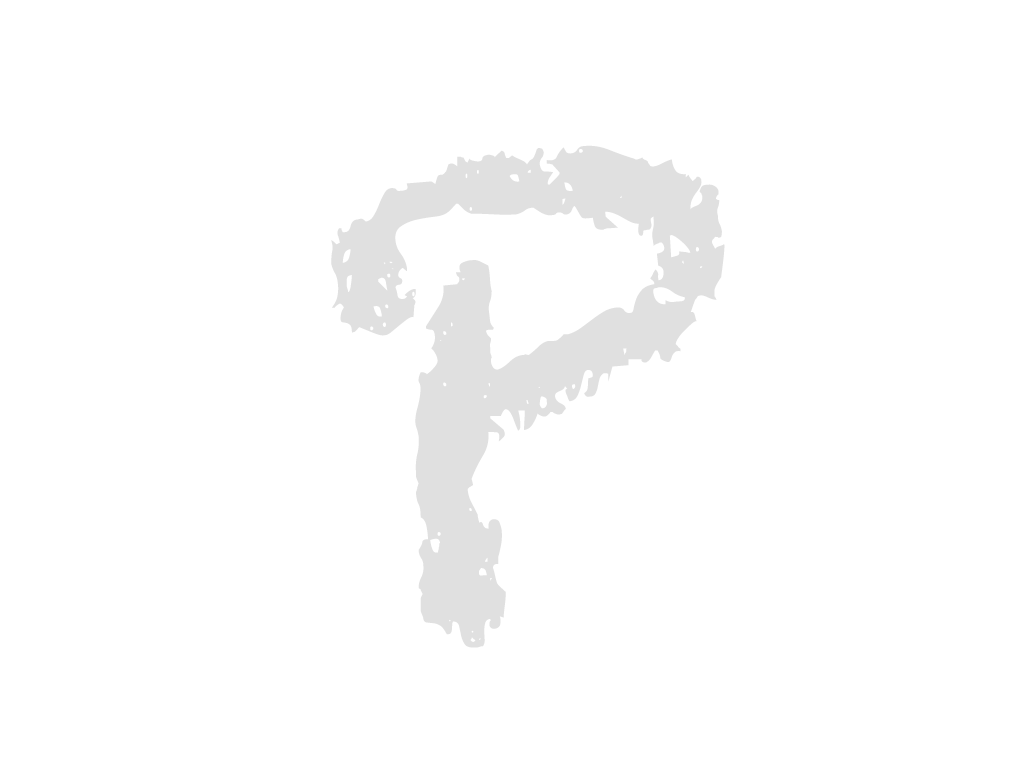
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
{"version":3,"names":["arrowFunctionExpression","assignmentExpression","binaryExpression","blockStatement","callExpression","conditionalExpression","expressionStatement","identifier","isIdentifier","jsxIdentifier","logicalExpression","LOGICAL_OPERATORS","memberExpression","metaProperty","numericLiteral","objectExpression","restElement","returnStatement","sequenceExpression","spreadElement","stringLiteral","super","_super","thisExpression","toExpression","unaryExpression","toComputedKey","key","isMemberExpression","node","property","isProperty","isMethod","ReferenceError","computed","name","ensureBlock","body","get","bodyNode","Array","isArray","Error","isBlockStatement","statements","stringPath","listKey","isStatement","push","isFunction","parentPath","setup","arrowFunctionToShadowed","isArrowFunctionExpression","arrowFunctionToExpression","unwrapFunctionEnvironment","isFunctionExpression","isFunctionDeclaration","buildCodeFrameError","hoistFunctionEnvironment","setType","path","type","allowInsertArrow","specCompliant","noNewArrows","thisBinding","fnPath","fn","checkBinding","scope","generateUidIdentifier","id","init","unshiftContainer","hub","addHelper","replaceWith","nameFunction","getSuperCallsVisitor","mergeVisitors","CallExpression","child","allSuperCalls","isSuper","environmentVisitor","arrowParent","thisEnvFn","findParent","p","isProgram","isClassProperty","static","isClassPrivateProperty","inConstructor","isClassMethod","kind","thisPaths","argumentsPaths","newTargetPaths","superProps","superCalls","getScopeInformation","length","traverse","superBinding","getSuperBinding","forEach","superCall","callee","loc","argumentsBinding","getBinding","args","buildUndefinedNode","argumentsChild","argsRef","newTargetBinding","targetChild","targetRef","flatSuperProps","reduce","acc","superProp","concat","standardizeSuperProperty","superParentPath","isAssignment","isAssignmentExpression","left","isCall","isCallExpression","getSuperPropBinding","value","right","call","getThisBinding","hasSuperClass","thisChild","thisRef","isJSX","isLogicalOp","op","includes","operator","assignmentPath","slice","isLogicalAssignment","tmp","generateDeclaredUidIdentifier","object","rightExpression","isUpdateExpression","updateExpr","computedKey","parts","prefix","superClass","assignSuperThisVisitor","supers","has","add","replaceWithMultiple","WeakSet","argsBinding","propName","argsList","fnBody","method","unshift","valueIdent","cacheKey","data","getData","setData","getScopeInformationVisitor","ThisExpression","JSXIdentifier","isJSXMemberExpression","isJSXOpeningElement","MemberExpression","Identifier","isReferencedIdentifier","curr","hasOwnBinding","rename","parent","MetaProperty"],"sources":["../../src/path/conversion.ts"],"sourcesContent":["// This file contains methods that convert the path node into another node or some other type of data.\n\nimport {\n arrowFunctionExpression,\n assignmentExpression,\n binaryExpression,\n blockStatement,\n callExpression,\n conditionalExpression,\n expressionStatement,\n identifier,\n isIdentifier,\n jsxIdentifier,\n logicalExpression,\n LOGICAL_OPERATORS,\n memberExpression,\n metaProperty,\n numericLiteral,\n objectExpression,\n restElement,\n returnStatement,\n sequenceExpression,\n spreadElement,\n stringLiteral,\n super as _super,\n thisExpression,\n toExpression,\n unaryExpression,\n} from \"@babel/types\";\nimport type * as t from \"@babel/types\";\nimport environmentVisitor from \"@babel/helper-environment-visitor\";\nimport nameFunction from \"@babel/helper-function-name\";\nimport { merge as mergeVisitors } from \"../visitors\";\nimport type NodePath from \"./index\";\n\nexport function toComputedKey(this: NodePath) {\n let key;\n if (this.isMemberExpression()) {\n key = this.node.property;\n } else if (this.isProperty() || this.isMethod()) {\n key = this.node.key;\n } else {\n throw new ReferenceError(\"todo\");\n }\n\n // @ts-expect-error todo(flow->ts) computed does not exist in ClassPrivateProperty\n if (!this.node.computed) {\n if (isIdentifier(key)) key = stringLiteral(key.name);\n }\n\n return key;\n}\n\nexport function ensureBlock(\n this: NodePath<\n t.Loop | t.WithStatement | t.Function | t.LabeledStatement | t.CatchClause\n >,\n) {\n const body = this.get(\"body\");\n const bodyNode = body.node;\n\n if (Array.isArray(body)) {\n throw new Error(\"Can't convert array path to a block statement\");\n }\n if (!bodyNode) {\n throw new Error(\"Can't convert node without a body\");\n }\n\n if (body.isBlockStatement()) {\n return bodyNode;\n }\n\n const statements: Array<t.Statement> = [];\n\n let stringPath = \"body\";\n let key;\n let listKey;\n if (body.isStatement()) {\n listKey = \"body\";\n key = 0;\n statements.push(body.node);\n } else {\n stringPath += \".body.0\";\n if (this.isFunction()) {\n key = \"argument\";\n statements.push(returnStatement(body.node as t.Expression));\n } else {\n key = \"expression\";\n statements.push(expressionStatement(body.node as t.Expression));\n }\n }\n\n this.node.body = blockStatement(statements);\n const parentPath = this.get(stringPath) as NodePath;\n body.setup(\n parentPath,\n listKey\n ? // @ts-expect-error listKey must present in parent path\n parentPath.node[listKey]\n : parentPath.node,\n listKey,\n key,\n );\n\n return this.node;\n}\n\n/**\n * Keeping this for backward-compatibility. You should use arrowFunctionToExpression() for >=7.x.\n */\n// TODO(Babel 8): Remove this\nexport function arrowFunctionToShadowed(this: NodePath) {\n if (!this.isArrowFunctionExpression()) return;\n\n this.arrowFunctionToExpression();\n}\n\n/**\n * Given an arbitrary function, process its content as if it were an arrow function, moving references\n * to \"this\", \"arguments\", \"super\", and such into the function's parent scope. This method is useful if\n * you have wrapped some set of items in an IIFE or other function, but want \"this\", \"arguments\", and super\"\n * to continue behaving as expected.\n */\nexport function unwrapFunctionEnvironment(this: NodePath) {\n if (\n !this.isArrowFunctionExpression() &&\n !this.isFunctionExpression() &&\n !this.isFunctionDeclaration()\n ) {\n throw this.buildCodeFrameError(\n \"Can only unwrap the environment of a function.\",\n );\n }\n\n hoistFunctionEnvironment(this);\n}\n\nfunction setType<N extends t.Node, T extends N[\"type\"]>(\n path: NodePath<N>,\n type: T,\n): asserts path is NodePath<Extract<N, { type: T }>> {\n path.node.type = type;\n}\n\n/**\n * Convert a given arrow function into a normal ES5 function expression.\n */\nexport function arrowFunctionToExpression(\n this: NodePath<t.ArrowFunctionExpression>,\n {\n allowInsertArrow = true,\n /** @deprecated Use `noNewArrows` instead */\n specCompliant = false,\n // TODO(Babel 8): Consider defaulting to `false` for spec compliancy\n noNewArrows = !specCompliant,\n }: {\n allowInsertArrow?: boolean | void;\n specCompliant?: boolean | void;\n noNewArrows?: boolean;\n } = {},\n): NodePath<Exclude<t.Function, t.Method | t.ArrowFunctionExpression>> {\n if (!this.isArrowFunctionExpression()) {\n throw (this as NodePath).buildCodeFrameError(\n \"Cannot convert non-arrow function to a function expression.\",\n );\n }\n\n const { thisBinding, fnPath: fn } = hoistFunctionEnvironment(\n this,\n noNewArrows,\n allowInsertArrow,\n );\n\n // @ts-expect-error TS requires explicit fn type annotation\n fn.ensureBlock();\n setType(fn, \"FunctionExpression\");\n\n if (!noNewArrows) {\n const checkBinding = thisBinding\n ? null\n : fn.scope.generateUidIdentifier(\"arrowCheckId\");\n if (checkBinding) {\n fn.parentPath.scope.push({\n id: checkBinding,\n init: objectExpression([]),\n });\n }\n\n fn.get(\"body\").unshiftContainer(\n \"body\",\n expressionStatement(\n callExpression(this.hub.addHelper(\"newArrowCheck\"), [\n thisExpression(),\n checkBinding\n ? identifier(checkBinding.name)\n : identifier(thisBinding),\n ]),\n ),\n );\n\n fn.replaceWith(\n callExpression(\n memberExpression(\n // @ts-expect-error TS can't infer nameFunction returns CallExpression | ArrowFunctionExpression here\n nameFunction(this, true) || fn.node,\n identifier(\"bind\"),\n ),\n [checkBinding ? identifier(checkBinding.name) : thisExpression()],\n ),\n );\n\n return fn.get(\"callee.object\");\n }\n\n return fn;\n}\n\nconst getSuperCallsVisitor = mergeVisitors<{\n allSuperCalls: NodePath<t.CallExpression>[];\n}>([\n {\n CallExpression(child, { allSuperCalls }) {\n if (!child.get(\"callee\").isSuper()) return;\n allSuperCalls.push(child);\n },\n },\n environmentVisitor,\n]);\n\n/**\n * Given a function, traverse its contents, and if there are references to \"this\", \"arguments\", \"super\",\n * or \"new.target\", ensure that these references reference the parent environment around this function.\n *\n * @returns `thisBinding`: the name of the injected reference to `this`; for example \"_this\"\n * @returns `fnPath`: the new path to the function node. This is different from the fnPath\n * parameter when the function node is wrapped in another node.\n */\nfunction hoistFunctionEnvironment(\n fnPath: NodePath<t.Function>,\n // TODO(Babel 8): Consider defaulting to `false` for spec compliancy\n noNewArrows: boolean | void = true,\n allowInsertArrow: boolean | void = true,\n): { thisBinding: string; fnPath: NodePath<t.Function> } {\n let arrowParent;\n let thisEnvFn: NodePath<t.Function> = fnPath.findParent(p => {\n if (p.isArrowFunctionExpression()) {\n arrowParent ??= p;\n return false;\n }\n return (\n p.isFunction() ||\n p.isProgram() ||\n p.isClassProperty({ static: false }) ||\n p.isClassPrivateProperty({ static: false })\n );\n }) as NodePath<t.Function>;\n const inConstructor = thisEnvFn.isClassMethod({ kind: \"constructor\" });\n\n if (thisEnvFn.isClassProperty() || thisEnvFn.isClassPrivateProperty()) {\n if (arrowParent) {\n thisEnvFn = arrowParent;\n } else if (allowInsertArrow) {\n // It's safe to wrap this function in another and not hoist to the\n // top level because the 'this' binding is constant in class\n // properties (since 'super()' has already been called), so we don't\n // need to capture/reassign it at the top level.\n fnPath.replaceWith(\n callExpression(\n arrowFunctionExpression([], toExpression(fnPath.node)),\n [],\n ),\n );\n thisEnvFn = fnPath.get(\"callee\") as NodePath<t.ArrowFunctionExpression>;\n fnPath = thisEnvFn.get(\"body\") as NodePath<t.FunctionExpression>;\n } else {\n throw fnPath.buildCodeFrameError(\n \"Unable to transform arrow inside class property\",\n );\n }\n }\n\n const { thisPaths, argumentsPaths, newTargetPaths, superProps, superCalls } =\n getScopeInformation(fnPath);\n\n // Convert all super() calls in the constructor, if super is used in an arrow.\n if (inConstructor && superCalls.length > 0) {\n if (!allowInsertArrow) {\n throw superCalls[0].buildCodeFrameError(\n \"Unable to handle nested super() usage in arrow\",\n );\n }\n const allSuperCalls: NodePath<t.CallExpression>[] = [];\n thisEnvFn.traverse(getSuperCallsVisitor, { allSuperCalls });\n const superBinding = getSuperBinding(thisEnvFn);\n allSuperCalls.forEach(superCall => {\n const callee = identifier(superBinding);\n callee.loc = superCall.node.callee.loc;\n\n superCall.get(\"callee\").replaceWith(callee);\n });\n }\n\n // Convert all \"arguments\" references in the arrow to point at the alias.\n if (argumentsPaths.length > 0) {\n const argumentsBinding = getBinding(thisEnvFn, \"arguments\", () => {\n const args = () => identifier(\"arguments\");\n if (thisEnvFn.scope.path.isProgram()) {\n return conditionalExpression(\n binaryExpression(\n \"===\",\n unaryExpression(\"typeof\", args()),\n stringLiteral(\"undefined\"),\n ),\n thisEnvFn.scope.buildUndefinedNode(),\n args(),\n );\n } else {\n return args();\n }\n });\n\n argumentsPaths.forEach(argumentsChild => {\n const argsRef = identifier(argumentsBinding);\n argsRef.loc = argumentsChild.node.loc;\n\n argumentsChild.replaceWith(argsRef);\n });\n }\n\n // Convert all \"new.target\" references in the arrow to point at the alias.\n if (newTargetPaths.length > 0) {\n const newTargetBinding = getBinding(thisEnvFn, \"newtarget\", () =>\n metaProperty(identifier(\"new\"), identifier(\"target\")),\n );\n\n newTargetPaths.forEach(targetChild => {\n const targetRef = identifier(newTargetBinding);\n targetRef.loc = targetChild.node.loc;\n\n targetChild.replaceWith(targetRef);\n });\n }\n\n // Convert all \"super.prop\" references to point at aliases.\n if (superProps.length > 0) {\n if (!allowInsertArrow) {\n throw superProps[0].buildCodeFrameError(\n \"Unable to handle nested super.prop usage\",\n );\n }\n\n const flatSuperProps: NodePath<t.MemberExpression>[] = superProps.reduce(\n (acc, superProp) => acc.concat(standardizeSuperProperty(superProp)),\n [],\n );\n\n flatSuperProps.forEach(superProp => {\n const key = superProp.node.computed\n ? \"\"\n : // @ts-expect-error super property must not contain private name\n superProp.get(\"property\").node.name;\n\n const superParentPath = superProp.parentPath;\n\n const isAssignment = superParentPath.isAssignmentExpression({\n left: superProp.node,\n });\n const isCall = superParentPath.isCallExpression({\n callee: superProp.node,\n });\n const superBinding = getSuperPropBinding(thisEnvFn, isAssignment, key);\n\n const args: t.Expression[] = [];\n if (superProp.node.computed) {\n // SuperProperty must not be a private name\n args.push(superProp.get(\"property\").node as t.Expression);\n }\n\n if (isAssignment) {\n const value = superParentPath.node.right;\n args.push(value);\n }\n\n const call = callExpression(identifier(superBinding), args);\n\n if (isCall) {\n superParentPath.unshiftContainer(\"arguments\", thisExpression());\n superProp.replaceWith(memberExpression(call, identifier(\"call\")));\n\n thisPaths.push(\n superParentPath.get(\"arguments.0\") as NodePath<t.ThisExpression>,\n );\n } else if (isAssignment) {\n // Replace not only the super.prop, but the whole assignment\n superParentPath.replaceWith(call);\n } else {\n superProp.replaceWith(call);\n }\n });\n }\n\n // Convert all \"this\" references in the arrow to point at the alias.\n let thisBinding: string | null;\n if (thisPaths.length > 0 || !noNewArrows) {\n thisBinding = getThisBinding(thisEnvFn, inConstructor);\n\n if (\n noNewArrows ||\n // In subclass constructors, still need to rewrite because \"this\" can't be bound in spec mode\n // because it might not have been initialized yet.\n (inConstructor && hasSuperClass(thisEnvFn))\n ) {\n thisPaths.forEach(thisChild => {\n const thisRef = thisChild.isJSX()\n ? jsxIdentifier(thisBinding)\n : identifier(thisBinding);\n\n thisRef.loc = thisChild.node.loc;\n thisChild.replaceWith(thisRef);\n });\n\n if (!noNewArrows) thisBinding = null;\n }\n }\n\n return { thisBinding, fnPath };\n}\n\ntype LogicalOp = Parameters<typeof logicalExpression>[0];\ntype BinaryOp = Parameters<typeof binaryExpression>[0];\n\nfunction isLogicalOp(op: string): op is LogicalOp {\n return LOGICAL_OPERATORS.includes(op);\n}\n\nfunction standardizeSuperProperty(\n superProp: NodePath<t.MemberExpression>,\n):\n | [NodePath<t.MemberExpression>]\n | [NodePath<t.MemberExpression>, NodePath<t.MemberExpression>] {\n if (\n superProp.parentPath.isAssignmentExpression() &&\n superProp.parentPath.node.operator !== \"=\"\n ) {\n const assignmentPath = superProp.parentPath;\n\n const op = assignmentPath.node.operator.slice(0, -1) as\n | LogicalOp\n | BinaryOp;\n\n const value = assignmentPath.node.right;\n\n const isLogicalAssignment = isLogicalOp(op);\n\n if (superProp.node.computed) {\n // from: super[foo] **= 4;\n // to: super[tmp = foo] = super[tmp] ** 4;\n\n // from: super[foo] ??= 4;\n // to: super[tmp = foo] ?? super[tmp] = 4;\n\n const tmp = superProp.scope.generateDeclaredUidIdentifier(\"tmp\");\n\n const object = superProp.node.object;\n const property = superProp.node.property as t.Expression;\n\n assignmentPath\n .get(\"left\")\n .replaceWith(\n memberExpression(\n object,\n assignmentExpression(\"=\", tmp, property),\n true /* computed */,\n ),\n );\n\n assignmentPath\n .get(\"right\")\n .replaceWith(\n rightExpression(\n isLogicalAssignment ? \"=\" : op,\n memberExpression(object, identifier(tmp.name), true /* computed */),\n value,\n ),\n );\n } else {\n // from: super.foo **= 4;\n // to: super.foo = super.foo ** 4;\n\n // from: super.foo ??= 4;\n // to: super.foo ?? super.foo = 4;\n\n const object = superProp.node.object;\n const property = superProp.node.property as t.Identifier;\n\n assignmentPath\n .get(\"left\")\n .replaceWith(memberExpression(object, property));\n\n assignmentPath\n .get(\"right\")\n .replaceWith(\n rightExpression(\n isLogicalAssignment ? \"=\" : op,\n memberExpression(object, identifier(property.name)),\n value,\n ),\n );\n }\n\n if (isLogicalAssignment) {\n assignmentPath.replaceWith(\n logicalExpression(\n op,\n assignmentPath.node.left as t.MemberExpression,\n assignmentPath.node.right as t.Expression,\n ),\n );\n } else {\n assignmentPath.node.operator = \"=\";\n }\n\n return [\n assignmentPath.get(\"left\") as NodePath<t.MemberExpression>,\n assignmentPath.get(\"right\").get(\"left\"),\n ];\n } else if (superProp.parentPath.isUpdateExpression()) {\n const updateExpr = superProp.parentPath;\n\n const tmp = superProp.scope.generateDeclaredUidIdentifier(\"tmp\");\n const computedKey = superProp.node.computed\n ? superProp.scope.generateDeclaredUidIdentifier(\"prop\")\n : null;\n\n const parts: t.Expression[] = [\n assignmentExpression(\n \"=\",\n tmp,\n memberExpression(\n superProp.node.object,\n computedKey\n ? assignmentExpression(\n \"=\",\n computedKey,\n superProp.node.property as t.Expression,\n )\n : superProp.node.property,\n superProp.node.computed,\n ),\n ),\n assignmentExpression(\n \"=\",\n memberExpression(\n superProp.node.object,\n computedKey ? identifier(computedKey.name) : superProp.node.property,\n superProp.node.computed,\n ),\n binaryExpression(\n // map `++` to `+`, and `--` to `-`\n superProp.parentPath.node.operator[0] as \"+\" | \"-\",\n identifier(tmp.name),\n numericLiteral(1),\n ),\n ),\n ];\n\n if (!superProp.parentPath.node.prefix) {\n parts.push(identifier(tmp.name));\n }\n\n updateExpr.replaceWith(sequenceExpression(parts));\n\n const left = updateExpr.get(\n \"expressions.0.right\",\n ) as NodePath<t.MemberExpression>;\n const right = updateExpr.get(\n \"expressions.1.left\",\n ) as NodePath<t.MemberExpression>;\n return [left, right];\n }\n\n return [superProp];\n\n function rightExpression(\n op: BinaryOp | \"=\",\n left: t.MemberExpression,\n right: t.Expression,\n ) {\n if (op === \"=\") {\n return assignmentExpression(\"=\", left, right);\n } else {\n return binaryExpression(op, left, right);\n }\n }\n}\n\nfunction hasSuperClass(thisEnvFn: NodePath<t.Function>) {\n return (\n thisEnvFn.isClassMethod() &&\n !!(thisEnvFn.parentPath.parentPath.node as t.Class).superClass\n );\n}\n\nconst assignSuperThisVisitor = mergeVisitors<{\n supers: WeakSet<t.CallExpression>;\n thisBinding: string;\n}>([\n {\n CallExpression(child, { supers, thisBinding }) {\n if (!child.get(\"callee\").isSuper()) return;\n if (supers.has(child.node)) return;\n supers.add(child.node);\n\n child.replaceWithMultiple([\n child.node,\n assignmentExpression(\"=\", identifier(thisBinding), identifier(\"this\")),\n ]);\n },\n },\n environmentVisitor,\n]);\n\n// Create a binding that evaluates to the \"this\" of the given function.\nfunction getThisBinding(\n thisEnvFn: NodePath<t.Function>,\n inConstructor: boolean,\n) {\n return getBinding(thisEnvFn, \"this\", thisBinding => {\n if (!inConstructor || !hasSuperClass(thisEnvFn)) return thisExpression();\n\n thisEnvFn.traverse(assignSuperThisVisitor, {\n supers: new WeakSet(),\n thisBinding,\n });\n });\n}\n\n// Create a binding for a function that will call \"super()\" with arguments passed through.\nfunction getSuperBinding(thisEnvFn: NodePath<t.Function>) {\n return getBinding(thisEnvFn, \"supercall\", () => {\n const argsBinding = thisEnvFn.scope.generateUidIdentifier(\"args\");\n return arrowFunctionExpression(\n [restElement(argsBinding)],\n callExpression(_super(), [spreadElement(identifier(argsBinding.name))]),\n );\n });\n}\n\n// Create a binding for a function that will call \"super.foo\" or \"super[foo]\".\nfunction getSuperPropBinding(\n thisEnvFn: NodePath<t.Function>,\n isAssignment: boolean,\n propName: string,\n) {\n const op = isAssignment ? \"set\" : \"get\";\n\n return getBinding(thisEnvFn, `superprop_${op}:${propName || \"\"}`, () => {\n const argsList = [];\n\n let fnBody;\n if (propName) {\n // () => super.foo\n fnBody = memberExpression(_super(), identifier(propName));\n } else {\n const method = thisEnvFn.scope.generateUidIdentifier(\"prop\");\n // (method) => super[method]\n argsList.unshift(method);\n fnBody = memberExpression(\n _super(),\n identifier(method.name),\n true /* computed */,\n );\n }\n\n if (isAssignment) {\n const valueIdent = thisEnvFn.scope.generateUidIdentifier(\"value\");\n argsList.push(valueIdent);\n\n fnBody = assignmentExpression(\"=\", fnBody, identifier(valueIdent.name));\n }\n\n return arrowFunctionExpression(argsList, fnBody);\n });\n}\n\nfunction getBinding(\n thisEnvFn: NodePath,\n key: string,\n init: (name: string) => t.Expression,\n) {\n const cacheKey = \"binding:\" + key;\n let data: string | undefined = thisEnvFn.getData(cacheKey);\n if (!data) {\n const id = thisEnvFn.scope.generateUidIdentifier(key);\n data = id.name;\n thisEnvFn.setData(cacheKey, data);\n\n thisEnvFn.scope.push({\n id: id,\n init: init(data),\n });\n }\n\n return data;\n}\n\ntype ScopeInfo = {\n thisPaths: NodePath<t.ThisExpression | t.JSXIdentifier>[];\n superCalls: NodePath<t.CallExpression>[];\n superProps: NodePath<t.MemberExpression>[];\n argumentsPaths: NodePath<t.Identifier | t.JSXIdentifier>[];\n newTargetPaths: NodePath<t.MetaProperty>[];\n};\n\nconst getScopeInformationVisitor = mergeVisitors<ScopeInfo>([\n {\n ThisExpression(child, { thisPaths }) {\n thisPaths.push(child);\n },\n JSXIdentifier(child, { thisPaths }) {\n if (child.node.name !== \"this\") return;\n if (\n !child.parentPath.isJSXMemberExpression({ object: child.node }) &&\n !child.parentPath.isJSXOpeningElement({ name: child.node })\n ) {\n return;\n }\n\n thisPaths.push(child);\n },\n CallExpression(child, { superCalls }) {\n if (child.get(\"callee\").isSuper()) superCalls.push(child);\n },\n MemberExpression(child, { superProps }) {\n if (child.get(\"object\").isSuper()) superProps.push(child);\n },\n Identifier(child, { argumentsPaths }) {\n if (!child.isReferencedIdentifier({ name: \"arguments\" })) return;\n\n let curr = child.scope;\n do {\n if (curr.hasOwnBinding(\"arguments\")) {\n curr.rename(\"arguments\");\n return;\n }\n if (curr.path.isFunction() && !curr.path.isArrowFunctionExpression()) {\n break;\n }\n } while ((curr = curr.parent));\n\n argumentsPaths.push(child);\n },\n MetaProperty(child, { newTargetPaths }) {\n if (!child.get(\"meta\").isIdentifier({ name: \"new\" })) return;\n if (!child.get(\"property\").isIdentifier({ name: \"target\" })) return;\n\n newTargetPaths.push(child);\n },\n },\n environmentVisitor,\n]);\n\nfunction getScopeInformation(fnPath: NodePath) {\n const thisPaths: ScopeInfo[\"thisPaths\"] = [];\n const argumentsPaths: ScopeInfo[\"argumentsPaths\"] = [];\n const newTargetPaths: ScopeInfo[\"newTargetPaths\"] = [];\n const superProps: ScopeInfo[\"superProps\"] = [];\n const superCalls: ScopeInfo[\"superCalls\"] = [];\n\n fnPath.traverse(getScopeInformationVisitor, {\n thisPaths,\n argumentsPaths,\n newTargetPaths,\n superProps,\n superCalls,\n });\n\n return {\n thisPaths,\n argumentsPaths,\n newTargetPaths,\n superProps,\n superCalls,\n };\n}\n"],"mappings":";;;;;;;;;;;AAEA;;AA4BA;;AACA;;AACA;;;EA7BEA,uB;EACAC,oB;EACAC,gB;EACAC,c;EACAC,c;EACAC,qB;EACAC,mB;EACAC,U;EACAC,Y;EACAC,a;EACAC,iB;EACAC,iB;EACAC,gB;EACAC,Y;EACAC,c;EACAC,gB;EACAC,W;EACAC,e;EACAC,kB;EACAC,a;EACAC,a;EACAC,K,EAASC,M;EACTC,c;EACAC,Y;EACAC;;;AAQK,SAASC,aAAT,GAAuC;EAC5C,IAAIC,GAAJ;;EACA,IAAI,KAAKC,kBAAL,EAAJ,EAA+B;IAC7BD,GAAG,GAAG,KAAKE,IAAL,CAAUC,QAAhB;EACD,CAFD,MAEO,IAAI,KAAKC,UAAL,MAAqB,KAAKC,QAAL,EAAzB,EAA0C;IAC/CL,GAAG,GAAG,KAAKE,IAAL,CAAUF,GAAhB;EACD,CAFM,MAEA;IACL,MAAM,IAAIM,cAAJ,CAAmB,MAAnB,CAAN;EACD;;EAGD,IAAI,CAAC,KAAKJ,IAAL,CAAUK,QAAf,EAAyB;IACvB,IAAI1B,YAAY,CAACmB,GAAD,CAAhB,EAAuBA,GAAG,GAAGP,aAAa,CAACO,GAAG,CAACQ,IAAL,CAAnB;EACxB;;EAED,OAAOR,GAAP;AACD;;AAEM,SAASS,WAAT,GAIL;EACA,MAAMC,IAAI,GAAG,KAAKC,GAAL,CAAS,MAAT,CAAb;EACA,MAAMC,QAAQ,GAAGF,IAAI,CAACR,IAAtB;;EAEA,IAAIW,KAAK,CAACC,OAAN,CAAcJ,IAAd,CAAJ,EAAyB;IACvB,MAAM,IAAIK,KAAJ,CAAU,+CAAV,CAAN;EACD;;EACD,IAAI,CAACH,QAAL,EAAe;IACb,MAAM,IAAIG,KAAJ,CAAU,mCAAV,CAAN;EACD;;EAED,IAAIL,IAAI,CAACM,gBAAL,EAAJ,EAA6B;IAC3B,OAAOJ,QAAP;EACD;;EAED,MAAMK,UAA8B,GAAG,EAAvC;EAEA,IAAIC,UAAU,GAAG,MAAjB;EACA,IAAIlB,GAAJ;EACA,IAAImB,OAAJ;;EACA,IAAIT,IAAI,CAACU,WAAL,EAAJ,EAAwB;IACtBD,OAAO,GAAG,MAAV;IACAnB,GAAG,GAAG,CAAN;IACAiB,UAAU,CAACI,IAAX,CAAgBX,IAAI,CAACR,IAArB;EACD,CAJD,MAIO;IACLgB,UAAU,IAAI,SAAd;;IACA,IAAI,KAAKI,UAAL,EAAJ,EAAuB;MACrBtB,GAAG,GAAG,UAAN;MACAiB,UAAU,CAACI,IAAX,CAAgB/B,eAAe,CAACoB,IAAI,CAACR,IAAN,CAA/B;IACD,CAHD,MAGO;MACLF,GAAG,GAAG,YAAN;MACAiB,UAAU,CAACI,IAAX,CAAgB1C,mBAAmB,CAAC+B,IAAI,CAACR,IAAN,CAAnC;IACD;EACF;;EAED,KAAKA,IAAL,CAAUQ,IAAV,GAAiBlC,cAAc,CAACyC,UAAD,CAA/B;EACA,MAAMM,UAAU,GAAG,KAAKZ,GAAL,CAASO,UAAT,CAAnB;EACAR,IAAI,CAACc,KAAL,CACED,UADF,EAEEJ,OAAO,GAEHI,UAAU,CAACrB,IAAX,CAAgBiB,OAAhB,CAFG,GAGHI,UAAU,CAACrB,IALjB,EAMEiB,OANF,EAOEnB,GAPF;EAUA,OAAO,KAAKE,IAAZ;AACD;;AAMM,SAASuB,uBAAT,GAAiD;EACtD,IAAI,CAAC,KAAKC,yBAAL,EAAL,EAAuC;EAEvC,KAAKC,yBAAL;AACD;;AAQM,SAASC,yBAAT,GAAmD;EACxD,IACE,CAAC,KAAKF,yBAAL,EAAD,IACA,CAAC,KAAKG,oBAAL,EADD,IAEA,CAAC,KAAKC,qBAAL,EAHH,EAIE;IACA,MAAM,KAAKC,mBAAL,CACJ,gDADI,CAAN;EAGD;;EAEDC,wBAAwB,CAAC,IAAD,CAAxB;AACD;;AAED,SAASC,OAAT,CACEC,IADF,EAEEC,IAFF,EAGqD;EACnDD,IAAI,CAAChC,IAAL,CAAUiC,IAAV,GAAiBA,IAAjB;AACD;;AAKM,SAASR,yBAAT,CAEL;EACES,gBAAgB,GAAG,IADrB;EAGEC,aAAa,GAAG,KAHlB;EAKEC,WAAW,GAAG,CAACD;AALjB,IAUI,EAZC,EAagE;EACrE,IAAI,CAAC,KAAKX,yBAAL,EAAL,EAAuC;IACrC,MAAO,IAAD,CAAmBK,mBAAnB,CACJ,6DADI,CAAN;EAGD;;EAED,MAAM;IAAEQ,WAAF;IAAeC,MAAM,EAAEC;EAAvB,IAA8BT,wBAAwB,CAC1D,IAD0D,EAE1DM,WAF0D,EAG1DF,gBAH0D,CAA5D;EAOAK,EAAE,CAAChC,WAAH;EACAwB,OAAO,CAACQ,EAAD,EAAK,oBAAL,CAAP;;EAEA,IAAI,CAACH,WAAL,EAAkB;IAChB,MAAMI,YAAY,GAAGH,WAAW,GAC5B,IAD4B,GAE5BE,EAAE,CAACE,KAAH,CAASC,qBAAT,CAA+B,cAA/B,CAFJ;;IAGA,IAAIF,YAAJ,EAAkB;MAChBD,EAAE,CAAClB,UAAH,CAAcoB,KAAd,CAAoBtB,IAApB,CAAyB;QACvBwB,EAAE,EAAEH,YADmB;QAEvBI,IAAI,EAAE1D,gBAAgB,CAAC,EAAD;MAFC,CAAzB;IAID;;IAEDqD,EAAE,CAAC9B,GAAH,CAAO,MAAP,EAAeoC,gBAAf,CACE,MADF,EAEEpE,mBAAmB,CACjBF,cAAc,CAAC,KAAKuE,GAAL,CAASC,SAAT,CAAmB,eAAnB,CAAD,EAAsC,CAClDrD,cAAc,EADoC,EAElD8C,YAAY,GACR9D,UAAU,CAAC8D,YAAY,CAAClC,IAAd,CADF,GAER5B,UAAU,CAAC2D,WAAD,CAJoC,CAAtC,CADG,CAFrB;IAYAE,EAAE,CAACS,WAAH,CACEzE,cAAc,CACZQ,gBAAgB,CAEd,IAAAkE,2BAAA,EAAa,IAAb,EAAmB,IAAnB,KAA4BV,EAAE,CAACvC,IAFjB,EAGdtB,UAAU,CAAC,MAAD,CAHI,CADJ,EAMZ,CAAC8D,YAAY,GAAG9D,UAAU,CAAC8D,YAAY,CAAClC,IAAd,CAAb,GAAmCZ,cAAc,EAA9D,CANY,CADhB;IAWA,OAAO6C,EAAE,CAAC9B,GAAH,CAAO,eAAP,CAAP;EACD;;EAED,OAAO8B,EAAP;AACD;;AAED,MAAMW,oBAAoB,GAAG,IAAAC,eAAA,EAE1B,CACD;EACEC,cAAc,CAACC,KAAD,EAAQ;IAAEC;EAAF,CAAR,EAA2B;IACvC,IAAI,CAACD,KAAK,CAAC5C,GAAN,CAAU,QAAV,EAAoB8C,OAApB,EAAL,EAAoC;IACpCD,aAAa,CAACnC,IAAd,CAAmBkC,KAAnB;EACD;;AAJH,CADC,EAODG,iCAPC,CAF0B,CAA7B;;AAoBA,SAAS1B,wBAAT,CACEQ,MADF,EAGEF,WAA2B,GAAG,IAHhC,EAIEF,gBAAgC,GAAG,IAJrC,EAKyD;EACvD,IAAIuB,WAAJ;EACA,IAAIC,SAA+B,GAAGpB,MAAM,CAACqB,UAAP,CAAkBC,CAAC,IAAI;IAC3D,IAAIA,CAAC,CAACpC,yBAAF,EAAJ,EAAmC;MAAA;;MACjC,gBAAAiC,WAAW,SAAX,kBAAAA,WAAW,GAAKG,CAAhB;MACA,OAAO,KAAP;IACD;;IACD,OACEA,CAAC,CAACxC,UAAF,MACAwC,CAAC,CAACC,SAAF,EADA,IAEAD,CAAC,CAACE,eAAF,CAAkB;MAAEC,MAAM,EAAE;IAAV,CAAlB,CAFA,IAGAH,CAAC,CAACI,sBAAF,CAAyB;MAAED,MAAM,EAAE;IAAV,CAAzB,CAJF;EAMD,CAXqC,CAAtC;EAYA,MAAME,aAAa,GAAGP,SAAS,CAACQ,aAAV,CAAwB;IAAEC,IAAI,EAAE;EAAR,CAAxB,CAAtB;;EAEA,IAAIT,SAAS,CAACI,eAAV,MAA+BJ,SAAS,CAACM,sBAAV,EAAnC,EAAuE;IACrE,IAAIP,WAAJ,EAAiB;MACfC,SAAS,GAAGD,WAAZ;IACD,CAFD,MAEO,IAAIvB,gBAAJ,EAAsB;MAK3BI,MAAM,CAACU,WAAP,CACEzE,cAAc,CACZJ,uBAAuB,CAAC,EAAD,EAAKwB,YAAY,CAAC2C,MAAM,CAACtC,IAAR,CAAjB,CADX,EAEZ,EAFY,CADhB;MAMA0D,SAAS,GAAGpB,MAAM,CAAC7B,GAAP,CAAW,QAAX,CAAZ;MACA6B,MAAM,GAAGoB,SAAS,CAACjD,GAAV,CAAc,MAAd,CAAT;IACD,CAbM,MAaA;MACL,MAAM6B,MAAM,CAACT,mBAAP,CACJ,iDADI,CAAN;IAGD;EACF;;EAED,MAAM;IAAEuC,SAAF;IAAaC,cAAb;IAA6BC,cAA7B;IAA6CC,UAA7C;IAAyDC;EAAzD,IACJC,mBAAmB,CAACnC,MAAD,CADrB;;EAIA,IAAI2B,aAAa,IAAIO,UAAU,CAACE,MAAX,GAAoB,CAAzC,EAA4C;IAC1C,IAAI,CAACxC,gBAAL,EAAuB;MACrB,MAAMsC,UAAU,CAAC,CAAD,CAAV,CAAc3C,mBAAd,CACJ,gDADI,CAAN;IAGD;;IACD,MAAMyB,aAA2C,GAAG,EAApD;IACAI,SAAS,CAACiB,QAAV,CAAmBzB,oBAAnB,EAAyC;MAAEI;IAAF,CAAzC;IACA,MAAMsB,YAAY,GAAGC,eAAe,CAACnB,SAAD,CAApC;IACAJ,aAAa,CAACwB,OAAd,CAAsBC,SAAS,IAAI;MACjC,MAAMC,MAAM,GAAGtG,UAAU,CAACkG,YAAD,CAAzB;MACAI,MAAM,CAACC,GAAP,GAAaF,SAAS,CAAC/E,IAAV,CAAegF,MAAf,CAAsBC,GAAnC;MAEAF,SAAS,CAACtE,GAAV,CAAc,QAAd,EAAwBuC,WAAxB,CAAoCgC,MAApC;IACD,CALD;EAMD;;EAGD,IAAIX,cAAc,CAACK,MAAf,GAAwB,CAA5B,EAA+B;IAC7B,MAAMQ,gBAAgB,GAAGC,UAAU,CAACzB,SAAD,EAAY,WAAZ,EAAyB,MAAM;MAChE,MAAM0B,IAAI,GAAG,MAAM1G,UAAU,CAAC,WAAD,CAA7B;;MACA,IAAIgF,SAAS,CAACjB,KAAV,CAAgBT,IAAhB,CAAqB6B,SAArB,EAAJ,EAAsC;QACpC,OAAOrF,qBAAqB,CAC1BH,gBAAgB,CACd,KADc,EAEduB,eAAe,CAAC,QAAD,EAAWwF,IAAI,EAAf,CAFD,EAGd7F,aAAa,CAAC,WAAD,CAHC,CADU,EAM1BmE,SAAS,CAACjB,KAAV,CAAgB4C,kBAAhB,EAN0B,EAO1BD,IAAI,EAPsB,CAA5B;MASD,CAVD,MAUO;QACL,OAAOA,IAAI,EAAX;MACD;IACF,CAfkC,CAAnC;IAiBAf,cAAc,CAACS,OAAf,CAAuBQ,cAAc,IAAI;MACvC,MAAMC,OAAO,GAAG7G,UAAU,CAACwG,gBAAD,CAA1B;MACAK,OAAO,CAACN,GAAR,GAAcK,cAAc,CAACtF,IAAf,CAAoBiF,GAAlC;MAEAK,cAAc,CAACtC,WAAf,CAA2BuC,OAA3B;IACD,CALD;EAMD;;EAGD,IAAIjB,cAAc,CAACI,MAAf,GAAwB,CAA5B,EAA+B;IAC7B,MAAMc,gBAAgB,GAAGL,UAAU,CAACzB,SAAD,EAAY,WAAZ,EAAyB,MAC1D1E,YAAY,CAACN,UAAU,CAAC,KAAD,CAAX,EAAoBA,UAAU,CAAC,QAAD,CAA9B,CADqB,CAAnC;IAIA4F,cAAc,CAACQ,OAAf,CAAuBW,WAAW,IAAI;MACpC,MAAMC,SAAS,GAAGhH,UAAU,CAAC8G,gBAAD,CAA5B;MACAE,SAAS,CAACT,GAAV,GAAgBQ,WAAW,CAACzF,IAAZ,CAAiBiF,GAAjC;MAEAQ,WAAW,CAACzC,WAAZ,CAAwB0C,SAAxB;IACD,CALD;EAMD;;EAGD,IAAInB,UAAU,CAACG,MAAX,GAAoB,CAAxB,EAA2B;IACzB,IAAI,CAACxC,gBAAL,EAAuB;MACrB,MAAMqC,UAAU,CAAC,CAAD,CAAV,CAAc1C,mBAAd,CACJ,0CADI,CAAN;IAGD;;IAED,MAAM8D,cAA8C,GAAGpB,UAAU,CAACqB,MAAX,CACrD,CAACC,GAAD,EAAMC,SAAN,KAAoBD,GAAG,CAACE,MAAJ,CAAWC,wBAAwB,CAACF,SAAD,CAAnC,CADiC,EAErD,EAFqD,CAAvD;IAKAH,cAAc,CAACb,OAAf,CAAuBgB,SAAS,IAAI;MAClC,MAAMhG,GAAG,GAAGgG,SAAS,CAAC9F,IAAV,CAAeK,QAAf,GACR,EADQ,GAGRyF,SAAS,CAACrF,GAAV,CAAc,UAAd,EAA0BT,IAA1B,CAA+BM,IAHnC;MAKA,MAAM2F,eAAe,GAAGH,SAAS,CAACzE,UAAlC;MAEA,MAAM6E,YAAY,GAAGD,eAAe,CAACE,sBAAhB,CAAuC;QAC1DC,IAAI,EAAEN,SAAS,CAAC9F;MAD0C,CAAvC,CAArB;MAGA,MAAMqG,MAAM,GAAGJ,eAAe,CAACK,gBAAhB,CAAiC;QAC9CtB,MAAM,EAAEc,SAAS,CAAC9F;MAD4B,CAAjC,CAAf;MAGA,MAAM4E,YAAY,GAAG2B,mBAAmB,CAAC7C,SAAD,EAAYwC,YAAZ,EAA0BpG,GAA1B,CAAxC;MAEA,MAAMsF,IAAoB,GAAG,EAA7B;;MACA,IAAIU,SAAS,CAAC9F,IAAV,CAAeK,QAAnB,EAA6B;QAE3B+E,IAAI,CAACjE,IAAL,CAAU2E,SAAS,CAACrF,GAAV,CAAc,UAAd,EAA0BT,IAApC;MACD;;MAED,IAAIkG,YAAJ,EAAkB;QAChB,MAAMM,KAAK,GAAGP,eAAe,CAACjG,IAAhB,CAAqByG,KAAnC;QACArB,IAAI,CAACjE,IAAL,CAAUqF,KAAV;MACD;;MAED,MAAME,IAAI,GAAGnI,cAAc,CAACG,UAAU,CAACkG,YAAD,CAAX,EAA2BQ,IAA3B,CAA3B;;MAEA,IAAIiB,MAAJ,EAAY;QACVJ,eAAe,CAACpD,gBAAhB,CAAiC,WAAjC,EAA8CnD,cAAc,EAA5D;QACAoG,SAAS,CAAC9C,WAAV,CAAsBjE,gBAAgB,CAAC2H,IAAD,EAAOhI,UAAU,CAAC,MAAD,CAAjB,CAAtC;QAEA0F,SAAS,CAACjD,IAAV,CACE8E,eAAe,CAACxF,GAAhB,CAAoB,aAApB,CADF;MAGD,CAPD,MAOO,IAAIyF,YAAJ,EAAkB;QAEvBD,eAAe,CAACjD,WAAhB,CAA4B0D,IAA5B;MACD,CAHM,MAGA;QACLZ,SAAS,CAAC9C,WAAV,CAAsB0D,IAAtB;MACD;IACF,CA1CD;EA2CD;;EAGD,IAAIrE,WAAJ;;EACA,IAAI+B,SAAS,CAACM,MAAV,GAAmB,CAAnB,IAAwB,CAACtC,WAA7B,EAA0C;IACxCC,WAAW,GAAGsE,cAAc,CAACjD,SAAD,EAAYO,aAAZ,CAA5B;;IAEA,IACE7B,WAAW,IAGV6B,aAAa,IAAI2C,aAAa,CAAClD,SAAD,CAJjC,EAKE;MACAU,SAAS,CAACU,OAAV,CAAkB+B,SAAS,IAAI;QAC7B,MAAMC,OAAO,GAAGD,SAAS,CAACE,KAAV,KACZnI,aAAa,CAACyD,WAAD,CADD,GAEZ3D,UAAU,CAAC2D,WAAD,CAFd;QAIAyE,OAAO,CAAC7B,GAAR,GAAc4B,SAAS,CAAC7G,IAAV,CAAeiF,GAA7B;QACA4B,SAAS,CAAC7D,WAAV,CAAsB8D,OAAtB;MACD,CAPD;MASA,IAAI,CAAC1E,WAAL,EAAkBC,WAAW,GAAG,IAAd;IACnB;EACF;;EAED,OAAO;IAAEA,WAAF;IAAeC;EAAf,CAAP;AACD;;AAKD,SAAS0E,WAAT,CAAqBC,EAArB,EAAkD;EAChD,OAAOnI,iBAAiB,CAACoI,QAAlB,CAA2BD,EAA3B,CAAP;AACD;;AAED,SAASjB,wBAAT,CACEF,SADF,EAIiE;EAC/D,IACEA,SAAS,CAACzE,UAAV,CAAqB8E,sBAArB,MACAL,SAAS,CAACzE,UAAV,CAAqBrB,IAArB,CAA0BmH,QAA1B,KAAuC,GAFzC,EAGE;IACA,MAAMC,cAAc,GAAGtB,SAAS,CAACzE,UAAjC;IAEA,MAAM4F,EAAE,GAAGG,cAAc,CAACpH,IAAf,CAAoBmH,QAApB,CAA6BE,KAA7B,CAAmC,CAAnC,EAAsC,CAAC,CAAvC,CAAX;IAIA,MAAMb,KAAK,GAAGY,cAAc,CAACpH,IAAf,CAAoByG,KAAlC;IAEA,MAAMa,mBAAmB,GAAGN,WAAW,CAACC,EAAD,CAAvC;;IAEA,IAAInB,SAAS,CAAC9F,IAAV,CAAeK,QAAnB,EAA6B;MAO3B,MAAMkH,GAAG,GAAGzB,SAAS,CAACrD,KAAV,CAAgB+E,6BAAhB,CAA8C,KAA9C,CAAZ;MAEA,MAAMC,MAAM,GAAG3B,SAAS,CAAC9F,IAAV,CAAeyH,MAA9B;MACA,MAAMxH,QAAQ,GAAG6F,SAAS,CAAC9F,IAAV,CAAeC,QAAhC;MAEAmH,cAAc,CACX3G,GADH,CACO,MADP,EAEGuC,WAFH,CAGIjE,gBAAgB,CACd0I,MADc,EAEdrJ,oBAAoB,CAAC,GAAD,EAAMmJ,GAAN,EAAWtH,QAAX,CAFN,EAGd,IAHc,CAHpB;MAUAmH,cAAc,CACX3G,GADH,CACO,OADP,EAEGuC,WAFH,CAGI0E,eAAe,CACbJ,mBAAmB,GAAG,GAAH,GAASL,EADf,EAEblI,gBAAgB,CAAC0I,MAAD,EAAS/I,UAAU,CAAC6I,GAAG,CAACjH,IAAL,CAAnB,EAA+B,IAA/B,CAFH,EAGbkG,KAHa,CAHnB;IASD,CA/BD,MA+BO;MAOL,MAAMiB,MAAM,GAAG3B,SAAS,CAAC9F,IAAV,CAAeyH,MAA9B;MACA,MAAMxH,QAAQ,GAAG6F,SAAS,CAAC9F,IAAV,CAAeC,QAAhC;MAEAmH,cAAc,CACX3G,GADH,CACO,MADP,EAEGuC,WAFH,CAEejE,gBAAgB,CAAC0I,MAAD,EAASxH,QAAT,CAF/B;MAIAmH,cAAc,CACX3G,GADH,CACO,OADP,EAEGuC,WAFH,CAGI0E,eAAe,CACbJ,mBAAmB,GAAG,GAAH,GAASL,EADf,EAEblI,gBAAgB,CAAC0I,MAAD,EAAS/I,UAAU,CAACuB,QAAQ,CAACK,IAAV,CAAnB,CAFH,EAGbkG,KAHa,CAHnB;IASD;;IAED,IAAIc,mBAAJ,EAAyB;MACvBF,cAAc,CAACpE,WAAf,CACEnE,iBAAiB,CACfoI,EADe,EAEfG,cAAc,CAACpH,IAAf,CAAoBoG,IAFL,EAGfgB,cAAc,CAACpH,IAAf,CAAoByG,KAHL,CADnB;IAOD,CARD,MAQO;MACLW,cAAc,CAACpH,IAAf,CAAoBmH,QAApB,GAA+B,GAA/B;IACD;;IAED,OAAO,CACLC,cAAc,CAAC3G,GAAf,CAAmB,MAAnB,CADK,EAEL2G,cAAc,CAAC3G,GAAf,CAAmB,OAAnB,EAA4BA,GAA5B,CAAgC,MAAhC,CAFK,CAAP;EAID,CAtFD,MAsFO,IAAIqF,SAAS,CAACzE,UAAV,CAAqBsG,kBAArB,EAAJ,EAA+C;IACpD,MAAMC,UAAU,GAAG9B,SAAS,CAACzE,UAA7B;IAEA,MAAMkG,GAAG,GAAGzB,SAAS,CAACrD,KAAV,CAAgB+E,6BAAhB,CAA8C,KAA9C,CAAZ;IACA,MAAMK,WAAW,GAAG/B,SAAS,CAAC9F,IAAV,CAAeK,QAAf,GAChByF,SAAS,CAACrD,KAAV,CAAgB+E,6BAAhB,CAA8C,MAA9C,CADgB,GAEhB,IAFJ;IAIA,MAAMM,KAAqB,GAAG,CAC5B1J,oBAAoB,CAClB,GADkB,EAElBmJ,GAFkB,EAGlBxI,gBAAgB,CACd+G,SAAS,CAAC9F,IAAV,CAAeyH,MADD,EAEdI,WAAW,GACPzJ,oBAAoB,CAClB,GADkB,EAElByJ,WAFkB,EAGlB/B,SAAS,CAAC9F,IAAV,CAAeC,QAHG,CADb,GAMP6F,SAAS,CAAC9F,IAAV,CAAeC,QARL,EASd6F,SAAS,CAAC9F,IAAV,CAAeK,QATD,CAHE,CADQ,EAgB5BjC,oBAAoB,CAClB,GADkB,EAElBW,gBAAgB,CACd+G,SAAS,CAAC9F,IAAV,CAAeyH,MADD,EAEdI,WAAW,GAAGnJ,UAAU,CAACmJ,WAAW,CAACvH,IAAb,CAAb,GAAkCwF,SAAS,CAAC9F,IAAV,CAAeC,QAF9C,EAGd6F,SAAS,CAAC9F,IAAV,CAAeK,QAHD,CAFE,EAOlBhC,gBAAgB,CAEdyH,SAAS,CAACzE,UAAV,CAAqBrB,IAArB,CAA0BmH,QAA1B,CAAmC,CAAnC,CAFc,EAGdzI,UAAU,CAAC6I,GAAG,CAACjH,IAAL,CAHI,EAIdrB,cAAc,CAAC,CAAD,CAJA,CAPE,CAhBQ,CAA9B;;IAgCA,IAAI,CAAC6G,SAAS,CAACzE,UAAV,CAAqBrB,IAArB,CAA0B+H,MAA/B,EAAuC;MACrCD,KAAK,CAAC3G,IAAN,CAAWzC,UAAU,CAAC6I,GAAG,CAACjH,IAAL,CAArB;IACD;;IAEDsH,UAAU,CAAC5E,WAAX,CAAuB3D,kBAAkB,CAACyI,KAAD,CAAzC;IAEA,MAAM1B,IAAI,GAAGwB,UAAU,CAACnH,GAAX,CACX,qBADW,CAAb;IAGA,MAAMgG,KAAK,GAAGmB,UAAU,CAACnH,GAAX,CACZ,oBADY,CAAd;IAGA,OAAO,CAAC2F,IAAD,EAAOK,KAAP,CAAP;EACD;;EAED,OAAO,CAACX,SAAD,CAAP;;EAEA,SAAS4B,eAAT,CACET,EADF,EAEEb,IAFF,EAGEK,KAHF,EAIE;IACA,IAAIQ,EAAE,KAAK,GAAX,EAAgB;MACd,OAAO7I,oBAAoB,CAAC,GAAD,EAAMgI,IAAN,EAAYK,KAAZ,CAA3B;IACD,CAFD,MAEO;MACL,OAAOpI,gBAAgB,CAAC4I,EAAD,EAAKb,IAAL,EAAWK,KAAX,CAAvB;IACD;EACF;AACF;;AAED,SAASG,aAAT,CAAuBlD,SAAvB,EAAwD;EACtD,OACEA,SAAS,CAACQ,aAAV,MACA,CAAC,CAAER,SAAS,CAACrC,UAAV,CAAqBA,UAArB,CAAgCrB,IAAjC,CAAkDgI,UAFtD;AAID;;AAED,MAAMC,sBAAsB,GAAG,IAAA9E,eAAA,EAG5B,CACD;EACEC,cAAc,CAACC,KAAD,EAAQ;IAAE6E,MAAF;IAAU7F;EAAV,CAAR,EAAiC;IAC7C,IAAI,CAACgB,KAAK,CAAC5C,GAAN,CAAU,QAAV,EAAoB8C,OAApB,EAAL,EAAoC;IACpC,IAAI2E,MAAM,CAACC,GAAP,CAAW9E,KAAK,CAACrD,IAAjB,CAAJ,EAA4B;IAC5BkI,MAAM,CAACE,GAAP,CAAW/E,KAAK,CAACrD,IAAjB;IAEAqD,KAAK,CAACgF,mBAAN,CAA0B,CACxBhF,KAAK,CAACrD,IADkB,EAExB5B,oBAAoB,CAAC,GAAD,EAAMM,UAAU,CAAC2D,WAAD,CAAhB,EAA+B3D,UAAU,CAAC,MAAD,CAAzC,CAFI,CAA1B;EAID;;AAVH,CADC,EAaD8E,iCAbC,CAH4B,CAA/B;;AAoBA,SAASmD,cAAT,CACEjD,SADF,EAEEO,aAFF,EAGE;EACA,OAAOkB,UAAU,CAACzB,SAAD,EAAY,MAAZ,EAAoBrB,WAAW,IAAI;IAClD,IAAI,CAAC4B,aAAD,IAAkB,CAAC2C,aAAa,CAAClD,SAAD,CAApC,EAAiD,OAAOhE,cAAc,EAArB;IAEjDgE,SAAS,CAACiB,QAAV,CAAmBsD,sBAAnB,EAA2C;MACzCC,MAAM,EAAE,IAAII,OAAJ,EADiC;MAEzCjG;IAFyC,CAA3C;EAID,CAPgB,CAAjB;AAQD;;AAGD,SAASwC,eAAT,CAAyBnB,SAAzB,EAA0D;EACxD,OAAOyB,UAAU,CAACzB,SAAD,EAAY,WAAZ,EAAyB,MAAM;IAC9C,MAAM6E,WAAW,GAAG7E,SAAS,CAACjB,KAAV,CAAgBC,qBAAhB,CAAsC,MAAtC,CAApB;IACA,OAAOvE,uBAAuB,CAC5B,CAACgB,WAAW,CAACoJ,WAAD,CAAZ,CAD4B,EAE5BhK,cAAc,CAACkB,MAAM,EAAP,EAAW,CAACH,aAAa,CAACZ,UAAU,CAAC6J,WAAW,CAACjI,IAAb,CAAX,CAAd,CAAX,CAFc,CAA9B;EAID,CANgB,CAAjB;AAOD;;AAGD,SAASiG,mBAAT,CACE7C,SADF,EAEEwC,YAFF,EAGEsC,QAHF,EAIE;EACA,MAAMvB,EAAE,GAAGf,YAAY,GAAG,KAAH,GAAW,KAAlC;EAEA,OAAOf,UAAU,CAACzB,SAAD,EAAa,aAAYuD,EAAG,IAAGuB,QAAQ,IAAI,EAAG,EAA9C,EAAiD,MAAM;IACtE,MAAMC,QAAQ,GAAG,EAAjB;IAEA,IAAIC,MAAJ;;IACA,IAAIF,QAAJ,EAAc;MAEZE,MAAM,GAAG3J,gBAAgB,CAACU,MAAM,EAAP,EAAWf,UAAU,CAAC8J,QAAD,CAArB,CAAzB;IACD,CAHD,MAGO;MACL,MAAMG,MAAM,GAAGjF,SAAS,CAACjB,KAAV,CAAgBC,qBAAhB,CAAsC,MAAtC,CAAf;MAEA+F,QAAQ,CAACG,OAAT,CAAiBD,MAAjB;MACAD,MAAM,GAAG3J,gBAAgB,CACvBU,MAAM,EADiB,EAEvBf,UAAU,CAACiK,MAAM,CAACrI,IAAR,CAFa,EAGvB,IAHuB,CAAzB;IAKD;;IAED,IAAI4F,YAAJ,EAAkB;MAChB,MAAM2C,UAAU,GAAGnF,SAAS,CAACjB,KAAV,CAAgBC,qBAAhB,CAAsC,OAAtC,CAAnB;MACA+F,QAAQ,CAACtH,IAAT,CAAc0H,UAAd;MAEAH,MAAM,GAAGtK,oBAAoB,CAAC,GAAD,EAAMsK,MAAN,EAAchK,UAAU,CAACmK,UAAU,CAACvI,IAAZ,CAAxB,CAA7B;IACD;;IAED,OAAOnC,uBAAuB,CAACsK,QAAD,EAAWC,MAAX,CAA9B;EACD,CA1BgB,CAAjB;AA2BD;;AAED,SAASvD,UAAT,CACEzB,SADF,EAEE5D,GAFF,EAGE8C,IAHF,EAIE;EACA,MAAMkG,QAAQ,GAAG,aAAahJ,GAA9B;EACA,IAAIiJ,IAAwB,GAAGrF,SAAS,CAACsF,OAAV,CAAkBF,QAAlB,CAA/B;;EACA,IAAI,CAACC,IAAL,EAAW;IACT,MAAMpG,EAAE,GAAGe,SAAS,CAACjB,KAAV,CAAgBC,qBAAhB,CAAsC5C,GAAtC,CAAX;IACAiJ,IAAI,GAAGpG,EAAE,CAACrC,IAAV;IACAoD,SAAS,CAACuF,OAAV,CAAkBH,QAAlB,EAA4BC,IAA5B;IAEArF,SAAS,CAACjB,KAAV,CAAgBtB,IAAhB,CAAqB;MACnBwB,EAAE,EAAEA,EADe;MAEnBC,IAAI,EAAEA,IAAI,CAACmG,IAAD;IAFS,CAArB;EAID;;EAED,OAAOA,IAAP;AACD;;AAUD,MAAMG,0BAA0B,GAAG,IAAA/F,eAAA,EAAyB,CAC1D;EACEgG,cAAc,CAAC9F,KAAD,EAAQ;IAAEe;EAAF,CAAR,EAAuB;IACnCA,SAAS,CAACjD,IAAV,CAAekC,KAAf;EACD,CAHH;;EAIE+F,aAAa,CAAC/F,KAAD,EAAQ;IAAEe;EAAF,CAAR,EAAuB;IAClC,IAAIf,KAAK,CAACrD,IAAN,CAAWM,IAAX,KAAoB,MAAxB,EAAgC;;IAChC,IACE,CAAC+C,KAAK,CAAChC,UAAN,CAAiBgI,qBAAjB,CAAuC;MAAE5B,MAAM,EAAEpE,KAAK,CAACrD;IAAhB,CAAvC,CAAD,IACA,CAACqD,KAAK,CAAChC,UAAN,CAAiBiI,mBAAjB,CAAqC;MAAEhJ,IAAI,EAAE+C,KAAK,CAACrD;IAAd,CAArC,CAFH,EAGE;MACA;IACD;;IAEDoE,SAAS,CAACjD,IAAV,CAAekC,KAAf;EACD,CAdH;;EAeED,cAAc,CAACC,KAAD,EAAQ;IAAEmB;EAAF,CAAR,EAAwB;IACpC,IAAInB,KAAK,CAAC5C,GAAN,CAAU,QAAV,EAAoB8C,OAApB,EAAJ,EAAmCiB,UAAU,CAACrD,IAAX,CAAgBkC,KAAhB;EACpC,CAjBH;;EAkBEkG,gBAAgB,CAAClG,KAAD,EAAQ;IAAEkB;EAAF,CAAR,EAAwB;IACtC,IAAIlB,KAAK,CAAC5C,GAAN,CAAU,QAAV,EAAoB8C,OAApB,EAAJ,EAAmCgB,UAAU,CAACpD,IAAX,CAAgBkC,KAAhB;EACpC,CApBH;;EAqBEmG,UAAU,CAACnG,KAAD,EAAQ;IAAEgB;EAAF,CAAR,EAA4B;IACpC,IAAI,CAAChB,KAAK,CAACoG,sBAAN,CAA6B;MAAEnJ,IAAI,EAAE;IAAR,CAA7B,CAAL,EAA0D;IAE1D,IAAIoJ,IAAI,GAAGrG,KAAK,CAACZ,KAAjB;;IACA,GAAG;MACD,IAAIiH,IAAI,CAACC,aAAL,CAAmB,WAAnB,CAAJ,EAAqC;QACnCD,IAAI,CAACE,MAAL,CAAY,WAAZ;QACA;MACD;;MACD,IAAIF,IAAI,CAAC1H,IAAL,CAAUZ,UAAV,MAA0B,CAACsI,IAAI,CAAC1H,IAAL,CAAUR,yBAAV,EAA/B,EAAsE;QACpE;MACD;IACF,CARD,QAQUkI,IAAI,GAAGA,IAAI,CAACG,MARtB;;IAUAxF,cAAc,CAAClD,IAAf,CAAoBkC,KAApB;EACD,CApCH;;EAqCEyG,YAAY,CAACzG,KAAD,EAAQ;IAAEiB;EAAF,CAAR,EAA4B;IACtC,IAAI,CAACjB,KAAK,CAAC5C,GAAN,CAAU,MAAV,EAAkB9B,YAAlB,CAA+B;MAAE2B,IAAI,EAAE;IAAR,CAA/B,CAAL,EAAsD;IACtD,IAAI,CAAC+C,KAAK,CAAC5C,GAAN,CAAU,UAAV,EAAsB9B,YAAtB,CAAmC;MAAE2B,IAAI,EAAE;IAAR,CAAnC,CAAL,EAA6D;IAE7DgE,cAAc,CAACnD,IAAf,CAAoBkC,KAApB;EACD;;AA1CH,CAD0D,EA6C1DG,iCA7C0D,CAAzB,CAAnC;;AAgDA,SAASiB,mBAAT,CAA6BnC,MAA7B,EAA+C;EAC7C,MAAM8B,SAAiC,GAAG,EAA1C;EACA,MAAMC,cAA2C,GAAG,EAApD;EACA,MAAMC,cAA2C,GAAG,EAApD;EACA,MAAMC,UAAmC,GAAG,EAA5C;EACA,MAAMC,UAAmC,GAAG,EAA5C;EAEAlC,MAAM,CAACqC,QAAP,CAAgBuE,0BAAhB,EAA4C;IAC1C9E,SAD0C;IAE1CC,cAF0C;IAG1CC,cAH0C;IAI1CC,UAJ0C;IAK1CC;EAL0C,CAA5C;EAQA,OAAO;IACLJ,SADK;IAELC,cAFK;IAGLC,cAHK;IAILC,UAJK;IAKLC;EALK,CAAP;AAOD"}