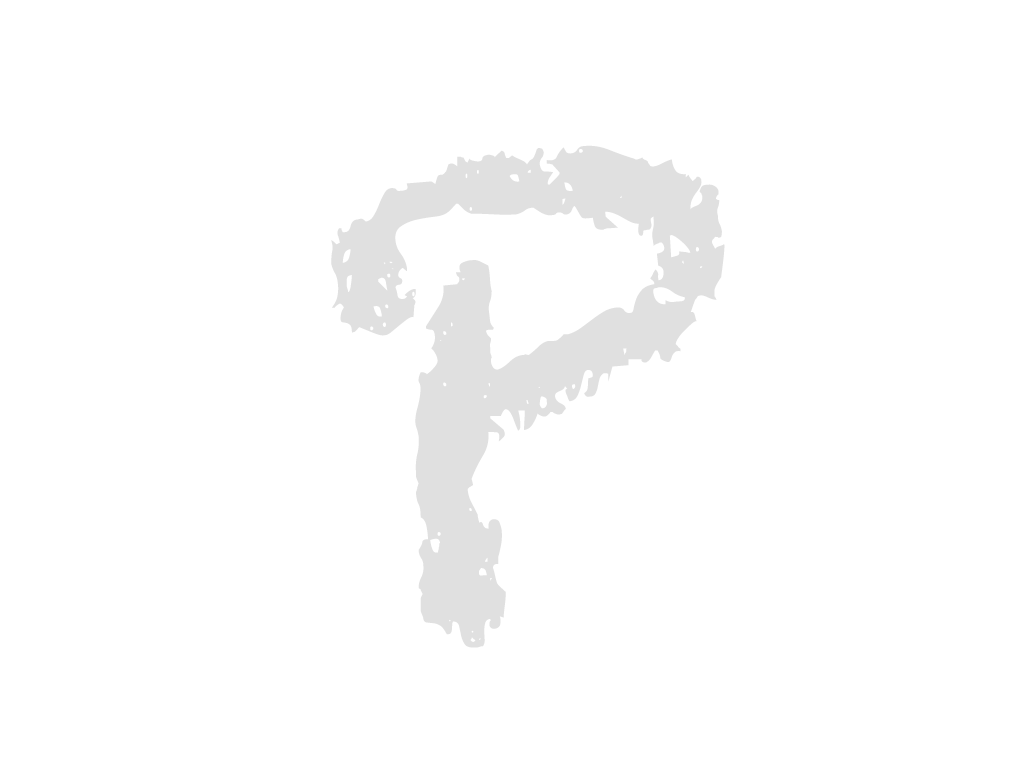
File name
Commit message
Commit date
2023-04-18
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React from "react";
import { useNavigate, useLocation } from "react-router";
import { useSelector } from "react-redux";
import ContentTitle from "../../component/ContentTitle.jsx";
import Modal from "../../component/Modal.jsx";
import SubTitle from "../../component/SubTitle.jsx";
import Pagination from "../../component/Pagination.jsx";
import CommonUtil from "../../../resources/js/CommonUtil.js";
export default function OrgSelect() {
const navigate = useNavigate();
const location = useLocation();
//전역 변수 저장 객체
const state = useSelector((state) => { return state });
// 시스템 코드 - 기관 종류
const [orgTypes, setOrgTypes] = React.useState({});
// 시스템 코드 - 기관 종류 조회
const orgTypesSelect = () => {
//fetch post
fetch("/common/systemCode/orgTypesSelect.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({})
}).then((response) => response.json()).then((data) => {
console.log('orgTypesSelect response : ', data);
setOrgTypes(data);
}).catch((error) => {
console.log('orgTypesSelect error : ', error);
});
}
//관리기관 모달 오픈 여부
const [isGovermentModalOpen, setIsGovermentModalOpen] = React.useState(false);
//관리기관 모달 오픈
const governmentModalOpen = () => {
setIsGovermentModalOpen(true);
};
//관리기관 모달 닫기
const governmentModalClose = () => {
setIsGovermentModalOpen(false);
};
//관리기관 초기화 정보
const governmentInit = {
'government_id': state.loginUser['government_id'],
'government_type': 'WELFARE',
'government_name': null,
'government_address': null,
'government_phonenumber': null,
'government_insert_datetime': null,
'government_insert_user_id': null,
'government_update_datetime': null,
'government_update_user_id': null,
}
//관리기관 데이터
const [government, setGovernment] = React.useState({
search: { currentPage: 1, perPage: 5, 'government_id': state.loginUser['government_id'] },
government: { ...governmentInit },
governmentList: [],
governmentListCount: 0,
})
//관리기관 Ref
const governmentRef = React.useRef(governmentInit);
//관리기관 정보 수정
const governmentValueChange = (targetKey, value) => {
government.government[targetKey] = value;
setGovernment({ ...government });
}
//관리기관 유효성 검사
const governmentValidation = () => {
const target = government.government;
const targetRef = governmentRef;
if (CommonUtil.isEmpty(target['government_name']) == true) {
targetRef.current['government_name'].focus();
alert("기관명을 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['government_phonenumber']) == true) {
targetRef.current['government_phonenumber'].focus();
alert("대표 연락처를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['government_address']) == true) {
targetRef.current['government_address'].focus();
alert("주소를 입력해 주세요.");
return false;
}
return true;
}
//관리기관 등록
const governmentInsert = () => {
if (governmentValidation() == false) {
return;
}
fetch("/org/governmentInsert.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(government.government),
}).then((response) => response.json()).then((data) => {
console.log("관리기관 등록 결과 : ", data);
if (data > 0) {
alert("등록완료");
governmentSelectList();
governmentModalClose();
} else {
alert("등록에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('governmentInsert() /org/governmentInsert.json error : ', error);
});
}
//관리기관 수정
const governmentUpdate = () => {
if (governmentValidation() == false) {
return;
}
fetch("/org/governmentUpdate.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(government.government),
}).then((response) => response.json()).then((data) => {
console.log("관리기관 수정 결과 : ", data);
if (data > 0) {
alert("수정완료");
governmentSelectList();
governmentModalClose();
} else {
alert("수정에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('governmentUpdate() /org/governmentUpdate.json error : ', error);
});
}
//관리기관 삭제
const governmentDelete = (item) => {
if (item['government_user_count'] > 0 || item['agent_user_count'] > 0
|| item['guardian_user_count'] > 0 || item['senior_user_count'] > 0) {
alert('해당 기관에 사용자가 존재합니다. (삭제 불가능)');
return;
}
if (item['equipment_count'] > 0) {
alert('해당 기관에 납품된 장비가 존재합니다. (삭제 불가능)');
return;
}
if (item['agency_count'] > 0) {
alert('해당 기관의 하위기관이 존재합니다. (삭제 불가능)');
return;
}
if (confirm("해당 기관을 삭제하시겠습니까?") == false) {
return;
}
fetch("/org/governmentDelete.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(item),
}).then((response) => response.json()).then((data) => {
console.log("관리기관 삭제 결과 : ", data);
if (data > 0) {
alert("삭제완료");
governmentSelectList();
governmentModalClose();
} else {
alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('governmentDelete() /org/governmentDelete.json error : ', error);
});
}
//관리기관 목록 조회
const governmentSelectList = () => {
fetch("/org/governmentSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(government.search),
}).then((response) => response.json()).then((data) => {
console.log("관리기관 목록 조회 결과 : ", data);
government.governmentList = data.governmentList;
government.governmentListCount = data.governmentListCount;
setGovernment({ ...government });
}).catch((error) => {
console.log('governmentSelectList() /org/governmentSelectList.json error : ', error);
});
}
//시행기관 모달 오픈 여부
const [isAgencyModalOpen, setIsAgencyModalOpen] = React.useState(false);
//시행기관 모달 오픈
const agencyModalOpen = () => {
setIsAgencyModalOpen(true);
};
//시행기관 모달 닫기
const agencyModalClose = () => {
setIsAgencyModalOpen(false);
};
//시행기관 초기화 정보
const agencyInit = {
'government_id': state.loginUser['government_id'],
'agency_id': state.loginUser['agency_id'],
'agency_type': 'WELFARE',
'agency_name': null,
'agency_address': null,
'agency_phonenumber': null,
'agency_insert_datetime': null,
'agency_insert_user_id': null,
'agency_update_datetime': null,
'agency_update_user_id': null
}
//시행기관 데이터
const [agency, setAgency] = React.useState({
search: { currentPage: 1, perPage: 5, 'government_id': state.loginUser['government_id'], 'agency_id': state.loginUser['agency_id'] },
agency: { ...agencyInit },
agencyList: [],
agencyListCount: 0,
})
//시행기관 Ref
const agencyRef = React.useRef(agencyInit);
//시행기관 정보 수정
const agencyValueChange = (targetKey, value) => {
agency.agency[targetKey] = value;
//'시행기관 구분' 정보는 '관리기관의 구분'으로 자동 선택
if (targetKey == 'government_id') {
const findGovernment = government.governmentList.find(item => item['government_id'] == value);
if (CommonUtil.isEmpty(findGovernment) == false) {
agency.agency['agency_type'] = findGovernment['government_type'];
} else {
agency.agency['agency_type'] = 'WELFARE';
}
}
setAgency({ ...agency });
}
//시행기관 유효성 검사
const agencyValidation = () => {
const target = agency.agency;
const targetRef = agencyRef;
if (CommonUtil.isEmpty(target['government_id']) == true) {
targetRef.current['government_id'].focus();
alert("관리기관을 선택해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['agency_name']) == true) {
targetRef.current['agency_name'].focus();
alert("기관명을 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['agency_phonenumber']) == true) {
targetRef.current['agency_phonenumber'].focus();
alert("대표 연락처를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['agency_address']) == true) {
targetRef.current['agency_address'].focus();
alert("주소를 입력해 주세요.");
return false;
}
return true;
}
//시행기관 등록
const agencyInsert = () => {
if (agencyValidation() == false) {
return;
}
fetch("/org/agencyInsert.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(agency.agency),
}).then((response) => response.json()).then((data) => {
console.log("시행기관 등록 결과 : ", data);
if (data > 0) {
alert("등록완료");
agencySelectList();
agencyModalClose();
} else {
alert("등록에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('agencyInsert() /org/agencyInsert.json error : ', error);
});
}
//시행기관 수정
const agencyUpdate = () => {
if (agencyValidation() == false) {
return;
}
fetch("/org/agencyUpdate.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(agency.agency),
}).then((response) => response.json()).then((data) => {
console.log("시행기관 수정 결과 : ", data);
if (data > 0) {
alert("수정완료");
agencySelectList();
agencyModalClose();
} else {
alert("수정에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('agencyUpdate() /org/agencyUpdate.json error : ', error);
});
}
//시행기관 삭제
const agencyDelete = (item) => {
if (item['agent_user_count'] > 0 || item['guardian_user_count'] > 0
|| item['senior_user_count'] > 0) {
alert('해당 기관에 사용자가 존재합니다. (삭제 불가능)');
return;
}
if (confirm("해당 기관을 삭제하시겠습니까?") == false) {
return;
}
fetch("/org/agencyDelete.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(item),
}).then((response) => response.json()).then((data) => {
console.log("시행기관 삭제 결과 : ", data);
if (data > 0) {
alert("삭제완료");
agencySelectList();
agencyModalClose();
} else {
alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('agencyDelete() /org/agencyDelete.json error : ', error);
});
}
//시행기관 목록 조회
const agencySelectList = () => {
console.log('government.government : ', government.government);
fetch("/org/agencySelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(agency.search),
}).then((response) => response.json()).then((data) => {
console.log("시행기관 목록 조회 결과 : ", data);
agency.agencyList = data.agencyList;
agency.agencyListCount = data.agencyListCount;
setAgency({ ...agency });
}).catch((error) => {
console.log('agencySelectList() /org/agencySelectList.json error : ', error);
});
}
//사용자 관리 페이지 이동
const userManagementPage = (governmentId, agencyId, auth) => {
navigate("/UserSelect", {
state: {
'government_id': governmentId,
'agency_id': agencyId,
'tabActiveByRoleType': auth,
}
})
};
React.useEffect(() => {
orgTypesSelect();
governmentSelectList();
agencySelectList();
}, [])
return (
<main>
<Modal open={isGovermentModalOpen} close={governmentModalClose} header={CommonUtil.isEmpty(government.government['government_id']) ? '관리기관 등록' : '관리기관 수정'}>
<div className="board-wrap">
<table className="flex70 margin-bottom agency-insert">
<tbody className="">
<tr>
<th><span style={{ color: "red" }}>*</span>구분</th>
<td colSpan={5}>
<select name="division" id="section"
onChange={(e) => { governmentValueChange('government_type', e.target.value) }}
ref={el => governmentRef.current['government_type'] = el}>
{Object.keys(orgTypes).map((key, idx) => {
return (
<option key={key} value={key} selected={key == government.government['government_type']}>
{orgTypes[key]}
</option>
)
})}
</select>
</td>
</tr>
<tr>
<th><span style={{ color: "red" }}>*</span>기관명</th>
<td colSpan={5}>
<input type="text"
value={government.government['government_name']}
onChange={(e) => { governmentValueChange('government_name', e.target.value) }}
ref={el => governmentRef.current['government_name'] = el} />
</td>
</tr>
<tr>
<th><span style={{ color: "red" }}>*</span>대표 연락처</th>
<td colSpan={5}>
<input type="number"
value={government.government['government_phonenumber']}
onChange={(e) => { governmentValueChange('government_phonenumber', e.target.value) }}
ref={el => governmentRef.current['government_phonenumber'] = el} />
</td>
</tr>
<tr>
<th><span style={{ color: "red" }}>*</span>주소</th>
<td colSpan={5}>
<input type="text"
value={government.government['government_address']}
onChange={(e) => { governmentValueChange('government_address', e.target.value) }}
ref={el => governmentRef.current['government_address'] = el} />
</td>
</tr>
</tbody>
</table>
<div className="btn-wrap flex-center">
{CommonUtil.isEmpty(government.government['government_id'])
? <button className={"btn-small gray-btn"} onClick={governmentInsert}>등록</button>
: <button className={"btn-small gray-btn"} onClick={governmentUpdate}>수정</button>
}
</div>
</div>
</Modal>
<Modal open={isAgencyModalOpen} close={agencyModalClose} header={CommonUtil.isEmpty(agency.agency['agency_id']) ? '관리기관 등록' : '관리기관 수정'}>
<div className="board-wrap">
<table className="flex70 margin-bottom agency-insert">
<tbody className="">
<tr>
<th><span style={{ color: "red" }}>*</span>관리기관</th>
<td colSpan={5}>
<select name="division" id="section" disabled={CommonUtil.isEmpty(agency.agency['agency_id']) == false}
onChange={(e) => { agencyValueChange('government_id', e.target.value) }}
ref={el => agencyRef.current['government_id'] = el}>
<option value="" selected={agency.agency['government_id'] == null}>관리기관선택</option>
{government.governmentList.map((item, idx) => {
return (
<option key={idx} value={item['government_id']} selected={item['government_id'] == agency.agency['government_id']}>
{item['government_name']}
</option>
)
})}
</select>
</td>
</tr>
<tr>
<th><span style={{ color: "red" }}>*</span>구분</th>
<td colSpan={5}>
<select name="division" id="section" disabled={true}
onChange={(e) => { agencyValueChange('agency_type', e.target.value) }}
ref={el => agencyRef.current['agency_type'] = el}>
{Object.keys(orgTypes).map((key, idx) => {
return (
<option key={key} value={key} selected={key == agency.agency['agency_type']}>
{orgTypes[key]}
</option>
)
})}
</select>
</td>
</tr>
<tr>
<th><span style={{ color: "red" }}>*</span>기관명</th>
<td colSpan={5}>
<input type="text"
value={agency.agency['agency_name']}
onChange={(e) => { agencyValueChange('agency_name', e.target.value) }}
ref={el => agencyRef.current['agency_name'] = el} />
</td>
</tr>
<tr>
<th><span style={{ color: "red" }}>*</span>대표 연락처</th>
<td colSpan={5}>
<input type="number"
value={agency.agency['agency_phonenumber']}
onChange={(e) => { agencyValueChange('agency_phonenumber', e.target.value) }}
ref={el => agencyRef.current['agency_phonenumber'] = el} />
</td>
</tr>
<tr>
<th><span style={{ color: "red" }}>*</span>주소</th>
<td colSpan={5}>
<input type="text"
value={agency.agency['agency_address']}
onChange={(e) => { agencyValueChange('agency_address', e.target.value) }}
ref={el => agencyRef.current['agency_address'] = el} />
</td>
</tr>
</tbody>
</table>
<div className="btn-wrap flex-center">
{CommonUtil.isEmpty(agency.agency['agency_id'])
? <button className={"btn-small gray-btn"} onClick={agencyInsert}>등록</button>
: <button className={"btn-small gray-btn"} onClick={agencyUpdate}>수정</button>
}
</div>
</div>
</Modal>
<div className="content-wrap">
<ContentTitle contentTitle={`관리기관 조회 (${government.governmentListCount})`} />
<div className="margin-bottom10">
{/* <div className="search-management flex-start margin-bottom2">
<select id="searchType" style={{maxWidth: 'fit-content'}} onChange={(e) => {}}>
<option value="">전체</option>
<option value="user_name">기관명</option>
<option value="user_phonenumber">기관연락처</option>
</select>
<input id="searchText" type="text"
onChange={(e) => {}}
onKeyUp={(e) => {}}
/>
<button className={"btn-small gray-btn"} onClick={() => {}}>검색</button>
</div> */}
<div className="board-wrap">
{/* <SubTitle explanation={"장비 클릭 시 지난 매칭이력을 확인할 수 있습니다."} /> */}
<div className="btn-wrap flex-end margin-bottom ">
{state.loginUser['authority'] == 'ROLE_ADMIN' ?
<button className={"btn-small gray-btn"} onClick={() => { governmentValueChange('government_id', null); governmentModalOpen() }}>등록</button>
: null}
</div>
<table className={"senior-user protector-user"}>
<thead>
<tr>
<th>No</th>
<th>구분</th>
<th>기관명</th>
<th>관리장비개수</th>
<th>관리자(명)</th>
<th>생활보호사(명)</th>
<th>돌봄 대상자(명)</th>
<th>주소</th>
<th>대표연락처</th>
{state.loginUser['authority'] == 'ROLE_ADMIN' ?
<th>시행기관조회</th>
: null}
{/* <th>장비관리</th> */}
<th>사용자관리</th>
<th>관리</th>
</tr>
</thead>
<tbody>
{government.governmentList.map((item, idx) => {
return (
<tr key={idx}>
<td data-label="No">{government.governmentListCount - idx - (government.search.currentPage - 1) * government.search.perPage}</td>
<td data-label="구분">{orgTypes[item['government_type']]}</td>
<td data-label="기관명">{item['government_name']}</td>
<td data-label="관리 장비 개수">{item['equipment_count']}</td>
<td data-label="관리자(명)">{item['government_user_count']}</td>
<td data-label="생활보호사(명)">{item['agent_user_count']}</td>
<td data-label="돌봄 대상자(명)">{item['senior_user_count']}</td>
<td data-label="주소">{item['government_address']}</td>
<td data-label="대표 연락처">{item['government_phonenumber']}</td>
{state.loginUser['authority'] == 'ROLE_ADMIN' ?
<td>
<button className={"btn-small lightgray-btn"} onClick={() => {
government.government = item;
setGovernment({ ...government });
agency.search['government_id'] = item['government_id'];
agency.search['ageny_id'] = null;
setAgency({ ...agency });
agencySelectList();
}}>시행기관조회</button>
</td>
: null}
{/* <td>
<button className={"btn-small gray-btn"} onClick={() => {userManagementPage(item['government_id'], null, 'ROLE_GOVERNMENT')}}>장비관리</button>
</td> */}
<td>
<button className={"btn-small gray-btn"} onClick={() => { userManagementPage(item['government_id'], null, 'ROLE_GOVERNMENT') }}>사용자관리</button>
</td>
<td data-label="관리">
<button className={"btn-small lightgray-btn"} onClick={() => { government.government = item; setGovernment({ ...government }); governmentModalOpen() }}>정보 수정</button>
{state.loginUser['authority'] == 'ROLE_ADMIN' ?
<button className={"btn-small red-btn"} onClick={() => { governmentDelete(item) }}>삭제</button>
: null}
</td>
</tr>
)
})}
{CommonUtil.isEmpty(government.governmentList) ?
<tr>
<td colSpan={state.loginUser['authority'] == 'ROLE_ADMIN' ? 12 : 11}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={government.search.currentPage}
perPage={government.search.perPage}
totalCount={government.governmentListCount}
maxRange={5}
click={governmentSelectList}
/>
</div>
</div>
{state.loginUser['authority'] == 'ROLE_ADMIN'
? <ContentTitle contentTitle={government.government['government_id'] != null ? `[${government.government['government_name']}] 시행기관 조회 (${agency.agencyListCount})` : `시행기관 조회 (${agency.agencyListCount})`} />
: <ContentTitle contentTitle={`시행기관 조회 (${agency.agencyListCount})`} />
}
<div>
{/* <div className="search-management flex-start margin-bottom2">
<select id="searchType" style={{maxWidth: 'fit-content'}} onChange={(e) => {}}>
<option value="">전체</option>
<option value="user_name">기관명</option>
<option value="user_phonenumber">기관연락처</option>
</select>
<input id="searchText" type="text"
onChange={(e) => {}}
onKeyUp={(e) => {}}
/>
<button className={"btn-small gray-btn"} onClick={() => {}}>검색</button>
</div> */}
<div className="board-wrap">
{/* <SubTitle explanation={"장비 클릭 시 지난 매칭이력을 확인할 수 있습니다."} /> */}
<div className="btn-wrap flex-end margin-bottom ">
{state.loginUser['authority'] == 'ROLE_ADMIN' || state.loginUser['authority'] == 'ROLE_GOVERNMENT' ?
<button className={"btn-small gray-btn"} onClick={() => {
agency.agency['agency_id'] = null;
if (CommonUtil.isEmpty(government.government['government_id']) == false) {
agency.agency['government_id'] = government.government['government_id'];
agency.agency['agency_type'] = government.government['government_type'];
}
setAgency({ ...agency });
agencyModalOpen();
}}>등록</button>
: null}
</div>
<table className={"senior-user protector-user"}>
<thead>
<tr>
<th>No</th>
<th>구분</th>
<th>관리기관명</th>
<th>기관명</th>
<th>생활보호사(명)</th>
<th>돌봄 대상자(명)</th>
<th>주소</th>
<th>대표연락처</th>
{/* <th>장비관리</th> */}
<th>사용자관리</th>
<th>관리</th>
</tr>
</thead>
<tbody>
{agency.agencyList.map((item, idx) => {
return (
<tr key={idx}>
<td data-label="No">{agency.agencyListCount - idx - (agency.search.currentPage - 1) * agency.search.perPage}</td>
<td data-label="구분">{orgTypes[item['agency_type']]}</td>
<td data-label="관리기관명">{item['government_name']}</td>
<td data-label="기관명">{item['agency_name']}</td>
<td data-label="생활보호사(명)">{item['agent_user_count']}</td>
<td data-label="돌봄 대상자(명)">{item['senior_user_count']}</td>
<td data-label="주소">{item['agency_address']}</td>
<td data-label="대표 연락처">{item['agency_phonenumber']}</td>
{/* <td>
<button className={"btn-small gray-btn"} onClick={() => {userManagementPage(item['agency_id'], null, 'ROLE_AGENCY')}}>장비관리</button>
</td> */}
<td>
<button className={"btn-small gray-btn"} onClick={() => { userManagementPage(item['government_id'], item['agency_id'], 'ROLE_AGENCY') }}>사용자관리</button>
</td>
<td data-label="관리">
<button className={"btn-small lightgray-btn"} onClick={() => { agency.agency = item; setAgency({ ...agency }); agencyModalOpen() }}>정보 수정</button>
{state.loginUser['authority'] == 'ROLE_ADMIN' || state.loginUser['authority'] == 'ROLE_GOVERNMENT' ?
<button className={"btn-small red-btn"} onClick={() => { agencyDelete(item) }}>삭제</button>
: null}
</td>
</tr>
)
})}
{CommonUtil.isEmpty(agency.agencyList) ?
<tr>
<td colSpan={12}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={agency.search.currentPage}
perPage={agency.search.perPage}
totalCount={agency.agencyListCount}
maxRange={5}
click={agencySelectList}
/>
</div>
</div>
</div>
</main>
);
}