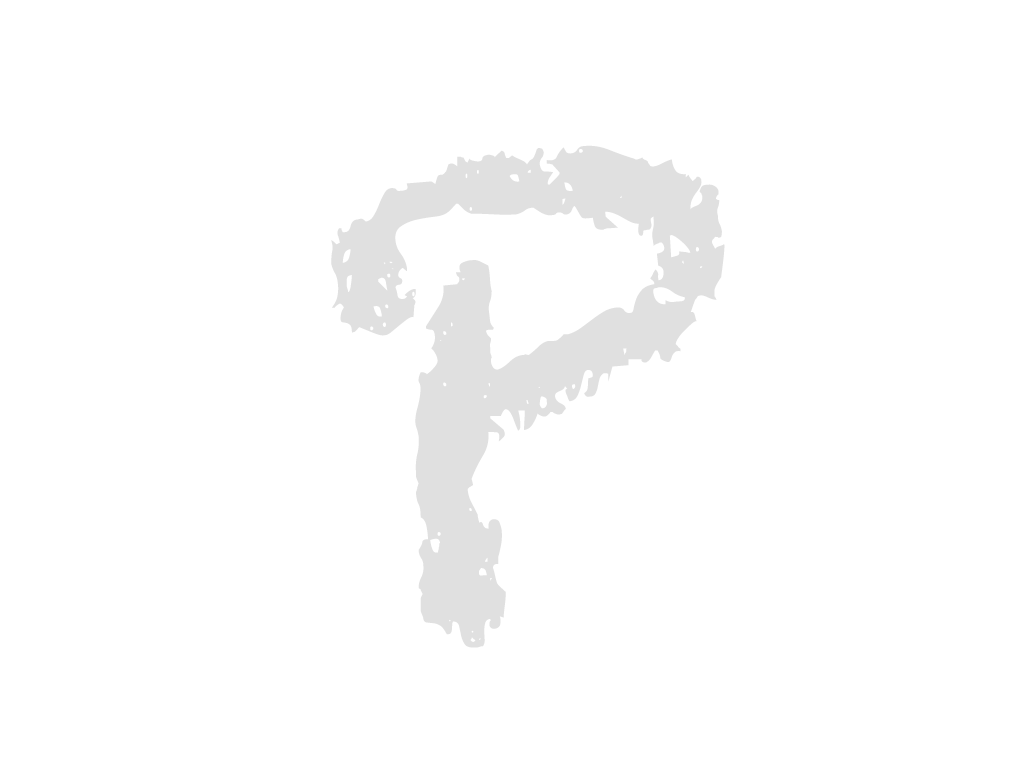
File name
Commit message
Commit date
File name
Commit message
Commit date
import React, { useState } from "react";
import { useNavigate, useLocation } from "react-router";
import { useSelector } from "react-redux";
import Calendar from "react-calendar";
import "react-calendar/dist/Calendar.css";
import Modal from "./Modal.jsx";
import moment from "moment";
import CommonUtil from "../../resources/js/CommonUtil.js";
export default function CalendarComponent({ data }) {
const navigate = useNavigate();
const location = useLocation();
//전역 변수 저장 객체
const state = useSelector((state) => { return state });
const [value, setValue] = useState(new Date());
const activeDate = moment(value).format('YYYY-MM-DD');
const monthOfActiveDate = moment(value).format('YYYY-MM');
const [activeMonth, setActiveMonth] = useState(monthOfActiveDate);
const getActiveMonth = (activeStartDate) => {
const newActiveMonth = moment(activeStartDate).format('YYYY-MM');
setActiveMonth(newActiveMonth);
};
const CalendarInner = (date) => {
var medicationData = '';
var temperatureData = '';
var todayTemperature = [];
data['equipmentRecordDataList'].map((item, idx) => {
if (item['date'] === date['date']) {
var medicationTotal = item['DAWN'] + item['MORNING'] + item['AFTERNOON'] + item['NIGHT'] + item['MIDNIGHT'];
if (typeof medicationTotal === 'string') {
medicationData = '-';
} else {
medicationData = medicationTotal + '/5'
}
if (CommonUtil.isEmpty(item['02:00']) == false) {
if (typeof item['02:00'] != 'string') {
todayTemperature.push(item['02:00']);
}
}
if (CommonUtil.isEmpty(item['10:00']) == false) {
if (typeof item['10:00'] != 'string') {
todayTemperature.push(item['10:00']);
}
}
if (CommonUtil.isEmpty(item['14:00']) == false) {
if (typeof item['14:00'] != 'string') {
todayTemperature.push(item['14:00']);
}
}
if (CommonUtil.isEmpty(item['23:00']) == false) {
if (typeof item['23:00'] != 'string') {
todayTemperature.push(item['23:00']);
}
}
}
})
if (todayTemperature == '-') {
temperatureData = '-'
} else {
if (todayTemperature.length > 0) {
var temperatures = "";
if (moment(date['date']).format("MM") >= 11 || moment(date['date']).format("MM") <= 2) {
temperatures = Math.min.apply(null, todayTemperature);
} else {
temperatures = Math.max.apply(null, todayTemperature);
}
temperatureData = temperatures + '℃'
}
}
return (
<>
<div>{medicationData}</div>
<div>{temperatureData}</div>
</>
)
}
React.useEffect(() => {
data.onChangeMonth(activeMonth);
}, [activeMonth])
return (
<>
<Calendar
onChange={setValue}
value={value}
tileContent={({ date, view }) => {
return (
<>
<div className="calendar-data">
<CalendarInner date={moment(date).format("YYYY-MM-DD")} />
</div>
</>
);
}}
onActiveStartDateChange={({ activeStartDate }) =>
getActiveMonth(activeStartDate)
}
onClickDay={(date, event) => {
let sendData = { date: moment(date).format("YYYY-MM-DD") };
data.onClick(sendData);
}}
/>
</>
);
}