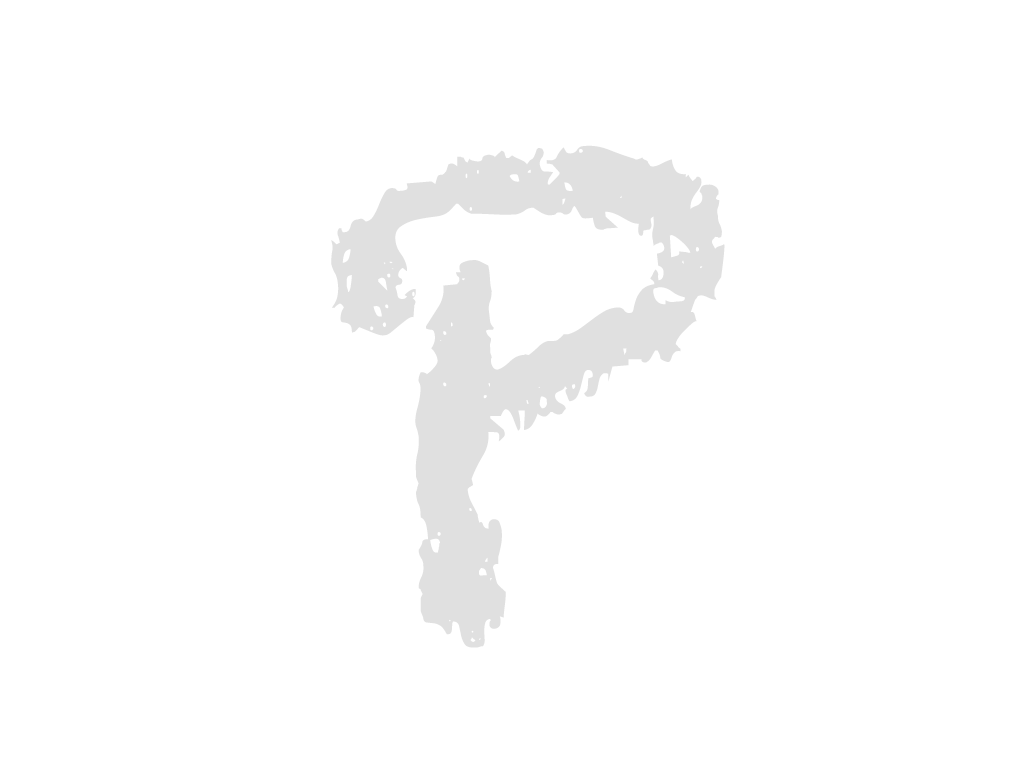
--- client/views/component/Modal_Guadian.jsx
+++ client/views/component/Modal_Guadian.jsx
... | ... | @@ -68,39 +68,45 @@ |
68 | 68 |
}); |
69 | 69 |
}; |
70 | 70 |
// 보호자 등록 영역 |
71 |
- const test = () => { |
|
72 |
- console.log("userName : ", userName); |
|
73 |
- console.log("gender : ", gender); |
|
74 |
- console.log("brith : ", brith); |
|
75 |
- console.log("telNum : ", telNum); |
|
76 |
- console.log("homeAddress : ", homeAddress); |
|
77 |
- console.log("relationship : ", relationship); |
|
78 |
- console.log("matchState : ", matchState); |
|
79 |
- } |
|
80 | 71 |
const insertUser = () => { |
81 |
- fetch("/user/insertSeniorData.json", { |
|
82 |
- method: "POST", |
|
83 |
- headers: { |
|
84 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
85 |
- }, |
|
86 |
- body: JSON.stringify({ |
|
87 |
- user_name: userName, |
|
88 |
- user_gender: gender != "" ? gender : null, |
|
89 |
- user_birth: brith, |
|
90 |
- user_phonenumber: telNum, |
|
91 |
- user_address: homeAddress != "" ? homeAddress : null, |
|
92 |
- agency_id: 'agency01', |
|
93 |
- government_id: 'government01', |
|
94 |
- user_code: '3', |
|
72 |
+ if(userName == ""){ |
|
73 |
+ alert("이름을 입력해 주세요."); |
|
74 |
+ return ; |
|
75 |
+ } else if(brith == "") { |
|
76 |
+ alert("생년월일을 선택해 주세요."); |
|
77 |
+ return ; |
|
78 |
+ } else if(telNum == "") { |
|
79 |
+ alert("연락처를 입력해 선택해 주세요."); |
|
80 |
+ return ; |
|
81 |
+ } |
|
82 |
+ var insertBtn = confirm("등록하시겠습니까?"); |
|
83 |
+ if (insertBtn) { |
|
84 |
+ fetch("/user/insertSeniorData.json", { |
|
85 |
+ method: "POST", |
|
86 |
+ headers: { |
|
87 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
88 |
+ }, |
|
89 |
+ body: JSON.stringify({ |
|
90 |
+ user_name: userName, |
|
91 |
+ user_gender: gender != "" ? gender : null, |
|
92 |
+ user_birth: brith, |
|
93 |
+ user_phonenumber: telNum, |
|
94 |
+ user_address: homeAddress != "" ? homeAddress : null, |
|
95 |
+ agency_id: 'agency01', |
|
96 |
+ government_id: 'government01', |
|
97 |
+ user_code: '3', |
|
95 | 98 |
|
96 |
- }), |
|
97 |
- }).then((response) => response.json()).then((data) => { |
|
98 |
- console.log("보호자 등록"); |
|
99 |
- insertGuadian(); |
|
100 |
- }).catch((error) => { |
|
101 |
- console.log('insertUser() /user/insertSeniorData.json error : ', error); |
|
102 |
- }); |
|
103 |
- }; |
|
99 |
+ }), |
|
100 |
+ }).then((response) => response.json()).then((data) => { |
|
101 |
+ console.log("보호자 등록"); |
|
102 |
+ insertGuadian(); |
|
103 |
+ }).catch((error) => { |
|
104 |
+ console.log('insertUser() /user/insertSeniorData.json error : ', error); |
|
105 |
+ }); |
|
106 |
+ } else { |
|
107 |
+ return ; |
|
108 |
+ } |
|
109 |
+ } |
|
104 | 110 |
|
105 | 111 |
const insertGuadian = () => { |
106 | 112 |
fetch("/user/insertGuardian.json", { |
... | ... | @@ -203,7 +209,6 @@ |
203 | 209 |
className={"btn-small green-btn"} |
204 | 210 |
btnName={"추가"} |
205 | 211 |
onClick={() => { |
206 |
- test(); |
|
207 | 212 |
insertUser() |
208 | 213 |
getselectMatchList(); |
209 | 214 |
}} |
--- client/views/component/Modal_SeniorInsert.jsx
+++ client/views/component/Modal_SeniorInsert.jsx
... | ... | @@ -64,6 +64,23 @@ |
64 | 64 |
//-------- 등록 버튼 동작 수행 영역 시작 --------// |
65 | 65 |
// 대상자 정보 등록을 위한 함수 |
66 | 66 |
const InsertUserData = () => { |
67 |
+ //-------- 유효성 체크 --------// |
|
68 |
+ if(userName == ""){ |
|
69 |
+ alert("이름을 입력해 주세요."); |
|
70 |
+ return ; |
|
71 |
+ } else if(gender == "") { |
|
72 |
+ alert("성별을 선택해 주세요."); |
|
73 |
+ return ; |
|
74 |
+ } else if(brith == "") { |
|
75 |
+ alert("생년월일을 선택해 주세요."); |
|
76 |
+ return ; |
|
77 |
+ } else if(telNum == "") { |
|
78 |
+ alert("연락처를 입력해 선택해 주세요."); |
|
79 |
+ return ; |
|
80 |
+ } else if(homeAddress == "") { |
|
81 |
+ alert("주소를 선택해 주세요."); |
|
82 |
+ return ; |
|
83 |
+ } |
|
67 | 84 |
var insertBtn = confirm("등록하시겠습니까?"); |
68 | 85 |
if (insertBtn) { |
69 | 86 |
fetch("/user/insertSeniorData.json", { |
--- client/views/component/Table.jsx
+++ client/views/component/Table.jsx
... | ... | @@ -3,7 +3,6 @@ |
3 | 3 |
import Modal_Matching from "./Modal_Matching.jsx"; |
4 | 4 |
import Modal_Guadian from "./Modal_Guadian.jsx"; |
5 | 5 |
import { useNavigate } from "react-router"; |
6 |
-import { Link } from 'react-router-dom'; |
|
7 | 6 |
// import styled from "styled-components"; |
8 | 7 |
|
9 | 8 |
export default function Table({ head, contents, contentKey, onClick, className, view, offset, limit }) { |
... | ... | @@ -106,8 +105,17 @@ |
106 | 105 |
return ( |
107 | 106 |
<> |
108 | 107 |
<td onClick={() => |
109 |
- navigate(`/SeniorSelectOne/${i.user_id}`, { state: { index } })}> |
|
108 |
+ { |
|
109 |
+ // 대상자 페이지일 때만 상세페이지로 이동하도록 설정 |
|
110 |
+ if(view == 'mySenior' || view == 'allSenior') { |
|
111 |
+ navigate(`/SeniorSelectOne/${i.user_id}`) |
|
112 |
+ } else { |
|
113 |
+ return ; |
|
114 |
+ } |
|
115 |
+ } |
|
116 |
+ }> |
|
110 | 117 |
{i[kes]} |
118 |
+ |
|
111 | 119 |
</td> |
112 | 120 |
</> |
113 | 121 |
) |
--- client/views/pages/AppRoute.jsx
+++ client/views/pages/AppRoute.jsx
... | ... | @@ -22,7 +22,7 @@ |
22 | 22 |
import Main_guardian from "./main/Main_guardian.jsx"; |
23 | 23 |
import Main_agency from "./main/Main_agency.jsx"; |
24 | 24 |
import Main from "./main/Main.jsx"; |
25 |
-import SeniorInsert from "./senior_management/SeniorInsert.jsx"; |
|
25 |
+import SeniorInsert from "./senior_management/SeniorEdit.jsx"; |
|
26 | 26 |
import SeniorSelectOne from "./senior_management/SeniorSelectOne.jsx"; |
27 | 27 |
import MedicineCareSelectOne from "./healthcare/medicinecare/MedicineCareSelectOne.jsx"; |
28 | 28 |
import TemperatureManagementSelectOne from "./healthcare/temperature/TemperatureManagementSelectOne.jsx"; |
--- client/views/pages/senior_management/SeniorInsert.jsx
+++ client/views/pages/senior_management/SeniorEdit.jsx
No changes |
--- client/views/pages/senior_management/SeniorSelectOne.jsx
+++ client/views/pages/senior_management/SeniorSelectOne.jsx
... | ... | @@ -9,6 +9,33 @@ |
9 | 9 |
const navigate = useNavigate(); |
10 | 10 |
let { seniorId } = useParams(); |
11 | 11 |
console.log("seniorId : ", seniorId); |
12 |
+ |
|
13 |
+ const [seniortOne, setSeniorOne] = React.useState([]); |
|
14 |
+ |
|
15 |
+ //-------- 상세페이지 선택된 대상자의 정보 불러오기 --------// |
|
16 |
+ const getSeniorDataOne = () => { |
|
17 |
+ fetch("/user/selectSeniorOne.json", { |
|
18 |
+ method: "POST", |
|
19 |
+ headers: { |
|
20 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
21 |
+ }, |
|
22 |
+ body: JSON.stringify({ |
|
23 |
+ user_id: seniorId |
|
24 |
+ }), |
|
25 |
+ }).then((response) => response.json()).then((data) => { |
|
26 |
+ console.log("data : ", data[0]); |
|
27 |
+ setSeniorOne(data[0]); |
|
28 |
+ |
|
29 |
+ }).catch((error) => { |
|
30 |
+ console.log('getSeniorDataOne() /user/selectSeniorOne.json error : ', error); |
|
31 |
+ }); |
|
32 |
+ }; |
|
33 |
+ |
|
34 |
+ |
|
35 |
+ React.useEffect(() => { |
|
36 |
+ getSeniorDataOne(); |
|
37 |
+ |
|
38 |
+ }, []) |
|
12 | 39 |
return ( |
13 | 40 |
<main> |
14 | 41 |
<div className="content-wrap row"> |
... | ... | @@ -19,48 +46,48 @@ |
19 | 46 |
<tr> |
20 | 47 |
<th>이름</th> |
21 | 48 |
<td> |
22 |
- <span></span> |
|
49 |
+ <span>{seniortOne.user_name}</span> |
|
23 | 50 |
</td> |
24 | 51 |
<th>성별</th> |
25 | 52 |
<td> |
26 |
- <span></span> |
|
53 |
+ <span>{seniortOne.user_gender}</span> |
|
27 | 54 |
</td> |
28 | 55 |
</tr> |
29 | 56 |
<tr> |
30 | 57 |
<th>생년월일</th> |
31 | 58 |
<td> |
32 |
- <span></span> |
|
59 |
+ <span>{seniortOne.user_birth}</span> |
|
33 | 60 |
</td> |
34 | 61 |
<th>연락처</th> |
35 | 62 |
<td> |
36 |
- <span></span> |
|
63 |
+ <span>{seniortOne.user_phonenumber}</span> |
|
37 | 64 |
</td> |
38 | 65 |
</tr> |
39 | 66 |
<tr> |
40 | 67 |
<th>주소</th> |
41 | 68 |
<td> |
42 |
- <span>1등급</span> |
|
69 |
+ <span>{seniortOne.user_address}</span> |
|
43 | 70 |
</td> |
44 | 71 |
|
45 | 72 |
</tr> |
46 | 73 |
<tr> |
47 | 74 |
<th>필요복약</th> |
48 | 75 |
<td className="flex-start"> |
49 |
- <span>아침</span> |
|
50 |
- <span>점심</span> |
|
51 |
- <span>저녁</span> |
|
76 |
+ {seniortOne.breakfast_medication_check ? <span>아침</span> : <></>} |
|
77 |
+ {seniortOne.lunch_medication_check ? <span>점심</span> : <></>} |
|
78 |
+ {seniortOne.dinner_medication_check ? <span>저녁</span> : <></>} |
|
52 | 79 |
</td> |
53 | 80 |
</tr> |
54 | 81 |
<tr> |
55 | 82 |
<th>복용중인 약</th> |
56 | 83 |
<td colSpan={3}> |
57 |
- <span>혈압약</span> |
|
84 |
+ <span className="medicine" cols="30" rows="2">{seniortOne.medication_pill}</span> |
|
58 | 85 |
</td> |
59 | 86 |
</tr> |
60 | 87 |
<tr> |
61 | 88 |
<th>비고</th> |
62 |
- <td colSpan={3} style={{height:"300px"}}> |
|
63 |
- <span></span> |
|
89 |
+ <td colSpan={3}> |
|
90 |
+ <span className="note" cols="30" rows="2">{seniortOne.senior_note}</span> |
|
64 | 91 |
</td> |
65 | 92 |
</tr> |
66 | 93 |
</tbody> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?