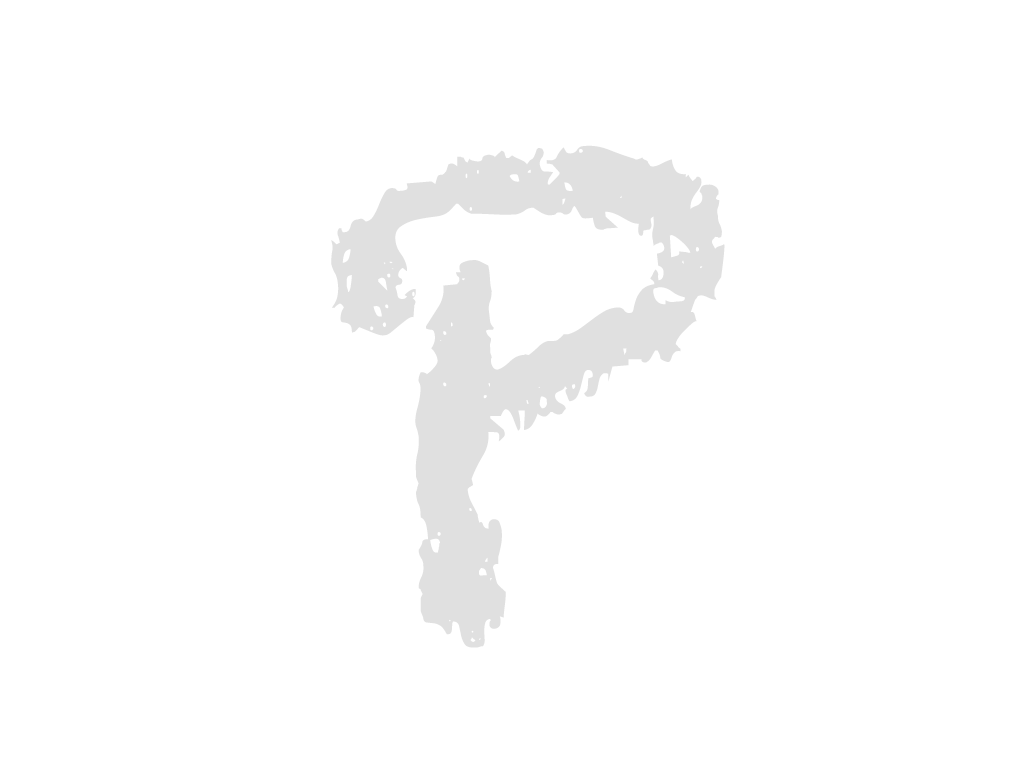
+++ .vscode/settings.json
... | ... | @@ -0,0 +1,3 @@ |
1 | +{ | |
2 | + "editor.fontFamily": "Consolas, 'Courier New', monospace" | |
3 | +}(No newline at end of file) |
--- client/views/component/Calendar_admin.jsx
+++ client/views/component/Calendar_admin.jsx
... | ... | @@ -1,21 +1,13 @@ |
1 | 1 |
import React, { useState } from "react"; |
2 |
-import { useNavigate, useLocation } from "react-router"; |
|
3 |
-import { useSelector } from "react-redux"; |
|
4 | 2 |
|
5 | 3 |
import Calendar from "react-calendar"; |
6 | 4 |
import "react-calendar/dist/Calendar.css"; |
7 |
-import Modal from "./Modal.jsx"; |
|
8 | 5 |
import moment from "moment"; |
9 | 6 |
|
10 | 7 |
import CommonUtil from "../../resources/js/CommonUtil.js"; |
11 | 8 |
|
12 | 9 |
export default function CalendarComponent({ data }) { |
13 |
- const navigate = useNavigate(); |
|
14 |
- const location = useLocation(); |
|
15 |
- |
|
16 | 10 |
//전역 변수 저장 객체 |
17 |
- const state = useSelector((state) => { return state }); |
|
18 |
- |
|
19 | 11 |
const [value, setValue] = useState(new Date()); |
20 | 12 |
const activeDate = moment(value).format('YYYY-MM-DD'); |
21 | 13 |
|
... | ... | @@ -68,15 +60,11 @@ |
68 | 60 |
temperatureData = '-' |
69 | 61 |
} else { |
70 | 62 |
if (todayTemperature.length > 0) { |
71 |
- var temperatures = ""; |
|
72 |
- |
|
73 | 63 |
if (moment(date['date']).format("MM") >= 11 || moment(date['date']).format("MM") <= 2) { |
74 |
- temperatures = Math.min.apply(null, todayTemperature); |
|
64 |
+ temperatureData = Math.min.apply(null, todayTemperature) + '℃'; |
|
75 | 65 |
} else { |
76 |
- temperatures = Math.max.apply(null, todayTemperature); |
|
66 |
+ temperatureData = Math.max.apply(null, todayTemperature) + '℃'; |
|
77 | 67 |
} |
78 |
- |
|
79 |
- temperatureData = temperatures + '℃' |
|
80 | 68 |
} |
81 | 69 |
} |
82 | 70 |
|
--- client/views/pages/main/Main_agency.jsx
+++ client/views/pages/main/Main_agency.jsx
... | ... | @@ -30,26 +30,38 @@ |
30 | 30 |
const location = useLocation(); |
31 | 31 |
|
32 | 32 |
//전역 변수 저장 객체 |
33 |
- const state = useSelector((state) => { return state }); |
|
33 |
+ const state = useSelector((state) => { |
|
34 |
+ return state; |
|
35 |
+ }); |
|
34 | 36 |
|
35 | 37 |
const thead = ["No", "계약업체명", "구분", "담당자 연락처", "주소"]; |
36 |
- const key = ["No", "agency", "division", "phone", "address",]; |
|
38 |
+ const key = ["No", "agency", "division", "phone", "address"]; |
|
37 | 39 |
const content = [ |
38 | 40 |
{ |
39 | 41 |
No: ( |
40 |
- <p><span>{thead[0]}</span> 1948.11.15</p> |
|
42 |
+ <p> |
|
43 |
+ <span>{thead[0]}</span> 1948.11.15 |
|
44 |
+ </p> |
|
41 | 45 |
), |
42 | 46 |
agency: ( |
43 |
- <p><span>{thead[1]}</span> A복지관</p> |
|
47 |
+ <p> |
|
48 |
+ <span>{thead[1]}</span> A복지관 |
|
49 |
+ </p> |
|
44 | 50 |
), |
45 | 51 |
division: ( |
46 |
- <p><span>{thead[2]}</span>교환</p> |
|
52 |
+ <p> |
|
53 |
+ <span>{thead[2]}</span>교환 |
|
54 |
+ </p> |
|
47 | 55 |
), |
48 | 56 |
phone: ( |
49 |
- <p><span>{thead[3]}</span>010-1234-5678</p> |
|
57 |
+ <p> |
|
58 |
+ <span>{thead[3]}</span>010-1234-5678 |
|
59 |
+ </p> |
|
50 | 60 |
), |
51 | 61 |
address: ( |
52 |
- <p><span>{thead[4]}</span>경상북도 군위군 삼국유사면</p> |
|
62 |
+ <p> |
|
63 |
+ <span>{thead[4]}</span>경상북도 군위군 삼국유사면 |
|
64 |
+ </p> |
|
53 | 65 |
), |
54 | 66 |
}, |
55 | 67 |
]; |
... | ... | @@ -59,9 +71,9 @@ |
59 | 71 |
seniorList: [], |
60 | 72 |
seniorListCount: 0, |
61 | 73 |
search: { |
62 |
- 'agent_id': state.loginUser['user_id'], |
|
63 |
- 'user_use': true, |
|
64 |
- } |
|
74 |
+ agent_id: state.loginUser["user_id"], |
|
75 |
+ user_use: true, |
|
76 |
+ }, |
|
65 | 77 |
}); |
66 | 78 |
// 내 시니어 목록 조회 |
67 | 79 |
const [seniorGenderData, setSeniorGenderData] = React.useState([]); |
... | ... | @@ -69,113 +81,167 @@ |
69 | 81 |
fetch("/user/seniorSelectList.json", { |
70 | 82 |
method: "POST", |
71 | 83 |
headers: { |
72 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
84 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
73 | 85 |
}, |
74 | 86 |
body: JSON.stringify(mySenior.search), |
75 |
- }).then((response) => response.json()).then((data) => { |
|
76 |
- data.search = mySenior.search; |
|
77 |
- console.log("내 시니어 목록 조회 : ", data); |
|
78 |
- setMySenior(data); |
|
87 |
+ }) |
|
88 |
+ .then((response) => response.json()) |
|
89 |
+ .then((data) => { |
|
90 |
+ data.search = mySenior.search; |
|
91 |
+ console.log("내 시니어 목록 조회 : ", data); |
|
92 |
+ setMySenior(data); |
|
79 | 93 |
|
80 |
- let seniorGenderArr = []; |
|
81 |
- const today = new Date(); |
|
82 |
- data['seniorList'].map((item, idx) => { |
|
83 |
- const birth = new Date(item['user_birth']); |
|
84 |
- let age = today.getFullYear() - birth.getFullYear() + 1; |
|
85 |
- seniorGenderArr.push({ "gender": item['user_gender'], "age": age }) |
|
86 |
- }) |
|
87 |
- console.log("seniorGenderArr: ", seniorGenderArr) |
|
94 |
+ let seniorGenderArr = []; |
|
95 |
+ const today = new Date(); |
|
96 |
+ data["seniorList"].map((item, idx) => { |
|
97 |
+ const birth = new Date(item["user_birth"]); |
|
98 |
+ let age = today.getFullYear() - birth.getFullYear() + 1; |
|
99 |
+ seniorGenderArr.push({ gender: item["user_gender"], age: age }); |
|
100 |
+ }); |
|
101 |
+ console.log("seniorGenderArr: ", seniorGenderArr); |
|
88 | 102 |
|
89 |
- let genderData = []; |
|
90 |
- for (let i = 0; i < 18; i++) { |
|
91 |
- let age = i * 5; |
|
92 |
- let age1 = ((i + 1) * 5) - 1; |
|
93 |
- if (i == 17) { |
|
94 |
- genderData.push({ "age": age + '+', "male": 0, "female": 0 }) |
|
95 |
- } else { |
|
96 |
- genderData.push({ "age": age + '-' + age1, "male": 0, "female": 0 }) |
|
97 |
- } |
|
98 |
- } |
|
99 |
- console.log("genderData: ", genderData) |
|
100 |
- |
|
101 |
- seniorGenderArr.map((item, idx) => { |
|
103 |
+ let genderData = []; |
|
102 | 104 |
for (let i = 0; i < 18; i++) { |
103 |
- if (item['age'] >= i * 5 && item['age'] <= ((i + 1) * 5) - 1) { |
|
104 |
- if (item['gender'] == "남") { |
|
105 |
- genderData[i]['male']-- |
|
106 |
- } else if (item['gender'] == "여") { |
|
107 |
- genderData[i]['female']++ |
|
108 |
- } |
|
105 |
+ let age = i * 5; |
|
106 |
+ let age1 = (i + 1) * 5 - 1; |
|
107 |
+ if (i == 17) { |
|
108 |
+ genderData.push({ age: age + "+", male: 0, female: 0 }); |
|
109 |
+ } else { |
|
110 |
+ genderData.push({ age: age + "-" + age1, male: 0, female: 0 }); |
|
109 | 111 |
} |
110 | 112 |
} |
111 |
- }) |
|
113 |
+ console.log("genderData: ", genderData); |
|
112 | 114 |
|
113 |
- setSeniorGenderData(genderData) |
|
114 |
- console.log("genderData: ", genderData) |
|
115 |
- }).catch((error) => { |
|
116 |
- console.log('seniorSelectList() /user/seniorSelectList.json error : ', error); |
|
117 |
- }); |
|
118 |
- } |
|
115 |
+ seniorGenderArr.map((item, idx) => { |
|
116 |
+ for (let i = 0; i < 18; i++) { |
|
117 |
+ if (item["age"] >= i * 5 && item["age"] <= (i + 1) * 5 - 1) { |
|
118 |
+ if (item["gender"] == "남") { |
|
119 |
+ genderData[i]["male"]--; |
|
120 |
+ } else if (item["gender"] == "여") { |
|
121 |
+ genderData[i]["female"]++; |
|
122 |
+ } |
|
123 |
+ } |
|
124 |
+ } |
|
125 |
+ }); |
|
126 |
+ |
|
127 |
+ setSeniorGenderData(genderData); |
|
128 |
+ console.log("genderData: ", genderData); |
|
129 |
+ }) |
|
130 |
+ .catch((error) => { |
|
131 |
+ console.log( |
|
132 |
+ "seniorSelectList() /user/seniorSelectList.json error : ", |
|
133 |
+ error |
|
134 |
+ ); |
|
135 |
+ }); |
|
136 |
+ }; |
|
119 | 137 |
|
120 | 138 |
//온도 위험 대상자(시니어) 수 조회 |
121 | 139 |
const [temperatureCount, setTemperatureCount] = React.useState(0); |
122 | 140 |
const temperatureRiskCount = () => { |
123 |
- fetch("/stats/agentTemperatureRisk.json", { |
|
141 |
+ fetch("/stats/temperatureRisk.json", { |
|
124 | 142 |
method: "POST", |
125 | 143 |
headers: { |
126 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
144 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
127 | 145 |
}, |
128 | 146 |
body: JSON.stringify({ |
129 |
- 'agent_id': state.loginUser['user_id'], |
|
147 |
+ agent_id: state.loginUser["user_id"], |
|
130 | 148 |
}), |
131 |
- }).then((response) => response.json()).then((data) => { |
|
132 |
- console.log("온도 위험 대상자(시니어) 수 조회 : ", data); |
|
133 |
- setTemperatureCount(data); |
|
134 |
- }).catch((error) => { |
|
135 |
- console.log('temperatureRiskCount() /stats/agentTemperatureRisk.json error : ', error); |
|
136 |
- }); |
|
137 |
- } |
|
149 |
+ }) |
|
150 |
+ .then((response) => response.json()) |
|
151 |
+ .then((data) => { |
|
152 |
+ console.log("온도 위험 대상자(시니어) 수 조회 : ", data); |
|
153 |
+ setTemperatureCount(data); |
|
154 |
+ }) |
|
155 |
+ .catch((error) => { |
|
156 |
+ console.log( |
|
157 |
+ "temperatureRiskCount() /stats/temperatureRisk.json error : ", |
|
158 |
+ error |
|
159 |
+ ); |
|
160 |
+ }); |
|
161 |
+ }; |
|
138 | 162 |
|
139 | 163 |
//배터리 부족 대상자(시니어) 수 조회 |
140 | 164 |
const [batteryCount, setbatteryCount] = React.useState(0); |
141 | 165 |
const batteryRiskCount = () => { |
142 |
- fetch("/stats/agentBatteryRisk.json", { |
|
166 |
+ fetch("/stats/batteryRisk.json", { |
|
143 | 167 |
method: "POST", |
144 | 168 |
headers: { |
145 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
169 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
146 | 170 |
}, |
147 | 171 |
body: JSON.stringify({ |
148 |
- 'agent_id': state.loginUser['user_id'], |
|
172 |
+ agent_id: state.loginUser["user_id"], |
|
149 | 173 |
}), |
150 |
- }).then((response) => response.json()).then((data) => { |
|
151 |
- console.log("배터리 부족 대상자(시니어) 수 조회 : ", data); |
|
152 |
- setbatteryCount(data); |
|
153 |
- }).catch((error) => { |
|
154 |
- console.log('batteryRiskCount() /stats/agentBatteryRisk.json error : ', error); |
|
155 |
- }); |
|
156 |
- } |
|
174 |
+ }) |
|
175 |
+ .then((response) => response.json()) |
|
176 |
+ .then((data) => { |
|
177 |
+ console.log("배터리 부족 대상자(시니어) 수 조회 : ", data); |
|
178 |
+ setbatteryCount(data); |
|
179 |
+ }) |
|
180 |
+ .catch((error) => { |
|
181 |
+ console.log( |
|
182 |
+ "batteryRiskCount() /stats/batteryRisk.json error : ", |
|
183 |
+ error |
|
184 |
+ ); |
|
185 |
+ }); |
|
186 |
+ }; |
|
187 |
+ |
|
188 |
+ //데이터 미확인 대상자(시니어) 수 조회 |
|
189 |
+ const [receiveDataMissCount, setReceiveDataMissCount] = React.useState(0); |
|
190 |
+ const receiveDataMissRiskCount = () => { |
|
191 |
+ fetch("/stats/receiveDataMissCount.json", { |
|
192 |
+ method: "POST", |
|
193 |
+ headers: { |
|
194 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
195 |
+ }, |
|
196 |
+ body: JSON.stringify({ |
|
197 |
+ agent_id: state.loginUser["user_id"], |
|
198 |
+ }), |
|
199 |
+ }) |
|
200 |
+ .then((response) => response.json()) |
|
201 |
+ .then((data) => { |
|
202 |
+ console.log("데이터 미확인 대상자(시니어) 수 조회 : ", data); |
|
203 |
+ setReceiveDataMissCount(data); |
|
204 |
+ }) |
|
205 |
+ .catch((error) => { |
|
206 |
+ console.log( |
|
207 |
+ "receiveDataMissRiskCount() /stats/receiveDataMissCount.json error : ", |
|
208 |
+ error |
|
209 |
+ ); |
|
210 |
+ }); |
|
211 |
+ }; |
|
157 | 212 |
|
158 | 213 |
// 최근 복약률 |
159 |
- const [medicationSelectListByNew, setMedicationSelectListByNew] = React.useState([]); |
|
214 |
+ const [medicationSelectListByNew, setMedicationSelectListByNew] = |
|
215 |
+ React.useState([]); |
|
160 | 216 |
// 최근 복약률 조회 |
161 | 217 |
const seniorMedicationSelectListByNew = () => { |
162 | 218 |
fetch("/user/seniorMedicationSelectListByNew.json", { |
163 | 219 |
method: "POST", |
164 | 220 |
headers: { |
165 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
221 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
166 | 222 |
}, |
167 |
- body: JSON.stringify({ "agent_id": state.loginUser['user_id'] }), |
|
168 |
- }).then((response) => response.json()).then((data) => { |
|
169 |
- console.log("seniorMedicationSelectListByNew : ", data); |
|
170 |
- setMedicationSelectListByNew(data) |
|
171 |
- }).catch((error) => { |
|
172 |
- console.log('seniorMedicationSelectListByNew() /user/seniorMedicationSelectListByNew.json error : ', error); |
|
173 |
- }); |
|
223 |
+ body: JSON.stringify({ |
|
224 |
+ agent_id: state.loginUser["user_id"], |
|
225 |
+ }), |
|
226 |
+ }) |
|
227 |
+ .then((response) => response.json()) |
|
228 |
+ .then((data) => { |
|
229 |
+ console.log("seniorMedicationSelectListByNew : ", data); |
|
230 |
+ setMedicationSelectListByNew(data); |
|
231 |
+ }) |
|
232 |
+ .catch((error) => { |
|
233 |
+ console.log( |
|
234 |
+ "seniorMedicationSelectListByNew() /user/seniorMedicationSelectListByNew.json error : ", |
|
235 |
+ error |
|
236 |
+ ); |
|
237 |
+ }); |
|
174 | 238 |
}; |
175 | 239 |
|
176 | 240 |
// 복약률 목록 |
177 |
- const [medicationSelectListByMonth, setMedicationSelectListByMonth] = React.useState([]); |
|
178 |
- const [medicationSelectListByYear, setMedicationSelectListByYear] = React.useState([]); |
|
241 |
+ const [medicationSelectListByMonth, setMedicationSelectListByMonth] = |
|
242 |
+ React.useState([]); |
|
243 |
+ const [medicationSelectListByYear, setMedicationSelectListByYear] = |
|
244 |
+ React.useState([]); |
|
179 | 245 |
// 복약률 목록 조회 |
180 | 246 |
const seniorMedicationSelectListByMonth = () => { |
181 | 247 |
let totalYearArr = []; |
... | ... | @@ -184,53 +250,72 @@ |
184 | 250 |
fetch("/user/seniorMedicationSelectListByMonth.json", { |
185 | 251 |
method: "POST", |
186 | 252 |
headers: { |
187 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
253 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
188 | 254 |
}, |
189 |
- body: JSON.stringify({ "agency_id": state.loginUser['agency_id'] }), |
|
190 |
- }).then((response) => response.json()).then((data) => { |
|
191 |
- data.map((item, idx) => { |
|
192 |
- item['year'] = item['medication_default_date'].substr(0, 4); |
|
193 |
- item['month'] = item['medication_default_date'].substr(5, 2); |
|
194 |
- totalYearArr.push(item['medication_default_date'].substr(0, 4)); |
|
195 |
- item['date'] = new Date(item['medication_default_date']).getTime(); |
|
196 |
- }) |
|
197 |
- |
|
198 |
- const setYearArr = [...new Set(totalYearArr)]; |
|
199 |
- console.log("totalYearArr: ", setYearArr); |
|
200 |
- |
|
201 |
- setYearArr.map((year, yearIdx) => { |
|
202 |
- let sum = 0; |
|
203 |
- let count = 0; |
|
204 |
- let avg = 0; |
|
205 |
- |
|
206 |
- let monthArr = ['-', '-', '-', '-', '-', '-', '-', '-', '-', '-', '-', '-']; |
|
207 |
- |
|
255 |
+ body: JSON.stringify({ agency_id: state.loginUser["agency_id"] }), |
|
256 |
+ }) |
|
257 |
+ .then((response) => response.json()) |
|
258 |
+ .then((data) => { |
|
208 | 259 |
data.map((item, idx) => { |
209 |
- if (item['year'] == year) { |
|
210 |
- sum += item['average']; |
|
211 |
- count++; |
|
212 |
- monthArr[Number(item['month']) - 1] = item['average']; |
|
213 |
- } |
|
214 |
- }) |
|
260 |
+ item["year"] = item["medication_default_date"].substr(0, 4); |
|
261 |
+ item["month"] = item["medication_default_date"].substr(5, 2); |
|
262 |
+ totalYearArr.push(item["medication_default_date"].substr(0, 4)); |
|
263 |
+ item["date"] = new Date(item["medication_default_date"]).getTime(); |
|
264 |
+ }); |
|
215 | 265 |
|
216 |
- avg = parseInt(sum / count); |
|
217 |
- yearArr.push({ "year": [year], "average": avg, "data": monthArr }); |
|
266 |
+ const setYearArr = [...new Set(totalYearArr)]; |
|
267 |
+ console.log("totalYearArr: ", setYearArr); |
|
268 |
+ |
|
269 |
+ setYearArr.map((year, yearIdx) => { |
|
270 |
+ let sum = 0; |
|
271 |
+ let count = 0; |
|
272 |
+ let avg = 0; |
|
273 |
+ |
|
274 |
+ let monthArr = [ |
|
275 |
+ "-", |
|
276 |
+ "-", |
|
277 |
+ "-", |
|
278 |
+ "-", |
|
279 |
+ "-", |
|
280 |
+ "-", |
|
281 |
+ "-", |
|
282 |
+ "-", |
|
283 |
+ "-", |
|
284 |
+ "-", |
|
285 |
+ "-", |
|
286 |
+ "-", |
|
287 |
+ ]; |
|
288 |
+ |
|
289 |
+ data.map((item, idx) => { |
|
290 |
+ if (item["year"] == year) { |
|
291 |
+ sum += item["average"]; |
|
292 |
+ count++; |
|
293 |
+ monthArr[Number(item["month"]) - 1] = item["average"]; |
|
294 |
+ } |
|
295 |
+ }); |
|
296 |
+ |
|
297 |
+ avg = parseInt(sum / count); |
|
298 |
+ yearArr.push({ year: [year], average: avg, data: monthArr }); |
|
299 |
+ }); |
|
300 |
+ |
|
301 |
+ console.log("yearArr : ", yearArr); |
|
302 |
+ setMedicationSelectListByYear(yearArr); |
|
303 |
+ |
|
304 |
+ console.log("seniorMedicationSelectListByMonth : ", data); |
|
305 |
+ setMedicationSelectListByMonth(data); |
|
218 | 306 |
}) |
219 |
- |
|
220 |
- console.log("yearArr : ", yearArr); |
|
221 |
- setMedicationSelectListByYear(yearArr); |
|
222 |
- |
|
223 |
- console.log("seniorMedicationSelectListByMonth : ", data); |
|
224 |
- setMedicationSelectListByMonth(data); |
|
225 |
- }).catch((error) => { |
|
226 |
- console.log('seniorMedicationSelectListByMonth() /user/seniorMedicationSelectListByMonth.json error : ', error); |
|
227 |
- }); |
|
307 |
+ .catch((error) => { |
|
308 |
+ console.log( |
|
309 |
+ "seniorMedicationSelectListByMonth() /user/seniorMedicationSelectListByMonth.json error : ", |
|
310 |
+ error |
|
311 |
+ ); |
|
312 |
+ }); |
|
228 | 313 |
}; |
229 |
- |
|
230 | 314 |
|
231 | 315 |
React.useEffect(() => { |
232 | 316 |
temperatureRiskCount(); |
233 | 317 |
batteryRiskCount(); |
318 |
+ receiveDataMissRiskCount(); |
|
234 | 319 |
seniorMedicationSelectListByNew(); |
235 | 320 |
seniorMedicationSelectListByMonth(); |
236 | 321 |
mySeniorSelectList(); |
... | ... | @@ -238,10 +323,26 @@ |
238 | 323 |
|
239 | 324 |
return ( |
240 | 325 |
<main> |
241 |
- <div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"대상자 현황"} explanation={new Date(new Date().setDate(new Date().getDate() - 1)).toLocaleDateString().replace(/\./g, '').replace(/\s/g, '-') + " 기준"} /></div> |
|
326 |
+ <div className="flex-start margin-bottom2"> |
|
327 |
+ <img src={medicinebox} alt="" /> |
|
328 |
+ <TitleSmall |
|
329 |
+ title={"대상자 현황"} |
|
330 |
+ explanation={ |
|
331 |
+ new Date(new Date().setDate(new Date().getDate() - 1)) |
|
332 |
+ .toLocaleDateString() |
|
333 |
+ .replace(/\./g, "") |
|
334 |
+ .replace(/\s/g, "-") + " 기준" |
|
335 |
+ } |
|
336 |
+ /> |
|
337 |
+ </div> |
|
242 | 338 |
<div className="main-grid-agency margin-bottom2"> |
243 | 339 |
<ul className="content-box statistics-agency" background="#8ef3d1"> |
244 |
- <li className="flex-start" onClick={() => { navigate("/Healthcare") }}> |
|
340 |
+ <li |
|
341 |
+ className="flex-start" |
|
342 |
+ onClick={() => { |
|
343 |
+ navigate("/Healthcare"); |
|
344 |
+ }} |
|
345 |
+ > |
|
245 | 346 |
<img src={medicineAgency} alt="" /> |
246 | 347 |
<div className="text"> |
247 | 348 |
<p>미복약 위험 대상자</p> |
... | ... | @@ -249,8 +350,13 @@ |
249 | 350 |
</div> |
250 | 351 |
</li> |
251 | 352 |
</ul> |
252 |
- <ul className="content-box statistics-agency" background="#ebe7b9" > |
|
253 |
- <li className="flex-start" onClick={() => { navigate("/Healthcare") }}> |
|
353 |
+ <ul className="content-box statistics-agency" background="#ebe7b9"> |
|
354 |
+ <li |
|
355 |
+ className="flex-start" |
|
356 |
+ onClick={() => { |
|
357 |
+ navigate("/Healthcare"); |
|
358 |
+ }} |
|
359 |
+ > |
|
254 | 360 |
<img src={temperatureAgency} alt="" /> |
255 | 361 |
<div className="text"> |
256 | 362 |
<p>댁내 온도 위험 대상자</p> |
... | ... | @@ -259,7 +365,12 @@ |
259 | 365 |
</li> |
260 | 366 |
</ul> |
261 | 367 |
<ul className="content-box statistics-agency" background="#5f9af3"> |
262 |
- <li className="flex-start" onClick={() => { navigate("/Healthcare") }}> |
|
368 |
+ <li |
|
369 |
+ className="flex-start" |
|
370 |
+ onClick={() => { |
|
371 |
+ navigate("/Healthcare"); |
|
372 |
+ }} |
|
373 |
+ > |
|
263 | 374 |
<img src={batteryAgency} alt="" /> |
264 | 375 |
<div className="text"> |
265 | 376 |
<p>배터리 부족 대상자</p> |
... | ... | @@ -268,24 +379,34 @@ |
268 | 379 |
</li> |
269 | 380 |
</ul> |
270 | 381 |
<ul className="content-box statistics-agency" background="#8080FF"> |
271 |
- <li className="flex-start" onClick={() => { navigate("/Healthcare") }}> |
|
382 |
+ <li |
|
383 |
+ className="flex-start" |
|
384 |
+ onClick={() => { |
|
385 |
+ navigate("/Healthcare"); |
|
386 |
+ }} |
|
387 |
+ > |
|
272 | 388 |
<img src={wifioff} alt="" /> |
273 | 389 |
<div className="text"> |
274 | 390 |
<p>데이터 미확인 대상자</p> |
275 |
- <p className="peoplecount">{batteryCount}</p> |
|
391 |
+ <p className="peoplecount">{receiveDataMissCount}</p> |
|
276 | 392 |
</div> |
277 | 393 |
</li> |
278 | 394 |
</ul> |
279 | 395 |
</div> |
280 |
- <div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"대상자 복약률 평균"} /></div> |
|
396 |
+ <div className="flex-start margin-bottom2"> |
|
397 |
+ <img src={medicinebox} alt="" /> |
|
398 |
+ <TitleSmall title={"대상자 복약률 평균"} /> |
|
399 |
+ </div> |
|
281 | 400 |
<div className="main-grid-agency margin-bottom2"> |
282 | 401 |
<div className="content-box combine-left-government4"> |
283 | 402 |
<div className="height-50"> |
284 |
- { |
|
285 |
- medicationSelectListByNew.length > 0 ? |
|
286 |
- <Chart10 data={medicationSelectListByMonth} /> |
|
287 |
- : <div className="no-data"><p>데이터가 없습니다.</p></div> |
|
288 |
- } |
|
403 |
+ {medicationSelectListByNew.length > 0 ? ( |
|
404 |
+ <Chart10 data={medicationSelectListByMonth} /> |
|
405 |
+ ) : ( |
|
406 |
+ <div className="no-data"> |
|
407 |
+ <p>데이터가 없습니다.</p> |
|
408 |
+ </div> |
|
409 |
+ )} |
|
289 | 410 |
</div> |
290 | 411 |
<div> |
291 | 412 |
<table className="visit-data-table"> |
... | ... | @@ -312,25 +433,27 @@ |
312 | 433 |
medicationSelectListByYear.map((item, idx) => { |
313 | 434 |
return ( |
314 | 435 |
<tr> |
315 |
- <td>{item['year']}</td> |
|
316 |
- <td>{item['average'] == '-' ? '-' : item['average'] + '%'}</td> |
|
317 |
- {CommonUtil.isEmpty(item['data']) == false ? ( |
|
318 |
- item['data'].map((item1, idx1) => { |
|
319 |
- return ( |
|
320 |
- <> |
|
321 |
- <td>{item1 == '-' ? '-' : item1 + '%'}</td> |
|
322 |
- </> |
|
323 |
- ) |
|
324 |
- }) |
|
325 |
- ) : null} |
|
436 |
+ <td>{item["year"]}</td> |
|
437 |
+ <td> |
|
438 |
+ {item["average"] == "-" ? "-" : item["average"] + "%"} |
|
439 |
+ </td> |
|
440 |
+ {CommonUtil.isEmpty(item["data"]) == false |
|
441 |
+ ? item["data"].map((item1, idx1) => { |
|
442 |
+ return ( |
|
443 |
+ <> |
|
444 |
+ <td>{item1 == "-" ? "-" : item1 + "%"}</td> |
|
445 |
+ </> |
|
446 |
+ ); |
|
447 |
+ }) |
|
448 |
+ : null} |
|
326 | 449 |
</tr> |
327 |
- ) |
|
450 |
+ ); |
|
328 | 451 |
}) |
329 |
- ) : |
|
452 |
+ ) : ( |
|
330 | 453 |
<tr> |
331 | 454 |
<td colSpan={14}>데이터가 없습니다.</td> |
332 | 455 |
</tr> |
333 |
- } |
|
456 |
+ )} |
|
334 | 457 |
</tbody> |
335 | 458 |
</table> |
336 | 459 |
</div> |
... | ... | @@ -349,29 +472,37 @@ |
349 | 472 |
</div> */} |
350 | 473 |
<div className="main-grid-agency2"> |
351 | 474 |
<div> |
352 |
- <div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"시니어 복약수 순위"} /></div> |
|
475 |
+ <div className="flex-start margin-bottom2"> |
|
476 |
+ <img src={medicinebox} alt="" /> |
|
477 |
+ <TitleSmall title={"시니어 복약수 순위"} /> |
|
478 |
+ </div> |
|
353 | 479 |
<div className="content-box combine-left-government3 visitlist margin-bottom2"> |
354 |
- { |
|
355 |
- medicationSelectListByNew.length > 0 ? |
|
356 |
- <Chart8 data={medicationSelectListByNew} /> |
|
357 |
- : <div className="no-data"><p>데이터가 없습니다.</p></div> |
|
358 |
- } |
|
480 |
+ {medicationSelectListByNew.length > 0 ? ( |
|
481 |
+ <Chart8 data={medicationSelectListByNew} /> |
|
482 |
+ ) : ( |
|
483 |
+ <div className="no-data"> |
|
484 |
+ <p>데이터가 없습니다.</p> |
|
485 |
+ </div> |
|
486 |
+ )} |
|
359 | 487 |
</div> |
360 | 488 |
</div> |
361 | 489 |
<div> |
362 |
- <div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"연령대별 통계"} /></div> |
|
490 |
+ <div className="flex-start margin-bottom2"> |
|
491 |
+ <img src={medicinebox} alt="" /> |
|
492 |
+ <TitleSmall title={"연령대별 통계"} /> |
|
493 |
+ </div> |
|
363 | 494 |
<div className="content-box combine-left-government3 visitlist margin-bottom2"> |
364 |
- { |
|
365 |
- seniorGenderData.length > 0 ? |
|
366 |
- <Chart7 data={seniorGenderData} /> |
|
367 |
- : <div className="no-data"><p>데이터가 없습니다.</p></div> |
|
368 |
- } |
|
495 |
+ {seniorGenderData.length > 0 ? ( |
|
496 |
+ <Chart7 data={seniorGenderData} /> |
|
497 |
+ ) : ( |
|
498 |
+ <div className="no-data"> |
|
499 |
+ <p>데이터가 없습니다.</p> |
|
500 |
+ </div> |
|
501 |
+ )} |
|
369 | 502 |
</div> |
370 | 503 |
</div> |
371 | 504 |
</div> |
372 |
- <div> |
|
373 |
- |
|
374 |
- </div> |
|
505 |
+ <div></div> |
|
375 | 506 |
</main> |
376 | 507 |
); |
377 | 508 |
} |
--- client/views/pages/main/Main_agencyAdmin.jsx
+++ client/views/pages/main/Main_agencyAdmin.jsx
... | ... | @@ -63,7 +63,7 @@ |
63 | 63 |
//온도 위험 대상자(시니어) 수 조회 |
64 | 64 |
const [temperatureCount, setTemperatureCount] = React.useState(0); |
65 | 65 |
const temperatureRiskCount = () => { |
66 |
- fetch("/stats/agencyTemperatureRisk.json", { |
|
66 |
+ fetch("/stats/temperatureRisk.json", { |
|
67 | 67 |
method: "POST", |
68 | 68 |
headers: { |
69 | 69 |
'Content-Type': 'application/json; charset=UTF-8' |
... | ... | @@ -75,14 +75,14 @@ |
75 | 75 |
console.log("온도 위험 대상자(시니어) 수 조회 : ", data); |
76 | 76 |
setTemperatureCount(data); |
77 | 77 |
}).catch((error) => { |
78 |
- console.log('temperatureRiskCount() /stats/agencyTemperatureRisk.json error : ', error); |
|
78 |
+ console.log('temperatureRiskCount() /stats/temperatureRisk.json error : ', error); |
|
79 | 79 |
}); |
80 | 80 |
} |
81 | 81 |
|
82 | 82 |
//배터리 부족 대상자(시니어) 수 조회 |
83 | 83 |
const [batteryCount, setbatteryCount] = React.useState(0); |
84 | 84 |
const batteryRiskCount = () => { |
85 |
- fetch("/stats/agencyBatteryRisk.json", { |
|
85 |
+ fetch("/stats/batteryRisk.json", { |
|
86 | 86 |
method: "POST", |
87 | 87 |
headers: { |
88 | 88 |
'Content-Type': 'application/json; charset=UTF-8' |
... | ... | @@ -94,7 +94,26 @@ |
94 | 94 |
console.log("배터리 부족 대상자(시니어) 수 조회 : ", data); |
95 | 95 |
setbatteryCount(data); |
96 | 96 |
}).catch((error) => { |
97 |
- console.log('batteryRiskCount() /stats/agencyBatteryRisk.json error : ', error); |
|
97 |
+ console.log('batteryRiskCount() /stats/batteryRisk.json error : ', error); |
|
98 |
+ }); |
|
99 |
+ } |
|
100 |
+ |
|
101 |
+ //데이터 미확인 대상자(시니어) 수 조회 |
|
102 |
+ const [receiveDataMissCount, setReceiveDataMissCount] = React.useState(0); |
|
103 |
+ const receiveDataMissRiskCount = () => { |
|
104 |
+ fetch("/stats/receiveDataMissCount.json", { |
|
105 |
+ method: "POST", |
|
106 |
+ headers: { |
|
107 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
108 |
+ }, |
|
109 |
+ body: JSON.stringify({ |
|
110 |
+ 'agency_id': state.loginUser['agency_id'], |
|
111 |
+ }), |
|
112 |
+ }).then((response) => response.json()).then((data) => { |
|
113 |
+ console.log("데이터 미확인 대상자(시니어) 수 조회 : ", data); |
|
114 |
+ setReceiveDataMissCount(data); |
|
115 |
+ }).catch((error) => { |
|
116 |
+ console.log('receiveDataMissRiskCount() /stats/receiveDataMissCount.json error : ', error); |
|
98 | 117 |
}); |
99 | 118 |
} |
100 | 119 |
|
... | ... | @@ -224,6 +243,7 @@ |
224 | 243 |
seniorSelectList(); |
225 | 244 |
temperatureRiskCount(); |
226 | 245 |
batteryRiskCount(); |
246 |
+ receiveDataMissRiskCount(); |
|
227 | 247 |
visitByMonthList(); |
228 | 248 |
seniorByAgent(); |
229 | 249 |
seniorMedicationSelectListByNew(); |
... | ... | @@ -259,7 +279,7 @@ |
259 | 279 |
<li onClick={() => { navigate("/HealthcareAdmin") }}> |
260 | 280 |
<p><WifiOffIcon sx={{ width: "50px", height: "50px", padding: "5px", color: "#ffffff", background: "#8080FF", borderRadius: "50px", boxSizing: "border-box" }} /></p> |
261 | 281 |
<p>{cityName} 데이터 미확인 대상자 </p> |
262 |
- <p>{batteryCount}</p> |
|
282 |
+ <p>{receiveDataMissCount}</p> |
|
263 | 283 |
</li> |
264 | 284 |
</ul> |
265 | 285 |
</div> |
--- client/views/pages/main/Main_government.jsx
+++ client/views/pages/main/Main_government.jsx
... | ... | @@ -2,11 +2,18 @@ |
2 | 2 |
import { useNavigate, useLocation } from "react-router"; |
3 | 3 |
import { useSelector } from "react-redux"; |
4 | 4 |
|
5 |
- |
|
6 |
- |
|
7 |
-import { MapContainer, TileLayer, LayerGroup, Marker, Circle, CircleMarker, Tooltip, Popup, useMap } from 'react-leaflet'; |
|
8 |
-import L, { CRS, latLng, bounds } from 'leaflet'; |
|
9 |
- |
|
5 |
+import { |
|
6 |
+ MapContainer, |
|
7 |
+ TileLayer, |
|
8 |
+ LayerGroup, |
|
9 |
+ Marker, |
|
10 |
+ Circle, |
|
11 |
+ CircleMarker, |
|
12 |
+ Tooltip, |
|
13 |
+ Popup, |
|
14 |
+ useMap, |
|
15 |
+} from "react-leaflet"; |
|
16 |
+import L, { CRS, latLng, bounds } from "leaflet"; |
|
10 | 17 |
|
11 | 18 |
import Title from "../../component/Title.jsx"; |
12 | 19 |
/* import Map from "../../component/chart/Map.jsx"; */ |
... | ... | @@ -16,11 +23,11 @@ |
16 | 23 |
import Chart8 from "../../component/chart/Chart8.jsx"; |
17 | 24 |
import RowChart_govern from "../../component/chart/RowChart_govern.jsx"; |
18 | 25 |
import AddCircleIcon from "@mui/icons-material/AddCircle"; |
19 |
-import BatteryCharging20Icon from '@mui/icons-material/BatteryCharging20'; |
|
20 |
-import DeviceThermostatIcon from '@mui/icons-material/DeviceThermostat'; |
|
21 |
-import MedicationIcon from '@mui/icons-material/Medication'; |
|
22 |
-import ElderlyIcon from '@mui/icons-material/Elderly'; |
|
23 |
-import WifiOffIcon from '@mui/icons-material/WifiOff'; |
|
26 |
+import BatteryCharging20Icon from "@mui/icons-material/BatteryCharging20"; |
|
27 |
+import DeviceThermostatIcon from "@mui/icons-material/DeviceThermostat"; |
|
28 |
+import MedicationIcon from "@mui/icons-material/Medication"; |
|
29 |
+import ElderlyIcon from "@mui/icons-material/Elderly"; |
|
30 |
+import WifiOffIcon from "@mui/icons-material/WifiOff"; |
|
24 | 31 |
|
25 | 32 |
import CommonUtil from "../../../resources/js/CommonUtil.js"; |
26 | 33 |
|
... | ... | @@ -31,68 +38,116 @@ |
31 | 38 |
const location = useLocation(); |
32 | 39 |
|
33 | 40 |
//전역 변수 저장 객체 |
34 |
- const state = useSelector((state) => { return state }); |
|
41 |
+ const state = useSelector((state) => { |
|
42 |
+ return state; |
|
43 |
+ }); |
|
35 | 44 |
|
36 |
- const [cityName, setCityName] = useState(state.loginUser['government_name']); |
|
45 |
+ const [cityName, setCityName] = useState(state.loginUser["government_name"]); |
|
37 | 46 |
|
38 | 47 |
//대상자(시니어) 목록 조회 |
39 |
- const [senior, setSenior] = React.useState({ userList: [], userListCount: 0 }); |
|
48 |
+ const [senior, setSenior] = React.useState({ |
|
49 |
+ userList: [], |
|
50 |
+ userListCount: 0, |
|
51 |
+ }); |
|
40 | 52 |
const seniorSelectList = () => { |
41 | 53 |
fetch("/user/userSelectList.json", { |
42 | 54 |
method: "POST", |
43 | 55 |
headers: { |
44 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
56 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
45 | 57 |
}, |
46 | 58 |
body: JSON.stringify({ |
47 |
- 'government_id': state.loginUser['government_id'], |
|
48 |
- 'agency_id': state.loginUser['agency_id'], |
|
49 |
- 'authority': 'ROLE_SENIOR', |
|
59 |
+ government_id: state.loginUser["government_id"], |
|
60 |
+ agency_id: state.loginUser["agency_id"], |
|
61 |
+ authority: "ROLE_SENIOR", |
|
50 | 62 |
}), |
51 |
- }).then((response) => response.json()).then((data) => { |
|
52 |
- console.log("대상자(시니어) 목록 조회 : ", data); |
|
53 |
- setSenior(data); |
|
54 |
- }).catch((error) => { |
|
55 |
- console.log('seniorSelectList() /user/userSelectList.json error : ', error); |
|
56 |
- }); |
|
57 |
- } |
|
63 |
+ }) |
|
64 |
+ .then((response) => response.json()) |
|
65 |
+ .then((data) => { |
|
66 |
+ console.log("대상자(시니어) 목록 조회 : ", data); |
|
67 |
+ setSenior(data); |
|
68 |
+ }) |
|
69 |
+ .catch((error) => { |
|
70 |
+ console.log( |
|
71 |
+ "seniorSelectList() /user/userSelectList.json error : ", |
|
72 |
+ error |
|
73 |
+ ); |
|
74 |
+ }); |
|
75 |
+ }; |
|
58 | 76 |
|
59 | 77 |
//온도 위험 대상자(시니어) 수 조회 |
60 | 78 |
const [temperatureCount, setTemperatureCount] = React.useState(0); |
61 | 79 |
const temperatureRiskCount = () => { |
62 |
- fetch("/stats/governmentTemperatureRisk.json", { |
|
80 |
+ fetch("/stats/temperatureRisk.json", { |
|
63 | 81 |
method: "POST", |
64 | 82 |
headers: { |
65 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
83 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
66 | 84 |
}, |
67 | 85 |
body: JSON.stringify({ |
68 |
- 'government_id': state.loginUser['government_id'], |
|
86 |
+ government_id: state.loginUser["government_id"], |
|
69 | 87 |
}), |
70 |
- }).then((response) => response.json()).then((data) => { |
|
71 |
- console.log("온도 위험 대상자(시니어) 수 조회 : ", data); |
|
72 |
- setTemperatureCount(data); |
|
73 |
- }).catch((error) => { |
|
74 |
- console.log('temperatureRiskCount() /stats/governmentTemperatureRisk.json error : ', error); |
|
75 |
- }); |
|
76 |
- } |
|
88 |
+ }) |
|
89 |
+ .then((response) => response.json()) |
|
90 |
+ .then((data) => { |
|
91 |
+ console.log("온도 위험 대상자(시니어) 수 조회 : ", data); |
|
92 |
+ setTemperatureCount(data); |
|
93 |
+ }) |
|
94 |
+ .catch((error) => { |
|
95 |
+ console.log( |
|
96 |
+ "temperatureRiskCount() /stats/temperatureRisk.json error : ", |
|
97 |
+ error |
|
98 |
+ ); |
|
99 |
+ }); |
|
100 |
+ }; |
|
77 | 101 |
|
78 | 102 |
//배터리 부족 대상자(시니어) 수 조회 |
79 | 103 |
const [batteryCount, setbatteryCount] = React.useState(0); |
80 | 104 |
const batteryRiskCount = () => { |
81 |
- fetch("/stats/governmentBatteryRisk.json", { |
|
105 |
+ fetch("/stats/batteryRisk.json", { |
|
82 | 106 |
method: "POST", |
83 | 107 |
headers: { |
84 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
108 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
85 | 109 |
}, |
86 | 110 |
body: JSON.stringify({ |
87 |
- 'government_id': state.loginUser['government_id'], |
|
111 |
+ government_id: state.loginUser["government_id"], |
|
88 | 112 |
}), |
89 |
- }).then((response) => response.json()).then((data) => { |
|
90 |
- console.log("배터리 부족 대상자(시니어) 수 조회 : ", data); |
|
91 |
- setbatteryCount(data); |
|
92 |
- }).catch((error) => { |
|
93 |
- console.log('batteryRiskCount() /stats/governmentBatteryRisk.json error : ', error); |
|
94 |
- }); |
|
95 |
- } |
|
113 |
+ }) |
|
114 |
+ .then((response) => response.json()) |
|
115 |
+ .then((data) => { |
|
116 |
+ console.log("배터리 부족 대상자(시니어) 수 조회 : ", data); |
|
117 |
+ setbatteryCount(data); |
|
118 |
+ }) |
|
119 |
+ .catch((error) => { |
|
120 |
+ console.log( |
|
121 |
+ "batteryRiskCount() /stats/batteryRisk.json error : ", |
|
122 |
+ error |
|
123 |
+ ); |
|
124 |
+ }); |
|
125 |
+ }; |
|
126 |
+ |
|
127 |
+ //데이터 미확인 대상자(시니어) 수 조회 |
|
128 |
+ const [receiveDataMissCount, setReceiveDataMissCount] = React.useState(0); |
|
129 |
+ const receiveDataMissRiskCount = () => { |
|
130 |
+ fetch("/stats/receiveDataMissCount.json", { |
|
131 |
+ method: "POST", |
|
132 |
+ headers: { |
|
133 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
134 |
+ }, |
|
135 |
+ body: JSON.stringify({ |
|
136 |
+ government_id: state.loginUser["government_id"], |
|
137 |
+ }), |
|
138 |
+ }) |
|
139 |
+ .then((response) => response.json()) |
|
140 |
+ .then((data) => { |
|
141 |
+ console.log("데이터 미확인 대상자(시니어) 수 조회 : ", data); |
|
142 |
+ setReceiveDataMissCount(data); |
|
143 |
+ }) |
|
144 |
+ .catch((error) => { |
|
145 |
+ console.log( |
|
146 |
+ "receiveDataMissRiskCount() /stats/receiveDataMissCount.json error : ", |
|
147 |
+ error |
|
148 |
+ ); |
|
149 |
+ }); |
|
150 |
+ }; |
|
96 | 151 |
|
97 | 152 |
//월별 방문 횟수 조회 |
98 | 153 |
const [visit, setVisit] = React.useState([]); |
... | ... | @@ -100,18 +155,24 @@ |
100 | 155 |
fetch("/stats/visitByMonthList.json", { |
101 | 156 |
method: "POST", |
102 | 157 |
headers: { |
103 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
158 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
104 | 159 |
}, |
105 | 160 |
body: JSON.stringify({ |
106 |
- 'government_id': state.loginUser['government_id'], |
|
161 |
+ government_id: state.loginUser["government_id"], |
|
107 | 162 |
}), |
108 |
- }).then((response) => response.json()).then((data) => { |
|
109 |
- console.log("월별 방문 횟수 조회 : ", data); |
|
110 |
- setVisit(data); |
|
111 |
- }).catch((error) => { |
|
112 |
- console.log('visitByMonthList() /stats/visitByMonthList.json error : ', error); |
|
113 |
- }); |
|
114 |
- } |
|
163 |
+ }) |
|
164 |
+ .then((response) => response.json()) |
|
165 |
+ .then((data) => { |
|
166 |
+ console.log("월별 방문 횟수 조회 : ", data); |
|
167 |
+ setVisit(data); |
|
168 |
+ }) |
|
169 |
+ .catch((error) => { |
|
170 |
+ console.log( |
|
171 |
+ "visitByMonthList() /stats/visitByMonthList.json error : ", |
|
172 |
+ error |
|
173 |
+ ); |
|
174 |
+ }); |
|
175 |
+ }; |
|
115 | 176 |
|
116 | 177 |
//시행 기관별 약상자 사용 현황 |
117 | 178 |
const [equipmentUsage, setEquipmentUsage] = React.useState([]); |
... | ... | @@ -119,20 +180,29 @@ |
119 | 180 |
fetch("/stats/equipmentByAgency.json", { |
120 | 181 |
method: "POST", |
121 | 182 |
headers: { |
122 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
183 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
123 | 184 |
}, |
124 | 185 |
body: JSON.stringify({ |
125 |
- 'government_id': state.loginUser['government_id'], |
|
186 |
+ government_id: state.loginUser["government_id"], |
|
126 | 187 |
}), |
127 |
- }).then((response) => response.json()).then((data) => { |
|
128 |
- console.log("시행기관별 약상자 사용 현황 : ", data); |
|
129 |
- let newEquipmentList = updateList(data['agencyList'], data['equipmentList']); |
|
130 |
- console.log("new equipment list", newEquipmentList); |
|
131 |
- setEquipmentUsage(newEquipmentList); |
|
132 |
- }).catch((error) => { |
|
133 |
- console.log('equipmentByAgency() /stats/equipmentByAgency.json error : ', error); |
|
134 |
- }); |
|
135 |
- } |
|
188 |
+ }) |
|
189 |
+ .then((response) => response.json()) |
|
190 |
+ .then((data) => { |
|
191 |
+ console.log("시행기관별 약상자 사용 현황 : ", data); |
|
192 |
+ let newEquipmentList = updateList( |
|
193 |
+ data["agencyList"], |
|
194 |
+ data["equipmentList"] |
|
195 |
+ ); |
|
196 |
+ console.log("new equipment list", newEquipmentList); |
|
197 |
+ setEquipmentUsage(newEquipmentList); |
|
198 |
+ }) |
|
199 |
+ .catch((error) => { |
|
200 |
+ console.log( |
|
201 |
+ "equipmentByAgency() /stats/equipmentByAgency.json error : ", |
|
202 |
+ error |
|
203 |
+ ); |
|
204 |
+ }); |
|
205 |
+ }; |
|
136 | 206 |
|
137 | 207 |
//시행 기관별 대상자(시니어) 등록 현황 |
138 | 208 |
const [seniorEnroll, setSeniorEnroll] = React.useState([]); |
... | ... | @@ -140,42 +210,57 @@ |
140 | 210 |
fetch("/stats/seniorByAgency.json", { |
141 | 211 |
method: "POST", |
142 | 212 |
headers: { |
143 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
213 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
144 | 214 |
}, |
145 | 215 |
body: JSON.stringify({ |
146 |
- 'government_id': state.loginUser['government_id'], |
|
216 |
+ government_id: state.loginUser["government_id"], |
|
147 | 217 |
}), |
148 |
- }).then((response) => response.json()).then((data) => { |
|
149 |
- console.log("시행기관별 시니어 등록 현황 : ", data); |
|
150 |
- let newSeniorList = updateList(data['agencyList'], data['seniorList']); |
|
151 |
- console.log("new senior list", newSeniorList); |
|
152 |
- setSeniorEnroll(newSeniorList); |
|
153 |
- }).catch((error) => { |
|
154 |
- console.log('seniorByAgency() /stats/seniorByAgency.json error : ', error); |
|
155 |
- }); |
|
156 |
- } |
|
218 |
+ }) |
|
219 |
+ .then((response) => response.json()) |
|
220 |
+ .then((data) => { |
|
221 |
+ console.log("시행기관별 시니어 등록 현황 : ", data); |
|
222 |
+ let newSeniorList = updateList(data["agencyList"], data["seniorList"]); |
|
223 |
+ console.log("new senior list", newSeniorList); |
|
224 |
+ setSeniorEnroll(newSeniorList); |
|
225 |
+ }) |
|
226 |
+ .catch((error) => { |
|
227 |
+ console.log( |
|
228 |
+ "seniorByAgency() /stats/seniorByAgency.json error : ", |
|
229 |
+ error |
|
230 |
+ ); |
|
231 |
+ }); |
|
232 |
+ }; |
|
157 | 233 |
|
158 | 234 |
// 최근 복약률 |
159 |
- const [medicationSelectListByNew, setMedicationSelectListByNew] = React.useState([]); |
|
235 |
+ const [medicationSelectListByNew, setMedicationSelectListByNew] = |
|
236 |
+ React.useState([]); |
|
160 | 237 |
// 최근 복약률 조회 |
161 | 238 |
const seniorMedicationSelectListByNew = () => { |
162 | 239 |
fetch("/user/seniorMedicationSelectListByNew.json", { |
163 | 240 |
method: "POST", |
164 | 241 |
headers: { |
165 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
242 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
166 | 243 |
}, |
167 |
- body: JSON.stringify({ "government_id": state.loginUser['government_id'] }), |
|
168 |
- }).then((response) => response.json()).then((data) => { |
|
169 |
- console.log("seniorMedicationSelectListByNew : ", data); |
|
170 |
- setMedicationSelectListByNew(data) |
|
171 |
- }).catch((error) => { |
|
172 |
- console.log('seniorMedicationSelectListByNew() /user/seniorMedicationSelectListByNew.json error : ', error); |
|
173 |
- }); |
|
244 |
+ body: JSON.stringify({ government_id: state.loginUser["government_id"] }), |
|
245 |
+ }) |
|
246 |
+ .then((response) => response.json()) |
|
247 |
+ .then((data) => { |
|
248 |
+ console.log("seniorMedicationSelectListByNew : ", data); |
|
249 |
+ setMedicationSelectListByNew(data); |
|
250 |
+ }) |
|
251 |
+ .catch((error) => { |
|
252 |
+ console.log( |
|
253 |
+ "seniorMedicationSelectListByNew() /user/seniorMedicationSelectListByNew.json error : ", |
|
254 |
+ error |
|
255 |
+ ); |
|
256 |
+ }); |
|
174 | 257 |
}; |
175 | 258 |
|
176 | 259 |
// 복약률 목록 |
177 |
- const [medicationSelectListByMonth, setMedicationSelectListByMonth] = React.useState([]); |
|
178 |
- const [medicationSelectListByYear, setMedicationSelectListByYear] = React.useState([]); |
|
260 |
+ const [medicationSelectListByMonth, setMedicationSelectListByMonth] = |
|
261 |
+ React.useState([]); |
|
262 |
+ const [medicationSelectListByYear, setMedicationSelectListByYear] = |
|
263 |
+ React.useState([]); |
|
179 | 264 |
// 복약률 목록 조회 |
180 | 265 |
const seniorMedicationSelectListByMonth = () => { |
181 | 266 |
let totalYearArr = []; |
... | ... | @@ -184,49 +269,67 @@ |
184 | 269 |
fetch("/user/seniorMedicationSelectListByMonth.json", { |
185 | 270 |
method: "POST", |
186 | 271 |
headers: { |
187 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
272 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
188 | 273 |
}, |
189 |
- body: JSON.stringify({ "government_id": state.loginUser['government_id'] }), |
|
190 |
- }).then((response) => response.json()).then((data) => { |
|
191 |
- data.map((item, idx) => { |
|
192 |
- item['year'] = item['medication_default_date'].substr(0, 4); |
|
193 |
- item['month'] = item['medication_default_date'].substr(5, 2); |
|
194 |
- totalYearArr.push(item['medication_default_date'].substr(0, 4)); |
|
195 |
- item['date'] = new Date(item['medication_default_date']).getTime(); |
|
196 |
- }) |
|
197 |
- |
|
198 |
- const setYearArr = [...new Set(totalYearArr)]; |
|
199 |
- console.log("totalYearArr: ", setYearArr); |
|
200 |
- |
|
201 |
- setYearArr.map((year, yearIdx) => { |
|
202 |
- let sum = 0; |
|
203 |
- let count = 0; |
|
204 |
- let avg = 0; |
|
205 |
- |
|
206 |
- let monthArr = ['-', '-', '-', '-', '-', '-', '-', '-', '-', '-', '-', '-']; |
|
207 |
- |
|
274 |
+ body: JSON.stringify({ government_id: state.loginUser["government_id"] }), |
|
275 |
+ }) |
|
276 |
+ .then((response) => response.json()) |
|
277 |
+ .then((data) => { |
|
208 | 278 |
data.map((item, idx) => { |
209 |
- if (item['year'] == year) { |
|
210 |
- sum += item['average']; |
|
211 |
- count++; |
|
212 |
- monthArr[Number(item['month']) - 1] = item['average']; |
|
213 |
- } |
|
214 |
- }) |
|
279 |
+ item["year"] = item["medication_default_date"].substr(0, 4); |
|
280 |
+ item["month"] = item["medication_default_date"].substr(5, 2); |
|
281 |
+ totalYearArr.push(item["medication_default_date"].substr(0, 4)); |
|
282 |
+ item["date"] = new Date(item["medication_default_date"]).getTime(); |
|
283 |
+ }); |
|
215 | 284 |
|
216 |
- avg = parseInt(sum / count); |
|
217 |
- yearArr.push({ "year": [year], "average": avg, "data": monthArr }); |
|
285 |
+ const setYearArr = [...new Set(totalYearArr)]; |
|
286 |
+ console.log("totalYearArr: ", setYearArr); |
|
287 |
+ |
|
288 |
+ setYearArr.map((year, yearIdx) => { |
|
289 |
+ let sum = 0; |
|
290 |
+ let count = 0; |
|
291 |
+ let avg = 0; |
|
292 |
+ |
|
293 |
+ let monthArr = [ |
|
294 |
+ "-", |
|
295 |
+ "-", |
|
296 |
+ "-", |
|
297 |
+ "-", |
|
298 |
+ "-", |
|
299 |
+ "-", |
|
300 |
+ "-", |
|
301 |
+ "-", |
|
302 |
+ "-", |
|
303 |
+ "-", |
|
304 |
+ "-", |
|
305 |
+ "-", |
|
306 |
+ ]; |
|
307 |
+ |
|
308 |
+ data.map((item, idx) => { |
|
309 |
+ if (item["year"] == year) { |
|
310 |
+ sum += item["average"]; |
|
311 |
+ count++; |
|
312 |
+ monthArr[Number(item["month"]) - 1] = item["average"]; |
|
313 |
+ } |
|
314 |
+ }); |
|
315 |
+ |
|
316 |
+ avg = parseInt(sum / count); |
|
317 |
+ yearArr.push({ year: [year], average: avg, data: monthArr }); |
|
318 |
+ }); |
|
319 |
+ |
|
320 |
+ console.log("yearArr : ", yearArr); |
|
321 |
+ setMedicationSelectListByYear(yearArr); |
|
322 |
+ |
|
323 |
+ console.log("seniorMedicationSelectListByMonth : ", data); |
|
324 |
+ setMedicationSelectListByMonth(data); |
|
218 | 325 |
}) |
219 |
- |
|
220 |
- console.log("yearArr : ", yearArr); |
|
221 |
- setMedicationSelectListByYear(yearArr); |
|
222 |
- |
|
223 |
- console.log("seniorMedicationSelectListByMonth : ", data); |
|
224 |
- setMedicationSelectListByMonth(data); |
|
225 |
- }).catch((error) => { |
|
226 |
- console.log('seniorMedicationSelectListByMonth() /user/seniorMedicationSelectListByMonth.json error : ', error); |
|
227 |
- }); |
|
326 |
+ .catch((error) => { |
|
327 |
+ console.log( |
|
328 |
+ "seniorMedicationSelectListByMonth() /user/seniorMedicationSelectListByMonth.json error : ", |
|
329 |
+ error |
|
330 |
+ ); |
|
331 |
+ }); |
|
228 | 332 |
}; |
229 |
- |
|
230 | 333 |
|
231 | 334 |
function updateList(agencyList, countList) { |
232 | 335 |
const result = []; |
... | ... | @@ -239,24 +342,27 @@ |
239 | 342 |
break; |
240 | 343 |
} |
241 | 344 |
} |
242 |
- result.push({ agency_id: agency.agency_id, agency_name: agency.agency_name, count: count }); |
|
345 |
+ result.push({ |
|
346 |
+ agency_id: agency.agency_id, |
|
347 |
+ agency_name: agency.agency_name, |
|
348 |
+ count: count, |
|
349 |
+ }); |
|
243 | 350 |
} |
244 | 351 |
return result; |
245 | 352 |
} |
246 | 353 |
|
247 |
- |
|
248 |
- |
|
249 | 354 |
const iconHouse = new L.Icon({ |
250 |
- iconUrl: '/client/resources/files/images/house.png', |
|
251 |
- iconRetinaUrl: '/client/resources/files/images/house.png', |
|
355 |
+ iconUrl: "/client/resources/files/images/house.png", |
|
356 |
+ iconRetinaUrl: "/client/resources/files/images/house.png", |
|
252 | 357 |
iconSize: [20, 20], |
253 |
- className: 'leaflet-background-radius-icon'//leaflet-div-icon |
|
358 |
+ className: "leaflet-background-radius-icon", //leaflet-div-icon |
|
254 | 359 |
}); |
255 | 360 |
|
256 | 361 |
React.useEffect(() => { |
257 | 362 |
seniorSelectList(); |
258 | 363 |
temperatureRiskCount(); |
259 | 364 |
batteryRiskCount(); |
365 |
+ receiveDataMissRiskCount(); |
|
260 | 366 |
visitByMonthList(); |
261 | 367 |
equipmentByAgency(); |
262 | 368 |
seniorByAgency(); |
... | ... | @@ -270,39 +376,93 @@ |
270 | 376 |
<div className="sub-grid-government"> |
271 | 377 |
<ul className="content-box statistics-govern" background="#8ef3d1"> |
272 | 378 |
<li> |
273 |
- <p><MedicationIcon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#076143", borderRadius: "50px" }} /></p> |
|
379 |
+ <p> |
|
380 |
+ <MedicationIcon |
|
381 |
+ sx={{ |
|
382 |
+ width: "50px", |
|
383 |
+ height: "50px", |
|
384 |
+ color: "#ffffff", |
|
385 |
+ background: "#076143", |
|
386 |
+ borderRadius: "50px", |
|
387 |
+ }} |
|
388 |
+ /> |
|
389 |
+ </p> |
|
274 | 390 |
<p>{cityName} 미복약 위험 대상자</p> |
275 | 391 |
<p>0</p> |
276 | 392 |
</li> |
277 | 393 |
</ul> |
278 |
- <ul className="content-box statistics-govern" background="#ebe7b9" > |
|
394 |
+ <ul className="content-box statistics-govern" background="#ebe7b9"> |
|
279 | 395 |
<li> |
280 |
- <p><DeviceThermostatIcon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#f1de05", borderRadius: "50px" }} /></p> |
|
396 |
+ <p> |
|
397 |
+ <DeviceThermostatIcon |
|
398 |
+ sx={{ |
|
399 |
+ width: "50px", |
|
400 |
+ height: "50px", |
|
401 |
+ color: "#ffffff", |
|
402 |
+ background: "#f1de05", |
|
403 |
+ borderRadius: "50px", |
|
404 |
+ }} |
|
405 |
+ /> |
|
406 |
+ </p> |
|
281 | 407 |
<p>{cityName} 댁내 온도 위험 대상자</p> |
282 |
- <p className={temperatureCount > 0 ? "red" : null}>{temperatureCount}</p> |
|
408 |
+ <p className={temperatureCount > 0 ? "red" : null}> |
|
409 |
+ {temperatureCount} |
|
410 |
+ </p> |
|
283 | 411 |
</li> |
284 | 412 |
</ul> |
285 | 413 |
<ul className="content-box statistics-govern" background="#5f9af3"> |
286 | 414 |
<li> |
287 |
- <p><BatteryCharging20Icon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#5f9af3", borderRadius: "50px" }} /></p> |
|
415 |
+ <p> |
|
416 |
+ <BatteryCharging20Icon |
|
417 |
+ sx={{ |
|
418 |
+ width: "50px", |
|
419 |
+ height: "50px", |
|
420 |
+ color: "#ffffff", |
|
421 |
+ background: "#5f9af3", |
|
422 |
+ borderRadius: "50px", |
|
423 |
+ }} |
|
424 |
+ /> |
|
425 |
+ </p> |
|
288 | 426 |
<p>{cityName} 배터리 부족 대상자 </p> |
289 | 427 |
<p>{batteryCount}</p> |
290 | 428 |
</li> |
291 | 429 |
</ul> |
292 | 430 |
<ul className="content-box statistics-govern" background="#8080FF"> |
293 | 431 |
<li> |
294 |
- <p><WifiOffIcon sx={{ width: "50px", height: "50px", padding: "5px", color: "#ffffff", background: "#8080FF", borderRadius: "50px", boxSizing: "border-box" }} /></p> |
|
432 |
+ <p> |
|
433 |
+ <WifiOffIcon |
|
434 |
+ sx={{ |
|
435 |
+ width: "50px", |
|
436 |
+ height: "50px", |
|
437 |
+ padding: "5px", |
|
438 |
+ color: "#ffffff", |
|
439 |
+ background: "#8080FF", |
|
440 |
+ borderRadius: "50px", |
|
441 |
+ boxSizing: "border-box", |
|
442 |
+ }} |
|
443 |
+ /> |
|
444 |
+ </p> |
|
295 | 445 |
<p>{cityName} 데이터 미확인 대상자 </p> |
296 |
- <p>{batteryCount}</p> |
|
446 |
+ <p>{receiveDataMissCount}</p> |
|
297 | 447 |
</li> |
298 | 448 |
</ul> |
299 | 449 |
</div> |
300 | 450 |
<div className="content-box combine-left-government combine-bottom-government2 main-main"> |
301 | 451 |
<div className="flex"> |
302 |
- <Title title={"지역별 케어 대상자 분포 현황"} explanation={"지역 선택 시 해당 지역의 대상자리스트가 보여집니다."} /> |
|
452 |
+ <Title |
|
453 |
+ title={"지역별 케어 대상자 분포 현황"} |
|
454 |
+ explanation={ |
|
455 |
+ "지역 선택 시 해당 지역의 대상자리스트가 보여집니다." |
|
456 |
+ } |
|
457 |
+ /> |
|
303 | 458 |
</div> |
304 |
- <div style={{ height: 'calc(100% - 60px)' }}> |
|
305 |
- <MapContainer center={latLng(35.8713802646197, 128.601805491072)} zoom={13} scrollWheelZoom={true} style={{ height: '100%' }}> |
|
459 |
+ <div style={{ height: "calc(100% - 60px)" }}> |
|
460 |
+ <MapContainer |
|
461 |
+ center={latLng(35.8713802646197, 128.601805491072)} |
|
462 |
+ zoom={13} |
|
463 |
+ scrollWheelZoom={true} |
|
464 |
+ style={{ height: "100%" }} |
|
465 |
+ > |
|
306 | 466 |
<TileLayer |
307 | 467 |
attribution='© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors' |
308 | 468 |
url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png" |
... | ... | @@ -315,15 +475,16 @@ |
315 | 475 |
|
316 | 476 |
<LayerGroup> |
317 | 477 |
{senior.userList.map((item, idx) => { |
318 |
- return item['y'] != null && item['x'] != null ? ( |
|
319 |
- <Marker position={[item['y'], item['x']]} icon={iconHouse}> |
|
478 |
+ return item["y"] != null && item["x"] != null ? ( |
|
479 |
+ <Marker position={[item["y"], item["x"]]} icon={iconHouse}> |
|
320 | 480 |
<Popup> |
321 | 481 |
<div> |
322 |
- {item['user_name']}({item['user_birth']} - {item['user_gender']}) |
|
482 |
+ {item["user_name"]}({item["user_birth"]} -{" "} |
|
483 |
+ {item["user_gender"]}) |
|
323 | 484 |
</div> |
324 | 485 |
</Popup> |
325 | 486 |
</Marker> |
326 |
- ) : null |
|
487 |
+ ) : null; |
|
327 | 488 |
})} |
328 | 489 |
</LayerGroup> |
329 | 490 |
</MapContainer> |
... | ... | @@ -340,48 +501,65 @@ |
340 | 501 |
<div className="flex"> |
341 | 502 |
<Title title={`${cityName} 복약수 순위`} /> |
342 | 503 |
</div> |
343 |
- <div style={{ height: 'calc(100% - 60px)' }}> |
|
344 |
- { |
|
345 |
- medicationSelectListByNew.length > 0 ? |
|
346 |
- <Chart8 data={medicationSelectListByNew} /> |
|
347 |
- : <div className="no-data"><p>데이터가 없습니다.</p></div> |
|
348 |
- } |
|
504 |
+ <div style={{ height: "calc(100% - 60px)" }}> |
|
505 |
+ {medicationSelectListByNew.length > 0 ? ( |
|
506 |
+ <Chart8 data={medicationSelectListByNew} /> |
|
507 |
+ ) : ( |
|
508 |
+ <div className="no-data"> |
|
509 |
+ <p>데이터가 없습니다.</p> |
|
510 |
+ </div> |
|
511 |
+ )} |
|
349 | 512 |
</div> |
350 | 513 |
</div> |
351 |
- <div className="content-box combine-left-government2" style={{ minHeight: '300px' }}> |
|
514 |
+ <div |
|
515 |
+ className="content-box combine-left-government2" |
|
516 |
+ style={{ minHeight: "300px" }} |
|
517 |
+ > |
|
352 | 518 |
<div className="flex"> |
353 |
- <Title title={`${cityName} 복약률 평균`} explanation={"해당 지역의 대상자 복약률이 그래프로 보여집니다."} /> |
|
519 |
+ <Title |
|
520 |
+ title={`${cityName} 복약률 평균`} |
|
521 |
+ explanation={"해당 지역의 대상자 복약률이 그래프로 보여집니다."} |
|
522 |
+ /> |
|
354 | 523 |
</div> |
355 |
- <div style={{ height: 'calc(100% - 60px)' }}> |
|
356 |
- { |
|
357 |
- medicationSelectListByMonth.length > 0 ? |
|
358 |
- <Chart2_govern data={medicationSelectListByMonth} /> |
|
359 |
- : <div className="no-data"><p>데이터가 없습니다.</p></div> |
|
360 |
- } |
|
524 |
+ <div style={{ height: "calc(100% - 60px)" }}> |
|
525 |
+ {medicationSelectListByMonth.length > 0 ? ( |
|
526 |
+ <Chart2_govern data={medicationSelectListByMonth} /> |
|
527 |
+ ) : ( |
|
528 |
+ <div className="no-data"> |
|
529 |
+ <p>데이터가 없습니다.</p> |
|
530 |
+ </div> |
|
531 |
+ )} |
|
361 | 532 |
</div> |
362 | 533 |
</div> |
363 | 534 |
<div className="content-box combine-right-government2"> |
364 | 535 |
<div className="flex"> |
365 |
- <Title title={`기관별 대상자 등록 현황`} explanation={"약상자 사용자의 데이터 차트가 보여집니다."} /> |
|
536 |
+ <Title |
|
537 |
+ title={`기관별 대상자 등록 현황`} |
|
538 |
+ explanation={"약상자 사용자의 데이터 차트가 보여집니다."} |
|
539 |
+ /> |
|
366 | 540 |
</div> |
367 |
- <div style={{ height: 'calc(100% - 60px)' }}> |
|
368 |
- { |
|
369 |
- seniorEnroll.length > 0 ? |
|
370 |
- <Chart5 data={seniorEnroll} /> |
|
371 |
- : <div className="no-data"><p>데이터가 없습니다.</p></div> |
|
372 |
- } |
|
541 |
+ <div style={{ height: "calc(100% - 60px)" }}> |
|
542 |
+ {seniorEnroll.length > 0 ? ( |
|
543 |
+ <Chart5 data={seniorEnroll} /> |
|
544 |
+ ) : ( |
|
545 |
+ <div className="no-data"> |
|
546 |
+ <p>데이터가 없습니다.</p> |
|
547 |
+ </div> |
|
548 |
+ )} |
|
373 | 549 |
</div> |
374 | 550 |
</div> |
375 | 551 |
<div className="content-box combine-right-government"> |
376 | 552 |
<div className="flex"> |
377 | 553 |
<Title title={`기관별 약상자 사용 현황`} explanation={""} /> |
378 | 554 |
</div> |
379 |
- <div style={{ height: 'calc(100% - 60px)' }}> |
|
380 |
- { |
|
381 |
- equipmentUsage.length > 0 ? |
|
382 |
- <Donut1_govern data={equipmentUsage} /> |
|
383 |
- : <div className="no-data"><p>데이터가 없습니다.</p></div> |
|
384 |
- } |
|
555 |
+ <div style={{ height: "calc(100% - 60px)" }}> |
|
556 |
+ {equipmentUsage.length > 0 ? ( |
|
557 |
+ <Donut1_govern data={equipmentUsage} /> |
|
558 |
+ ) : ( |
|
559 |
+ <div className="no-data"> |
|
560 |
+ <p>데이터가 없습니다.</p> |
|
561 |
+ </div> |
|
562 |
+ )} |
|
385 | 563 |
</div> |
386 | 564 |
</div> |
387 | 565 |
<div className="content-box span4 table-size-fix"> |
... | ... | @@ -408,19 +586,21 @@ |
408 | 586 |
{medicationSelectListByYear.map((item, idx) => { |
409 | 587 |
return ( |
410 | 588 |
<tr> |
411 |
- <td>{item['year']}</td> |
|
412 |
- <td>{item['average'] == '-' ? '-' : item['average'] + '%'}</td> |
|
413 |
- {CommonUtil.isEmpty(item['data']) == false ? ( |
|
414 |
- item['data'].map((item1, idx1) => { |
|
415 |
- return ( |
|
416 |
- <> |
|
417 |
- <td>{item1 == '-' ? '-' : item1 + '%'}</td> |
|
418 |
- </> |
|
419 |
- ) |
|
420 |
- }) |
|
421 |
- ) : null} |
|
589 |
+ <td>{item["year"]}</td> |
|
590 |
+ <td> |
|
591 |
+ {item["average"] == "-" ? "-" : item["average"] + "%"} |
|
592 |
+ </td> |
|
593 |
+ {CommonUtil.isEmpty(item["data"]) == false |
|
594 |
+ ? item["data"].map((item1, idx1) => { |
|
595 |
+ return ( |
|
596 |
+ <> |
|
597 |
+ <td>{item1 == "-" ? "-" : item1 + "%"}</td> |
|
598 |
+ </> |
|
599 |
+ ); |
|
600 |
+ }) |
|
601 |
+ : null} |
|
422 | 602 |
</tr> |
423 |
- ) |
|
603 |
+ ); |
|
424 | 604 |
})} |
425 | 605 |
</tbody> |
426 | 606 |
</table> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?