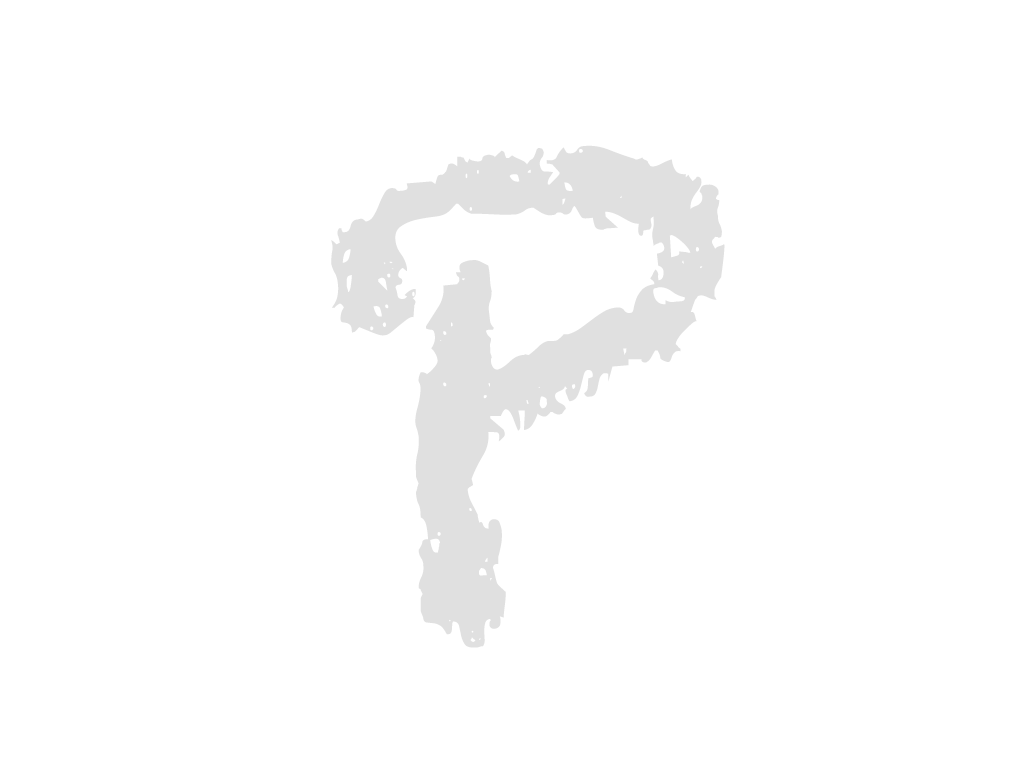
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
2023-09-26
import pandas as pd
def check_missing(df):
# Convert the '관측시각' column to a datetime object
# Also, doing copy() to prevent pass by reference in which is not intended.
df_copy = df.copy()
df_copy['관측시각'] = pd.to_datetime(df_copy['관측시각'], format='%Y%m%d%H%M')
# Calculate the difference between each row and its subsequent row
df_copy['time_diff'] = df_copy['관측시각'].diff()
# Check for differences that aren't 1 hour, excluding the first row
errors = df_copy[df_copy['time_diff'] != pd.Timedelta(hours=1)][1:]
if not errors.empty:
print("Errors found:")
print(errors[['관측시각', 'time_diff']])
return False
else:
print("All time differences are correct.")
return True
if __name__ == "__main__":
file = ".csv"
check_missing(file)