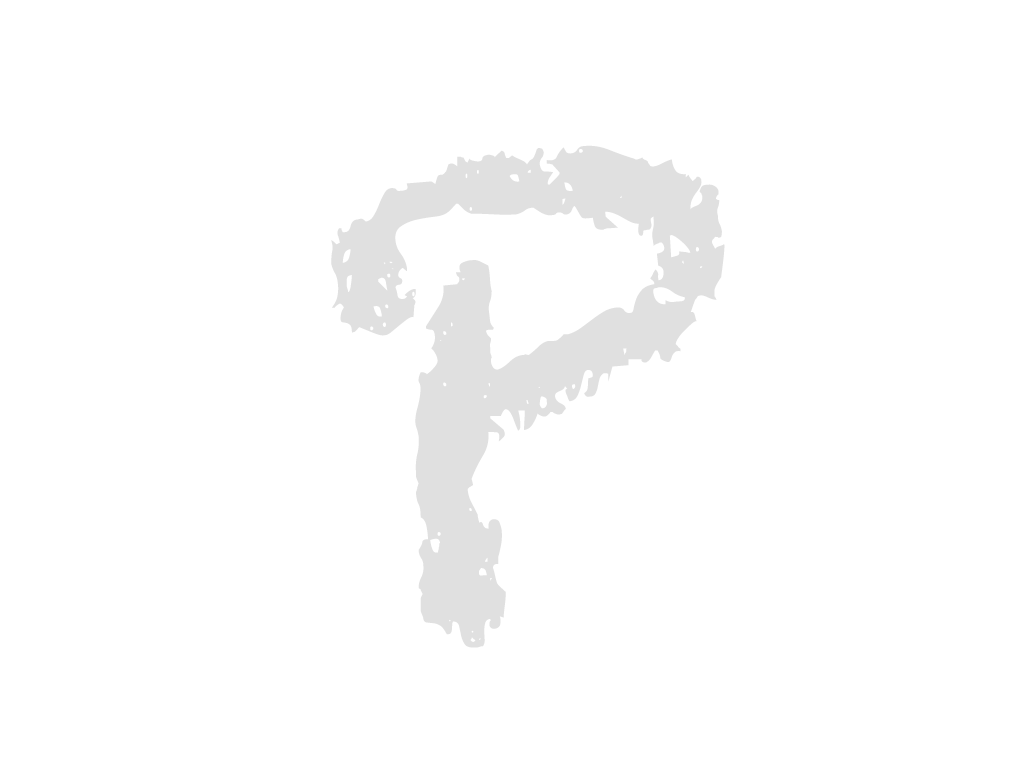
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="title-box flex justify-between mb40">
<p class="title">지문 상세 보기</p>
</div>
<div class="board-wrap">
<div class="flex align-center mb20">
<label for="" class="title2">제목</label>
<input v-if="isEditing" type="text" v-model="post.textTtl" class=" data-wrap">
<p class="data-wrap" v-else>{{ post.textTtl }}</p>
</div>
<div class="flex align-center mb20">
<label for="" class="title2">URL</label>
<input v-if="isEditing" type="text" v-model="post.textUrl" class="data-wrap">
<p v-else class="data-wrap">{{ post.textUrl }}</p>
</div>
<div class="flex align-center mb20" style="display: flex; flex-direction: row;">
<label class="title2">형식</label>
<label class="title2">
<input type="radio" v-model="post.textTypeId" value="1" class="data-wrap" :disabled="!isEditing"> 일반
</label>
<label class="title2">
<input type="radio" v-model="post.textTypeId" value="2" class="data-wrap" :disabled="!isEditing"> 대화
</label>
<label class="title2">
<input type="radio" v-model="post.textTypeId" value="3" class="data-wrap" :disabled="!isEditing"> 책 리스닝
</label>
<select v-if="isEditing" id="" v-model="post.bookId" @change="fetchUnits" class="ml20">
<option value="" disabled>교재를 선택하세요</option>
<option v-for="book in books" :key="book.book_id" :value="book.book_id">
{{ book.book_nm }}
</option>
</select>
<label v-else for="" class="title2 flex align-center ml20" style="display: flex; width: 20rem;">교재:
<p class="title2">{{ post.bookName }}</p>
</label>
<select v-if="isEditing" name="" id="" v-model="post.unitId" class="ml20">
<option value="" disabled>단원을 선택하세요</option>
<option v-for="unit in units" :key="unit.unitId" :value="unit.unitId">
{{ unit.unitName }}
</option>
</select>
<label v-else for="" class="title2 flex align-center ml20"
style="display: flex; width: 20rem; text-align: right;">단원:
<p class="title2">{{ post.unitName }}</p>
</label>
</div>
<hr>
<div class="flex align-center">
<label for="" class="title2">스크립트</label>
<textarea v-if="isEditing" v-model="post.textCnt" class="data-wrap"></textarea>
<p v-else class="data-wrap">{{ post.textCnt }}</p>
</div>
<hr>
<div class="flex align-center">
<label for="" class="title2">첨부파일</label>
<input v-if="isEditing" type="file" ref="fileInput" @change="handleFileUpload" multiple />
<label v-else for="" class="title2">{{ file.fileNm }}</label>
</div>
</div>
<div class="flex justify-between mt50">
<button type="button" title="목록으로" class="new-btn" @click="goToPage('TextList')">
목록
</button>
<div class="flex">
<button type="button" title="수정" class="new-btn mr10" @click="toggleEdit">
{{ isEditing ? '저장' : '수정' }}
</button>
<button type="button" title="삭제" class="new-btn" @click="deletePost">
삭제
</button>
</div>
</div>
</template>
<script>
import axios from "axios";
import SvgIcon from '@jamescoyle/vue-icon';
import { mdiMagnify } from '@mdi/js';
export default {
data() {
return {
mdiMagnify: mdiMagnify,
post: {
textId: "",
textTtl: "",
textCnt: "",
textUrl: "",
textTypeId: "",
bookId: "",
unitId: "",
bookName: "",
unitName: "",
fileMngId: "",
},
books: [],
units: [],
file: { fileNm : '' },
isEditing: false,
selectedFiles: {},
};
},
computed: {
textId() {
return this.$route.query.textId;
},
selectedBookName() {
const book = this.books.find(book => book.book_id === this.post.bookId);
return book ? book.book_nm : '';
},
selectedUnitName() {
const unit = this.units.find(unit => unit.unit_id === this.post.unitId);
return unit ? unit.unit_nm : '';
}
},
methods: {
goToPage(page) {
this.$router.push({ name: page });
},
handleFileUpload(e) {
this.selectedFiles = e.target.files;
},
fetchPostDetail() {
const textId = this.$route.query.textId;
axios.post('/text/selectOneText.json', { textId }, {
headers: {
"Content-Type": "application/json; charset=UTF-8",
}
})
.then(response => {
if (response.data && response.data[0]) {
this.post.textId = response.data[0].text_id;
this.post.textTtl = response.data[0].text_ttl;
this.post.textCnt = response.data[0].text_cnt;
this.post.textUrl = response.data[0].text_url;
this.post.textTypeId = response.data[0].text_type_id;
this.post.bookId = response.data[0].book_id;
this.post.unitId = response.data[0].unit_id;
this.post.bookName = response.data[0].book_name;
this.post.unitName = response.data[0].unit_name;
this.post.fileMngId = response.data[0].file_mng_id;
if(this.post.fileMngId) {
this.findFile();
}
} else {
this.error = "Failed to fetch post details.";
}
})
.catch(error => {
console.error("Error fetching post detail:", error);
this.error = "Failed to fetch post details.";
});
},
findFile() {
const vm = this;
axios({
url: "/file/find.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
file_mng_id: vm.post.fileMngId,
},
})
.then(function (res) {
console.log("fileInfo - response : ", res.data.list[0]);
vm.file = res.data.list[0];
})
.catch(function (error) {
console.log("result - error : ", error);
});
},
async dataInsert() {
this.newPost = {
textId: this.post.textId,
textTtl: this.post.textTtl,
textCnt: this.post.textCnt,
textUrl: this.post.textUrl,
textTypeId: this.post.textTypeId,
bookId: this.post.bookId,
unitId: this.post.unitId,
fileMngId: this.post.fileMngId,
};
if (this.selectedFiles && this.selectedFiles.length > 0) {
// 파일 업로드
const formData = new FormData();
for (let i = 0; i < this.selectedFiles.length; i++) {
formData.append("files", this.selectedFiles[i]);
}
const fileUploadResponse = await axios.post(
"/file/upload.json",
formData,
{
headers: {
"Content-Type": "multipart/form-data",
},
}
);
// 업로드 후 파일 매니지 아이디 호출
vm.newPost.fileMngId = fileUploadResponse.data.fileMngId;
}
console.log(this.newPost)
axios.post("/text/textUpdate.json", this.newPost, {
headers: {
"Content-Type": "application/json; charset=UTF-8",
}
})
.then(response => {
alert(response.data.message);
this.fetchPostDetail();
})
.catch(error => {
console.log("dataInsert - error:", error.response.data);
alert("지문 업데이트에 오류가 발생했습니다.");
});
},
toggleEdit() {
if (this.isEditing) {
this.dataInsert();
this.isEditing = false;
} else {
this.isEditing = true;
this.fetchBooks();
this.fetchUnits();
}
},
deletePost() {
const result = confirm('데이터를 삭제 하시겠습니까?')
if (result) {
} else {
alert("삭제를 취소했습니다")
return;
}
axios.post("/text/textDelete.json", { textId: this.textId }, {
headers: {
"Content-Type": "application/json; charset=UTF-8",
}
})
.then(response => {
alert(response.data.message);
this.goToPage('TextList');
})
.catch(error => {
console.error("Error deleting post:", error);
alert("지문 삭제에 오류가 발생했습니다.");
});
},
fetchBooks() {
axios.post("/book/findAll.json", {}, {
headers: {
"Content-Type": "application/json; charset=UTF-8",
}
})
.then(response => {
this.books = response.data;
})
.catch(error => {
console.error("fetchBooks - error:", error);
alert("교재 목록을 불러오는 중 오류가 발생했습니다.");
});
},
fetchUnits() {
if (!this.post.bookId) return;
axios.post("/unit/unitList.json", { bookId: this.post.bookId }, {
headers: {
"Content-Type": "application/json; charset=UTF-8",
}
})
.then(response => {
this.units = response.data;
})
.catch(error => {
console.error("fetchUnits - error:", error);
alert("단원 목록을 불러오는 중 오류가 발생했습니다.");
});
}
},
mounted() {
this.fetchPostDetail();
},
components: {
SvgIcon
}
};
</script>
<style scoped></style>