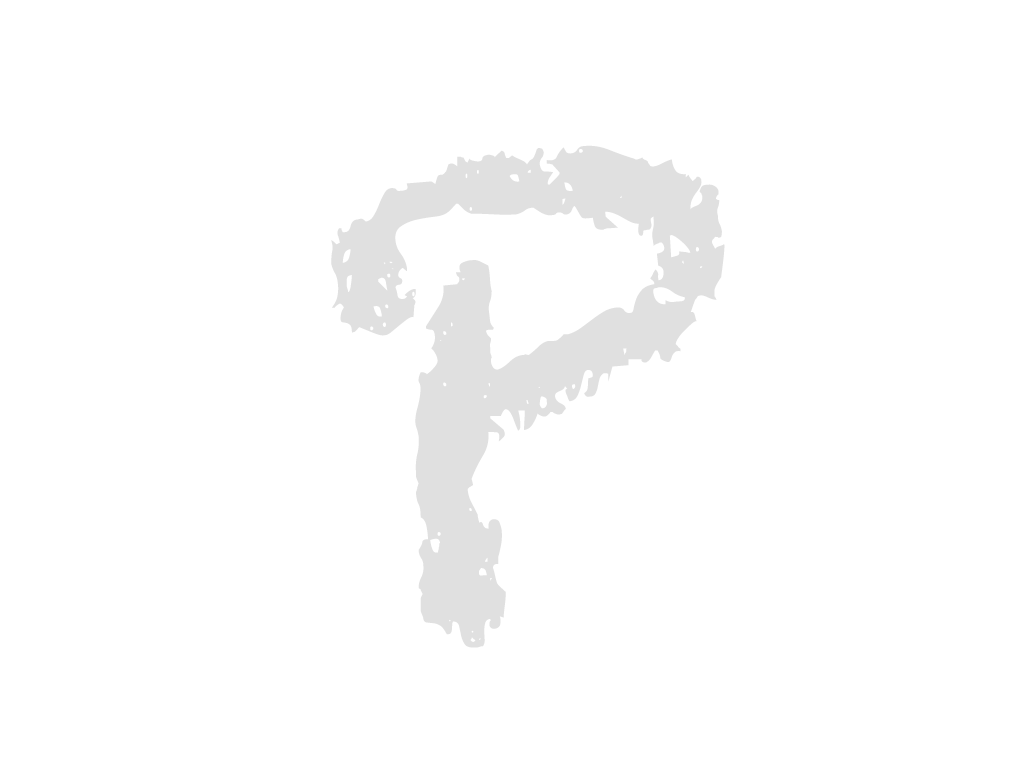
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="title-box flex justify-between mb40">
<p class="title">{{ titleLabel }}</p>
</div>
<div class="board-wrap">
<form @submit.prevent="handleButtonAction">
<div class="flex align-center">
<label for="title" class="title2">제목</label>
<input type="text" id="title" class="data-wrap" v-model="title" />
</div>
<hr />
<textarea name="" id="content" v-model="content"></textarea>
<hr />
<div class="flex align-center">
<label for="file" class="title2">첨부파일</label>
<label for="file" class="title2"> {{ file.fileNm }}</label>
<input
type="file"
ref="fileInput"
@change="handleFileUpload"
multiple
/>
</div>
<div class="flex justify-between mt50">
<button title="글쓰기" class="new-btn" @click="goToPage('Board')">
목록
</button>
<button title="글쓰기" class="new-btn">
{{ buttonLabel }}
</button>
</div>
</form>
</div>
</template>
<script>
import SvgIcon from "@jamescoyle/vue-icon";
import { mdiMagnify } from "@mdi/js";
import axios from "axios";
export default {
data() {
return {
mdiMagnify: mdiMagnify,
title: "",
content: "",
category: "Notice",
file: "",
sclsId: "",
bbsId: "",
fileMngId: null,
selectedFiles: [],
dataList: "",
// 데이터 편집 상태 (true = 수정, false = 등록)
isEditMode: false,
};
},
methods: {
goToPage(page) {
this.$router.push({ name: page });
},
handleFileUpload(e) {
this.selectedFiles = e.target.files;
},
created() {
const vm = this;
const sclsId = JSON.parse(sessionStorage.getItem("sclsId"));
const bbsId = JSON.parse(sessionStorage.getItem("bbsId"));
const file = JSON.parse(sessionStorage.getItem("file"));
if (sclsId) {
vm.sclsId = sclsId;
if (bbsId && file) {
vm.bbsId = bbsId;
vm.file = file;
vm.fileMngId = file.fileMngId;
vm.isEditMode = true;
vm.findBoard();
} else {
vm.isEditMode = false;
}
}
},
// 게시글 상세 조회
findBoard() {
const vm = this;
vm.bbsId = JSON.parse(sessionStorage.getItem("bbsId"));
console.log(vm.bbsId);
axios({
url: "/board/find.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
bbsId: vm.bbsId,
},
})
.then(function (res) {
console.log("boardData - response : ", res.data);
vm.dataList = res.data.result[0].boardClass[0].board[0];
vm.title = vm.dataList.bbsTtl;
vm.content = vm.dataList.bbsCnt;
vm.category = vm.dataList.bbsCls;
vm.time = vm.dataList.bbsTm;
})
.catch(function (error) {
console.log("boardData - error : ", error);
});
},
// 게시글 등록
async dataInsert() {
const vm = this;
try {
// 파일 업로드
if (this.selectedFiles && this.selectedFiles.length > 0) {
// 파일 업로드
const formData = new FormData();
for (let i = 0; i < this.selectedFiles.length; i++) {
formData.append("files", this.selectedFiles[i]);
}
const fileUploadResponse = await axios.post(
"http://localhost:9080/file/upload.json",
formData,
{
headers: {
"Content-Type": "multipart/form-data",
},
}
);
// 업로드 후 파일 매니지 아이디 호출
fileMngId = fileUploadResponse.data.fileMngId;
}
// 게시물 등록
const newData = {
bbsTtl: vm.title,
bbsCls: vm.category,
bbsCnt: vm.content,
fileMngId: vm.fileMngId,
sclsId: vm.sclsId,
};
await axios.post("/board/insert.json", newData);
alert("게시물 등록 성공");
vm.$router.push({ name: "Board" });
} catch (error) {
console.error("게시물 등록 실패 ", error);
alert("게시물 등록 실패");
}
},
// 게시글 수정
async boardUpdate() {
const vm = this;
// 파일 업로드
if (this.selectedFiles && this.selectedFiles.length > 0) {
// 파일 업로드
const formData = new FormData();
for (let i = 0; i < this.selectedFiles.length; i++) {
formData.append("files", this.selectedFiles[i]);
}
const fileUploadResponse = await axios.post(
"http://localhost:9080/file/upload.json",
formData,
{
headers: {
"Content-Type": "multipart/form-data",
},
}
);
// 업로드 후 파일 매니지 아이디 호출
fileMngId = fileUploadResponse.data.fileMngId;
}
const newData = {
bbsTtl: vm.title,
bbsCls: vm.category,
bbsCnt: vm.content,
fileMngId: vm.fileMngId,
bbsId: vm.bbsId,
};
await axios({
url: "/board/update.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: newData,
})
.then(function (res) {
console.log("dataUpdate - response : ", res.data);
})
.catch(function (error) {
console.log("result - error : ", error);
});
},
handleButtonAction() {
if (this.isEditMode) {
this.boardUpdate();
} else {
this.dataInsert();
}
},
},
watch: {},
computed: {
titleLabel() {
return this.isEditMode ? "수정" : "공지 등록";
},
buttonLabel() {
return this.isEditMode ? "수정" : "등록";
},
},
components: {
SvgIcon,
},
mounted() {
console.log("Main2 mounted");
this.created();
},
};
</script>