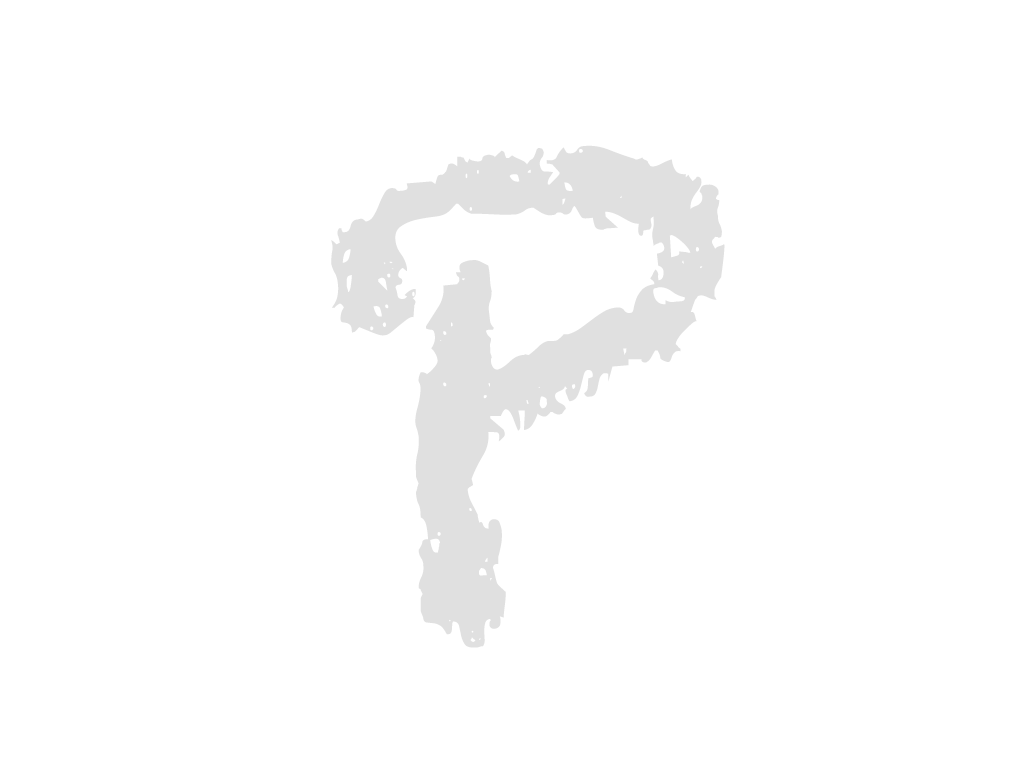
File name
Commit message
Commit date
File name
Commit message
Commit date
import cv2
import os
import glob
from joblib import Parallel, delayed
def crop_image(image_path, crop_size, start_point):
if image_path.endswith(".jpg") or image_path.endswith(".png"):
image = cv2.imread(image_path)
height, width = image.shape[:2]
if width > start_point[0] + crop_size[0] and height > start_point[1] + crop_size[1]:
cropped_image = image[start_point[1]:start_point[1]+crop_size[1], start_point[0]:start_point[0]+crop_size[0]]
return cropped_image
else:
print(f"Image {os.path.basename(image_path)} is too small to be cropped with the current settings.")
return False
def crop_images_parallel(image_paths, output_directory, crop_size, start_point):
if not os.path.exists(output_directory):
os.makedirs(output_directory)
# run the cropping function in parallel
Parallel(n_jobs=-1)(delayed(crop_image)(image_path, output_directory, crop_size, start_point) for image_path in image_paths)
if __name__ == "__main__":
output_directory = "/home/takensoft/Pictures/test512_512/rainy/"
# get all image paths in the directory
# image_paths = glob.glob("/home/takensoft/Pictures/화창한날, 비오는날 프레임2000장/화창한날 프레임 추출/하드디스크 화창한날(17개)/**/*.png")
# image_paths += glob.glob("/home/takensoft/Pictures/화창한날, 비오는날 프레임2000장/화창한날 프레임 추출/7월19일 화창한날(8개)/**/*.png")
image_paths = glob.glob("/home/takensoft/Pictures/화창한날, 비오는날 프레임2000장/비오는날 프레임 추출/7월11일 폭우(3개)/**/*.png")
image_paths += glob.glob("/home/takensoft/Pictures/폭우 빗방울 (475개)/*.png")
crop_size = (512, 512) # width and height you want for your cropped images
start_point = (750, 450) # upper left point where the crop should start
crop_images_parallel(image_paths, output_directory, crop_size, start_point)