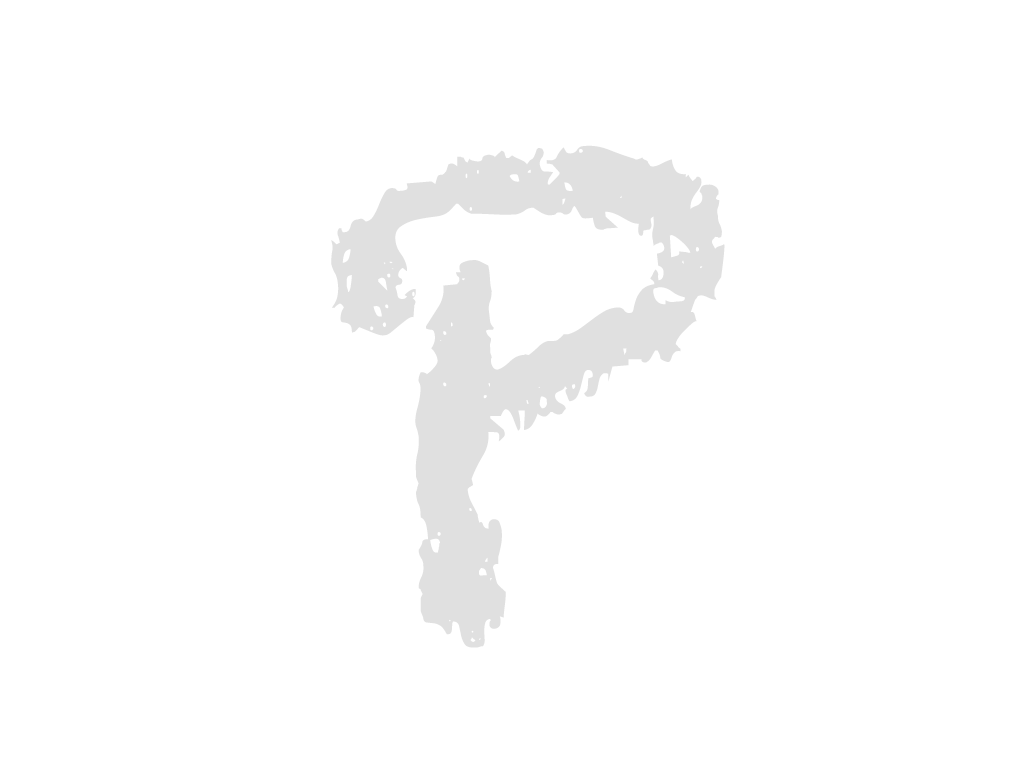
File name
Commit message
Commit date
2023-09-26
File name
Commit message
Commit date
File name
Commit message
Commit date
import plotly.express as px
import pandas as pd
import re
from io import StringIO
def extract_range(line):
values = re.findall(r"[-+]?\d*\.\d+|\d+", line)
if len(values) >= 2:
return [float(values[0]), float(values[1])]
else:
raise ValueError(f"Could not extract range from line: {line}")
def draw_gcode(file_name):
with open(file_name, "r") as f:
data = f.read()
# Extracting necessary info from the first 9 lines
info_lines = data.split('"X,Y,Z,G,M,S,F"')[0].split('\n')
name = info_lines[2].split('=')[1].strip()
# Using the function to extract the ranges
x_range = extract_range(info_lines[6]) # Corrected index
y_range = extract_range(info_lines[7]) # Corrected index
z_range = extract_range(info_lines[8]) # Corrected index
# Parsing the coordinate data
data_coords = data.split('"X,Y,Z,G,M,S,F"')[1].strip()
# The data was refined, but he made a mistake of... putting all in a string.
df = pd.read_csv(StringIO(data_coords), delimiter=',', names=['X', 'Y', 'Z', 'G', 'M', 'S', 'F'])
# Visualizing using Plotly
fig = px.scatter_3d(df, x='X', y='Y', z='Z', color='M',
title=name,
range_x=x_range,
range_y=y_range,
range_z=z_range,
size_max=8,
opacity=0.3)
fig.update_traces(marker=dict(size=1))
fig.show()
if __name__ == "__main__":
file_name = "/home/juni/PycharmProjects/failure_analysis/data/ncdata/cleaned/26.csv"
draw_gcode(file_name)