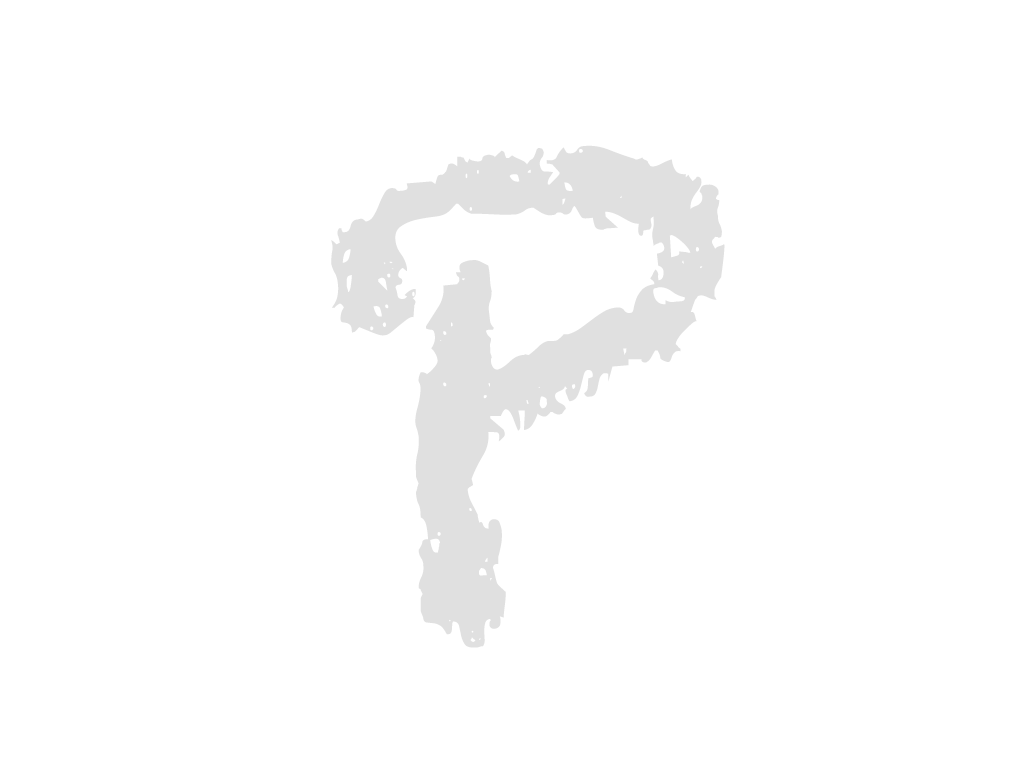
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
2023-03-06
2023-03-08
File name
Commit message
Commit date
2023-03-08
import React, { useState } from "react";
import ContentTitle from "../../component/ContentTitle.jsx";
import SubTitle from "../../component/SubTitle.jsx";
import Modal from "../../component/Modal.jsx";
import Table from "../../component/Table.jsx";
import Button from "../../component/Button.jsx";
import Category from "../../component/Category.jsx";
import { width } from "@mui/system";
export default function UserAuthoriySelect() {
const [agencyName, setAgencyName] = useState("시행기관")
const [modalOpen, setModalOpen] = React.useState(false);
const openModal = () => {
setModalOpen(true);
};
const closeModal = () => {
setModalOpen(false);
};
const [modalOpen2, setModalOpen2] = React.useState(false);
const openModal2 = () => {
setModalOpen2(true);
};
const closeModal2 = () => {
setModalOpen2(false);
};
const thead = [
"No",
"소속기관명",
"이름",
"연락처",
"성별",
"주소",
"담당 대상자(어르신) 인원",
];
const key = [
"No",
"center",
"name",
"phone",
"gender",
"address",
"worker",
];
const content = [
{
No: 1,
center: "A복지관",
name: "홍길동",
phone: "010-1234-1234",
gender: "여",
address: "경상북도 군위군 삼국유사면",
worker: "10명"
},
];
const thead1 = [
"No",
"이름",
"대상자등록번호",
"생년월일",
"성별",
"연락처",
"주소",
"보호자",
];
const key1 = ["No",
"name",
"management_number",
"birth",
"gender",
"phone",
"address",
"family",
];
const content1 = [
{
No: 1,
name: "김복남",
management_number: 2022080101,
birth: "1950.02.03",
gender: "남",
phone: "010-1234-1234",
address: "경상북도 군위군 삼국유사면",
family: (
<Button
className={"btn-small lightgray-btn"}
btnName={"보호자(가족) 보기"}
onClick={openModal}
/>
),
},
];
const thead3 = [
"No",
"사용자명",
"사용자ID",
"대상자와의 관계",
"보호자 연락처",
];
const key3 = [
"No",
"name",
"Id",
"relationship",
"phone",
];
const content3 = [
{
No: 1,
name: "김훈",
Id: "admin2",
relationship: "아들",
phone: "010-1234-1234",
},
];
const data = [
{
id: 1,
title: "대상자(사용자)",
description: (
<Table
className={"protector-user"}
head={thead1}
contents={content1}
contentKey={key1}
onClick={() => {
navigate("/SeniorSelectOne");
}}
/>
),
},
{
id: 2,
title: "복지사(간호사)",
description: (
<Table
className={"senior-table"}
head={thead}
contents={content}
contentKey={key}
onClick={() => {
navigate("/SeniorSelectOne");
}}
/>
),
},
]
const [index, setIndex] = React.useState(1);
return (
<main>
<Modal open={modalOpen} close={closeModal} header="'김복남'님의 가족">
<div className="board-wrap">
<SubTitle explanation={"최초 로그인 ID는 연락처, PW는 생년월일 8자리입니다."} className="margin-bottom" />
<table className="margin-bottom2 senior-insert">
<tr>
<th>이름</th>
<td>
<input type="text" />
</td>
<th>생년월일</th>
<td>
<div className="flex">
<select name="year" id="year">
<option value="">년</option>
</select>
<select name="month" id="month">
<option value="">월</option>
</select>
<select name="days" id="days">
<option value="">일</option>
</select>
</div>
</td>
</tr>
<tr>
<th>연락처</th>
<td colSpan={3}>
<input type="input" maxLength="11" />
</td>
</tr>
<tr>
<th>대상자와의 관계</th>
<td colSpan={3}>
<input type="text" />
</td>
</tr>
</table>
<div className="btn-wrap flex-center margin-bottom5">
<Button
className={"btn-small red-btn"}
btnName={"추가"}
onClick={() => {
navigate("/SeniorInsert");
}}
/>
</div>
<div>
<Table
className={"caregiver-user"}
head={thead3}
contents={content3}
contentKey={key3}
/>
</div>
</div>
</Modal>
<Modal open={modalOpen2} close={closeModal2} header="대상자(사용자) 등록">
<div className="board-wrap">
<SubTitle explanation={"회원 등록 시 ID는 연락처, 패스워드는 생년월일 8자리입니다."} className="margin-bottom" />
<table className="margin-bottom2 senior-insert">
<tr>
<th>대상자등록번호</th>
<td colSpan={3} className="flex">
<input type="text" placeholder="생성하기 버튼 클릭 시 자동으로 생성됩니다." />
<Button
className={"btn-short red-btn margin-left"}
btnName={"생성"}
/>
</td>
</tr>
<tr>
<th>이름</th>
<td>
<input type="text" />
</td>
</tr>
<tr>
<th>성별</th>
<td className="flex-start gender">
<div className="flex-start">
<input type="radio" name="genderSelect"></input>
<label for="gender">남</label>
</div>
<div className="flex-start">
<input type="radio" name="genderSelect"></input>
<label for="gender">여</label>
</div>
</td>
</tr>
<tr>
<th>생년월일</th>
<td>
<div className="flex">
<select name="year" id="year">
<option value="">년</option>
</select>
<select name="month" id="month">
<option value="">월</option>
</select>
<select name="days" id="days">
<option value="">일</option>
</select>
</div>
</td>
{/* <th>요양등급</th>
<td>
<input type="text" />
</td> */}
</tr>
<tr>
<th>연락처</th>
<td colSpan={3}>
<input type="text" />
</td>
</tr>
<tr>
<th>주소</th>
<td colSpan={3}>
<input type="text" />
</td>
</tr>
<tr>
<th>비고</th>
<td colSpan={3}>
<textarea className="medicine" cols="30" rows="2"></textarea>
</td>
</tr>
{/* <tr>
<th>복용중인 약</th>
<td colSpan={3}>
<textarea className="medicine" cols="30" rows="2"></textarea>
</td>
</tr>
<tr>
<th>기저질환</th>
<td colSpan={3}>
<textarea cols="30" rows="10"></textarea>
</td>
</tr> */}
</table>
<div className="flex-center">
<Button
className={"btn-small red-btn"}
btnName={"등록"}
onClick={closeModal2}
/>
</div>
</div>
</Modal>
<ContentTitle explanation={"사용자 관리"} />
<div className="content-wrap">
<div
className="flex-align-start userauthoriylist"
style={{ height: "calc(100% - 61px)" }}
>
<div className="left" style={{ height: "100%", }}>
<div style={{ height: "100%" }}>
<SubTitle
explanation={"관리기관 리스트"}
className="margin-bottom"
/>
<Category />
</div>
</div>
<div className="right" style={{ height: "100%", }}>
<div style={{ height: "100%" }}>
<SubTitle
explanation={`${agencyName} 사용자 리스트`}
className="margin-bottom"
/>
<div className="tab-container">
<ul className="tab-menu flex-end">
{data.map((item) => (
<li
key={item.id}
className={index === item.id ? "active" : null}
onClick={() => setIndex(item.id)}
>
{item.title}
</li>
))}
</ul>
<div className="content-wrap userlist">
<div className="search-management flex-start margin-bottom2">
<select>
<option value="이름">이름</option>
<option value="아이디">사용자등록번호</option>
<option value="아이디">ID</option>
</select>
<input type="text" />
<Button
className={"btn-small gray-btn"}
btnName={"검색"}
onClick={() => navigate("/SeniorInsert")}
/>
</div>
<div className="btn-wrap flex-end margin-bottom">
<Button
className={"btn-small gray-btn"}
btnName={"등록"}
onClick={openModal2}
/>
<Button className={"btn-small red-btn"} btnName={"삭제"} />
</div>
<ul className="tab-content">
{data
.filter((item) => index === item.id)
.map((item) => (
<li>{item.description}</li>
))}
</ul>
</div>
</div>
</div>
</div>
</div>
</div>
</main>
);
}