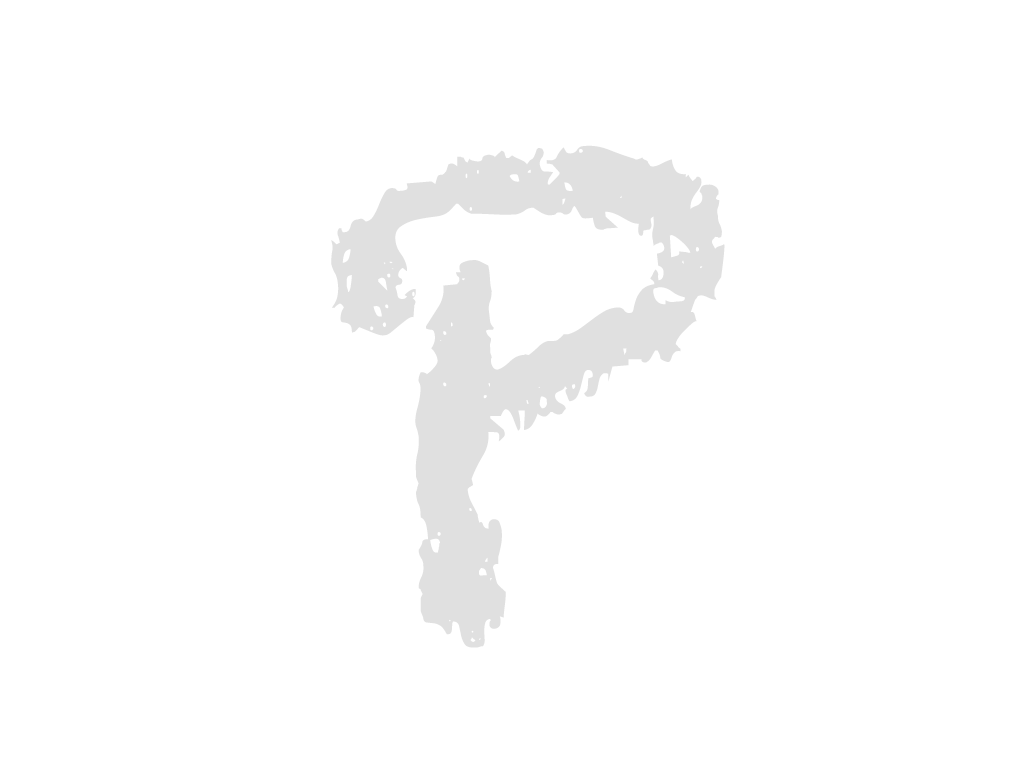
File name
Commit message
Commit date
2023-05-06
2023-04-18
File name
Commit message
Commit date
2023-04-18
File name
Commit message
Commit date
2023-04-18
2023-04-18
File name
Commit message
Commit date
2023-04-18
import React from "react";
import { useNavigate, useLocation } from "react-router";
import { useSelector } from "react-redux";
import SubTitle from "../../component/SubTitle.jsx";
import DetailTitle from "../../component/DetailTitle.jsx";
import Pagination from "../../component/Pagination.jsx";
import CommonUtil from "../../../resources/js/CommonUtil.js";
export default function UserAuthoriySelect() {
const navigate = useNavigate();
const location = useLocation();
//전역 변수 저장 객체
const state = useSelector((state) => {return state});
//검색(엔터)
const searchingEnter = (key) => {
if (key == 'Enter') {
searching();
} else {
return;
}
}
//검색
const searching = () => {
if (CommonUtil.isEmpty(state.loginUser) == false
&& state.loginUser['authority'] == 'ROLE_AGENCY' && isMySenior) {
senior.search['agent_id'] = state.loginUser['user_id'];
} else {
senior.search['agent_id'] = null;
}
setSenior({...senior});
seniorSelectList(1);
}
const [isMySenior, setIsMySenior] = React.useState(true);
React.useEffect(() => {
searching();
}, [isMySenior])
//보호사(간호사)의 돌봄 대상자(시니어)
const [senior, setSenior] = React.useState({seniorList: [], seniorListCount: 0, search: {
'government_id': CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['government_id'],
'agency_id': CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['agency_id'],
'searchType': null,
'searchText': null,
'currentPage': 1,
'perPage': 10,
}});
//보호사(간호사)의 돌봄 대상자(시니어) 목록 조회
const seniorSelectList = (currentPage) => {
senior.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage;
fetch("/user/seniorSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(senior.search),
}).then((response) => response.json()).then((data) => {
data.search = senior.search;
console.log("돌봄 대상자(시니어) 목록 조회 : ", data);
setSenior(data);
}).catch((error) => {
console.log('seniorSelectList() /user/seniorSelectList.json error : ', error);
});
}
React.useEffect(() => {
searching();
}, []);
//현재 탭 Index
const [tabIndex, setTabIndex] = React.useState(0);
//탭 초기화
const tab = [{
title: `복약관리`,
content: (
<div>
<div className="flex equip-tab">
<SubTitle explanation={"돌봄 대상자 클릭 시, 상세페이지로 이동합니다."} />
</div>
<table className={"protector-user"}>
<thead>
<tr>
<th>No</th>
<th>소속기관명</th>
<th>이름</th>
<th>생년월일</th>
<th>성별</th>
<th>연락처</th>
<th>최근복약률</th>
</tr>
</thead>
<tbody>
{senior.seniorList.map((item, idx) => { return (
<tr key={idx} onClick={() => {
navigate("/MedicineCareSelectOne");
}}>
<td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td>
<td data-label="소속기관명">{item['agency_name']}</td>
<td data-label="이름">{item['user_name']}</td>
<td data-label="생년월일">{item['user_birth']}</td>
<td data-label="성별">{item['user_gender']}</td>
<td data-label="연락처">{item['user_phonenumber']}</td>
<td data-label="문진표관리">
80%
</td>
</tr>
)})}
{CommonUtil.isEmpty(senior.seniorList) ?
<tr>
<td colSpan={7}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={senior.search.currentPage}
perPage={senior.search.perPage}
totalCount={senior.seniorListCount}
maxRange={5}
click={seniorSelectList}
/>
</div>
)
}, {
title: `댁내온도관리`,
content: (
<div>
<div className="flex equip-tab">
<SubTitle explanation={"돌봄 대상자 클릭 시, 상세페이지로 이동합니다."} />
</div>
<table className={"protector-user"}>
<thead>
<tr>
<th>No</th>
<th>소속기관명</th>
<th>이름</th>
<th>생년월일</th>
<th>성별</th>
<th>연락처</th>
<th>최근최저온도</th>
<th>최근최고온도</th>
</tr>
</thead>
<tbody>
{senior.seniorList.map((item, idx) => { return (
<tr key={idx} onClick={() => {
navigate("/TemperatureManagementSelectOne");
}}>
<td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td>
<td data-label="소속기관명">{item['agency_name']}</td>
<td data-label="이름">{item['user_name']}</td>
<td data-label="생년월일">{item['user_birth']}</td>
<td data-label="성별">{item['user_gender']}</td>
<td data-label="연락처">{item['user_phonenumber']}</td>
<td data-label="최근최저온도">
18℃
</td>
<td data-label="최근최고온도">
26℃
</td>
</tr>
)})}
{CommonUtil.isEmpty(senior.seniorList) ?
<tr>
<td colSpan={8}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={senior.search.currentPage}
perPage={senior.search.perPage}
totalCount={senior.seniorListCount}
maxRange={5}
click={seniorSelectList}
/>
</div>
)
}, {
title: `방문관리`,
content: (
<div>
<div className="flex equip-tab">
<SubTitle explanation={"돌봄 대상자 클릭 시, 상세페이지로 이동합니다."} />
</div>
<table className={"protector-user"}>
<thead>
<tr>
<th>No</th>
<th>소속기관명</th>
<th>이름</th>
<th>생년월일</th>
<th>성별</th>
<th>연락처</th>
{/* <th>최근방문일</th>
<th>방문목적</th> */}
</tr>
</thead>
<tbody>
{senior.seniorList.map((item, idx) => { return (
<tr key={idx} onClick={() => {
navigate("/VisitSelectOne", {state: {
'senior_id': item['senior_id'],
}})
}}>
<td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td>
<td data-label="소속기관명">{item['agency_name']}</td>
<td data-label="이름">{item['user_name']}</td>
<td data-label="생년월일">{item['user_birth']}</td>
<td data-label="성별">{item['user_gender']}</td>
<td data-label="연락처">{item['user_phonenumber']}</td>
{/* <td>2023-04-11</td>
<td>정기방문</td> */}
</tr>
)})}
{CommonUtil.isEmpty(senior.seniorList) ?
<tr>
<td colSpan={6}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={senior.search.currentPage}
perPage={senior.search.perPage}
totalCount={senior.seniorListCount}
maxRange={5}
click={seniorSelectList}
/>
</div>
)
}];
return (
<main>
<div className="search-management flex-start margin-bottom2">
<select style={{maxWidth: '150px'}}
onChange={(e) => {senior.search.searchType = e.target.value; setSenior({...senior});}}>
<option value="" selected={CommonUtil.isEmpty(senior.search.searchType)}>전체</option>
<option value="user_name" selected={senior.search.searchType == 'user_name'}>이름</option>
<option value="user_id" selected={senior.search.searchType == 'user_id'}>아이디</option>
<option value="user_phonenumber" selected={senior.search.searchType == 'user_phonenumber'}>연락처</option>
</select>
<input type="text" className="senior-search" value={senior.search.searchText}
onChange={(e) => {senior.search.searchText = e.target.value; setSenior({...senior});}}
onKeyUp={(e) => searchingEnter(e.key)}/>
<button className="btn-small gray-btn" onClick={searching}>검색</button>
</div>
<div className="content-wrap">
<DetailTitle contentTitle={"대상자의 문진표 / 심전도 / 혈압 관리를 할 수 있습니다."} />
<div style={{ height: "calc(100% - 61px)" }}>
<div className="right" style={{ height: "100%", }}>
<div style={{ height: "100%" }}>
<div className="tab-container" style={{ marginTop: "5rem"}}>
{CommonUtil.isEmpty(state.loginUser) == false && state.loginUser['authority'] == 'ROLE_AGENCY' ?
<div className="flex-end margin-bottom">
<div className="flex searchselect" style={{width: 'auto'}}>
<input type="radio" id="my_senior" name="senior" checked={isMySenior}
onChange={(e) => {e.target.checked ? setIsMySenior(true) : null}}/>
<label for="my_senior" style={{marginRight: '3rem'}}>나의 대상자 보기</label>
<input type="radio" id="all_senior" name="senior" checked={!isMySenior}
onChange={(e) => {e.target.checked ? setIsMySenior(false) : null}}/>
<label for="all_senior" style={{marginRight: '0'}}>전체 대상자 보기</label>
</div>
</div>
:null}
<ul className="tab-menu flex-end">
{tab.map((item, idx) => { return (
<li onClick={() => setTabIndex(idx)} className={idx == tabIndex ? 'active' : null}>
{item.title}
</li>
)})}
</ul>
<div className="content-wrap userlist">
<ul className="tab-content">
<li>
{tab[tabIndex].content}
</li>
</ul>
</div>
</div>
</div>
</div>
</div>
</div>
</main>
);
}