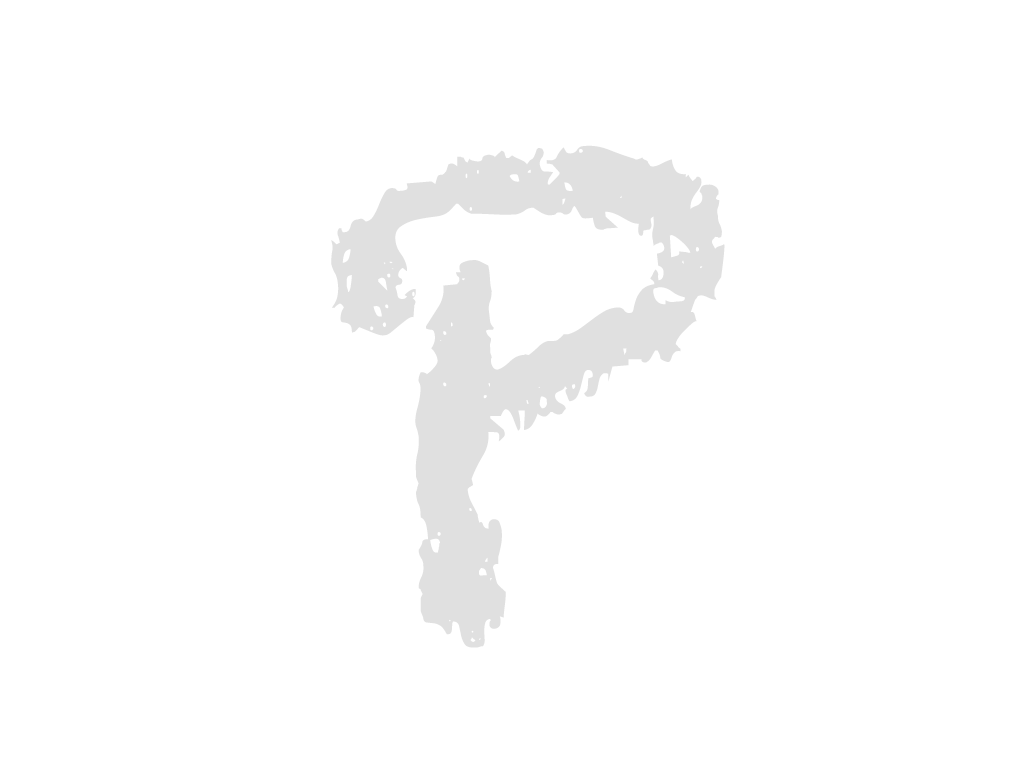
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="title-box flex justify-between mb40">
<p class="title">평가</p>
<select name="" id="" v-model="selectedBook" @change="fetchUnits">
<option value="" disabled selected>교재를 선택하세요</option>
<option v-for="book in books" :key="book.book_id" :value="book.book_id">
{{ book.book_nm }}
</option>
</select>
</div>
<div class="content-t">
<label for="" class="title2">단원</label>
<div class="unit-pagination flex mt10 mb20" style="gap: 10px;">
<button v-for="(unit, index) in units" :key="index"
:class="{ 'selected-btn': selectedUnit === unit.unitId }" @click="selectUnit(unit.unitId)">
{{ unit.unitName }}
</button>
</div>
<div class="search-wrap flex justify-end mb20">
<select id="evalType" class="mr10 data-wrap">
<option value="">전체</option>
<option value="중간평가">중간</option>
<option value="최종평가">최종</option>
</select>
<button type="button" title="위원회 검색" @click="fetchData">
<img src="../../../resources/img/look_t.png" alt="">
</button>
</div>
<div class="table-wrap">
<table>
<thead>
<td>No.</td>
<td>중간/최종</td>
<td>문항 갯수</td>
<td>보기</td>
</thead>
<tbody>
<tr v-for="(post, index) in posts" :key="post.evalId">
<td>{{ index + 1 }}</td>
<td>{{ post.evalType }}</td>
<td>{{ post.problemCount }}</td>
<td><button type="button" title="보기" class="new-btn"
@click="goToPage('ExamDetail', post.evalId)">
보기
</button></td>
</tr>
<!-- <tr :class="{ 'hidden-tr': !isRowVisible }" class="show-tr">
<td colspan="7">
<div>
<table>
<colgroup>
<col style="width: 10%;">
<col style="width: 70%;">
<col style="width: 20%;">
</colgroup>
<thead>
<td>No.</td>
<td>문제</td>
<td></td>
</thead>
<tbody>
<tr>
<td>1</td>
<td>1</td>
<td><button type="button" title="수정" class="new-btn" @click="goToPage('ExamDetail')">
수정
</button></td>
</tr>
</tbody>
</table>
</div>
</td>
</tr> -->
</tbody>
</table>
<div class="flex justify-end ">
<button type="button" title="등록" class="new-btn" @click="goToPage('ExamInsert')">
등록
</button>
</div>
</div>
</div>
</template>
<script>
import SvgIcon from '@jamescoyle/vue-icon';
import { mdiMagnify, mdiWindowClose } from '@mdi/js';
import axios from 'axios';
export default {
data() {
return {
mdiMagnify: mdiMagnify,
mdiWindowClose: mdiWindowClose,
showModal: false,
searchOpen: false,
isRowVisible: false,
books: [],
units: [],
selectedBook: "",
currentPage: 1,
pageSize: 10,
totalPosts: 0,
posts: [],
selectedUnit: null,
selectedEval: null,
}
},
methods: {
toggleRow() {
this.isRowVisible = !this.isRowVisible;
},
goToPage(page, evalId) {
this.$router.push({ name: page, query: { unit_id: this.selectedUnit, eval_id: evalId } });
},
showConfirm(type) {
let message = '';
if (type === 'cancel') {
message = '삭제하시겠습니까?';
} else if (type === 'reset') {
message = '초기화하시겠습니까?';
} else if (type === 'save') {
message = '등록하시겠습니까?';
}
if (confirm(message)) {
this.goBack();
}
},
selectUnit(unitId) {
this.selectedUnit = unitId;
this.fetchData();
},
closeModal() {
this.showModal = false;
},
buttonSearch() {
this.searchOpen = true;
},
closeBtn() {
this.searchOpen = false;
},
// 평가 정보 가져오기
fetchData() {
const evalType = document.getElementById('evalType').value;
const keyword = '';
const idx = (this.currentPage - 1) * this.pageSize;
let option = null;
let searchKeyword = null;
if (evalType !== '') {
option = 'eval_type';
searchKeyword = evalType;
} else if (keyword !== '') {
option = 'keyword';
searchKeyword = keyword;
}
axios({
url: "/evaluation/evaluationUnitList.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
"option": option,
"keyword": searchKeyword,
"pageSize": this.pageSize,
"startIndex": idx,
"unitId": this.selectedUnit
},
})
.then(response => {
this.posts = response.data;
})
.catch(error => {
console.error("fetchData - error: ", error);
alert("검색 중 오류가 발생했습니다.");
});
},
fetchBooks() {
axios({
url: "/book/findAll.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
})
.then(response => {
console.log(response.data)
this.books = response.data;
})
.catch(error => {
console.error("fetchBooks - error: ", error);
alert("교재 목록을 불러오는 중 오류가 발생했습니다.");
});
},
fetchUnits() {
if (!this.selectedBook) return;
axios({
url: "/unit/unitList.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
"bookId": this.selectedBook
},
})
.then(response => {
console.log(response.data)
this.units = response.data;
})
.catch(error => {
console.error("fetchUnits - error: ", error);
alert("단원 목록을 불러오는 중 오류가 발생했습니다.");
});
},
},
watch: {
},
computed: {
},
components: {
SvgIcon
},
mounted() {
this.selectedUnit = this.$route.query.unit_id || '';
this.selectedBook = this.$route.query.book_id || '';
this.fetchBooks();
this.fetchUnits();
if(this.selectedUnit){
this.fetchData();
}
}
}
</script>