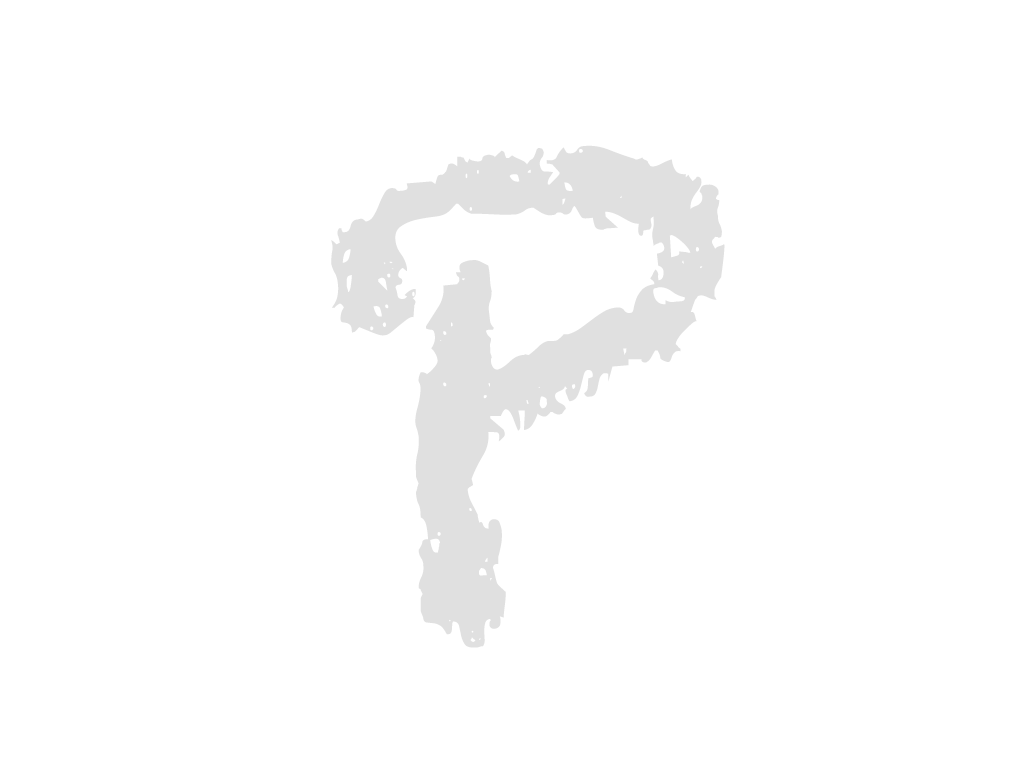
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React from "react";
import { useNavigate, useLocation } from "react-router";
import { useSelector } from "react-redux";
import Title from "../../component/Title.jsx";
import Table from "../../component/Table.jsx";
import Map from "../../component/chart/Map.jsx";
import Chart from "../../component/chart/Chart.jsx";
import Chart5_agency from "../../component/chart/Chart5_agency.jsx";
import Chart2_agency from "../../component/chart/Chart2_agency.jsx";
import AddCircleIcon from "@mui/icons-material/AddCircle";
import Calendar_agency from "../../component/Calendar_agency.jsx";
import Chart10 from "../../component/chart/Chart10.jsx";
import Chart7 from "../../component/chart/Chart7.jsx";
import Chart8 from "../../component/chart/Chart8.jsx";
import tool from "../../../resources/files/images/tool.png";
import medicinebox from "../../../resources/files/images/medicinebox.png";
import box from "../../../resources/files/images/box.png";
import exchange from "../../../resources/files/images/exchange.png";
import error from "../../../resources/files/images/error.png";
import batteryAgency from "../../../resources/files/images/batteryAgency.png";
import medicineAgency from "../../../resources/files/images/medicineAgency.png";
import temperatureAgency from "../../../resources/files/images/temperatureAgency.png";
import wifioff from "../../../resources/files/images/wifioff.png";
import TitleSmall from "../../component/TitleSmall.jsx";
import CommonUtil from "../../../resources/js/CommonUtil.js";
export default function Main2() {
const navigate = useNavigate();
const location = useLocation();
//전역 변수 저장 객체
const state = useSelector((state) => { return state });
const thead = ["No", "계약업체명", "구분", "담당자 연락처", "주소"];
const key = ["No", "agency", "division", "phone", "address",];
const content = [
{
No: (
<p><span>{thead[0]}</span> 1948.11.15</p>
),
agency: (
<p><span>{thead[1]}</span> A복지관</p>
),
division: (
<p><span>{thead[2]}</span>교환</p>
),
phone: (
<p><span>{thead[3]}</span>010-1234-5678</p>
),
address: (
<p><span>{thead[4]}</span>경상북도 군위군 삼국유사면</p>
),
},
];
// 내 시니어
const [mySenior, setMySenior] = React.useState({
seniorList: [],
seniorListCount: 0,
search: {
'agent_id': state.loginUser['user_id'],
'user_use': true,
}
});
// 내 시니어 목록 조회
const [seniorGenderData, setSeniorGenderData] = React.useState([]);
const mySeniorSelectList = () => {
fetch("/user/seniorSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(mySenior.search),
}).then((response) => response.json()).then((data) => {
data.search = mySenior.search;
console.log("내 시니어 목록 조회 : ", data);
setMySenior(data);
let seniorGenderArr = [];
const today = new Date();
data['seniorList'].map((item, idx) => {
const birth = new Date(item['user_birth']);
let age = today.getFullYear() - birth.getFullYear() + 1;
seniorGenderArr.push({ "gender": item['user_gender'], "age": age })
})
console.log("seniorGenderArr: ", seniorGenderArr)
let genderData = [];
for (let i = 0; i < 18; i++) {
let age = i * 5;
let age1 = ((i + 1) * 5) - 1;
if (i == 17) {
genderData.push({ "age": age + '+', "male": 0, "female": 0 })
} else {
genderData.push({ "age": age + '-' + age1, "male": 0, "female": 0 })
}
}
console.log("genderData: ", genderData)
seniorGenderArr.map((item, idx) => {
for (let i = 0; i < 18; i++) {
if (item['age'] >= i * 5 && item['age'] <= ((i + 1) * 5) - 1) {
if (item['gender'] == "남") {
genderData[i]['male']--
} else if (item['gender'] == "여") {
genderData[i]['female']++
}
}
}
})
setSeniorGenderData(genderData)
console.log("genderData: ", genderData)
}).catch((error) => {
console.log('seniorSelectList() /user/seniorSelectList.json error : ', error);
});
}
//온도 위험 대상자(시니어) 수 조회
const [temperatureCount, setTemperatureCount] = React.useState(0);
const temperatureRiskCount = () => {
fetch("/stats/agentTemperatureRisk.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({
'agent_id': state.loginUser['user_id'],
}),
}).then((response) => response.json()).then((data) => {
console.log("온도 위험 대상자(시니어) 수 조회 : ", data);
setTemperatureCount(data);
}).catch((error) => {
console.log('temperatureRiskCount() /stats/agentTemperatureRisk.json error : ', error);
});
}
//배터리 부족 대상자(시니어) 수 조회
const [batteryCount, setbatteryCount] = React.useState(0);
const batteryRiskCount = () => {
fetch("/stats/agentBatteryRisk.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({
'agent_id': state.loginUser['user_id'],
}),
}).then((response) => response.json()).then((data) => {
console.log("배터리 부족 대상자(시니어) 수 조회 : ", data);
setbatteryCount(data);
}).catch((error) => {
console.log('batteryRiskCount() /stats/agentBatteryRisk.json error : ', error);
});
}
// 최근 복약률
const [medicationSelectListByNew, setMedicationSelectListByNew] = React.useState([]);
// 최근 복약률 조회
const seniorMedicationSelectListByNew = () => {
fetch("/user/seniorMedicationSelectListByNew.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({ "agent_id": state.loginUser['user_id'] }),
}).then((response) => response.json()).then((data) => {
console.log("seniorMedicationSelectListByNew : ", data);
setMedicationSelectListByNew(data)
}).catch((error) => {
console.log('seniorMedicationSelectListByNew() /user/seniorMedicationSelectListByNew.json error : ', error);
});
};
// 복약률 목록
const [medicationSelectListByMonth, setMedicationSelectListByMonth] = React.useState([]);
const [medicationSelectListByYear, setMedicationSelectListByYear] = React.useState([]);
// 복약률 목록 조회
const seniorMedicationSelectListByMonth = () => {
let totalYearArr = [];
let yearArr = [];
fetch("/user/seniorMedicationSelectListByMonth.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({ "agency_id": state.loginUser['agency_id'] }),
}).then((response) => response.json()).then((data) => {
data.map((item, idx) => {
item['year'] = item['medication_default_date'].substr(0, 4);
item['month'] = item['medication_default_date'].substr(5, 2);
totalYearArr.push(item['medication_default_date'].substr(0, 4));
item['date'] = new Date(item['medication_default_date']).getTime();
})
const setYearArr = [...new Set(totalYearArr)];
console.log("totalYearArr: ", setYearArr);
setYearArr.map((year, yearIdx) => {
let sum = 0;
let count = 0;
let avg = 0;
let monthArr = ['-', '-', '-', '-', '-', '-', '-', '-', '-', '-', '-', '-'];
data.map((item, idx) => {
if (item['year'] == year) {
sum += item['average'];
count++;
monthArr[Number(item['month']) - 1] = item['average'];
}
})
avg = parseInt(sum / count);
yearArr.push({ "year": [year], "average": avg, "data": monthArr });
})
console.log("yearArr : ", yearArr);
setMedicationSelectListByYear(yearArr);
console.log("seniorMedicationSelectListByMonth : ", data);
setMedicationSelectListByMonth(data);
}).catch((error) => {
console.log('seniorMedicationSelectListByMonth() /user/seniorMedicationSelectListByMonth.json error : ', error);
});
};
React.useEffect(() => {
temperatureRiskCount();
batteryRiskCount();
seniorMedicationSelectListByNew();
seniorMedicationSelectListByMonth();
mySeniorSelectList();
}, []);
return (
<main>
<div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"대상자 현황"} explanation={new Date(new Date().setDate(new Date().getDate() - 1)).toLocaleDateString().replace(/\./g, '').replace(/\s/g, '-') + " 기준"} /></div>
<div className="main-grid-agency margin-bottom2">
<ul className="content-box statistics-agency" background="#8ef3d1">
<li className="flex-start" onClick={() => { navigate("/Healthcare") }}>
<img src={medicineAgency} alt="" />
<div className="text">
<p>미복약 위험 대상자</p>
<p className="peoplecount">0</p>
</div>
</li>
</ul>
<ul className="content-box statistics-agency" background="#ebe7b9" >
<li className="flex-start" onClick={() => { navigate("/Healthcare") }}>
<img src={temperatureAgency} alt="" />
<div className="text">
<p>댁내 온도 위험 대상자</p>
<p className="peoplecount">{temperatureCount}</p>
</div>
</li>
</ul>
<ul className="content-box statistics-agency" background="#5f9af3">
<li className="flex-start" onClick={() => { navigate("/Healthcare") }}>
<img src={batteryAgency} alt="" />
<div className="text">
<p>배터리 부족 대상자</p>
<p className="peoplecount">{batteryCount}</p>
</div>
</li>
</ul>
<ul className="content-box statistics-agency" background="#8080FF">
<li className="flex-start" onClick={() => { navigate("/Healthcare") }}>
<img src={wifioff} alt="" />
<div className="text">
<p>데이터 미확인 대상자</p>
<p className="peoplecount">{batteryCount}</p>
</div>
</li>
</ul>
</div>
<div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"대상자 복약률 평균"} /></div>
<div className="main-grid-agency margin-bottom2">
<div className="content-box combine-left-government4">
<div className="height-50">
{
medicationSelectListByNew.length > 0 ?
<Chart10 data={medicationSelectListByMonth} />
: <div className="no-data"><p>데이터가 없습니다.</p></div>
}
</div>
<div>
<table className="visit-data-table">
<thead>
<tr>
<th>년도</th>
<th>총계</th>
<th>1월</th>
<th>2월</th>
<th>3월</th>
<th>4월</th>
<th>5월</th>
<th>6월</th>
<th>7월</th>
<th>8월</th>
<th>9월</th>
<th>10월</th>
<th>11월</th>
<th>12월</th>
</tr>
</thead>
<tbody>
{medicationSelectListByYear.length > 0 ? (
medicationSelectListByYear.map((item, idx) => {
return (
<tr>
<td>{item['year']}</td>
<td>{item['average'] == '-' ? '-' : item['average'] + '%'}</td>
{CommonUtil.isEmpty(item['data']) == false ? (
item['data'].map((item1, idx1) => {
return (
<>
<td>{item1 == '-' ? '-' : item1 + '%'}</td>
</>
)
})
) : null}
</tr>
)
})
) :
<tr>
<td colSpan={14}>데이터가 없습니다.</td>
</tr>
}
</tbody>
</table>
</div>
</div>
</div>
{/* <div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"방문/전화 관리 리스트"} /></div>
<div className="main-grid-agency margin-bottom2">
<div className="content-box combine-left-government3 visitlist">
<div className="margin-bottom2">
<Calendar_agency />
</div>
<div className="margin-bottom2">
<Table className="agency-visitlist" head={thead} contents={content} contentKey={key} />
</div>
</div>
</div> */}
<div className="main-grid-agency2">
<div>
<div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"시니어 복약수 순위"} /></div>
<div className="content-box combine-left-government3 visitlist margin-bottom2">
{
medicationSelectListByNew.length > 0 ?
<Chart8 data={medicationSelectListByNew} />
: <div className="no-data"><p>데이터가 없습니다.</p></div>
}
</div>
</div>
<div>
<div className="flex-start margin-bottom2"><img src={medicinebox} alt="" /><TitleSmall title={"연령대별 통계"} /></div>
<div className="content-box combine-left-government3 visitlist margin-bottom2">
{
seniorGenderData.length > 0 ?
<Chart7 data={seniorGenderData} />
: <div className="no-data"><p>데이터가 없습니다.</p></div>
}
</div>
</div>
</div>
<div>
</div>
</main>
);
}