
|
92 |
+ strokeOpacity: 0, |
|
93 |
+ fillOpacity: 0.8 |
|
94 |
+ }); |
|
103 | 95 |
|
104 |
- var xAxis = chart.xAxes.push( |
|
105 |
- am5xy.ValueAxis.new(root, { |
|
106 |
- renderer: am5xy.AxisRendererX.new(root, { |
|
107 |
- strokeOpacity: 0.1 |
|
96 |
+ series.bullets.push(function () { |
|
97 |
+ return am5.Bullet.new(root, { |
|
98 |
+ locationX: 1, |
|
99 |
+ locationY: 0.5, |
|
100 |
+ sprite: am5.Label.new(root, { |
|
101 |
+ centerY: am5.p50, |
|
102 |
+ text: "{valueX}", |
|
103 |
+ populateText: true, |
|
104 |
+ centerX: labelCenterX |
|
108 | 105 |
}) |
109 |
- }) |
|
110 |
- ); |
|
111 |
- |
|
112 |
- // Add series |
|
113 |
- // https://www.amcharts.com/docs/v5/charts/xy-chart/series/ |
|
114 |
- function createSeries(field, labelCenterX, pointerOrientation, rangeValue) { |
|
115 |
- var series = chart.series.push( |
|
116 |
- am5xy.ColumnSeries.new(root, { |
|
117 |
- xAxis: xAxis, |
|
118 |
- yAxis: yAxis, |
|
119 |
- valueXField: field, |
|
120 |
- categoryYField: "age", |
|
121 |
- sequencedInterpolation: true, |
|
122 |
- clustered: false, |
|
123 |
- tooltip: am5.Tooltip.new(root, { |
|
124 |
- pointerOrientation: pointerOrientation, |
|
125 |
- labelText: "{categoryY}: {valueX}" |
|
126 |
- }) |
|
127 |
- }) |
|
128 |
- ); |
|
129 |
- |
|
130 |
- series.columns.template.setAll({ |
|
131 |
- height: am5.p100, |
|
132 |
- strokeOpacity: 0, |
|
133 |
- fillOpacity: 0.8 |
|
134 | 106 |
}); |
107 |
+ }); |
|
135 | 108 |
|
136 |
- series.bullets.push(function () { |
|
137 |
- return am5.Bullet.new(root, { |
|
138 |
- locationX: 1, |
|
139 |
- locationY: 0.5, |
|
140 |
- sprite: am5.Label.new(root, { |
|
141 |
- centerY: am5.p50, |
|
142 |
- text: "{valueX}", |
|
143 |
- populateText: true, |
|
144 |
- centerX: labelCenterX |
|
145 |
- }) |
|
146 |
- }); |
|
147 |
- }); |
|
109 |
+ series.data.setAll(data); |
|
110 |
+ series.appear(); |
|
148 | 111 |
|
149 |
- series.data.setAll(data); |
|
150 |
- series.appear(); |
|
112 |
+ let rangeDataItem = xAxis.makeDataItem({ |
|
113 |
+ value: rangeValue |
|
114 |
+ }); |
|
115 |
+ xAxis.createAxisRange(rangeDataItem); |
|
116 |
+ rangeDataItem.get("grid").setAll({ |
|
117 |
+ strokeOpacity: 1, |
|
118 |
+ stroke: series.get("stroke") |
|
119 |
+ }); |
|
151 | 120 |
|
152 |
- var rangeDataItem = xAxis.makeDataItem({ |
|
153 |
- value: rangeValue |
|
154 |
- }); |
|
155 |
- xAxis.createAxisRange(rangeDataItem); |
|
156 |
- rangeDataItem.get("grid").setAll({ |
|
157 |
- strokeOpacity: 1, |
|
158 |
- stroke: series.get("stroke") |
|
159 |
- }); |
|
121 |
+ let label = rangeDataItem.get("label"); |
|
122 |
+ label.setAll({ |
|
123 |
+ text: field.toUpperCase(), |
|
124 |
+ fontSize: "1.1em", |
|
125 |
+ fill: series.get("stroke"), |
|
126 |
+ paddingTop: 10, |
|
127 |
+ isMeasured: false, |
|
128 |
+ centerX: labelCenterX |
|
129 |
+ }); |
|
130 |
+ label.adapters.add("dy", function () { |
|
131 |
+ return -chart.plotContainer.height(); |
|
132 |
+ }); |
|
160 | 133 |
|
161 |
- var label = rangeDataItem.get("label"); |
|
162 |
- label.setAll({ |
|
163 |
- text: field.toUpperCase(), |
|
164 |
- fontSize: "1.1em", |
|
165 |
- fill: series.get("stroke"), |
|
166 |
- paddingTop: 10, |
|
167 |
- isMeasured: false, |
|
168 |
- centerX: labelCenterX |
|
169 |
- }); |
|
170 |
- label.adapters.add("dy", function () { |
|
171 |
- return -chart.plotContainer.height(); |
|
172 |
- }); |
|
173 |
- |
|
174 |
- return series; |
|
175 |
- } |
|
176 |
- |
|
177 |
- createSeries("male", am5.p100, "right", -3); |
|
178 |
- createSeries("female", 0, "left", 4); |
|
179 |
- |
|
180 |
- // Add cursor |
|
181 |
- // https://www.amcharts.com/docs/v5/charts/xy-chart/cursor/ |
|
182 |
- var cursor = chart.set("cursor", am5xy.XYCursor.new(root, { |
|
183 |
- behavior: "zoomY" |
|
184 |
- })); |
|
185 |
- cursor.lineY.set("forceHidden", true); |
|
186 |
- cursor.lineX.set("forceHidden", true); |
|
187 |
- |
|
188 |
- // Make stuff animate on load |
|
189 |
- // https://www.amcharts.com/docs/v5/concepts/animations/ |
|
190 |
- chart.appear(1000, 100); |
|
191 |
- |
|
192 |
- }); // end am5.ready() |
|
193 |
- } |
|
194 |
- |
|
195 |
- componentWillUnmount() { |
|
196 |
- if (this.root) { |
|
197 |
- this.root.dispose(); |
|
134 |
+ return series; |
|
198 | 135 |
} |
136 |
+ |
|
137 |
+ createSeries("male", am5.p100, "right", -2); |
|
138 |
+ createSeries("female", 0, "left", 2); |
|
139 |
+ |
|
140 |
+ // Add cursor |
|
141 |
+ // https://www.amcharts.com/docs/v5/charts/xy-chart/cursor/ |
|
142 |
+ let cursor = chart.set("cursor", am5xy.XYCursor.new(root, { |
|
143 |
+ behavior: "zoomY" |
|
144 |
+ })); |
|
145 |
+ cursor.lineY.set("forceHidden", true); |
|
146 |
+ cursor.lineX.set("forceHidden", true); |
|
147 |
+ |
|
148 |
+ // Make stuff animate on load |
|
149 |
+ // https://www.amcharts.com/docs/v5/concepts/animations/ |
|
150 |
+ chart.appear(1000, 100); |
|
199 | 151 |
} |
200 | 152 |
|
201 |
- render() { |
|
202 |
- return <div id="chart7" style={{ width: "100%", height: "100%" }}></div>; |
|
203 |
- } |
|
204 |
-} |
|
153 |
+ React.useEffect(() => { |
|
154 |
+ console.log('React.useEffect chart8 data : ', data); |
|
155 |
+ if (CommonUtil.isEmpty(data) == false) { |
|
156 |
+ createChart(); |
|
157 |
+ } |
|
158 |
+ }, [data]) |
|
205 | 159 |
|
206 |
-export default Chart6; |
|
160 |
+ return ( |
|
161 |
+ <div id="Chart7" style={{ width: "100%", height: "100%" }}></div> |
|
162 |
+ ) |
|
163 |
+}(파일 끝에 줄바꿈 문자 없음) |
--- client/views/pages/main/Main_agency.jsx
+++ client/views/pages/main/Main_agency.jsx
... | ... | @@ -9,7 +9,7 @@ |
9 | 9 |
import AddCircleIcon from "@mui/icons-material/AddCircle"; |
10 | 10 |
import Calendar_agency from "../../component/Calendar_agency.jsx"; |
11 | 11 |
import Chart10 from "../../component/chart/Chart10.jsx"; |
12 |
-import Cart7 from "../../component/chart/Chart7.jsx"; |
|
12 |
+import Chart7 from "../../component/chart/Chart7.jsx"; |
|
13 | 13 |
import Chart8 from "../../component/chart/Chart8.jsx"; |
14 | 14 |
import tool from "../../../resources/files/images/tool.png"; |
15 | 15 |
import medicinebox from "../../../resources/files/images/medicinebox.png"; |
... | ... | @@ -59,6 +59,7 @@ |
59 | 59 |
} |
60 | 60 |
}); |
61 | 61 |
// 내 시니어 목록 조회 |
62 |
+ const [seniorGenderData, setSeniorGenderData] = React.useState([]); |
|
62 | 63 |
const mySeniorSelectList = () => { |
63 | 64 |
fetch("/user/seniorSelectList.json", { |
64 | 65 |
method: "POST", |
... | ... | @@ -78,26 +79,31 @@ |
78 | 79 |
let age = today.getFullYear() - birth.getFullYear() + 1; |
79 | 80 |
seniorGenderArr.push({ "gender": item['user_gender'], "age": age }) |
80 | 81 |
}) |
81 |
- console.log("seniorGenderArr : ", seniorGenderArr) |
|
82 | 82 |
|
83 | 83 |
let genderData = []; |
84 |
- for (let i = 4; i < 14; i++) { |
|
84 |
+ for (let i = 0; i < 18; i++) { |
|
85 | 85 |
let age = i * 5; |
86 |
- genderData.push({ "age": age, "male": 0, "female": 0 }) |
|
86 |
+ let age1 = ((i + 1) * 5) - 1; |
|
87 |
+ if (i == 17) { |
|
88 |
+ genderData.push({ "age": age + '+', "male": 0, "female": 0 }) |
|
89 |
+ } else { |
|
90 |
+ genderData.push({ "age": age + '-' + age1, "male": 0, "female": 0 }) |
|
91 |
+ } |
|
87 | 92 |
} |
88 | 93 |
|
89 | 94 |
seniorGenderArr.map((item, idx) => { |
90 | 95 |
for (let i = 0; i < 18; i++) { |
91 | 96 |
if (item['age'] > i * 5 && item['age'] < ((i + 1) * 5) - 1) { |
92 | 97 |
if (item['gender'] == "남") { |
93 |
- genderData[i]['male'] = genderData[i]['male'] + 1 |
|
98 |
+ genderData[i]['male'] = genderData[i]['male'] - 1 |
|
94 | 99 |
} else if (item['gender'] == "여") { |
95 | 100 |
genderData[i]['female'] = genderData[i]['female'] + 1 |
96 | 101 |
} |
97 | 102 |
} |
98 | 103 |
} |
99 | 104 |
}) |
100 |
- console.log("genderData: ", genderData) |
|
105 |
+ |
|
106 |
+ setSeniorGenderData(genderData) |
|
101 | 107 |
}).catch((error) => { |
102 | 108 |
console.log('seniorSelectList() /user/seniorSelectList.json error : ', error); |
103 | 109 |
}); |
... | ... | @@ -353,7 +359,7 @@ |
353 | 359 |
<div> |
354 | 360 |
<div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"연령대별 통계"} /></div> |
355 | 361 |
<div className="content-box combine-left-government3 visitlist margin-bottom2"> |
356 |
- <Cart7 /> |
|
362 |
+ <Chart7 data={seniorGenderData} /> |
|
357 | 363 |
</div> |
358 | 364 |
</div> |
359 | 365 |
</div> |
--- server/modules/web/Server.js
+++ server/modules/web/Server.js
... | ... | @@ -17,12 +17,12 @@ |
17 | 17 |
|
18 | 18 |
http.createServer(webServer).listen(PORT); |
19 | 19 |
https.createServer({ |
20 |
- key: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.com/u-dolbom.com_nopass.key`), |
|
20 |
+ /* key: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.com/u-dolbom.com_nopass.key`), |
|
21 | 21 |
cert: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.com/u-dolbom.com.pem`), |
22 |
- ca: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.com/Chain_RootCA_Bundle.crt`), |
|
23 |
- /* key: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.co.kr/u-dolbom.co.kr_nopass.key`), |
|
22 |
+ ca: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.com/Chain_RootCA_Bundle.crt`), */ |
|
23 |
+ key: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.co.kr/u-dolbom.co.kr_nopass.key`), |
|
24 | 24 |
cert: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.co.kr/u-dolbom.co.kr.pem`), |
25 |
- ca: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.co.kr/Chain_RootCA_Bundle.crt`), */ |
|
25 |
+ ca: fs.readFileSync(`${BASE_DIR}/server/modules/web/ssl/u-dolbom.co.kr/Chain_RootCA_Bundle.crt`), |
|
26 | 26 |
/* requestCert: false, |
27 | 27 |
rejectUnauthorized: false */ |
28 | 28 |
}, webServer).listen(443); |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?