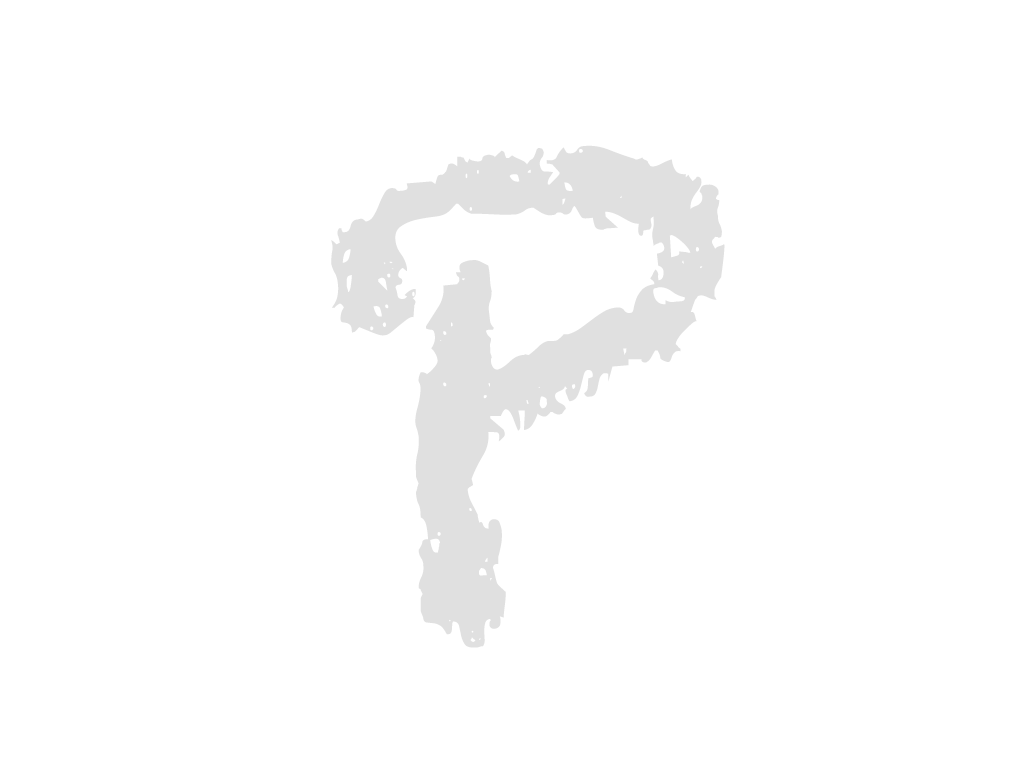
--- android/app/src/main/AndroidManifest.xml
+++ android/app/src/main/AndroidManifest.xml
... | ... | @@ -1,4 +1,5 @@ |
1 |
-<manifest xmlns:android="http://schemas.android.com/apk/res/android"> |
|
1 |
+<manifest xmlns:android="http://schemas.android.com/apk/res/android" |
|
2 |
+package="com.send_location_ta"> |
|
2 | 3 |
|
3 | 4 |
<uses-permission android:name="android.permission.INTERNET" /> |
4 | 5 |
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> |
... | ... | @@ -16,6 +17,7 @@ |
16 | 17 |
android:allowBackup="false" |
17 | 18 |
android:theme="@style/AppTheme" |
18 | 19 |
android:exported="true" |
20 |
+ android:requestLegacyExternalStorage="true" |
|
19 | 21 |
android:usesCleartextTraffic="true"> |
20 | 22 |
<activity |
21 | 23 |
android:name=".MainActivity" |
--- android/app/src/main/assets/index.android.bundle
+++ android/app/src/main/assets/index.android.bundle
This diff is too big to display. |
--- package-lock.json
+++ package-lock.json
This diff is too big to display. |
--- src/screens/LoginScreen.tsx
+++ src/screens/LoginScreen.tsx
... | ... | @@ -12,6 +12,7 @@ |
12 | 12 |
import {useLoginAPI} from '../utils/loginUtils'; |
13 | 13 |
import {RootStackParam} from '../utils/RootStackParam'; |
14 | 14 |
import {useFocusEffect} from '@react-navigation/native'; |
15 |
+import AsyncStorage from '@react-native-async-storage/async-storage'; |
|
15 | 16 |
|
16 | 17 |
export default function LoginScreen() { |
17 | 18 |
const navigation = useNavigation<NativeStackNavigationProp<RootStackParam>>(); |
... | ... | @@ -43,6 +44,7 @@ |
43 | 44 |
console.log(loginData); // |
44 | 45 |
try { |
45 | 46 |
const response = await loginAPI(loginData); |
47 |
+ await AsyncStorage.setItem('user_id', loginData.id); |
|
46 | 48 |
console.log('Response from server:', response); |
47 | 49 |
} catch (error) { |
48 | 50 |
console.error('Error submitting agreement:', error); |
--- src/screens/SendLocation.tsx
+++ src/screens/SendLocation.tsx
... | ... | @@ -1,4 +1,4 @@ |
1 |
-import React, {useEffect, useRef, useState} from 'react'; |
|
1 |
+import React, { useEffect, useRef, useState } from 'react'; |
|
2 | 2 |
import { |
3 | 3 |
StyleSheet, |
4 | 4 |
View, |
... | ... | @@ -6,58 +6,97 @@ |
6 | 6 |
TouchableOpacity, |
7 | 7 |
Dimensions, |
8 | 8 |
} from 'react-native'; |
9 |
-import {Shadow} from 'react-native-shadow-2'; |
|
9 |
+import { Shadow } from 'react-native-shadow-2'; |
|
10 | 10 |
import Icon from 'react-native-vector-icons/FontAwesome5'; |
11 |
-import {getCurrentPosition} from '../utils/useGeolocationAPI'; |
|
12 |
-import {useNavigation} from '@react-navigation/native'; |
|
13 |
-import {NativeStackNavigationProp} from '@react-navigation/native-stack'; |
|
14 |
-import {RootStackParam} from '../utils/RootStackParam'; |
|
11 |
+import Geolocation from '@react-native-community/geolocation'; |
|
12 |
+import { sendLocationData } from '../utils/useGeolocationAPI'; |
|
13 |
+import { useNavigation } from '@react-navigation/native'; |
|
14 |
+import { NativeStackNavigationProp } from '@react-navigation/native-stack'; |
|
15 |
+import { RootStackParam } from '../utils/RootStackParam'; |
|
15 | 16 |
import AsyncStorage from '@react-native-async-storage/async-storage'; |
16 | 17 |
import BackgroundService from 'react-native-background-actions'; |
17 | 18 |
import crypto from 'crypto-js'; |
18 | 19 |
|
20 |
+let intervalId: NodeJS.Timeout | null = null; |
|
21 |
+ |
|
19 | 22 |
export default function SendLocation() { |
20 | 23 |
const navigation = useNavigation<NativeStackNavigationProp<RootStackParam>>(); |
21 |
- |
|
22 | 24 |
const [time, setTime] = useState<number>(0); |
23 | 25 |
const [time2, setTime2] = useState<number>(0); |
24 | 26 |
const [isRunning, setIsRunning] = useState<boolean>(false); |
25 |
- |
|
27 |
+ const [locations, setLocations] = useState<{ |
|
28 |
+ longitudes: string[], |
|
29 |
+ latitudes: string[], |
|
30 |
+ timestamps: string[] |
|
31 |
+ }>({ longitudes: [], latitudes: [], timestamps: [] }); |
|
32 |
+ const locationsRef = useRef(locations); |
|
26 | 33 |
const [hash, setHash] = useState(""); |
27 |
- |
|
28 | 34 |
const tripIdRef = useRef<string>(hash); |
35 |
+ const userIdRef = useRef<string>(''); |
|
36 |
+ const [watchId, setWatchId] = useState<number | null>(null); |
|
29 | 37 |
|
30 |
- // 해시 |
|
38 |
+ // `hash`가 업데이트될 때마다 `tripIdRef`도 업데이트 |
|
31 | 39 |
useEffect(() => { |
32 | 40 |
tripIdRef.current = hash; |
33 | 41 |
}, [hash]); |
34 | 42 |
|
43 |
+ // `locations`가 업데이트될 때마다 `locationsRef`도 업데이트 |
|
35 | 44 |
useEffect(() => { |
36 |
- var rand = Math.random().toString(); |
|
37 |
- var date = new Date(); |
|
38 |
- var sha256Hash = crypto.SHA256(rand + ',' + date.toString()).toString(crypto.enc.Hex); |
|
39 |
- console.log(rand, date, sha256Hash); |
|
40 |
- setHash(sha256Hash); |
|
45 |
+ locationsRef.current = locations; |
|
46 |
+ }, [locations]); |
|
41 | 47 |
|
42 |
- if (isRunning) { |
|
43 |
- startBackgroundService(); |
|
44 |
- } else { |
|
45 |
- stopBackgroundService(); |
|
46 |
- } |
|
48 |
+ // 컴포넌트 초기화 및 `isRunning` 상태 변경 시 초기화 |
|
49 |
+ useEffect(() => { |
|
50 |
+ const initialize = async () => { |
|
51 |
+ // `user_id`를 AsyncStorage에서 가져옴 |
|
52 |
+ const userId = await AsyncStorage.getItem('user_id'); |
|
53 |
+ if (userId) { |
|
54 |
+ userIdRef.current = userId; |
|
55 |
+ } |
|
56 |
+ |
|
57 |
+ // 해시 값을 생성 |
|
58 |
+ const rand = Math.random().toString(); |
|
59 |
+ const date = new Date(); |
|
60 |
+ const sha256Hash = crypto.SHA256(rand + ',' + date.toString()).toString(crypto.enc.Hex); |
|
61 |
+ setHash(sha256Hash); |
|
62 |
+ |
|
63 |
+ // `isRunning` 상태에 따라 백그라운드 서비스 시작 또는 중지 |
|
64 |
+ if (isRunning) { |
|
65 |
+ await startBackgroundService(); |
|
66 |
+ } else { |
|
67 |
+ await stopBackgroundService(); |
|
68 |
+ } |
|
69 |
+ }; |
|
70 |
+ |
|
71 |
+ initialize(); |
|
47 | 72 |
}, [isRunning]); |
48 | 73 |
|
74 |
+ // `time2` 밀리초 동안 대기하는 함수 |
|
49 | 75 |
const sleep = (time2: number) => new Promise(resolve => setTimeout(() => resolve(true), time2)); |
50 | 76 |
|
77 |
+ // 위치 데이터를 서버로 전송하는 함수 |
|
78 |
+ const sendData = async () => { |
|
79 |
+ const currentLocations = { ...locationsRef.current }; |
|
80 |
+ // 전송할 위치 데이터가 있는지 확인 |
|
81 |
+ if (currentLocations.longitudes.length === 0 || currentLocations.latitudes.length === 0 || currentLocations.timestamps.length === 0) { |
|
82 |
+ console.log("No data to send."); |
|
83 |
+ return; |
|
84 |
+ } |
|
85 |
+ await sendLocationData(userIdRef.current, tripIdRef.current, currentLocations, navigation); |
|
86 |
+ setLocations({ longitudes: [], latitudes: [], timestamps: [] }); |
|
87 |
+ }; |
|
88 |
+ |
|
89 |
+ // 백그라운드 서비스에서 실행될 함수 |
|
51 | 90 |
const veryIntensiveTask = async (taskDataArguments: any) => { |
52 | 91 |
const { delay } = taskDataArguments; |
53 | 92 |
|
54 | 93 |
while (BackgroundService.isRunning()) { |
55 |
- setTime(prevTime => prevTime + 1); |
|
56 |
- getCurrentPosition(tripIdRef, navigation); |
|
57 | 94 |
await sleep(delay); |
95 |
+ await sendData(); |
|
58 | 96 |
} |
59 | 97 |
}; |
60 | 98 |
|
99 |
+ // 백그라운드 서비스 옵션 |
|
61 | 100 |
const options = { |
62 | 101 |
taskName: '측정 서비스', |
63 | 102 |
taskTitle: '측정 중', |
... | ... | @@ -69,42 +108,184 @@ |
69 | 108 |
color: '#ff00ff', |
70 | 109 |
linkingURI: 'sendLocation', |
71 | 110 |
parameters: { |
72 |
- delay: 1000, |
|
111 |
+ delay: 15000, |
|
73 | 112 |
}, |
74 | 113 |
}; |
75 | 114 |
|
115 |
+ // 백그라운드 서비스를 시작하는 함수 |
|
76 | 116 |
const startBackgroundService = async () => { |
77 |
- await BackgroundService.start(veryIntensiveTask, options); |
|
117 |
+ return new Promise<void>((resolve, reject) => { |
|
118 |
+ let latestPosition: GeolocationPosition | null = null; // 마지막 위치를 저장할 변수 |
|
119 |
+ let locationCheckInterval: NodeJS.Timeout | null = null; // 위치 체크 인터벌을 저장할 변수 |
|
120 |
+ let watchId: number | null = null; // 위치 감시 ID를 저장할 변수 |
|
121 |
+ let lastUpdateTime = Date.now(); // 마지막 업데이트 시간을 저장할 변수 |
|
122 |
+ |
|
123 |
+ // 위치 업데이트를 처리하는 함수 |
|
124 |
+ const handleLocationUpdate = (position: GeolocationPosition) => { |
|
125 |
+ latestPosition = position; // 최신 위치를 업데이트 |
|
126 |
+ lastUpdateTime = Date.now(); // 마지막 업데이트 시간 갱신 |
|
127 |
+ |
|
128 |
+ const { longitude, latitude } = position.coords; |
|
129 |
+ const time = new Date().toISOString(); |
|
130 |
+ |
|
131 |
+ // 한국 시간으로 변환하는 함수 |
|
132 |
+ const formatDate = (time) => { |
|
133 |
+ const date = new Date(time); |
|
134 |
+ const year = date.getFullYear(); |
|
135 |
+ const month = `0${date.getMonth() + 1}`.slice(-2); |
|
136 |
+ const day = `0${date.getDate()}`.slice(-2); |
|
137 |
+ const hours = `0${date.getHours()}`.slice(-2); |
|
138 |
+ const minutes = `0${date.getMinutes()}`.slice(-2); |
|
139 |
+ const seconds = `0${date.getSeconds()}`.slice(-2); |
|
140 |
+ const milliseconds = `00${date.getMilliseconds()}`.slice(-3); |
|
141 |
+ |
|
142 |
+ return `${year}-${month}-${day} ${hours}:${minutes}:${seconds}.${milliseconds}`; |
|
143 |
+ }; |
|
144 |
+ |
|
145 |
+ const timestamp = formatDate(time); |
|
146 |
+ |
|
147 |
+ // 위치 데이터를 상태에 추가 |
|
148 |
+ setLocations(prevData => ({ |
|
149 |
+ longitudes: [...prevData.longitudes, longitude.toString()], |
|
150 |
+ latitudes: [...prevData.latitudes, latitude.toString()], |
|
151 |
+ timestamps: [...prevData.timestamps, timestamp] |
|
152 |
+ })); |
|
153 |
+ }; |
|
154 |
+ |
|
155 |
+ // 1초마다 위치 업데이트를 확인하고 3초 동안 업데이트가 없을 경우 마지막 위치를 추가 |
|
156 |
+ locationCheckInterval = setInterval(() => { |
|
157 |
+ if (latestPosition && (Date.now() - lastUpdateTime > 3000)) { |
|
158 |
+ const { longitude, latitude } = latestPosition.coords; |
|
159 |
+ const time = new Date().toISOString(); |
|
160 |
+ const timestamp = formatDate(time); |
|
161 |
+ |
|
162 |
+ setLocations(prevData => ({ |
|
163 |
+ longitudes: [...prevData.longitudes, longitude.toString()], |
|
164 |
+ latitudes: [...prevData.latitudes, latitude.toString()], |
|
165 |
+ timestamps: [...prevData.timestamps, timestamp] |
|
166 |
+ })); |
|
167 |
+ lastUpdateTime = Date.now(); |
|
168 |
+ } |
|
169 |
+ }, 1000); |
|
170 |
+ |
|
171 |
+ // 위치 업데이트를 시작하는 함수 |
|
172 |
+ const startWatchingPosition = () => { |
|
173 |
+ watchId = Geolocation.watchPosition( |
|
174 |
+ handleLocationUpdate, |
|
175 |
+ error => { |
|
176 |
+ console.log('watchPosition Error:', error); |
|
177 |
+ reject(error); |
|
178 |
+ }, |
|
179 |
+ { |
|
180 |
+ enableHighAccuracy: false, // 높은 정확도를 사용할지 여부 |
|
181 |
+ distanceFilter: 0, // 최소 거리 필터 |
|
182 |
+ interval: 1000, // 업데이트 간격 (밀리초) |
|
183 |
+ } |
|
184 |
+ ); |
|
185 |
+ }; |
|
186 |
+ |
|
187 |
+ // watchId가 유효한 경우 백그라운드 서비스 시작 |
|
188 |
+ if (watchId === null) { |
|
189 |
+ (async () => { |
|
190 |
+ try { |
|
191 |
+ await BackgroundService.start(veryIntensiveTask, options); |
|
192 |
+ startWatchingPosition(); // 위치 업데이트 시작 |
|
193 |
+ resolve(); |
|
194 |
+ } catch (error) { |
|
195 |
+ reject(error); |
|
196 |
+ } |
|
197 |
+ })(); |
|
198 |
+ } |
|
199 |
+ |
|
200 |
+ // 백그라운드 서비스를 중지할 때의 정리 함수 |
|
201 |
+ return () => { |
|
202 |
+ if (locationCheckInterval !== null) { |
|
203 |
+ clearInterval(locationCheckInterval); |
|
204 |
+ locationCheckInterval = null; |
|
205 |
+ } |
|
206 |
+ if (watchId !== null) { |
|
207 |
+ Geolocation.clearWatch(watchId); |
|
208 |
+ watchId = null; |
|
209 |
+ } |
|
210 |
+ BackgroundService.stop(); |
|
211 |
+ }; |
|
212 |
+ }); |
|
78 | 213 |
}; |
79 | 214 |
|
215 |
+ // 백그라운드 서비스를 중지하는 함수 |
|
80 | 216 |
const stopBackgroundService = async () => { |
217 |
+ if (watchId !== null) { |
|
218 |
+ Geolocation.clearWatch(watchId); |
|
219 |
+ setWatchId(null); |
|
220 |
+ } |
|
221 |
+ if (intervalId) { |
|
222 |
+ clearInterval(intervalId); |
|
223 |
+ intervalId = null; |
|
224 |
+ } |
|
81 | 225 |
await BackgroundService.stop(); |
82 | 226 |
}; |
83 | 227 |
|
84 |
- // 측정 시작 |
|
228 |
+ // 측정을 시작하는 함수 |
|
85 | 229 |
const handleStart = () => { |
86 | 230 |
setIsRunning(true); |
231 |
+ intervalId = setInterval(() => { |
|
232 |
+ setTime(prevTime => prevTime + 1); |
|
233 |
+ }, 1000); |
|
87 | 234 |
}; |
88 | 235 |
|
89 |
- // 측정 종료 |
|
236 |
+ // 측정을 중지하는 함수 |
|
90 | 237 |
const handleStop = () => { |
238 |
+ // `watchPosition` 제거 |
|
239 |
+ if (watchId !== null) { |
|
240 |
+ Geolocation.clearWatch(watchId); // watchId 제거 |
|
241 |
+ setWatchId(null); // watchId 초기화 |
|
242 |
+ } |
|
243 |
+ |
|
244 |
+ // `intervalId` 제거 |
|
245 |
+ if (intervalId) { |
|
246 |
+ clearInterval(intervalId); |
|
247 |
+ intervalId = null; |
|
248 |
+ } |
|
249 |
+ |
|
250 |
+ // 상태 초기화 |
|
91 | 251 |
setTime(0); |
92 | 252 |
setIsRunning(false); |
253 |
+ |
|
254 |
+ // 백그라운드 서비스가 실행 중인지 확인 |
|
255 |
+ if (BackgroundService.isRunning()) { |
|
256 |
+ // 백그라운드 서비스 중지 |
|
257 |
+ BackgroundService.stop().then(() => { |
|
258 |
+ console.log("Background service stopped successfully."); |
|
259 |
+ // 중지 후 즉시 데이터 전송 |
|
260 |
+ sendData().then(() => { |
|
261 |
+ console.log("Data sent successfully after stopping background service."); |
|
262 |
+ }).catch(error => { |
|
263 |
+ console.log("Error sending data after stopping background service:", error); |
|
264 |
+ }); |
|
265 |
+ }).catch(error => { |
|
266 |
+ console.log("Error stopping background service:", error); |
|
267 |
+ }); |
|
268 |
+ } else { |
|
269 |
+ // 백그라운드 서비스가 실행 중이 아니면 데이터만 전송 |
|
270 |
+ sendData().then(() => { |
|
271 |
+ console.log("Data sent successfully when background service is not running."); |
|
272 |
+ }).catch(error => { |
|
273 |
+ console.log("Error sending data when background service is not running:", error); |
|
274 |
+ }); |
|
275 |
+ } |
|
93 | 276 |
}; |
94 | 277 |
|
95 |
- // 시간 포맷 |
|
278 |
+ // 시간을 형식화하는 함수 |
|
96 | 279 |
const formatTime = (time: number): string => { |
97 |
- console.log(time) |
|
98 |
- const hours = Math.floor(time / 3600); |
|
280 |
+ const hours = Math.floor(time / 3600); |
|
99 | 281 |
const minutes = Math.floor((time % 3600) / 60); |
100 | 282 |
const seconds = time % 60; |
101 |
- return `${hours.toString().padStart(2, '0')}:${minutes.toString().padStart(2, '0')}:${seconds |
|
102 |
- .toString().padStart(2, '0')}`; |
|
283 |
+ return `${hours.toString().padStart(2, '0')}:${minutes.toString().padStart(2, '0')}:${seconds.toString().padStart(2, '0')}`; |
|
103 | 284 |
}; |
104 | 285 |
|
105 | 286 |
return ( |
106 | 287 |
<View style={styles.container}> |
107 |
- <View style={{alignItems: 'center'}}> |
|
288 |
+ <View style={{ alignItems: 'center' }}> |
|
108 | 289 |
<Shadow |
109 | 290 |
distance={8} |
110 | 291 |
startColor={'#3872ef33'} |
... | ... | @@ -112,7 +293,7 @@ |
112 | 293 |
offset={[5, 6]} |
113 | 294 |
containerStyle={styles.watchContainer}> |
114 | 295 |
<View style={styles.watchContainer}> |
115 |
- <Text style={{fontWeight: 'bold', fontSize: 20}}> |
|
296 |
+ <Text style={{ fontWeight: 'bold', fontSize: 20 }}> |
|
116 | 297 |
측정 경과 시간 |
117 | 298 |
</Text> |
118 | 299 |
<Text style={styles.time}> {formatTime(time)} </Text> |
--- src/utils/useGeolocationAPI.ts
+++ src/utils/useGeolocationAPI.ts
... | ... | @@ -1,18 +1,16 @@ |
1 | 1 |
import React from 'react'; |
2 |
-//import {Alert} from 'react-native'; |
|
3 |
-import Geolocation from '@react-native-community/geolocation'; |
|
4 |
- |
|
5 |
-import {getTokenFromStorage, verifyTokens} from './loginUtils'; |
|
2 |
+import { getTokenFromStorage, verifyTokens } from './loginUtils'; |
|
6 | 3 |
import AsyncStorage from '@react-native-async-storage/async-storage'; |
7 |
- |
|
8 |
-import {NativeStackNavigationProp} from '@react-navigation/native-stack'; |
|
9 |
-import {RootStackParam} from '../utils/RootStackParam'; |
|
4 |
+import { NativeStackNavigationProp } from '@react-navigation/native-stack'; |
|
5 |
+import { RootStackParam } from '../utils/RootStackParam'; |
|
10 | 6 |
|
11 | 7 |
// API 주소 |
12 | 8 |
const url = 'http://takensoftai.iptime.org:15857/action/gps_update'; |
13 | 9 |
|
14 |
-export const getCurrentPosition = async ( |
|
15 |
- tripId:String, |
|
10 |
+export const sendLocationData = async ( |
|
11 |
+ userId: string, |
|
12 |
+ tripId: string, |
|
13 |
+ locations: { longitudes: string[], latitudes: string[], timestamps: string[] }, |
|
16 | 14 |
navigation: NativeStackNavigationProp<RootStackParam>, |
17 | 15 |
) => { |
18 | 16 |
try { |
... | ... | @@ -23,71 +21,42 @@ |
23 | 21 |
return; |
24 | 22 |
} |
25 | 23 |
|
26 |
- let initialTimeout = 10000; |
|
27 |
- Geolocation.getCurrentPosition( |
|
28 |
- async pos => { |
|
29 |
- const longitude = JSON.stringify(pos.coords.longitude); |
|
30 |
- const latitude = JSON.stringify(pos.coords.latitude); |
|
31 |
- const date = new Date(); |
|
32 |
- |
|
33 |
- const year = date.getFullYear(); |
|
34 |
- const month = String(date.getMonth() + 1).padStart(2, '0'); |
|
35 |
- const day = String(date.getDate()).padStart(2, '0'); |
|
36 |
- const hours = String(date.getHours()).padStart(2, '0'); |
|
37 |
- const minutes = String(date.getMinutes()).padStart(2, '0'); |
|
38 |
- const seconds = String(date.getSeconds()).padStart(2, '0'); |
|
39 |
- const milliSeconds = date.getMilliseconds().toString().padStart(3, '0'); |
|
40 |
- |
|
41 |
- const date_now = `${year}-${month}-${day} ${hours}:${minutes}:${seconds}.${milliSeconds}`; |
|
42 |
- |
|
43 |
- console.log('trip_id:', tripId, '현재 위치: ', longitude, latitude, '현재 시간:', date_now); |
|
44 |
- try { |
|
45 |
- const response = await fetch(url, { |
|
46 |
- method: 'POST', |
|
47 |
- headers: { |
|
48 |
- 'Content-Type': 'application/json', |
|
49 |
- Authorization: `${token}`, |
|
50 |
- }, |
|
51 |
- body: JSON.stringify({ |
|
52 |
- trip_id: tripId, |
|
53 |
- location_x: longitude, |
|
54 |
- location_y: latitude, |
|
55 |
- timestamp: date_now |
|
56 |
- }), |
|
57 |
- }); |
|
58 |
- |
|
59 |
- const data = await response.json(); |
|
60 |
- console.log('대답 : ', data); |
|
61 |
- |
|
62 |
- if (!response.ok) { |
|
63 |
- console.log('서버 응답 실패 : ', data.msg || response.statusText); |
|
64 |
- } |
|
65 |
- if (data.result === 'fail') { |
|
66 |
- await AsyncStorage.removeItem('token'); |
|
67 |
- } |
|
68 |
- } catch (error) { |
|
69 |
- console.log('서버 요청 오류:', error); |
|
70 |
- } |
|
24 |
+ const requestBody = { |
|
25 |
+ user_id: userId, |
|
26 |
+ trip_id: tripId, |
|
27 |
+ trip_log: { |
|
28 |
+ latitude: locations.latitudes, |
|
29 |
+ longitude: locations.longitudes, |
|
30 |
+ timestamp: locations.timestamps, |
|
71 | 31 |
}, |
72 |
- //error => Alert.alert('GetCurrentPosition Error', JSON.stringify(error)), |
|
73 |
- error => { |
|
74 |
- console.log('GetCurrentPosition Error:', error); |
|
75 |
- }, |
|
76 |
- { |
|
77 |
- enableHighAccuracy: false, |
|
78 |
- timeout: initialTimeout, |
|
79 |
- maximumAge: 10000, |
|
80 |
- }, |
|
81 |
- ); |
|
32 |
+ }; |
|
33 |
+ |
|
34 |
+ console.log('Request body:', requestBody); |
|
35 |
+ |
|
36 |
+ try { |
|
37 |
+ const response = await fetch(url, { |
|
38 |
+ method: 'POST', |
|
39 |
+ headers: { |
|
40 |
+ 'Content-Type': 'application/json', |
|
41 |
+ Authorization: `${token}`, |
|
42 |
+ }, |
|
43 |
+ body: JSON.stringify(requestBody), |
|
44 |
+ }); |
|
45 |
+ |
|
46 |
+ const data = await response.json(); |
|
47 |
+ console.log('Response:', data); |
|
48 |
+ |
|
49 |
+ if (!response.ok) { |
|
50 |
+ console.log('Server response error:', data.msg || response.statusText); |
|
51 |
+ } |
|
52 |
+ if (data.result === 'fail') { |
|
53 |
+ await AsyncStorage.removeItem('token'); |
|
54 |
+ } |
|
55 |
+ } catch (error) { |
|
56 |
+ console.log('Server request error:', error); |
|
57 |
+ } |
|
82 | 58 |
} catch (error) { |
83 |
- console.log('토큰 가져오기 오류:', error); |
|
59 |
+ console.log('Token retrieval error:', error); |
|
84 | 60 |
} |
85 | 61 |
}; |
86 | 62 |
|
87 |
-export default function GetCurrentLocation() { |
|
88 |
- React.useEffect(() => { |
|
89 |
- Geolocation.requestAuthorization(); |
|
90 |
- }, []); |
|
91 |
- |
|
92 |
- return null; |
|
93 |
-} |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?