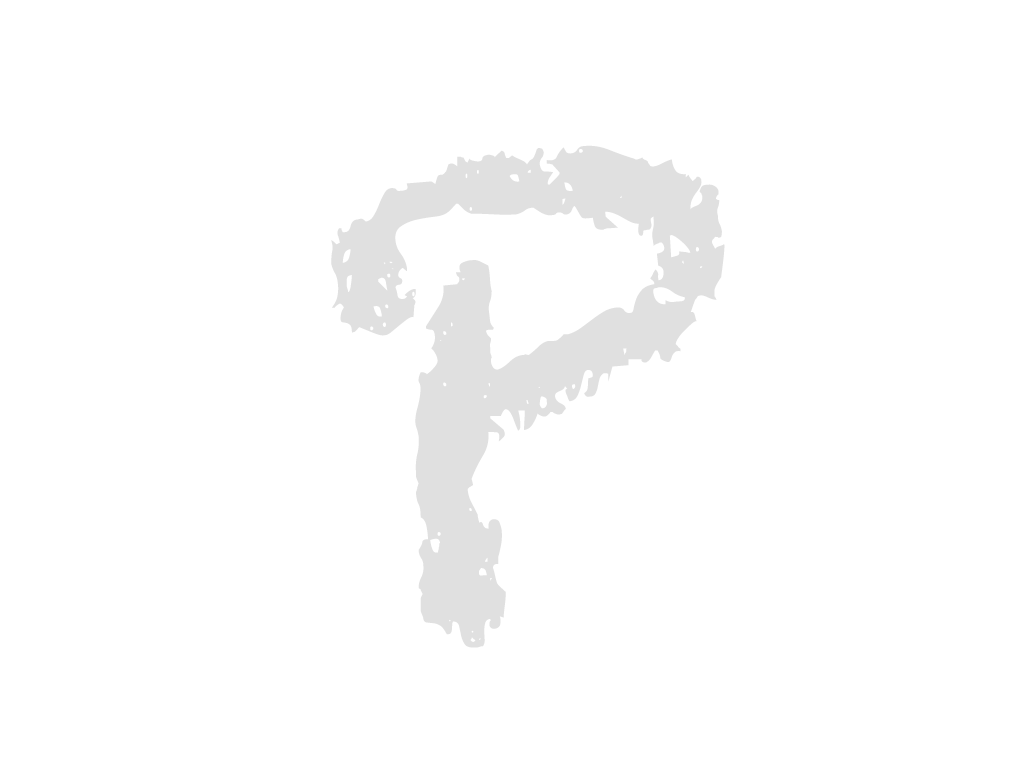
--- client/views/component/Calendar_admin.jsx
+++ client/views/component/Calendar_admin.jsx
... | ... | @@ -15,8 +15,78 @@ |
15 | 15 |
|
16 | 16 |
//전역 변수 저장 객체 |
17 | 17 |
const state = useSelector((state) => { return state }); |
18 |
- |
|
18 |
+ |
|
19 | 19 |
const [value, setValue] = useState(new Date()); |
20 |
+ const activeDate = moment(value).format('YYYY-MM-DD'); |
|
21 |
+ |
|
22 |
+ const monthOfActiveDate = moment(value).format('YYYY-MM'); |
|
23 |
+ const [activeMonth, setActiveMonth] = useState(monthOfActiveDate); |
|
24 |
+ |
|
25 |
+ const getActiveMonth = (activeStartDate) => { |
|
26 |
+ const newActiveMonth = moment(activeStartDate).format('YYYY-MM'); |
|
27 |
+ setActiveMonth(newActiveMonth); |
|
28 |
+ }; |
|
29 |
+ |
|
30 |
+ const CalendarInner = (date) => { |
|
31 |
+ var medicationData = ''; |
|
32 |
+ var temperatureData = ''; |
|
33 |
+ var todayTemperature = []; |
|
34 |
+ |
|
35 |
+ data['equipmentRecordDataList'].map((item, idx) => { |
|
36 |
+ if (item['date'] === date['date']) { |
|
37 |
+ var medicationTotal = item['DAWN'] + item['MORNING'] + item['AFTERNOON'] + item['NIGHT'] + item['MIDNIGHT']; |
|
38 |
+ if (typeof medicationTotal === 'string') { |
|
39 |
+ medicationData = '-'; |
|
40 |
+ } else { |
|
41 |
+ medicationData = medicationTotal + '/5' |
|
42 |
+ } |
|
43 |
+ |
|
44 |
+ if (CommonUtil.isEmpty(item['02:00']) == false) { |
|
45 |
+ if (typeof item['02:00'] != 'string') { |
|
46 |
+ todayTemperature.push(item['02:00']); |
|
47 |
+ } |
|
48 |
+ } |
|
49 |
+ if (CommonUtil.isEmpty(item['10:00']) == false) { |
|
50 |
+ if (typeof item['10:00'] != 'string') { |
|
51 |
+ todayTemperature.push(item['10:00']); |
|
52 |
+ } |
|
53 |
+ } |
|
54 |
+ if (CommonUtil.isEmpty(item['14:00']) == false) { |
|
55 |
+ if (typeof item['14:00'] != 'string') { |
|
56 |
+ todayTemperature.push(item['14:00']); |
|
57 |
+ } |
|
58 |
+ } |
|
59 |
+ if (CommonUtil.isEmpty(item['23:00']) == false) { |
|
60 |
+ if (typeof item['23:00'] != 'string') { |
|
61 |
+ todayTemperature.push(item['23:00']); |
|
62 |
+ } |
|
63 |
+ } |
|
64 |
+ } |
|
65 |
+ }) |
|
66 |
+ |
|
67 |
+ if (todayTemperature == '-') { |
|
68 |
+ temperatureData = '-' |
|
69 |
+ } else { |
|
70 |
+ if (todayTemperature.length > 0) { |
|
71 |
+ if (moment(date['date']).format("MM") >= 11 || moment(date['date']).format("MM") <= 2) { |
|
72 |
+ temperatureData = Math.min.apply(null, todayTemperature) + '℃' |
|
73 |
+ } else { |
|
74 |
+ temperatureData = Math.max.apply(null, todayTemperature) + '℃' |
|
75 |
+ } |
|
76 |
+ } |
|
77 |
+ } |
|
78 |
+ |
|
79 |
+ return ( |
|
80 |
+ <> |
|
81 |
+ <div>{medicationData}</div> |
|
82 |
+ <div>{temperatureData}</div> |
|
83 |
+ </> |
|
84 |
+ ) |
|
85 |
+ } |
|
86 |
+ |
|
87 |
+ React.useEffect(() => { |
|
88 |
+ data.onChangeMonth(activeMonth); |
|
89 |
+ }, [activeMonth]) |
|
20 | 90 |
|
21 | 91 |
return ( |
22 | 92 |
<> |
... | ... | @@ -27,12 +97,18 @@ |
27 | 97 |
return ( |
28 | 98 |
<> |
29 | 99 |
<div className="calendar-data"> |
30 |
- <div>3/5</div> |
|
31 |
- <div>30℃</div> |
|
100 |
+ <CalendarInner date={moment(date).format("YYYY-MM-DD")} /> |
|
32 | 101 |
</div> |
33 | 102 |
</> |
34 | 103 |
); |
35 | 104 |
}} |
105 |
+ onActiveStartDateChange={({ activeStartDate }) => |
|
106 |
+ getActiveMonth(activeStartDate) |
|
107 |
+ } |
|
108 |
+ onClickDay={(date, event) => { |
|
109 |
+ let sendData = { date: moment(date).format("YYYY-MM-DD") }; |
|
110 |
+ data.onClick(sendData); |
|
111 |
+ }} |
|
36 | 112 |
/> |
37 | 113 |
</> |
38 | 114 |
); |
+++ client/views/component/chart/Chart12.jsx
... | ... | @@ -0,0 +1,105 @@ |
1 | +import React, { Component } from "react"; | |
2 | +import * as am5 from "@amcharts/amcharts5"; | |
3 | +import * as am5xy from "@amcharts/amcharts5/xy"; | |
4 | +import am5themes_Animated from "@amcharts/amcharts5/themes/Animated"; | |
5 | +import CommonUtil from "../../../resources/js/CommonUtil"; | |
6 | + | |
7 | +var globalObject = {} | |
8 | + | |
9 | +export default function Chart12({ data }) { | |
10 | + const createChart = (drawChart) => { | |
11 | + if (globalObject[drawChart]) { | |
12 | + globalObject[drawChart].dispose() | |
13 | + } | |
14 | + | |
15 | + console.log('createChart12 data : ', data); | |
16 | + | |
17 | + let root = am5.Root.new("Chart12"); | |
18 | + root._logo.dispose(); | |
19 | + | |
20 | + | |
21 | + // Set themes | |
22 | + // https://www.amcharts.com/docs/v5/concepts/themes/ | |
23 | + root.setThemes([ | |
24 | + am5themes_Animated.new(root) | |
25 | + ]); | |
26 | + | |
27 | + | |
28 | + // Create chart | |
29 | + // https://www.amcharts.com/docs/v5/charts/xy-chart/ | |
30 | + let chart = root.container.children.push(am5xy.XYChart.new(root, { | |
31 | + panX: true, | |
32 | + panY: true, | |
33 | + wheelX: "panX", | |
34 | + wheelY: "zoomX", | |
35 | + pinchZoomX: true | |
36 | + })); | |
37 | + | |
38 | + | |
39 | + // Add cursor | |
40 | + // https://www.amcharts.com/docs/v5/charts/xy-chart/cursor/ | |
41 | + let cursor = chart.set("cursor", am5xy.XYCursor.new(root, { | |
42 | + behavior: "none" | |
43 | + })); | |
44 | + cursor.lineY.set("visible", false); | |
45 | + | |
46 | + | |
47 | + // Create axes | |
48 | + // https://www.amcharts.com/docs/v5/charts/xy-chart/axes/ | |
49 | + let xAxis = chart.xAxes.push(am5xy.DateAxis.new(root, { | |
50 | + baseInterval: { | |
51 | + timeUnit: "day", | |
52 | + count: 1 | |
53 | + }, | |
54 | + renderer: am5xy.AxisRendererX.new(root, {}), | |
55 | + tooltip: am5.Tooltip.new(root, {}) | |
56 | + })); | |
57 | + | |
58 | + let yAxis = chart.yAxes.push(am5xy.ValueAxis.new(root, { | |
59 | + min: 0, | |
60 | + max: 100, | |
61 | + renderer: am5xy.AxisRendererY.new(root, {}) | |
62 | + })); | |
63 | + | |
64 | + | |
65 | + // Add series | |
66 | + // https://www.amcharts.com/docs/v5/charts/xy-chart/series/ | |
67 | + let series = chart.series.push(am5xy.LineSeries.new(root, { | |
68 | + name: "Series", | |
69 | + xAxis: xAxis, | |
70 | + yAxis: yAxis, | |
71 | + valueYField: "medicationAvg", | |
72 | + valueXField: "datetime", | |
73 | + tooltip: am5.Tooltip.new(root, { | |
74 | + labelText: "{valueY}" | |
75 | + }) | |
76 | + })); | |
77 | + series.strokes.template.setAll({ | |
78 | + strokeWidth: 2, | |
79 | + strokeDasharray: [3, 3], | |
80 | + }); | |
81 | + | |
82 | + | |
83 | + // Set data | |
84 | + series.data.setAll(data); | |
85 | + | |
86 | + | |
87 | + // Make stuff animate on load | |
88 | + // https://www.amcharts.com/docs/v5/concepts/animations/ | |
89 | + series.appear(1000); | |
90 | + chart.appear(1000, 100); | |
91 | + | |
92 | + globalObject[drawChart] = root | |
93 | + } | |
94 | + | |
95 | + React.useEffect(() => { | |
96 | + if (CommonUtil.isEmpty(data) == false) { | |
97 | + console.log('React.useEffect chart2 data : ', data); | |
98 | + createChart(); | |
99 | + } | |
100 | + }, [data]) | |
101 | + | |
102 | + return ( | |
103 | + <div id="Chart12" style={{ width: "100%", height: "100%" }}></div> | |
104 | + ) | |
105 | +}(파일 끝에 줄바꿈 문자 없음) |
--- client/views/pages/equipment/EquipmentDataSelect.jsx
+++ client/views/pages/equipment/EquipmentDataSelect.jsx
... | ... | @@ -1,4 +1,4 @@ |
1 |
-import React from "react"; |
|
1 |
+import React, { useState } from "react"; |
|
2 | 2 |
import { useNavigate, useLocation } from "react-router"; |
3 | 3 |
import { useSelector } from "react-redux"; |
4 | 4 |
|
... | ... | @@ -8,8 +8,9 @@ |
8 | 8 |
import DetailTitle from "../../component/DetailTitle.jsx"; |
9 | 9 |
import Modal from "../../component/Modal.jsx"; |
10 | 10 |
import CalendarAdmin from "../../component/Calendar_admin.jsx"; |
11 |
-import Chart9 from "../../component/chart/Chart9.jsx"; |
|
11 |
+import Chart12 from "../../component/chart/Chart12.jsx"; |
|
12 | 12 |
import Senior from '../../../resources/files/images/senior.png'; |
13 |
+import CommonUtil from "../../../resources/js/CommonUtil.js"; |
|
13 | 14 |
|
14 | 15 |
export default function EquipmentDataSelect() { |
15 | 16 |
const navigate = useNavigate(); |
... | ... | @@ -17,25 +18,16 @@ |
17 | 18 |
|
18 | 19 |
//전역 변수 저장 객체 |
19 | 20 |
const state = useSelector((state) => { return state }); |
20 |
- console.log("state: ", state); |
|
21 | 21 |
|
22 |
- //장비 초기값 |
|
23 |
- const equipment = useLocation()['state']['equipment']; |
|
22 |
+ const now = new Date(); |
|
23 |
+ const [equipmentSearch, setEquipmentSearch] = React.useState({ |
|
24 |
+ 'equipment_serial_number': location.state['equipment_serial_number'], |
|
25 |
+ 'search_date': now.getFullYear() + "-" + (now.getMonth() + 1) |
|
26 |
+ }) |
|
24 | 27 |
|
25 |
- //장비 데이터 조회 |
|
26 |
- const equipmentDataSelect = () => { |
|
27 |
- fetch("/user/equipmentDataSelect.json", { |
|
28 |
- method: "POST", |
|
29 |
- headers: { |
|
30 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
31 |
- }, |
|
32 |
- body: JSON.stringify(equipment), |
|
33 |
- }).then((response) => response.json()).then((data) => { |
|
34 |
- console.log("equipmentDataSelect data : ", data); |
|
35 |
- }).catch((error) => { |
|
36 |
- console.log('equipmentDataSelect() /user/equipmentDataSelect.json error : ', error); |
|
37 |
- }); |
|
38 |
- }; |
|
28 |
+ const [equipmentRecordDataList, setEquipmentRecordDataList] = useState([]); |
|
29 |
+ |
|
30 |
+ const [modalDate, setModalDate] = React.useState(null); |
|
39 | 31 |
|
40 | 32 |
const [modalOpen, setModalOpen] = React.useState(false); |
41 | 33 |
const openModal = () => { |
... | ... | @@ -47,10 +39,130 @@ |
47 | 39 |
|
48 | 40 |
const calerndarClick = (data) => { |
49 | 41 |
console.log("calerndarClick - date, visitRecord", data); |
42 |
+ setModalDate(data.date); |
|
50 | 43 |
openModal(); |
51 | 44 |
} |
52 | 45 |
|
53 |
- const DateMedication = () => { |
|
46 |
+ const calerndarChangeMonth = (data) => { |
|
47 |
+ equipmentRecordDataSearchList(data); |
|
48 |
+ } |
|
49 |
+ |
|
50 |
+ //장비 데이터 조회 |
|
51 |
+ const equipmentRecordDataSearchList = (search_date) => { |
|
52 |
+ equipmentSearch['search_date'] = CommonUtil.isEmpty(search_date) ? now.getFullYear() + "-" + (now.getMonth() + 1) : search_date; |
|
53 |
+ setEquipmentSearch({ ...equipmentSearch }); |
|
54 |
+ |
|
55 |
+ console.log("search_date : ", equipmentSearch) |
|
56 |
+ |
|
57 |
+ fetch("/equipment/equipmentRecordDataList.json", { |
|
58 |
+ method: "POST", |
|
59 |
+ headers: { |
|
60 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
61 |
+ }, |
|
62 |
+ body: JSON.stringify(equipmentSearch), |
|
63 |
+ }).then((response) => response.json()).then((data) => { |
|
64 |
+ var codeList = []; |
|
65 |
+ data.map((item, idx) => { |
|
66 |
+ var code = { |
|
67 |
+ date: item['date'], |
|
68 |
+ datetime: new Date(item['date']).getTime(), |
|
69 |
+ DAWN: "-", |
|
70 |
+ MORNING: "-", |
|
71 |
+ AFTERNOON: "-", |
|
72 |
+ NIGHT: "-", |
|
73 |
+ MIDNIGHT: "-", |
|
74 |
+ "02:00": "-", |
|
75 |
+ "10:00": "-", |
|
76 |
+ "14:00": "-", |
|
77 |
+ "23:00": "-", |
|
78 |
+ battery: "-", |
|
79 |
+ } |
|
80 |
+ |
|
81 |
+ if (CommonUtil.isEmpty(item['medication_time_code_list']) == false) { |
|
82 |
+ code['DAWN'] = 0; |
|
83 |
+ code['MORNING'] = 0; |
|
84 |
+ code['AFTERNOON'] = 0; |
|
85 |
+ code['NIGHT'] = 0; |
|
86 |
+ code['MIDNIGHT'] = 0; |
|
87 |
+ |
|
88 |
+ item['medication_time_code_list'].map((item1, idx1) => { |
|
89 |
+ if (item1 == "DAWN") { |
|
90 |
+ code['DAWN'] = 1 |
|
91 |
+ } |
|
92 |
+ if (item1 == "MORNING") { |
|
93 |
+ code['MORNING'] = 1 |
|
94 |
+ } |
|
95 |
+ if (item1 == "AFTERNOON") { |
|
96 |
+ code['AFTERNOON'] = 1 |
|
97 |
+ } |
|
98 |
+ if (item1 == "NIGHT") { |
|
99 |
+ code['NIGHT'] = 1 |
|
100 |
+ } |
|
101 |
+ if (item1 == "MIDNIGHT") { |
|
102 |
+ code['MIDNIGHT'] = 1 |
|
103 |
+ } |
|
104 |
+ }) |
|
105 |
+ |
|
106 |
+ code['medicationAvg'] = ((code['DAWN'] + code['MORNING'] + code['AFTERNOON'] + code['NIGHT'] + code['MIDNIGHT']) / 5) * 100; |
|
107 |
+ } |
|
108 |
+ |
|
109 |
+ if (CommonUtil.isEmpty(item['temperature_datetime']) == false) { |
|
110 |
+ for (let i = 0; i < item['temperature_datetime'].length; i++) { |
|
111 |
+ code[item['temperature_datetime'][i].substr(11, 5)] = item['temperature_data'][i] |
|
112 |
+ } |
|
113 |
+ } |
|
114 |
+ if (CommonUtil.isEmpty(item['battery_power_data']) == false) { |
|
115 |
+ code['battery'] = item['battery_power_data'] |
|
116 |
+ }; |
|
117 |
+ codeList.push(code); |
|
118 |
+ }) |
|
119 |
+ setEquipmentRecordDataList(codeList); |
|
120 |
+ }).catch((error) => { |
|
121 |
+ console.log('equipmentRecordDataList() /equipment/equipmentRecordDataList.json error : ', error); |
|
122 |
+ }); |
|
123 |
+ }; |
|
124 |
+ |
|
125 |
+ const ModalInner = () => { |
|
126 |
+ var clickDayData = {}; |
|
127 |
+ equipmentRecordDataList.map((item, idx) => { |
|
128 |
+ if (item['date'] == modalDate) { |
|
129 |
+ if (CommonUtil.isEmpty(item['DAWN']) == false && CommonUtil.isEmpty(item['MORNING']) == false && CommonUtil.isEmpty(item['AFTERNOON']) == false && CommonUtil.isEmpty(item['NIGHT']) == false && CommonUtil.isEmpty(item['MIDNIGHT']) == false) { |
|
130 |
+ item['medication'] = true; |
|
131 |
+ } |
|
132 |
+ if (CommonUtil.isEmpty(item['02:00']) == false && CommonUtil.isEmpty(item['10:00']) == false && CommonUtil.isEmpty(item['14:00']) == false && CommonUtil.isEmpty(item['23:00']) == false) { |
|
133 |
+ item['temperature'] = true; |
|
134 |
+ if (item['02:00'] == '-') { |
|
135 |
+ item['02:00'] = item['02:00']; |
|
136 |
+ } else { |
|
137 |
+ item['02:00'] = item['02:00'] + '℃'; |
|
138 |
+ } |
|
139 |
+ if (item['10:00'] == '-') { |
|
140 |
+ item['10:00'] = item['10:00']; |
|
141 |
+ } else { |
|
142 |
+ item['10:00'] = item['10:00'] + '℃'; |
|
143 |
+ } |
|
144 |
+ if (item['14:00'] == '-') { |
|
145 |
+ item['14:00'] = item['14:00']; |
|
146 |
+ } else { |
|
147 |
+ item['14:00'] = item['14:00'] + '℃'; |
|
148 |
+ } |
|
149 |
+ if (item['23:00'] == '-') { |
|
150 |
+ item['23:00'] = item['23:00']; |
|
151 |
+ } else { |
|
152 |
+ item['23:00'] = item['23:00'] + '℃'; |
|
153 |
+ } |
|
154 |
+ } |
|
155 |
+ if (CommonUtil.isEmpty(item['battery']) == false) { |
|
156 |
+ item['battery_check'] = true; |
|
157 |
+ if (item['battery'] == '-') { |
|
158 |
+ item['battery'] = item['battery']; |
|
159 |
+ } else { |
|
160 |
+ item['battery'] = item['battery'] + '%'; |
|
161 |
+ } |
|
162 |
+ } |
|
163 |
+ clickDayData = item; |
|
164 |
+ } |
|
165 |
+ }) |
|
54 | 166 |
return ( |
55 | 167 |
<> |
56 | 168 |
<tr> |
... | ... | @@ -62,15 +174,16 @@ |
62 | 174 |
<th>취침</th> |
63 | 175 |
</tr> |
64 | 176 |
<tr> |
65 |
- <td colSpan={5}>정보가 없습니다.</td> |
|
177 |
+ {clickDayData['medication'] == true ? ( |
|
178 |
+ <> |
|
179 |
+ <td>{clickDayData['DAWN']}</td> |
|
180 |
+ <td>{clickDayData['MORNING']}</td> |
|
181 |
+ <td>{clickDayData['AFTERNOON']}</td> |
|
182 |
+ <td>{clickDayData['NIGHT']}</td> |
|
183 |
+ <td>{clickDayData['MIDNIGHT']}</td> |
|
184 |
+ </> |
|
185 |
+ ) : <td colSpan={5}>데이터가 없습니다.</td>} |
|
66 | 186 |
</tr> |
67 |
- </> |
|
68 |
- ) |
|
69 |
- } |
|
70 |
- |
|
71 |
- const DateTemperature = () => { |
|
72 |
- return ( |
|
73 |
- <> |
|
74 | 187 |
<tr> |
75 | 188 |
<th rowSpan={2}>온도</th> |
76 | 189 |
<th>02:00</th> |
... | ... | @@ -79,30 +192,40 @@ |
79 | 192 |
<th>23:00</th> |
80 | 193 |
</tr> |
81 | 194 |
<tr> |
82 |
- <td colSpan={4}>정보가 없습니다.</td> |
|
195 |
+ {clickDayData['temperature'] == true ? ( |
|
196 |
+ <> |
|
197 |
+ <td>{clickDayData['02:00']}</td> |
|
198 |
+ <td>{clickDayData['10:00']}</td> |
|
199 |
+ <td>{clickDayData['14:00']}</td> |
|
200 |
+ <td>{clickDayData['23:00']}</td> |
|
201 |
+ </> |
|
202 |
+ ) : <td colSpan={4}>데이터가 없습니다.</td>} |
|
203 |
+ </tr> |
|
204 |
+ <tr> |
|
205 |
+ <th>배터리</th> |
|
206 |
+ <td colSpan={5}> |
|
207 |
+ {clickDayData['battery_check'] == true ? ( |
|
208 |
+ clickDayData['battery'] |
|
209 |
+ ) : '데이터가 없습니다.'} |
|
210 |
+ </td> |
|
83 | 211 |
</tr> |
84 | 212 |
</> |
85 | 213 |
) |
86 | 214 |
} |
87 | 215 |
|
88 |
- const DateBattery = () => { |
|
89 |
- return ( |
|
90 |
- <tr> |
|
91 |
- <th>배터리</th> |
|
92 |
- <td colSpan={5}>정보가 없습니다.</td> |
|
93 |
- </tr> |
|
94 |
- ) |
|
95 |
- } |
|
216 |
+ React.useEffect(() => { |
|
217 |
+ equipmentRecordDataSearchList(); |
|
218 |
+ }, []) |
|
96 | 219 |
|
97 | 220 |
|
98 | 221 |
return ( |
99 | 222 |
<> |
100 | 223 |
<main className="pink"> |
101 | 224 |
<div className="flex-start main-guardian"><img src={Senior} alt="" /> |
102 |
- <Title title={equipment['equipment_serial_number']} explanation={"복약, 온도, 배터리 현황을 확인하세요."} /> |
|
225 |
+ <Title title={equipmentSearch['equipment_serial_number']} explanation={"복약, 온도, 배터리 현황을 확인하세요."} /> |
|
103 | 226 |
</div> |
104 | 227 |
|
105 |
- <CalendarAdmin data={{ onClick: calerndarClick }} /> |
|
228 |
+ <CalendarAdmin data={{ equipmentRecordDataList: equipmentRecordDataList, onClick: calerndarClick, onChangeMonth: calerndarChangeMonth }} /> |
|
106 | 229 |
|
107 | 230 |
<div className="content-wrap margin-top"> |
108 | 231 |
<div className="margin-top"> |
... | ... | @@ -128,28 +251,34 @@ |
128 | 251 |
</thead> |
129 | 252 |
|
130 | 253 |
<tbody> |
131 |
- <tr> |
|
132 |
- <td>2023-11-07</td> |
|
133 |
- <td>0</td> |
|
134 |
- <td>1</td> |
|
135 |
- <td>1</td> |
|
136 |
- <td>1</td> |
|
137 |
- <td>0</td> |
|
138 |
- <td>20℃</td> |
|
139 |
- <td>25℃</td> |
|
140 |
- <td>30℃</td> |
|
141 |
- <td>20℃</td> |
|
142 |
- <td>99%</td> |
|
143 |
- </tr> |
|
254 |
+ {CommonUtil.isEmpty(equipmentRecordDataList) == false ? |
|
255 |
+ equipmentRecordDataList.map((item, idx) => { |
|
256 |
+ return ( |
|
257 |
+ <tr> |
|
258 |
+ <td>{item['date']}</td> |
|
259 |
+ <td>{item['DAWN']}</td> |
|
260 |
+ <td>{item['MORNING']}</td> |
|
261 |
+ <td>{item['AFTERNOON']}</td> |
|
262 |
+ <td>{item['NIGHT']}</td> |
|
263 |
+ <td>{item['MIDNIGHT']}</td> |
|
264 |
+ <td>{item['02:00'] != '-' ? item['02:00'] + '℃' : '-'}</td> |
|
265 |
+ <td>{item['10:00'] != '-' ? item['10:00'] + '℃' : '-'}</td> |
|
266 |
+ <td>{item['14:00'] != '-' ? item['14:00'] + '℃' : '-'}</td> |
|
267 |
+ <td>{item['23:00'] != '-' ? item['23:00'] + '℃' : '-'}</td> |
|
268 |
+ <td>{item['battery'] != '-' ? item['battery'] + '%' : '-'}</td> |
|
269 |
+ </tr> |
|
270 |
+ ) |
|
271 |
+ }) |
|
272 |
+ : <tr><td colSpan={11}>조회된 데이터가 없습니다.</td></tr>} |
|
144 | 273 |
</tbody> |
145 | 274 |
</table> |
146 | 275 |
</div> |
147 | 276 |
</div> |
148 | 277 |
|
149 | 278 |
<div className="content-wrap margin-top"> |
150 |
- <DetailTitle contentTitle={"대상자의 복약률을 확인 할 수 있습니다."} /> |
|
279 |
+ <DetailTitle contentTitle={"기기의 복약률을 확인 할 수 있습니다."} /> |
|
151 | 280 |
<div className="main-guardian-chart margin-top"> |
152 |
- <Chart9 /> |
|
281 |
+ <Chart12 data={equipmentRecordDataList} /> |
|
153 | 282 |
</div> |
154 | 283 |
</div> |
155 | 284 |
|
... | ... | @@ -161,9 +290,7 @@ |
161 | 290 |
<div className="modal-visit board-wrap"> |
162 | 291 |
<table className="margin-bottom"> |
163 | 292 |
<tbody> |
164 |
- <DateMedication /> |
|
165 |
- <DateTemperature /> |
|
166 |
- <DateBattery /> |
|
293 |
+ <ModalInner /> |
|
167 | 294 |
</tbody> |
168 | 295 |
</table> |
169 | 296 |
</div> |
--- client/views/pages/equipment/EquipmentSelect.jsx
+++ client/views/pages/equipment/EquipmentSelect.jsx
... | ... | @@ -583,7 +583,7 @@ |
583 | 583 |
<td data-label="상태">{equipmentStates[item['equipment_state']]}</td> |
584 | 584 |
<td data-label="관리"> |
585 | 585 |
<button className={"btn-small gray-btn"} onClick={() => modalEquipmentOpen(item)}>정보 수정</button> |
586 |
- <button className={"btn-small gray-btn"} onClick={() => { navigate("/EquipmentDataSelect", { state: { equipment: item } }) }}>기기데이터 정보 조회</button> |
|
586 |
+ <button className={"btn-small gray-btn"} onClick={() => { navigate("/EquipmentDataSelect", { state: { equipment_serial_number: item['equipment_serial_number'] } }) }}>정보 조회</button> |
|
587 | 587 |
</td> |
588 | 588 |
</tr> |
589 | 589 |
) |
... | ... | @@ -665,9 +665,9 @@ |
665 | 665 |
{isEquipmentDelivery == false ? |
666 | 666 |
<td data-label="관리"> |
667 | 667 |
<button className={"btn-small gray-btn"} onClick={() => modalEquipmentOpen(item)}>정보 수정</button> |
668 |
+ <button className={"btn-small gray-btn"} onClick={() => { navigate("/EquipmentDataSelect", { state: { equipment_serial_number: item['equipment_serial_number'] } }) }}>정보 조회</button> |
|
668 | 669 |
</td> |
669 | 670 |
: null} |
670 |
- |
|
671 | 671 |
</tr> |
672 | 672 |
) |
673 | 673 |
})} |
... | ... | @@ -776,6 +776,7 @@ |
776 | 776 |
</td> |
777 | 777 |
<td data-label="관리"> |
778 | 778 |
<button className={"btn-small gray-btn"} onClick={() => modalEquipmentOpen(item)}>정보 수정</button> |
779 |
+ <button className={"btn-small gray-btn"} onClick={() => { navigate("/EquipmentDataSelect", { state: { equipment_serial_number: item['equipment_serial_number'] } }) }}>정보 조회</button> |
|
779 | 780 |
</td> |
780 | 781 |
</tr> |
781 | 782 |
) |
--- client/views/pages/healthcare/HealthcareSelectOne.jsx
+++ client/views/pages/healthcare/HealthcareSelectOne.jsx
... | ... | @@ -510,7 +510,7 @@ |
510 | 510 |
<div className="flex-start main-guardian"><img src={Senior} alt="" /> |
511 | 511 |
<Title title={`${senior['user_name']} 어르신`} explanation={"방문, 복약, 온도, 배터리 현황을 확인하세요."} /> |
512 | 512 |
</div> |
513 |
- |
|
513 |
+ |
|
514 | 514 |
<div className="btn-wrap flex-end margin-bottom"> |
515 | 515 |
<button className="btn-small gray-btn" onClick={() => { setVisitRecordInit(); openModal(); }}>방문등록</button> |
516 | 516 |
</div> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?