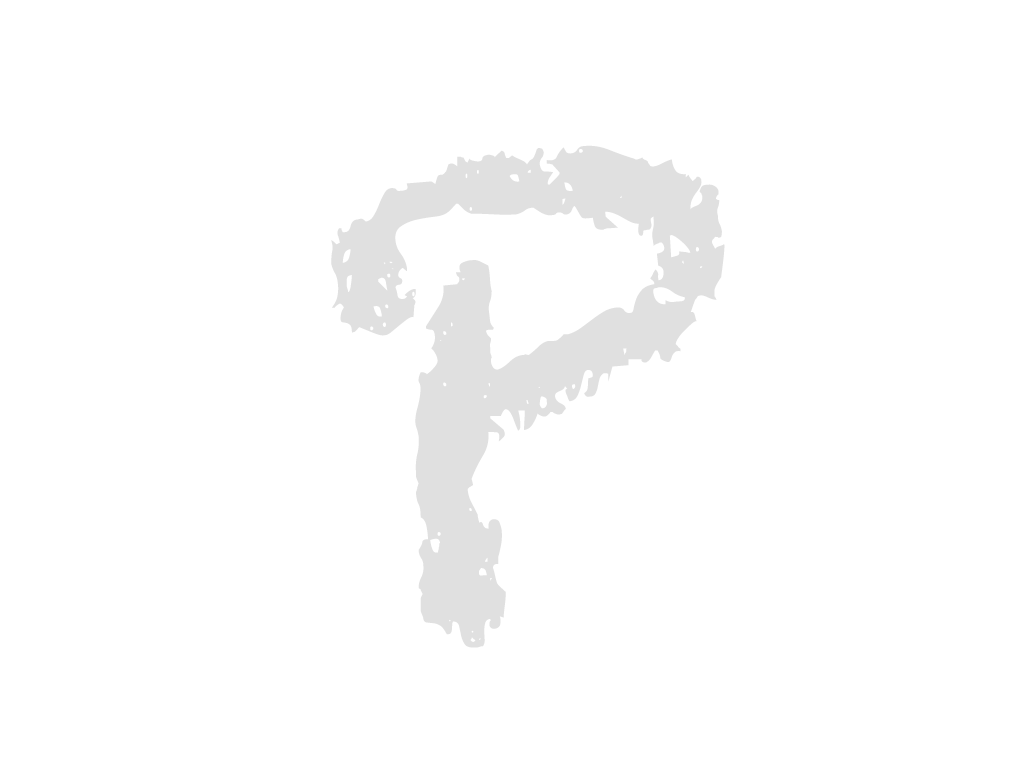
--- action.py
+++ action.py
... | ... | @@ -1,14 +1,41 @@ |
1 | 1 |
import sched |
2 |
- |
|
2 |
+import psycopg2 |
|
3 | 3 |
from flask_restx import Resource, Api, Namespace, fields |
4 | 4 |
from flask import request |
5 |
-from flask import Flask, render_template, request |
|
5 |
+from flask import Flask, render_template, request, jsonify, Response |
|
6 |
+from statsmodels.tsa.statespace.sarimax import SARIMAX |
|
7 |
+from datetime import datetime, timedelta |
|
6 | 8 |
import pandas as pd |
9 |
+import numpy as np |
|
10 |
+import pickle |
|
11 |
+import time |
|
7 | 12 |
|
8 | 13 |
Action = Namespace( |
9 | 14 |
name="Action", |
10 | 15 |
description="노드 분석을 위해 사용하는 api.", |
11 | 16 |
) |
17 |
+ |
|
18 |
+db_config = { |
|
19 |
+ 'dbname': 'welding', |
|
20 |
+ 'user': 'postgres', |
|
21 |
+ 'password': 'ts4430!@', |
|
22 |
+ 'host': 'localhost', # e.g., 'localhost' |
|
23 |
+ 'port': '5432', # e.g., '5432' |
|
24 |
+} |
|
25 |
+ |
|
26 |
+key_columns = ["temperature", "relative_humidity", "absolute_humidity"] |
|
27 |
+ |
|
28 |
+def buck_equation(temperature): # temp in Celsius |
|
29 |
+ saturation_vapor_pressure = 0.61121 * np.exp((18.678 - temperature / 234.5) * (temperature / (257.14 + temperature))) |
|
30 |
+ return saturation_vapor_pressure * 1000 # KPa -> Pa |
|
31 |
+ |
|
32 |
+def absolute_humidity(relative_humidity, temperature): |
|
33 |
+ relative_humidity = np.array(relative_humidity) |
|
34 |
+ temperature = np.array(temperature) |
|
35 |
+ saturation_vapor_pressure = buck_equation(temperature) |
|
36 |
+ # 461.5/Kg Kelvin is specific gas constant |
|
37 |
+ return saturation_vapor_pressure * relative_humidity * 0.01 /(461.5 * (temperature + 273.15)) # g/m^3 |
|
38 |
+ |
|
12 | 39 |
# @sched.scheduler |
13 | 40 |
# def weather_update |
14 | 41 |
|
... | ... | @@ -31,4 +58,94 @@ |
31 | 58 |
else: |
32 | 59 |
return { |
33 | 60 |
'report': "safe" |
34 |
- }, 200(파일 끝에 줄바꿈 문자 없음) |
|
61 |
+ }, 200 |
|
62 |
+ |
|
63 |
[email protected]('/train_sarima', methods=['POST']) |
|
64 |
+class TrainSARIMA(Resource): |
|
65 |
+ @Action.doc(responses={200: 'Success'}) |
|
66 |
+ @Action.doc(responses={500: 'Register Failed'}) |
|
67 |
+ def post(self): |
|
68 |
+ query = "SELECT * FROM weather_data ORDER BY time DESC LIMIT 600" |
|
69 |
+ |
|
70 |
+ try: |
|
71 |
+ view_past = int(request.form.get('past_data_for_prediction',72)) |
|
72 |
+ future_hours = int(request.form.get('future_hours', 24)) |
|
73 |
+ save_name = request.form.get('save_name', 'prediction') |
|
74 |
+ |
|
75 |
+ with psycopg2.connect(**db_config) as conn: |
|
76 |
+ df = pd.read_sql_query(query, conn) |
|
77 |
+ df_sarima = df.iloc[:view_past] |
|
78 |
+ df_sarima = df_sarima.iloc[::-1].reset_index(drop=True) |
|
79 |
+ df = df.iloc[::-1].reset_index(drop=True) |
|
80 |
+ |
|
81 |
+ return_index = [df_sarima['time'].iloc[-1] + timedelta(hours=i) for i in range(1, future_hours + 1)] |
|
82 |
+ forecast_return = pd.DataFrame(None, columns=["time", "temperature", "relative_humidity", "absolute_humidity"]) |
|
83 |
+ forecast_return['time'] = return_index |
|
84 |
+ seasonal_order = { |
|
85 |
+ f"{key_columns[0]}": (0, 1, 1, 24), |
|
86 |
+ f"{key_columns[1]}": (0, 1, 1, 24), |
|
87 |
+ f"{key_columns[2]}": (0, 1, 1, 24), |
|
88 |
+ } |
|
89 |
+ t1 = time.time() |
|
90 |
+ for col_key in key_columns: |
|
91 |
+ |
|
92 |
+ model = SARIMAX(df_sarima[col_key], order=(1, 0, 2), seasonal_order=seasonal_order[col_key]) |
|
93 |
+ model_fit = model.fit(disp=False) |
|
94 |
+ |
|
95 |
+ |
|
96 |
+ forecast_values = model_fit.forecast(steps=future_hours) |
|
97 |
+ forecast_return[col_key] = forecast_values.values |
|
98 |
+ # with open(f'predictions/sarima_model_{save_name}_{col_key}.pkl', 'wb') as pkl_file: |
|
99 |
+ # pickle.dump(model_fit, pkl_file) |
|
100 |
+ t2 = -(t1 - time.time()) |
|
101 |
+ print(f"{t2} seconds per {future_hours*3}\n" |
|
102 |
+ f"that is {future_hours*3 / t2} per seconds") |
|
103 |
+ return Response((pd.concat((df, forecast_return)).reset_index(drop=True)).to_json(orient='columns'), mimetype='application/json') |
|
104 |
+ |
|
105 |
+ except Exception as e: |
|
106 |
+ return jsonify({"error": str(e)}), 500 |
|
107 |
+ |
|
108 |
+ |
|
109 |
+def forecast_from_saved_model(df, trained_weight="predictions" , future_hours=24): |
|
110 |
+ # Load the saved model |
|
111 |
+ forecast_df = None |
|
112 |
+ |
|
113 |
+ for key_col in key_columns: |
|
114 |
+ with open(trained_weight, 'rb') as pkl_file: |
|
115 |
+ loaded_model = pickle.load(pkl_file) |
|
116 |
+ |
|
117 |
+ print("files loaded") |
|
118 |
+ t1 = time.time() |
|
119 |
+ # Forecast the next 'future_hours' using the loaded model |
|
120 |
+ forecast_values = loaded_model.forecast(steps=future_hours) |
|
121 |
+ forecast_index = [df['time'].iloc[-1] + timedelta(hours=i) for i in range(1, future_hours + 1)] |
|
122 |
+ forecast_df = pd.DataFrame({ |
|
123 |
+ 'time': forecast_index, |
|
124 |
+ 'forecast': forecast_values |
|
125 |
+ }) |
|
126 |
+ |
|
127 |
+ # forecast_df.to_csv(f"{file.split('.')[0]}_forecast.csv", index=False) |
|
128 |
+ t2 = -(t1 - time.time()) |
|
129 |
+ # print(forecast_df) |
|
130 |
+ print(f"{t2} seconds per {future_hours}\n" |
|
131 |
+ f"that is {future_hours/t2} per seconds") |
|
132 |
+ return forecast_df |
|
133 |
+ |
|
134 |
[email protected]('/fetch_sensor') |
|
135 |
+class FetchSensorData(Resource): |
|
136 |
+ @Action.doc(responses={200: 'Success', 500: 'Failed'}) |
|
137 |
+ def get(self): |
|
138 |
+ conn_params = db_config # Define or fetch your connection parameters here |
|
139 |
+ query = "SELECT * FROM weather_data ORDER BY time DESC LIMIT 600" |
|
140 |
+ try: |
|
141 |
+ with psycopg2.connect(**conn_params) as conn: |
|
142 |
+ df = pd.read_sql_query(query, conn) |
|
143 |
+ # predictions |
|
144 |
+ # Convert Timestamp columns to string |
|
145 |
+ for column in df.columns: |
|
146 |
+ if df[column].dtype == "datetime64[ns]": |
|
147 |
+ df[column] = df[column].astype(str) |
|
148 |
+ |
|
149 |
+ return df.to_dict(orient='list'), 200 |
|
150 |
+ except Exception as e: |
|
151 |
+ return {"message": str(e)}, 500(파일 끝에 줄바꿈 문자 없음) |
--- app.py
+++ app.py
... | ... | @@ -17,25 +17,23 @@ |
17 | 17 |
description="API Server", |
18 | 18 |
terms_url="/", |
19 | 19 |
contact="[email protected]", |
20 |
- license="MIT") |
|
20 |
+ ) |
|
21 | 21 |
|
22 | 22 |
|
23 | 23 |
scheduler = BackgroundScheduler() |
24 | 24 |
scheduler.start() |
25 | 25 |
today = datetime.today().strftime('%Y-%m-%d') |
26 |
-# Schedule task_function to be called every 6 hours |
|
26 |
+# Schedule task_function to be called every 1 hour |
|
27 | 27 |
scheduler.add_job( |
28 | 28 |
func=update_weather_info_to_today, |
29 |
- trigger=IntervalTrigger(hours=6), |
|
30 |
- args=("data/weather/weather_data.csv",), |
|
31 |
- # comma to make it a tuple, so that python won't confuse this as a list of char |
|
29 |
+ trigger=IntervalTrigger(hours=1), |
|
32 | 30 |
id='weather_data_update', |
33 | 31 |
name='update weather time every 6 hours', |
34 | 32 |
replace_existing=True |
35 | 33 |
) |
36 | 34 |
scheduler.add_job( |
37 | 35 |
func=sarima, |
38 |
- trigger=IntervalTrigger(hours=6), |
|
36 |
+ trigger=IntervalTrigger(hours=1), |
|
39 | 37 |
args=("data/weather/weather_data.csv", f"{today}"), |
40 | 38 |
# comma to make it a tuple, so that python won't confuse this as a list of char |
41 | 39 |
id='weather_data_update', |
... | ... | @@ -44,8 +42,8 @@ |
44 | 42 |
) |
45 | 43 |
|
46 | 44 |
api.add_namespace(Action, '/action') |
47 |
-update_weather_info_to_today("data/weather/weather_data.csv") |
|
48 |
-sarima("data/weather/weather_data.csv",f"{today}") |
|
45 |
+# update_weather_info_to_today("data/weather/weather_data.csv") |
|
46 |
+# sarima("data/weather/weather_data.csv",f"{today}") |
|
49 | 47 |
|
50 | 48 |
api.add_namespace(Auth, '/auth') |
51 | 49 |
print("Api Add Auth") |
--- data/weather/weather_data.csv
+++ data/weather/weather_data.csv
... | ... | @@ -28345,532 +28345,606 @@ |
28345 | 28345 |
202309242300,281,29,1.4,-9,-9.0,-9,1007.9,1019.1,-9,-9.0,18.4,15.0,81.0,17.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,18,-,-9,-9,-9,5000,-9.0,-9.0,-9,17.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
28346 | 28346 |
202309250000,281,32,2.2,-9,-9.0,-9,1008.0,1019.3,5,-0.4,18.2,14.8,81.0,16.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,6,18,-,-9,-9,-9,5000,-9.0,-9.0,-9,17.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
28347 | 28347 |
202309250100,281,27,0.5,-9,-9.0,-9,1007.4,1018.6,-9,-9.0,18.4,15.0,81.0,17.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,13,-,-9,-9,-9,5000,-9.0,-9.0,-9,17.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
28348 |
-202309250200,281,32,1.9,-9,-9.0,-9,1007.1,1018.4,-9,-9.0,17.9,14.9,83.0,16.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,29,-,-9,-9,-9,5000,-9.0,-9.00,-9,17.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28349 |
-202309250300,281,29,1.0,-9,-9.0,-9,1006.6,1017.9,7,-1.4,17.7,15.1,85.0,17.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,14,-,-9,-9,-9,5000,-9.0,-9.00,-9,17.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28350 |
-202309250400,281,29,1.4,-9,-9.0,-9,1006.5,1017.8,-9,-9.0,17.6,15.2,86.0,17.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,4,11,-,-9,-9,-9,5000,-9.0,-9.00,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28351 |
-202309250500,281,29,1.4,-9,-9.0,-9,1006.5,1017.8,-9,-9.0,17.8,14.6,82.0,16.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,10,-,-9,-9,-9,5000,-9.0,-9.00,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28352 |
-202309250600,281,27,1.2,-9,-9.0,-9,1006.9,1018.2,3,0.3,17.6,14.8,84.0,16.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,11,-,-9,-9,-9,5000,-9.0,-9.00,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28353 |
-202309250700,281,0,0.0,-9,-9.0,-9,1007.1,1018.4,-9,-9.0,17.8,15.6,87.0,17.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,13,-,-9,-9,-9,4979,0.0,-9.00,-9,18.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28354 |
-202309250800,281,32,0.8,-9,-9.0,-9,1007.2,1018.4,-9,-9.0,18.3,15.1,82.0,17.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,33,-,-9,-9,-9,5000,0.0,-9.00,-9,19.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28355 |
-202309250900,281,0,0.2,-9,-9.0,-9,1007.0,1018.2,4,0.0,19.4,15.4,78.0,17.5,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,28,-,-9,-9,-9,4264,0.0,-9.00,-9,22.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28356 |
-202309251000,281,0,0.3,-9,-9.0,-9,1007.2,1018.3,-9,-9.0,21.8,15.8,69.0,17.9,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,9,-,-9,-9,-9,4880,0.1,-9.00,-9,29.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28357 |
-202309251100,281,5,0.9,-9,-9.0,-9,1006.6,1017.6,-9,-9.0,22.9,16.4,67.0,18.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,9,-,-9,-9,-9,4890,0.1,-9.00,-9,29.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28358 |
-202309251200,281,7,2.7,-9,-9.0,-9,1006.3,1017.3,8,-0.9,23.4,17.1,68.0,19.5,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,9,-,-9,-9,-9,5000,0.0,-9.00,-9,27.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28359 |
-202309251300,281,9,2.7,-9,-9.0,-9,1005.4,1016.4,-9,-9.0,23.8,17.2,67.0,19.6,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,8,-,-9,-9,-9,5000,0.1,-9.00,-9,31.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28360 |
-202309251400,281,11,2.1,-9,-9.0,-9,1005.1,1016.1,-9,-9.0,23.4,17.8,71.0,20.4,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,8,-,-9,-9,-9,5000,0.0,-9.00,-9,27.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28361 |
-202309251500,281,11,2.4,-9,-9.0,-9,1004.6,1015.5,7,-1.8,24.5,17.7,66.0,20.2,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,12,-,-9,-9,-9,4924,0.2,-9.00,-9,35.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28362 |
-202309251600,281,9,2.0,-9,-9.0,-9,1004.5,1015.5,-9,-9.0,23.4,17.3,69.0,19.7,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,12,-,-9,-9,-9,5000,0.2,-9.00,-9,26.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28363 |
-202309251700,281,9,2.1,-9,-9.0,-9,1004.5,1015.5,-9,-9.0,23.0,17.6,72.0,20.1,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,8,-,-9,-9,-9,5000,0.0,-9.00,-9,24.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28364 |
-202309251800,281,11,1.6,-9,-9.0,-9,1004.7,1015.8,2,0.3,22.4,18.1,77.0,20.8,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,1,13,-,-9,-9,-9,4970,0.0,-9.00,-9,22.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28365 |
-202309251900,281,11,0.9,-9,-9.0,-9,1004.8,1015.9,-9,-9.0,21.5,18.0,81.0,20.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,3,14,-,-9,-9,-9,3136,0.0,-9.00,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28366 |
-202309252000,281,9,0.5,-9,-9.0,-9,1005.2,1016.3,-9,-9.0,21.1,17.8,82.0,20.4,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,12,-,-9,-9,-9,4857,-9.0,-9.00,-9,20.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28367 |
-202309252100,281,0,0.1,-9,-9.0,-9,1005.6,1016.8,2,1.0,20.3,18.5,90.0,21.3,0.1,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,22,-,-9,-9,-9,1045,-9.0,-9.00,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28368 |
-202309252200,281,0,0.1,-9,-9.0,-9,1005.6,1016.8,-9,-9.0,20.1,19.2,95.0,22.2,0.4,0.5,0.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,22,-,-9,-9,-9,793,-9.0,-9.00,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28369 |
-202309252300,281,0,0.2,-9,-9.0,-9,1005.4,1016.5,-9,-9.0,19.7,19.5,99.0,22.7,0.4,0.9,0.9,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,11,-,-9,-9,-9,2458,-9.0,-9.00,-9,20.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28370 |
-202309260000,281,0,0.4,-9,-9.0,-9,1004.7,1015.8,7,-1.0,19.5,19.4,100.0,22.5,0.6,1.5,1.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,12,-,-9,-9,-9,1228,-9.0,-9.00,-9,20.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28371 |
-202309260100,281,27,0.8,-9,-9.0,-9,1004.3,1015.4,-9,-9.0,19.4,19.3,100.0,22.4,0.1,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,4,-,-9,-9,-9,1772,-9.0,-9.00,-9,20.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28372 |
-202309260200,281,0,0.3,-9,-9.0,-9,1003.9,1015.0,-9,-9.0,19.3,19.2,100.0,22.2,-9.0,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,23,-,-9,-9,-9,2173,-9.0,-9.00,-9,20.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28373 |
-202309260300,281,0,0.3,-9,-9.0,-9,1003.7,1014.8,7,-1.0,19.5,19.4,100.0,22.5,-9.0,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,10,-,-9,-9,-9,2389,-9.0,-9.00,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28374 |
-202309260400,281,0,0.0,-9,-9.0,-9,1003.2,1014.3,-9,-9.0,19.5,19.4,100.0,22.5,-9.0,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,6,-,-9,-9,-9,2634,-9.0,-9.00,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28375 |
-202309260500,281,0,0.3,-9,-9.0,-9,1003.2,1014.3,-9,-9.0,19.6,19.4,99.0,22.5,0.1,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,4,-,-9,-9,-9,2164,-9.0,-9.00,-9,20.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28376 |
-202309260600,281,0,0.3,-9,-9.0,-9,1003.5,1014.6,5,-0.2,19.5,19.4,100.0,22.5,1.1,1.3,1.3,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,4,-,-9,-9,-9,1192,-9.0,-9.00,-9,20.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28377 |
-202309260700,281,27,0.6,-9,-9.0,-9,1004.3,1015.4,-9,-9.0,19.5,19.4,100.0,22.5,4.8,6.1,6.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,8,-,-9,-9,-9,175,0.0,-9.00,-9,19.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28378 |
-202309260800,281,5,0.9,-9,-9.0,-9,1004.3,1015.4,-9,-9.0,19.7,19.6,100.0,22.8,7.1,13.2,13.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,42,-,-9,-9,-9,549,0.0,-9.00,-9,20.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28379 |
-202309260900,281,5,1.1,-9,-9.0,-9,1004.4,1015.6,2,1.0,20.0,19.9,100.0,23.2,3.1,16.3,16.3,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,1,-,-9,-9,-9,474,0.0,-9.00,-9,21.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28380 |
-202309261000,281,7,1.0,-9,-9.0,-9,1004.1,1015.3,-9,-9.0,20.1,20.0,100.0,23.4,2.7,19.0,19.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,-9,-,-9,-9,-9,1687,0.0,-9.00,-9,22.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28381 |
-202309261100,281,9,0.9,-9,-9.0,-9,1003.5,1014.6,-9,-9.0,20.8,20.7,100.0,24.4,0.4,19.4,19.4,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,3,-,-9,-9,-9,1717,0.0,-9.00,-9,23.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28382 |
-202309261200,281,11,0.9,-9,-9.0,-9,1003.3,1014.4,7,-1.2,21.0,20.6,98.0,24.3,0.3,19.7,19.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,4,-,-9,-9,-9,4694,0.0,-9.00,-9,22.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28383 |
-202309261300,281,9,1.4,-9,-9.0,-9,1002.7,1013.8,-9,-9.0,20.7,20.2,97.0,23.7,0.1,19.8,19.8,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,6,-,-9,-9,-9,4207,0.0,-9.00,-9,22.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28384 |
-202309261400,281,7,1.4,-9,-9.0,-9,1002.0,1013.1,-9,-9.0,20.7,20.5,99.0,24.1,0.2,20.0,20.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,18,-,-9,-9,-9,3138,0.0,-9.00,-9,22.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28385 |
-202309261500,281,9,1.0,-9,-9.0,-9,1001.7,1012.8,7,-1.6,20.7,20.5,99.0,24.1,0.2,20.2,20.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,4,-,-9,-9,-9,836,0.0,-9.00,-9,21.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28386 |
-202309261600,281,7,1.3,-9,-9.0,-9,1001.5,1012.6,-9,-9.0,20.6,20.5,100.0,24.1,0.5,20.7,20.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,5,-,-9,-9,-9,1328,0.0,-9.00,-9,22.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28387 |
-202309261700,281,9,0.8,-9,-9.0,-9,1001.4,1012.5,-9,-9.0,20.6,20.5,100.0,24.1,0.1,20.8,20.8,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,-9,-,-9,-9,-9,1334,0.0,-9.00,-9,21.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28388 |
-202309261800,281,7,1.2,-9,-9.0,-9,1001.6,1012.7,5,-0.1,20.5,20.4,100.0,24.0,0.3,21.1,21.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,582,0.0,-9.00,-9,21.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28389 |
-202309261900,281,7,0.9,-9,-9.0,-9,1001.5,1012.6,-9,-9.0,20.5,20.4,100.0,24.0,0.5,21.6,21.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,4,5,-,-9,-9,-9,764,0.0,-9.00,-9,21.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28390 |
-202309262000,281,36,0.6,-9,-9.0,-9,1001.7,1012.8,-9,-9.0,20.6,20.5,100.0,24.1,0.4,22.0,22.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,-9,-,-9,-9,-9,294,-9.0,-9.00,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28391 |
-202309262100,281,0,0.4,-9,-9.0,-9,1001.9,1013.0,3,0.3,20.5,20.4,100.0,24.0,1.0,23.0,23.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,1,-,-9,-9,-9,487,-9.0,-9.00,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28392 |
-202309262200,281,34,0.8,-9,-9.0,-9,1002.0,1013.1,-9,-9.0,20.5,20.4,100.0,24.0,0.5,23.5,23.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,3,-,-9,-9,-9,1381,-9.0,-9.00,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28393 |
-202309262300,281,29,0.5,-9,-9.0,-9,1002.0,1013.1,-9,-9.0,20.6,20.5,100.0,24.1,-9.0,23.5,23.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,3,-,-9,-9,-9,1125,-9.0,-9.00,-9,21.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28394 |
-202309270000,281,0,0.3,-9,-9.0,-9,1001.5,1012.6,8,-0.4,20.6,20.5,100.0,24.1,-9.0,23.5,23.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,3,-,-9,-9,-9,892,-9.0,-9.00,-9,21.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28395 |
-202309270100,281,0,0.0,-9,-9.0,-9,1001.0,1012.1,-9,-9.0,20.4,20.3,100.0,23.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,1,-,-9,-9,-9,727,-9.0,-9.00,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28396 |
-202309270200,281,27,1.1,-9,-9.0,-9,1001.0,1012.1,-9,-9.0,20.5,20.4,100.0,24.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,4,-,-9,-9,-9,515,-9.0,-9.00,-9,20.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28397 |
-202309270300,281,0,0.2,-9,-9.0,-9,1000.7,1011.8,7,-0.8,20.3,20.2,100.0,23.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,5,3,-,-9,-9,-9,690,-9.0,-9.00,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28398 |
-202309270400,281,25,0.7,-9,-9.0,-9,1000.6,1011.7,-9,-9.0,20.3,20.2,100.0,23.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,1,-,-9,-9,-9,712,-9.0,-9.00,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28399 |
-202309270500,281,0,0.2,-9,-9.0,-9,1000.5,1011.6,-9,-9.0,20.3,20.2,100.0,23.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,-9,-,-9,-9,-9,432,-9.0,-9.00,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28400 |
-202309270600,281,0,0.1,-9,-9.0,-9,1000.7,1011.8,4,0.0,20.4,20.3,100.0,23.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,-9,-,-9,-9,-9,573,-9.0,-9.00,-9,20.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28401 |
-202309270700,281,0,0.3,-9,-9.0,-9,1001.1,1012.2,-9,-9.0,20.5,20.4,100.0,24.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,-9,-,-9,-9,-9,502,0.0,-9.00,-9,21.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28402 |
-202309270800,281,7,0.7,-9,-9.0,-9,1001.4,1012.5,-9,-9.0,20.8,20.7,100.0,24.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,-9,-,-9,-9,-9,636,0.0,-9.00,-9,22.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28403 |
-202309270900,281,0,0.4,-9,-9.0,-9,1001.5,1012.6,2,0.8,21.7,21.1,97.0,25.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,1,-,-9,-9,-9,735,0.0,-9.00,-9,24.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28404 |
-202309271000,281,20,0.9,-9,-9.0,-9,1001.3,1012.3,-9,-9.0,23.4,20.9,86.0,24.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,8,3,-,-9,-9,-9,1030,0.1,-9.00,-9,31.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28405 |
-202309271100,281,0,0.4,-9,-9.0,-9,1001.2,1012.1,-9,-9.0,23.9,20.8,83.0,24.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,4,-,-9,-9,-9,1441,0.0,-9.00,-9,31.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28406 |
-202309271200,281,32,1.4,-9,-9.0,-9,1000.6,1011.6,7,-1.0,25.0,21.3,80.0,25.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,8,-,-9,-9,-9,2235,0.2,-9.00,-9,30.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28407 |
-202309271300,281,29,1.5,-9,-9.0,-9,1000.1,1011.0,-9,-9.0,24.7,20.5,78.0,24.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,10,-,-9,-9,-9,3104,0.0,-9.00,-9,27.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28408 |
-202309271400,281,34,0.9,-9,-9.0,-9,999.3,1010.2,-9,-9.0,24.6,21.1,81.0,25.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,11,-,-9,-9,-9,2728,0.0,-9.00,-9,26.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28409 |
-202309271500,281,5,1.2,-9,-9.0,-9,998.9,1009.8,7,-1.8,24.9,20.7,78.0,24.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,17,-,-9,-9,-9,4413,0.0,-9.00,-9,29.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28410 |
-202309271600,281,32,0.7,-9,-9.0,-9,998.9,1009.8,-9,-9.0,25.3,20.7,76.0,24.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,4,11,-,-9,-9,-9,5000,0.0,-9.00,-9,27.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28411 |
-202309271700,281,29,2.1,-9,-9.0,-9,998.9,1009.8,-9,-9.0,24.8,20.0,75.0,23.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,13,-,-9,-9,-9,5000,0.1,-9.00,-9,25.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28412 |
-202309271800,281,29,2.0,-9,-9.0,-9,999.1,1010.0,2,0.2,23.7,20.0,80.0,23.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,10,-,-9,-9,-9,5000,0.1,-9.00,-9,23.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28413 |
-202309271900,281,32,2.0,-9,-9.0,-9,999.5,1010.4,-9,-9.0,23.4,18.9,76.0,21.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,13,-,-9,-9,-9,5000,0.0,-9.00,-9,22.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28414 |
-202309272000,281,29,2.2,-9,-9.0,-9,1000.1,1011.1,-9,-9.0,23.0,19.1,79.0,22.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,12,-,-9,-9,-9,4954,-9.0,-9.00,-9,21.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28415 |
-202309272100,281,27,1.3,-9,-9.0,-9,1000.8,1011.8,2,1.8,22.8,19.3,81.0,22.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,8,-,-9,-9,-9,5000,-9.0,-9.00,-9,21.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28416 |
-202309272200,281,27,1.6,-9,-9.0,-9,1001.1,1012.1,-9,-9.0,22.3,19.0,82.0,22.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,11,-,-9,-9,-9,5000,-9.0,-9.00,-9,21.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28417 |
-202309272300,281,29,1.3,-9,-9.0,-9,1001.1,1012.1,-9,-9.0,22.0,18.9,83.0,21.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,10,-,-9,-9,-9,5000,-9.0,-9.00,-9,21.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28418 |
-202309280000,281,27,1.8,-9,-9.0,-9,1001.0,1012.1,1,0.3,21.8,18.7,83.0,21.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,7,9,-,-9,-9,-9,4592,-9.0,-9.00,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28419 |
-202309280100,281,29,1.6,-9,-9.0,-9,1000.5,1011.6,-9,-9.0,21.3,18.4,84.0,21.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,5,9,-,-9,-9,-9,390,-9.0,-9.00,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28420 |
-202309280200,281,27,1.7,-9,-9.0,-9,1000.5,1011.6,-9,-9.0,21.1,19.0,88.0,22.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,8,-,-9,-9,-9,4489,-9.0,-9.00,-9,20.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28421 |
-202309280300,281,27,2.4,-9,-9.0,-9,1000.4,1011.5,7,-0.6,20.8,18.9,89.0,21.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,11,-,-9,-9,-9,3795,-9.0,-9.00,-9,19.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28422 |
-202309280400,281,27,1.8,-9,-9.0,-9,1000.9,1012.0,-9,-9.0,21.0,18.7,87.0,21.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,9,-,-9,-9,-9,4053,-9.0,-9.00,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28423 |
-202309280500,281,25,1.5,-9,-9.0,-9,1001.0,1012.1,-9,-9.0,20.7,18.4,87.0,21.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,6,14,-,-9,-9,-9,3734,-9.0,-9.00,-9,19.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28424 |
-202309280600,281,27,1.9,-9,-9.0,-9,1001.4,1012.6,2,1.1,20.2,18.3,89.0,21.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,15,-,-9,-9,-9,2786,-9.0,-9.00,-9,19.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28425 |
-202309280700,281,29,1.2,-9,-9.0,-9,1002.5,1013.6,-9,-9.0,20.8,18.3,86.0,21.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,4,27,-,-9,-9,-9,2989,0.4,-9.00,-9,19.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28426 |
-202309280800,281,27,2.1,-9,-9.0,-9,1002.9,1014.0,-9,-9.0,22.1,18.2,79.0,20.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,20,-,-9,-9,-9,3305,0.6,-9.00,-9,23.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28427 |
-202309280900,281,29,2.0,-9,-9.0,-9,1003.3,1014.3,2,1.7,23.4,18.0,72.0,20.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,8,-,-9,-9,-9,3442,0.8,-9.00,-9,27.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28428 |
-202309281000,281,27,2.6,-9,-9.0,-9,1003.4,1014.4,-9,-9.0,24.9,18.1,66.0,20.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,11,-,-9,-9,-9,4616,0.7,-9.00,-9,32.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28429 |
-202309281100,281,29,3.0,-9,-9.0,-9,1002.9,1013.9,-9,-9.0,25.5,18.4,65.0,21.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,11,-,-9,-9,-9,4665,0.8,-9.00,-9,34.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28430 |
-202309281200,281,29,3.2,-9,-9.0,-9,1002.6,1013.5,8,-0.8,25.8,17.7,61.0,20.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,11,-,-9,-9,-9,4804,0.8,-9.00,-9,33.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28431 |
-202309281300,281,27,2.8,-9,-9.0,-9,1002.0,1012.8,-9,-9.0,27.7,18.1,56.0,20.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,2,11,-,-9,-9,-9,4901,0.7,-9.00,-9,38.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28432 |
-202309281400,281,27,3.1,-9,-9.0,-9,1001.3,1012.1,-9,-9.0,28.0,17.8,54.0,20.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,2,12,-,-9,-9,-9,4609,1.0,-9.00,-9,37.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28433 |
-202309281500,281,27,3.9,-9,-9.0,-9,1000.9,1011.7,7,-1.8,27.3,16.5,52.0,18.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4193,1.0,-9.00,-9,33.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28434 |
-202309281600,281,29,3.3,-9,-9.0,-9,1001.2,1012.1,-9,-9.0,27.1,16.9,54.0,19.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3986,1.0,-9.00,-9,30.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28435 |
-202309281700,281,27,2.7,-9,-9.0,-9,1001.5,1012.4,-9,-9.0,26.3,16.8,56.0,19.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3555,1.0,-9.00,-9,26.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28436 |
-202309281800,281,29,1.8,-9,-9.0,-9,1001.6,1012.5,2,0.8,24.4,17.1,64.0,19.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2799,0.4,-9.00,-9,22.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28437 |
-202309281900,281,29,1.9,-9,-9.0,-9,1001.8,1012.8,-9,-9.0,23.3,16.8,67.0,19.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,2244,0.0,-9.00,-9,20.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28438 |
-202309282000,281,32,1.4,-9,-9.0,-9,1002.5,1013.6,-9,-9.0,21.3,17.0,77.0,19.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1813,-9.0,-9.00,-9,19.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28439 |
-202309282100,281,7,0.8,-9,-9.0,-9,1003.0,1014.1,2,1.6,19.5,18.1,92.0,20.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1056,-9.0,-9.00,-9,18.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28440 |
-202309282200,281,0,0.2,-9,-9.0,-9,1003.3,1014.5,-9,-9.0,18.6,18.1,97.0,20.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,692,-9.0,-9.00,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28441 |
-202309282300,281,34,0.7,-9,-9.0,-9,1003.5,1014.7,-9,-9.0,18.0,17.6,98.0,20.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,601,-9.0,-9.00,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28442 |
-202309290000,281,0,0.2,-9,-9.0,-9,1003.6,1014.9,2,0.8,17.5,17.3,99.0,19.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,538,-9.0,-9.00,-9,17.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28443 |
-202309290100,281,0,0.4,-9,-9.0,-9,1003.4,1014.7,-9,-9.0,17.1,17.0,100.0,19.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,439,-9.0,-9.00,-9,16.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28444 |
-202309290200,281,27,1.1,-9,-9.0,-9,1003.5,1014.7,-9,-9.0,17.7,17.3,98.0,19.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,529,-9.0,-9.00,-9,16.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28445 |
-202309290300,281,0,0.2,-9,-9.0,-9,1003.4,1014.7,6,-0.2,17.3,17.2,100.0,19.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,483,-9.0,-9.00,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28446 |
-202309290400,281,23,0.8,-9,-9.0,-9,1003.8,1015.1,-9,-9.0,16.6,16.2,98.0,18.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,502,-9.0,-9.00,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28447 |
-202309290500,281,29,0.5,-9,-9.0,-9,1004.4,1015.8,-9,-9.0,15.9,15.8,100.0,17.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,330,-9.0,-9.00,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28448 |
-202309290600,281,20,0.9,-9,-9.0,-9,1004.3,1015.7,0,1.0,15.5,15.4,100.0,17.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,306,-9.0,-9.00,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28449 |
-202309290700,281,0,0.3,-9,-9.0,-9,1004.6,1016.0,-9,-9.0,15.3,15.2,100.0,17.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,327,0.0,-9.00,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28450 |
-202309290800,281,29,0.7,-9,-9.0,-9,1004.8,1016.0,-9,-9.0,18.3,17.4,95.0,19.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,554,0.9,-9.00,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28451 |
-202309290900,281,11,0.7,-9,-9.0,-9,1005.3,1016.4,2,0.7,19.5,17.2,87.0,19.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,711,1.0,-9.00,-9,27.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28452 |
-202309291000,281,18,1.1,-9,-9.0,-9,1005.2,1016.3,-9,-9.0,22.5,16.9,71.0,19.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,3,63,-,-9,-9,-9,921,1.0,-9.00,-9,32.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28453 |
-202309291100,281,36,1.6,-9,-9.0,-9,1004.8,1015.8,-9,-9.0,23.5,16.0,63.0,18.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,75,-,-9,-9,-9,1030,1.0,-9.00,-9,36.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28454 |
-202309291200,281,7,1.1,-9,-9.0,-9,1003.9,1014.9,7,-1.5,24.8,16.5,60.0,18.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,48,-,-9,-9,-9,1361,0.9,-9.00,-9,36.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28455 |
-202309291300,281,9,1.0,-9,-9.0,-9,1003.1,1014.1,-9,-9.0,25.4,15.1,53.0,17.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,6,44,-,-9,-9,-9,1956,0.6,-9.00,-9,36.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28456 |
-202309291400,281,27,1.5,-9,-9.0,-9,1002.4,1013.3,-9,-9.0,26.1,14.8,50.0,16.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,44,-,-9,-9,-9,2215,0.0,-9.00,-9,31.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28457 |
-202309291500,281,32,1.1,-9,-9.0,-9,1002.0,1012.9,7,-2.0,25.7,15.0,52.0,17.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,41,-,-9,-9,-9,2237,0.0,-9.00,-9,28.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28458 |
-202309291600,281,34,0.8,-9,-9.0,-9,1001.5,1012.4,-9,-9.0,25.6,15.5,54.0,17.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,39,-,-9,-9,-9,2305,0.0,-9.00,-9,28.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28459 |
-202309291700,281,11,1.9,-9,-9.0,-9,1001.9,1012.8,-9,-9.0,24.2,17.4,66.0,19.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,42,-,-9,-9,-9,1832,0.0,-9.00,-9,25.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28460 |
-202309291800,281,7,1.5,-9,-9.0,-9,1001.9,1012.9,4,0.0,22.6,17.9,75.0,20.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,8,52,-,-9,-9,-9,1295,0.0,-9.00,-9,22.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28461 |
-202309291900,281,7,1.5,-9,-9.0,-9,1002.0,1013.1,-9,-9.0,22.0,17.9,78.0,20.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,45,-,-9,-9,-9,1238,0.0,-9.00,-9,21.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28462 |
-202309292000,281,9,0.9,-9,-9.0,-9,1001.9,1013.0,-9,-9.0,21.3,18.2,83.0,20.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,4,49,-,-9,-9,-9,1075,-9.0,-9.00,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28463 |
-202309292100,281,0,0.3,-9,-9.0,-9,1002.0,1013.1,1,0.2,20.7,18.6,88.0,21.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,1,68,-,-9,-9,-9,874,-9.0,-9.00,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28464 |
-202309292200,281,29,0.7,-9,-9.0,-9,1002.1,1013.2,-9,-9.0,19.6,18.4,93.0,21.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,5,50,-,-9,-9,-9,1021,-9.0,-9.00,-9,19.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28465 |
-202309292300,281,0,0.2,-9,-9.0,-9,1001.9,1013.0,-9,-9.0,19.5,18.8,96.0,21.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,47,-,-9,-9,-9,874,-9.0,-9.00,-9,19.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28466 |
-202309300000,281,0,0.1,-9,-9.0,-9,1000.8,1011.9,8,-1.2,19.1,18.7,98.0,21.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,45,-,-9,-9,-9,789,-9.0,-9.00,-9,19.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28467 |
-202309300100,281,0,0.4,-9,-9.0,-9,1000.3,1011.4,-9,-9.0,19.1,18.6,97.0,21.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,42,-,-9,-9,-9,869,-9.0,-9.00,-9,19.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28468 |
-202309300200,281,29,1.0,-9,-9.0,-9,1000.4,1011.5,-9,-9.0,19.0,18.5,97.0,21.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,39,-,-9,-9,-9,712,-9.0,-9.00,-9,19.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28469 |
-202309300300,281,32,0.5,-9,-9.0,-9,1000.2,1011.3,7,-0.6,19.1,18.6,97.0,21.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,34,-,-9,-9,-9,699,-9.0,-9.00,-9,19.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28470 |
-202309300400,281,27,1.8,-9,-9.0,-9,1000.8,1011.9,-9,-9.0,19.4,17.5,89.0,20.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,10,-,-9,-9,-9,925,-9.0,-9.00,-9,19.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28471 |
-202309300500,281,27,2.6,-9,-9.0,-9,1000.9,1012.1,-9,-9.0,18.3,16.4,89.0,18.6,0.1,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,31,-,-9,-9,-9,757,-9.0,-9.00,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28472 |
-202309300600,281,32,3.0,-9,-9.0,-9,1001.8,1013.1,2,1.8,16.9,14.3,85.0,16.3,0.5,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,28,-,-9,-9,-9,1078,-9.0,-9.00,-9,17.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28473 |
-202309300700,281,29,1.8,-9,-9.0,-9,1002.2,1013.6,-9,-9.0,15.4,14.2,93.0,16.2,1.4,2.0,2.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,22,-,-9,-9,-9,971,0.0,-9.00,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28474 |
-202309300800,281,27,2.9,-9,-9.0,-9,1003.0,1014.4,-9,-9.0,15.0,13.5,91.0,15.5,0.8,2.8,2.8,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,20,-,-9,-9,-9,1293,0.0,-9.00,-9,16.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28475 |
-202309300900,281,0,0.2,-9,-9.0,-9,1002.1,1013.5,0,0.4,15.2,13.3,89.0,15.3,0.9,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,3,18,-,-9,-9,-9,3489,0.0,-9.00,-9,18.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28476 |
-202309301000,281,23,1.4,-9,-9.0,-9,1001.5,1012.8,-9,-9.0,15.8,14.1,90.0,16.1,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,20,-,-9,-9,-9,2223,0.0,-9.00,-9,19.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28477 |
-202309301100,281,32,1.6,-9,-9.0,-9,1001.1,1012.4,-9,-9.0,16.8,14.7,88.0,16.7,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,3,-,-9,-9,-9,2271,0.0,-9.00,-9,21.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28478 |
-202309301200,281,27,1.6,-9,-9.0,-9,1000.4,1011.5,7,-2.0,19.5,15.7,79.0,17.8,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,6,4,-,-9,-9,-9,3171,0.3,-9.00,-9,34.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28479 |
-202309301300,281,27,1.8,-9,-9.0,-9,999.6,1010.7,-9,-9.0,20.9,14.9,69.0,16.9,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,69,-,-9,-9,-9,4538,0.6,-9.00,-9,32.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28480 |
-202309301400,281,25,2.0,-9,-9.0,-9,998.9,1009.9,-9,-9.0,22.4,14.2,60.0,16.2,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,33.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28481 |
-202309301500,281,27,3.0,-9,-9.0,-9,998.3,1009.2,7,-2.3,23.5,13.0,52.0,15.0,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,30.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28482 |
-202309301600,281,29,2.8,-9,-9.0,-9,998.3,1009.2,-9,-9.0,23.6,14.0,55.0,16.0,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,27.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28483 |
-202309301700,281,32,1.5,-9,-9.0,-9,998.4,1009.4,-9,-9.0,23.0,13.7,56.0,15.7,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,23.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28484 |
-202309301800,281,29,1.3,-9,-9.0,-9,998.6,1009.7,2,0.5,21.0,14.6,67.0,16.6,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,5000,0.4,-9.00,-9,19.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28485 |
-202309301900,281,29,1.5,-9,-9.0,-9,998.7,1009.8,-9,-9.0,19.3,14.9,76.0,16.9,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,-9,-,-9,-9,-9,4983,0.0,-9.00,-9,18.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28486 |
-202309302000,281,27,2.8,-9,-9.0,-9,999.3,1010.4,-9,-9.0,20.1,14.8,72.0,16.8,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,3802,-9.0,-9.00,-9,17.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28487 |
-202309302100,281,27,3.0,-9,-9.0,-9,999.5,1010.7,2,1.0,20.0,14.7,72.0,16.7,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,3654,-9.0,-9.00,-9,17.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28488 |
-202309302200,281,27,1.9,-9,-9.0,-9,1000.0,1011.1,-9,-9.0,18.5,14.9,80.0,16.9,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,3141,-9.0,-9.00,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28489 |
-202309302300,281,29,1.1,-9,-9.0,-9,999.9,1011.1,-9,-9.0,17.9,14.7,82.0,16.7,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,3120,-9.0,-9.00,-9,16.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28490 |
-202310010000,281,29,1.3,-9,-9.0,-9,1000.0,1011.3,2,0.6,16.4,15.0,92.0,17.0,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2252,-9.0,-9.00,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28491 |
-202310010100,281,27,0.5,-9,-9.0,-9,1000.2,1011.5,-9,-9.0,15.9,15.2,96.0,17.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1815,-9.0,-9.00,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28492 |
-202310010200,281,0,0.2,-9,-9.0,-9,1000.0,1011.4,-9,-9.0,15.3,14.8,97.0,16.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,1598,-9.0,-9.00,-9,15.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28493 |
-202310010300,281,0,0.3,-9,-9.0,-9,999.7,1011.1,8,-0.2,15.1,14.7,98.0,16.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,1433,-9.0,-9.00,-9,14.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28494 |
-202310010400,281,25,1.3,-9,-9.0,-9,999.9,1011.2,-9,-9.0,16.1,14.9,93.0,16.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,1779,-9.0,-9.00,-9,15.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28495 |
-202310010500,281,27,2.7,-9,-9.0,-9,1000.1,1011.4,-9,-9.0,16.9,14.3,85.0,16.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2334,-9.0,-9.00,-9,15.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28496 |
-202310010600,281,27,2.5,-9,-9.0,-9,1000.9,1012.2,2,1.1,16.2,13.6,85.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2562,-9.0,-9.00,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28497 |
-202310010700,281,29,1.0,-9,-9.0,-9,1001.6,1012.9,-9,-9.0,15.8,12.1,79.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3997,0.0,-9.00,-9,14.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28498 |
-202310010800,281,27,2.1,-9,-9.0,-9,1002.1,1013.3,-9,-9.0,18.2,11.5,65.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,4875,0.8,-9.00,-9,19.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28499 |
-202310010900,281,29,2.2,-9,-9.0,-9,1002.6,1013.8,2,1.6,20.0,11.9,60.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4768,1.0,-9.00,-9,24.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28500 |
-202310011000,281,27,2.1,-9,-9.0,-9,1002.9,1014.0,-9,-9.0,21.1,11.4,54.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4964,1.0,-9.00,-9,30.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28501 |
-202310011100,281,34,2.2,-9,-9.0,-9,1002.9,1014.0,-9,-9.0,21.5,9.3,46.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,29.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28502 |
-202310011200,281,27,3.4,-9,-9.0,-9,1002.7,1013.7,8,-0.1,22.9,9.2,42.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,1,26,-,-9,-9,-9,5000,0.9,-9.00,-9,32.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28503 |
-202310011300,281,34,2.6,-9,-9.0,-9,1002.2,1013.2,-9,-9.0,22.6,8.6,41.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.9,-9.00,-9,30.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28504 |
-202310011400,281,34,2.5,-9,-9.0,-9,1002.1,1013.1,-9,-9.0,23.1,7.6,37.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.7,-9.00,-9,31.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28505 |
-202310011500,281,27,3.6,-9,-9.0,-9,1002.3,1013.3,5,-0.4,23.6,8.4,38.0,11.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,30.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28506 |
-202310011600,281,34,2.0,-9,-9.0,-9,1002.5,1013.5,-9,-9.0,23.2,7.2,36.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,28.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28507 |
-202310011700,281,32,2.2,-9,-9.0,-9,1002.7,1013.7,-9,-9.0,22.3,7.2,38.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,22.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28508 |
-202310011800,281,29,1.4,-9,-9.0,-9,1003.2,1014.4,2,1.1,20.1,8.7,48.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.4,-9.00,-9,17.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28509 |
-202310011900,281,32,1.8,-9,-9.0,-9,1003.6,1014.9,-9,-9.0,16.8,10.6,67.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.0,-9.00,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28510 |
-202310012000,281,11,1.0,-9,-9.0,-9,1004.2,1015.6,-9,-9.0,15.4,11.5,78.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4241,-9.0,-9.00,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28511 |
-202310012100,281,7,1.0,-9,-9.0,-9,1004.7,1016.1,2,1.7,14.1,11.7,86.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3412,-9.0,-9.00,-9,13.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28512 |
-202310012200,281,0,0.2,-9,-9.0,-9,1004.9,1016.4,-9,-9.0,13.0,11.7,92.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3231,-9.0,-9.00,-9,12.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28513 |
-202310012300,281,0,0.4,-9,-9.0,-9,1005.7,1017.2,-9,-9.0,12.4,11.1,92.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3354,-9.0,-9.00,-9,12.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28514 |
-202310020000,281,9,0.6,-9,-9.0,-9,1005.8,1017.4,2,1.3,11.5,11.0,97.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3207,-9.0,-9.00,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28515 |
-202310020100,281,0,0.4,-9,-9.0,-9,1005.8,1017.4,-9,-9.0,11.1,10.6,97.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2846,-9.0,-9.00,-9,11.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28516 |
-202310020200,281,9,1.0,-9,-9.0,-9,1006.3,1017.9,-9,-9.0,10.7,10.3,98.0,12.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,2970,-9.0,-9.00,-9,11.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28517 |
-202310020300,281,0,0.2,-9,-9.0,-9,1006.3,1018.0,2,0.6,10.2,10.0,99.0,12.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,2925,-9.0,-9.00,-9,10.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28518 |
-202310020400,281,0,0.4,-9,-9.0,-9,1006.4,1018.1,-9,-9.0,10.0,9.8,99.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,2725,-9.0,-9.00,-9,10.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28519 |
-202310020500,281,7,0.8,-9,-9.0,-9,1006.4,1018.0,-9,-9.0,9.7,9.5,99.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2884,-9.0,-9.00,-9,10.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28520 |
-202310020600,281,7,0.5,-9,-9.0,-9,1006.8,1018.5,2,0.5,9.5,9.3,99.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2842,-9.0,-9.00,-9,10.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28521 |
-202310020700,281,0,0.4,-9,-9.0,-9,1007.2,1018.9,-9,-9.0,9.7,9.5,99.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2733,0.0,-9.00,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28522 |
-202310020800,281,0,0.0,-9,-9.0,-9,1007.5,1019.1,-9,-9.0,10.9,10.2,96.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,2453,0.0,-9.00,-9,13.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28523 |
-202310020900,281,16,0.7,-9,-9.0,-9,1008.2,1019.7,2,1.2,13.8,10.7,82.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,3885,1.0,-9.00,-9,24.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28524 |
-202310021000,281,34,1.1,-9,-9.0,-9,1007.8,1019.0,-9,-9.0,18.7,10.7,60.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4852,1.0,-9.00,-9,31.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28525 |
-202310021100,281,18,1.2,-9,-9.0,-9,1007.7,1019.0,-9,-9.0,19.9,11.3,58.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,34.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28526 |
-202310021200,281,25,2.0,-9,-9.0,-9,1007.2,1018.3,7,-1.4,22.3,8.0,40.0,10.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,4837,1.0,-9.00,-9,36.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28527 |
-202310021300,281,29,2.7,-9,-9.0,-9,1006.6,1017.6,-9,-9.0,23.2,6.4,34.0,9.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,35.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28528 |
-202310021400,281,32,2.3,-9,-9.0,-9,1005.9,1016.9,-9,-9.0,23.4,6.1,33.0,9.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,34.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28529 |
-202310021500,281,32,1.7,-9,-9.0,-9,1005.6,1016.6,7,-1.7,23.5,7.1,35.0,10.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,32.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28530 |
-202310021600,281,32,1.7,-9,-9.0,-9,1005.4,1016.4,-9,-9.0,23.2,8.4,39.0,11.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,29.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28531 |
-202310021700,281,11,2.2,-9,-9.0,-9,1005.5,1016.6,-9,-9.0,22.0,9.5,45.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,23.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28532 |
-202310021800,281,9,1.3,-9,-9.0,-9,1006.1,1017.3,3,0.7,19.4,9.2,52.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.5,-9.00,-9,17.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28533 |
-202310021900,281,9,0.7,-9,-9.0,-9,1006.7,1018.0,-9,-9.0,17.5,10.3,63.0,12.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4992,0.0,-9.00,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28534 |
-202310022000,281,0,0.4,-9,-9.0,-9,1007.1,1018.6,-9,-9.0,15.0,11.3,79.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,3705,-9.0,-9.00,-9,14.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28535 |
-202310022100,281,0,0.2,-9,-9.0,-9,1007.5,1019.0,2,1.7,13.5,11.0,85.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4536,-9.0,-9.00,-9,13.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28536 |
-202310022200,281,0,0.3,-9,-9.0,-9,1008.1,1019.6,-9,-9.0,12.6,11.1,91.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3504,-9.0,-9.00,-9,12.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28537 |
-202310022300,281,9,0.7,-9,-9.0,-9,1008.5,1020.1,-9,-9.0,11.8,10.6,93.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,3,65,-,-9,-9,-9,3085,-9.0,-9.00,-9,12.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28538 |
-202310030000,281,9,0.5,-9,-9.0,-9,1008.7,1020.3,2,1.3,11.4,10.4,94.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,5,61,-,-9,-9,-9,3521,-9.0,-9.00,-9,12.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28539 |
-202310030100,281,0,0.2,-9,-9.0,-9,1008.6,1020.2,-9,-9.0,11.2,10.4,95.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,5,63,-,-9,-9,-9,4309,-9.0,-9.00,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28540 |
-202310030200,281,0,0.2,-9,-9.0,-9,1008.8,1020.4,-9,-9.0,10.9,10.4,97.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,60,-,-9,-9,-9,3399,-9.0,-9.00,-9,11.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28541 |
-202310030300,281,0,0.1,-9,-9.0,-9,1008.2,1019.9,8,-0.4,10.6,10.1,97.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,5,54,-,-9,-9,-9,3789,-9.0,-9.00,-9,11.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28542 |
-202310030400,281,9,0.5,-9,-9.0,-9,1007.9,1019.6,-9,-9.0,10.3,9.9,98.0,12.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,3,52,-,-9,-9,-9,3650,-9.0,-9.00,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28543 |
-202310030500,281,0,0.1,-9,-9.0,-9,1007.9,1019.6,-9,-9.0,10.1,9.7,98.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,5,56,-,-9,-9,-9,3770,-9.0,-9.00,-9,10.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28544 |
-202310030600,281,7,0.7,-9,-9.0,-9,1007.4,1019.1,7,-0.8,10.0,9.6,98.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,5,51,-,-9,-9,-9,3768,-9.0,-9.00,-9,10.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28545 |
-202310030700,281,0,0.1,-9,-9.0,-9,1007.7,1019.4,-9,-9.0,10.5,10.1,98.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,52,-,-9,-9,-9,3449,0.0,-9.00,-9,12.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28546 |
-202310030800,281,0,0.4,-9,-9.0,-9,1008.3,1019.9,-9,-9.0,11.8,10.6,93.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,51,-,-9,-9,-9,3882,0.0,-9.00,-9,16.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28547 |
-202310030900,281,14,0.6,-9,-9.0,-9,1008.3,1019.8,0,0.7,13.5,11.7,89.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,52,-,-9,-9,-9,3926,0.0,-9.00,-9,18.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28548 |
-202310031000,281,25,0.7,-9,-9.0,-9,1008.4,1019.8,-9,-9.0,16.0,12.1,78.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,55,-,-9,-9,-9,4884,0.1,-9.00,-9,23.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28549 |
-202310031100,281,2,0.6,-9,-9.0,-9,1008.1,1019.3,-9,-9.0,18.7,12.2,66.0,14.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,55,-,-9,-9,-9,5000,0.3,-9.00,-9,27.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28550 |
-202310031200,281,32,0.7,-9,-9.0,-9,1007.8,1019.0,7,-0.8,20.0,12.7,63.0,14.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,48,-,-9,-9,-9,5000,0.0,-9.00,-9,28.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28551 |
-202310031300,281,27,1.4,-9,-9.0,-9,1007.5,1018.7,-9,-9.0,20.6,13.0,62.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,13,-,-9,-9,-9,5000,0.0,-9.00,-9,25.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28552 |
-202310031400,281,27,0.9,-9,-9.0,-9,1006.3,1017.5,-9,-9.0,20.4,13.1,63.0,15.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,43,-,-9,-9,-9,5000,0.0,-9.00,-9,22.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28553 |
-202310031500,281,36,0.6,-9,-9.0,-9,1005.8,1017.0,7,-2.0,20.6,13.5,64.0,15.5,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,4992,0.0,-9.00,-9,24.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28554 |
-202310031600,281,29,1.1,-9,-9.0,-9,1005.7,1016.9,-9,-9.0,19.2,14.4,74.0,16.4,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,1,21,-,-9,-9,-9,4856,0.0,-9.00,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28555 |
-202310031700,281,27,1.4,-9,-9.0,-9,1005.8,1017.0,-9,-9.0,18.1,14.5,80.0,16.5,0.2,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,6,26,-,-9,-9,-9,2624,0.0,-9.00,-9,18.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28556 |
-202310031800,281,0,0.1,-9,-9.0,-9,1005.5,1016.8,7,-0.2,17.8,15.4,86.0,17.5,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,27,-,-9,-9,-9,2066,0.0,-9.00,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28557 |
-202310031900,281,7,0.6,-9,-9.0,-9,1005.8,1017.1,-9,-9.0,17.1,15.9,93.0,18.1,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,29,-,-9,-9,-9,1765,0.0,-9.00,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28558 |
-202310032000,281,27,0.6,-9,-9.0,-9,1005.9,1017.2,-9,-9.0,16.5,15.5,94.0,17.6,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,4,13,-,-9,-9,-9,1734,-9.0,-9.00,-9,16.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28559 |
-202310032100,281,32,0.6,-9,-9.0,-9,1006.3,1017.7,2,0.9,15.5,15.1,98.0,17.2,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,13,-,-9,-9,-9,1253,-9.0,-9.00,-9,15.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28560 |
-202310032200,281,0,0.3,-9,-9.0,-9,1006.1,1017.6,-9,-9.0,14.9,14.5,98.0,16.5,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,1,-9,-,-9,-9,-9,1194,-9.0,-9.00,-9,14.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28561 |
-202310032300,281,0,0.2,-9,-9.0,-9,1005.9,1017.3,-9,-9.0,14.1,14.0,100.0,16.0,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,670,-9.0,-9.00,-9,13.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28562 |
-202310040000,281,0,0.1,-9,-9.0,-9,1005.8,1017.3,6,-0.4,13.3,13.2,100.0,15.2,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,797,-9.0,-9.00,-9,13.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28563 |
-202310040100,281,0,0.1,-9,-9.0,-9,1005.6,1017.1,-9,-9.0,13.7,13.6,100.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,3,38,-,-9,-9,-9,547,-9.0,-9.00,-9,14.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28564 |
-202310040200,281,32,0.6,-9,-9.0,-9,1005.2,1016.7,-9,-9.0,13.6,13.5,100.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,61,-,-9,-9,-9,175,-9.0,-9.00,-9,15.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28565 |
-202310040300,281,27,1.1,-9,-9.0,-9,1004.7,1016.1,7,-1.2,14.1,14.0,100.0,16.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,-9,-,-9,-9,-9,71,-9.0,-9.00,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28566 |
-202310040400,281,34,0.8,-9,-9.0,-9,1004.3,1015.7,-9,-9.0,13.6,13.5,100.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,46,-,-9,-9,-9,159,-9.0,-9.00,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28567 |
-202310040500,281,32,0.7,-9,-9.0,-9,1004.7,1016.2,-9,-9.0,13.4,13.3,100.0,15.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,4,-9,-,-9,-9,-9,35,-9.0,-9.00,-9,15.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28568 |
-202310040600,281,32,1.2,-9,-9.0,-9,1004.7,1016.2,1,0.1,13.0,12.9,100.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,-9,-,-9,-9,-9,1045,-9.0,-9.00,-9,14.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28569 |
-202310040700,281,29,1.2,-9,-9.0,-9,1004.8,1016.3,-9,-9.0,13.4,13.3,100.0,15.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,-9,-,-9,-9,-9,1706,0.0,-9.00,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28570 |
-202310040800,281,25,1.1,-9,-9.0,-9,1005.2,1016.6,-9,-9.0,14.5,14.3,99.0,16.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,1,-,-9,-9,-9,823,0.0,-9.00,-9,17.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28571 |
-202310040900,281,27,2.0,-9,-9.0,-9,1005.0,1016.4,0,0.2,15.8,13.9,89.0,15.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,2,-,-9,-9,-9,1346,0.4,-9.00,-9,23.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28572 |
-202310041000,281,25,1.6,-9,-9.0,-9,1004.6,1015.9,-9,-9.0,17.7,13.6,77.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,2,59,-,-9,-9,-9,1732,1.0,-9.00,-9,30.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28573 |
-202310041100,281,25,2.2,-9,-9.0,-9,1003.7,1014.9,-9,-9.0,20.4,13.5,65.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2449,1.0,-9.00,-9,33.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28574 |
-202310041200,281,27,3.4,-9,-9.0,-9,1002.7,1013.7,7,-2.7,22.6,12.5,53.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,35.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28575 |
-202310041300,281,32,2.7,-9,-9.0,-9,1001.8,1012.7,-9,-9.0,23.9,12.2,48.0,14.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,37.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28576 |
-202310041400,281,27,3.0,-9,-9.0,-9,1000.9,1011.8,-9,-9.0,23.7,11.3,46.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,34.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28577 |
-202310041500,281,32,2.2,-9,-9.0,-9,1000.7,1011.6,7,-2.1,23.7,11.3,46.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,31.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28578 |
-202310041600,281,29,2.5,-9,-9.0,-9,1000.3,1011.3,-9,-9.0,23.1,12.1,50.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,27.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28579 |
-202310041700,281,29,1.5,-9,-9.0,-9,1000.4,1011.4,-9,-9.0,22.8,13.5,56.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,5000,0.5,-9.00,-9,23.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28580 |
-202310041800,281,29,1.2,-9,-9.0,-9,1000.5,1011.6,4,0.0,20.9,13.8,64.0,15.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,5000,0.0,-9.00,-9,19.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28581 |
-202310041900,281,27,1.4,-9,-9.0,-9,1000.6,1011.7,-9,-9.0,20.4,13.5,65.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,15,-,-9,-9,-9,4783,0.0,-9.00,-9,19.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28582 |
-202310042000,281,29,1.1,-9,-9.0,-9,1000.7,1011.8,-9,-9.0,19.7,12.9,65.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,74,-,-9,-9,-9,4862,-9.0,-9.00,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28583 |
-202310042100,281,27,1.0,-9,-9.0,-9,1000.5,1011.6,4,0.0,19.5,13.2,67.0,15.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,59,-,-9,-9,-9,4011,-9.0,-9.00,-9,17.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28584 |
-202310042200,281,0,0.4,-9,-9.0,-9,1000.5,1011.7,-9,-9.0,17.5,14.7,84.0,16.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,18,-,-9,-9,-9,2875,-9.0,-9.00,-9,17.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28585 |
-202310042300,281,29,1.0,-9,-9.0,-9,1000.1,1011.3,-9,-9.0,18.4,13.4,73.0,15.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,59,-,-9,-9,-9,3491,-9.0,-9.00,-9,17.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28586 |
-202310050000,281,27,2.2,-9,-9.0,-9,1000.0,1011.1,8,-0.5,18.7,12.8,69.0,14.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,13,-,-9,-9,-9,3603,-9.0,-9.00,-9,17.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28587 |
-202310050100,281,29,2.6,-9,-9.0,-9,1000.2,1011.3,-9,-9.0,18.8,10.8,60.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,13,-,-9,-9,-9,4956,-9.0,-9.00,-9,16.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28588 |
-202310050200,281,29,3.0,-9,-9.0,-9,1000.9,1012.1,-9,-9.0,17.8,9.1,57.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,5000,-9.0,-9.00,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28589 |
-202310050300,281,29,2.4,29,10.0,233,1001.0,1012.3,2,1.2,16.8,5.7,48.0,9.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,5000,-9.0,-9.00,-9,13.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28590 |
-202310050400,281,29,2.9,-9,-9.0,-9,1001.2,1012.5,-9,-9.0,16.4,2.3,39.0,7.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,5000,-9.0,-9.00,-9,13.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28591 |
-202310050500,281,32,2.5,-9,-9.0,-9,1001.8,1013.2,-9,-9.0,15.1,2.5,43.0,7.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,5000,-9.0,-9.00,-9,12.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28592 |
-202310050600,281,32,2.9,-9,-9.0,-9,1003.2,1014.6,2,2.3,14.4,0.2,38.0,6.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,1522,-9.0,-9.00,-9,11.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28593 |
-202310050700,281,29,2.2,-9,-9.0,-9,1003.7,1015.1,-9,-9.0,13.7,0.9,42.0,6.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,674,0.0,-9.00,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28594 |
-202310050800,281,29,2.9,-9,-9.0,-9,1004.2,1015.6,-9,-9.0,13.7,0.9,42.0,6.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,5000,0.0,-9.00,-9,13.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28595 |
-202310050900,281,29,1.0,-9,-9.0,-9,1005.0,1016.4,2,1.8,13.8,1.4,43.0,6.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,5000,0.0,-9.00,-9,16.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28596 |
-202310051000,281,34,3.1,-9,-9.0,-9,1004.7,1016.0,-9,-9.0,16.5,2.1,38.0,7.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,5000,0.9,-9.00,-9,28.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28597 |
-202310051100,281,27,4.4,-9,-9.0,-9,1004.4,1015.7,-9,-9.0,17.3,2.0,36.0,7.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,31.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28598 |
-202310051200,281,29,4.0,-9,-9.0,-9,1003.7,1014.9,7,-1.5,18.8,0.8,30.0,6.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,34.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28599 |
-202310051300,281,29,2.3,-9,-9.0,-9,1003.1,1014.2,-9,-9.0,19.2,2.0,32.0,7.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4628,1.0,-9.00,-9,36.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28600 |
-202310051400,281,29,2.9,-9,-9.0,-9,1002.7,1013.9,-9,-9.0,20.3,0.6,27.0,6.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,34.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28601 |
-202310051500,281,25,2.9,-9,-9.0,-9,1002.3,1013.5,7,-1.4,19.9,2.6,32.0,7.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,31.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28602 |
-202310051600,281,27,3.8,-9,-9.0,-9,1002.3,1013.4,-9,-9.0,19.6,2.4,32.0,7.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,27.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28603 |
-202310051700,281,29,2.8,-9,-9.0,-9,1002.9,1014.1,-9,-9.0,18.8,2.9,35.0,7.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28604 |
-202310051800,281,29,1.3,-9,-9.0,-9,1003.1,1014.4,3,0.9,16.7,4.6,45.0,8.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4866,0.5,-9.00,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28605 |
-202310051900,281,29,1.2,-9,-9.0,-9,1003.8,1015.2,-9,-9.0,14.2,5.7,57.0,9.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4826,0.0,-9.00,-9,13.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28606 |
-202310052000,281,29,1.3,-9,-9.0,-9,1004.5,1016.0,-9,-9.0,12.4,6.2,66.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4777,-9.0,-9.00,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28607 |
-202310052100,281,27,2.1,-9,-9.0,-9,1005.5,1017.0,2,2.6,13.4,5.0,57.0,8.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4241,-9.0,-9.00,-9,11.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28608 |
-202310052200,281,29,1.4,-9,-9.0,-9,1005.9,1017.4,-9,-9.0,13.3,5.4,59.0,9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,4302,-9.0,-9.00,-9,12.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28609 |
-202310052300,281,27,1.0,-9,-9.0,-9,1006.2,1017.7,-9,-9.0,12.8,5.2,60.0,8.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,4647,-9.0,-9.00,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28610 |
-202310060000,281,32,1.7,-9,-9.0,-9,1006.1,1017.7,1,0.7,10.8,6.3,74.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,4603,-9.0,-9.00,-9,11.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28611 |
-202310060100,281,32,0.5,-9,-9.0,-9,1006.0,1017.6,-9,-9.0,11.3,6.2,71.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,4247,-9.0,-9.00,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28612 |
-202310060200,281,29,0.7,-9,-9.0,-9,1006.5,1018.2,-9,-9.0,9.9,7.3,84.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,3886,-9.0,-9.00,-9,10.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28613 |
-202310060300,281,0,0.4,-9,-9.0,-9,1006.6,1018.3,3,0.6,10.0,7.2,83.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,2,64,-,-9,-9,-9,3976,-9.0,-9.00,-9,10.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28614 |
-202310060400,281,27,0.7,-9,-9.0,-9,1006.7,1018.4,-9,-9.0,10.0,6.5,79.0,9.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,2,65,-,-9,-9,-9,4572,-9.0,-9.00,-9,10.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28615 |
-202310060500,281,11,0.5,-9,-9.0,-9,1007.0,1018.7,-9,-9.0,9.2,7.8,91.0,10.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,3645,-9.0,-9.00,-9,10.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28616 |
-202310060600,281,0,0.2,-9,-9.0,-9,1007.2,1018.9,2,0.6,8.9,7.9,94.0,10.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,3239,-9.0,-9.00,-9,10.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28617 |
-202310060700,281,11,0.5,-9,-9.0,-9,1007.8,1019.5,-9,-9.0,8.9,7.8,93.0,10.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,3441,0.0,-9.00,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28618 |
-202310060800,281,9,0.7,-9,-9.0,-9,1008.5,1020.2,-9,-9.0,10.7,8.0,84.0,10.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,62,-,-9,-9,-9,3268,0.6,-9.00,-9,14.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28619 |
-202310060900,281,25,1.2,-9,-9.0,-9,1008.3,1019.7,0,0.8,14.6,6.4,58.0,9.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,66,-,-9,-9,-9,4412,0.8,-9.00,-9,22.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28620 |
-202310061000,281,27,1.3,-9,-9.0,-9,1008.6,1020.0,-9,-9.0,16.8,6.0,49.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,4923,1.0,-9.00,-9,29.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28621 |
-202310061100,281,32,1.6,-9,-9.0,-9,1008.0,1019.3,-9,-9.0,18.2,5.4,43.0,9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4976,1.0,-9.00,-9,33.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28622 |
-202310061200,281,16,1.1,-9,-9.0,-9,1007.5,1018.7,8,-1.0,19.3,6.3,43.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1856,1.0,-9.00,-9,36.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28623 |
-202310061300,281,25,1.4,-9,-9.0,-9,1006.6,1017.8,-9,-9.0,20.9,5.2,36.0,8.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,-9,1.0,-9.00,-9,37.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28624 |
-202310061400,281,23,1.0,-9,-9.0,-9,1005.7,1016.8,-9,-9.0,21.4,4.0,32.0,8.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,-9,1.0,-9.00,-9,36.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28625 |
-202310061500,281,25,1.3,-9,-9.0,-9,1005.6,1016.7,7,-2.0,21.5,4.0,32.0,8.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,-9,1.0,-9.00,-9,33.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28626 |
-202310061600,281,23,0.9,-9,-9.0,-9,1005.5,1016.6,-9,-9.0,21.4,5.6,36.0,9.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,30.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28627 |
-202310061700,281,23,0.8,-9,-9.0,-9,1005.8,1017.0,-9,-9.0,20.8,5.9,38.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,4991,0.5,-9.00,-9,22.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28628 |
-202310061800,281,29,1.2,-9,-9.0,-9,1006.3,1017.5,3,0.8,18.3,8.8,54.0,11.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,2,62,-,-9,-9,-9,4476,0.1,-9.00,-9,17.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28629 |
-202310061900,281,0,0.0,-9,-9.0,-9,1007.2,1018.5,-9,-9.0,16.8,9.4,62.0,11.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,62,-,-9,-9,-9,4826,0.0,-9.00,-9,16.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28630 |
-202310062000,281,9,0.7,-9,-9.0,-9,1007.6,1019.0,-9,-9.0,15.3,11.6,79.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,3,71,-,-9,-9,-9,2918,-9.0,-9.00,-9,15.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28631 |
-202310062100,281,0,0.4,-9,-9.0,-9,1008.3,1019.8,2,2.3,14.8,11.5,81.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,3766,-9.0,-9.00,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28632 |
-202310062200,281,32,1.2,-9,-9.0,-9,1008.6,1020.1,-9,-9.0,14.8,10.2,74.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,4873,-9.0,-9.00,-9,15.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28633 |
-202310062300,281,16,0.5,-9,-9.0,-9,1008.7,1020.2,-9,-9.0,14.8,11.1,79.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,1305,-9.0,-9.00,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28634 |
-202310070000,281,0,0.2,-9,-9.0,-9,1009.1,1020.6,2,0.8,14.4,10.6,78.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,1493,-9.0,-9.00,-9,14.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28635 |
-202310070100,281,9,0.5,-9,-9.0,-9,1009.3,1020.8,-9,-9.0,13.7,11.9,89.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,2918,-9.0,-9.00,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28636 |
-202310070200,281,0,0.4,-9,-9.0,-9,1009.2,1020.7,-9,-9.0,13.4,11.6,89.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,3664,-9.0,-9.00,-9,14.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28637 |
-202310070300,281,14,0.6,-9,-9.0,-9,1009.1,1020.6,4,0.0,13.0,11.8,93.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,62,-,-9,-9,-9,3404,-9.0,-9.00,-9,13.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28638 |
-202310070400,281,0,0.2,-9,-9.0,-9,1009.2,1020.8,-9,-9.0,12.5,11.7,95.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,1,60,-,-9,-9,-9,3236,-9.0,-9.00,-9,12.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28639 |
-202310070500,281,0,0.0,-9,-9.0,-9,1009.6,1021.2,-9,-9.0,11.9,11.4,97.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,60,-,-9,-9,-9,2835,-9.0,-9.00,-9,11.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28640 |
-202310070600,281,0,0.0,-9,-9.0,-9,1009.9,1021.5,2,0.9,11.3,10.9,98.0,13.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2070,-9.0,-9.00,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28641 |
-202310070700,281,9,0.6,-9,-9.0,-9,1010.5,1022.2,-9,-9.0,11.2,10.7,97.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,1673,0.0,-9.00,-9,12.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28642 |
-202310070800,281,0,0.1,-9,-9.0,-9,1011.7,1023.3,-9,-9.0,12.4,11.4,94.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,2216,0.2,-9.00,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28643 |
-202310070900,281,18,0.7,-9,-9.0,-9,1011.8,1023.3,1,1.8,14.0,11.3,84.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2732,0.3,-9.00,-9,19.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28644 |
-202310071000,281,0,0.3,-9,-9.0,-9,1012.0,1023.5,-9,-9.0,15.7,10.6,72.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,3719,0.0,-9.00,-9,21.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28645 |
-202310071100,281,23,0.9,-9,-9.0,-9,1011.8,1023.1,-9,-9.0,18.5,11.0,62.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,4472,0.8,-9.00,-9,30.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28646 |
-202310071200,281,7,3.0,-9,-9.0,-9,1011.4,1022.6,8,-0.7,21.2,8.7,45.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,36.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28647 |
-202310071300,281,11,2.0,-9,-9.0,-9,1010.7,1021.9,-9,-9.0,21.6,9.7,47.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,4,70,-,-9,-9,-9,4948,0.7,-9.00,-9,32.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28648 |
-202310071400,281,9,2.4,-9,-9.0,-9,1010.3,1021.5,-9,-9.0,21.0,10.7,52.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,72,-,-9,-9,-9,5000,0.3,-9.00,-9,26.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28649 |
-202310071500,281,9,2.7,-9,-9.0,-9,1010.4,1021.6,5,-1.0,20.8,10.5,52.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,61,-,-9,-9,-9,5000,0.0,-9.00,-9,25.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28650 |
-202310071600,281,7,3.6,-9,-9.0,-9,1010.6,1021.8,-9,-9.0,19.4,12.1,63.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,51,-,-9,-9,-9,5000,0.0,-9.00,-9,22.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28651 |
-202310071700,281,7,3.1,-9,-9.0,-9,1011.0,1022.3,-9,-9.0,18.2,12.4,69.0,14.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,5000,0.0,-9.00,-9,19.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28652 |
-202310071800,281,9,1.3,-9,-9.0,-9,1011.4,1022.7,2,1.1,17.4,12.5,73.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,16,-,-9,-9,-9,4984,0.0,-9.00,-9,18.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28653 |
-202310071900,281,14,0.8,-9,-9.0,-9,1011.8,1023.2,-9,-9.0,17.1,12.8,76.0,14.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,18,-,-9,-9,-9,4295,0.0,-9.00,-9,17.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28654 |
-202310072000,281,0,0.2,-9,-9.0,-9,1012.7,1024.1,-9,-9.0,16.9,12.6,76.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,20,-,-9,-9,-9,4990,-9.0,-9.00,-9,17.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28655 |
-202310072100,281,0,0.2,-9,-9.0,-9,1012.9,1024.3,2,1.6,16.6,12.9,79.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,21,-,-9,-9,-9,4266,-9.0,-9.00,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28656 |
-202310072200,281,32,0.8,-9,-9.0,-9,1013.0,1024.5,-9,-9.0,15.9,13.0,83.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,32,-,-9,-9,-9,4678,-9.0,-9.00,-9,16.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28657 |
-202310072300,281,0,0.3,-9,-9.0,-9,1012.8,1024.3,-9,-9.0,15.6,13.4,87.0,15.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,25,-,-9,-9,-9,4029,-9.0,-9.00,-9,16.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28658 |
-202310080000,281,0,0.1,-9,-9.0,-9,1012.5,1024.0,8,-0.3,15.4,12.8,85.0,14.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,24,-,-9,-9,-9,4700,-9.0,-9.00,-9,16.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28659 |
-202310080100,281,0,0.1,-9,-9.0,-9,1012.5,1024.0,-9,-9.0,15.1,13.6,91.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,25,-,-9,-9,-9,4646,-9.0,-9.00,-9,16.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28660 |
-202310080200,281,9,0.7,-9,-9.0,-9,1012.5,1024.0,-9,-9.0,15.1,13.6,91.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,14,-,-9,-9,-9,4326,-9.0,-9.00,-9,16.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28661 |
-202310080300,281,0,0.2,-9,-9.0,-9,1012.4,1023.9,7,-0.1,15.4,13.4,88.0,15.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,16,-,-9,-9,-9,4475,-9.0,-9.00,-9,16.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28662 |
-202310080400,281,0,0.1,-9,-9.0,-9,1012.1,1023.6,-9,-9.0,15.5,13.1,86.0,15.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,17,-,-9,-9,-9,4580,-9.0,-9.00,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28663 |
-202310080500,281,9,0.6,-9,-9.0,-9,1012.3,1023.7,-9,-9.0,16.2,12.1,77.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,14,-,-9,-9,-9,5000,-9.0,-9.00,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28664 |
-202310080600,281,0,0.2,-9,-9.0,-9,1012.2,1023.7,5,-0.2,15.6,12.3,81.0,14.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,15,-,-9,-9,-9,4918,-9.0,-9.00,-9,15.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28665 |
-202310080700,281,0,0.3,-9,-9.0,-9,1012.5,1024.0,-9,-9.0,15.7,12.6,82.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,14,-,-9,-9,-9,4919,0.0,-9.00,-9,16.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28666 |
-202310080800,281,7,0.6,-9,-9.0,-9,1012.7,1024.1,-9,-9.0,16.4,12.7,79.0,14.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,14,-,-9,-9,-9,4312,0.0,-9.00,-9,17.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28667 |
-202310080900,281,9,2.0,-9,-9.0,-9,1012.8,1024.1,1,0.4,18.1,11.1,64.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,31,-,-9,-9,-9,5000,0.0,-9.00,-9,19.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28668 |
-202310081000,281,9,3.3,-9,-9.0,-9,1012.7,1024.0,-9,-9.0,18.7,9.7,56.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,43,-,-9,-9,-9,5000,0.0,-9.00,-9,21.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28669 |
-202310081100,281,7,3.0,-9,-9.0,-9,1012.4,1023.6,-9,-9.0,19.1,9.2,53.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,50,-,-9,-9,-9,4223,0.0,-9.00,-9,23.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28670 |
-202310081200,281,7,2.6,-9,-9.0,-9,1011.9,1023.1,7,-1.0,19.3,9.4,53.0,11.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,38,-,-9,-9,-9,5000,0.0,-9.00,-9,22.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28671 |
-202310081300,281,7,2.2,-9,-9.0,-9,1011.0,1022.2,-9,-9.0,19.5,10.2,55.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,38,-,-9,-9,-9,5000,0.0,-9.00,-9,23.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28672 |
-202310081400,281,7,2.2,-9,-9.0,-9,1010.9,1022.1,-9,-9.0,19.3,11.0,59.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,12,-,-9,-9,-9,5000,0.0,-9.00,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28673 |
-202310081500,281,11,2.7,-9,-9.0,-9,1011.0,1022.4,5,-0.7,17.3,13.8,80.0,15.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,8,-,-9,-9,-9,4653,0.0,-9.00,-9,20.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28674 |
-202310081600,281,9,3.3,-9,-9.0,-9,1011.2,1022.5,-9,-9.0,17.9,12.5,71.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,9,-,-9,-9,-9,4997,0.0,-9.00,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28675 |
-202310081700,281,9,0.9,-9,-9.0,-9,1011.0,1022.4,-9,-9.0,17.3,12.6,74.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,5,15,-,-9,-9,-9,4997,0.0,-9.00,-9,18.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28676 |
-202310081800,281,0,0.4,-9,-9.0,-9,1010.9,1022.3,8,-0.1,17.0,12.9,77.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,4,18,-,-9,-9,-9,2574,0.0,-9.00,-9,17.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28677 |
-202310081900,281,5,1.1,-9,-9.0,-9,1011.0,1022.3,-9,-9.0,17.5,11.5,68.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,12,-,-9,-9,-9,4976,-9.0,-9.00,-9,16.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28678 |
-202310082000,281,2,0.7,-9,-9.0,-9,1011.3,1022.7,-9,-9.0,17.0,11.0,68.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,37,-,-9,-9,-9,5000,-9.0,-9.00,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28679 |
-202310082100,281,0,0.4,-9,-9.0,-9,1011.5,1023.0,2,0.7,15.2,11.5,79.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,6,13,-,-9,-9,-9,5000,-9.0,-9.00,-9,15.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28680 |
-202310082200,281,29,0.6,-9,-9.0,-9,1011.1,1022.6,-9,-9.0,15.1,11.8,81.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,13,-,-9,-9,-9,5000,-9.0,-9.00,-9,14.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28681 |
-202310082300,281,29,1.5,-9,-9.0,-9,1010.8,1022.3,-9,-9.0,14.1,11.9,87.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,2,11,-,-9,-9,-9,981,-9.0,-9.00,-9,14.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28682 |
-202310090000,281,27,0.9,-9,-9.0,-9,1010.6,1022.1,7,-0.9,15.2,11.3,78.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,10,-,-9,-9,-9,1992,-9.0,-9.00,-9,15.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28683 |
-202310090100,281,27,0.6,-9,-9.0,-9,1010.4,1021.9,-9,-9.0,15.1,11.4,79.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,9,-,-9,-9,-9,2420,-9.0,-9.00,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28684 |
-202310090200,281,0,0.1,-9,-9.0,-9,1010.2,1021.7,-9,-9.0,15.2,11.7,80.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,5,-,-9,-9,-9,4961,-9.0,-9.00,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28685 |
-202310090300,281,0,0.3,-9,-9.0,-9,1009.7,1021.2,7,-0.9,15.3,11.4,78.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,3,-,-9,-9,-9,4483,-9.0,-9.00,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28686 |
-202310090400,281,29,0.8,-9,-9.0,-9,1009.4,1020.9,-9,-9.0,15.2,11.3,78.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,4,-,-9,-9,-9,2060,-9.0,-9.00,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28687 |
-202310090500,281,27,0.7,-9,-9.0,-9,1009.0,1020.5,-9,-9.0,15.1,11.6,80.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,7,-,-9,-9,-9,5000,-9.0,-9.00,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28688 |
-202310090600,281,0,0.3,-9,-9.0,-9,1009.2,1020.7,5,-0.5,14.8,11.3,80.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,8,-,-9,-9,-9,5000,-9.0,-9.00,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28689 |
-202310090700,281,29,0.5,-9,-9.0,-9,1009.5,1021.0,-9,-9.0,14.9,11.6,81.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,9,-,-9,-9,-9,4987,0.0,-9.00,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28690 |
-202310090800,281,27,1.0,-9,-9.0,-9,1009.3,1020.7,-9,-9.0,15.9,11.6,76.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,11,-,-9,-9,-9,2335,0.0,-9.00,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28691 |
-202310090900,281,23,0.9,-9,-9.0,-9,1009.8,1021.1,2,0.4,17.9,11.9,68.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,6,12,-,-9,-9,-9,3584,0.4,-9.00,-9,26.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28692 |
-202310091000,281,0,0.4,-9,-9.0,-9,1009.1,1020.3,-9,-9.0,18.6,12.5,68.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,2,45,-,-9,-9,-9,4915,0.4,-9.00,-9,28.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28693 |
-202310091100,281,32,0.6,-9,-9.0,-9,1008.8,1020.1,-9,-9.0,19.8,13.2,66.0,15.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,5000,0.4,-9.00,-9,25.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28694 |
-202310091200,281,27,1.2,-9,-9.0,-9,1008.4,1019.7,7,-1.4,20.0,13.4,66.0,15.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,9,-,-9,-9,-9,4979,0.0,-9.00,-9,25.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28695 |
-202310091300,281,27,1.0,-9,-9.0,-9,1007.8,1019.0,-9,-9.0,20.1,13.7,67.0,15.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,9,-,-9,-9,-9,4971,0.0,-9.00,-9,24.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28696 |
-202310091400,281,20,0.6,-9,-9.0,-9,1007.1,1018.3,-9,-9.0,20.7,13.6,64.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,10,-,-9,-9,-9,4931,0.0,-9.00,-9,28.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28697 |
-202310091500,281,11,1.5,-9,-9.0,-9,1006.6,1017.7,7,-2.0,21.5,14.1,63.0,16.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,9,-,-9,-9,-9,4764,0.2,-9.00,-9,32.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28698 |
-202310091600,281,11,1.4,-9,-9.0,-9,1006.8,1018.0,-9,-9.0,20.4,13.8,66.0,15.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,9,-,-9,-9,-9,5000,0.1,-9.00,-9,23.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28699 |
-202310091700,281,9,1.4,-9,-9.0,-9,1006.9,1018.1,-9,-9.0,19.9,14.6,72.0,16.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,9,-,-9,-9,-9,4992,0.0,-9.00,-9,21.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28700 |
-202310091800,281,11,1.0,-9,-9.0,-9,1007.0,1018.2,2,0.5,18.9,14.7,77.0,16.7,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,17,-,-9,-9,-9,3800,0.0,-9.00,-9,18.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28701 |
-202310091900,281,9,0.7,-9,-9.0,-9,1007.4,1018.7,-9,-9.0,17.8,14.4,81.0,16.4,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,22,-,-9,-9,-9,4143,-9.0,-9.00,-9,16.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28702 |
-202310092000,281,7,0.6,-9,-9.0,-9,1007.5,1018.9,-9,-9.0,16.2,14.3,89.0,16.3,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,2521,-9.0,-9.00,-9,15.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28703 |
-202310092100,281,7,0.5,-9,-9.0,-9,1007.7,1019.1,2,0.9,15.3,14.6,96.0,16.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,2691,-9.0,-9.00,-9,14.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28704 |
-202310092200,281,9,0.7,-9,-9.0,-9,1007.8,1019.3,-9,-9.0,14.2,13.7,97.0,15.7,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,12,-,-9,-9,-9,2445,-9.0,-9.00,-9,14.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28705 |
-202310092300,281,7,0.6,-9,-9.0,-9,1007.6,1019.1,-9,-9.0,14.1,13.7,98.0,15.7,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,1,31,-,-9,-9,-9,2529,-9.0,-9.00,-9,13.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28706 |
-202310100000,281,0,0.1,-9,-9.0,-9,1007.2,1018.7,8,-0.4,14.0,13.6,98.0,15.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,27,-,-9,-9,-9,2778,-9.0,-9.00,-9,13.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28707 |
-202310100100,281,0,0.4,-9,-9.0,-9,1007.3,1018.8,-9,-9.0,13.2,13.0,99.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,11,-,-9,-9,-9,2560,-9.0,-9.00,-9,13.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28708 |
-202310100200,281,0,0.2,-9,-9.0,-9,1007.5,1019.0,-9,-9.0,12.6,12.4,99.0,14.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2210,-9.0,-9.00,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28709 |
-202310100300,281,27,1.7,-9,-9.0,-9,1007.2,1018.6,8,-0.1,14.5,13.0,91.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1662,-9.0,-9.00,-9,12.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28710 |
-202310100400,281,29,1.1,-9,-9.0,-9,1006.8,1018.3,-9,-9.0,13.9,12.6,92.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1369,-9.0,-9.00,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28711 |
-202310100500,281,29,1.5,-9,-9.0,-9,1007.6,1019.1,-9,-9.0,14.1,12.1,88.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,1482,-9.0,-9.00,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28712 |
-202310100600,281,29,1.9,-9,-9.0,-9,1008.0,1019.5,3,0.9,12.8,12.1,96.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,947,-9.0,-9.00,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28713 |
-202310100700,281,27,2.8,-9,-9.0,-9,1008.1,1019.5,-9,-9.0,14.5,12.5,88.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1507,0.2,-9.00,-9,13.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28714 |
-202310100800,281,25,1.7,-9,-9.0,-9,1008.7,1020.1,-9,-9.0,15.8,11.9,78.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2018,1.0,-9.00,-9,19.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28715 |
-202310100900,281,29,1.5,-9,-9.0,-9,1009.4,1020.7,2,1.2,17.6,11.8,69.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,2466,1.0,-9.00,-9,24.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28716 |
-202310101000,281,32,1.9,-9,-9.0,-9,1009.5,1020.7,-9,-9.0,19.3,10.5,57.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3933,1.0,-9.00,-9,29.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28717 |
-202310101100,281,29,1.6,-9,-9.0,-9,1009.2,1020.4,-9,-9.0,20.4,10.7,54.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4870,1.0,-9.00,-9,33.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28718 |
-202310101200,281,29,1.3,-9,-9.0,-9,1008.7,1019.9,7,-0.8,21.3,9.8,48.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,14,-,-9,-9,-9,5000,0.9,-9.00,-9,38.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28719 |
-202310101300,281,2,1.8,-9,-9.0,-9,1008.5,1019.7,-9,-9.0,21.0,9.5,48.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,15,-,-9,-9,-9,5000,0.3,-9.00,-9,28.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28720 |
-202310101400,281,9,1.4,-9,-9.0,-9,1008.1,1019.2,-9,-9.0,21.9,9.7,46.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,2,19,-,-9,-9,-9,5000,0.4,-9.00,-9,34.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28721 |
-202310101500,281,9,3.3,-9,-9.0,-9,1008.4,1019.6,5,-0.3,21.3,10.7,51.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,22,-,-9,-9,-9,5000,0.4,-9.00,-9,30.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28722 |
-202310101600,281,9,2.4,-9,-9.0,-9,1008.6,1019.8,-9,-9.0,20.6,9.8,50.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,16,-,-9,-9,-9,4903,0.3,-9.00,-9,23.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28723 |
-202310101700,281,11,1.7,-9,-9.0,-9,1009.1,1020.4,-9,-9.0,20.0,10.6,55.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,4690,0.4,-9.00,-9,21.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28724 |
-202310101800,281,11,1.3,-9,-9.0,-9,1009.4,1020.7,2,1.1,18.1,11.6,66.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,1,-9,-,-9,-9,-9,4081,0.0,-9.00,-9,16.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28725 |
-202310101900,281,0,0.2,-9,-9.0,-9,1010.0,1021.4,-9,-9.0,16.7,11.8,73.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,3819,-9.0,-9.00,-9,15.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28726 |
-202310102000,281,9,0.5,-9,-9.0,-9,1010.5,1022.0,-9,-9.0,14.3,12.1,87.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2907,-9.0,-9.00,-9,14.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28727 |
-202310102100,281,7,0.5,-9,-9.0,-9,1011.0,1022.5,2,1.8,14.2,11.8,86.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2736,-9.0,-9.00,-9,13.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28728 |
-202310102200,281,0,0.3,-9,-9.0,-9,1011.4,1022.9,-9,-9.0,13.6,11.9,90.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2202,-9.0,-9.00,-9,12.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28729 |
-202310102300,281,0,0.2,-9,-9.0,-9,1011.7,1023.3,-9,-9.0,13.2,11.9,92.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,2331,-9.0,-9.00,-9,12.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28730 |
-202310110000,281,0,0.3,-9,-9.0,-9,1011.9,1023.5,2,1.0,12.3,11.6,96.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2129,-9.0,-9.00,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28731 |
-202310110100,281,0,0.1,-9,-9.0,-9,1012.2,1023.8,-9,-9.0,11.8,11.3,97.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2030,-9.0,-9.00,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28732 |
-202310110200,281,0,0.4,-9,-9.0,-9,1012.5,1024.2,-9,-9.0,11.1,10.7,98.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1971,-9.0,-9.00,-9,10.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28733 |
-202310110300,281,9,0.5,-9,-9.0,-9,1012.4,1024.1,0,0.6,10.4,10.2,99.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1718,-9.0,-9.00,-9,9.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28734 |
-202310110400,281,0,0.1,-9,-9.0,-9,1012.7,1024.5,-9,-9.0,9.8,9.6,99.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1323,-9.0,-9.00,-9,9.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28735 |
-202310110500,281,7,0.5,-9,-9.0,-9,1012.8,1024.5,-9,-9.0,9.4,9.2,99.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1522,-9.0,-9.00,-9,9.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28736 |
-202310110600,281,0,0.4,-9,-9.0,-9,1013.4,1025.2,2,1.1,9.1,8.9,99.0,11.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1596,-9.0,-9.00,-9,8.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28737 |
-202310110700,281,9,0.6,-9,-9.0,-9,1013.9,1025.7,-9,-9.0,8.8,8.6,99.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1529,0.1,-9.00,-9,9.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28738 |
-202310110800,281,11,0.7,-9,-9.0,-9,1014.4,1026.1,-9,-9.0,10.6,9.8,95.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1797,1.0,-9.00,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28739 |
-202310110900,281,16,0.5,-9,-9.0,-9,1014.8,1026.3,2,1.1,13.9,10.1,78.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2495,1.0,-9.00,-9,22.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28740 |
-202310111000,281,27,0.7,-9,-9.0,-9,1014.7,1026.0,-9,-9.0,18.0,11.7,67.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,2802,1.0,-9.00,-9,30.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28741 |
-202310111100,281,14,0.9,-9,-9.0,-9,1014.1,1025.4,-9,-9.0,20.1,9.0,49.0,11.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,4264,1.0,-9.00,-9,34.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28742 |
-202310111200,281,9,3.1,-9,-9.0,-9,1013.6,1024.8,7,-1.5,21.2,8.1,43.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,17,-,-9,-9,-9,5000,0.5,-9.00,-9,33.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28743 |
-202310111300,281,7,3.5,-9,-9.0,-9,1012.8,1024.0,-9,-9.0,21.8,8.3,42.0,10.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,4,21,-,-9,-9,-9,5000,0.7,-9.00,-9,33.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28744 |
-202310111400,281,9,3.2,-9,-9.0,-9,1012.5,1023.7,-9,-9.0,21.2,7.4,41.0,10.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,17,-,-9,-9,-9,4553,0.5,-9.00,-9,29.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28745 |
-202310111500,281,9,2.9,-9,-9.0,-9,1012.5,1023.8,5,-1.0,20.6,7.5,43.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,5,19,-,-9,-9,-9,5000,0.5,-9.00,-9,25.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28746 |
-202310111600,281,9,2.8,-9,-9.0,-9,1012.5,1023.7,-9,-9.0,21.0,7.5,42.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,3,57,-,-9,-9,-9,5000,0.6,-9.00,-9,26.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28747 |
-202310111700,281,9,2.0,-9,-9.0,-9,1012.6,1023.8,-9,-9.0,19.3,6.7,44.0,9.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,5000,0.5,-9.00,-9,18.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28748 |
-202310111800,281,9,1.3,-9,-9.0,-9,1012.8,1024.2,3,0.4,17.1,5.9,48.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.1,-9.00,-9,14.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28749 |
-202310111900,281,7,0.8,-9,-9.0,-9,1013.2,1024.7,-9,-9.0,15.1,7.6,61.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3939,-9.0,-9.00,-9,12.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28750 |
-202310112000,281,0,0.3,-9,-9.0,-9,1013.5,1025.1,-9,-9.0,12.6,8.4,76.0,11.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3656,-9.0,-9.00,-9,11.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28751 |
-202310112100,281,0,0.4,-9,-9.0,-9,1013.9,1025.6,2,1.4,11.5,8.3,81.0,10.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3889,-9.0,-9.00,-9,10.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28752 |
-202310112200,281,0,0.2,-9,-9.0,-9,1014.4,1026.1,-9,-9.0,10.5,8.5,88.0,11.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3162,-9.0,-9.00,-9,9.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28753 |
-202310112300,281,0,0.2,-9,-9.0,-9,1014.4,1026.1,-9,-9.0,9.6,8.0,90.0,10.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3721,-9.0,-9.00,-9,9.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28754 |
-202310120000,281,9,0.5,-9,-9.0,-9,1014.6,1026.4,2,0.8,9.0,7.9,93.0,10.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3784,-9.0,-9.00,-9,8.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28755 |
-202310120100,281,7,0.6,-9,-9.0,-9,1014.5,1026.3,-9,-9.0,8.4,7.6,95.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3399,-9.0,-9.00,-9,8.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28756 |
-202310120200,281,0,0.3,-9,-9.0,-9,1014.3,1026.1,-9,-9.0,7.9,7.4,97.0,10.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,2769,-9.0,-9.00,-9,7.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28757 |
-202310120300,281,0,0.2,-9,-9.0,-9,1014.0,1025.8,7,-0.6,7.6,7.1,97.0,10.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,3003,-9.0,-9.00,-9,7.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28758 |
-202310120400,281,7,0.8,-9,-9.0,-9,1014.4,1026.3,-9,-9.0,7.2,6.7,97.0,9.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3021,-9.0,-9.00,-9,7.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28759 |
-202310120500,281,11,0.7,-9,-9.0,-9,1014.6,1026.5,-9,-9.0,6.7,6.4,98.0,9.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2629,-9.0,-9.00,-9,7.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28760 |
-202310120600,281,0,0.2,-9,-9.0,-9,1014.7,1026.6,2,0.8,6.8,6.5,98.0,9.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2768,-9.0,-9.00,-9,6.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28761 |
-202310120700,281,0,0.4,-9,-9.0,-9,1014.8,1026.7,-9,-9.0,6.8,6.5,98.0,9.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2450,0.2,-9.00,-9,7.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28762 |
-202310120800,281,0,0.3,-9,-9.0,-9,1015.4,1027.2,-9,-9.0,8.8,7.0,89.0,10.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,2881,1.0,-9.00,-9,15.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28763 |
-202310120900,281,0,0.3,-9,-9.0,-9,1015.7,1027.3,2,0.7,12.7,8.1,74.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3468,1.0,-9.00,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28764 |
-202310121000,281,27,0.9,-9,-9.0,-9,1015.4,1026.9,-9,-9.0,16.3,8.5,60.0,11.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4293,1.0,-9.00,-9,28.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28765 |
-202310121100,281,18,0.8,-9,-9.0,-9,1014.9,1026.2,-9,-9.0,18.6,9.0,54.0,11.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4727,1.0,-9.00,-9,33.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28766 |
-202310121200,281,34,1.0,-9,-9.0,-9,1013.9,1025.2,7,-2.1,20.4,7.7,44.0,10.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,36.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28767 |
-202310121300,281,25,1.2,-9,-9.0,-9,1012.5,1023.7,-9,-9.0,22.0,8.1,41.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,5000,1.0,-9.00,-9,38.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28768 |
-202310121400,281,32,1.5,-9,-9.0,-9,1011.7,1022.9,-9,-9.0,21.9,8.3,42.0,10.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,1,22,-,-9,-9,-9,5000,0.9,-9.00,-9,29.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28769 |
-202310121500,281,9,1.2,11,11.0,1437,1011.2,1022.3,7,-2.9,22.3,8.4,41.0,11.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,18,-,-9,-9,-9,4985,0.9,-9.00,-9,33.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28770 |
-202310121600,281,9,2.2,-9,-9.0,-9,1011.2,1022.4,-9,-9.0,21.6,9.7,47.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,5000,0.8,-9.00,-9,26.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28771 |
-202310121700,281,9,2.8,-9,-9.0,-9,1011.4,1022.7,-9,-9.0,20.1,10.2,53.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4946,0.4,-9.00,-9,20.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28772 |
-202310121800,281,7,1.5,-9,-9.0,-9,1011.6,1022.9,2,0.6,17.8,10.4,62.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4589,0.4,-9.00,-9,16.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28773 |
-202310121900,281,7,0.8,-9,-9.0,-9,1012.0,1023.4,-9,-9.0,16.5,10.5,68.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3680,-9.0,-9.00,-9,14.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28774 |
-202310122000,281,0,0.1,-9,-9.0,-9,1012.3,1023.8,-9,-9.0,14.5,10.8,79.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3220,-9.0,-9.00,-9,12.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28775 |
-202310122100,281,0,0.2,-9,-9.0,-9,1012.5,1024.1,2,1.2,13.3,10.6,84.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,3149,-9.0,-9.00,-9,12.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28776 |
-202310122200,281,0,0.4,-9,-9.0,-9,1012.6,1024.2,-9,-9.0,12.2,10.7,91.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2723,-9.0,-9.00,-9,11.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28777 |
-202310122300,281,11,0.7,-9,-9.0,-9,1012.2,1023.8,-9,-9.0,11.8,10.6,93.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2393,-9.0,-9.00,-9,12.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28778 |
-202310130000,281,0,0.4,-9,-9.0,-9,1011.9,1023.5,8,-0.6,11.7,10.5,93.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,2574,-9.0,-9.00,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28779 |
-202310130100,281,9,0.9,-9,-9.0,-9,1011.5,1023.1,-9,-9.0,11.7,10.7,94.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,2745,-9.0,-9.00,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28780 |
-202310130200,281,5,0.5,-9,-9.0,-9,1011.4,1023.1,-9,-9.0,11.4,10.4,94.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,1,18,-,-9,-9,-9,2686,-9.0,-9.00,-9,11.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28781 |
-202310130300,281,0,0.2,-9,-9.0,-9,1011.0,1022.7,7,-0.8,11.2,10.5,96.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,2,34,-,-9,-9,-9,2586,-9.0,-9.00,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28782 |
-202310130400,281,0,0.2,-9,-9.0,-9,1010.7,1022.3,-9,-9.0,11.3,10.6,96.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,58,-,-9,-9,-9,2684,-9.0,-9.00,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28783 |
-202310130500,281,9,0.5,-9,-9.0,-9,1010.3,1022.0,-9,-9.0,10.8,10.3,97.0,12.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,2251,-9.0,-9.00,-9,10.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28784 |
-202310130600,281,0,0.2,-9,-9.0,-9,1010.1,1021.8,7,-0.9,10.4,10.0,98.0,12.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,-9,-,-9,-9,-9,1845,-9.0,-9.00,-9,10.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28785 |
-202310130700,281,5,0.6,-9,-9.0,-9,1010.2,1021.8,-9,-9.0,11.2,10.5,96.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,2272,0.0,-9.00,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28786 |
-202310130800,281,9,0.9,-9,-9.0,-9,1010.3,1021.9,-9,-9.0,11.6,10.9,96.0,13.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,3,65,-,-9,-9,-9,1828,0.0,-9.00,-9,14.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28787 |
-202310130900,281,9,0.6,-9,-9.0,-9,1010.3,1021.8,4,0.0,14.0,11.5,85.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2680,0.5,-9.00,-9,22.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28788 |
-202310131000,281,11,0.8,-9,-9.0,-9,1009.5,1020.8,-9,-9.0,17.2,11.6,70.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,1,70,-,-9,-9,-9,3316,1.0,-9.00,-9,29.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,2, |
|
28789 |
-202310131100,281,23,0.9,-9,-9.0,-9,1008.5,1019.7,-9,-9.0,19.7,11.1,58.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,3550,1.0,-9.00,-9,32.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28790 |
-202310131200,281,16,1.6,-9,-9.0,-9,1007.6,1018.8,7,-3.0,21.0,9.2,47.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,2,65,-,-9,-9,-9,4894,1.0,-9.00,-9,36.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28791 |
-202310131300,281,23,1.0,-9,-9.0,-9,1006.3,1017.4,-9,-9.0,22.1,9.9,46.0,12.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,1,74,-,-9,-9,-9,4854,1.0,-9.00,-9,38.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28792 |
-202310131400,281,14,1.6,-9,-9.0,-9,1005.0,1016.1,-9,-9.0,22.2,9.3,44.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,3,59,-,-9,-9,-9,4899,1.0,-9.00,-9,37.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28793 |
-202310131500,281,18,0.7,-9,-9.0,-9,1004.5,1015.6,7,-3.2,21.6,8.8,44.0,11.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,1,17,-,-9,-9,-9,4523,0.4,-9.00,-9,27.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28794 |
-202310131600,281,11,1.5,-9,-9.0,-9,1004.1,1015.2,-9,-9.0,21.8,9.6,46.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,17,-,-9,-9,-9,4727,0.5,-9.00,-9,26.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28795 |
-202310131700,281,9,0.8,-9,-9.0,-9,1003.8,1014.9,-9,-9.0,20.8,10.5,52.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,3,17,-,-9,-9,-9,3865,0.0,-9.00,-9,21.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28796 |
-202310131800,281,9,1.1,-9,-9.0,-9,1004.0,1015.2,5,-0.4,19.1,11.6,62.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,17,-,-9,-9,-9,3617,0.0,-9.00,-9,18.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28797 |
-202310131900,281,7,0.8,-9,-9.0,-9,1004.0,1015.3,-9,-9.0,17.5,12.1,71.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,46,-,-9,-9,-9,2412,-9.0,-9.00,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28798 |
-202310132000,281,7,1.2,-9,-9.0,-9,1004.0,1015.3,-9,-9.0,16.5,12.2,76.0,14.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,49,-,-9,-9,-9,2823,-9.0,-9.00,-9,15.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28799 |
-202310132100,281,0,0.4,-9,-9.0,-9,1004.1,1015.5,2,0.3,15.7,12.4,81.0,14.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,48,-,-9,-9,-9,2624,-9.0,-9.00,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28800 |
-202310132200,281,0,0.3,-9,-9.0,-9,1004.2,1015.6,-9,-9.0,15.1,12.5,85.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,43,-,-9,-9,-9,1727,-9.0,-9.00,-9,15.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28801 |
-202310132300,281,0,0.2,-9,-9.0,-9,1003.9,1015.3,-9,-9.0,14.9,12.9,88.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,1,18,-,-9,-9,-9,1396,-9.0,-9.00,-9,15.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28802 |
-202310140000,281,11,0.5,-9,-9.0,-9,1003.3,1014.7,8,-0.8,14.5,13.0,91.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,3,17,-,-9,-9,-9,1398,-9.0,-9.00,-9,14.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28803 |
-202310140100,281,7,0.6,-9,-9.0,-9,1002.7,1014.1,-9,-9.0,14.1,12.9,93.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,43,-,-9,-9,-9,1835,-9.0,-9.00,-9,14.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28804 |
-202310140200,281,0,0.3,-9,-9.0,-9,1002.4,1013.8,-9,-9.0,13.9,12.9,94.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,43,-,-9,-9,-9,1997,-9.0,-9.00,-9,14.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28805 |
-202310140300,281,0,0.4,-9,-9.0,-9,1002.0,1013.4,7,-1.3,13.4,12.7,96.0,14.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,42,-,-9,-9,-9,1975,-9.0,-9.00,-9,13.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28806 |
-202310140400,281,0,0.1,-9,-9.0,-9,1001.3,1012.7,-9,-9.0,13.2,12.7,97.0,14.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,41,-,-9,-9,-9,1939,-9.0,-9.00,-9,13.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28807 |
-202310140500,281,0,0.3,-9,-9.0,-9,1001.4,1012.9,-9,-9.0,12.6,12.2,98.0,14.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,-9,-,-9,-9,-9,1710,-9.0,-9.00,-9,11.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28808 |
-202310140600,281,0,0.1,-9,-9.0,-9,1001.2,1012.7,6,-0.7,12.1,11.9,99.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,42,-,-9,-9,-9,1047,-9.0,-9.00,-9,12.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28809 |
-202310140700,281,0,0.1,-9,-9.0,-9,1001.2,1012.7,-9,-9.0,11.7,11.5,99.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,46,-,-9,-9,-9,1495,0.0,-9.00,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28810 |
-202310140800,281,0,0.1,-9,-9.0,-9,1001.4,1012.9,-9,-9.0,12.8,12.1,96.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1656,0.3,-9.00,-9,17.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28811 |
-202310140900,281,9,0.7,-9,-9.0,-9,1001.3,1012.7,4,0.0,15.2,12.6,85.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2053,0.9,-9.00,-9,22.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28812 |
-202310141000,281,7,0.8,-9,-9.0,-9,1000.9,1012.1,-9,-9.0,17.8,12.4,71.0,14.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2276,1.0,-9.00,-9,30.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28813 |
-202310141100,281,25,1.4,-9,-9.0,-9,1000.3,1011.4,-9,-9.0,20.2,11.9,59.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,2,40,-,-9,-9,-9,2311,1.0,-9.00,-9,31.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28814 |
-202310141200,281,25,1.6,-9,-9.0,-9,999.1,1010.1,7,-2.6,22.4,10.5,47.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,35,-,-9,-9,-9,2750,1.0,-9.00,-9,38.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28815 |
-202310141300,281,29,2.6,-9,-9.0,-9,998.1,1009.1,-9,-9.0,22.0,11.0,50.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,5,15,-,-9,-9,-9,2717,0.7,-9.00,-9,30.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28816 |
-202310141400,281,27,2.2,-9,-9.0,-9,997.1,1008.1,-9,-9.0,22.2,9.3,44.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,7,15,-,-9,-9,-9,3922,0.3,-9.00,-9,28.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28817 |
-202310141500,281,32,2.2,-9,-9.0,-9,996.6,1007.6,7,-2.5,22.2,9.6,45.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,6,17,-,-9,-9,-9,3346,0.2,-9.00,-9,26.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28818 |
-202310141600,281,32,2.5,-9,-9.0,-9,997.1,1008.3,-9,-9.0,17.6,13.5,77.0,15.5,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,20,-,-9,-9,-9,1265,0.0,-9.00,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28819 |
-202310141700,281,11,0.9,-9,-9.0,-9,997.0,1008.2,-9,-9.0,16.8,13.5,81.0,15.5,0.6,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,22,-,-9,-9,-9,2281,0.0,-9.00,-9,18.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28820 |
-202310141800,281,11,0.7,-9,-9.0,-9,996.7,1008.0,0,0.4,15.8,14.4,92.0,16.4,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,3,18,-,-9,-9,-9,1386,0.1,-9.00,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28821 |
-202310141900,281,0,0.2,-9,-9.0,-9,997.0,1008.4,-9,-9.0,14.9,14.5,98.0,16.5,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,2,17,-,-9,-9,-9,702,-9.0,-9.00,-9,14.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28822 |
-202310142000,281,7,0.8,-9,-9.0,-9,997.5,1008.9,-9,-9.0,13.9,13.7,99.0,15.7,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,16,-,-9,-9,-9,784,-9.0,-9.00,-9,14.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28823 |
-202310142100,281,32,1.9,-9,-9.0,-9,998.1,1009.5,2,1.5,13.9,13.8,100.0,15.8,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,6,-,-9,-9,-9,673,-9.0,-9.00,-9,14.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28824 |
-202310142200,281,20,0.6,-9,-9.0,-9,998.1,1009.5,-9,-9.0,14.0,12.8,93.0,14.8,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,2,47,-,-9,-9,-9,1793,-9.0,-9.00,-9,12.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28825 |
-202310142300,281,0,0.4,-9,-9.0,-9,998.1,1009.5,-9,-9.0,12.3,12.1,99.0,14.1,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,928,-9.0,-9.00,-9,11.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28826 |
-202310150000,281,0,0.4,-9,-9.0,-9,997.7,1009.2,7,-0.3,12.0,11.9,100.0,13.9,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,701,-9.0,-9.00,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28827 |
-202310150100,281,0,0.3,-9,-9.0,-9,997.6,1009.1,-9,-9.0,10.9,10.8,100.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,470,-9.0,-9.00,-9,10.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28828 |
-202310150200,281,0,0.4,-9,-9.0,-9,997.6,1009.1,-9,-9.0,10.7,10.6,100.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,13,-,-9,-9,-9,124,-9.0,-9.00,-9,12.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28829 |
-202310150300,281,9,0.7,-9,-9.0,-9,997.5,1009.0,7,-0.2,10.6,10.5,100.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,2,18,-,-9,-9,-9,397,-9.0,-9.00,-9,11.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28830 |
-202310150400,281,29,1.1,-9,-9.0,-9,997.5,1009.0,-9,-9.0,10.9,10.8,100.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,731,-9.0,-9.00,-9,10.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28831 |
-202310150500,281,25,0.7,-9,-9.0,-9,997.8,1009.4,-9,-9.0,10.4,10.3,100.0,12.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,92,-9.0,-9.00,-9,9.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28832 |
-202310150600,281,18,0.7,-9,-9.0,-9,998.4,1010.0,2,1.0,10.3,10.2,100.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,528,-9.0,-9.00,-9,9.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28833 |
-202310150700,281,27,1.1,-9,-9.0,-9,998.7,1010.2,-9,-9.0,12.1,11.1,94.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,1104,0.0,-9.00,-9,10.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28834 |
-202310150800,281,23,1.0,-9,-9.0,-9,999.1,1010.5,-9,-9.0,13.5,11.1,86.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1340,1.0,-9.00,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28835 |
-202310150900,281,27,2.1,-9,-9.0,-9,999.4,1010.8,2,0.8,15.2,11.1,77.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1496,0.9,-9.00,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28836 |
-202310151000,281,29,3.7,-9,-9.0,-9,999.5,1010.7,-9,-9.0,17.6,10.9,65.0,13.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2247,1.0,-9.00,-9,26.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28837 |
-202310151100,281,29,3.9,-9,-9.0,-9,999.4,1010.5,-9,-9.0,18.9,10.9,60.0,13.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2998,1.0,-9.00,-9,29.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28838 |
-202310151200,281,29,3.1,-9,-9.0,-9,999.1,1010.2,7,-0.6,19.5,11.2,59.0,13.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,10,-,-9,-9,-9,3183,0.8,-9.00,-9,30.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28839 |
-202310151300,281,32,2.6,-9,-9.0,-9,998.6,1009.7,-9,-9.0,19.9,10.8,56.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,6,13,-,-9,-9,-9,4313,0.4,-9.00,-9,29.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28840 |
-202310151400,281,29,2.7,-9,-9.0,-9,998.2,1009.3,-9,-9.0,21.3,10.1,49.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,13,-,-9,-9,-9,4712,0.7,-9.00,-9,31.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28841 |
-202310151500,281,29,3.6,29,10.0,1420,998.1,1009.2,7,-1.0,20.7,9.2,48.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,15,-,-9,-9,-9,4780,0.8,-9.00,-9,27.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28842 |
-202310151600,281,29,3.1,-9,-9.0,-9,998.6,1009.7,-9,-9.0,20.4,9.6,50.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,4633,0.8,-9.00,-9,24.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28843 |
-202310151700,281,29,2.6,-9,-9.0,-9,998.8,1009.9,-9,-9.0,19.4,9.2,52.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,4060,0.2,-9.00,-9,18.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28844 |
-202310151800,281,29,1.0,-9,-9.0,-9,999.4,1010.7,2,1.5,16.8,10.6,67.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3401,0.4,-9.00,-9,14.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28845 |
-202310151900,281,29,1.5,-9,-9.0,-9,1000.0,1011.3,-9,-9.0,14.6,10.6,77.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2589,-9.0,-9.00,-9,13.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28846 |
-202310152000,281,27,2.5,-9,-9.0,-9,1000.4,1011.8,-9,-9.0,15.3,10.4,73.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2430,-9.0,-9.00,-9,12.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28847 |
-202310152100,281,29,1.7,-9,-9.0,-9,1000.7,1012.1,2,1.4,14.2,10.4,78.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2120,-9.0,-9.00,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28848 |
-202310152200,281,27,1.4,-9,-9.0,-9,1001.2,1012.6,-9,-9.0,14.8,9.7,72.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2480,-9.0,-9.00,-9,11.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28849 |
-202310152300,281,27,2.5,-9,-9.0,-9,1001.4,1012.8,-9,-9.0,15.3,9.4,68.0,11.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2787,-9.0,-9.00,-9,11.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28850 |
-202310160000,281,27,2.6,-9,-9.0,-9,1001.5,1012.9,2,0.8,14.5,9.5,72.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,2479,-9.0,-9.00,-9,11.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28851 |
-202310160100,281,29,1.5,-9,-9.0,-9,1002.1,1013.5,-9,-9.0,13.7,9.1,74.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2565,-9.0,-9.00,-9,10.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28852 |
-202310160200,281,29,1.3,-9,-9.0,-9,1002.4,1013.9,-9,-9.0,12.0,9.3,84.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1985,-9.0,-9.00,-9,10.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28853 |
-202310160300,281,0,0.3,-9,-9.0,-9,1002.3,1013.9,1,1.0,11.3,9.5,89.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,1558,-9.0,-9.00,-9,9.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28854 |
-202310160400,281,0,0.0,-9,-9.0,-9,1001.9,1013.5,-9,-9.0,10.2,9.1,93.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1266,-9.0,-9.00,-9,9.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28855 |
-202310160500,281,18,0.7,-9,-9.0,-9,1002.4,1014.0,-9,-9.0,10.1,8.6,91.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,1507,-9.0,-9.00,-9,8.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28856 |
-202310160600,281,7,0.5,-9,-9.0,-9,1002.8,1014.4,3,0.5,9.0,8.6,98.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,1015,-9.0,-9.00,-9,8.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28857 |
-202310160700,281,0,0.1,-9,-9.0,-9,1003.2,1014.8,-9,-9.0,9.6,9.1,97.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,11,-,-9,-9,-9,960,0.0,-9.00,-9,9.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28858 |
-202310160800,281,0,0.1,-9,-9.0,-9,1004.0,1015.5,-9,-9.0,11.7,9.9,89.0,12.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1162,0.9,-9.00,-9,16.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28859 |
-202310160900,281,29,2.0,-9,-9.0,-9,1004.3,1015.6,2,1.2,17.0,11.4,70.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,2174,0.9,-9.00,-9,20.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28860 |
-202310161000,281,27,3.8,-9,-9.0,-9,1004.1,1015.3,-9,-9.0,18.8,9.8,56.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2598,1.0,-9.00,-9,28.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28861 |
-202310161100,281,36,2.8,-9,-9.0,-9,1003.9,1015.1,-9,-9.0,18.5,9.2,55.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,11,-,-9,-9,-9,3071,0.7,-9.00,-9,30.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28862 |
-202310161200,281,32,3.2,-9,-9.0,-9,1003.5,1014.7,7,-0.9,20.1,8.1,46.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,12,-,-9,-9,-9,3435,0.9,-9.00,-9,34.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28863 |
-202310161300,281,32,3.0,-9,-9.0,-9,1002.7,1013.8,-9,-9.0,20.8,8.7,46.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,1,54,-,-9,-9,-9,3734,0.9,-9.00,-9,34.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28864 |
-202310161400,281,29,3.0,-9,-9.0,-9,1002.3,1013.4,-9,-9.0,21.4,7.2,40.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4031,1.0,-9.00,-9,34.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28865 |
-202310161500,281,27,2.0,-9,-9.0,-9,1002.4,1013.5,5,-1.2,21.3,6.7,39.0,9.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4483,1.0,-9.00,-9,30.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28866 |
-202310161600,281,27,3.2,-9,-9.0,-9,1002.5,1013.7,-9,-9.0,20.4,6.6,41.0,9.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4366,1.0,-9.00,-9,25.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28867 |
-202310161700,281,29,2.6,-9,-9.0,-9,1002.6,1013.7,-9,-9.0,19.6,7.3,45.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4196,0.3,-9.00,-9,18.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28868 |
-202310161800,281,29,1.3,-9,-9.0,-9,1002.9,1014.2,2,0.7,16.5,8.9,61.0,11.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3686,0.4,-9.00,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28869 |
-202310161900,281,29,1.8,-9,-9.0,-9,1003.2,1014.6,-9,-9.0,14.4,8.7,69.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2928,-9.0,-9.00,-9,12.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28870 |
-202310162000,281,7,0.9,-9,-9.0,-9,1003.7,1015.2,-9,-9.0,12.7,9.1,79.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2143,-9.0,-9.00,-9,11.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28871 |
-202310162100,281,0,0.2,-9,-9.0,-9,1004.2,1015.8,2,1.6,11.6,9.1,85.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1854,-9.0,-9.00,-9,10.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28872 |
-202310162200,281,0,0.2,-9,-9.0,-9,1004.7,1016.3,-9,-9.0,10.4,8.6,89.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1925,-9.0,-9.00,-9,9.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28873 |
-202310162300,281,29,2.1,-9,-9.0,-9,1004.8,1016.3,-9,-9.0,12.7,8.1,74.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2750,-9.0,-9.00,-9,9.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28874 |
-202310170000,281,32,3.2,-9,-9.0,-9,1005.1,1016.6,2,0.8,13.3,6.8,65.0,9.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3909,-9.0,-9.00,-9,10.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28875 |
-202310170100,281,27,2.1,-9,-9.0,-9,1005.6,1017.1,-9,-9.0,12.1,6.3,68.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3949,-9.0,-9.00,-9,9.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28876 |
-202310170200,281,29,2.0,-9,-9.0,-9,1005.8,1017.4,-9,-9.0,12.0,4.9,62.0,8.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4874,-9.0,-9.00,-9,8.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28348 |
+202309250200,281,32,1.9,-9,-9.0,-9,1007.1,1018.4,-9,-9.0,17.9,14.9,83.0,16.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,29,-,-9,-9,-9,5000,-9.0,-9.0,-9,17.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28349 |
+202309250300,281,29,1.0,-9,-9.0,-9,1006.6,1017.9,7,-1.4,17.7,15.1,85.0,17.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,14,-,-9,-9,-9,5000,-9.0,-9.0,-9,17.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28350 |
+202309250400,281,29,1.4,-9,-9.0,-9,1006.5,1017.8,-9,-9.0,17.6,15.2,86.0,17.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,4,11,-,-9,-9,-9,5000,-9.0,-9.0,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28351 |
+202309250500,281,29,1.4,-9,-9.0,-9,1006.5,1017.8,-9,-9.0,17.8,14.6,82.0,16.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,10,-,-9,-9,-9,5000,-9.0,-9.0,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28352 |
+202309250600,281,27,1.2,-9,-9.0,-9,1006.9,1018.2,3,0.3,17.6,14.8,84.0,16.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,11,-,-9,-9,-9,5000,-9.0,-9.0,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28353 |
+202309250700,281,0,0.0,-9,-9.0,-9,1007.1,1018.4,-9,-9.0,17.8,15.6,87.0,17.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,13,-,-9,-9,-9,4979,0.0,-9.0,-9,18.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28354 |
+202309250800,281,32,0.8,-9,-9.0,-9,1007.2,1018.4,-9,-9.0,18.3,15.1,82.0,17.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,33,-,-9,-9,-9,5000,0.0,-9.0,-9,19.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28355 |
+202309250900,281,0,0.2,-9,-9.0,-9,1007.0,1018.2,4,0.0,19.4,15.4,78.0,17.5,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,28,-,-9,-9,-9,4264,0.0,-9.0,-9,22.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28356 |
+202309251000,281,0,0.3,-9,-9.0,-9,1007.2,1018.3,-9,-9.0,21.8,15.8,69.0,17.9,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,9,-,-9,-9,-9,4880,0.1,-9.0,-9,29.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28357 |
+202309251100,281,5,0.9,-9,-9.0,-9,1006.6,1017.6,-9,-9.0,22.9,16.4,67.0,18.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,9,-,-9,-9,-9,4890,0.1,-9.0,-9,29.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28358 |
+202309251200,281,7,2.7,-9,-9.0,-9,1006.3,1017.3,8,-0.9,23.4,17.1,68.0,19.5,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,9,-,-9,-9,-9,5000,0.0,-9.0,-9,27.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28359 |
+202309251300,281,9,2.7,-9,-9.0,-9,1005.4,1016.4,-9,-9.0,23.8,17.2,67.0,19.6,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,8,-,-9,-9,-9,5000,0.1,-9.0,-9,31.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28360 |
+202309251400,281,11,2.1,-9,-9.0,-9,1005.1,1016.1,-9,-9.0,23.4,17.8,71.0,20.4,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,8,-,-9,-9,-9,5000,0.0,-9.0,-9,27.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28361 |
+202309251500,281,11,2.4,-9,-9.0,-9,1004.6,1015.5,7,-1.8,24.5,17.7,66.0,20.2,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,12,-,-9,-9,-9,4924,0.2,-9.0,-9,35.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28362 |
+202309251600,281,9,2.0,-9,-9.0,-9,1004.5,1015.5,-9,-9.0,23.4,17.3,69.0,19.7,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,12,-,-9,-9,-9,5000,0.2,-9.0,-9,26.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28363 |
+202309251700,281,9,2.1,-9,-9.0,-9,1004.5,1015.5,-9,-9.0,23.0,17.6,72.0,20.1,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,8,-,-9,-9,-9,5000,0.0,-9.0,-9,24.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28364 |
+202309251800,281,11,1.6,-9,-9.0,-9,1004.7,1015.8,2,0.3,22.4,18.1,77.0,20.8,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,1,13,-,-9,-9,-9,4970,0.0,-9.0,-9,22.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28365 |
+202309251900,281,11,0.9,-9,-9.0,-9,1004.8,1015.9,-9,-9.0,21.5,18.0,81.0,20.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,3,14,-,-9,-9,-9,3136,0.0,-9.0,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28366 |
+202309252000,281,9,0.5,-9,-9.0,-9,1005.2,1016.3,-9,-9.0,21.1,17.8,82.0,20.4,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,12,-,-9,-9,-9,4857,-9.0,-9.0,-9,20.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28367 |
+202309252100,281,0,0.1,-9,-9.0,-9,1005.6,1016.8,2,1.0,20.3,18.5,90.0,21.3,0.1,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,22,-,-9,-9,-9,1045,-9.0,-9.0,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28368 |
+202309252200,281,0,0.1,-9,-9.0,-9,1005.6,1016.8,-9,-9.0,20.1,19.2,95.0,22.2,0.4,0.5,0.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,22,-,-9,-9,-9,793,-9.0,-9.0,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28369 |
+202309252300,281,0,0.2,-9,-9.0,-9,1005.4,1016.5,-9,-9.0,19.7,19.5,99.0,22.7,0.4,0.9,0.9,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,11,-,-9,-9,-9,2458,-9.0,-9.0,-9,20.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28370 |
+202309260000,281,0,0.4,-9,-9.0,-9,1004.7,1015.8,7,-1.0,19.5,19.4,100.0,22.5,0.6,1.5,1.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,12,-,-9,-9,-9,1228,-9.0,-9.0,-9,20.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28371 |
+202309260100,281,27,0.8,-9,-9.0,-9,1004.3,1015.4,-9,-9.0,19.4,19.3,100.0,22.4,0.1,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,4,-,-9,-9,-9,1772,-9.0,-9.0,-9,20.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28372 |
+202309260200,281,0,0.3,-9,-9.0,-9,1003.9,1015.0,-9,-9.0,19.3,19.2,100.0,22.2,-9.0,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,23,-,-9,-9,-9,2173,-9.0,-9.0,-9,20.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28373 |
+202309260300,281,0,0.3,-9,-9.0,-9,1003.7,1014.8,7,-1.0,19.5,19.4,100.0,22.5,-9.0,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,10,-,-9,-9,-9,2389,-9.0,-9.0,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28374 |
+202309260400,281,0,0.0,-9,-9.0,-9,1003.2,1014.3,-9,-9.0,19.5,19.4,100.0,22.5,-9.0,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,6,-,-9,-9,-9,2634,-9.0,-9.0,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28375 |
+202309260500,281,0,0.3,-9,-9.0,-9,1003.2,1014.3,-9,-9.0,19.6,19.4,99.0,22.5,0.1,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,4,-,-9,-9,-9,2164,-9.0,-9.0,-9,20.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28376 |
+202309260600,281,0,0.3,-9,-9.0,-9,1003.5,1014.6,5,-0.2,19.5,19.4,100.0,22.5,1.1,1.3,1.3,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,4,-,-9,-9,-9,1192,-9.0,-9.0,-9,20.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28377 |
+202309260700,281,27,0.6,-9,-9.0,-9,1004.3,1015.4,-9,-9.0,19.5,19.4,100.0,22.5,4.8,6.1,6.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,8,-,-9,-9,-9,175,0.0,-9.0,-9,19.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28378 |
+202309260800,281,5,0.9,-9,-9.0,-9,1004.3,1015.4,-9,-9.0,19.7,19.6,100.0,22.8,7.1,13.2,13.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,42,-,-9,-9,-9,549,0.0,-9.0,-9,20.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28379 |
+202309260900,281,5,1.1,-9,-9.0,-9,1004.4,1015.6,2,1.0,20.0,19.9,100.0,23.2,3.1,16.3,16.3,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,1,-,-9,-9,-9,474,0.0,-9.0,-9,21.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28380 |
+202309261000,281,7,1.0,-9,-9.0,-9,1004.1,1015.3,-9,-9.0,20.1,20.0,100.0,23.4,2.7,19.0,19.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,-9,-,-9,-9,-9,1687,0.0,-9.0,-9,22.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28381 |
+202309261100,281,9,0.9,-9,-9.0,-9,1003.5,1014.6,-9,-9.0,20.8,20.7,100.0,24.4,0.4,19.4,19.4,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,3,-,-9,-9,-9,1717,0.0,-9.0,-9,23.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28382 |
+202309261200,281,11,0.9,-9,-9.0,-9,1003.3,1014.4,7,-1.2,21.0,20.6,98.0,24.3,0.3,19.7,19.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,4,-,-9,-9,-9,4694,0.0,-9.0,-9,22.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28383 |
+202309261300,281,9,1.4,-9,-9.0,-9,1002.7,1013.8,-9,-9.0,20.7,20.2,97.0,23.7,0.1,19.8,19.8,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,6,-,-9,-9,-9,4207,0.0,-9.0,-9,22.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28384 |
+202309261400,281,7,1.4,-9,-9.0,-9,1002.0,1013.1,-9,-9.0,20.7,20.5,99.0,24.1,0.2,20.0,20.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,18,-,-9,-9,-9,3138,0.0,-9.0,-9,22.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28385 |
+202309261500,281,9,1.0,-9,-9.0,-9,1001.7,1012.8,7,-1.6,20.7,20.5,99.0,24.1,0.2,20.2,20.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,4,-,-9,-9,-9,836,0.0,-9.0,-9,21.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28386 |
+202309261600,281,7,1.3,-9,-9.0,-9,1001.5,1012.6,-9,-9.0,20.6,20.5,100.0,24.1,0.5,20.7,20.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,5,-,-9,-9,-9,1328,0.0,-9.0,-9,22.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28387 |
+202309261700,281,9,0.8,-9,-9.0,-9,1001.4,1012.5,-9,-9.0,20.6,20.5,100.0,24.1,0.1,20.8,20.8,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,-9,-,-9,-9,-9,1334,0.0,-9.0,-9,21.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28388 |
+202309261800,281,7,1.2,-9,-9.0,-9,1001.6,1012.7,5,-0.1,20.5,20.4,100.0,24.0,0.3,21.1,21.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,582,0.0,-9.0,-9,21.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28389 |
+202309261900,281,7,0.9,-9,-9.0,-9,1001.5,1012.6,-9,-9.0,20.5,20.4,100.0,24.0,0.5,21.6,21.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,4,5,-,-9,-9,-9,764,0.0,-9.0,-9,21.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28390 |
+202309262000,281,36,0.6,-9,-9.0,-9,1001.7,1012.8,-9,-9.0,20.6,20.5,100.0,24.1,0.4,22.0,22.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,-9,-,-9,-9,-9,294,-9.0,-9.0,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28391 |
+202309262100,281,0,0.4,-9,-9.0,-9,1001.9,1013.0,3,0.3,20.5,20.4,100.0,24.0,1.0,23.0,23.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,1,-,-9,-9,-9,487,-9.0,-9.0,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28392 |
+202309262200,281,34,0.8,-9,-9.0,-9,1002.0,1013.1,-9,-9.0,20.5,20.4,100.0,24.0,0.5,23.5,23.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,3,-,-9,-9,-9,1381,-9.0,-9.0,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28393 |
+202309262300,281,29,0.5,-9,-9.0,-9,1002.0,1013.1,-9,-9.0,20.6,20.5,100.0,24.1,-9.0,23.5,23.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,3,-,-9,-9,-9,1125,-9.0,-9.0,-9,21.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28394 |
+202309270000,281,0,0.3,-9,-9.0,-9,1001.5,1012.6,8,-0.4,20.6,20.5,100.0,24.1,-9.0,23.5,23.5,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,3,-,-9,-9,-9,892,-9.0,-9.0,-9,21.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28395 |
+202309270100,281,0,0.0,-9,-9.0,-9,1001.0,1012.1,-9,-9.0,20.4,20.3,100.0,23.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,1,-,-9,-9,-9,727,-9.0,-9.0,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28396 |
+202309270200,281,27,1.1,-9,-9.0,-9,1001.0,1012.1,-9,-9.0,20.5,20.4,100.0,24.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,4,-,-9,-9,-9,515,-9.0,-9.0,-9,20.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28397 |
+202309270300,281,0,0.2,-9,-9.0,-9,1000.7,1011.8,7,-0.8,20.3,20.2,100.0,23.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,5,3,-,-9,-9,-9,690,-9.0,-9.0,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28398 |
+202309270400,281,25,0.7,-9,-9.0,-9,1000.6,1011.7,-9,-9.0,20.3,20.2,100.0,23.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,1,-,-9,-9,-9,712,-9.0,-9.0,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28399 |
+202309270500,281,0,0.2,-9,-9.0,-9,1000.5,1011.6,-9,-9.0,20.3,20.2,100.0,23.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,-9,-,-9,-9,-9,432,-9.0,-9.0,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28400 |
+202309270600,281,0,0.1,-9,-9.0,-9,1000.7,1011.8,4,0.0,20.4,20.3,100.0,23.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,-9,-,-9,-9,-9,573,-9.0,-9.0,-9,20.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28401 |
+202309270700,281,0,0.3,-9,-9.0,-9,1001.1,1012.2,-9,-9.0,20.5,20.4,100.0,24.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,-9,-,-9,-9,-9,502,0.0,-9.0,-9,21.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28402 |
+202309270800,281,7,0.7,-9,-9.0,-9,1001.4,1012.5,-9,-9.0,20.8,20.7,100.0,24.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,-9,-,-9,-9,-9,636,0.0,-9.0,-9,22.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28403 |
+202309270900,281,0,0.4,-9,-9.0,-9,1001.5,1012.6,2,0.8,21.7,21.1,97.0,25.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,1,-,-9,-9,-9,735,0.0,-9.0,-9,24.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28404 |
+202309271000,281,20,0.9,-9,-9.0,-9,1001.3,1012.3,-9,-9.0,23.4,20.9,86.0,24.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,8,3,-,-9,-9,-9,1030,0.1,-9.0,-9,31.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28405 |
+202309271100,281,0,0.4,-9,-9.0,-9,1001.2,1012.1,-9,-9.0,23.9,20.8,83.0,24.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,4,-,-9,-9,-9,1441,0.0,-9.0,-9,31.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28406 |
+202309271200,281,32,1.4,-9,-9.0,-9,1000.6,1011.6,7,-1.0,25.0,21.3,80.0,25.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,8,-,-9,-9,-9,2235,0.2,-9.0,-9,30.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28407 |
+202309271300,281,29,1.5,-9,-9.0,-9,1000.1,1011.0,-9,-9.0,24.7,20.5,78.0,24.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,10,-,-9,-9,-9,3104,0.0,-9.0,-9,27.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28408 |
+202309271400,281,34,0.9,-9,-9.0,-9,999.3,1010.2,-9,-9.0,24.6,21.1,81.0,25.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,11,-,-9,-9,-9,2728,0.0,-9.0,-9,26.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28409 |
+202309271500,281,5,1.2,-9,-9.0,-9,998.9,1009.8,7,-1.8,24.9,20.7,78.0,24.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,17,-,-9,-9,-9,4413,0.0,-9.0,-9,29.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28410 |
+202309271600,281,32,0.7,-9,-9.0,-9,998.9,1009.8,-9,-9.0,25.3,20.7,76.0,24.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,4,11,-,-9,-9,-9,5000,0.0,-9.0,-9,27.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28411 |
+202309271700,281,29,2.1,-9,-9.0,-9,998.9,1009.8,-9,-9.0,24.8,20.0,75.0,23.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,13,-,-9,-9,-9,5000,0.1,-9.0,-9,25.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28412 |
+202309271800,281,29,2.0,-9,-9.0,-9,999.1,1010.0,2,0.2,23.7,20.0,80.0,23.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,10,-,-9,-9,-9,5000,0.1,-9.0,-9,23.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28413 |
+202309271900,281,32,2.0,-9,-9.0,-9,999.5,1010.4,-9,-9.0,23.4,18.9,76.0,21.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,13,-,-9,-9,-9,5000,0.0,-9.0,-9,22.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28414 |
+202309272000,281,29,2.2,-9,-9.0,-9,1000.1,1011.1,-9,-9.0,23.0,19.1,79.0,22.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,12,-,-9,-9,-9,4954,-9.0,-9.0,-9,21.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28415 |
+202309272100,281,27,1.3,-9,-9.0,-9,1000.8,1011.8,2,1.8,22.8,19.3,81.0,22.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,8,-,-9,-9,-9,5000,-9.0,-9.0,-9,21.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28416 |
+202309272200,281,27,1.6,-9,-9.0,-9,1001.1,1012.1,-9,-9.0,22.3,19.0,82.0,22.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,11,-,-9,-9,-9,5000,-9.0,-9.0,-9,21.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28417 |
+202309272300,281,29,1.3,-9,-9.0,-9,1001.1,1012.1,-9,-9.0,22.0,18.9,83.0,21.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,10,-,-9,-9,-9,5000,-9.0,-9.0,-9,21.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28418 |
+202309280000,281,27,1.8,-9,-9.0,-9,1001.0,1012.1,1,0.3,21.8,18.7,83.0,21.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,7,9,-,-9,-9,-9,4592,-9.0,-9.0,-9,21.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28419 |
+202309280100,281,29,1.6,-9,-9.0,-9,1000.5,1011.6,-9,-9.0,21.3,18.4,84.0,21.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,5,9,-,-9,-9,-9,390,-9.0,-9.0,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28420 |
+202309280200,281,27,1.7,-9,-9.0,-9,1000.5,1011.6,-9,-9.0,21.1,19.0,88.0,22.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,8,-,-9,-9,-9,4489,-9.0,-9.0,-9,20.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28421 |
+202309280300,281,27,2.4,-9,-9.0,-9,1000.4,1011.5,7,-0.6,20.8,18.9,89.0,21.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,11,-,-9,-9,-9,3795,-9.0,-9.0,-9,19.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28422 |
+202309280400,281,27,1.8,-9,-9.0,-9,1000.9,1012.0,-9,-9.0,21.0,18.7,87.0,21.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,9,-,-9,-9,-9,4053,-9.0,-9.0,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28423 |
+202309280500,281,25,1.5,-9,-9.0,-9,1001.0,1012.1,-9,-9.0,20.7,18.4,87.0,21.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,6,14,-,-9,-9,-9,3734,-9.0,-9.0,-9,19.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28424 |
+202309280600,281,27,1.9,-9,-9.0,-9,1001.4,1012.6,2,1.1,20.2,18.3,89.0,21.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,15,-,-9,-9,-9,2786,-9.0,-9.0,-9,19.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28425 |
+202309280700,281,29,1.2,-9,-9.0,-9,1002.5,1013.6,-9,-9.0,20.8,18.3,86.0,21.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,4,27,-,-9,-9,-9,2989,0.4,-9.0,-9,19.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28426 |
+202309280800,281,27,2.1,-9,-9.0,-9,1002.9,1014.0,-9,-9.0,22.1,18.2,79.0,20.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,20,-,-9,-9,-9,3305,0.6,-9.0,-9,23.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28427 |
+202309280900,281,29,2.0,-9,-9.0,-9,1003.3,1014.3,2,1.7,23.4,18.0,72.0,20.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,8,-,-9,-9,-9,3442,0.8,-9.0,-9,27.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28428 |
+202309281000,281,27,2.6,-9,-9.0,-9,1003.4,1014.4,-9,-9.0,24.9,18.1,66.0,20.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,11,-,-9,-9,-9,4616,0.7,-9.0,-9,32.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28429 |
+202309281100,281,29,3.0,-9,-9.0,-9,1002.9,1013.9,-9,-9.0,25.5,18.4,65.0,21.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,11,-,-9,-9,-9,4665,0.8,-9.0,-9,34.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28430 |
+202309281200,281,29,3.2,-9,-9.0,-9,1002.6,1013.5,8,-0.8,25.8,17.7,61.0,20.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,11,-,-9,-9,-9,4804,0.8,-9.0,-9,33.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28431 |
+202309281300,281,27,2.8,-9,-9.0,-9,1002.0,1012.8,-9,-9.0,27.7,18.1,56.0,20.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,2,11,-,-9,-9,-9,4901,0.7,-9.0,-9,38.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28432 |
+202309281400,281,27,3.1,-9,-9.0,-9,1001.3,1012.1,-9,-9.0,28.0,17.8,54.0,20.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,2,12,-,-9,-9,-9,4609,1.0,-9.0,-9,37.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28433 |
+202309281500,281,27,3.9,-9,-9.0,-9,1000.9,1011.7,7,-1.8,27.3,16.5,52.0,18.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4193,1.0,-9.0,-9,33.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28434 |
+202309281600,281,29,3.3,-9,-9.0,-9,1001.2,1012.1,-9,-9.0,27.1,16.9,54.0,19.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3986,1.0,-9.0,-9,30.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28435 |
+202309281700,281,27,2.7,-9,-9.0,-9,1001.5,1012.4,-9,-9.0,26.3,16.8,56.0,19.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3555,1.0,-9.0,-9,26.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28436 |
+202309281800,281,29,1.8,-9,-9.0,-9,1001.6,1012.5,2,0.8,24.4,17.1,64.0,19.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2799,0.4,-9.0,-9,22.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28437 |
+202309281900,281,29,1.9,-9,-9.0,-9,1001.8,1012.8,-9,-9.0,23.3,16.8,67.0,19.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,2244,0.0,-9.0,-9,20.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28438 |
+202309282000,281,32,1.4,-9,-9.0,-9,1002.5,1013.6,-9,-9.0,21.3,17.0,77.0,19.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1813,-9.0,-9.0,-9,19.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28439 |
+202309282100,281,7,0.8,-9,-9.0,-9,1003.0,1014.1,2,1.6,19.5,18.1,92.0,20.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1056,-9.0,-9.0,-9,18.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28440 |
+202309282200,281,0,0.2,-9,-9.0,-9,1003.3,1014.5,-9,-9.0,18.6,18.1,97.0,20.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,692,-9.0,-9.0,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28441 |
+202309282300,281,34,0.7,-9,-9.0,-9,1003.5,1014.7,-9,-9.0,18.0,17.6,98.0,20.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,601,-9.0,-9.0,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28442 |
+202309290000,281,0,0.2,-9,-9.0,-9,1003.6,1014.9,2,0.8,17.5,17.3,99.0,19.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,538,-9.0,-9.0,-9,17.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28443 |
+202309290100,281,0,0.4,-9,-9.0,-9,1003.4,1014.7,-9,-9.0,17.1,17.0,100.0,19.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,439,-9.0,-9.0,-9,16.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28444 |
+202309290200,281,27,1.1,-9,-9.0,-9,1003.5,1014.7,-9,-9.0,17.7,17.3,98.0,19.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,529,-9.0,-9.0,-9,16.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28445 |
+202309290300,281,0,0.2,-9,-9.0,-9,1003.4,1014.7,6,-0.2,17.3,17.2,100.0,19.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,483,-9.0,-9.0,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28446 |
+202309290400,281,23,0.8,-9,-9.0,-9,1003.8,1015.1,-9,-9.0,16.6,16.2,98.0,18.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,502,-9.0,-9.0,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28447 |
+202309290500,281,29,0.5,-9,-9.0,-9,1004.4,1015.8,-9,-9.0,15.9,15.8,100.0,17.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,330,-9.0,-9.0,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28448 |
+202309290600,281,20,0.9,-9,-9.0,-9,1004.3,1015.7,0,1.0,15.5,15.4,100.0,17.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,306,-9.0,-9.0,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28449 |
+202309290700,281,0,0.3,-9,-9.0,-9,1004.6,1016.0,-9,-9.0,15.3,15.2,100.0,17.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,327,0.0,-9.0,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28450 |
+202309290800,281,29,0.7,-9,-9.0,-9,1004.8,1016.0,-9,-9.0,18.3,17.4,95.0,19.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,554,0.9,-9.0,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28451 |
+202309290900,281,11,0.7,-9,-9.0,-9,1005.3,1016.4,2,0.7,19.5,17.2,87.0,19.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,711,1.0,-9.0,-9,27.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28452 |
+202309291000,281,18,1.1,-9,-9.0,-9,1005.2,1016.3,-9,-9.0,22.5,16.9,71.0,19.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,3,63,-,-9,-9,-9,921,1.0,-9.0,-9,32.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28453 |
+202309291100,281,36,1.6,-9,-9.0,-9,1004.8,1015.8,-9,-9.0,23.5,16.0,63.0,18.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,75,-,-9,-9,-9,1030,1.0,-9.0,-9,36.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28454 |
+202309291200,281,7,1.1,-9,-9.0,-9,1003.9,1014.9,7,-1.5,24.8,16.5,60.0,18.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,48,-,-9,-9,-9,1361,0.9,-9.0,-9,36.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28455 |
+202309291300,281,9,1.0,-9,-9.0,-9,1003.1,1014.1,-9,-9.0,25.4,15.1,53.0,17.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,6,44,-,-9,-9,-9,1956,0.6,-9.0,-9,36.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28456 |
+202309291400,281,27,1.5,-9,-9.0,-9,1002.4,1013.3,-9,-9.0,26.1,14.8,50.0,16.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,44,-,-9,-9,-9,2215,0.0,-9.0,-9,31.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28457 |
+202309291500,281,32,1.1,-9,-9.0,-9,1002.0,1012.9,7,-2.0,25.7,15.0,52.0,17.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,41,-,-9,-9,-9,2237,0.0,-9.0,-9,28.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28458 |
+202309291600,281,34,0.8,-9,-9.0,-9,1001.5,1012.4,-9,-9.0,25.6,15.5,54.0,17.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,39,-,-9,-9,-9,2305,0.0,-9.0,-9,28.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28459 |
+202309291700,281,11,1.9,-9,-9.0,-9,1001.9,1012.8,-9,-9.0,24.2,17.4,66.0,19.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,42,-,-9,-9,-9,1832,0.0,-9.0,-9,25.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28460 |
+202309291800,281,7,1.5,-9,-9.0,-9,1001.9,1012.9,4,0.0,22.6,17.9,75.0,20.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,8,52,-,-9,-9,-9,1295,0.0,-9.0,-9,22.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28461 |
+202309291900,281,7,1.5,-9,-9.0,-9,1002.0,1013.1,-9,-9.0,22.0,17.9,78.0,20.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,45,-,-9,-9,-9,1238,0.0,-9.0,-9,21.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28462 |
+202309292000,281,9,0.9,-9,-9.0,-9,1001.9,1013.0,-9,-9.0,21.3,18.2,83.0,20.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,4,49,-,-9,-9,-9,1075,-9.0,-9.0,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28463 |
+202309292100,281,0,0.3,-9,-9.0,-9,1002.0,1013.1,1,0.2,20.7,18.6,88.0,21.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,1,68,-,-9,-9,-9,874,-9.0,-9.0,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28464 |
+202309292200,281,29,0.7,-9,-9.0,-9,1002.1,1013.2,-9,-9.0,19.6,18.4,93.0,21.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,5,50,-,-9,-9,-9,1021,-9.0,-9.0,-9,19.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28465 |
+202309292300,281,0,0.2,-9,-9.0,-9,1001.9,1013.0,-9,-9.0,19.5,18.8,96.0,21.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,47,-,-9,-9,-9,874,-9.0,-9.0,-9,19.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28466 |
+202309300000,281,0,0.1,-9,-9.0,-9,1000.8,1011.9,8,-1.2,19.1,18.7,98.0,21.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,45,-,-9,-9,-9,789,-9.0,-9.0,-9,19.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28467 |
+202309300100,281,0,0.4,-9,-9.0,-9,1000.3,1011.4,-9,-9.0,19.1,18.6,97.0,21.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,42,-,-9,-9,-9,869,-9.0,-9.0,-9,19.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28468 |
+202309300200,281,29,1.0,-9,-9.0,-9,1000.4,1011.5,-9,-9.0,19.0,18.5,97.0,21.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,39,-,-9,-9,-9,712,-9.0,-9.0,-9,19.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28469 |
+202309300300,281,32,0.5,-9,-9.0,-9,1000.2,1011.3,7,-0.6,19.1,18.6,97.0,21.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,34,-,-9,-9,-9,699,-9.0,-9.0,-9,19.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28470 |
+202309300400,281,27,1.8,-9,-9.0,-9,1000.8,1011.9,-9,-9.0,19.4,17.5,89.0,20.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,10,-,-9,-9,-9,925,-9.0,-9.0,-9,19.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28471 |
+202309300500,281,27,2.6,-9,-9.0,-9,1000.9,1012.1,-9,-9.0,18.3,16.4,89.0,18.6,0.1,0.1,0.1,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,31,-,-9,-9,-9,757,-9.0,-9.0,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28472 |
+202309300600,281,32,3.0,-9,-9.0,-9,1001.8,1013.1,2,1.8,16.9,14.3,85.0,16.3,0.5,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,28,-,-9,-9,-9,1078,-9.0,-9.0,-9,17.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28473 |
+202309300700,281,29,1.8,-9,-9.0,-9,1002.2,1013.6,-9,-9.0,15.4,14.2,93.0,16.2,1.4,2.0,2.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,22,-,-9,-9,-9,971,0.0,-9.0,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28474 |
+202309300800,281,27,2.9,-9,-9.0,-9,1003.0,1014.4,-9,-9.0,15.0,13.5,91.0,15.5,0.8,2.8,2.8,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,20,-,-9,-9,-9,1293,0.0,-9.0,-9,16.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28475 |
+202309300900,281,0,0.2,-9,-9.0,-9,1002.1,1013.5,0,0.4,15.2,13.3,89.0,15.3,0.9,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,3,18,-,-9,-9,-9,3489,0.0,-9.0,-9,18.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28476 |
+202309301000,281,23,1.4,-9,-9.0,-9,1001.5,1012.8,-9,-9.0,15.8,14.1,90.0,16.1,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,20,-,-9,-9,-9,2223,0.0,-9.0,-9,19.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28477 |
+202309301100,281,32,1.6,-9,-9.0,-9,1001.1,1012.4,-9,-9.0,16.8,14.7,88.0,16.7,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,3,-,-9,-9,-9,2271,0.0,-9.0,-9,21.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28478 |
+202309301200,281,27,1.6,-9,-9.0,-9,1000.4,1011.5,7,-2.0,19.5,15.7,79.0,17.8,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,6,4,-,-9,-9,-9,3171,0.3,-9.0,-9,34.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28479 |
+202309301300,281,27,1.8,-9,-9.0,-9,999.6,1010.7,-9,-9.0,20.9,14.9,69.0,16.9,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,69,-,-9,-9,-9,4538,0.6,-9.0,-9,32.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28480 |
+202309301400,281,25,2.0,-9,-9.0,-9,998.9,1009.9,-9,-9.0,22.4,14.2,60.0,16.2,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,33.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28481 |
+202309301500,281,27,3.0,-9,-9.0,-9,998.3,1009.2,7,-2.3,23.5,13.0,52.0,15.0,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,30.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28482 |
+202309301600,281,29,2.8,-9,-9.0,-9,998.3,1009.2,-9,-9.0,23.6,14.0,55.0,16.0,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,27.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28483 |
+202309301700,281,32,1.5,-9,-9.0,-9,998.4,1009.4,-9,-9.0,23.0,13.7,56.0,15.7,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,23.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28484 |
+202309301800,281,29,1.3,-9,-9.0,-9,998.6,1009.7,2,0.5,21.0,14.6,67.0,16.6,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,5000,0.4,-9.0,-9,19.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28485 |
+202309301900,281,29,1.5,-9,-9.0,-9,998.7,1009.8,-9,-9.0,19.3,14.9,76.0,16.9,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,-9,-,-9,-9,-9,4983,0.0,-9.0,-9,18.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28486 |
+202309302000,281,27,2.8,-9,-9.0,-9,999.3,1010.4,-9,-9.0,20.1,14.8,72.0,16.8,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,3802,-9.0,-9.0,-9,17.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28487 |
+202309302100,281,27,3.0,-9,-9.0,-9,999.5,1010.7,2,1.0,20.0,14.7,72.0,16.7,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,3654,-9.0,-9.0,-9,17.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28488 |
+202309302200,281,27,1.9,-9,-9.0,-9,1000.0,1011.1,-9,-9.0,18.5,14.9,80.0,16.9,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,3141,-9.0,-9.0,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28489 |
+202309302300,281,29,1.1,-9,-9.0,-9,999.9,1011.1,-9,-9.0,17.9,14.7,82.0,16.7,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,3120,-9.0,-9.0,-9,16.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28490 |
+202310010000,281,29,1.3,-9,-9.0,-9,1000.0,1011.3,2,0.6,16.4,15.0,92.0,17.0,-9.0,3.7,3.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2252,-9.0,-9.0,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28491 |
+202310010100,281,27,0.5,-9,-9.0,-9,1000.2,1011.5,-9,-9.0,15.9,15.2,96.0,17.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1815,-9.0,-9.0,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28492 |
+202310010200,281,0,0.2,-9,-9.0,-9,1000.0,1011.4,-9,-9.0,15.3,14.8,97.0,16.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,1598,-9.0,-9.0,-9,15.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28493 |
+202310010300,281,0,0.3,-9,-9.0,-9,999.7,1011.1,8,-0.2,15.1,14.7,98.0,16.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,1433,-9.0,-9.0,-9,14.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28494 |
+202310010400,281,25,1.3,-9,-9.0,-9,999.9,1011.2,-9,-9.0,16.1,14.9,93.0,16.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,1779,-9.0,-9.0,-9,15.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28495 |
+202310010500,281,27,2.7,-9,-9.0,-9,1000.1,1011.4,-9,-9.0,16.9,14.3,85.0,16.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2334,-9.0,-9.0,-9,15.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28496 |
+202310010600,281,27,2.5,-9,-9.0,-9,1000.9,1012.2,2,1.1,16.2,13.6,85.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2562,-9.0,-9.0,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28497 |
+202310010700,281,29,1.0,-9,-9.0,-9,1001.6,1012.9,-9,-9.0,15.8,12.1,79.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3997,0.0,-9.0,-9,14.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28498 |
+202310010800,281,27,2.1,-9,-9.0,-9,1002.1,1013.3,-9,-9.0,18.2,11.5,65.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,4875,0.8,-9.0,-9,19.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28499 |
+202310010900,281,29,2.2,-9,-9.0,-9,1002.6,1013.8,2,1.6,20.0,11.9,60.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4768,1.0,-9.0,-9,24.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28500 |
+202310011000,281,27,2.1,-9,-9.0,-9,1002.9,1014.0,-9,-9.0,21.1,11.4,54.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4964,1.0,-9.0,-9,30.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28501 |
+202310011100,281,34,2.2,-9,-9.0,-9,1002.9,1014.0,-9,-9.0,21.5,9.3,46.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,29.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28502 |
+202310011200,281,27,3.4,-9,-9.0,-9,1002.7,1013.7,8,-0.1,22.9,9.2,42.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,1,26,-,-9,-9,-9,5000,0.9,-9.0,-9,32.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28503 |
+202310011300,281,34,2.6,-9,-9.0,-9,1002.2,1013.2,-9,-9.0,22.6,8.6,41.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.9,-9.0,-9,30.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28504 |
+202310011400,281,34,2.5,-9,-9.0,-9,1002.1,1013.1,-9,-9.0,23.1,7.6,37.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.7,-9.0,-9,31.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28505 |
+202310011500,281,27,3.6,-9,-9.0,-9,1002.3,1013.3,5,-0.4,23.6,8.4,38.0,11.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,30.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28506 |
+202310011600,281,34,2.0,-9,-9.0,-9,1002.5,1013.5,-9,-9.0,23.2,7.2,36.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,28.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28507 |
+202310011700,281,32,2.2,-9,-9.0,-9,1002.7,1013.7,-9,-9.0,22.3,7.2,38.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,22.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28508 |
+202310011800,281,29,1.4,-9,-9.0,-9,1003.2,1014.4,2,1.1,20.1,8.7,48.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.4,-9.0,-9,17.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28509 |
+202310011900,281,32,1.8,-9,-9.0,-9,1003.6,1014.9,-9,-9.0,16.8,10.6,67.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.0,-9.0,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28510 |
+202310012000,281,11,1.0,-9,-9.0,-9,1004.2,1015.6,-9,-9.0,15.4,11.5,78.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4241,-9.0,-9.0,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28511 |
+202310012100,281,7,1.0,-9,-9.0,-9,1004.7,1016.1,2,1.7,14.1,11.7,86.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3412,-9.0,-9.0,-9,13.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28512 |
+202310012200,281,0,0.2,-9,-9.0,-9,1004.9,1016.4,-9,-9.0,13.0,11.7,92.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3231,-9.0,-9.0,-9,12.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28513 |
+202310012300,281,0,0.4,-9,-9.0,-9,1005.7,1017.2,-9,-9.0,12.4,11.1,92.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3354,-9.0,-9.0,-9,12.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28514 |
+202310020000,281,9,0.6,-9,-9.0,-9,1005.8,1017.4,2,1.3,11.5,11.0,97.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3207,-9.0,-9.0,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28515 |
+202310020100,281,0,0.4,-9,-9.0,-9,1005.8,1017.4,-9,-9.0,11.1,10.6,97.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2846,-9.0,-9.0,-9,11.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28516 |
+202310020200,281,9,1.0,-9,-9.0,-9,1006.3,1017.9,-9,-9.0,10.7,10.3,98.0,12.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,2970,-9.0,-9.0,-9,11.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28517 |
+202310020300,281,0,0.2,-9,-9.0,-9,1006.3,1018.0,2,0.6,10.2,10.0,99.0,12.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,2925,-9.0,-9.0,-9,10.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28518 |
+202310020400,281,0,0.4,-9,-9.0,-9,1006.4,1018.1,-9,-9.0,10.0,9.8,99.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,2725,-9.0,-9.0,-9,10.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28519 |
+202310020500,281,7,0.8,-9,-9.0,-9,1006.4,1018.0,-9,-9.0,9.7,9.5,99.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2884,-9.0,-9.0,-9,10.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28520 |
+202310020600,281,7,0.5,-9,-9.0,-9,1006.8,1018.5,2,0.5,9.5,9.3,99.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2842,-9.0,-9.0,-9,10.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28521 |
+202310020700,281,0,0.4,-9,-9.0,-9,1007.2,1018.9,-9,-9.0,9.7,9.5,99.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2733,0.0,-9.0,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28522 |
+202310020800,281,0,0.0,-9,-9.0,-9,1007.5,1019.1,-9,-9.0,10.9,10.2,96.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,2453,0.0,-9.0,-9,13.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28523 |
+202310020900,281,16,0.7,-9,-9.0,-9,1008.2,1019.7,2,1.2,13.8,10.7,82.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,3885,1.0,-9.0,-9,24.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28524 |
+202310021000,281,34,1.1,-9,-9.0,-9,1007.8,1019.0,-9,-9.0,18.7,10.7,60.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4852,1.0,-9.0,-9,31.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28525 |
+202310021100,281,18,1.2,-9,-9.0,-9,1007.7,1019.0,-9,-9.0,19.9,11.3,58.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,34.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28526 |
+202310021200,281,25,2.0,-9,-9.0,-9,1007.2,1018.3,7,-1.4,22.3,8.0,40.0,10.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,4837,1.0,-9.0,-9,36.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28527 |
+202310021300,281,29,2.7,-9,-9.0,-9,1006.6,1017.6,-9,-9.0,23.2,6.4,34.0,9.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,35.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28528 |
+202310021400,281,32,2.3,-9,-9.0,-9,1005.9,1016.9,-9,-9.0,23.4,6.1,33.0,9.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,34.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28529 |
+202310021500,281,32,1.7,-9,-9.0,-9,1005.6,1016.6,7,-1.7,23.5,7.1,35.0,10.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,32.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28530 |
+202310021600,281,32,1.7,-9,-9.0,-9,1005.4,1016.4,-9,-9.0,23.2,8.4,39.0,11.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,29.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28531 |
+202310021700,281,11,2.2,-9,-9.0,-9,1005.5,1016.6,-9,-9.0,22.0,9.5,45.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,23.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28532 |
+202310021800,281,9,1.3,-9,-9.0,-9,1006.1,1017.3,3,0.7,19.4,9.2,52.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.5,-9.0,-9,17.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28533 |
+202310021900,281,9,0.7,-9,-9.0,-9,1006.7,1018.0,-9,-9.0,17.5,10.3,63.0,12.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4992,0.0,-9.0,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28534 |
+202310022000,281,0,0.4,-9,-9.0,-9,1007.1,1018.6,-9,-9.0,15.0,11.3,79.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,3705,-9.0,-9.0,-9,14.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28535 |
+202310022100,281,0,0.2,-9,-9.0,-9,1007.5,1019.0,2,1.7,13.5,11.0,85.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4536,-9.0,-9.0,-9,13.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28536 |
+202310022200,281,0,0.3,-9,-9.0,-9,1008.1,1019.6,-9,-9.0,12.6,11.1,91.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3504,-9.0,-9.0,-9,12.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28537 |
+202310022300,281,9,0.7,-9,-9.0,-9,1008.5,1020.1,-9,-9.0,11.8,10.6,93.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,3,65,-,-9,-9,-9,3085,-9.0,-9.0,-9,12.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28538 |
+202310030000,281,9,0.5,-9,-9.0,-9,1008.7,1020.3,2,1.3,11.4,10.4,94.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,5,61,-,-9,-9,-9,3521,-9.0,-9.0,-9,12.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28539 |
+202310030100,281,0,0.2,-9,-9.0,-9,1008.6,1020.2,-9,-9.0,11.2,10.4,95.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,5,63,-,-9,-9,-9,4309,-9.0,-9.0,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28540 |
+202310030200,281,0,0.2,-9,-9.0,-9,1008.8,1020.4,-9,-9.0,10.9,10.4,97.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,60,-,-9,-9,-9,3399,-9.0,-9.0,-9,11.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28541 |
+202310030300,281,0,0.1,-9,-9.0,-9,1008.2,1019.9,8,-0.4,10.6,10.1,97.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,5,54,-,-9,-9,-9,3789,-9.0,-9.0,-9,11.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28542 |
+202310030400,281,9,0.5,-9,-9.0,-9,1007.9,1019.6,-9,-9.0,10.3,9.9,98.0,12.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,3,52,-,-9,-9,-9,3650,-9.0,-9.0,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28543 |
+202310030500,281,0,0.1,-9,-9.0,-9,1007.9,1019.6,-9,-9.0,10.1,9.7,98.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,5,56,-,-9,-9,-9,3770,-9.0,-9.0,-9,10.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28544 |
+202310030600,281,7,0.7,-9,-9.0,-9,1007.4,1019.1,7,-0.8,10.0,9.6,98.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,5,51,-,-9,-9,-9,3768,-9.0,-9.0,-9,10.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28545 |
+202310030700,281,0,0.1,-9,-9.0,-9,1007.7,1019.4,-9,-9.0,10.5,10.1,98.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,52,-,-9,-9,-9,3449,0.0,-9.0,-9,12.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28546 |
+202310030800,281,0,0.4,-9,-9.0,-9,1008.3,1019.9,-9,-9.0,11.8,10.6,93.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,51,-,-9,-9,-9,3882,0.0,-9.0,-9,16.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28547 |
+202310030900,281,14,0.6,-9,-9.0,-9,1008.3,1019.8,0,0.7,13.5,11.7,89.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,52,-,-9,-9,-9,3926,0.0,-9.0,-9,18.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28548 |
+202310031000,281,25,0.7,-9,-9.0,-9,1008.4,1019.8,-9,-9.0,16.0,12.1,78.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,55,-,-9,-9,-9,4884,0.1,-9.0,-9,23.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28549 |
+202310031100,281,2,0.6,-9,-9.0,-9,1008.1,1019.3,-9,-9.0,18.7,12.2,66.0,14.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,55,-,-9,-9,-9,5000,0.3,-9.0,-9,27.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28550 |
+202310031200,281,32,0.7,-9,-9.0,-9,1007.8,1019.0,7,-0.8,20.0,12.7,63.0,14.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,48,-,-9,-9,-9,5000,0.0,-9.0,-9,28.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28551 |
+202310031300,281,27,1.4,-9,-9.0,-9,1007.5,1018.7,-9,-9.0,20.6,13.0,62.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,13,-,-9,-9,-9,5000,0.0,-9.0,-9,25.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28552 |
+202310031400,281,27,0.9,-9,-9.0,-9,1006.3,1017.5,-9,-9.0,20.4,13.1,63.0,15.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,43,-,-9,-9,-9,5000,0.0,-9.0,-9,22.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28553 |
+202310031500,281,36,0.6,-9,-9.0,-9,1005.8,1017.0,7,-2.0,20.6,13.5,64.0,15.5,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,4992,0.0,-9.0,-9,24.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28554 |
+202310031600,281,29,1.1,-9,-9.0,-9,1005.7,1016.9,-9,-9.0,19.2,14.4,74.0,16.4,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,1,21,-,-9,-9,-9,4856,0.0,-9.0,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28555 |
+202310031700,281,27,1.4,-9,-9.0,-9,1005.8,1017.0,-9,-9.0,18.1,14.5,80.0,16.5,0.2,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,6,26,-,-9,-9,-9,2624,0.0,-9.0,-9,18.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28556 |
+202310031800,281,0,0.1,-9,-9.0,-9,1005.5,1016.8,7,-0.2,17.8,15.4,86.0,17.5,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,27,-,-9,-9,-9,2066,0.0,-9.0,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28557 |
+202310031900,281,7,0.6,-9,-9.0,-9,1005.8,1017.1,-9,-9.0,17.1,15.9,93.0,18.1,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,29,-,-9,-9,-9,1765,0.0,-9.0,-9,17.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28558 |
+202310032000,281,27,0.6,-9,-9.0,-9,1005.9,1017.2,-9,-9.0,16.5,15.5,94.0,17.6,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,4,13,-,-9,-9,-9,1734,-9.0,-9.0,-9,16.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28559 |
+202310032100,281,32,0.6,-9,-9.0,-9,1006.3,1017.7,2,0.9,15.5,15.1,98.0,17.2,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,13,-,-9,-9,-9,1253,-9.0,-9.0,-9,15.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28560 |
+202310032200,281,0,0.3,-9,-9.0,-9,1006.1,1017.6,-9,-9.0,14.9,14.5,98.0,16.5,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,1,-9,-,-9,-9,-9,1194,-9.0,-9.0,-9,14.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28561 |
+202310032300,281,0,0.2,-9,-9.0,-9,1005.9,1017.3,-9,-9.0,14.1,14.0,100.0,16.0,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,670,-9.0,-9.0,-9,13.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28562 |
+202310040000,281,0,0.1,-9,-9.0,-9,1005.8,1017.3,6,-0.4,13.3,13.2,100.0,15.2,-9.0,0.2,0.2,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,797,-9.0,-9.0,-9,13.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28563 |
+202310040100,281,0,0.1,-9,-9.0,-9,1005.6,1017.1,-9,-9.0,13.7,13.6,100.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,3,38,-,-9,-9,-9,547,-9.0,-9.0,-9,14.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28564 |
+202310040200,281,32,0.6,-9,-9.0,-9,1005.2,1016.7,-9,-9.0,13.6,13.5,100.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,61,-,-9,-9,-9,175,-9.0,-9.0,-9,15.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28565 |
+202310040300,281,27,1.1,-9,-9.0,-9,1004.7,1016.1,7,-1.2,14.1,14.0,100.0,16.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,-9,-,-9,-9,-9,71,-9.0,-9.0,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28566 |
+202310040400,281,34,0.8,-9,-9.0,-9,1004.3,1015.7,-9,-9.0,13.6,13.5,100.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,46,-,-9,-9,-9,159,-9.0,-9.0,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28567 |
+202310040500,281,32,0.7,-9,-9.0,-9,1004.7,1016.2,-9,-9.0,13.4,13.3,100.0,15.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,4,-9,-,-9,-9,-9,35,-9.0,-9.0,-9,15.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28568 |
+202310040600,281,32,1.2,-9,-9.0,-9,1004.7,1016.2,1,0.1,13.0,12.9,100.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,-9,-,-9,-9,-9,1045,-9.0,-9.0,-9,14.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28569 |
+202310040700,281,29,1.2,-9,-9.0,-9,1004.8,1016.3,-9,-9.0,13.4,13.3,100.0,15.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,-9,-,-9,-9,-9,1706,0.0,-9.0,-9,15.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28570 |
+202310040800,281,25,1.1,-9,-9.0,-9,1005.2,1016.6,-9,-9.0,14.5,14.3,99.0,16.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,1,-,-9,-9,-9,823,0.0,-9.0,-9,17.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28571 |
+202310040900,281,27,2.0,-9,-9.0,-9,1005.0,1016.4,0,0.2,15.8,13.9,89.0,15.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,2,-,-9,-9,-9,1346,0.4,-9.0,-9,23.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28572 |
+202310041000,281,25,1.6,-9,-9.0,-9,1004.6,1015.9,-9,-9.0,17.7,13.6,77.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,2,59,-,-9,-9,-9,1732,1.0,-9.0,-9,30.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28573 |
+202310041100,281,25,2.2,-9,-9.0,-9,1003.7,1014.9,-9,-9.0,20.4,13.5,65.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2449,1.0,-9.0,-9,33.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28574 |
+202310041200,281,27,3.4,-9,-9.0,-9,1002.7,1013.7,7,-2.7,22.6,12.5,53.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,35.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28575 |
+202310041300,281,32,2.7,-9,-9.0,-9,1001.8,1012.7,-9,-9.0,23.9,12.2,48.0,14.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,37.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28576 |
+202310041400,281,27,3.0,-9,-9.0,-9,1000.9,1011.8,-9,-9.0,23.7,11.3,46.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,34.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28577 |
+202310041500,281,32,2.2,-9,-9.0,-9,1000.7,1011.6,7,-2.1,23.7,11.3,46.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,31.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28578 |
+202310041600,281,29,2.5,-9,-9.0,-9,1000.3,1011.3,-9,-9.0,23.1,12.1,50.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,27.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28579 |
+202310041700,281,29,1.5,-9,-9.0,-9,1000.4,1011.4,-9,-9.0,22.8,13.5,56.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,5000,0.5,-9.0,-9,23.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28580 |
+202310041800,281,29,1.2,-9,-9.0,-9,1000.5,1011.6,4,0.0,20.9,13.8,64.0,15.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,5000,0.0,-9.0,-9,19.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28581 |
+202310041900,281,27,1.4,-9,-9.0,-9,1000.6,1011.7,-9,-9.0,20.4,13.5,65.0,15.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,15,-,-9,-9,-9,4783,0.0,-9.0,-9,19.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28582 |
+202310042000,281,29,1.1,-9,-9.0,-9,1000.7,1011.8,-9,-9.0,19.7,12.9,65.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,74,-,-9,-9,-9,4862,-9.0,-9.0,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28583 |
+202310042100,281,27,1.0,-9,-9.0,-9,1000.5,1011.6,4,0.0,19.5,13.2,67.0,15.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,59,-,-9,-9,-9,4011,-9.0,-9.0,-9,17.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28584 |
+202310042200,281,0,0.4,-9,-9.0,-9,1000.5,1011.7,-9,-9.0,17.5,14.7,84.0,16.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,18,-,-9,-9,-9,2875,-9.0,-9.0,-9,17.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28585 |
+202310042300,281,29,1.0,-9,-9.0,-9,1000.1,1011.3,-9,-9.0,18.4,13.4,73.0,15.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,4,59,-,-9,-9,-9,3491,-9.0,-9.0,-9,17.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28586 |
+202310050000,281,27,2.2,-9,-9.0,-9,1000.0,1011.1,8,-0.5,18.7,12.8,69.0,14.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,13,-,-9,-9,-9,3603,-9.0,-9.0,-9,17.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28587 |
+202310050100,281,29,2.6,-9,-9.0,-9,1000.2,1011.3,-9,-9.0,18.8,10.8,60.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,13,-,-9,-9,-9,4956,-9.0,-9.0,-9,16.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28588 |
+202310050200,281,29,3.0,-9,-9.0,-9,1000.9,1012.1,-9,-9.0,17.8,9.1,57.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,5000,-9.0,-9.0,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28589 |
+202310050300,281,29,2.4,29,10.0,233,1001.0,1012.3,2,1.2,16.8,5.7,48.0,9.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,5000,-9.0,-9.0,-9,13.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28590 |
+202310050400,281,29,2.9,-9,-9.0,-9,1001.2,1012.5,-9,-9.0,16.4,2.3,39.0,7.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,5000,-9.0,-9.0,-9,13.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28591 |
+202310050500,281,32,2.5,-9,-9.0,-9,1001.8,1013.2,-9,-9.0,15.1,2.5,43.0,7.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,5000,-9.0,-9.0,-9,12.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28592 |
+202310050600,281,32,2.9,-9,-9.0,-9,1003.2,1014.6,2,2.3,14.4,0.2,38.0,6.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,1522,-9.0,-9.0,-9,11.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28593 |
+202310050700,281,29,2.2,-9,-9.0,-9,1003.7,1015.1,-9,-9.0,13.7,0.9,42.0,6.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,674,0.0,-9.0,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28594 |
+202310050800,281,29,2.9,-9,-9.0,-9,1004.2,1015.6,-9,-9.0,13.7,0.9,42.0,6.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,5000,0.0,-9.0,-9,13.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28595 |
+202310050900,281,29,1.0,-9,-9.0,-9,1005.0,1016.4,2,1.8,13.8,1.4,43.0,6.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,5000,0.0,-9.0,-9,16.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28596 |
+202310051000,281,34,3.1,-9,-9.0,-9,1004.7,1016.0,-9,-9.0,16.5,2.1,38.0,7.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,5000,0.9,-9.0,-9,28.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28597 |
+202310051100,281,27,4.4,-9,-9.0,-9,1004.4,1015.7,-9,-9.0,17.3,2.0,36.0,7.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,31.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28598 |
+202310051200,281,29,4.0,-9,-9.0,-9,1003.7,1014.9,7,-1.5,18.8,0.8,30.0,6.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,34.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28599 |
+202310051300,281,29,2.3,-9,-9.0,-9,1003.1,1014.2,-9,-9.0,19.2,2.0,32.0,7.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4628,1.0,-9.0,-9,36.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28600 |
+202310051400,281,29,2.9,-9,-9.0,-9,1002.7,1013.9,-9,-9.0,20.3,0.6,27.0,6.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,34.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28601 |
+202310051500,281,25,2.9,-9,-9.0,-9,1002.3,1013.5,7,-1.4,19.9,2.6,32.0,7.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,31.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28602 |
+202310051600,281,27,3.8,-9,-9.0,-9,1002.3,1013.4,-9,-9.0,19.6,2.4,32.0,7.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,27.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28603 |
+202310051700,281,29,2.8,-9,-9.0,-9,1002.9,1014.1,-9,-9.0,18.8,2.9,35.0,7.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,20.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28604 |
+202310051800,281,29,1.3,-9,-9.0,-9,1003.1,1014.4,3,0.9,16.7,4.6,45.0,8.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4866,0.5,-9.0,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28605 |
+202310051900,281,29,1.2,-9,-9.0,-9,1003.8,1015.2,-9,-9.0,14.2,5.7,57.0,9.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4826,0.0,-9.0,-9,13.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28606 |
+202310052000,281,29,1.3,-9,-9.0,-9,1004.5,1016.0,-9,-9.0,12.4,6.2,66.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4777,-9.0,-9.0,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28607 |
+202310052100,281,27,2.1,-9,-9.0,-9,1005.5,1017.0,2,2.6,13.4,5.0,57.0,8.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4241,-9.0,-9.0,-9,11.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28608 |
+202310052200,281,29,1.4,-9,-9.0,-9,1005.9,1017.4,-9,-9.0,13.3,5.4,59.0,9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,4302,-9.0,-9.0,-9,12.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28609 |
+202310052300,281,27,1.0,-9,-9.0,-9,1006.2,1017.7,-9,-9.0,12.8,5.2,60.0,8.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,4647,-9.0,-9.0,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28610 |
+202310060000,281,32,1.7,-9,-9.0,-9,1006.1,1017.7,1,0.7,10.8,6.3,74.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,4603,-9.0,-9.0,-9,11.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28611 |
+202310060100,281,32,0.5,-9,-9.0,-9,1006.0,1017.6,-9,-9.0,11.3,6.2,71.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,4247,-9.0,-9.0,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28612 |
+202310060200,281,29,0.7,-9,-9.0,-9,1006.5,1018.2,-9,-9.0,9.9,7.3,84.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,3886,-9.0,-9.0,-9,10.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28613 |
+202310060300,281,0,0.4,-9,-9.0,-9,1006.6,1018.3,3,0.6,10.0,7.2,83.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,2,64,-,-9,-9,-9,3976,-9.0,-9.0,-9,10.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28614 |
+202310060400,281,27,0.7,-9,-9.0,-9,1006.7,1018.4,-9,-9.0,10.0,6.5,79.0,9.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,2,65,-,-9,-9,-9,4572,-9.0,-9.0,-9,10.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28615 |
+202310060500,281,11,0.5,-9,-9.0,-9,1007.0,1018.7,-9,-9.0,9.2,7.8,91.0,10.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,3645,-9.0,-9.0,-9,10.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28616 |
+202310060600,281,0,0.2,-9,-9.0,-9,1007.2,1018.9,2,0.6,8.9,7.9,94.0,10.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,3239,-9.0,-9.0,-9,10.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28617 |
+202310060700,281,11,0.5,-9,-9.0,-9,1007.8,1019.5,-9,-9.0,8.9,7.8,93.0,10.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,3441,0.0,-9.0,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28618 |
+202310060800,281,9,0.7,-9,-9.0,-9,1008.5,1020.2,-9,-9.0,10.7,8.0,84.0,10.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,62,-,-9,-9,-9,3268,0.6,-9.0,-9,14.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28619 |
+202310060900,281,25,1.2,-9,-9.0,-9,1008.3,1019.7,0,0.8,14.6,6.4,58.0,9.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,66,-,-9,-9,-9,4412,0.8,-9.0,-9,22.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28620 |
+202310061000,281,27,1.3,-9,-9.0,-9,1008.6,1020.0,-9,-9.0,16.8,6.0,49.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,4923,1.0,-9.0,-9,29.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28621 |
+202310061100,281,32,1.6,-9,-9.0,-9,1008.0,1019.3,-9,-9.0,18.2,5.4,43.0,9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4976,1.0,-9.0,-9,33.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28622 |
+202310061200,281,16,1.1,-9,-9.0,-9,1007.5,1018.7,8,-1.0,19.3,6.3,43.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1856,1.0,-9.0,-9,36.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28623 |
+202310061300,281,25,1.4,-9,-9.0,-9,1006.6,1017.8,-9,-9.0,20.9,5.2,36.0,8.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,-9,1.0,-9.0,-9,37.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28624 |
+202310061400,281,23,1.0,-9,-9.0,-9,1005.7,1016.8,-9,-9.0,21.4,4.0,32.0,8.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,-9,1.0,-9.0,-9,36.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28625 |
+202310061500,281,25,1.3,-9,-9.0,-9,1005.6,1016.7,7,-2.0,21.5,4.0,32.0,8.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,-9,1.0,-9.0,-9,33.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28626 |
+202310061600,281,23,0.9,-9,-9.0,-9,1005.5,1016.6,-9,-9.0,21.4,5.6,36.0,9.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,30.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28627 |
+202310061700,281,23,0.8,-9,-9.0,-9,1005.8,1017.0,-9,-9.0,20.8,5.9,38.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,4991,0.5,-9.0,-9,22.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28628 |
+202310061800,281,29,1.2,-9,-9.0,-9,1006.3,1017.5,3,0.8,18.3,8.8,54.0,11.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,2,62,-,-9,-9,-9,4476,0.1,-9.0,-9,17.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28629 |
+202310061900,281,0,0.0,-9,-9.0,-9,1007.2,1018.5,-9,-9.0,16.8,9.4,62.0,11.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,62,-,-9,-9,-9,4826,0.0,-9.0,-9,16.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28630 |
+202310062000,281,9,0.7,-9,-9.0,-9,1007.6,1019.0,-9,-9.0,15.3,11.6,79.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,3,71,-,-9,-9,-9,2918,-9.0,-9.0,-9,15.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28631 |
+202310062100,281,0,0.4,-9,-9.0,-9,1008.3,1019.8,2,2.3,14.8,11.5,81.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,3766,-9.0,-9.0,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28632 |
+202310062200,281,32,1.2,-9,-9.0,-9,1008.6,1020.1,-9,-9.0,14.8,10.2,74.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,4873,-9.0,-9.0,-9,15.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28633 |
+202310062300,281,16,0.5,-9,-9.0,-9,1008.7,1020.2,-9,-9.0,14.8,11.1,79.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,1305,-9.0,-9.0,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28634 |
+202310070000,281,0,0.2,-9,-9.0,-9,1009.1,1020.6,2,0.8,14.4,10.6,78.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,1493,-9.0,-9.0,-9,14.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28635 |
+202310070100,281,9,0.5,-9,-9.0,-9,1009.3,1020.8,-9,-9.0,13.7,11.9,89.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,2918,-9.0,-9.0,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28636 |
+202310070200,281,0,0.4,-9,-9.0,-9,1009.2,1020.7,-9,-9.0,13.4,11.6,89.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,3664,-9.0,-9.0,-9,14.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28637 |
+202310070300,281,14,0.6,-9,-9.0,-9,1009.1,1020.6,4,0.0,13.0,11.8,93.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,62,-,-9,-9,-9,3404,-9.0,-9.0,-9,13.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28638 |
+202310070400,281,0,0.2,-9,-9.0,-9,1009.2,1020.8,-9,-9.0,12.5,11.7,95.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,1,60,-,-9,-9,-9,3236,-9.0,-9.0,-9,12.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28639 |
+202310070500,281,0,0.0,-9,-9.0,-9,1009.6,1021.2,-9,-9.0,11.9,11.4,97.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,60,-,-9,-9,-9,2835,-9.0,-9.0,-9,11.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28640 |
+202310070600,281,0,0.0,-9,-9.0,-9,1009.9,1021.5,2,0.9,11.3,10.9,98.0,13.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2070,-9.0,-9.0,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28641 |
+202310070700,281,9,0.6,-9,-9.0,-9,1010.5,1022.2,-9,-9.0,11.2,10.7,97.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,1673,0.0,-9.0,-9,12.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28642 |
+202310070800,281,0,0.1,-9,-9.0,-9,1011.7,1023.3,-9,-9.0,12.4,11.4,94.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,2216,0.2,-9.0,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28643 |
+202310070900,281,18,0.7,-9,-9.0,-9,1011.8,1023.3,1,1.8,14.0,11.3,84.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2732,0.3,-9.0,-9,19.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28644 |
+202310071000,281,0,0.3,-9,-9.0,-9,1012.0,1023.5,-9,-9.0,15.7,10.6,72.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,3719,0.0,-9.0,-9,21.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28645 |
+202310071100,281,23,0.9,-9,-9.0,-9,1011.8,1023.1,-9,-9.0,18.5,11.0,62.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,4472,0.8,-9.0,-9,30.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28646 |
+202310071200,281,7,3.0,-9,-9.0,-9,1011.4,1022.6,8,-0.7,21.2,8.7,45.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,36.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28647 |
+202310071300,281,11,2.0,-9,-9.0,-9,1010.7,1021.9,-9,-9.0,21.6,9.7,47.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,4,70,-,-9,-9,-9,4948,0.7,-9.0,-9,32.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28648 |
+202310071400,281,9,2.4,-9,-9.0,-9,1010.3,1021.5,-9,-9.0,21.0,10.7,52.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,72,-,-9,-9,-9,5000,0.3,-9.0,-9,26.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28649 |
+202310071500,281,9,2.7,-9,-9.0,-9,1010.4,1021.6,5,-1.0,20.8,10.5,52.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,61,-,-9,-9,-9,5000,0.0,-9.0,-9,25.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28650 |
+202310071600,281,7,3.6,-9,-9.0,-9,1010.6,1021.8,-9,-9.0,19.4,12.1,63.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,5,51,-,-9,-9,-9,5000,0.0,-9.0,-9,22.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28651 |
+202310071700,281,7,3.1,-9,-9.0,-9,1011.0,1022.3,-9,-9.0,18.2,12.4,69.0,14.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,5000,0.0,-9.0,-9,19.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28652 |
+202310071800,281,9,1.3,-9,-9.0,-9,1011.4,1022.7,2,1.1,17.4,12.5,73.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,16,-,-9,-9,-9,4984,0.0,-9.0,-9,18.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28653 |
+202310071900,281,14,0.8,-9,-9.0,-9,1011.8,1023.2,-9,-9.0,17.1,12.8,76.0,14.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,18,-,-9,-9,-9,4295,0.0,-9.0,-9,17.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28654 |
+202310072000,281,0,0.2,-9,-9.0,-9,1012.7,1024.1,-9,-9.0,16.9,12.6,76.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,20,-,-9,-9,-9,4990,-9.0,-9.0,-9,17.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28655 |
+202310072100,281,0,0.2,-9,-9.0,-9,1012.9,1024.3,2,1.6,16.6,12.9,79.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,21,-,-9,-9,-9,4266,-9.0,-9.0,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28656 |
+202310072200,281,32,0.8,-9,-9.0,-9,1013.0,1024.5,-9,-9.0,15.9,13.0,83.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,32,-,-9,-9,-9,4678,-9.0,-9.0,-9,16.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28657 |
+202310072300,281,0,0.3,-9,-9.0,-9,1012.8,1024.3,-9,-9.0,15.6,13.4,87.0,15.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,25,-,-9,-9,-9,4029,-9.0,-9.0,-9,16.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28658 |
+202310080000,281,0,0.1,-9,-9.0,-9,1012.5,1024.0,8,-0.3,15.4,12.8,85.0,14.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,24,-,-9,-9,-9,4700,-9.0,-9.0,-9,16.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28659 |
+202310080100,281,0,0.1,-9,-9.0,-9,1012.5,1024.0,-9,-9.0,15.1,13.6,91.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,25,-,-9,-9,-9,4646,-9.0,-9.0,-9,16.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28660 |
+202310080200,281,9,0.7,-9,-9.0,-9,1012.5,1024.0,-9,-9.0,15.1,13.6,91.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,7,14,-,-9,-9,-9,4326,-9.0,-9.0,-9,16.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28661 |
+202310080300,281,0,0.2,-9,-9.0,-9,1012.4,1023.9,7,-0.1,15.4,13.4,88.0,15.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,16,-,-9,-9,-9,4475,-9.0,-9.0,-9,16.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28662 |
+202310080400,281,0,0.1,-9,-9.0,-9,1012.1,1023.6,-9,-9.0,15.5,13.1,86.0,15.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,17,-,-9,-9,-9,4580,-9.0,-9.0,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28663 |
+202310080500,281,9,0.6,-9,-9.0,-9,1012.3,1023.7,-9,-9.0,16.2,12.1,77.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,14,-,-9,-9,-9,5000,-9.0,-9.0,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28664 |
+202310080600,281,0,0.2,-9,-9.0,-9,1012.2,1023.7,5,-0.2,15.6,12.3,81.0,14.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,15,-,-9,-9,-9,4918,-9.0,-9.0,-9,15.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28665 |
+202310080700,281,0,0.3,-9,-9.0,-9,1012.5,1024.0,-9,-9.0,15.7,12.6,82.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,14,-,-9,-9,-9,4919,0.0,-9.0,-9,16.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28666 |
+202310080800,281,7,0.6,-9,-9.0,-9,1012.7,1024.1,-9,-9.0,16.4,12.7,79.0,14.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,14,-,-9,-9,-9,4312,0.0,-9.0,-9,17.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28667 |
+202310080900,281,9,2.0,-9,-9.0,-9,1012.8,1024.1,1,0.4,18.1,11.1,64.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,31,-,-9,-9,-9,5000,0.0,-9.0,-9,19.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28668 |
+202310081000,281,9,3.3,-9,-9.0,-9,1012.7,1024.0,-9,-9.0,18.7,9.7,56.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,43,-,-9,-9,-9,5000,0.0,-9.0,-9,21.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28669 |
+202310081100,281,7,3.0,-9,-9.0,-9,1012.4,1023.6,-9,-9.0,19.1,9.2,53.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,50,-,-9,-9,-9,4223,0.0,-9.0,-9,23.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28670 |
+202310081200,281,7,2.6,-9,-9.0,-9,1011.9,1023.1,7,-1.0,19.3,9.4,53.0,11.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,38,-,-9,-9,-9,5000,0.0,-9.0,-9,22.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28671 |
+202310081300,281,7,2.2,-9,-9.0,-9,1011.0,1022.2,-9,-9.0,19.5,10.2,55.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,38,-,-9,-9,-9,5000,0.0,-9.0,-9,23.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28672 |
+202310081400,281,7,2.2,-9,-9.0,-9,1010.9,1022.1,-9,-9.0,19.3,11.0,59.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,12,-,-9,-9,-9,5000,0.0,-9.0,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28673 |
+202310081500,281,11,2.7,-9,-9.0,-9,1011.0,1022.4,5,-0.7,17.3,13.8,80.0,15.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,8,-,-9,-9,-9,4653,0.0,-9.0,-9,20.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28674 |
+202310081600,281,9,3.3,-9,-9.0,-9,1011.2,1022.5,-9,-9.0,17.9,12.5,71.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,9,-,-9,-9,-9,4997,0.0,-9.0,-9,20.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28675 |
+202310081700,281,9,0.9,-9,-9.0,-9,1011.0,1022.4,-9,-9.0,17.3,12.6,74.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,5,15,-,-9,-9,-9,4997,0.0,-9.0,-9,18.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28676 |
+202310081800,281,0,0.4,-9,-9.0,-9,1010.9,1022.3,8,-0.1,17.0,12.9,77.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,4,18,-,-9,-9,-9,2574,0.0,-9.0,-9,17.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28677 |
+202310081900,281,5,1.1,-9,-9.0,-9,1011.0,1022.3,-9,-9.0,17.5,11.5,68.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,12,-,-9,-9,-9,4976,-9.0,-9.0,-9,16.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28678 |
+202310082000,281,2,0.7,-9,-9.0,-9,1011.3,1022.7,-9,-9.0,17.0,11.0,68.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,37,-,-9,-9,-9,5000,-9.0,-9.0,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28679 |
+202310082100,281,0,0.4,-9,-9.0,-9,1011.5,1023.0,2,0.7,15.2,11.5,79.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,6,13,-,-9,-9,-9,5000,-9.0,-9.0,-9,15.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28680 |
+202310082200,281,29,0.6,-9,-9.0,-9,1011.1,1022.6,-9,-9.0,15.1,11.8,81.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,13,-,-9,-9,-9,5000,-9.0,-9.0,-9,14.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28681 |
+202310082300,281,29,1.5,-9,-9.0,-9,1010.8,1022.3,-9,-9.0,14.1,11.9,87.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,2,11,-,-9,-9,-9,981,-9.0,-9.0,-9,14.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28682 |
+202310090000,281,27,0.9,-9,-9.0,-9,1010.6,1022.1,7,-0.9,15.2,11.3,78.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,10,-,-9,-9,-9,1992,-9.0,-9.0,-9,15.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28683 |
+202310090100,281,27,0.6,-9,-9.0,-9,1010.4,1021.9,-9,-9.0,15.1,11.4,79.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,9,-,-9,-9,-9,2420,-9.0,-9.0,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28684 |
+202310090200,281,0,0.1,-9,-9.0,-9,1010.2,1021.7,-9,-9.0,15.2,11.7,80.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,5,-,-9,-9,-9,4961,-9.0,-9.0,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28685 |
+202310090300,281,0,0.3,-9,-9.0,-9,1009.7,1021.2,7,-0.9,15.3,11.4,78.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,3,-,-9,-9,-9,4483,-9.0,-9.0,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28686 |
+202310090400,281,29,0.8,-9,-9.0,-9,1009.4,1020.9,-9,-9.0,15.2,11.3,78.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,4,-,-9,-9,-9,2060,-9.0,-9.0,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28687 |
+202310090500,281,27,0.7,-9,-9.0,-9,1009.0,1020.5,-9,-9.0,15.1,11.6,80.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,7,-,-9,-9,-9,5000,-9.0,-9.0,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28688 |
+202310090600,281,0,0.3,-9,-9.0,-9,1009.2,1020.7,5,-0.5,14.8,11.3,80.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,8,-,-9,-9,-9,5000,-9.0,-9.0,-9,15.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28689 |
+202310090700,281,29,0.5,-9,-9.0,-9,1009.5,1021.0,-9,-9.0,14.9,11.6,81.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,9,-,-9,-9,-9,4987,0.0,-9.0,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28690 |
+202310090800,281,27,1.0,-9,-9.0,-9,1009.3,1020.7,-9,-9.0,15.9,11.6,76.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,11,-,-9,-9,-9,2335,0.0,-9.0,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28691 |
+202310090900,281,23,0.9,-9,-9.0,-9,1009.8,1021.1,2,0.4,17.9,11.9,68.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,6,12,-,-9,-9,-9,3584,0.4,-9.0,-9,26.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28692 |
+202310091000,281,0,0.4,-9,-9.0,-9,1009.1,1020.3,-9,-9.0,18.6,12.5,68.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,2,45,-,-9,-9,-9,4915,0.4,-9.0,-9,28.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28693 |
+202310091100,281,32,0.6,-9,-9.0,-9,1008.8,1020.1,-9,-9.0,19.8,13.2,66.0,15.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,5000,0.4,-9.0,-9,25.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28694 |
+202310091200,281,27,1.2,-9,-9.0,-9,1008.4,1019.7,7,-1.4,20.0,13.4,66.0,15.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,9,-,-9,-9,-9,4979,0.0,-9.0,-9,25.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28695 |
+202310091300,281,27,1.0,-9,-9.0,-9,1007.8,1019.0,-9,-9.0,20.1,13.7,67.0,15.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,9,-,-9,-9,-9,4971,0.0,-9.0,-9,24.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28696 |
+202310091400,281,20,0.6,-9,-9.0,-9,1007.1,1018.3,-9,-9.0,20.7,13.6,64.0,15.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,10,-,-9,-9,-9,4931,0.0,-9.0,-9,28.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28697 |
+202310091500,281,11,1.5,-9,-9.0,-9,1006.6,1017.7,7,-2.0,21.5,14.1,63.0,16.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,9,-,-9,-9,-9,4764,0.2,-9.0,-9,32.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28698 |
+202310091600,281,11,1.4,-9,-9.0,-9,1006.8,1018.0,-9,-9.0,20.4,13.8,66.0,15.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,9,-,-9,-9,-9,5000,0.1,-9.0,-9,23.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28699 |
+202310091700,281,9,1.4,-9,-9.0,-9,1006.9,1018.1,-9,-9.0,19.9,14.6,72.0,16.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,9,-,-9,-9,-9,4992,0.0,-9.0,-9,21.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28700 |
+202310091800,281,11,1.0,-9,-9.0,-9,1007.0,1018.2,2,0.5,18.9,14.7,77.0,16.7,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,17,-,-9,-9,-9,3800,0.0,-9.0,-9,18.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28701 |
+202310091900,281,9,0.7,-9,-9.0,-9,1007.4,1018.7,-9,-9.0,17.8,14.4,81.0,16.4,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,22,-,-9,-9,-9,4143,-9.0,-9.0,-9,16.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28702 |
+202310092000,281,7,0.6,-9,-9.0,-9,1007.5,1018.9,-9,-9.0,16.2,14.3,89.0,16.3,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,2521,-9.0,-9.0,-9,15.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28703 |
+202310092100,281,7,0.5,-9,-9.0,-9,1007.7,1019.1,2,0.9,15.3,14.6,96.0,16.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,2691,-9.0,-9.0,-9,14.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28704 |
+202310092200,281,9,0.7,-9,-9.0,-9,1007.8,1019.3,-9,-9.0,14.2,13.7,97.0,15.7,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,12,-,-9,-9,-9,2445,-9.0,-9.0,-9,14.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28705 |
+202310092300,281,7,0.6,-9,-9.0,-9,1007.6,1019.1,-9,-9.0,14.1,13.7,98.0,15.7,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,1,31,-,-9,-9,-9,2529,-9.0,-9.0,-9,13.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28706 |
+202310100000,281,0,0.1,-9,-9.0,-9,1007.2,1018.7,8,-0.4,14.0,13.6,98.0,15.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,27,-,-9,-9,-9,2778,-9.0,-9.0,-9,13.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28707 |
+202310100100,281,0,0.4,-9,-9.0,-9,1007.3,1018.8,-9,-9.0,13.2,13.0,99.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,11,-,-9,-9,-9,2560,-9.0,-9.0,-9,13.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28708 |
+202310100200,281,0,0.2,-9,-9.0,-9,1007.5,1019.0,-9,-9.0,12.6,12.4,99.0,14.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2210,-9.0,-9.0,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28709 |
+202310100300,281,27,1.7,-9,-9.0,-9,1007.2,1018.6,8,-0.1,14.5,13.0,91.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1662,-9.0,-9.0,-9,12.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28710 |
+202310100400,281,29,1.1,-9,-9.0,-9,1006.8,1018.3,-9,-9.0,13.9,12.6,92.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1369,-9.0,-9.0,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28711 |
+202310100500,281,29,1.5,-9,-9.0,-9,1007.6,1019.1,-9,-9.0,14.1,12.1,88.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,1482,-9.0,-9.0,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28712 |
+202310100600,281,29,1.9,-9,-9.0,-9,1008.0,1019.5,3,0.9,12.8,12.1,96.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,947,-9.0,-9.0,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28713 |
+202310100700,281,27,2.8,-9,-9.0,-9,1008.1,1019.5,-9,-9.0,14.5,12.5,88.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1507,0.2,-9.0,-9,13.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28714 |
+202310100800,281,25,1.7,-9,-9.0,-9,1008.7,1020.1,-9,-9.0,15.8,11.9,78.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2018,1.0,-9.0,-9,19.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28715 |
+202310100900,281,29,1.5,-9,-9.0,-9,1009.4,1020.7,2,1.2,17.6,11.8,69.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,2466,1.0,-9.0,-9,24.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28716 |
+202310101000,281,32,1.9,-9,-9.0,-9,1009.5,1020.7,-9,-9.0,19.3,10.5,57.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3933,1.0,-9.0,-9,29.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28717 |
+202310101100,281,29,1.6,-9,-9.0,-9,1009.2,1020.4,-9,-9.0,20.4,10.7,54.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4870,1.0,-9.0,-9,33.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28718 |
+202310101200,281,29,1.3,-9,-9.0,-9,1008.7,1019.9,7,-0.8,21.3,9.8,48.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,14,-,-9,-9,-9,5000,0.9,-9.0,-9,38.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28719 |
+202310101300,281,2,1.8,-9,-9.0,-9,1008.5,1019.7,-9,-9.0,21.0,9.5,48.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,15,-,-9,-9,-9,5000,0.3,-9.0,-9,28.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28720 |
+202310101400,281,9,1.4,-9,-9.0,-9,1008.1,1019.2,-9,-9.0,21.9,9.7,46.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,2,19,-,-9,-9,-9,5000,0.4,-9.0,-9,34.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28721 |
+202310101500,281,9,3.3,-9,-9.0,-9,1008.4,1019.6,5,-0.3,21.3,10.7,51.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,22,-,-9,-9,-9,5000,0.4,-9.0,-9,30.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28722 |
+202310101600,281,9,2.4,-9,-9.0,-9,1008.6,1019.8,-9,-9.0,20.6,9.8,50.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,16,-,-9,-9,-9,4903,0.3,-9.0,-9,23.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28723 |
+202310101700,281,11,1.7,-9,-9.0,-9,1009.1,1020.4,-9,-9.0,20.0,10.6,55.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,4690,0.4,-9.0,-9,21.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28724 |
+202310101800,281,11,1.3,-9,-9.0,-9,1009.4,1020.7,2,1.1,18.1,11.6,66.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,1,-9,-,-9,-9,-9,4081,0.0,-9.0,-9,16.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28725 |
+202310101900,281,0,0.2,-9,-9.0,-9,1010.0,1021.4,-9,-9.0,16.7,11.8,73.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,3819,-9.0,-9.0,-9,15.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28726 |
+202310102000,281,9,0.5,-9,-9.0,-9,1010.5,1022.0,-9,-9.0,14.3,12.1,87.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2907,-9.0,-9.0,-9,14.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28727 |
+202310102100,281,7,0.5,-9,-9.0,-9,1011.0,1022.5,2,1.8,14.2,11.8,86.0,13.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2736,-9.0,-9.0,-9,13.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28728 |
+202310102200,281,0,0.3,-9,-9.0,-9,1011.4,1022.9,-9,-9.0,13.6,11.9,90.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2202,-9.0,-9.0,-9,12.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28729 |
+202310102300,281,0,0.2,-9,-9.0,-9,1011.7,1023.3,-9,-9.0,13.2,11.9,92.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,0,-9,-,-9,-9,-9,2331,-9.0,-9.0,-9,12.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28730 |
+202310110000,281,0,0.3,-9,-9.0,-9,1011.9,1023.5,2,1.0,12.3,11.6,96.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2129,-9.0,-9.0,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28731 |
+202310110100,281,0,0.1,-9,-9.0,-9,1012.2,1023.8,-9,-9.0,11.8,11.3,97.0,13.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2030,-9.0,-9.0,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28732 |
+202310110200,281,0,0.4,-9,-9.0,-9,1012.5,1024.2,-9,-9.0,11.1,10.7,98.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1971,-9.0,-9.0,-9,10.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28733 |
+202310110300,281,9,0.5,-9,-9.0,-9,1012.4,1024.1,0,0.6,10.4,10.2,99.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1718,-9.0,-9.0,-9,9.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28734 |
+202310110400,281,0,0.1,-9,-9.0,-9,1012.7,1024.5,-9,-9.0,9.8,9.6,99.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1323,-9.0,-9.0,-9,9.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28735 |
+202310110500,281,7,0.5,-9,-9.0,-9,1012.8,1024.5,-9,-9.0,9.4,9.2,99.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1522,-9.0,-9.0,-9,9.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28736 |
+202310110600,281,0,0.4,-9,-9.0,-9,1013.4,1025.2,2,1.1,9.1,8.9,99.0,11.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1596,-9.0,-9.0,-9,8.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28737 |
+202310110700,281,9,0.6,-9,-9.0,-9,1013.9,1025.7,-9,-9.0,8.8,8.6,99.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1529,0.1,-9.0,-9,9.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28738 |
+202310110800,281,11,0.7,-9,-9.0,-9,1014.4,1026.1,-9,-9.0,10.6,9.8,95.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1797,1.0,-9.0,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28739 |
+202310110900,281,16,0.5,-9,-9.0,-9,1014.8,1026.3,2,1.1,13.9,10.1,78.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2495,1.0,-9.0,-9,22.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28740 |
+202310111000,281,27,0.7,-9,-9.0,-9,1014.7,1026.0,-9,-9.0,18.0,11.7,67.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,2802,1.0,-9.0,-9,30.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28741 |
+202310111100,281,14,0.9,-9,-9.0,-9,1014.1,1025.4,-9,-9.0,20.1,9.0,49.0,11.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,4264,1.0,-9.0,-9,34.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28742 |
+202310111200,281,9,3.1,-9,-9.0,-9,1013.6,1024.8,7,-1.5,21.2,8.1,43.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,17,-,-9,-9,-9,5000,0.5,-9.0,-9,33.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28743 |
+202310111300,281,7,3.5,-9,-9.0,-9,1012.8,1024.0,-9,-9.0,21.8,8.3,42.0,10.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,4,21,-,-9,-9,-9,5000,0.7,-9.0,-9,33.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28744 |
+202310111400,281,9,3.2,-9,-9.0,-9,1012.5,1023.7,-9,-9.0,21.2,7.4,41.0,10.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,17,-,-9,-9,-9,4553,0.5,-9.0,-9,29.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28745 |
+202310111500,281,9,2.9,-9,-9.0,-9,1012.5,1023.8,5,-1.0,20.6,7.5,43.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,5,19,-,-9,-9,-9,5000,0.5,-9.0,-9,25.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28746 |
+202310111600,281,9,2.8,-9,-9.0,-9,1012.5,1023.7,-9,-9.0,21.0,7.5,42.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,3,57,-,-9,-9,-9,5000,0.6,-9.0,-9,26.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28747 |
+202310111700,281,9,2.0,-9,-9.0,-9,1012.6,1023.8,-9,-9.0,19.3,6.7,44.0,9.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,5000,0.5,-9.0,-9,18.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28748 |
+202310111800,281,9,1.3,-9,-9.0,-9,1012.8,1024.2,3,0.4,17.1,5.9,48.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.1,-9.0,-9,14.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28749 |
+202310111900,281,7,0.8,-9,-9.0,-9,1013.2,1024.7,-9,-9.0,15.1,7.6,61.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3939,-9.0,-9.0,-9,12.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28750 |
+202310112000,281,0,0.3,-9,-9.0,-9,1013.5,1025.1,-9,-9.0,12.6,8.4,76.0,11.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3656,-9.0,-9.0,-9,11.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28751 |
+202310112100,281,0,0.4,-9,-9.0,-9,1013.9,1025.6,2,1.4,11.5,8.3,81.0,10.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3889,-9.0,-9.0,-9,10.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28752 |
+202310112200,281,0,0.2,-9,-9.0,-9,1014.4,1026.1,-9,-9.0,10.5,8.5,88.0,11.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3162,-9.0,-9.0,-9,9.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28753 |
+202310112300,281,0,0.2,-9,-9.0,-9,1014.4,1026.1,-9,-9.0,9.6,8.0,90.0,10.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3721,-9.0,-9.0,-9,9.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28754 |
+202310120000,281,9,0.5,-9,-9.0,-9,1014.6,1026.4,2,0.8,9.0,7.9,93.0,10.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3784,-9.0,-9.0,-9,8.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28755 |
+202310120100,281,7,0.6,-9,-9.0,-9,1014.5,1026.3,-9,-9.0,8.4,7.6,95.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3399,-9.0,-9.0,-9,8.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28756 |
+202310120200,281,0,0.3,-9,-9.0,-9,1014.3,1026.1,-9,-9.0,7.9,7.4,97.0,10.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,2769,-9.0,-9.0,-9,7.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28757 |
+202310120300,281,0,0.2,-9,-9.0,-9,1014.0,1025.8,7,-0.6,7.6,7.1,97.0,10.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,3003,-9.0,-9.0,-9,7.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28758 |
+202310120400,281,7,0.8,-9,-9.0,-9,1014.4,1026.3,-9,-9.0,7.2,6.7,97.0,9.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3021,-9.0,-9.0,-9,7.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28759 |
+202310120500,281,11,0.7,-9,-9.0,-9,1014.6,1026.5,-9,-9.0,6.7,6.4,98.0,9.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2629,-9.0,-9.0,-9,7.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28760 |
+202310120600,281,0,0.2,-9,-9.0,-9,1014.7,1026.6,2,0.8,6.8,6.5,98.0,9.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2768,-9.0,-9.0,-9,6.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28761 |
+202310120700,281,0,0.4,-9,-9.0,-9,1014.8,1026.7,-9,-9.0,6.8,6.5,98.0,9.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2450,0.2,-9.0,-9,7.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28762 |
+202310120800,281,0,0.3,-9,-9.0,-9,1015.4,1027.2,-9,-9.0,8.8,7.0,89.0,10.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,2881,1.0,-9.0,-9,15.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28763 |
+202310120900,281,0,0.3,-9,-9.0,-9,1015.7,1027.3,2,0.7,12.7,8.1,74.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3468,1.0,-9.0,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28764 |
+202310121000,281,27,0.9,-9,-9.0,-9,1015.4,1026.9,-9,-9.0,16.3,8.5,60.0,11.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4293,1.0,-9.0,-9,28.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28765 |
+202310121100,281,18,0.8,-9,-9.0,-9,1014.9,1026.2,-9,-9.0,18.6,9.0,54.0,11.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4727,1.0,-9.0,-9,33.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28766 |
+202310121200,281,34,1.0,-9,-9.0,-9,1013.9,1025.2,7,-2.1,20.4,7.7,44.0,10.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,36.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28767 |
+202310121300,281,25,1.2,-9,-9.0,-9,1012.5,1023.7,-9,-9.0,22.0,8.1,41.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,38.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28768 |
+202310121400,281,32,1.5,-9,-9.0,-9,1011.7,1022.9,-9,-9.0,21.9,8.3,42.0,10.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,1,22,-,-9,-9,-9,5000,0.9,-9.0,-9,29.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28769 |
+202310121500,281,9,1.2,11,11.0,1437,1011.2,1022.3,7,-2.9,22.3,8.4,41.0,11.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,18,-,-9,-9,-9,4985,0.9,-9.0,-9,33.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28770 |
+202310121600,281,9,2.2,-9,-9.0,-9,1011.2,1022.4,-9,-9.0,21.6,9.7,47.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,5000,0.8,-9.0,-9,26.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28771 |
+202310121700,281,9,2.8,-9,-9.0,-9,1011.4,1022.7,-9,-9.0,20.1,10.2,53.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4946,0.4,-9.0,-9,20.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28772 |
+202310121800,281,7,1.5,-9,-9.0,-9,1011.6,1022.9,2,0.6,17.8,10.4,62.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,4589,0.4,-9.0,-9,16.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28773 |
+202310121900,281,7,0.8,-9,-9.0,-9,1012.0,1023.4,-9,-9.0,16.5,10.5,68.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3680,-9.0,-9.0,-9,14.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28774 |
+202310122000,281,0,0.1,-9,-9.0,-9,1012.3,1023.8,-9,-9.0,14.5,10.8,79.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3220,-9.0,-9.0,-9,12.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28775 |
+202310122100,281,0,0.2,-9,-9.0,-9,1012.5,1024.1,2,1.2,13.3,10.6,84.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,3149,-9.0,-9.0,-9,12.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28776 |
+202310122200,281,0,0.4,-9,-9.0,-9,1012.6,1024.2,-9,-9.0,12.2,10.7,91.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2723,-9.0,-9.0,-9,11.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28777 |
+202310122300,281,11,0.7,-9,-9.0,-9,1012.2,1023.8,-9,-9.0,11.8,10.6,93.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2393,-9.0,-9.0,-9,12.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28778 |
+202310130000,281,0,0.4,-9,-9.0,-9,1011.9,1023.5,8,-0.6,11.7,10.5,93.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,2574,-9.0,-9.0,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28779 |
+202310130100,281,9,0.9,-9,-9.0,-9,1011.5,1023.1,-9,-9.0,11.7,10.7,94.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,2745,-9.0,-9.0,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28780 |
+202310130200,281,5,0.5,-9,-9.0,-9,1011.4,1023.1,-9,-9.0,11.4,10.4,94.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,1,18,-,-9,-9,-9,2686,-9.0,-9.0,-9,11.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28781 |
+202310130300,281,0,0.2,-9,-9.0,-9,1011.0,1022.7,7,-0.8,11.2,10.5,96.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,2,34,-,-9,-9,-9,2586,-9.0,-9.0,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28782 |
+202310130400,281,0,0.2,-9,-9.0,-9,1010.7,1022.3,-9,-9.0,11.3,10.6,96.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,58,-,-9,-9,-9,2684,-9.0,-9.0,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28783 |
+202310130500,281,9,0.5,-9,-9.0,-9,1010.3,1022.0,-9,-9.0,10.8,10.3,97.0,12.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,2251,-9.0,-9.0,-9,10.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28784 |
+202310130600,281,0,0.2,-9,-9.0,-9,1010.1,1021.8,7,-0.9,10.4,10.0,98.0,12.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,-9,-,-9,-9,-9,1845,-9.0,-9.0,-9,10.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28785 |
+202310130700,281,5,0.6,-9,-9.0,-9,1010.2,1021.8,-9,-9.0,11.2,10.5,96.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,0,-9,-,-9,-9,-9,2272,0.0,-9.0,-9,11.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28786 |
+202310130800,281,9,0.9,-9,-9.0,-9,1010.3,1021.9,-9,-9.0,11.6,10.9,96.0,13.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,3,65,-,-9,-9,-9,1828,0.0,-9.0,-9,14.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28787 |
+202310130900,281,9,0.6,-9,-9.0,-9,1010.3,1021.8,4,0.0,14.0,11.5,85.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2680,0.5,-9.0,-9,22.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28788 |
+202310131000,281,11,0.8,-9,-9.0,-9,1009.5,1020.8,-9,-9.0,17.2,11.6,70.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,1,70,-,-9,-9,-9,3316,1.0,-9.0,-9,29.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,2, |
|
28789 |
+202310131100,281,23,0.9,-9,-9.0,-9,1008.5,1019.7,-9,-9.0,19.7,11.1,58.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,3550,1.0,-9.0,-9,32.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28790 |
+202310131200,281,16,1.6,-9,-9.0,-9,1007.6,1018.8,7,-3.0,21.0,9.2,47.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,2,65,-,-9,-9,-9,4894,1.0,-9.0,-9,36.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28791 |
+202310131300,281,23,1.0,-9,-9.0,-9,1006.3,1017.4,-9,-9.0,22.1,9.9,46.0,12.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,1,74,-,-9,-9,-9,4854,1.0,-9.0,-9,38.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28792 |
+202310131400,281,14,1.6,-9,-9.0,-9,1005.0,1016.1,-9,-9.0,22.2,9.3,44.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,3,59,-,-9,-9,-9,4899,1.0,-9.0,-9,37.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28793 |
+202310131500,281,18,0.7,-9,-9.0,-9,1004.5,1015.6,7,-3.2,21.6,8.8,44.0,11.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,1,17,-,-9,-9,-9,4523,0.4,-9.0,-9,27.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28794 |
+202310131600,281,11,1.5,-9,-9.0,-9,1004.1,1015.2,-9,-9.0,21.8,9.6,46.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,17,-,-9,-9,-9,4727,0.5,-9.0,-9,26.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28795 |
+202310131700,281,9,0.8,-9,-9.0,-9,1003.8,1014.9,-9,-9.0,20.8,10.5,52.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,3,17,-,-9,-9,-9,3865,0.0,-9.0,-9,21.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28796 |
+202310131800,281,9,1.1,-9,-9.0,-9,1004.0,1015.2,5,-0.4,19.1,11.6,62.0,13.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,5,17,-,-9,-9,-9,3617,0.0,-9.0,-9,18.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28797 |
+202310131900,281,7,0.8,-9,-9.0,-9,1004.0,1015.3,-9,-9.0,17.5,12.1,71.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,46,-,-9,-9,-9,2412,-9.0,-9.0,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28798 |
+202310132000,281,7,1.2,-9,-9.0,-9,1004.0,1015.3,-9,-9.0,16.5,12.2,76.0,14.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,49,-,-9,-9,-9,2823,-9.0,-9.0,-9,15.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28799 |
+202310132100,281,0,0.4,-9,-9.0,-9,1004.1,1015.5,2,0.3,15.7,12.4,81.0,14.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,48,-,-9,-9,-9,2624,-9.0,-9.0,-9,15.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28800 |
+202310132200,281,0,0.3,-9,-9.0,-9,1004.2,1015.6,-9,-9.0,15.1,12.5,85.0,14.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,43,-,-9,-9,-9,1727,-9.0,-9.0,-9,15.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28801 |
+202310132300,281,0,0.2,-9,-9.0,-9,1003.9,1015.3,-9,-9.0,14.9,12.9,88.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,1,18,-,-9,-9,-9,1396,-9.0,-9.0,-9,15.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28802 |
+202310140000,281,11,0.5,-9,-9.0,-9,1003.3,1014.7,8,-0.8,14.5,13.0,91.0,15.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,3,17,-,-9,-9,-9,1398,-9.0,-9.0,-9,14.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28803 |
+202310140100,281,7,0.6,-9,-9.0,-9,1002.7,1014.1,-9,-9.0,14.1,12.9,93.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,43,-,-9,-9,-9,1835,-9.0,-9.0,-9,14.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28804 |
+202310140200,281,0,0.3,-9,-9.0,-9,1002.4,1013.8,-9,-9.0,13.9,12.9,94.0,14.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,43,-,-9,-9,-9,1997,-9.0,-9.0,-9,14.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28805 |
+202310140300,281,0,0.4,-9,-9.0,-9,1002.0,1013.4,7,-1.3,13.4,12.7,96.0,14.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,42,-,-9,-9,-9,1975,-9.0,-9.0,-9,13.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28806 |
+202310140400,281,0,0.1,-9,-9.0,-9,1001.3,1012.7,-9,-9.0,13.2,12.7,97.0,14.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,41,-,-9,-9,-9,1939,-9.0,-9.0,-9,13.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28807 |
+202310140500,281,0,0.3,-9,-9.0,-9,1001.4,1012.9,-9,-9.0,12.6,12.2,98.0,14.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,-9,-,-9,-9,-9,1710,-9.0,-9.0,-9,11.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28808 |
+202310140600,281,0,0.1,-9,-9.0,-9,1001.2,1012.7,6,-0.7,12.1,11.9,99.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,42,-,-9,-9,-9,1047,-9.0,-9.0,-9,12.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28809 |
+202310140700,281,0,0.1,-9,-9.0,-9,1001.2,1012.7,-9,-9.0,11.7,11.5,99.0,13.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,46,-,-9,-9,-9,1495,0.0,-9.0,-9,11.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28810 |
+202310140800,281,0,0.1,-9,-9.0,-9,1001.4,1012.9,-9,-9.0,12.8,12.1,96.0,14.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1656,0.3,-9.0,-9,17.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28811 |
+202310140900,281,9,0.7,-9,-9.0,-9,1001.3,1012.7,4,0.0,15.2,12.6,85.0,14.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2053,0.9,-9.0,-9,22.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28812 |
+202310141000,281,7,0.8,-9,-9.0,-9,1000.9,1012.1,-9,-9.0,17.8,12.4,71.0,14.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2276,1.0,-9.0,-9,30.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28813 |
+202310141100,281,25,1.4,-9,-9.0,-9,1000.3,1011.4,-9,-9.0,20.2,11.9,59.0,13.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,2,40,-,-9,-9,-9,2311,1.0,-9.0,-9,31.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28814 |
+202310141200,281,25,1.6,-9,-9.0,-9,999.1,1010.1,7,-2.6,22.4,10.5,47.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,35,-,-9,-9,-9,2750,1.0,-9.0,-9,38.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28815 |
+202310141300,281,29,2.6,-9,-9.0,-9,998.1,1009.1,-9,-9.0,22.0,11.0,50.0,13.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,5,15,-,-9,-9,-9,2717,0.7,-9.0,-9,30.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28816 |
+202310141400,281,27,2.2,-9,-9.0,-9,997.1,1008.1,-9,-9.0,22.2,9.3,44.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,7,15,-,-9,-9,-9,3922,0.3,-9.0,-9,28.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28817 |
+202310141500,281,32,2.2,-9,-9.0,-9,996.6,1007.6,7,-2.5,22.2,9.6,45.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,6,17,-,-9,-9,-9,3346,0.2,-9.0,-9,26.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28818 |
+202310141600,281,32,2.5,-9,-9.0,-9,997.1,1008.3,-9,-9.0,17.6,13.5,77.0,15.5,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,20,-,-9,-9,-9,1265,0.0,-9.0,-9,18.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28819 |
+202310141700,281,11,0.9,-9,-9.0,-9,997.0,1008.2,-9,-9.0,16.8,13.5,81.0,15.5,0.6,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,22,-,-9,-9,-9,2281,0.0,-9.0,-9,18.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28820 |
+202310141800,281,11,0.7,-9,-9.0,-9,996.7,1008.0,0,0.4,15.8,14.4,92.0,16.4,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,3,18,-,-9,-9,-9,1386,0.1,-9.0,-9,15.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28821 |
+202310141900,281,0,0.2,-9,-9.0,-9,997.0,1008.4,-9,-9.0,14.9,14.5,98.0,16.5,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,2,17,-,-9,-9,-9,702,-9.0,-9.0,-9,14.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28822 |
+202310142000,281,7,0.8,-9,-9.0,-9,997.5,1008.9,-9,-9.0,13.9,13.7,99.0,15.7,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,16,-,-9,-9,-9,784,-9.0,-9.0,-9,14.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28823 |
+202310142100,281,32,1.9,-9,-9.0,-9,998.1,1009.5,2,1.5,13.9,13.8,100.0,15.8,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,6,-,-9,-9,-9,673,-9.0,-9.0,-9,14.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28824 |
+202310142200,281,20,0.6,-9,-9.0,-9,998.1,1009.5,-9,-9.0,14.0,12.8,93.0,14.8,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,2,47,-,-9,-9,-9,1793,-9.0,-9.0,-9,12.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28825 |
+202310142300,281,0,0.4,-9,-9.0,-9,998.1,1009.5,-9,-9.0,12.3,12.1,99.0,14.1,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,928,-9.0,-9.0,-9,11.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28826 |
+202310150000,281,0,0.4,-9,-9.0,-9,997.7,1009.2,7,-0.3,12.0,11.9,100.0,13.9,-9.0,0.6,0.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,701,-9.0,-9.0,-9,10.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28827 |
+202310150100,281,0,0.3,-9,-9.0,-9,997.6,1009.1,-9,-9.0,10.9,10.8,100.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,470,-9.0,-9.0,-9,10.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28828 |
+202310150200,281,0,0.4,-9,-9.0,-9,997.6,1009.1,-9,-9.0,10.7,10.6,100.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,13,-,-9,-9,-9,124,-9.0,-9.0,-9,12.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28829 |
+202310150300,281,9,0.7,-9,-9.0,-9,997.5,1009.0,7,-0.2,10.6,10.5,100.0,12.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,2,18,-,-9,-9,-9,397,-9.0,-9.0,-9,11.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28830 |
+202310150400,281,29,1.1,-9,-9.0,-9,997.5,1009.0,-9,-9.0,10.9,10.8,100.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,731,-9.0,-9.0,-9,10.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28831 |
+202310150500,281,25,0.7,-9,-9.0,-9,997.8,1009.4,-9,-9.0,10.4,10.3,100.0,12.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,92,-9.0,-9.0,-9,9.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28832 |
+202310150600,281,18,0.7,-9,-9.0,-9,998.4,1010.0,2,1.0,10.3,10.2,100.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,3,0,-9,-,-9,-9,-9,528,-9.0,-9.0,-9,9.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28833 |
+202310150700,281,27,1.1,-9,-9.0,-9,998.7,1010.2,-9,-9.0,12.1,11.1,94.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,1104,0.0,-9.0,-9,10.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28834 |
+202310150800,281,23,1.0,-9,-9.0,-9,999.1,1010.5,-9,-9.0,13.5,11.1,86.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1340,1.0,-9.0,-9,16.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28835 |
+202310150900,281,27,2.1,-9,-9.0,-9,999.4,1010.8,2,0.8,15.2,11.1,77.0,13.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1496,0.9,-9.0,-9,20.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28836 |
+202310151000,281,29,3.7,-9,-9.0,-9,999.5,1010.7,-9,-9.0,17.6,10.9,65.0,13.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2247,1.0,-9.0,-9,26.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28837 |
+202310151100,281,29,3.9,-9,-9.0,-9,999.4,1010.5,-9,-9.0,18.9,10.9,60.0,13.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2998,1.0,-9.0,-9,29.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28838 |
+202310151200,281,29,3.1,-9,-9.0,-9,999.1,1010.2,7,-0.6,19.5,11.2,59.0,13.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,10,-,-9,-9,-9,3183,0.8,-9.0,-9,30.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28839 |
+202310151300,281,32,2.6,-9,-9.0,-9,998.6,1009.7,-9,-9.0,19.9,10.8,56.0,12.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,6,13,-,-9,-9,-9,4313,0.4,-9.0,-9,29.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28840 |
+202310151400,281,29,2.7,-9,-9.0,-9,998.2,1009.3,-9,-9.0,21.3,10.1,49.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,13,-,-9,-9,-9,4712,0.7,-9.0,-9,31.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28841 |
+202310151500,281,29,3.6,29,10.0,1420,998.1,1009.2,7,-1.0,20.7,9.2,48.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,15,-,-9,-9,-9,4780,0.8,-9.0,-9,27.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28842 |
+202310151600,281,29,3.1,-9,-9.0,-9,998.6,1009.7,-9,-9.0,20.4,9.6,50.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,4633,0.8,-9.0,-9,24.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28843 |
+202310151700,281,29,2.6,-9,-9.0,-9,998.8,1009.9,-9,-9.0,19.4,9.2,52.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,4060,0.2,-9.0,-9,18.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28844 |
+202310151800,281,29,1.0,-9,-9.0,-9,999.4,1010.7,2,1.5,16.8,10.6,67.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3401,0.4,-9.0,-9,14.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28845 |
+202310151900,281,29,1.5,-9,-9.0,-9,1000.0,1011.3,-9,-9.0,14.6,10.6,77.0,12.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2589,-9.0,-9.0,-9,13.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28846 |
+202310152000,281,27,2.5,-9,-9.0,-9,1000.4,1011.8,-9,-9.0,15.3,10.4,73.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2430,-9.0,-9.0,-9,12.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28847 |
+202310152100,281,29,1.7,-9,-9.0,-9,1000.7,1012.1,2,1.4,14.2,10.4,78.0,12.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2120,-9.0,-9.0,-9,12.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28848 |
+202310152200,281,27,1.4,-9,-9.0,-9,1001.2,1012.6,-9,-9.0,14.8,9.7,72.0,12.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2480,-9.0,-9.0,-9,11.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28849 |
+202310152300,281,27,2.5,-9,-9.0,-9,1001.4,1012.8,-9,-9.0,15.3,9.4,68.0,11.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2787,-9.0,-9.0,-9,11.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28850 |
+202310160000,281,27,2.6,-9,-9.0,-9,1001.5,1012.9,2,0.8,14.5,9.5,72.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,2479,-9.0,-9.0,-9,11.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28851 |
+202310160100,281,29,1.5,-9,-9.0,-9,1002.1,1013.5,-9,-9.0,13.7,9.1,74.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2565,-9.0,-9.0,-9,10.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28852 |
+202310160200,281,29,1.3,-9,-9.0,-9,1002.4,1013.9,-9,-9.0,12.0,9.3,84.0,11.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1985,-9.0,-9.0,-9,10.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28853 |
+202310160300,281,0,0.3,-9,-9.0,-9,1002.3,1013.9,1,1.0,11.3,9.5,89.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,1558,-9.0,-9.0,-9,9.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28854 |
+202310160400,281,0,0.0,-9,-9.0,-9,1001.9,1013.5,-9,-9.0,10.2,9.1,93.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1266,-9.0,-9.0,-9,9.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28855 |
+202310160500,281,18,0.7,-9,-9.0,-9,1002.4,1014.0,-9,-9.0,10.1,8.6,91.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,1507,-9.0,-9.0,-9,8.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28856 |
+202310160600,281,7,0.5,-9,-9.0,-9,1002.8,1014.4,3,0.5,9.0,8.6,98.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,1015,-9.0,-9.0,-9,8.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28857 |
+202310160700,281,0,0.1,-9,-9.0,-9,1003.2,1014.8,-9,-9.0,9.6,9.1,97.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,1,11,-,-9,-9,-9,960,0.0,-9.0,-9,9.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28858 |
+202310160800,281,0,0.1,-9,-9.0,-9,1004.0,1015.5,-9,-9.0,11.7,9.9,89.0,12.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1162,0.9,-9.0,-9,16.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28859 |
+202310160900,281,29,2.0,-9,-9.0,-9,1004.3,1015.6,2,1.2,17.0,11.4,70.0,13.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,2174,0.9,-9.0,-9,20.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28860 |
+202310161000,281,27,3.8,-9,-9.0,-9,1004.1,1015.3,-9,-9.0,18.8,9.8,56.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,0,-9,-,-9,-9,-9,2598,1.0,-9.0,-9,28.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28861 |
+202310161100,281,36,2.8,-9,-9.0,-9,1003.9,1015.1,-9,-9.0,18.5,9.2,55.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,6,11,-,-9,-9,-9,3071,0.7,-9.0,-9,30.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28862 |
+202310161200,281,32,3.2,-9,-9.0,-9,1003.5,1014.7,7,-0.9,20.1,8.1,46.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,4,12,-,-9,-9,-9,3435,0.9,-9.0,-9,34.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28863 |
+202310161300,281,32,3.0,-9,-9.0,-9,1002.7,1013.8,-9,-9.0,20.8,8.7,46.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,1,54,-,-9,-9,-9,3734,0.9,-9.0,-9,34.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28864 |
+202310161400,281,29,3.0,-9,-9.0,-9,1002.3,1013.4,-9,-9.0,21.4,7.2,40.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4031,1.0,-9.0,-9,34.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28865 |
+202310161500,281,27,2.0,-9,-9.0,-9,1002.4,1013.5,5,-1.2,21.3,6.7,39.0,9.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4483,1.0,-9.0,-9,30.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28866 |
+202310161600,281,27,3.2,-9,-9.0,-9,1002.5,1013.7,-9,-9.0,20.4,6.6,41.0,9.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4366,1.0,-9.0,-9,25.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28867 |
+202310161700,281,29,2.6,-9,-9.0,-9,1002.6,1013.7,-9,-9.0,19.6,7.3,45.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4196,0.3,-9.0,-9,18.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28868 |
+202310161800,281,29,1.3,-9,-9.0,-9,1002.9,1014.2,2,0.7,16.5,8.9,61.0,11.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3686,0.4,-9.0,-9,14.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28869 |
+202310161900,281,29,1.8,-9,-9.0,-9,1003.2,1014.6,-9,-9.0,14.4,8.7,69.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2928,-9.0,-9.0,-9,12.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28870 |
+202310162000,281,7,0.9,-9,-9.0,-9,1003.7,1015.2,-9,-9.0,12.7,9.1,79.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2143,-9.0,-9.0,-9,11.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28871 |
+202310162100,281,0,0.2,-9,-9.0,-9,1004.2,1015.8,2,1.6,11.6,9.1,85.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1854,-9.0,-9.0,-9,10.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28872 |
+202310162200,281,0,0.2,-9,-9.0,-9,1004.7,1016.3,-9,-9.0,10.4,8.6,89.0,11.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,1925,-9.0,-9.0,-9,9.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28873 |
+202310162300,281,29,2.1,-9,-9.0,-9,1004.8,1016.3,-9,-9.0,12.7,8.1,74.0,10.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2750,-9.0,-9.0,-9,9.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28874 |
+202310170000,281,32,3.2,-9,-9.0,-9,1005.1,1016.6,2,0.8,13.3,6.8,65.0,9.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3909,-9.0,-9.0,-9,10.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28875 |
+202310170100,281,27,2.1,-9,-9.0,-9,1005.6,1017.1,-9,-9.0,12.1,6.3,68.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3949,-9.0,-9.0,-9,9.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28876 |
+202310170200,281,29,2.0,-9,-9.0,-9,1005.8,1017.4,-9,-9.0,12.0,4.9,62.0,8.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4874,-9.0,-9.0,-9,8.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28877 |
+202310170300,281,27,1.6,-9,-9.0,-9,1005.8,1017.4,1,0.8,10.4,4.7,68.0,8.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4679,-9.0,-9.0,-9,7.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28878 |
+202310170400,281,29,0.9,-9,-9.0,-9,1005.9,1017.6,-9,-9.0,8.2,4.9,80.0,8.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4482,-9.0,-9.0,-9,6.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28879 |
+202310170500,281,0,0.2,-9,-9.0,-9,1006.2,1018.0,-9,-9.0,7.0,4.9,87.0,8.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4023,-9.0,-9.0,-9,5.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28880 |
+202310170600,281,29,1.3,-9,-9.0,-9,1006.5,1018.3,2,0.9,6.8,5.1,89.0,8.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3232,-9.0,-9.0,-9,5.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28881 |
+202310170700,281,0,0.1,-9,-9.0,-9,1007.1,1018.9,-9,-9.0,7.8,5.9,88.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2912,0.1,-9.0,-9,5.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28882 |
+202310170800,281,0,0.2,-9,-9.0,-9,1007.8,1019.5,-9,-9.0,10.5,6.2,75.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2948,1.0,-9.0,-9,15.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28883 |
+202310170900,281,27,2.1,-9,-9.0,-9,1008.1,1019.6,2,1.3,14.1,5.6,57.0,9.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4582,0.9,-9.0,-9,16.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28884 |
+202310171000,281,27,2.8,-9,-9.0,-9,1008.1,1019.5,-9,-9.0,16.0,4.0,45.0,8.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4968,1.0,-9.0,-9,26.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28885 |
+202310171100,281,27,3.3,-9,-9.0,-9,1008.0,1019.3,-9,-9.0,17.3,3.9,41.0,8.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,30.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28886 |
+202310171200,281,27,2.5,-9,-9.0,-9,1007.6,1018.8,7,-0.8,19.2,3.3,35.0,7.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,34.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28887 |
+202310171300,281,29,2.3,-9,-9.0,-9,1007.0,1018.2,-9,-9.0,20.1,1.4,29.0,6.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,34.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28888 |
+202310171400,281,29,2.0,-9,-9.0,-9,1006.6,1017.8,-9,-9.0,21.1,2.3,29.0,7.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,34.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28889 |
+202310171500,281,25,2.1,-9,-9.0,-9,1006.5,1017.7,7,-1.1,20.8,2.5,30.0,7.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,30.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28890 |
+202310171600,281,27,2.5,-9,-9.0,-9,1006.4,1017.6,-9,-9.0,20.3,4.7,36.0,8.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,1.0,-9.0,-9,25.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28891 |
+202310171700,281,32,1.6,-9,-9.0,-9,1006.8,1018.0,-9,-9.0,20.0,3.2,33.0,7.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.4,-9.0,-9,17.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28892 |
+202310171800,281,29,1.4,-9,-9.0,-9,1007.6,1019.0,3,1.3,16.2,5.4,49.0,9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,5000,0.4,-9.0,-9,13.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28893 |
+202310171900,281,11,0.6,-9,-9.0,-9,1008.4,1019.9,-9,-9.0,13.4,6.9,65.0,9.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3455,-9.0,-9.0,-9,11.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28894 |
+202310172000,281,0,0.4,-9,-9.0,-9,1008.6,1020.2,-9,-9.0,11.6,7.1,74.0,10.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3599,-9.0,-9.0,-9,9.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28895 |
+202310172100,281,0,0.2,-9,-9.0,-9,1009.1,1020.8,2,1.8,10.2,7.2,82.0,10.2,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3615,-9.0,-9.0,-9,8.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28896 |
+202310172200,281,0,0.4,-9,-9.0,-9,1009.5,1021.2,-9,-9.0,9.3,7.0,86.0,10.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3010,-9.0,-9.0,-9,8.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28897 |
+202310172300,281,0,0.3,-9,-9.0,-9,1009.7,1021.5,-9,-9.0,8.3,7.0,92.0,10.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3293,-9.0,-9.0,-9,7.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28898 |
+202310180000,281,0,0.3,-9,-9.0,-9,1009.6,1021.4,0,0.6,7.3,5.9,91.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3752,-9.0,-9.0,-9,6.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28899 |
+202310180100,281,0,0.1,-9,-9.0,-9,1009.7,1021.5,-9,-9.0,6.8,6.2,96.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3133,-9.0,-9.0,-9,6.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28900 |
+202310180200,281,0,0.4,-9,-9.0,-9,1009.8,1021.7,-9,-9.0,6.1,5.3,95.0,8.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3466,-9.0,-9.0,-9,5.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28901 |
+202310180300,281,0,0.4,-9,-9.0,-9,1009.8,1021.7,1,0.3,5.6,5.0,96.0,8.7,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3266,-9.0,-9.0,-9,5.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28902 |
+202310180400,281,0,0.3,-9,-9.0,-9,1009.9,1021.8,-9,-9.0,5.2,4.6,96.0,8.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,3119,-9.0,-9.0,-9,4.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28903 |
+202310180500,281,0,0.2,-9,-9.0,-9,1010.0,1021.9,-9,-9.0,5.0,4.7,98.0,8.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2604,-9.0,-9.0,-9,4.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28904 |
+202310180600,281,0,0.3,-9,-9.0,-9,1010.0,1021.9,1,0.2,4.6,4.4,99.0,8.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,1,0,-9,-,-9,-9,-9,1484,-9.0,-9.0,-9,4.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28905 |
+202310180700,281,0,0.0,-9,-9.0,-9,1010.4,1022.3,-9,-9.0,4.6,4.3,98.0,8.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2706,0.0,-9.0,-9,5.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28906 |
+202310180800,281,14,0.5,-9,-9.0,-9,1010.9,1022.8,-9,-9.0,6.5,5.1,91.0,8.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,2493,1.0,-9.0,-9,11.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28907 |
+202310180900,281,20,0.7,-9,-9.0,-9,1010.9,1022.6,0,0.7,10.2,6.3,77.0,9.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,3309,0.9,-9.0,-9,13.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28908 |
+202310181000,281,23,1.4,-9,-9.0,-9,1010.9,1022.4,-9,-9.0,14.4,6.7,60.0,9.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,3495,1.0,-9.0,-9,24.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28909 |
+202310181100,281,27,1.0,-9,-9.0,-9,1010.3,1021.6,-9,-9.0,17.9,7.0,49.0,10.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3682,1.0,-9.0,-9,32.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28910 |
+202310181200,281,23,1.5,-9,-9.0,-9,1009.3,1020.5,7,-2.1,19.7,6.0,41.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,4335,1.0,-9.0,-9,34.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28911 |
+202310181300,281,27,1.7,-9,-9.0,-9,1007.8,1018.9,-9,-9.0,22.3,6.0,35.0,9.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4768,1.0,-9.0,-9,35.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28912 |
+202310181400,281,23,2.3,-9,-9.0,-9,1006.9,1018.0,-9,-9.0,22.4,5.3,33.0,8.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,0,0,-9,-,-9,-9,-9,4881,1.0,-9.0,-9,33.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28913 |
+202310181500,281,25,2.3,-9,-9.0,-9,1006.6,1017.7,7,-2.8,22.8,5.2,32.0,8.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,0,-9,-,-9,-9,-9,4990,1.0,-9.0,-9,30.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28914 |
+202310181600,281,25,1.5,-9,-9.0,-9,1006.0,1017.1,-9,-9.0,22.6,7.5,38.0,10.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,3606,0.9,-9.0,-9,26.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28915 |
+202310181700,281,29,1.6,-9,-9.0,-9,1006.2,1017.3,-9,-9.0,22.1,8.5,42.0,11.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,2,0,-9,-,-9,-9,-9,3419,0.4,-9.0,-9,19.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28916 |
+202310181800,281,29,0.8,-9,-9.0,-9,1006.7,1018.0,3,0.3,17.5,9.1,58.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,4638,0.3,-9.0,-9,15.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28917 |
+202310181900,281,0,0.2,-9,-9.0,-9,1006.8,1018.2,-9,-9.0,16.2,9.8,66.0,12.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2639,-9.0,-9.0,-9,13.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28918 |
+202310182000,281,0,0.2,-9,-9.0,-9,1007.2,1018.7,-9,-9.0,13.2,10.0,81.0,12.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2560,-9.0,-9.0,-9,11.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28919 |
+202310182100,281,0,0.2,-9,-9.0,-9,1007.6,1019.1,2,1.1,12.7,10.0,84.0,12.3,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2711,-9.0,-9.0,-9,11.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28920 |
+202310182200,281,7,0.8,-9,-9.0,-9,1007.8,1019.4,-9,-9.0,11.5,9.5,88.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,4,0,-9,-,-9,-9,-9,2484,-9.0,-9.0,-9,10.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28921 |
+202310182300,281,0,0.2,-9,-9.0,-9,1008.0,1019.6,-9,-9.0,10.9,9.6,92.0,11.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2321,-9.0,-9.0,-9,9.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28922 |
+202310190000,281,0,0.2,-9,-9.0,-9,1007.9,1019.6,1,0.5,10.4,9.4,94.0,11.8,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,5,1,51,-,-9,-9,-9,2342,-9.0,-9.0,-9,9.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28923 |
+202310190100,281,0,0.4,-9,-9.0,-9,1007.6,1019.3,-9,-9.0,9.9,9.1,95.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,4,48,-,-9,-9,-9,2686,-9.0,-9.0,-9,9.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28924 |
+202310190200,281,0,0.4,-9,-9.0,-9,1007.3,1019.0,-9,-9.0,9.7,9.0,96.0,11.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,55,-,-9,-9,-9,2418,-9.0,-9.0,-9,9.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28925 |
+202310190300,281,0,0.0,-9,-9.0,-9,1007.0,1018.6,7,-1.0,9.7,9.2,97.0,11.6,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,0,-9,-,-9,-9,-9,2314,-9.0,-9.0,-9,8.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28926 |
+202310190400,281,0,0.2,-9,-9.0,-9,1006.9,1018.6,-9,-9.0,8.9,8.5,98.0,11.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,41,-,-9,-9,-9,1990,-9.0,-9.0,-9,8.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28927 |
+202310190500,281,0,0.2,-9,-9.0,-9,1006.6,1018.3,-9,-9.0,8.8,8.4,98.0,11.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,6,40,-,-9,-9,-9,2095,-9.0,-9.0,-9,8.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28928 |
+202310190600,281,0,0.2,-9,-9.0,-9,1006.6,1018.3,6,-0.3,8.6,8.2,98.0,10.9,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,7,7,38,-,-9,-9,-9,1754,-9.0,-9.0,-9,7.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28929 |
+202310190700,281,27,0.5,-9,-9.0,-9,1006.9,1018.6,-9,-9.0,9.0,8.5,97.0,11.1,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,3,71,-,-9,-9,-9,2216,0.0,-9.0,-9,9.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28930 |
+202310190800,281,0,0.2,-9,-9.0,-9,1007.5,1019.2,-9,-9.0,10.0,8.9,93.0,11.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,0,-9,-,-9,-9,-9,2120,0.1,-9.0,-9,12.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28931 |
+202310190900,281,0,0.0,-9,-9.0,-9,1007.3,1018.8,0,0.5,12.6,10.3,86.0,12.5,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,7,37,-,-9,-9,-9,2411,0.1,-9.0,-9,17.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28932 |
+202310191000,281,25,0.9,-9,-9.0,-9,1007.2,1018.7,-9,-9.0,15.1,10.2,73.0,12.4,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,41,-,-9,-9,-9,2264,0.4,-9.0,-9,22.4,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28933 |
+202310191100,281,27,1.0,-9,-9.0,-9,1006.7,1018.1,-9,-9.0,16.3,11.0,71.0,13.1,0.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,6,33,-,-9,-9,-9,2105,0.0,-9.0,-9,20.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28934 |
+202310191200,281,23,1.6,-9,-9.0,-9,1005.8,1017.0,7,-1.8,18.9,12.6,67.0,14.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,38,-,-9,-9,-9,1943,0.3,-9.0,-9,31.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28935 |
+202310191300,281,27,1.8,-9,-9.0,-9,1004.7,1015.8,-9,-9.0,22.0,11.0,50.0,13.1,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,7,32,-,-9,-9,-9,3749,0.9,-9.0,-9,36.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28936 |
+202310191400,281,25,1.6,-9,-9.0,-9,1003.6,1014.7,-9,-9.0,21.6,8.4,43.0,11.0,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,5,42,-,-9,-9,-9,4896,0.6,-9.0,-9,33.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28937 |
+202310191500,281,27,1.0,-9,-9.0,-9,1003.1,1014.2,7,-2.8,22.2,7.9,40.0,10.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,6,2,32,-,-9,-9,-9,3950,0.9,-9.0,-9,29.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28938 |
+202310191600,281,23,0.9,-9,-9.0,-9,1002.6,1013.7,-9,-9.0,21.3,8.8,45.0,11.3,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,29,-,-9,-9,-9,2947,0.2,-9.0,-9,24.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28939 |
+202310191700,281,27,1.7,-9,-9.0,-9,1002.7,1013.9,-9,-9.0,20.6,8.2,45.0,10.9,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,27,-,-9,-9,-9,3915,0.0,-9.0,-9,20.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28940 |
+202310191800,281,27,0.9,-9,-9.0,-9,1002.7,1013.9,5,-0.3,19.8,9.3,51.0,11.7,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,20,-,-9,-9,-9,3449,0.0,-9.0,-9,18.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28941 |
+202310191900,281,0,0.4,-9,-9.0,-9,1002.6,1013.8,-9,-9.0,18.1,11.1,64.0,13.2,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,3,20,-,-9,-9,-9,2850,-9.0,-9.0,-9,17.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28942 |
+202310192000,281,29,2.0,-9,-9.0,-9,1002.9,1014.1,-9,-9.0,17.7,11.4,67.0,13.5,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,8,8,16,-,-9,-9,-9,2919,-9.0,-9.0,-9,17.1,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28943 |
+202310192100,281,32,1.4,-9,-9.0,-9,1002.9,1014.1,1,0.2,17.6,12.0,70.0,14.0,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,9,13,-,-9,-9,-9,3237,-9.0,-9.0,-9,16.9,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28944 |
+202310192200,281,34,1.6,-9,-9.0,-9,1003.4,1014.7,-9,-9.0,17.1,12.6,75.0,14.6,-9.0,0.0,0.0,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,3,-,-9,-9,-9,2605,-9.0,-9.0,-9,16.5,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,3,-9, |
|
28945 |
+202310192300,281,29,1.5,-9,-9.0,-9,1003.3,1014.7,-9,-9.0,15.1,14.9,99.0,16.9,1.3,1.3,1.3,-9.0,-9.0,-9.0,-9.0,-9,-9,-,9,6,1,-,-9,-9,-9,234,-9.0,-9.0,-9,15.7,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28946 |
+202310200000,281,27,2.2,-9,-9.0,-9,1003.4,1014.8,2,0.7,13.7,11.5,87.0,13.6,0.5,1.8,1.8,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,9,10,-,-9,-9,-9,3245,-9.0,-9.0,-9,14.2,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28947 |
+202310200100,281,27,0.8,-9,-9.0,-9,1003.7,1015.1,-9,-9.0,13.4,11.0,86.0,13.1,0.3,0.3,0.3,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,8,13,-,-9,-9,-9,2715,-9.0,-9.0,-9,13.8,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28948 |
+202310200200,281,29,0.8,-9,-9.0,-9,1003.0,1014.5,-9,-9.0,12.8,11.6,93.0,13.7,0.5,0.8,0.8,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,1,20,-,-9,-9,-9,1657,-9.0,-9.0,-9,13.6,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28949 |
+202310200300,281,27,1.9,-9,-9.0,-9,1002.8,1014.3,8,-0.5,12.9,11.7,93.0,13.7,0.9,1.7,1.7,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,2,18,-,-9,-9,-9,1396,-9.0,-9.0,-9,13.3,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
|
28950 |
+202310200400,281,27,1.2,-9,-9.0,-9,1002.4,1013.9,-9,-9.0,12.5,10.7,89.0,12.9,0.9,2.6,2.6,-9.0,-9.0,-9.0,-9.0,-9,-9,-,10,10,21,-,-9,-9,-9,4776,-9.0,-9.00,-9,13.0,-99.0,-99.0,-99.0,-99.0,-9,-9.0,-9,1,-9, |
--- database/DBupload.py
+++ database/DBupload_ncdata.py
No changes |
--- tools/algo/SARIMA.py
+++ tools/algo/SARIMA.py
... | ... | @@ -40,7 +40,7 @@ |
40 | 40 |
|
41 | 41 |
forecast_df.to_csv(f"{file.split('.')[0]}_forecast.csv", index=False) |
42 | 42 |
|
43 |
- with open(f'sarima_model_{save_name}.pkl', 'wb') as pkl_file: |
|
43 |
+ with open(f'sarima_model_{col_key}.pkl', 'wb') as pkl_file: |
|
44 | 44 |
pickle.dump(model_fit, pkl_file) |
45 | 45 |
|
46 | 46 |
def forecast_from_saved_model(file, model_file, future_hours=24): |
... | ... | @@ -70,10 +70,11 @@ |
70 | 70 |
return forecast_df |
71 | 71 |
|
72 | 72 |
if __name__ == "__main__": |
73 |
- # sarima("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308310000_f.csv", "test1", ) |
|
74 |
- forecast_from_saved_model("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308310000_f.csv", |
|
75 |
- "/home/juni/PycharmProjects/failure_analysis/tools/algo/sarima_model_test1.pkl", |
|
76 |
- 24) |
|
73 |
+ sarima("/home/juni/PycharmProjects/failure_analysis/data/weather/weather_data.csv", "test1", "상대습도") |
|
74 |
+ # forecast = forecast_from_saved_model("/home/juni/PycharmProjects/failure_analysis/data/weather/weather_data.csv", |
|
75 |
+ # "/home/juni/PycharmProjects/failure_analysis/tools/algo/sarima_model_test1.pkl", |
|
76 |
+ # 24) |
|
77 |
+ px.bar(forecast) |
|
77 | 78 |
# df = pd.read_csv("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308310000_f.csv") |
78 | 79 |
# ah = absolute_humidity(df["상대습도"], df["기온"]) |
79 | 80 |
# df['관측시각'] = df['관측시각'].apply(lambda x: datetime.strptime(f"{x}", '%Y%m%d%H%M')) |
--- tools/weather_agency_api/weather_api.py
+++ tools/weather_agency_api/weather_api.py
... | ... | @@ -5,7 +5,9 @@ |
5 | 5 |
from io import StringIO |
6 | 6 |
from tools.weather_agency_api.duplicate_check import duplicate_check |
7 | 7 |
from tools.weather_agency_api.check_missing import check_missing |
8 |
+from sqlalchemy import create_engine |
|
8 | 9 |
|
10 |
+DATABASE_URL = "postgresql+psycopg2://username:ts4430!@@localhost:5432/welding" |
|
9 | 11 |
# https://apihub.kma.go.kr/ 참고 |
10 | 12 |
weather_api_columns = [ |
11 | 13 |
"관측시각", |
... | ... | @@ -81,7 +83,57 @@ |
81 | 83 |
return response.text |
82 | 84 |
|
83 | 85 |
|
84 |
-def update_weather_info_to_today(file_name): |
|
86 |
+def update_weather_info_to_today(): |
|
87 |
+ """ |
|
88 |
+ Updates the weather information up to today at 00:00. |
|
89 |
+ """ |
|
90 |
+ |
|
91 |
+ # Set up a connection using SQLAlchemy (for Pandas operations) |
|
92 |
+ engine = create_engine(DATABASE_URL) |
|
93 |
+ |
|
94 |
+ # Load existing data |
|
95 |
+ query = "SELECT * FROM weather_data" |
|
96 |
+ df_weather_existing = pd.read_sql(query, engine) |
|
97 |
+ |
|
98 |
+ # Find the last date available in the database |
|
99 |
+ last_date_str = f'{df_weather_existing.iloc[-1]["관측시각"]}' |
|
100 |
+ last_date = datetime.strptime(last_date_str, '%Y%m%d%H%M') |
|
101 |
+ |
|
102 |
+ # Get today's date at 00:00 |
|
103 |
+ today_00 = datetime.now().replace(hour=0, minute=0, second=0, microsecond=0) |
|
104 |
+ |
|
105 |
+ # If the last date in the database is not today's date, fetch data and append |
|
106 |
+ if last_date < today_00: |
|
107 |
+ start_date = (last_date + timedelta(hours=1)).strftime('%Y%m%d%H%M') |
|
108 |
+ end_date = today_00.strftime('%Y%m%d%H%M') |
|
109 |
+ |
|
110 |
+ date_lists = generate_dates(start_date, end_date) # Ensure this function is defined |
|
111 |
+ |
|
112 |
+ df_weather_new = pd.DataFrame() |
|
113 |
+ k = 24 |
|
114 |
+ for i, date in enumerate(range(0, len(date_lists) - 1, k)): |
|
115 |
+ if date + k <= len(date_lists): |
|
116 |
+ end_day = date + k |
|
117 |
+ else: |
|
118 |
+ end_day = len(date_lists) - 1 |
|
119 |
+ text = call_administration_of_weather_api(date_lists[date], date_lists[end_day]) # Ensure this function is defined |
|
120 |
+ buffer = StringIO(text) |
|
121 |
+ df = pd.read_csv(buffer, skiprows=2, skipfooter=1, sep=r"\s+", header=None, index_col=False, engine="python").iloc[2:, :-1] |
|
122 |
+ df = df.set_axis(weather_api_columns, axis=1, inplace=False) # Ensure 'weather_api_columns' is defined |
|
123 |
+ if not check_missing(df): # Ensure this function is defined |
|
124 |
+ print("API is not working!") |
|
125 |
+ return { |
|
126 |
+ "responses": 500 |
|
127 |
+ } |
|
128 |
+ df_weather_new = pd.concat([df_weather_new, df], ignore_index=True) |
|
129 |
+ print(f"{i}/{len(range(0, len(date_lists) - 1, k)) - 1}") |
|
130 |
+ |
|
131 |
+ # Append the new data to the existing data in the database |
|
132 |
+ df_weather_new.to_sql('weather_data', engine, if_exists='append', index=False) |
|
133 |
+ else: |
|
134 |
+ print("Weather data is already up-to-date!") |
|
135 |
+ |
|
136 |
+def update_weather_info_to_today_csv(file_name): |
|
85 | 137 |
""" |
86 | 138 |
Updates the weather information up to today at 00:00. |
87 | 139 |
:param file_name: CSV file name containing weather data. |
... | ... | @@ -96,7 +148,8 @@ |
96 | 148 |
|
97 | 149 |
# Get today's date at 00:00 |
98 | 150 |
today_00 = datetime.now().replace(hour=0, minute=0, second=0, microsecond=0) |
99 |
- |
|
151 |
+ print(today_00) |
|
152 |
+ print(last_date) |
|
100 | 153 |
# If the last date in CSV is not today's date, fetch data and append |
101 | 154 |
if last_date < today_00: |
102 | 155 |
start_date = (last_date + timedelta(hours=1)).strftime('%Y%m%d%H%M') |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?