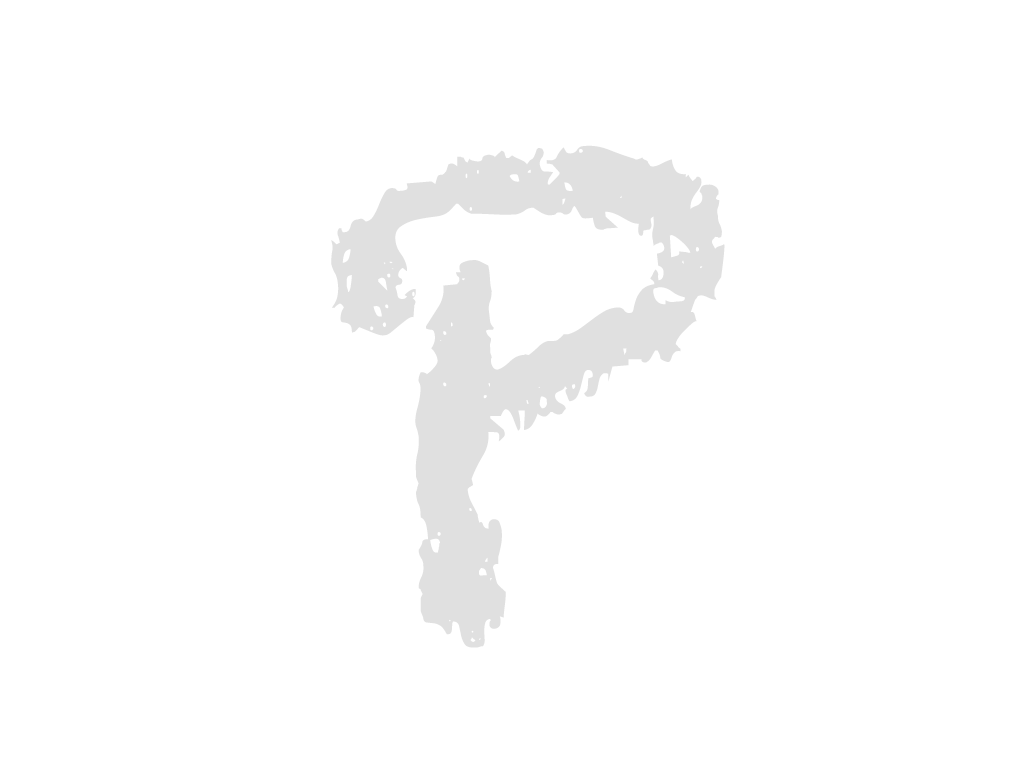
File name
Commit message
Commit date
2023-04-18
File name
Commit message
Commit date
import React from "react";
import { useSelector } from "react-redux";
import SubTitle from "./SubTitle.jsx";
import CommonUtil from "../../resources/js/CommonUtil.js";
import {JUSO_API_KEY, JUSO_CORRD_API_KEY} from "../../../Global.js";
import proj4 from "proj4";
export default function Modal({ open, close, seniorInsertCallback, defaultAgentId, defaultAgencyId, defaultGovernmentId }) {
//console.log(`seniorInsertModal - defaultGovernmentId:${defaultGovernmentId}, defaultAgencyId:${defaultAgencyId}`)
/**** 기본 조회 데이터 (시작) ****/
//전역 변수 저장 객체
const state = useSelector((state) => {return state});
//const defaultGovernmentId = CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['government_id'];
//기관 계층 구조 목록
const [orgListOfHierarchy, setOrgListOfHierarchy] = React.useState([]);
//기관(관리, 시행) 계층 구조 목록 조회
const orgSelectListOfHierarchy = () => {
fetch("/org/orgSelectListOfHierarchy.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({'government_id': defaultGovernmentId}),
}).then((response) => response.json()).then((data) => {
console.log("기관(관리, 시행) 계층 구조 목록 조회 : ", data);
setOrgListOfHierarchy(data);
}).catch((error) => {
console.log('orgSelectListOfHierarchy() /org/orgSelectListOfHierarchy.json error : ', error);
});
};
const getAgencyList = () => {
const government = orgListOfHierarchy.find(item => item['government_id'] == senior['government_id']);
if (CommonUtil.isEmpty(government) || CommonUtil.isEmpty(government['agencyList'])) {
return [];
} else {
return government['agencyList'];
}
}
const [medicationTimeCodeList, setMedicationTimeCodeList] = React.useState([]);
//복약 시간 코드 목록 조회
const medicationTimeCodeSelectList = () => {
fetch("/common/medicationTimeCodeSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({}),
}).then((response) => response.json()).then((data) => {
console.log("복약 시간 코드 목록 조회 : ", data);
setMedicationTimeCodeList(data);
//시니어 복약 정보 미리 세팅
let newSenior = JSON.parse(JSON.stringify(senior));
for (let i = 0; i < data.length; i++) {
newSenior['seniorMedicationList'].push(data[i]['medication_time_code']);
}
setSenior(newSenior);
}).catch((error) => {
console.log('medicationTimeCodeSelectList() /common/medicationTimeCodeSelectList.json error : ', error);
});
}
/**** 기본 조회 데이터 (종료) ****/
//등록할 시니어 정보
const [senior, setSenior] = React.useState({
'user_id': null,
'user_name': null,
'user_password': null,
'user_phonenumber': null,
'user_birth': null,
'user_gender': null,
'user_address': null,
'user_email': null,
'authority': 'ROLE_SENIOR',
'agency_id': !defaultAgencyId ? state.loginUser['agency_id'] : defaultAgencyId,
'government_id': !defaultGovernmentId ? state.loginUser['government_id'] : defaultGovernmentId,
'zip_no': null,
'adm_cd': null,
'rn_mgt_sn': null,
'bd_mgt_sn': null,
'si_nm': null,
'sgg_nm': null,
'emd_nm': null,
'li_nm': null,
'rn': null,
'emd_no': null,
'hemd_nm': null,
'road_addr': null,
'buld_mnnm': null,
'buld_slno': null,
'x': null,
'y': null,
'senior_id': null,
'care_grade': null,
'medication_pill': null,
'underlie_disease': null,
'senior_note': null,
'seniorMedicationList': [],
'agent_id': !defaultAgentId ? state.loginUser['agent_id'] : defaultAgentId,
});
//console.log(`seniorInsertModal - senior:`, senior);
//각 데이터별로 Dom 정보 담을 Ref 생성
const seniorRefInit = JSON.parse(JSON.stringify(senior));
seniorRefInit['user_gender'] = {};
seniorRefInit['user_id_check_button'] = null;
const seniorRef = React.useRef(seniorRefInit);
//등록할 시니어 정보 변경
const seniorValueChange = (targetKey, value) => {
let newSenior = JSON.parse(JSON.stringify(senior));
newSenior[targetKey] = value;
setSenior(newSenior);
}
//등록할 시니어의 관리기관 변경
const seniorGovernmentIdChange = (value) => {
let newSenior = JSON.parse(JSON.stringify(senior));
if (CommonUtil.isEmpty(value) == true) {
newSenior['government_id'] = null;
} else {
newSenior['government_id'] = value;
}
newSenior['agency_id'] = null;
setSenior(newSenior);
}
//등록할 시니어의 시행기관 변경
const seniorAgencyIdChange = (value) => {
let newSenior = JSON.parse(JSON.stringify(senior));
if (CommonUtil.isEmpty(value) == true) {
newSenior['agency_id'] = null;
} else {
newSenior['agency_id'] = value;
}
setSenior(newSenior);
}
//등록할 시니어의 기본 복약 정보 변경
const seniorMedicationChange = (value, isChecked) => {
let newSenior = JSON.parse(JSON.stringify(senior));
let index = newSenior['seniorMedicationList'].indexOf(value);
if (isChecked == true && index == -1) {
newSenior['seniorMedicationList'].push(value);
setSenior(newSenior);
} else if (isChecked == false && index > -1) {
newSenior['seniorMedicationList'].splice(index, 1);
setSenior(newSenior);
} else {
return;
}
}
//아이디 중복 확인
const [isIdCheck, setIsIdCheck] = React.useState(false);
//로그인 아이디 중복 검사
const userIdCheck = () => {
if (CommonUtil.isEmpty(senior['user_phonenumber']) == true) {
seniorRef.current['user_phonenumber'].focus();
alert("연락처를 입력해 주세요.");
return false;
}
senior['user_id'] = senior['user_phonenumber'];
fetch("/user/userSelectOne.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(senior),
}).then((response) => response.json()).then((data) => {
console.log("로그인 아이디 중복 검사(아이디를 통한 사용자 조회) : ", data);
if (CommonUtil.isEmpty(data) == true) {
setIsIdCheck(true);
seniorRef.current['user_address'].focus();
alert("사용가능한 연락처 입니다.");
} else {
setIsIdCheck(false);
seniorRef.current['user_phonenumber'].focus();
alert("이미 존재하는 연락처 입니다.");
}
}).catch((error) => {
console.log('userIdCheck() /user/userSelectOne.json error : ', error);
});
}
//시니어 등록 유효성 검사
const seniorInsertValidation = () => {
if (CommonUtil.isEmpty(senior['government_id']) == true) {
seniorRef.current['government_id'].focus();
alert("관리기관을 선택해 주세요.");
return false;
}
if (CommonUtil.isEmpty(senior['agency_id']) == true) {
seniorRef.current['agency_id'].focus();
alert("시행기관을 선택해 주세요.");
return false;
}
if (CommonUtil.isEmpty(senior['user_name']) == true) {
seniorRef.current['user_name'].focus();
alert("이름을 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(senior['user_gender']) == true) {
seniorRef.current['user_gender']['m'].focus();
alert("성별을 선택해 주세요.");
return false;
}
if (CommonUtil.isEmpty(senior['user_birth']) == true) {
seniorRef.current['user_birth'].focus();
alert("생년월일을 선택해 주세요.");
return false;
}
if (CommonUtil.isEmpty(senior['user_phonenumber']) == true) {
seniorRef.current['user_phonenumber'].focus();
alert("연락처를 입력해 주세요.");
return false;
}
if (isIdCheck == false) {
alert("연락처 중복확인을 해주세요.");
return false;
}
if (CommonUtil.isEmpty(senior['user_address']) == true) {
seniorRef.current['user_address'].focus();
alert("주소를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(senior['zip_no']) == true) {
seniorRef.current['user_address'].focus();
alert("검색을 통해 주소를 선택해 주세요.");
return false;
}
return true;
}
//시니어 등록
const seniorInsert = () => {
if (seniorInsertValidation() == false) {
return;
}
fetch("/user/seniorInsert.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(senior),
}).then((response) => response.json()).then((data) => {
console.log("시니어 등록 결과(건수) : ", data);
if (data > 0) {
alert("등록완료");
seniorInsertCallback();
} else {
alert("등록에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('seniorInsert() /user/seniorInsert.json error : ', error);
});
}
//주소 검색 결과 객체
const [jusoList, setJusoList] = React.useState({
common: {
countPerPage: 10,
currentPage: 1,
errorCode: '0',
errorMessage: '정상',
totalCount: 0
}, juso: [],
});
//주소 검색
const jusoSearch = (currentPage) => {
if (CommonUtil.isEmpty(senior['user_address']) == true) {
seniorRef.current['user_address'].focus();
alert("주소를 입력해 주세요.");
return false;
}
// console.log("check done");
const vm = this;
let url = 'https://business.juso.go.kr/addrlink/addrLinkApi.do'
url += `?currentPage=${CommonUtil.isEmpty(currentPage) ? 1 : currentPage}`
url += '&countPerPage=10'
url += '&resultType=json'
url += `&keyword=${senior['user_address']}`
url += `&confmKey=${JUSO_API_KEY}`;
fetch(url, {
method: "GET",
}).then((response) => response.json()).then((data) => {
console.log("주소 검색 결과(건수) : ", data);
setJusoList(data.results);
if (data.results.common.errorCode != '0') {
alert(data.results.common.errorMessage);
} else {
if (CommonUtil.isEmpty(data.results.juso)) {
alert('조회된 주소 정보가 없습니다.');
}
}
}).catch((error) => {
console.log('jusoSearch() : ', error);
});
};
const grs80 = "+proj=tmerc +lat_0=38 +lon_0=127.5 +k=0.9996 +x_0=1000000 +y_0=2000000 +ellps=GRS80 +towgs84=0,0,0,0,0,0,0 +units=m +no_defs";
const wgs84 = "+proj=longlat +ellps=WGS84 +datum=WGS84 +no_defs";
//주소 정보 활용 좌표 검색
const coordSearch = () => {
console.log("check senior : ", senior);
let url = 'https://business.juso.go.kr/addrlink/addrCoordApi.do'
url += `?admCd=${senior['adm_cd']}`
url += `&rnMgtSn=${senior['rn_mgt_sn']}`
url += `&udrtYn=${senior['udrt_yn']}`
url += `&buldMnnm=${senior['buld_mnnm']}`
url += `&buldSlno=${senior['buld_slno']}`
url += '&resultType=json'
url += `&confmKey=${JUSO_CORRD_API_KEY}`;
fetch(url, {
method: "GET",
}).then((response) => response.json()).then((data) => {
console.log("주소 정보 활용 좌표 검색 결과(건수) : ", data);
//setJusoList(data.results);
if (data.results.common.errorCode == '0' && CommonUtil.isEmpty(data.results.juso) == false) {
let grs80Point = [parseFloat(data.results.juso[0].entX), parseFloat(data.results.juso[0].entY)];
let wgs84Point = proj4(grs80, wgs84).forward(grs80Point);
senior['x'] = wgs84Point[0];
senior['y'] = wgs84Point[1];
setSenior({...senior});
}
}).catch((error) => {
console.log('jusoSearch() : ', error);
});
};
//Mounted
React.useEffect(() => {
orgSelectListOfHierarchy();
medicationTimeCodeSelectList();
}, []);
//Mounted
React.useEffect(() => {
const government_id = !defaultGovernmentId ? state.loginUser['government_id'] : defaultGovernmentId;
const agency_id = !defaultAgencyId ? state.loginUser['agency_id'] : defaultAgencyId;
let newSenior = JSON.parse(JSON.stringify(senior));
newSenior['government_id'] = government_id;
newSenior['agency_id'] = agency_id;
setSenior(newSenior);
}, [defaultGovernmentId, defaultAgencyId]);
return (
<div class={open ? "openModal modal" : "modal"}>
{open ? (
<div className="modal-inner">
<div className="modal-header flex">
대상자(시니어) 등록
<button className={"close"} onClick={() => {close()}}>X</button>
</div>
<div className="modal-main">
<div className="board-wrap">
<SubTitle explanation={"회원 등록 시 ID는 연락처, 패스워드는 생년월일 8자리입니다."} className="margin-bottom" />
<table className="margin-bottom2 senior-insert">
<tr>
<th><span style={{color : "red"}}>*</span>관리기관</th>
<td>
<select onChange={(e) => {seniorGovernmentIdChange(e.target.value)}}
ref={el => seniorRef.current['government_id'] = el}>
<option value={''} selected={senior['government_id'] == null}>관리기관선택</option>
{orgListOfHierarchy.map((item, idx) => { return (
<option key={idx} value={item['government_id']} selected={senior['government_id'] == item['government_id']}>
{item['government_name']}
</option>
)})}
</select>
</td>
<th><span style={{color : "red"}}>*</span>시행기관</th>
<td>
<select onChange={(e) => {seniorAgencyIdChange(e.target.value)}}
ref={el => seniorRef.current['agency_id'] = el}>
<option value={''} selected={senior['agency_id'] == null}>시행기관선택</option>
{getAgencyList().map((item, idx) => { return (
<option key={idx} value={item['agency_id']} selected={senior['agency_id'] == item['agency_id']}>
{item['agency_name']}
</option>
)})}
</select>
</td>
</tr>
<tr>
<th><span style={{color : "red"}}>*</span>이름</th>
<td>
<input type="text"
value={senior['user_name']}
onChange={(e) => {seniorValueChange('user_name', e.target.value)}}
ref={el => seniorRef.current['user_name'] = el}
/>
</td>
<th><span style={{color : "red"}}>*</span>성별</th>
<td className="">
<div className="gender flex-start">
<div className="flex-start">
<input type="radio" id="user_gender_m" name="user_gender" value="남" checked={senior['user_gender'] == '남'}
onChange={(e) => {e.target.checked ? seniorValueChange('user_gender', e.target.value) : null}}
ref={el => seniorRef.current['user_gender']['m'] = el}
/>
<label for="user_gender_m">남</label>
</div>
<div className="flex-start">
<input type="radio" id="user_gender_f" name="user_gender" value="여" checked={senior['user_gender'] == '여'}
onChange={(e) => {e.target.checked ? seniorValueChange('user_gender', e.target.value) : null}}
ref={el => seniorRef.current['user_gender']['f'] = el}
/>
<label for="user_gender_f">여</label>
</div>
</div>
</td>
</tr>
<tr>
<th><span style={{color : "red"}}>*</span>생년월일</th>
<td>
<div className="flex">
<input type='date'
value={senior['user_birth']}
onChange={(e) => {seniorValueChange('user_birth', e.target.value)}}
ref={el => seniorRef.current['user_birth'] = el}
/>
</div>
</td>
</tr>
<tr>
<th><span style={{color : "red"}}>*</span>연락처</th>
<td colSpan={3}>
<input type="number" maxLength="11" style={{width: 'calc(100% - 160px)'}}
value={senior['user_phonenumber']}
onChange={(e) => {seniorValueChange('user_phonenumber', e.target.value); setIsIdCheck(false);}}
ref={el => seniorRef.current['user_phonenumber'] = el}
/>
<button className={"red-btn btn-large"} onClick={userIdCheck}
ref={el => seniorRef.current['user_id_check_button'] = el}>
중복확인
</button>
</td>
</tr>
<tr>
<th><span style={{color : "red"}}>*</span>주소</th>
<td colSpan={3}>
<div>
<input type="text" style={{width: 'calc(100% - 160px)'}}
value={senior['user_address']} disabled={CommonUtil.isEmpty(senior['zip_no']) == false}
onChange={(e) => {seniorValueChange('user_address', e.target.value)}}
onKeyUp={(e) => {e.key == 'Enter' ? jusoSearch() : null}}
ref={el => seniorRef.current['user_address'] = el}
/>
{CommonUtil.isEmpty(senior['zip_no'])
? <button className={"red-btn btn-large"} onClick={() => {jusoSearch()}}>주소검색</button>
: <button className={"gray-btn btn-large"} onClick={() => {seniorValueChange('zip_no', null)}}>다시검색</button>
}
</div>
{CommonUtil.isEmpty(jusoList.juso) == false && CommonUtil.isEmpty(senior['zip_no']) ?
<div>
<ul className="list-box" style={{width: '100%'}}>
{CommonUtil.isEmpty(jusoList.juso) == false ? jusoList.juso.map((item, idx) => {return (
<li className={senior['zip_no'] == item['zipNo'] ? 'active' : null} onClick={() => {
senior['zip_no'] = item['zipNo']; senior['adm_cd'] = item['admCd'];
senior['rn_mgt_sn'] = item['rnMgtSn']; senior['bd_mgt_sn'] = item['bdMgtSn'];
senior['siNsi_nmm'] = item['siNm']; senior['sgg_nm'] = item['sggNm'];
senior['emd_nm'] = item['emdNm']; senior['li_nm'] = item['liNm'];
senior['rn'] = item['rn']; senior['emd_no'] = item['emdNo'];
senior['hemd_nm'] = item['hemdNm']; senior['road_addr'] = item['roadAddr'];
senior['buld_mnnm'] = item['buldMnnm']; senior['buld_slno'] = item['buldSlno'];
senior['user_address'] = item['roadAddr'];
console.log(`@@@@@@@@@@@@ item['udrtYn'] : ${item['udrtYn']}`);
if (CommonUtil.isEmpty(item['udrtYn'])) {
senior['udrt_yn'] = null;
} else {
if (item['udrtYn'] == 1 || item['udrtYn'] == true || item['udrtYn'] == '1'|| item['udrtYn'].toLowerCase() == 'true') {
senior['udrt_yn'] = true;
} else {
senior['udrt_yn'] = false;
}
}
setSenior({...senior}); coordSearch();
}}>
<span style={{fontWeight: 600}}>[지번]</span> {item['jibunAddr']}
<br/>
<span style={{fontWeight: 600}}>[도로명]</span> {item['roadAddr']}
</li>
)}): null}
</ul>
</div>
: null}
</td>
</tr>
<tr>
<th>필요 복약</th>
<td className="medicationTime-td">
<div className="flex">
{medicationTimeCodeList.map((item, idx) => { return (
<span key={idx}>
<input type="checkbox"
name="medicationTimeCodeList"
id={item['medication_time_code']}
value={item['medication_time_code']}
onChange={(e) => {seniorMedicationChange(e.target.value, e.target.checked)}}
checked={senior['seniorMedicationList'].indexOf(item['medication_time_code']) > -1}/>
<label for={item['medication_time_code']}>{item['medication_time_code_name']}</label>
</span>
)})}
</div>
</td>
</tr>
<tr>
<th>복용중인 약</th>
<td colSpan={3}>
<textarea className="medicine" cols="30" rows="2" value={senior['medication_pill']} onChange={(e) => {seniorValueChange('medication_pill', e.target.value)}} />
</td>
</tr>
<tr>
<th>비고</th>
<td colSpan={3}>
<textarea className="note" cols="30" rows="2" value={senior['senior_note']} onChange={(e) => {seniorValueChange('senior_note', e.target.value)}} />
</td>
</tr>
{/* <tr>
<th>기저질환</th>
<td colSpan={3}>
<textarea cols="30" rows="10"></textarea>
</td>
</tr> */}
</table>
<div className="btn-wrap flex-center">
<button className={"btn-small gray-btn"} onClick={seniorInsert}>등록</button>
</div>
</div>
</div>
</div>
) : null}
</div>
);
}