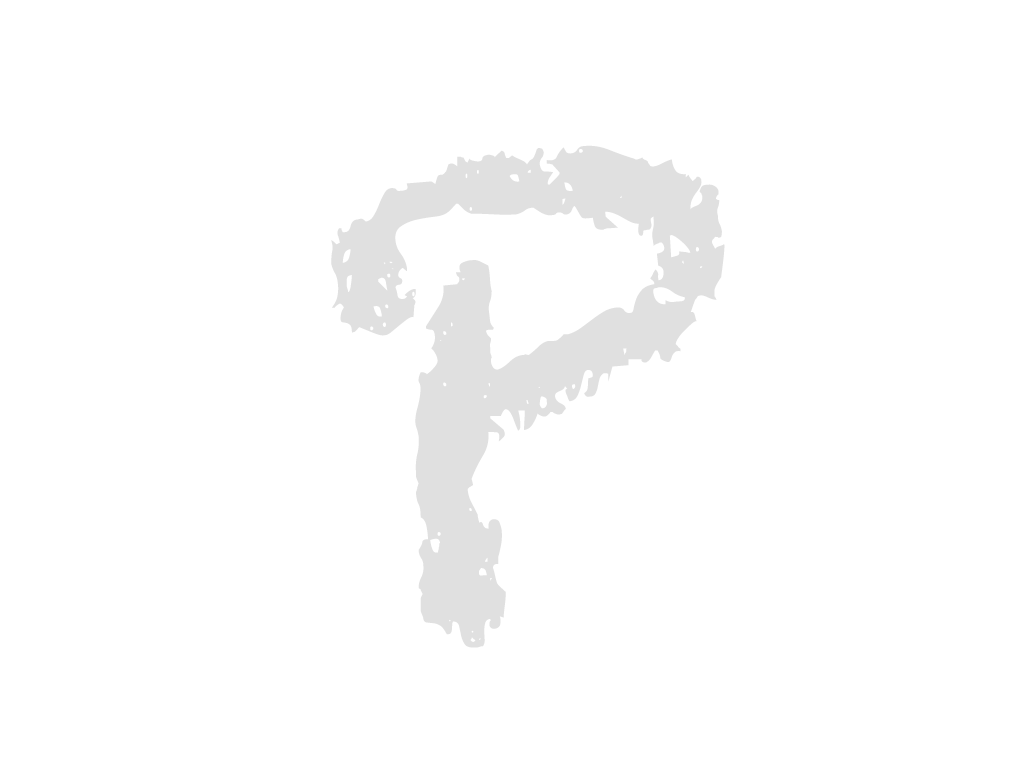
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="title-box flex justify-between mb40">
<p class="title">지문 등록</p>
</div>
<div class="board-wrap">
<div class="flex align-center mb20">
<label for="" class="title2">제목</label>
<input type="text" class="data-wrap" v-model="newData.textTtl">
</div>
<div class="flex align-center mb20">
<label for="" class="title2">URL</label>
<input type="text" class="data-wrap" v-model="newData.textUrl">
</div>
<div class="flex align-center">
<label class="title2">형식</label>
<label class="title2">
<input type="radio" v-model="newData.textTypeId" value="1" class="data-wrap"> 일반
</label>
<label class="title2">
<input type="radio" v-model="newData.textTypeId" value="2" class="data-wrap"> 대화
</label>
<label class="title2">
<input type="radio" v-model="newData.textTypeId" value="3" class="data-wrap"> 책 리스닝
</label>
<select name="" id="" v-model="newData.bookId" @change="fetchUnits" class="ml20">
<option value="" disabled>교재를 선택하세요</option>
<option v-for="book in books" :key="book.book_id" :value="book.book_id">
{{ book.book_nm }}
</option>
</select>
<select name="" id="" v-model="newData.unitId" class="ml20">
<option value="" disabled>단원을 선택하세요</option>
<option v-for="unit in units" :key="unit.unitId" :value="unit.unitId">
{{ unit.unitName }}
</option>
</select>
</div>
<hr>
<div class="flex align-center">
<label for="" class="title2">스크립트</label>
<textarea name="" id="" class="data-wrap" v-model="newData.textCnt"></textarea>
</div>
<hr>
<div class="flex align-center ">
<label for="file" class="title2">첨부파일</label>
<input type="file" ref="fileInput" @change="handleFileUpload" multiple />
</div>
</div>
<div class="flex justify-between mt50">
<button type="button" title="글쓰기" class="new-btn" @click="goToPage('TextList')">
목록
</button>
<div class="flex">
<button type="button" title="글쓰기" class="new-btn mr10" @click="handleButtonAction">
작성
</button>
</div>
</div>
</template>
<script>
import axios from "axios";
import SvgIcon from '@jamescoyle/vue-icon';
import { mdiMagnify } from '@mdi/js';
import store from '../AppStore';
export default {
computed: {
textId() {
return this.$route.query.textId;
}
},
data() {
return {
mdiMagnify: mdiMagnify,
post: null,
newData: {
textId: "",
textTtl: "",
textCnt: "",
textUrl: "",
textTypeId: "",
fileMngId: "",
userId: "",
bookId: "",
unitId: ""
},
books: [],
units: [],
file: "",
selectedFiles: [],
}
},
methods: {
goToPage(page) {
this.$router.push({ name: page });
},
handleFileUpload(e) {
this.selectedFiles = e.target.files;
},
fetchPostDetail() {
const textId = this.$route.query.textId;
axios({
url: `/text/selectOneText.json`,
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: { "textId": textId }
})
.then(response => {
if (response.data && response.data[0]) {
this.post = response.data[0];
this.editTitle = this.post.title;
this.editContent = this.post.content;
console.log(this.post);
} else {
this.error = "Failed to fetch post details.";
}
})
.catch(error => {
console.error("Error fetching post detail:", error);
this.error = "Failed to fetch post details.";
});
},
async dataInsert() {
const vm = this;
if (this.selectedFiles && this.selectedFiles.length > 0) {
// 파일 업로드
const formData = new FormData();
for (let i = 0; i < this.selectedFiles.length; i++) {
formData.append("files", this.selectedFiles[i]);
}
const fileUploadResponse = await axios.post(
"/file/upload.json",
formData,
{
headers: {
"Content-Type": "multipart/form-data",
},
}
);
// 업로드 후 파일 매니지 아이디 호출
vm.newData.fileMngId = fileUploadResponse.data.fileMngId;
}
axios({
url: "/text/insertText.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: vm.newData,
})
.then(response => {
alert(response.data.message);
this.goToPage('TextList')
})
.catch(error => {
console.log("dataInsert - error : ", error.response.data);
alert("게시글 등록에 오류가 발생했습니다.");
});
},
handleButtonAction() {
if (!this.newData.textTtl) {
alert("제목을 입력해 주세요.");
return;
}
if (!this.newData.textUrl) {
alert("url을 입력해 주세요.");
return;
}
if (!this.newData.textTypeId) {
alert("지문 형식을 입력해 주세요.");
return;
}
if (!this.newData.bookId) {
alert("교재를 선택해 주세요.");
return;
}
if (!this.newData.unitId) {
alert("단원을 선택해 주세요.");
return;
}
if (!this.newData.textCnt) {
alert("내용을 입력해 주세요.");
return;
}
this.dataInsert();
},
fetchBooks() {
axios({
url: "/book/findAll.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
})
.then(response => {
console.log(response.data)
this.books = response.data;
})
.catch(error => {
console.error("fetchBooks - error: ", error);
alert("교재 목록을 불러오는 중 오류가 발생했습니다.");
});
},
fetchUnits() {
if (!this.newData.bookId) return;
axios({
url: "/unit/unitList.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
"bookId": this.newData.bookId
},
})
.then(response => {
console.log(response.data)
this.units = response.data;
})
.catch(error => {
console.error("fetchUnits - error: ", error);
alert("단원 목록을 불러오는 중 오류가 발생했습니다.");
});
},
},
watch: {
},
computed: {
},
components: {
SvgIcon
},
mounted() {
this.fetchPostDetail();
this.newData.bookId = this.$route.query.book_id;
this.newData.unitId = this.$route.query.unit_id;
this.newData.userId = store.getters.getUserInfo.userId;
this.fetchBooks();
this.fetchUnits();
console.log('Main2 mounted');
}
}
</script>