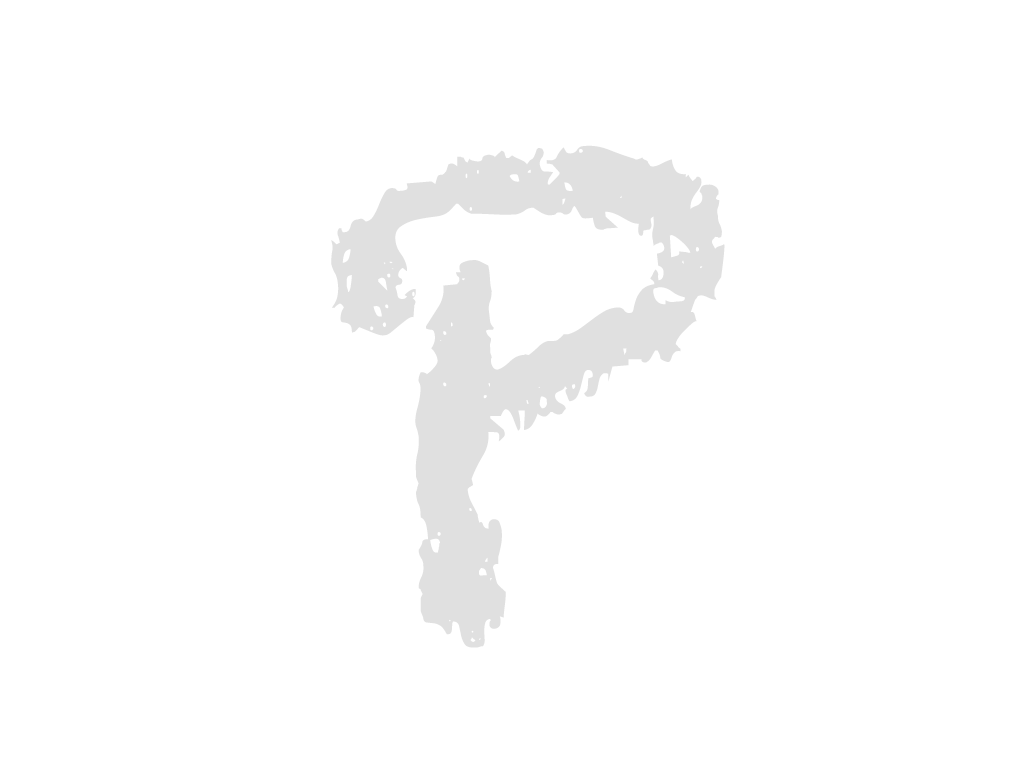
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React, {useEffect, useRef, useState} from 'react';
import {
StyleSheet,
View,
Text,
TouchableOpacity,
Dimensions,
} from 'react-native';
import {Shadow} from 'react-native-shadow-2';
import Icon from 'react-native-vector-icons/FontAwesome5';
import {getCurrentPosition} from '../utils/useGeolocationAPI';
import {useNavigation} from '@react-navigation/native';
import {NativeStackNavigationProp} from '@react-navigation/native-stack';
import {RootStackParam} from '../utils/RootStackParam';
import AsyncStorage from '@react-native-async-storage/async-storage';
import BackgroundService from 'react-native-background-actions';
import crypto from 'crypto-js';
export default function SendLocation() {
const navigation = useNavigation<NativeStackNavigationProp<RootStackParam>>();
const [time, setTime] = useState<number>(0);
const [time2, setTime2] = useState<number>(0);
const [isRunning, setIsRunning] = useState<boolean>(false);
const [hash, setHash] = useState("");
const tripIdRef = useRef<string>(hash);
// 해시
useEffect(() => {
tripIdRef.current = hash;
}, [hash]);
useEffect(() => {
var rand = Math.random().toString();
var date = new Date();
var sha256Hash = crypto.SHA256(rand + ',' + date.toString()).toString(crypto.enc.Hex);
console.log(rand, date, sha256Hash);
setHash(sha256Hash);
if (isRunning) {
startBackgroundService();
} else {
stopBackgroundService();
}
}, [isRunning]);
const sleep = (time2: number) => new Promise(resolve => setTimeout(() => resolve(true), time2));
const veryIntensiveTask = async (taskDataArguments: any) => {
const { delay } = taskDataArguments;
while (BackgroundService.isRunning()) {
setTime(prevTime => prevTime + 1);
getCurrentPosition(tripIdRef, navigation);
await sleep(delay);
}
};
const options = {
taskName: '측정 서비스',
taskTitle: '측정 중',
taskDesc: '측정 진행 중... ',
taskIcon: {
name: 'ic_launcher',
type: 'mipmap',
},
color: '#ff00ff',
linkingURI: 'sendLocation',
parameters: {
delay: 1000,
},
};
const startBackgroundService = async () => {
await BackgroundService.start(veryIntensiveTask, options);
};
const stopBackgroundService = async () => {
await BackgroundService.stop();
};
// 측정 시작
const handleStart = () => {
setIsRunning(true);
};
// 측정 종료
const handleStop = () => {
setTime(0);
setIsRunning(false);
};
// 시간 포맷
const formatTime = (time: number): string => {
console.log(time)
const hours = Math.floor(time / 3600);
const minutes = Math.floor((time % 3600) / 60);
const seconds = time % 60;
return `${hours.toString().padStart(2, '0')}:${minutes.toString().padStart(2, '0')}:${seconds
.toString().padStart(2, '0')}`;
};
return (
<View style={styles.container}>
<View style={{alignItems: 'center'}}>
<Shadow
distance={8}
startColor={'#3872ef33'}
endColor={'#ffffff'}
offset={[5, 6]}
containerStyle={styles.watchContainer}>
<View style={styles.watchContainer}>
<Text style={{fontWeight: 'bold', fontSize: 20}}>
측정 경과 시간
</Text>
<Text style={styles.time}> {formatTime(time)} </Text>
</View>
</Shadow>
<View style={styles.buttonContainer}>
<View style={styles.row}>
<TouchableOpacity style={styles.button} onPress={handleStart}>
<Icon name="play" size={25} color={'#717171'} />
</TouchableOpacity>
<Text> 측정 시작 </Text>
</View>
<View style={styles.row}>
<TouchableOpacity style={styles.button} onPress={handleStop}>
<Icon name="power-off" size={25} color={'#717171'} />
</TouchableOpacity>
<Text> 측정 종료 </Text>
</View>
</View>
<TouchableOpacity
onPress={() => navigation.navigate('CameraScreen')}
style={styles.navButton}>
<Text> 카메라 촬영 </Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.navButton}
onPress={() => navigation.navigate('AnalysisScreen')}>
<Text> 분석 결과 </Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.logout}
onPress={() => {
AsyncStorage.removeItem('token');
navigation.navigate('LoginScreen');
}}>
<Text> 로그아웃 </Text>
</TouchableOpacity>
</View>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingHorizontal: 16,
paddingVertical: 50,
justifyContent: 'center',
backgroundColor: '#ffffff',
},
watchContainer: {
alignItems: 'center',
justifyContent: 'center',
borderRadius:
Math.round(
Dimensions.get('window').width + Dimensions.get('window').height,
) * 0.35,
width: Dimensions.get('window').width * 0.7,
height: Dimensions.get('window').width * 0.7,
backgroundColor: '#ffffff',
},
time: {
marginTop: 30,
color: '#3872ef',
fontWeight: 'bold',
fontSize: 50,
},
buttonContainer: {
flexDirection: 'row',
justifyContent: 'space-between',
paddingHorizontal: 10,
marginTop: 65,
marginBottom: 70,
width: '55%',
},
row: {
alignItems: 'center',
},
button: {
backgroundColor: '#F0F0F0',
justifyContent: 'center',
alignItems: 'center',
borderRadius: 100,
width: 60,
height: 60,
marginBottom: 10,
},
navButton: {
backgroundColor: '#f0f0f0',
alignItems: 'center',
justifyContent: 'center',
height: Dimensions.get('screen').height * 0.05,
width: Dimensions.get('screen').width * 0.7,
marginTop: 15,
borderRadius: 10,
},
logout: {
borderWidth: 3,
borderColor: '#f0f0f0',
alignItems: 'center',
justifyContent: 'center',
height: Dimensions.get('screen').height * 0.05,
width: Dimensions.get('screen').width * 0.7,
marginTop: 15,
borderRadius: 10,
},
});