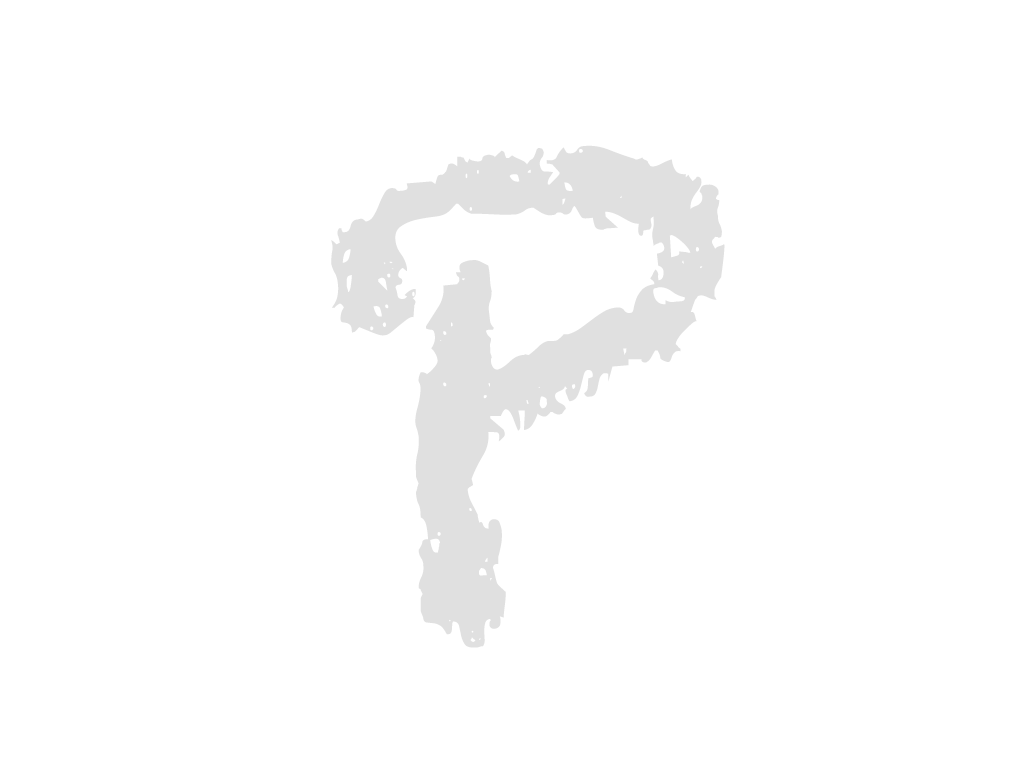
+++ .bundle/config
... | ... | @@ -0,0 +1,2 @@ |
1 | +BUNDLE_PATH: "vendor/bundle" | |
2 | +BUNDLE_FORCE_RUBY_PLATFORM: 1 |
+++ .eslintrc.js
... | ... | @@ -0,0 +1,12 @@ |
1 | +module.exports = { | |
2 | + root: true, | |
3 | + extends: ['plugin:prettier/recommended', '@react-native'], | |
4 | + rules: { | |
5 | + 'prettier/prettier': [ | |
6 | + 'error', | |
7 | + { | |
8 | + endOfLine: 'auto', | |
9 | + }, | |
10 | + ], | |
11 | + }, | |
12 | +}; |
+++ .git ignore
... | ... | @@ -0,0 +1,10 @@ |
1 | +*.iml | |
2 | +.gradle | |
3 | +/local.properties | |
4 | +/.idea/workspace.xml | |
5 | +/.idea/libraries | |
6 | +.idea | |
7 | +.DS_Store | |
8 | +/build | |
9 | +/captures | |
10 | +.externalNativeBuild(파일 끝에 줄바꿈 문자 없음) |
+++ .gitignore
... | ... | @@ -0,0 +1,74 @@ |
1 | +# OSX | |
2 | +# | |
3 | +.DS_Store | |
4 | + | |
5 | +# Xcode | |
6 | +# | |
7 | +build/ | |
8 | +*.pbxuser | |
9 | +!default.pbxuser | |
10 | +*.mode1v3 | |
11 | +!default.mode1v3 | |
12 | +*.mode2v3 | |
13 | +!default.mode2v3 | |
14 | +*.perspectivev3 | |
15 | +!default.perspectivev3 | |
16 | +xcuserdata | |
17 | +*.xccheckout | |
18 | +*.moved-aside | |
19 | +DerivedData | |
20 | +*.hmap | |
21 | +*.ipa | |
22 | +*.xcuserstate | |
23 | +**/.xcode.env.local | |
24 | + | |
25 | +# Android/IntelliJ | |
26 | +# | |
27 | +build/ | |
28 | +.idea | |
29 | +.gradle | |
30 | +local.properties | |
31 | +*.iml | |
32 | +*.hprof | |
33 | +.cxx/ | |
34 | +*.keystore | |
35 | +!debug.keystore | |
36 | + | |
37 | +# node.js | |
38 | +# | |
39 | +node_modules/ | |
40 | +npm-debug.log | |
41 | +yarn-error.log | |
42 | + | |
43 | +# fastlane | |
44 | +# | |
45 | +# It is recommended to not store the screenshots in the git repo. Instead, use fastlane to re-generate the | |
46 | +# screenshots whenever they are needed. | |
47 | +# For more information about the recommended setup visit: | |
48 | +# https://docs.fastlane.tools/best-practices/source-control/ | |
49 | + | |
50 | +**/fastlane/report.xml | |
51 | +**/fastlane/Preview.html | |
52 | +**/fastlane/screenshots | |
53 | +**/fastlane/test_output | |
54 | + | |
55 | +# Bundle artifact | |
56 | +*.jsbundle | |
57 | + | |
58 | +# Ruby / CocoaPods | |
59 | +**/Pods/ | |
60 | +/vendor/bundle/ | |
61 | + | |
62 | +# Temporary files created by Metro to check the health of the file watcher | |
63 | +.metro-health-check* | |
64 | + | |
65 | +# testing | |
66 | +/coverage | |
67 | + | |
68 | +# Yarn | |
69 | +.yarn/* | |
70 | +!.yarn/patches | |
71 | +!.yarn/plugins | |
72 | +!.yarn/releases | |
73 | +!.yarn/sdks | |
74 | +!.yarn/versions |
+++ .prettierrc.js
... | ... | @@ -0,0 +1,8 @@ |
1 | +module.exports = { | |
2 | + arrowParens: 'avoid', | |
3 | + bracketSameLine: true, | |
4 | + bracketSpacing: false, | |
5 | + singleQuote: true, | |
6 | + trailingComma: 'all', | |
7 | + endOfLine: 'auto', | |
8 | +}; |
+++ .watchmanconfig
... | ... | @@ -0,0 +1,1 @@ |
1 | +{} |
+++ App.tsx
... | ... | @@ -0,0 +1,84 @@ |
1 | +import React from 'react'; | |
2 | +import {NavigationContainer} from '@react-navigation/native'; | |
3 | +import { | |
4 | + createNativeStackNavigator, | |
5 | + NativeStackNavigationProp, | |
6 | +} from '@react-navigation/native-stack'; | |
7 | +import {useNavigation} from '@react-navigation/native'; | |
8 | + | |
9 | +import SendLocation from './src/screens/SendLocation.tsx'; | |
10 | +import CameraScreen from './src/screens/CameraScreen.tsx'; | |
11 | +import LoginScreen from './src/screens/LoginScreen.tsx'; | |
12 | +import AgreementScreen from './src/screens/AgreementScreen.tsx'; | |
13 | + | |
14 | +import AnalysisScreen from './src/screens/AnalysisScreen.tsx'; | |
15 | + | |
16 | +import {verifyTokens} from './src/utils/loginUtils.ts'; | |
17 | +import {RootStackParam} from './src/utils/RootStackParam.ts'; | |
18 | + | |
19 | +const Stack = createNativeStackNavigator<RootStackParam>(); | |
20 | + | |
21 | +const RootNavigator = () => { | |
22 | + const navigation = useNavigation<NativeStackNavigationProp<RootStackParam>>(); | |
23 | + | |
24 | + React.useEffect(() => { | |
25 | + const checkTokens = async () => { | |
26 | + await verifyTokens(navigation); | |
27 | + }; | |
28 | + checkTokens(); | |
29 | + }, [navigation]); | |
30 | + | |
31 | + return ( | |
32 | + <Stack.Navigator | |
33 | + initialRouteName="LoginScreen" | |
34 | + screenOptions={{ | |
35 | + headerShown: false, | |
36 | + }}> | |
37 | + <Stack.Screen name="SendLocation" component={SendLocation} /> | |
38 | + <Stack.Screen | |
39 | + name="CameraScreen" | |
40 | + component={CameraScreen} | |
41 | + options={{ | |
42 | + headerShown: true, | |
43 | + headerTransparent: true, | |
44 | + headerTintColor: '#ffffff', | |
45 | + title: '', | |
46 | + headerStyle: { | |
47 | + backgroundColor: 'rgba(255, 255, 255, 0.3)', | |
48 | + }, | |
49 | + }} | |
50 | + /> | |
51 | + <Stack.Screen name="LoginScreen" component={LoginScreen} /> | |
52 | + <Stack.Screen | |
53 | + name="AgreementScreen" | |
54 | + component={AgreementScreen} | |
55 | + options={{ | |
56 | + headerShown: true, | |
57 | + headerTransparent: true, | |
58 | + headerTintColor: '#cccccc', | |
59 | + title: '', | |
60 | + headerStyle: { | |
61 | + backgroundColor: 'rgba(255, 255, 255, 0.3)', | |
62 | + }, | |
63 | + }} | |
64 | + /> | |
65 | + <Stack.Screen | |
66 | + name="AnalysisScreen" | |
67 | + component={AnalysisScreen} | |
68 | + options={{ | |
69 | + headerShown: true, | |
70 | + headerTintColor: '#424242', | |
71 | + title: '운행 히스토리', | |
72 | + }} | |
73 | + /> | |
74 | + </Stack.Navigator> | |
75 | + ); | |
76 | +}; | |
77 | + | |
78 | +export default function App() { | |
79 | + return ( | |
80 | + <NavigationContainer> | |
81 | + <RootNavigator /> | |
82 | + </NavigationContainer> | |
83 | + ); | |
84 | +} |
+++ Gemfile
... | ... | @@ -0,0 +1,9 @@ |
1 | +source 'https://rubygems.org' | |
2 | + | |
3 | +# You may use http://rbenv.org/ or https://rvm.io/ to install and use this version | |
4 | +ruby ">= 2.6.10" | |
5 | + | |
6 | +# Cocoapods 1.15 introduced a bug which break the build. We will remove the upper | |
7 | +# bound in the template on Cocoapods with next React Native release. | |
8 | +gem 'cocoapods', '>= 1.13', '< 1.15' | |
9 | +gem 'activesupport', '>= 6.1.7.5', '< 7.1.0' |
+++ README.md
... | ... | @@ -0,0 +1,79 @@ |
1 | +This is a new [**React Native**](https://reactnative.dev) project, bootstrapped using [`@react-native-community/cli`](https://github.com/react-native-community/cli). | |
2 | + | |
3 | +# Getting Started | |
4 | + | |
5 | +>**Note**: Make sure you have completed the [React Native - Environment Setup](https://reactnative.dev/docs/environment-setup) instructions till "Creating a new application" step, before proceeding. | |
6 | + | |
7 | +## Step 1: Start the Metro Server | |
8 | + | |
9 | +First, you will need to start **Metro**, the JavaScript _bundler_ that ships _with_ React Native. | |
10 | + | |
11 | +To start Metro, run the following command from the _root_ of your React Native project: | |
12 | + | |
13 | +```bash | |
14 | +# using npm | |
15 | +npm start | |
16 | + | |
17 | +# OR using Yarn | |
18 | +yarn start | |
19 | +``` | |
20 | + | |
21 | +## Step 2: Start your Application | |
22 | + | |
23 | +Let Metro Bundler run in its _own_ terminal. Open a _new_ terminal from the _root_ of your React Native project. Run the following command to start your _Android_ or _iOS_ app: | |
24 | + | |
25 | +### For Android | |
26 | + | |
27 | +```bash | |
28 | +# using npm | |
29 | +npm run android | |
30 | + | |
31 | +# OR using Yarn | |
32 | +yarn android | |
33 | +``` | |
34 | + | |
35 | +### For iOS | |
36 | + | |
37 | +```bash | |
38 | +# using npm | |
39 | +npm run ios | |
40 | + | |
41 | +# OR using Yarn | |
42 | +yarn ios | |
43 | +``` | |
44 | + | |
45 | +If everything is set up _correctly_, you should see your new app running in your _Android Emulator_ or _iOS Simulator_ shortly provided you have set up your emulator/simulator correctly. | |
46 | + | |
47 | +This is one way to run your app — you can also run it directly from within Android Studio and Xcode respectively. | |
48 | + | |
49 | +## Step 3: Modifying your App | |
50 | + | |
51 | +Now that you have successfully run the app, let's modify it. | |
52 | + | |
53 | +1. Open `App.tsx` in your text editor of choice and edit some lines. | |
54 | +2. For **Android**: Press the <kbd>R</kbd> key twice or select **"Reload"** from the **Developer Menu** (<kbd>Ctrl</kbd> + <kbd>M</kbd> (on Window and Linux) or <kbd>Cmd ⌘</kbd> + <kbd>M</kbd> (on macOS)) to see your changes! | |
55 | + | |
56 | + For **iOS**: Hit <kbd>Cmd ⌘</kbd> + <kbd>R</kbd> in your iOS Simulator to reload the app and see your changes! | |
57 | + | |
58 | +## Congratulations! :tada: | |
59 | + | |
60 | +You've successfully run and modified your React Native App. :partying_face: | |
61 | + | |
62 | +### Now what? | |
63 | + | |
64 | +- If you want to add this new React Native code to an existing application, check out the [Integration guide](https://reactnative.dev/docs/integration-with-existing-apps). | |
65 | +- If you're curious to learn more about React Native, check out the [Introduction to React Native](https://reactnative.dev/docs/getting-started). | |
66 | + | |
67 | +# Troubleshooting | |
68 | + | |
69 | +If you can't get this to work, see the [Troubleshooting](https://reactnative.dev/docs/troubleshooting) page. | |
70 | + | |
71 | +# Learn More | |
72 | + | |
73 | +To learn more about React Native, take a look at the following resources: | |
74 | + | |
75 | +- [React Native Website](https://reactnative.dev) - learn more about React Native. | |
76 | +- [Getting Started](https://reactnative.dev/docs/environment-setup) - an **overview** of React Native and how setup your environment. | |
77 | +- [Learn the Basics](https://reactnative.dev/docs/getting-started) - a **guided tour** of the React Native **basics**. | |
78 | +- [Blog](https://reactnative.dev/blog) - read the latest official React Native **Blog** posts. | |
79 | +- [`@facebook/react-native`](https://github.com/facebook/react-native) - the Open Source; GitHub **repository** for React Native. |
+++ __tests__/App.test.tsx
... | ... | @@ -0,0 +1,17 @@ |
1 | +/** | |
2 | + * @format | |
3 | + */ | |
4 | + | |
5 | +import 'react-native'; | |
6 | +import React from 'react'; | |
7 | +import App from '../App'; | |
8 | + | |
9 | +// Note: import explicitly to use the types shipped with jest. | |
10 | +import {it} from '@jest/globals'; | |
11 | + | |
12 | +// Note: test renderer must be required after react-native. | |
13 | +import renderer from 'react-test-renderer'; | |
14 | + | |
15 | +it('renders correctly', () => { | |
16 | + renderer.create(<App />); | |
17 | +}); |
+++ android/app/build.gradle
... | ... | @@ -0,0 +1,119 @@ |
1 | +apply plugin: "com.android.application" | |
2 | +apply plugin: "org.jetbrains.kotlin.android" | |
3 | +apply plugin: "com.facebook.react" | |
4 | + | |
5 | +/** | |
6 | + * This is the configuration block to customize your React Native Android app. | |
7 | + * By default you don't need to apply any configuration, just uncomment the lines you need. | |
8 | + */ | |
9 | +react { | |
10 | + /* Folders */ | |
11 | + // The root of your project, i.e. where "package.json" lives. Default is '..' | |
12 | + // root = file("../") | |
13 | + // The folder where the react-native NPM package is. Default is ../node_modules/react-native | |
14 | + // reactNativeDir = file("../node_modules/react-native") | |
15 | + // The folder where the react-native Codegen package is. Default is ../node_modules/@react-native/codegen | |
16 | + // codegenDir = file("../node_modules/@react-native/codegen") | |
17 | + // The cli.js file which is the React Native CLI entrypoint. Default is ../node_modules/react-native/cli.js | |
18 | + // cliFile = file("../node_modules/react-native/cli.js") | |
19 | + | |
20 | + /* Variants */ | |
21 | + // The list of variants to that are debuggable. For those we're going to | |
22 | + // skip the bundling of the JS bundle and the assets. By default is just 'debug'. | |
23 | + // If you add flavors like lite, prod, etc. you'll have to list your debuggableVariants. | |
24 | + // debuggableVariants = ["liteDebug", "prodDebug"] | |
25 | + | |
26 | + /* Bundling */ | |
27 | + // A list containing the node command and its flags. Default is just 'node'. | |
28 | + // nodeExecutableAndArgs = ["node"] | |
29 | + // | |
30 | + // The command to run when bundling. By default is 'bundle' | |
31 | + // bundleCommand = "ram-bundle" | |
32 | + // | |
33 | + // The path to the CLI configuration file. Default is empty. | |
34 | + // bundleConfig = file(../rn-cli.config.js) | |
35 | + // | |
36 | + // The name of the generated asset file containing your JS bundle | |
37 | + // bundleAssetName = "MyApplication.android.bundle" | |
38 | + // | |
39 | + // The entry file for bundle generation. Default is 'index.android.js' or 'index.js' | |
40 | + // entryFile = file("../js/MyApplication.android.js") | |
41 | + // | |
42 | + // A list of extra flags to pass to the 'bundle' commands. | |
43 | + // See https://github.com/react-native-community/cli/blob/main/docs/commands.md#bundle | |
44 | + // extraPackagerArgs = [] | |
45 | + | |
46 | + /* Hermes Commands */ | |
47 | + // The hermes compiler command to run. By default it is 'hermesc' | |
48 | + // hermesCommand = "$rootDir/my-custom-hermesc/bin/hermesc" | |
49 | + // | |
50 | + // The list of flags to pass to the Hermes compiler. By default is "-O", "-output-source-map" | |
51 | + // hermesFlags = ["-O", "-output-source-map"] | |
52 | +} | |
53 | + | |
54 | +/** | |
55 | + * Set this to true to Run Proguard on Release builds to minify the Java bytecode. | |
56 | + */ | |
57 | +def enableProguardInReleaseBuilds = false | |
58 | + | |
59 | +/** | |
60 | + * The preferred build flavor of JavaScriptCore (JSC) | |
61 | + * | |
62 | + * For example, to use the international variant, you can use: | |
63 | + * `def jscFlavor = 'org.webkit:android-jsc-intl:+'` | |
64 | + * | |
65 | + * The international variant includes ICU i18n library and necessary data | |
66 | + * allowing to use e.g. `Date.toLocaleString` and `String.localeCompare` that | |
67 | + * give correct results when using with locales other than en-US. Note that | |
68 | + * this variant is about 6MiB larger per architecture than default. | |
69 | + */ | |
70 | +def jscFlavor = 'org.webkit:android-jsc:+' | |
71 | + | |
72 | +android { | |
73 | + ndkVersion rootProject.ext.ndkVersion | |
74 | + buildToolsVersion rootProject.ext.buildToolsVersion | |
75 | + compileSdk rootProject.ext.compileSdkVersion | |
76 | + | |
77 | + namespace "com.send_location_ta" | |
78 | + defaultConfig { | |
79 | + applicationId "com.send_location_ta" | |
80 | + minSdkVersion rootProject.ext.minSdkVersion | |
81 | + targetSdkVersion rootProject.ext.targetSdkVersion | |
82 | + versionCode 1 | |
83 | + versionName "1.0" | |
84 | + } | |
85 | + signingConfigs { | |
86 | + debug { | |
87 | + storeFile file('debug.keystore') | |
88 | + storePassword 'android' | |
89 | + keyAlias 'androiddebugkey' | |
90 | + keyPassword 'android' | |
91 | + } | |
92 | + } | |
93 | + buildTypes { | |
94 | + debug { | |
95 | + signingConfig signingConfigs.debug | |
96 | + } | |
97 | + release { | |
98 | + // Caution! In production, you need to generate your own keystore file. | |
99 | + // see https://reactnative.dev/docs/signed-apk-android. | |
100 | + signingConfig signingConfigs.debug | |
101 | + minifyEnabled enableProguardInReleaseBuilds | |
102 | + proguardFiles getDefaultProguardFile("proguard-android.txt"), "proguard-rules.pro" | |
103 | + } | |
104 | + } | |
105 | +} | |
106 | + | |
107 | +dependencies { | |
108 | + // The version of react-native is set by the React Native Gradle Plugin | |
109 | + implementation("com.facebook.react:react-android") | |
110 | + | |
111 | + if (hermesEnabled.toBoolean()) { | |
112 | + implementation("com.facebook.react:hermes-android") | |
113 | + } else { | |
114 | + implementation jscFlavor | |
115 | + } | |
116 | +} | |
117 | + | |
118 | +apply from: file("../../node_modules/@react-native-community/cli-platform-android/native_modules.gradle"); applyNativeModulesAppBuildGradle(project) | |
119 | +apply from: "../../node_modules/react-native-vector-icons/fonts.gradle" |
+++ android/app/debug.keystore
Binary file is not shown |
+++ android/app/proguard-rules.pro
... | ... | @@ -0,0 +1,10 @@ |
1 | +# Add project specific ProGuard rules here. | |
2 | +# By default, the flags in this file are appended to flags specified | |
3 | +# in /usr/local/Cellar/android-sdk/24.3.3/tools/proguard/proguard-android.txt | |
4 | +# You can edit the include path and order by changing the proguardFiles | |
5 | +# directive in build.gradle. | |
6 | +# | |
7 | +# For more details, see | |
8 | +# http://developer.android.com/guide/developing/tools/proguard.html | |
9 | + | |
10 | +# Add any project specific keep options here: |
+++ android/app/src/debug/AndroidManifest.xml
... | ... | @@ -0,0 +1,9 @@ |
1 | +<?xml version="1.0" encoding="utf-8"?> | |
2 | +<manifest xmlns:android="http://schemas.android.com/apk/res/android" | |
3 | + xmlns:tools="http://schemas.android.com/tools"> | |
4 | + | |
5 | + <application | |
6 | + android:usesCleartextTraffic="true" | |
7 | + tools:targetApi="28" | |
8 | + tools:ignore="GoogleAppIndexingWarning"/> | |
9 | +</manifest> |
+++ android/app/src/main/AndroidManifest.xml
... | ... | @@ -0,0 +1,34 @@ |
1 | +<manifest xmlns:android="http://schemas.android.com/apk/res/android"> | |
2 | + | |
3 | + <uses-permission android:name="android.permission.INTERNET" /> | |
4 | + <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> | |
5 | + <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> | |
6 | + <uses-permission android:name="android.permission.CAMERA" /> | |
7 | + <uses-permission android:name="android.permission.FOREGROUND_SERVICE" /> | |
8 | + <uses-permission android:name="android.permission.WAKE_LOCK" /> | |
9 | + <uses-permission android:name="android.permission.POST_NOTIFICATIONS" /> | |
10 | + | |
11 | + <application | |
12 | + android:name=".MainApplication" | |
13 | + android:label="@string/app_name" | |
14 | + android:icon="@mipmap/ic_launcher" | |
15 | + android:roundIcon="@mipmap/ic_launcher_round" | |
16 | + android:allowBackup="false" | |
17 | + android:theme="@style/AppTheme" | |
18 | + android:exported="true" | |
19 | + android:usesCleartextTraffic="true"> | |
20 | + <activity | |
21 | + android:name=".MainActivity" | |
22 | + android:label="@string/app_name" | |
23 | + android:configChanges="keyboard|keyboardHidden|orientation|screenLayout|screenSize|smallestScreenSize|uiMode" | |
24 | + android:launchMode="singleTask" | |
25 | + android:windowSoftInputMode="adjustResize" | |
26 | + android:exported="true"> | |
27 | + <intent-filter> | |
28 | + <action android:name="android.intent.action.MAIN" /> | |
29 | + <category android:name="android.intent.category.LAUNCHER" /> | |
30 | + </intent-filter> | |
31 | + </activity> | |
32 | + <service android:name="com.asterinet.react.bgactions.RNBackgroundActionsTask" android:foregroundServiceType="shortService"/> | |
33 | + </application> | |
34 | +</manifest> |
+++ android/app/src/main/assets/index.android.bundle
This file is too big to display. |
+++ android/app/src/main/java/com/send_location_ta/MainActivity.kt
This diff is skipped because there are too many other diffs. |
+++ android/app/src/main/java/com/send_location_ta/MainApplication.kt
This diff is skipped because there are too many other diffs. |
+++ android/app/src/main/res/drawable-mdpi/src_asset_bicycle.png
Binary file is not shown |
+++ android/app/src/main/res/drawable/rn_edit_text_material.xml
This diff is skipped because there are too many other diffs. |
+++ android/app/src/main/res/mipmap-hdpi/ic_launcher.png
Binary file is not shown |
+++ android/app/src/main/res/mipmap-hdpi/ic_launcher_round.png
Binary file is not shown |
+++ android/app/src/main/res/mipmap-mdpi/ic_launcher.png
Binary file is not shown |
+++ android/app/src/main/res/mipmap-mdpi/ic_launcher_round.png
Binary file is not shown |
+++ android/app/src/main/res/mipmap-xhdpi/ic_launcher.png
Binary file is not shown |
+++ android/app/src/main/res/mipmap-xhdpi/ic_launcher_round.png
Binary file is not shown |
+++ android/app/src/main/res/mipmap-xxhdpi/ic_launcher.png
Binary file is not shown |
+++ android/app/src/main/res/mipmap-xxhdpi/ic_launcher_round.png
Binary file is not shown |
+++ android/app/src/main/res/mipmap-xxxhdpi/ic_launcher.png
Binary file is not shown |
+++ android/app/src/main/res/mipmap-xxxhdpi/ic_launcher_round.png
Binary file is not shown |
+++ android/app/src/main/res/values/strings.xml
This diff is skipped because there are too many other diffs. |
+++ android/app/src/main/res/values/styles.xml
This diff is skipped because there are too many other diffs. |
+++ android/build.gradle
This diff is skipped because there are too many other diffs. |
+++ android/gradle.properties
This diff is skipped because there are too many other diffs. |
+++ android/gradle/wrapper/gradle-wrapper.jar
Binary file is not shown |
+++ android/gradle/wrapper/gradle-wrapper.properties
This diff is skipped because there are too many other diffs. |
+++ android/gradlew
This diff is skipped because there are too many other diffs. |
+++ android/gradlew.bat
This diff is skipped because there are too many other diffs. |
+++ android/settings.gradle
This diff is skipped because there are too many other diffs. |
+++ app.json
This diff is skipped because there are too many other diffs. |
+++ babel.config.js
This diff is skipped because there are too many other diffs. |
+++ index.js
This diff is skipped because there are too many other diffs. |
+++ ios/.xcode.env
This diff is skipped because there are too many other diffs. |
+++ ios/Podfile
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TA.xcodeproj/project.pbxproj
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TA/AppDelegate.h
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TA/AppDelegate.mm
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TA/Images.xcassets/AppIcon.appiconset/Contents.json
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TA/Images.xcassets/Contents.json
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TA/Info.plist
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TA/LaunchScreen.storyboard
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TA/PrivacyInfo.xcprivacy
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TA/main.m
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TATests/Info.plist
This diff is skipped because there are too many other diffs. |
+++ ios/Send_Location_TATests/Send_Location_TATests.m
This diff is skipped because there are too many other diffs. |
+++ jest.config.js
This diff is skipped because there are too many other diffs. |
+++ metro.config.js
This diff is skipped because there are too many other diffs. |
+++ package-lock.json
This diff is skipped because there are too many other diffs. |
+++ package.json
This diff is skipped because there are too many other diffs. |
+++ src/asset/bicycle.png
Binary file is not shown |
+++ src/component/RadioButton.tsx
This diff is skipped because there are too many other diffs. |
+++ src/screens/AgreementScreen.tsx
This diff is skipped because there are too many other diffs. |
+++ src/screens/AnalysisComponent.tsx
This diff is skipped because there are too many other diffs. |
+++ src/screens/AnalysisScreen.tsx
This diff is skipped because there are too many other diffs. |
+++ src/screens/CameraScreen.tsx
This diff is skipped because there are too many other diffs. |
+++ src/screens/LoginScreen.tsx
This diff is skipped because there are too many other diffs. |
+++ src/screens/SendLocation.tsx
This diff is skipped because there are too many other diffs. |
+++ src/styles/GlobalStyles.tsx
This diff is skipped because there are too many other diffs. |
+++ src/utils/RootStackParam.ts
This diff is skipped because there are too many other diffs. |
+++ src/utils/loginUtils.ts
This diff is skipped because there are too many other diffs. |
+++ src/utils/useCameraAPI.ts
This diff is skipped because there are too many other diffs. |
+++ src/utils/useGeolocationAPI.ts
This diff is skipped because there are too many other diffs. |
+++ tsconfig.json
This diff is skipped because there are too many other diffs. |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?