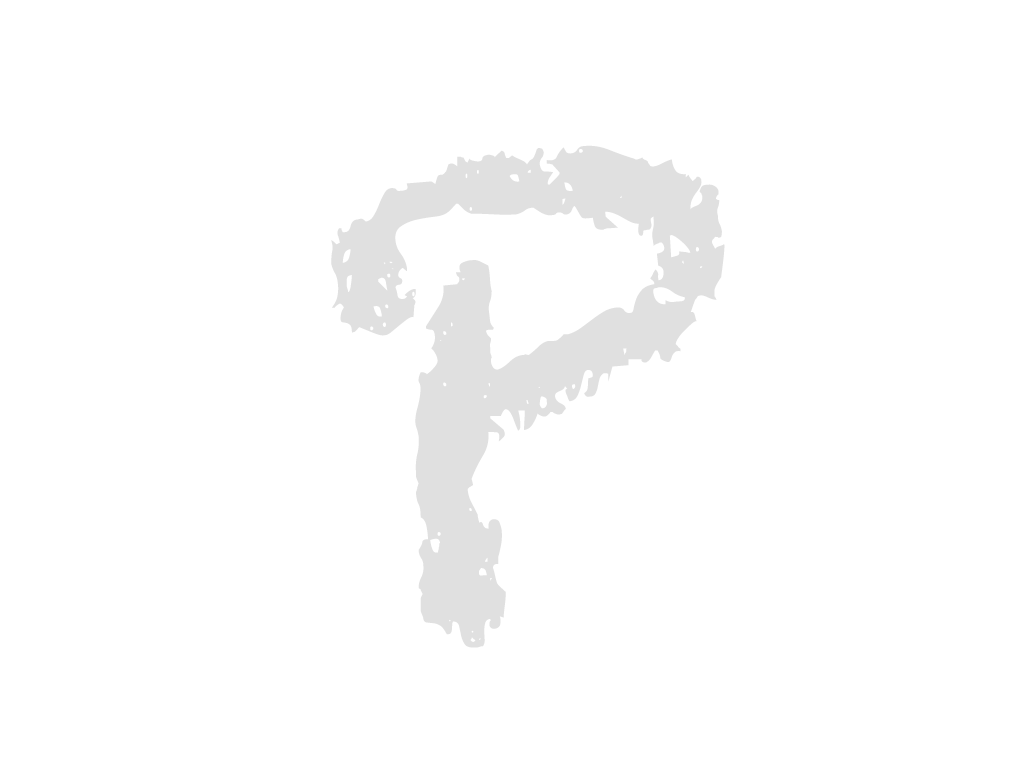
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React, { useEffect, useState, useRef } from 'react';
import { View, Button, StyleSheet, Alert, Text, Linking, TouchableOpacity } from 'react-native';
import { Camera, useCameraDevice } from 'react-native-vision-camera';
import { useNavigation } from '@react-navigation/native';
import Api from '../api/ApiUtils'
const CameraScreen = () => {
const [hasPermission, setHasPermission] = useState(null); // null로 초기화하여 권한 상태가 로딩 중임을 표시
const [isDeviceReady, setIsDeviceReady] = useState(false);
const device = useCameraDevice('front');
const camera = useRef(null);
const navigation = useNavigation();
// Function to check and request camera permission
const checkPermission = async () => {
try {
const cameraPermission = await Camera.getCameraPermissionStatus();
console.log('Current permission status:', cameraPermission);
if (cameraPermission === 'granted') {
setHasPermission(true);
setIsDeviceReady(true);
} else if (cameraPermission === 'not-determined') {
const newCameraPermission = await Camera.requestCameraPermission();
if (newCameraPermission === 'granted') {
setHasPermission(true);
setIsDeviceReady(true);
} else {
setHasPermission(false);
Alert.alert('카메라 권한 필요', '카메라 권한이 필요합니다.', [
{ text: '설정으로 이동', onPress: () => Linking.openSettings() },
{ text: '취소', style: 'cancel' },
]);
}
} else {
setHasPermission(false);
Alert.alert('카메라 권한 필요', '카메라 권한이 필요합니다.', [
{ text: '설정으로 이동', onPress: () => Linking.openSettings() },
{ text: '취소', style: 'cancel' },
]);
}
} catch (error) {
console.error('Permission check error:', error);
Alert.alert('오류', '권한 확인 중 오류가 발생했습니다.');
}
};
useEffect(() => {
checkPermission(); // Check permission once on component mount
}, []);
useEffect(() => {
// Debugging: Check if device is defined and ready
console.log('Device:', device);
if (device) {
setIsDeviceReady(true);
} else {
setIsDeviceReady(false);
}
}, [device]);
const handleCapture = async () => {
if (camera.current) {
try {
const photo = await camera.current.takePhoto({
flash: 'off',
qualityPrioritization: 'speed',
});
navigation.navigate('Gps');
// // API 호출을 비동기로 처리
// const response = await Api.uploadPhoto(uri);
// // 응답 상태 코드가 200일 경우 페이지 이동
// if (response.status === 200) {
// navigation.navigate('Gps');
// } else {
// // 상태 코드가 200이 아닐 경우 에러 메시지 표시
// Alert.alert('업로드 실패', '사진 업로드에 실패했습니다.');
// }
} catch (error) {
console.error('Photo capture error:', error.message);
Alert.alert('오류', '사진 캡처 중 오류가 발생했습니다.');
}
}
};
if (hasPermission === null) {
return (
<View style={styles.permissionContainer}>
<Text>권한을 확인 중입니다...</Text>
</View>
);
}
if (!hasPermission) {
return (
<View style={styles.permissionContainer}>
<Text>카메라 권한이 필요합니다. 설정에서 권한을 허용해 주세요.</Text>
</View>
);
}
return (
<View style={styles.container}>
{isDeviceReady && device ? (
<Camera
ref={camera}
style={StyleSheet.absoluteFill} // Use absoluteFill for full screen camera
device={device}
photo={true}
video={false}
isActive={true}
/>
) : (
<View style={styles.buttonContainer}>
<Text>카메라 장치 준비 중...</Text>
<Button title="다음 페이지로 이동" onPress={() => navigation.navigate('Gps')} />
</View>
)}
<TouchableOpacity onPress={handleCapture} style={styles.captureButton}>
<View style={styles.captureInnerButton} />
</TouchableOpacity>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
position: 'relative', // Ensures that absolute positioning works for capture button
},
buttonContainer: {
justifyContent: 'center',
alignItems: 'center',
flex: 1,
},
captureButton: {
position: 'absolute',
bottom: 40, // Adjust to move the button up or down
width: 70,
height: 70,
borderRadius: 35,
backgroundColor: '#ffffff', // White background for outer button
shadowColor: '#000000',
shadowOffset: { width: 0, height: 4 }, // Slight shadow offset
shadowOpacity: 0.3, // Slight shadow opacity
shadowRadius: 8, // Softer shadow radius
alignItems: 'center',
justifyContent: 'center',
elevation: 5, // For Android shadow effect
},
captureInnerButton: {
width: '70%', // Adjust size of inner button to create a border effect
height: '70%',
borderRadius: 35,
backgroundColor: '#ffffff', // White color for inner button
borderWidth: 4, // Border width to distinguish from outer button
borderColor: '#007AFF', // Blue border color
},
permissionContainer: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default CameraScreen;