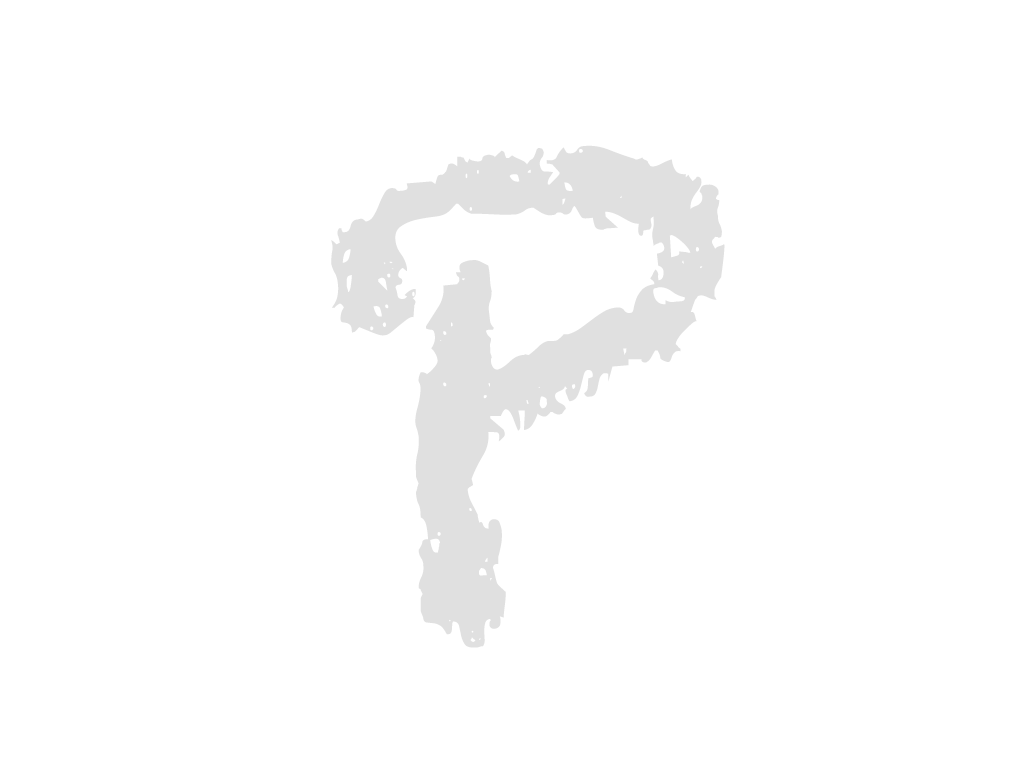
File name
Commit message
Commit date
File name
Commit message
Commit date
import React from "react";
import Button from "./Button.jsx";
import SubTitle from "./SubTitle.jsx";
export default function Modal({ open, close, header, setModalOpen3, setAddSenior }) {
// 사용자 등록 시 초기값 세팅
const [userName, setUserName] = React.useState("");
const [gender, setGender] = React.useState("");
const [brith, setBrith] = React.useState("");
const [telNum, setTelNum] = React.useState("");
const [homeAddress, setHomeAddress] = React.useState("");
const [medicineM, setMedicineM] = React.useState(false);
const [medicineL, setMedicineL] = React.useState(false);
const [medicineD, setMedicineD] = React.useState(false);
const [medication, setMedication] = React.useState("");
const [note, setNote] = React.useState("");
// 등록 후 초기화 진행
const dataReset = () => {
setUserName("");
setGender("");
setBrith("");
setTelNum("");
setHomeAddress("");
setMedicineM("");
setMedicineL("");
setMedicineD("");
setMedication("");
setNote("");
}
//-------- 변경되는 데이터 Handler 설정 --------//
const handleUserName = (e) => {
setUserName(e.target.value);
};
const handleGender = (e) => {
setGender(e.target.value);
};
const handleBrithday = (e) => {
setBrith(e.target.value);
};
const handleTelNum = (e) => {
e.target.value = e.target.value.replace(/[^0-9]/g, '').replace(/^(\d{2,3})(\d{3,4})(\d{4})$/, `$1-$2-$3`);
setTelNum(e.target.value);
};
const handleHomeAddress = (e) => {
setHomeAddress(e.target.value);
};
const handleMedicineM = (e) => {
setMedicineM(e.target.checked);
};
const handleMedicineL = (e) => {
setMedicineL(e.target.checked);
};
const handleMedicineD = (e) => {
setMedicineD(e.target.checked);
};
const handleMedication = (e) => {
setMedication(e.target.value);
};
const handleNote = (e) => {
setNote(e.target.value);
};
//-------- 등록 버튼 동작 수행 영역 시작 --------//
// 대상자 정보 등록을 위한 함수
const InsertUserData = () => {
var insertBtn = confirm("등록하시겠습니까?");
if (insertBtn) {
fetch("/user/insertSeniorData.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({
user_name: userName,
user_gender: gender,
user_birth: brith,
user_phonenumber: telNum,
user_address: homeAddress,
agency_id: 'agency01',
government_id: 'government01',
user_code: '4',
}),
}).then((response) => response.json()).then((data) => {
console.log("대상자 정보 등록");
InsertUserPillData();
}).catch((error) => {
console.log('insertSeniorData() /user/insertSeniorData.json error : ', error);
});
} else {
return;
}
};
// 대상자 약 복용 정보 등록을 위한 함수
const InsertUserPillData = () => {
fetch("/user/insertSeniorMadication.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({
user_phonenumber: telNum,
breakfast_medication_check: medicineM,
lunch_medication_check: medicineL,
dinner_medication_check: medicineD,
medication_pill: medication,
senior_note: note,
}),
}).then((response) => response.json()).then((data) => {
console.log("약 정보 등록");
dataReset();
setAddSenior(true)
alert("등록 되었습니다.");
noticeModalClose()
}).catch((error) => {
console.log('InsertUserPillData() /user/insertSeniorMadication.json error : ', error);
});
};
//-------- 등록 버튼 동작 수행 영역 끝 --------/
//-------- 모달 종료 -------->
const noticeModalClose = () => {
setModalOpen3(false);
};
return (
<div class={open ? "openModal modal" : "modal"}>
{open ? (
<div className="modal-inner">
<div className="modal-header flex">
{header}
<Button className={"close"} onClick={noticeModalClose} btnName={"X"} />
</div>
<div className="modal-main">
<div className="board-wrap">
<SubTitle explanation={"회원 등록 시 ID는 연락처, 패스워드는 생년월일 8자리입니다."} className="margin-bottom" />
<table className="margin-bottom2 senior-insert">
{/* <tr>
<th>대상자등록번호</th>
<td colSpan={3} className="flex">
<input type="text" placeholder="생성하기 버튼 클릭 시 자동으로 생성됩니다."/>
<Button
className={"btn-large gray-btn"}
btnName={"생성하기"}
/>
</td>
</tr> */}
<tr>
<th>이름</th>
<td>
<input type="text" value={userName} onChange={handleUserName} />
</td>
<th>성별</th>
<td className="flex-start gender">
<div className="flex-start">
<input type="radio" name="genderSelect" value="남" onChange={handleGender} />
<label for="gender">남</label>
</div>
<div className="flex-start">
<input type="radio" name="genderSelect" value="여" onChange={handleGender} />
<label for="gender">여</label>
</div>
</td>
</tr>
<tr>
<th>생년월일</th>
<td>
<div className="flex">
<input type='date' value={brith} onChange={handleBrithday} />
</div>
</td>
{/* <th>요양등급</th>
<td>
<input type="text" />
</td> */}
</tr>
<tr>
<th>연락처</th>
<td colSpan={3}>
<input type="text" maxLength="13" value={telNum} onChange={handleTelNum} />
</td>
</tr>
<tr>
<th>주소</th>
<td colSpan={3}>
<input type="text" value={homeAddress} onChange={handleHomeAddress} />
</td>
</tr>
<tr>
<th>필요 복약</th>
<td>
<div className="flex">
<input type="checkbox" name="medicationSelect" checked={medicineM} onClick={(e) => { handleMedicineM(e) }} /><label for="medicationTime">아침</label>
<input type="checkbox" name="medicationSelect" checked={medicineL} onClick={(e) => { handleMedicineL(e) }} /><label for="medicationTime">점심</label>
<input type="checkbox" name="medicationSelect" checked={medicineD} onClick={(e) => { handleMedicineD(e) }} /><label for="medicationTime">저녁</label>
</div>
</td>
</tr>
<tr>
<th>복용중인 약</th>
<td colSpan={3}>
<textarea className="medicine" cols="30" rows="2" value={medication} onChange={handleMedication}></textarea>
</td>
</tr>
<tr>
<th>비고</th>
<td colSpan={3}>
<textarea className="note" cols="30" rows="2" value={note} onChange={handleNote}></textarea>
</td>
</tr>
{/* <tr>
<th>기저질환</th>
<td colSpan={3}>
<textarea cols="30" rows="10"></textarea>
</td>
</tr> */}
</table>
<div className="btn-wrap flex-center">
<Button
className={"btn-small gray-btn"}
btnName={"등록"}
onClick={() => {
InsertUserData();
}}
/>
</div>
</div>
</div>
</div>
) : null}
</div>
);
}