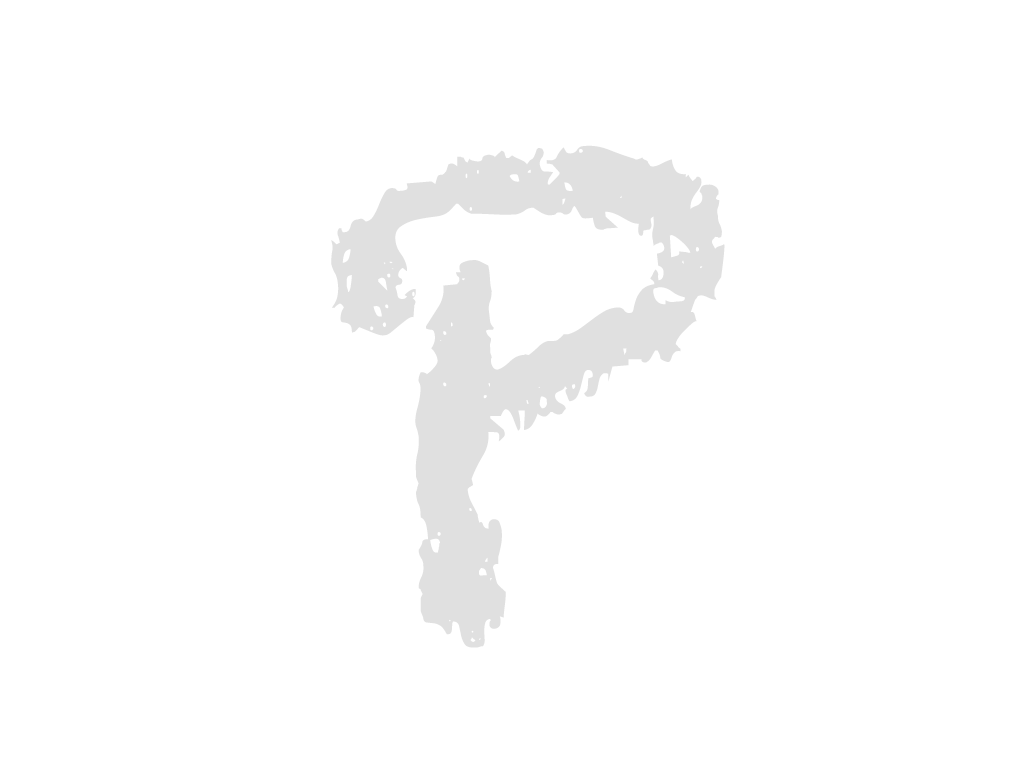
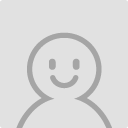
240805 류윤주 커밋
@72964671565916625d07ff37cc8a2090ae2e31e5
--- src/screen/CameraScreen.js
+++ src/screen/CameraScreen.js
... | ... | @@ -1,87 +1,169 @@ |
1 |
-import React, { useEffect, useState } from 'react'; |
|
2 |
-import { View, Text, StyleSheet, Alert } from 'react-native'; |
|
3 |
-import { Camera, useCameraDevice, useCameraPermission } from 'react-native-vision-camera'; |
|
1 |
+import React, { useEffect, useState, useRef } from 'react'; |
|
2 |
+import { View, Button, StyleSheet, Alert, Text, Linking, TouchableOpacity } from 'react-native'; |
|
3 |
+import { Camera, useCameraDevice } from 'react-native-vision-camera'; |
|
4 |
+import { useNavigation } from '@react-navigation/native'; |
|
5 |
+import Api from '../api/ApiUtils' |
|
4 | 6 |
|
5 | 7 |
const CameraScreen = () => { |
6 |
- const [loading, setLoading] = useState(true); |
|
7 |
- const { status: permissionStatus, requestPermission } = useCameraPermission(); |
|
8 |
- const device = useCameraDevice('back'); // 'front' 또는 'back' |
|
8 |
+ const [hasPermission, setHasPermission] = useState(null); // null로 초기화하여 권한 상태가 로딩 중임을 표시 |
|
9 |
+ const [isDeviceReady, setIsDeviceReady] = useState(false); |
|
10 |
+ const device = useCameraDevice('front'); |
|
11 |
+ const camera = useRef(null); |
|
12 |
+ const navigation = useNavigation(); |
|
9 | 13 |
|
10 |
- useEffect(() => { |
|
11 |
- const checkPermissions = async () => { |
|
12 |
- try { |
|
13 |
- console.log('Checking camera permission status...'); |
|
14 |
- if (permissionStatus === 'not-determined') { |
|
15 |
- console.log('Requesting camera permission...'); |
|
16 |
- const newStatus = await requestPermission(); |
|
17 |
- console.log('New camera permission status:', newStatus); |
|
18 |
- } else { |
|
19 |
- console.log('Current permission status:', permissionStatus); |
|
14 |
+ // Function to check and request camera permission |
|
15 |
+ const checkPermission = async () => { |
|
16 |
+ try { |
|
17 |
+ const cameraPermission = await Camera.getCameraPermissionStatus(); |
|
18 |
+ console.log('Current permission status:', cameraPermission); |
|
19 |
+ |
|
20 |
+ if (cameraPermission === 'granted') { |
|
21 |
+ setHasPermission(true); |
|
22 |
+ setIsDeviceReady(true); |
|
23 |
+ } else if (cameraPermission === 'not-determined') { |
|
24 |
+ const newCameraPermission = await Camera.requestCameraPermission(); |
|
25 |
+ if (newCameraPermission === 'granted') { |
|
26 |
+ setHasPermission(true); |
|
27 |
+ setIsDeviceReady(true); |
|
28 |
+ } else { |
|
29 |
+ setHasPermission(false); |
|
30 |
+ Alert.alert('카메라 권한 필요', '카메라 권한이 필요합니다.', [ |
|
31 |
+ { text: '설정으로 이동', onPress: () => Linking.openSettings() }, |
|
32 |
+ { text: '취소', style: 'cancel' }, |
|
33 |
+ ]); |
|
34 |
+ } |
|
35 |
+ } else { |
|
36 |
+ setHasPermission(false); |
|
37 |
+ Alert.alert('카메라 권한 필요', '카메라 권한이 필요합니다.', [ |
|
38 |
+ { text: '설정으로 이동', onPress: () => Linking.openSettings() }, |
|
39 |
+ { text: '취소', style: 'cancel' }, |
|
40 |
+ ]); |
|
41 |
+ } |
|
42 |
+ } catch (error) { |
|
43 |
+ console.error('Permission check error:', error); |
|
44 |
+ Alert.alert('오류', '권한 확인 중 오류가 발생했습니다.'); |
|
20 | 45 |
} |
21 |
- } catch (error) { |
|
22 |
- console.error('Error checking permissions:', error); |
|
23 |
- Alert.alert('Error', 'An error occurred while checking permissions.'); |
|
24 |
- } |
|
25 | 46 |
}; |
26 | 47 |
|
27 |
- checkPermissions(); |
|
28 |
- }, [permissionStatus, requestPermission]); |
|
48 |
+ useEffect(() => { |
|
49 |
+ checkPermission(); // Check permission once on component mount |
|
50 |
+ }, []); |
|
29 | 51 |
|
30 |
- useEffect(() => { |
|
31 |
- console.log('Device:', device); // 디바이스 정보 로그 추가 |
|
52 |
+ useEffect(() => { |
|
53 |
+ // Debugging: Check if device is defined and ready |
|
54 |
+ console.log('Device:', device); |
|
55 |
+ if (device) { |
|
56 |
+ setIsDeviceReady(true); |
|
57 |
+ } else { |
|
58 |
+ setIsDeviceReady(false); |
|
59 |
+ } |
|
60 |
+ }, [device]); |
|
32 | 61 |
|
33 |
- if (device) { |
|
34 |
- console.log('Camera device loaded:', device); |
|
35 |
- setLoading(false); |
|
36 |
- } else { |
|
37 |
- console.log('No camera device found'); |
|
38 |
- setLoading(false); // No device found 시에도 로딩 상태를 false로 설정 |
|
39 |
- } |
|
40 |
- }, [device]); |
|
62 |
+ const handleCapture = async () => { |
|
63 |
+ if (camera.current) { |
|
64 |
+ try { |
|
65 |
+ const photo = await camera.current.takePhoto({ |
|
66 |
+ flash: 'off', |
|
67 |
+ qualityPrioritization: 'speed', |
|
68 |
+ }); |
|
69 |
+ navigation.navigate('Gps'); |
|
70 |
+ // // API 호출을 비동기로 처리 |
|
71 |
+ // const response = await Api.uploadPhoto(uri); |
|
72 |
+ |
|
73 |
+ // // 응답 상태 코드가 200일 경우 페이지 이동 |
|
74 |
+ // if (response.status === 200) { |
|
75 |
+ // navigation.navigate('Gps'); |
|
76 |
+ // } else { |
|
77 |
+ // // 상태 코드가 200이 아닐 경우 에러 메시지 표시 |
|
78 |
+ // Alert.alert('업로드 실패', '사진 업로드에 실패했습니다.'); |
|
79 |
+ // } |
|
80 |
+ } catch (error) { |
|
81 |
+ console.error('Photo capture error:', error.message); |
|
82 |
+ Alert.alert('오류', '사진 캡처 중 오류가 발생했습니다.'); |
|
83 |
+ } |
|
84 |
+ } |
|
85 |
+ }; |
|
41 | 86 |
|
42 |
- const renderCamera = () => { |
|
43 |
- if (loading) { |
|
44 |
- return <Text>Loading camera...</Text>; |
|
87 |
+ if (hasPermission === null) { |
|
88 |
+ return ( |
|
89 |
+ <View style={styles.permissionContainer}> |
|
90 |
+ <Text>권한을 확인 중입니다...</Text> |
|
91 |
+ </View> |
|
92 |
+ ); |
|
45 | 93 |
} |
46 | 94 |
|
47 |
- if (!device) { |
|
48 |
- return <Text>No camera device found</Text>; |
|
95 |
+ if (!hasPermission) { |
|
96 |
+ return ( |
|
97 |
+ <View style={styles.permissionContainer}> |
|
98 |
+ <Text>카메라 권한이 필요합니다. 설정에서 권한을 허용해 주세요.</Text> |
|
99 |
+ </View> |
|
100 |
+ ); |
|
49 | 101 |
} |
50 | 102 |
|
51 |
- if (permissionStatus === 'granted') { |
|
52 |
- return <Camera style={styles.camera} device={device} isActive={true} photo={true}/>; |
|
53 |
- } else { |
|
54 |
- return ( |
|
55 |
- <View style={styles.noPermission}> |
|
56 |
- <Text>Camera access is not granted. Please enable camera permissions in settings.</Text> |
|
103 |
+ return ( |
|
104 |
+ <View style={styles.container}> |
|
105 |
+ {isDeviceReady && device ? ( |
|
106 |
+ <Camera |
|
107 |
+ ref={camera} |
|
108 |
+ style={StyleSheet.absoluteFill} // Use absoluteFill for full screen camera |
|
109 |
+ device={device} |
|
110 |
+ photo={true} |
|
111 |
+ video={false} |
|
112 |
+ isActive={true} |
|
113 |
+ /> |
|
114 |
+ ) : ( |
|
115 |
+ <View style={styles.buttonContainer}> |
|
116 |
+ <Text>카메라 장치 준비 중...</Text> |
|
117 |
+ <Button title="다음 페이지로 이동" onPress={() => navigation.navigate('Gps')} /> |
|
118 |
+ </View> |
|
119 |
+ )} |
|
120 |
+ <TouchableOpacity onPress={handleCapture} style={styles.captureButton}> |
|
121 |
+ <View style={styles.captureInnerButton} /> |
|
122 |
+ </TouchableOpacity> |
|
57 | 123 |
</View> |
58 |
- ); |
|
59 |
- } |
|
60 |
- }; |
|
61 |
- |
|
62 |
- return ( |
|
63 |
- <View style={styles.container}> |
|
64 |
- {renderCamera()} |
|
65 |
- </View> |
|
66 |
- ); |
|
124 |
+ ); |
|
67 | 125 |
}; |
68 | 126 |
|
69 | 127 |
const styles = StyleSheet.create({ |
70 |
- container: { |
|
71 |
- flex: 1, |
|
72 |
- justifyContent: 'center', |
|
73 |
- alignItems: 'center', |
|
74 |
- }, |
|
75 |
- camera: { |
|
76 |
- width: '100%', |
|
77 |
- height: '100%', |
|
78 |
- }, |
|
79 |
- noPermission: { |
|
80 |
- flex: 1, |
|
81 |
- justifyContent: 'center', |
|
82 |
- alignItems: 'center', |
|
83 |
- padding: 20, |
|
84 |
- }, |
|
128 |
+ container: { |
|
129 |
+ flex: 1, |
|
130 |
+ justifyContent: 'center', |
|
131 |
+ alignItems: 'center', |
|
132 |
+ position: 'relative', // Ensures that absolute positioning works for capture button |
|
133 |
+ }, |
|
134 |
+ buttonContainer: { |
|
135 |
+ justifyContent: 'center', |
|
136 |
+ alignItems: 'center', |
|
137 |
+ flex: 1, |
|
138 |
+ }, |
|
139 |
+ captureButton: { |
|
140 |
+ position: 'absolute', |
|
141 |
+ bottom: 40, // Adjust to move the button up or down |
|
142 |
+ width: 70, |
|
143 |
+ height: 70, |
|
144 |
+ borderRadius: 35, |
|
145 |
+ backgroundColor: '#ffffff', // White background for outer button |
|
146 |
+ shadowColor: '#000000', |
|
147 |
+ shadowOffset: { width: 0, height: 4 }, // Slight shadow offset |
|
148 |
+ shadowOpacity: 0.3, // Slight shadow opacity |
|
149 |
+ shadowRadius: 8, // Softer shadow radius |
|
150 |
+ alignItems: 'center', |
|
151 |
+ justifyContent: 'center', |
|
152 |
+ elevation: 5, // For Android shadow effect |
|
153 |
+ }, |
|
154 |
+ captureInnerButton: { |
|
155 |
+ width: '70%', // Adjust size of inner button to create a border effect |
|
156 |
+ height: '70%', |
|
157 |
+ borderRadius: 35, |
|
158 |
+ backgroundColor: '#ffffff', // White color for inner button |
|
159 |
+ borderWidth: 4, // Border width to distinguish from outer button |
|
160 |
+ borderColor: '#007AFF', // Blue border color |
|
161 |
+ }, |
|
162 |
+ permissionContainer: { |
|
163 |
+ flex: 1, |
|
164 |
+ justifyContent: 'center', |
|
165 |
+ alignItems: 'center', |
|
166 |
+ }, |
|
85 | 167 |
}); |
86 | 168 |
|
87 | 169 |
export default CameraScreen; |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?