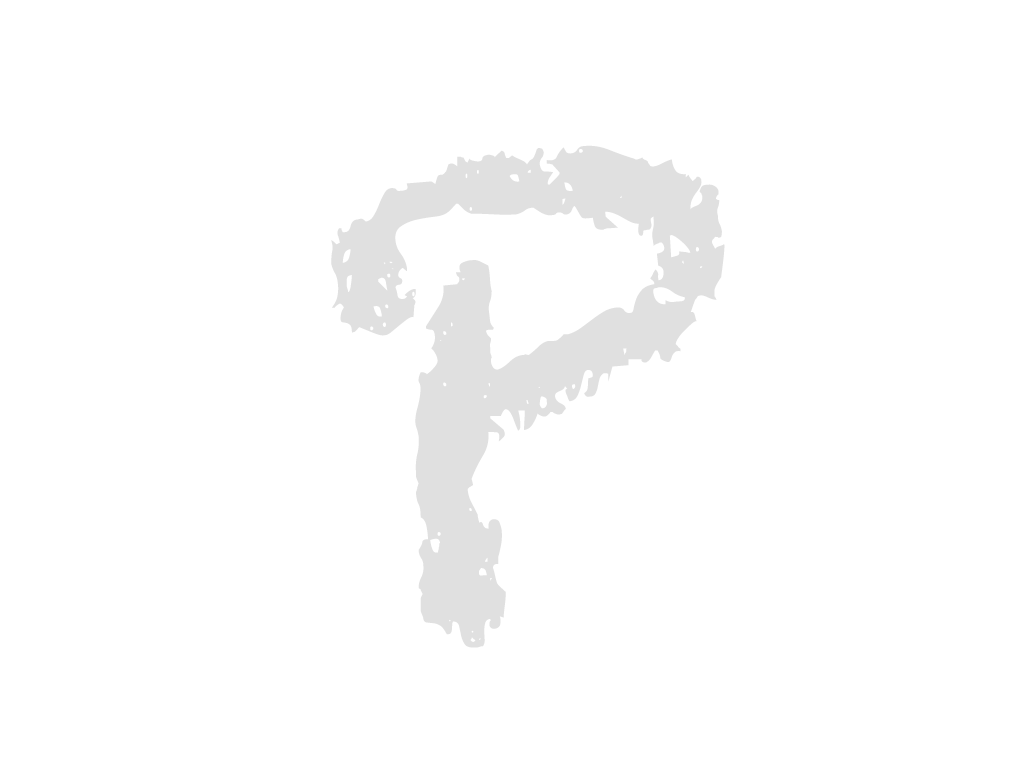
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import * as React from 'react';
import {View, Text, TouchableOpacity, StyleSheet} from 'react-native';
export interface RadioButtonProps {
children?: React.ReactNode;
isSelected?: boolean | undefined;
labelHorizontal?: boolean | undefined;
buttonColor?: string | undefined;
selectedButtonColor?: string | undefined;
labelColor?: string | undefined;
style?: any;
wrapStyle?: any;
onPress?: () => void;
}
export class RadioButton extends React.Component<RadioButtonProps> {
render() {
const {
isSelected,
buttonColor,
selectedButtonColor,
labelColor,
style,
wrapStyle,
children,
onPress,
} = this.props;
const color = isSelected
? selectedButtonColor || '#3872ef'
: buttonColor || '#ccc';
return (
<TouchableOpacity onPress={onPress} style={[styles.container, wrapStyle]}>
<View style={[styles.circle, {borderColor: color}]}>
{isSelected && (
<View style={[styles.checkedCircle, {backgroundColor: color}]} />
)}
</View>
{children && (
<Text style={[styles.label, {color: labelColor || '#000'}, style]}>
{children}
</Text>
)}
</TouchableOpacity>
);
}
}
const styles = StyleSheet.create({
container: {
flexDirection: 'row',
alignItems: 'center',
marginBottom: 10,
},
circle: {
height: 20,
width: 20,
borderRadius: 12,
borderWidth: 2,
borderColor: '#ccc',
alignItems: 'center',
justifyContent: 'center',
marginRight: 10,
},
checkedCircle: {
width: 10,
height: 10,
borderRadius: 6,
backgroundColor: '#2196f3',
},
label: {
fontSize: 16,
},
});
export default RadioButton;