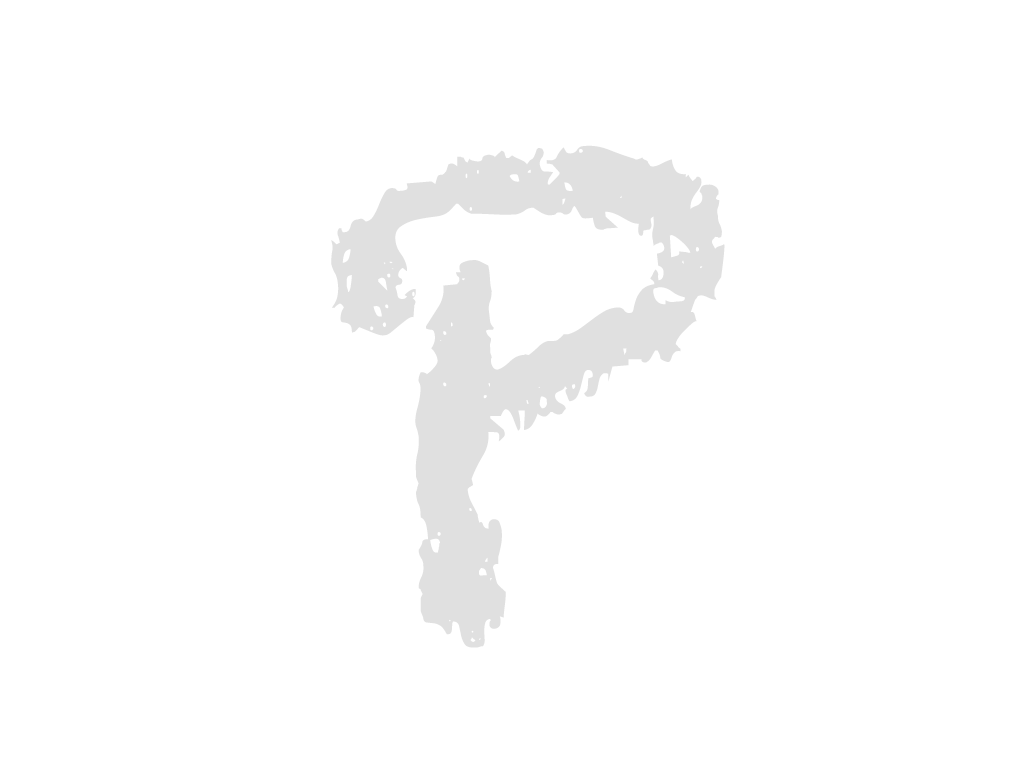
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
{"version":3,"names":["getBindingIdentifiers","_getBindingIdentifiers","getOuterBindingIdentifiers","_getOuterBindingIdentifiers","isDeclaration","numericLiteral","unaryExpression","NORMAL_COMPLETION","BREAK_COMPLETION","NormalCompletion","path","type","BreakCompletion","getOpposite","key","getSibling","addCompletionRecords","records","context","push","_getCompletionRecords","completionRecordForSwitch","cases","lastNormalCompletions","i","length","casePath","caseCompletions","normalCompletions","breakCompletions","c","normalCompletionToBreak","completions","forEach","replaceBreakStatementInBreakCompletion","reachable","isBreakStatement","label","replaceWith","remove","getStatementListCompletion","paths","canHaveBreak","newContext","inCaseClause","isBlockStatement","shouldPopulateBreak","statementCompletions","every","some","pathCompletions","isVariableDeclaration","isIfStatement","get","isDoExpression","isFor","isWhile","isLabeledStatement","isProgram","isFunction","isTryStatement","isCatchClause","isSwitchStatement","isSwitchCase","getCompletionRecords","map","r","NodePath","parentPath","parent","container","listKey","setContext","getPrevSibling","getNextSibling","getAllNextSiblings","_key","sibling","siblings","node","getAllPrevSiblings","parts","split","_getKey","_getPattern","Array","isArray","_","part","duplicates","getBindingIdentifierPaths","outerOnly","search","ids","Object","create","id","shift","keys","isIdentifier","_ids","name","isExportDeclaration","declaration","isFunctionDeclaration","isFunctionExpression","child","getOuterBindingIdentifierPaths"],"sources":["../../src/path/family.ts"],"sourcesContent":["// This file contains methods responsible for dealing with/retrieving children or siblings.\n\nimport type TraversalContext from \"../context\";\nimport NodePath from \"./index\";\nimport {\n getBindingIdentifiers as _getBindingIdentifiers,\n getOuterBindingIdentifiers as _getOuterBindingIdentifiers,\n isDeclaration,\n numericLiteral,\n unaryExpression,\n} from \"@babel/types\";\nimport type * as t from \"@babel/types\";\n\nconst NORMAL_COMPLETION = 0 as const;\nconst BREAK_COMPLETION = 1 as const;\n\ntype Completion = {\n path: NodePath;\n type: 0 | 1;\n};\n\ntype CompletionContext = {\n // whether the current context allows `break` statement. When it allows, we have\n // to search all the statements for potential `break`\n canHaveBreak: boolean;\n // whether the statement is an immediate descendant of a switch case clause\n inCaseClause: boolean;\n // whether the `break` statement record should be populated to upper level\n // when a `break` statement is an immediate descendant of a block statement, e.g.\n // `{ break }`, it can influence the control flow in the upper levels.\n shouldPopulateBreak: boolean;\n};\n\nfunction NormalCompletion(path: NodePath) {\n return { type: NORMAL_COMPLETION, path };\n}\n\nfunction BreakCompletion(path: NodePath) {\n return { type: BREAK_COMPLETION, path };\n}\n\nexport function getOpposite(this: NodePath): NodePath | null {\n if (this.key === \"left\") {\n return this.getSibling(\"right\");\n } else if (this.key === \"right\") {\n return this.getSibling(\"left\");\n }\n return null;\n}\n\nfunction addCompletionRecords(\n path: NodePath | null | undefined,\n records: Completion[],\n context: CompletionContext,\n): Completion[] {\n if (path) {\n records.push(..._getCompletionRecords(path, context));\n }\n return records;\n}\n\nfunction completionRecordForSwitch(\n cases: NodePath<t.SwitchCase>[],\n records: Completion[],\n context: CompletionContext,\n): Completion[] {\n // https://tc39.es/ecma262/#sec-runtime-semantics-caseblockevaluation\n let lastNormalCompletions: Completion[] = [];\n for (let i = 0; i < cases.length; i++) {\n const casePath = cases[i];\n const caseCompletions = _getCompletionRecords(casePath, context);\n const normalCompletions = [];\n const breakCompletions = [];\n for (const c of caseCompletions) {\n if (c.type === NORMAL_COMPLETION) {\n normalCompletions.push(c);\n }\n if (c.type === BREAK_COMPLETION) {\n breakCompletions.push(c);\n }\n }\n if (normalCompletions.length) {\n lastNormalCompletions = normalCompletions;\n }\n records.push(...breakCompletions);\n }\n records.push(...lastNormalCompletions);\n return records;\n}\n\nfunction normalCompletionToBreak(completions: Completion[]) {\n completions.forEach(c => {\n c.type = BREAK_COMPLETION;\n });\n}\n\n/**\n * Determine how we should handle the break statement for break completions\n *\n * @param {Completion[]} completions\n * @param {boolean} reachable Whether the break statement is reachable after\n we mark the normal completions _before_ the given break completions as the final\n completions. For example,\n `{ 0 }; break;` is transformed to `{ return 0 }; break;`, the `break` here is unreachable\n and thus can be removed without consequences. We may in the future reserve them instead since\n we do not consistently remove unreachable statements _after_ break\n `{ var x = 0 }; break;` is transformed to `{ var x = 0 }; return void 0;`, the `break` is reachable\n because we can not wrap variable declaration under a return statement\n */\nfunction replaceBreakStatementInBreakCompletion(\n completions: Completion[],\n reachable: boolean,\n) {\n completions.forEach(c => {\n if (c.path.isBreakStatement({ label: null })) {\n if (reachable) {\n c.path.replaceWith(unaryExpression(\"void\", numericLiteral(0)));\n } else {\n c.path.remove();\n }\n }\n });\n}\n\nfunction getStatementListCompletion(\n paths: NodePath[],\n context: CompletionContext,\n): Completion[] {\n const completions = [];\n if (context.canHaveBreak) {\n let lastNormalCompletions = [];\n for (let i = 0; i < paths.length; i++) {\n const path = paths[i];\n const newContext = { ...context, inCaseClause: false };\n if (\n path.isBlockStatement() &&\n (context.inCaseClause || // case test: { break }\n context.shouldPopulateBreak) // case test: { { break } }\n ) {\n newContext.shouldPopulateBreak = true;\n } else {\n newContext.shouldPopulateBreak = false;\n }\n const statementCompletions = _getCompletionRecords(path, newContext);\n if (\n statementCompletions.length > 0 &&\n // we can stop search `paths` when we have seen a `path` that is\n // effectively a `break` statement. Examples are\n // - `break`\n // - `if (true) { 1; break } else { 2; break }`\n // - `{ break }```\n // In other words, the paths after this `path` are unreachable\n statementCompletions.every(c => c.type === BREAK_COMPLETION)\n ) {\n if (\n lastNormalCompletions.length > 0 &&\n statementCompletions.every(c =>\n c.path.isBreakStatement({ label: null }),\n )\n ) {\n // when a break completion has a path as BreakStatement, it must be `{ break }`\n // whose completion value we can not determine, otherwise it would have been\n // replaced by `replaceBreakStatementInBreakCompletion`\n // When we have seen normal completions from the last statement\n // it is safe to stop populating break and mark normal completions as break\n normalCompletionToBreak(lastNormalCompletions);\n completions.push(...lastNormalCompletions);\n // Declarations have empty completion record, however they can not be nested\n // directly in return statement, i.e. `return (var a = 1)` is invalid.\n if (lastNormalCompletions.some(c => c.path.isDeclaration())) {\n completions.push(...statementCompletions);\n replaceBreakStatementInBreakCompletion(\n statementCompletions,\n /* reachable */ true,\n );\n }\n replaceBreakStatementInBreakCompletion(\n statementCompletions,\n /* reachable */ false,\n );\n } else {\n completions.push(...statementCompletions);\n if (!context.shouldPopulateBreak) {\n replaceBreakStatementInBreakCompletion(\n statementCompletions,\n /* reachable */ true,\n );\n }\n }\n break;\n }\n if (i === paths.length - 1) {\n completions.push(...statementCompletions);\n } else {\n lastNormalCompletions = [];\n for (let i = 0; i < statementCompletions.length; i++) {\n const c = statementCompletions[i];\n if (c.type === BREAK_COMPLETION) {\n completions.push(c);\n }\n if (c.type === NORMAL_COMPLETION) {\n lastNormalCompletions.push(c);\n }\n }\n }\n }\n } else if (paths.length) {\n // When we are in a context where `break` must not exist, we can skip linear\n // search on statement lists and assume that the last\n // non-variable-declaration statement determines the completion.\n for (let i = paths.length - 1; i >= 0; i--) {\n const pathCompletions = _getCompletionRecords(paths[i], context);\n if (\n pathCompletions.length > 1 ||\n (pathCompletions.length === 1 &&\n !pathCompletions[0].path.isVariableDeclaration())\n ) {\n completions.push(...pathCompletions);\n break;\n }\n }\n }\n return completions;\n}\n\nfunction _getCompletionRecords(\n path: NodePath,\n context: CompletionContext,\n): Completion[] {\n let records: Completion[] = [];\n if (path.isIfStatement()) {\n records = addCompletionRecords(path.get(\"consequent\"), records, context);\n records = addCompletionRecords(path.get(\"alternate\"), records, context);\n } else if (\n path.isDoExpression() ||\n path.isFor() ||\n path.isWhile() ||\n path.isLabeledStatement()\n ) {\n // @ts-expect-error(flow->ts): todo\n return addCompletionRecords(path.get(\"body\"), records, context);\n } else if (path.isProgram() || path.isBlockStatement()) {\n // @ts-expect-error(flow->ts): todo\n return getStatementListCompletion(path.get(\"body\"), context);\n } else if (path.isFunction()) {\n return _getCompletionRecords(path.get(\"body\"), context);\n } else if (path.isTryStatement()) {\n records = addCompletionRecords(path.get(\"block\"), records, context);\n records = addCompletionRecords(path.get(\"handler\"), records, context);\n } else if (path.isCatchClause()) {\n return addCompletionRecords(path.get(\"body\"), records, context);\n } else if (path.isSwitchStatement()) {\n return completionRecordForSwitch(path.get(\"cases\"), records, context);\n } else if (path.isSwitchCase()) {\n return getStatementListCompletion(path.get(\"consequent\"), {\n canHaveBreak: true,\n shouldPopulateBreak: false,\n inCaseClause: true,\n });\n } else if (path.isBreakStatement()) {\n records.push(BreakCompletion(path));\n } else {\n records.push(NormalCompletion(path));\n }\n\n return records;\n}\n\n/**\n * Retrieve the completion records of a given path.\n * Note: to ensure proper support on `break` statement, this method\n * will manipulate the AST around the break statement. Do not call the method\n * twice for the same path.\n *\n * @export\n * @param {NodePath} this\n * @returns {NodePath[]} Completion records\n */\nexport function getCompletionRecords(this: NodePath): NodePath[] {\n const records = _getCompletionRecords(this, {\n canHaveBreak: false,\n shouldPopulateBreak: false,\n inCaseClause: false,\n });\n return records.map(r => r.path);\n}\n\nexport function getSibling(this: NodePath, key: string | number): NodePath {\n return NodePath.get({\n parentPath: this.parentPath,\n parent: this.parent,\n container: this.container,\n listKey: this.listKey,\n key: key,\n }).setContext(this.context);\n}\n\nexport function getPrevSibling(this: NodePath): NodePath {\n // @ts-expect-error todo(flow->ts) this.key could be a string\n return this.getSibling(this.key - 1);\n}\n\nexport function getNextSibling(this: NodePath): NodePath {\n // @ts-expect-error todo(flow->ts) this.key could be a string\n return this.getSibling(this.key + 1);\n}\n\nexport function getAllNextSiblings(this: NodePath): NodePath[] {\n // @ts-expect-error todo(flow->ts) this.key could be a string\n let _key: number = this.key;\n let sibling = this.getSibling(++_key);\n const siblings = [];\n while (sibling.node) {\n siblings.push(sibling);\n sibling = this.getSibling(++_key);\n }\n return siblings;\n}\n\nexport function getAllPrevSiblings(this: NodePath): NodePath[] {\n // @ts-expect-error todo(flow->ts) this.key could be a string\n let _key: number = this.key;\n let sibling = this.getSibling(--_key);\n const siblings = [];\n while (sibling.node) {\n siblings.push(sibling);\n sibling = this.getSibling(--_key);\n }\n return siblings;\n}\n\n// convert \"1\" to 1 (string index to number index)\ntype MaybeToIndex<T extends string> = T extends `${bigint}` ? number : T;\n\ntype Pattern<Obj extends string, Prop extends string> = `${Obj}.${Prop}`;\n\n// split \"body.body.1\" to [\"body\", \"body\", 1]\ntype Split<P extends string> = P extends Pattern<infer O, infer U>\n ? [MaybeToIndex<O>, ...Split<U>]\n : [MaybeToIndex<P>];\n\n// get all K with Node[K] is t.Node | t.Node[]\ntype NodeKeyOf<Node extends t.Node | t.Node[]> = keyof Pick<\n Node,\n {\n [Key in keyof Node]-?: Node[Key] extends t.Node | t.Node[] ? Key : never;\n }[keyof Node]\n>;\n\n// traverse the Node with tuple path [\"body\", \"body\", 1]\n// Path should be created with Split\ntype Trav<\n Node extends t.Node | t.Node[],\n Path extends unknown[],\n> = Path extends [infer K, ...infer R]\n ? K extends NodeKeyOf<Node>\n ? R extends []\n ? Node[K]\n : // @ts-expect-error ignore since TS is not smart enough\n Trav<Node[K], R>\n : never\n : never;\n\ntype ToNodePath<T> = T extends Array<t.Node | null | undefined>\n ? Array<NodePath<T[number]>>\n : T extends t.Node | null | undefined\n ? NodePath<T>\n : never;\n\nfunction get<T extends t.Node, K extends keyof T>(\n this: NodePath<T>,\n key: K,\n context?: boolean | TraversalContext,\n): T[K] extends Array<t.Node | null | undefined>\n ? Array<NodePath<T[K][number]>>\n : T[K] extends t.Node | null | undefined\n ? NodePath<T[K]>\n : never;\n\nfunction get<T extends t.Node, K extends string>(\n this: NodePath<T>,\n key: K,\n context?: boolean | TraversalContext,\n): ToNodePath<Trav<T, Split<K>>>;\n\nfunction get<T extends t.Node>(\n this: NodePath<T>,\n key: string,\n context?: true | TraversalContext,\n): NodePath | NodePath[];\n\nfunction get<T extends t.Node>(\n this: NodePath<T>,\n key: string,\n context: true | TraversalContext = true,\n): NodePath | NodePath[] {\n if (context === true) context = this.context;\n const parts = key.split(\".\");\n if (parts.length === 1) {\n // \"foo\"\n // @ts-expect-error key may not index T\n return this._getKey(key, context);\n } else {\n // \"foo.bar\"\n return this._getPattern(parts, context);\n }\n}\n\nexport { get };\n\nexport function _getKey<T extends t.Node>(\n this: NodePath<T>,\n key: keyof T & string,\n context?: TraversalContext,\n): NodePath | NodePath[] {\n const node = this.node;\n const container = node[key];\n\n if (Array.isArray(container)) {\n // requested a container so give them all the paths\n return container.map((_, i) => {\n return NodePath.get({\n listKey: key,\n parentPath: this,\n parent: node,\n container: container,\n key: i,\n }).setContext(context);\n });\n } else {\n return NodePath.get({\n parentPath: this,\n parent: node,\n container: node,\n key: key,\n }).setContext(context);\n }\n}\n\nexport function _getPattern(\n this: NodePath,\n parts: string[],\n context?: TraversalContext,\n): NodePath | NodePath[] {\n let path: NodePath | NodePath[] = this;\n for (const part of parts) {\n if (part === \".\") {\n // @ts-expect-error todo(flow-ts): Can path be an array here?\n path = path.parentPath;\n } else {\n if (Array.isArray(path)) {\n // @ts-expect-error part may not index path\n path = path[part];\n } else {\n path = path.get(part, context);\n }\n }\n }\n return path;\n}\n\nfunction getBindingIdentifiers(\n duplicates: true,\n): Record<string, t.Identifier[]>;\nfunction getBindingIdentifiers(\n duplicates?: false,\n): Record<string, t.Identifier>;\nfunction getBindingIdentifiers(\n duplicates: boolean,\n): Record<string, t.Identifier[] | t.Identifier>;\n\nfunction getBindingIdentifiers(\n this: NodePath,\n duplicates?: boolean,\n): Record<string, t.Identifier[] | t.Identifier> {\n return _getBindingIdentifiers(this.node, duplicates);\n}\n\nexport { getBindingIdentifiers };\n\nfunction getOuterBindingIdentifiers(\n duplicates: true,\n): Record<string, t.Identifier[]>;\nfunction getOuterBindingIdentifiers(\n duplicates?: false,\n): Record<string, t.Identifier>;\nfunction getOuterBindingIdentifiers(\n duplicates: boolean,\n): Record<string, t.Identifier[] | t.Identifier>;\n\nfunction getOuterBindingIdentifiers(\n this: NodePath,\n duplicates?: boolean,\n): Record<string, t.Identifier[] | t.Identifier> {\n return _getOuterBindingIdentifiers(this.node, duplicates);\n}\n\nexport { getOuterBindingIdentifiers };\n\nfunction getBindingIdentifierPaths(\n duplicates: true,\n outerOnly?: boolean,\n): Record<string, NodePath<t.Identifier>[]>;\nfunction getBindingIdentifierPaths(\n duplicates: false,\n outerOnly?: boolean,\n): Record<string, NodePath<t.Identifier>>;\nfunction getBindingIdentifierPaths(\n duplicates?: boolean,\n outerOnly?: boolean,\n): Record<string, NodePath<t.Identifier> | NodePath<t.Identifier>[]>;\n\n// original source - https://github.com/babel/babel/blob/main/packages/babel-types/src/retrievers/getBindingIdentifiers.js\n// path.getBindingIdentifiers returns nodes where the following re-implementation returns paths\nfunction getBindingIdentifierPaths(\n this: NodePath,\n duplicates: boolean = false,\n outerOnly: boolean = false,\n): Record<string, NodePath<t.Identifier> | NodePath<t.Identifier>[]> {\n const path = this;\n const search = [path];\n const ids = Object.create(null);\n\n while (search.length) {\n const id = search.shift();\n if (!id) continue;\n if (!id.node) continue;\n\n const keys =\n // @ts-expect-error _getBindingIdentifiers.keys do not cover all node types\n _getBindingIdentifiers.keys[id.node.type];\n\n if (id.isIdentifier()) {\n if (duplicates) {\n const _ids = (ids[id.node.name] = ids[id.node.name] || []);\n _ids.push(id);\n } else {\n ids[id.node.name] = id;\n }\n continue;\n }\n\n if (id.isExportDeclaration()) {\n const declaration = id.get(\"declaration\");\n if (isDeclaration(declaration)) {\n search.push(declaration);\n }\n continue;\n }\n\n if (outerOnly) {\n if (id.isFunctionDeclaration()) {\n search.push(id.get(\"id\"));\n continue;\n }\n if (id.isFunctionExpression()) {\n continue;\n }\n }\n\n if (keys) {\n for (let i = 0; i < keys.length; i++) {\n const key = keys[i];\n const child = id.get(key);\n if (Array.isArray(child)) {\n search.push(...child);\n } else if (child.node) {\n search.push(child);\n }\n }\n }\n }\n\n return ids;\n}\n\nexport { getBindingIdentifierPaths };\n\nfunction getOuterBindingIdentifierPaths(\n duplicates: true,\n): Record<string, NodePath<t.Identifier>[]>;\nfunction getOuterBindingIdentifierPaths(\n duplicates?: false,\n): Record<string, NodePath<t.Identifier>>;\nfunction getOuterBindingIdentifierPaths(\n duplicates?: boolean,\n): Record<string, NodePath<t.Identifier> | NodePath<t.Identifier>[]>;\n\nfunction getOuterBindingIdentifierPaths(\n this: NodePath,\n duplicates: boolean = false,\n) {\n return this.getBindingIdentifierPaths(duplicates, true);\n}\n\nexport { getOuterBindingIdentifierPaths };\n"],"mappings":";;;;;;;;;;;;;;;;;;;;AAGA;;AACA;;;EACEA,qB,EAAyBC,sB;EACzBC,0B,EAA8BC,2B;EAC9BC,a;EACAC,c;EACAC;;AAIF,MAAMC,iBAAiB,GAAG,CAA1B;AACA,MAAMC,gBAAgB,GAAG,CAAzB;;AAmBA,SAASC,gBAAT,CAA0BC,IAA1B,EAA0C;EACxC,OAAO;IAAEC,IAAI,EAAEJ,iBAAR;IAA2BG;EAA3B,CAAP;AACD;;AAED,SAASE,eAAT,CAAyBF,IAAzB,EAAyC;EACvC,OAAO;IAAEC,IAAI,EAAEH,gBAAR;IAA0BE;EAA1B,CAAP;AACD;;AAEM,SAASG,WAAT,GAAsD;EAC3D,IAAI,KAAKC,GAAL,KAAa,MAAjB,EAAyB;IACvB,OAAO,KAAKC,UAAL,CAAgB,OAAhB,CAAP;EACD,CAFD,MAEO,IAAI,KAAKD,GAAL,KAAa,OAAjB,EAA0B;IAC/B,OAAO,KAAKC,UAAL,CAAgB,MAAhB,CAAP;EACD;;EACD,OAAO,IAAP;AACD;;AAED,SAASC,oBAAT,CACEN,IADF,EAEEO,OAFF,EAGEC,OAHF,EAIgB;EACd,IAAIR,IAAJ,EAAU;IACRO,OAAO,CAACE,IAAR,CAAa,GAAGC,qBAAqB,CAACV,IAAD,EAAOQ,OAAP,CAArC;EACD;;EACD,OAAOD,OAAP;AACD;;AAED,SAASI,yBAAT,CACEC,KADF,EAEEL,OAFF,EAGEC,OAHF,EAIgB;EAEd,IAAIK,qBAAmC,GAAG,EAA1C;;EACA,KAAK,IAAIC,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGF,KAAK,CAACG,MAA1B,EAAkCD,CAAC,EAAnC,EAAuC;IACrC,MAAME,QAAQ,GAAGJ,KAAK,CAACE,CAAD,CAAtB;;IACA,MAAMG,eAAe,GAAGP,qBAAqB,CAACM,QAAD,EAAWR,OAAX,CAA7C;;IACA,MAAMU,iBAAiB,GAAG,EAA1B;IACA,MAAMC,gBAAgB,GAAG,EAAzB;;IACA,KAAK,MAAMC,CAAX,IAAgBH,eAAhB,EAAiC;MAC/B,IAAIG,CAAC,CAACnB,IAAF,KAAWJ,iBAAf,EAAkC;QAChCqB,iBAAiB,CAACT,IAAlB,CAAuBW,CAAvB;MACD;;MACD,IAAIA,CAAC,CAACnB,IAAF,KAAWH,gBAAf,EAAiC;QAC/BqB,gBAAgB,CAACV,IAAjB,CAAsBW,CAAtB;MACD;IACF;;IACD,IAAIF,iBAAiB,CAACH,MAAtB,EAA8B;MAC5BF,qBAAqB,GAAGK,iBAAxB;IACD;;IACDX,OAAO,CAACE,IAAR,CAAa,GAAGU,gBAAhB;EACD;;EACDZ,OAAO,CAACE,IAAR,CAAa,GAAGI,qBAAhB;EACA,OAAON,OAAP;AACD;;AAED,SAASc,uBAAT,CAAiCC,WAAjC,EAA4D;EAC1DA,WAAW,CAACC,OAAZ,CAAoBH,CAAC,IAAI;IACvBA,CAAC,CAACnB,IAAF,GAASH,gBAAT;EACD,CAFD;AAGD;;AAeD,SAAS0B,sCAAT,CACEF,WADF,EAEEG,SAFF,EAGE;EACAH,WAAW,CAACC,OAAZ,CAAoBH,CAAC,IAAI;IACvB,IAAIA,CAAC,CAACpB,IAAF,CAAO0B,gBAAP,CAAwB;MAAEC,KAAK,EAAE;IAAT,CAAxB,CAAJ,EAA8C;MAC5C,IAAIF,SAAJ,EAAe;QACbL,CAAC,CAACpB,IAAF,CAAO4B,WAAP,CAAmBhC,eAAe,CAAC,MAAD,EAASD,cAAc,CAAC,CAAD,CAAvB,CAAlC;MACD,CAFD,MAEO;QACLyB,CAAC,CAACpB,IAAF,CAAO6B,MAAP;MACD;IACF;EACF,CARD;AASD;;AAED,SAASC,0BAAT,CACEC,KADF,EAEEvB,OAFF,EAGgB;EACd,MAAMc,WAAW,GAAG,EAApB;;EACA,IAAId,OAAO,CAACwB,YAAZ,EAA0B;IACxB,IAAInB,qBAAqB,GAAG,EAA5B;;IACA,KAAK,IAAIC,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGiB,KAAK,CAAChB,MAA1B,EAAkCD,CAAC,EAAnC,EAAuC;MACrC,MAAMd,IAAI,GAAG+B,KAAK,CAACjB,CAAD,CAAlB;MACA,MAAMmB,UAAU,qBAAQzB,OAAR;QAAiB0B,YAAY,EAAE;MAA/B,EAAhB;;MACA,IACElC,IAAI,CAACmC,gBAAL,OACC3B,OAAO,CAAC0B,YAAR,IACC1B,OAAO,CAAC4B,mBAFV,CADF,EAIE;QACAH,UAAU,CAACG,mBAAX,GAAiC,IAAjC;MACD,CAND,MAMO;QACLH,UAAU,CAACG,mBAAX,GAAiC,KAAjC;MACD;;MACD,MAAMC,oBAAoB,GAAG3B,qBAAqB,CAACV,IAAD,EAAOiC,UAAP,CAAlD;;MACA,IACEI,oBAAoB,CAACtB,MAArB,GAA8B,CAA9B,IAOAsB,oBAAoB,CAACC,KAArB,CAA2BlB,CAAC,IAAIA,CAAC,CAACnB,IAAF,KAAWH,gBAA3C,CARF,EASE;QACA,IACEe,qBAAqB,CAACE,MAAtB,GAA+B,CAA/B,IACAsB,oBAAoB,CAACC,KAArB,CAA2BlB,CAAC,IAC1BA,CAAC,CAACpB,IAAF,CAAO0B,gBAAP,CAAwB;UAAEC,KAAK,EAAE;QAAT,CAAxB,CADF,CAFF,EAKE;UAMAN,uBAAuB,CAACR,qBAAD,CAAvB;UACAS,WAAW,CAACb,IAAZ,CAAiB,GAAGI,qBAApB;;UAGA,IAAIA,qBAAqB,CAAC0B,IAAtB,CAA2BnB,CAAC,IAAIA,CAAC,CAACpB,IAAF,CAAON,aAAP,EAAhC,CAAJ,EAA6D;YAC3D4B,WAAW,CAACb,IAAZ,CAAiB,GAAG4B,oBAApB;YACAb,sCAAsC,CACpCa,oBADoC,EAEpB,IAFoB,CAAtC;UAID;;UACDb,sCAAsC,CACpCa,oBADoC,EAEpB,KAFoB,CAAtC;QAID,CA1BD,MA0BO;UACLf,WAAW,CAACb,IAAZ,CAAiB,GAAG4B,oBAApB;;UACA,IAAI,CAAC7B,OAAO,CAAC4B,mBAAb,EAAkC;YAChCZ,sCAAsC,CACpCa,oBADoC,EAEpB,IAFoB,CAAtC;UAID;QACF;;QACD;MACD;;MACD,IAAIvB,CAAC,KAAKiB,KAAK,CAAChB,MAAN,GAAe,CAAzB,EAA4B;QAC1BO,WAAW,CAACb,IAAZ,CAAiB,GAAG4B,oBAApB;MACD,CAFD,MAEO;QACLxB,qBAAqB,GAAG,EAAxB;;QACA,KAAK,IAAIC,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAGuB,oBAAoB,CAACtB,MAAzC,EAAiDD,CAAC,EAAlD,EAAsD;UACpD,MAAMM,CAAC,GAAGiB,oBAAoB,CAACvB,CAAD,CAA9B;;UACA,IAAIM,CAAC,CAACnB,IAAF,KAAWH,gBAAf,EAAiC;YAC/BwB,WAAW,CAACb,IAAZ,CAAiBW,CAAjB;UACD;;UACD,IAAIA,CAAC,CAACnB,IAAF,KAAWJ,iBAAf,EAAkC;YAChCgB,qBAAqB,CAACJ,IAAtB,CAA2BW,CAA3B;UACD;QACF;MACF;IACF;EACF,CA7ED,MA6EO,IAAIW,KAAK,CAAChB,MAAV,EAAkB;IAIvB,KAAK,IAAID,CAAC,GAAGiB,KAAK,CAAChB,MAAN,GAAe,CAA5B,EAA+BD,CAAC,IAAI,CAApC,EAAuCA,CAAC,EAAxC,EAA4C;MAC1C,MAAM0B,eAAe,GAAG9B,qBAAqB,CAACqB,KAAK,CAACjB,CAAD,CAAN,EAAWN,OAAX,CAA7C;;MACA,IACEgC,eAAe,CAACzB,MAAhB,GAAyB,CAAzB,IACCyB,eAAe,CAACzB,MAAhB,KAA2B,CAA3B,IACC,CAACyB,eAAe,CAAC,CAAD,CAAf,CAAmBxC,IAAnB,CAAwByC,qBAAxB,EAHL,EAIE;QACAnB,WAAW,CAACb,IAAZ,CAAiB,GAAG+B,eAApB;QACA;MACD;IACF;EACF;;EACD,OAAOlB,WAAP;AACD;;AAED,SAASZ,qBAAT,CACEV,IADF,EAEEQ,OAFF,EAGgB;EACd,IAAID,OAAqB,GAAG,EAA5B;;EACA,IAAIP,IAAI,CAAC0C,aAAL,EAAJ,EAA0B;IACxBnC,OAAO,GAAGD,oBAAoB,CAACN,IAAI,CAAC2C,GAAL,CAAS,YAAT,CAAD,EAAyBpC,OAAzB,EAAkCC,OAAlC,CAA9B;IACAD,OAAO,GAAGD,oBAAoB,CAACN,IAAI,CAAC2C,GAAL,CAAS,WAAT,CAAD,EAAwBpC,OAAxB,EAAiCC,OAAjC,CAA9B;EACD,CAHD,MAGO,IACLR,IAAI,CAAC4C,cAAL,MACA5C,IAAI,CAAC6C,KAAL,EADA,IAEA7C,IAAI,CAAC8C,OAAL,EAFA,IAGA9C,IAAI,CAAC+C,kBAAL,EAJK,EAKL;IAEA,OAAOzC,oBAAoB,CAACN,IAAI,CAAC2C,GAAL,CAAS,MAAT,CAAD,EAAmBpC,OAAnB,EAA4BC,OAA5B,CAA3B;EACD,CARM,MAQA,IAAIR,IAAI,CAACgD,SAAL,MAAoBhD,IAAI,CAACmC,gBAAL,EAAxB,EAAiD;IAEtD,OAAOL,0BAA0B,CAAC9B,IAAI,CAAC2C,GAAL,CAAS,MAAT,CAAD,EAAmBnC,OAAnB,CAAjC;EACD,CAHM,MAGA,IAAIR,IAAI,CAACiD,UAAL,EAAJ,EAAuB;IAC5B,OAAOvC,qBAAqB,CAACV,IAAI,CAAC2C,GAAL,CAAS,MAAT,CAAD,EAAmBnC,OAAnB,CAA5B;EACD,CAFM,MAEA,IAAIR,IAAI,CAACkD,cAAL,EAAJ,EAA2B;IAChC3C,OAAO,GAAGD,oBAAoB,CAACN,IAAI,CAAC2C,GAAL,CAAS,OAAT,CAAD,EAAoBpC,OAApB,EAA6BC,OAA7B,CAA9B;IACAD,OAAO,GAAGD,oBAAoB,CAACN,IAAI,CAAC2C,GAAL,CAAS,SAAT,CAAD,EAAsBpC,OAAtB,EAA+BC,OAA/B,CAA9B;EACD,CAHM,MAGA,IAAIR,IAAI,CAACmD,aAAL,EAAJ,EAA0B;IAC/B,OAAO7C,oBAAoB,CAACN,IAAI,CAAC2C,GAAL,CAAS,MAAT,CAAD,EAAmBpC,OAAnB,EAA4BC,OAA5B,CAA3B;EACD,CAFM,MAEA,IAAIR,IAAI,CAACoD,iBAAL,EAAJ,EAA8B;IACnC,OAAOzC,yBAAyB,CAACX,IAAI,CAAC2C,GAAL,CAAS,OAAT,CAAD,EAAoBpC,OAApB,EAA6BC,OAA7B,CAAhC;EACD,CAFM,MAEA,IAAIR,IAAI,CAACqD,YAAL,EAAJ,EAAyB;IAC9B,OAAOvB,0BAA0B,CAAC9B,IAAI,CAAC2C,GAAL,CAAS,YAAT,CAAD,EAAyB;MACxDX,YAAY,EAAE,IAD0C;MAExDI,mBAAmB,EAAE,KAFmC;MAGxDF,YAAY,EAAE;IAH0C,CAAzB,CAAjC;EAKD,CANM,MAMA,IAAIlC,IAAI,CAAC0B,gBAAL,EAAJ,EAA6B;IAClCnB,OAAO,CAACE,IAAR,CAAaP,eAAe,CAACF,IAAD,CAA5B;EACD,CAFM,MAEA;IACLO,OAAO,CAACE,IAAR,CAAaV,gBAAgB,CAACC,IAAD,CAA7B;EACD;;EAED,OAAOO,OAAP;AACD;;AAYM,SAAS+C,oBAAT,GAA0D;EAC/D,MAAM/C,OAAO,GAAGG,qBAAqB,CAAC,IAAD,EAAO;IAC1CsB,YAAY,EAAE,KAD4B;IAE1CI,mBAAmB,EAAE,KAFqB;IAG1CF,YAAY,EAAE;EAH4B,CAAP,CAArC;;EAKA,OAAO3B,OAAO,CAACgD,GAAR,CAAYC,CAAC,IAAIA,CAAC,CAACxD,IAAnB,CAAP;AACD;;AAEM,SAASK,UAAT,CAAoCD,GAApC,EAAoE;EACzE,OAAOqD,cAAA,CAASd,GAAT,CAAa;IAClBe,UAAU,EAAE,KAAKA,UADC;IAElBC,MAAM,EAAE,KAAKA,MAFK;IAGlBC,SAAS,EAAE,KAAKA,SAHE;IAIlBC,OAAO,EAAE,KAAKA,OAJI;IAKlBzD,GAAG,EAAEA;EALa,CAAb,EAMJ0D,UANI,CAMO,KAAKtD,OANZ,CAAP;AAOD;;AAEM,SAASuD,cAAT,GAAkD;EAEvD,OAAO,KAAK1D,UAAL,CAAgB,KAAKD,GAAL,GAAW,CAA3B,CAAP;AACD;;AAEM,SAAS4D,cAAT,GAAkD;EAEvD,OAAO,KAAK3D,UAAL,CAAgB,KAAKD,GAAL,GAAW,CAA3B,CAAP;AACD;;AAEM,SAAS6D,kBAAT,GAAwD;EAE7D,IAAIC,IAAY,GAAG,KAAK9D,GAAxB;EACA,IAAI+D,OAAO,GAAG,KAAK9D,UAAL,CAAgB,EAAE6D,IAAlB,CAAd;EACA,MAAME,QAAQ,GAAG,EAAjB;;EACA,OAAOD,OAAO,CAACE,IAAf,EAAqB;IACnBD,QAAQ,CAAC3D,IAAT,CAAc0D,OAAd;IACAA,OAAO,GAAG,KAAK9D,UAAL,CAAgB,EAAE6D,IAAlB,CAAV;EACD;;EACD,OAAOE,QAAP;AACD;;AAEM,SAASE,kBAAT,GAAwD;EAE7D,IAAIJ,IAAY,GAAG,KAAK9D,GAAxB;EACA,IAAI+D,OAAO,GAAG,KAAK9D,UAAL,CAAgB,EAAE6D,IAAlB,CAAd;EACA,MAAME,QAAQ,GAAG,EAAjB;;EACA,OAAOD,OAAO,CAACE,IAAf,EAAqB;IACnBD,QAAQ,CAAC3D,IAAT,CAAc0D,OAAd;IACAA,OAAO,GAAG,KAAK9D,UAAL,CAAgB,EAAE6D,IAAlB,CAAV;EACD;;EACD,OAAOE,QAAP;AACD;;AA8DD,SAASzB,GAAT,CAEEvC,GAFF,EAGEI,OAAgC,GAAG,IAHrC,EAIyB;EACvB,IAAIA,OAAO,KAAK,IAAhB,EAAsBA,OAAO,GAAG,KAAKA,OAAf;EACtB,MAAM+D,KAAK,GAAGnE,GAAG,CAACoE,KAAJ,CAAU,GAAV,CAAd;;EACA,IAAID,KAAK,CAACxD,MAAN,KAAiB,CAArB,EAAwB;IAGtB,OAAO,KAAK0D,OAAL,CAAarE,GAAb,EAAkBI,OAAlB,CAAP;EACD,CAJD,MAIO;IAEL,OAAO,KAAKkE,WAAL,CAAiBH,KAAjB,EAAwB/D,OAAxB,CAAP;EACD;AACF;;AAIM,SAASiE,OAAT,CAELrE,GAFK,EAGLI,OAHK,EAIkB;EACvB,MAAM6D,IAAI,GAAG,KAAKA,IAAlB;EACA,MAAMT,SAAS,GAAGS,IAAI,CAACjE,GAAD,CAAtB;;EAEA,IAAIuE,KAAK,CAACC,OAAN,CAAchB,SAAd,CAAJ,EAA8B;IAE5B,OAAOA,SAAS,CAACL,GAAV,CAAc,CAACsB,CAAD,EAAI/D,CAAJ,KAAU;MAC7B,OAAO2C,cAAA,CAASd,GAAT,CAAa;QAClBkB,OAAO,EAAEzD,GADS;QAElBsD,UAAU,EAAE,IAFM;QAGlBC,MAAM,EAAEU,IAHU;QAIlBT,SAAS,EAAEA,SAJO;QAKlBxD,GAAG,EAAEU;MALa,CAAb,EAMJgD,UANI,CAMOtD,OANP,CAAP;IAOD,CARM,CAAP;EASD,CAXD,MAWO;IACL,OAAOiD,cAAA,CAASd,GAAT,CAAa;MAClBe,UAAU,EAAE,IADM;MAElBC,MAAM,EAAEU,IAFU;MAGlBT,SAAS,EAAES,IAHO;MAIlBjE,GAAG,EAAEA;IAJa,CAAb,EAKJ0D,UALI,CAKOtD,OALP,CAAP;EAMD;AACF;;AAEM,SAASkE,WAAT,CAELH,KAFK,EAGL/D,OAHK,EAIkB;EACvB,IAAIR,IAA2B,GAAG,IAAlC;;EACA,KAAK,MAAM8E,IAAX,IAAmBP,KAAnB,EAA0B;IACxB,IAAIO,IAAI,KAAK,GAAb,EAAkB;MAEhB9E,IAAI,GAAGA,IAAI,CAAC0D,UAAZ;IACD,CAHD,MAGO;MACL,IAAIiB,KAAK,CAACC,OAAN,CAAc5E,IAAd,CAAJ,EAAyB;QAEvBA,IAAI,GAAGA,IAAI,CAAC8E,IAAD,CAAX;MACD,CAHD,MAGO;QACL9E,IAAI,GAAGA,IAAI,CAAC2C,GAAL,CAASmC,IAAT,EAAetE,OAAf,CAAP;MACD;IACF;EACF;;EACD,OAAOR,IAAP;AACD;;AAYD,SAASV,qBAAT,CAEEyF,UAFF,EAGiD;EAC/C,OAAOxF,sBAAsB,CAAC,KAAK8E,IAAN,EAAYU,UAAZ,CAA7B;AACD;;AAcD,SAASvF,0BAAT,CAEEuF,UAFF,EAGiD;EAC/C,OAAOtF,2BAA2B,CAAC,KAAK4E,IAAN,EAAYU,UAAZ,CAAlC;AACD;;AAmBD,SAASC,yBAAT,CAEED,UAAmB,GAAG,KAFxB,EAGEE,SAAkB,GAAG,KAHvB,EAIqE;EACnE,MAAMjF,IAAI,GAAG,IAAb;EACA,MAAMkF,MAAM,GAAG,CAAClF,IAAD,CAAf;EACA,MAAMmF,GAAG,GAAGC,MAAM,CAACC,MAAP,CAAc,IAAd,CAAZ;;EAEA,OAAOH,MAAM,CAACnE,MAAd,EAAsB;IACpB,MAAMuE,EAAE,GAAGJ,MAAM,CAACK,KAAP,EAAX;IACA,IAAI,CAACD,EAAL,EAAS;IACT,IAAI,CAACA,EAAE,CAACjB,IAAR,EAAc;IAEd,MAAMmB,IAAI,GAERjG,sBAAsB,CAACiG,IAAvB,CAA4BF,EAAE,CAACjB,IAAH,CAAQpE,IAApC,CAFF;;IAIA,IAAIqF,EAAE,CAACG,YAAH,EAAJ,EAAuB;MACrB,IAAIV,UAAJ,EAAgB;QACd,MAAMW,IAAI,GAAIP,GAAG,CAACG,EAAE,CAACjB,IAAH,CAAQsB,IAAT,CAAH,GAAoBR,GAAG,CAACG,EAAE,CAACjB,IAAH,CAAQsB,IAAT,CAAH,IAAqB,EAAvD;;QACAD,IAAI,CAACjF,IAAL,CAAU6E,EAAV;MACD,CAHD,MAGO;QACLH,GAAG,CAACG,EAAE,CAACjB,IAAH,CAAQsB,IAAT,CAAH,GAAoBL,EAApB;MACD;;MACD;IACD;;IAED,IAAIA,EAAE,CAACM,mBAAH,EAAJ,EAA8B;MAC5B,MAAMC,WAAW,GAAGP,EAAE,CAAC3C,GAAH,CAAO,aAAP,CAApB;;MACA,IAAIjD,aAAa,CAACmG,WAAD,CAAjB,EAAgC;QAC9BX,MAAM,CAACzE,IAAP,CAAYoF,WAAZ;MACD;;MACD;IACD;;IAED,IAAIZ,SAAJ,EAAe;MACb,IAAIK,EAAE,CAACQ,qBAAH,EAAJ,EAAgC;QAC9BZ,MAAM,CAACzE,IAAP,CAAY6E,EAAE,CAAC3C,GAAH,CAAO,IAAP,CAAZ;QACA;MACD;;MACD,IAAI2C,EAAE,CAACS,oBAAH,EAAJ,EAA+B;QAC7B;MACD;IACF;;IAED,IAAIP,IAAJ,EAAU;MACR,KAAK,IAAI1E,CAAC,GAAG,CAAb,EAAgBA,CAAC,GAAG0E,IAAI,CAACzE,MAAzB,EAAiCD,CAAC,EAAlC,EAAsC;QACpC,MAAMV,GAAG,GAAGoF,IAAI,CAAC1E,CAAD,CAAhB;QACA,MAAMkF,KAAK,GAAGV,EAAE,CAAC3C,GAAH,CAAOvC,GAAP,CAAd;;QACA,IAAIuE,KAAK,CAACC,OAAN,CAAcoB,KAAd,CAAJ,EAA0B;UACxBd,MAAM,CAACzE,IAAP,CAAY,GAAGuF,KAAf;QACD,CAFD,MAEO,IAAIA,KAAK,CAAC3B,IAAV,EAAgB;UACrBa,MAAM,CAACzE,IAAP,CAAYuF,KAAZ;QACD;MACF;IACF;EACF;;EAED,OAAOb,GAAP;AACD;;AAcD,SAASc,8BAAT,CAEElB,UAAmB,GAAG,KAFxB,EAGE;EACA,OAAO,KAAKC,yBAAL,CAA+BD,UAA/B,EAA2C,IAA3C,CAAP;AACD"}