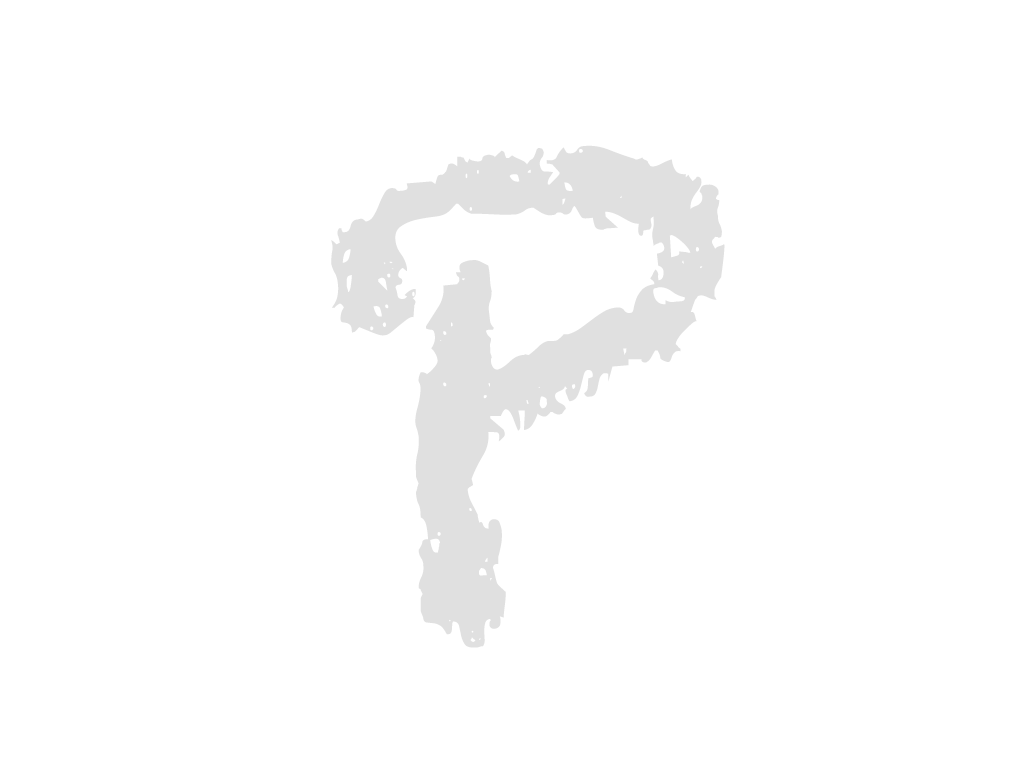
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React from "react";
import { useNavigate, useLocation } from "react-router";
import { useSelector } from "react-redux";
import SubTitle from "../../component/SubTitle.jsx";
import Modal from "../../component/Modal.jsx";
import DetailTitle from "../../component/DetailTitle.jsx";
import Pagination from "../../component/Pagination.jsx";
import CommonFile from "../../component/file/CommonFile.jsx";
import CommonUtil from "../../../resources/js/CommonUtil.js";
export default function UserAuthoriySelect() {
const navigate = useNavigate();
const location = useLocation();
//전역 변수 저장 객체
const state = useSelector((state) => {return state});
const defaultUserId = CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['user_id'];
//검색(엔터)
const searchingEnter = (key) => {
if (key == 'Enter') {
searching();
} else {
return;
}
}
//검색
const searching = () => {
if (CommonUtil.isEmpty(state.loginUser) == false
&& state.loginUser['authority'] == 'ROLE_AGENCY' && isMySenior) {
senior.search['agent_id'] = state.loginUser['user_id'];
} else {
senior.search['agent_id'] = null;
}
setSenior({...senior});
seniorSelectList(1);
}
const [isMySenior, setIsMySenior] = React.useState(true);
React.useEffect(() => {
searching();
}, [isMySenior])
//보호사(간호사)의 돌봄 대상자(시니어)
const [senior, setSenior] = React.useState({seniorList: [], seniorListCount: 0, search: {
'government_id': CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['government_id'],
'agency_id': CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['agency_id'],
'searchType': null,
'searchText': null,
'currentPage': 1,
'perPage': 10,
}});
//보호사(간호사)의 돌봄 대상자(시니어) 목록 조회
const seniorSelectList = (currentPage) => {
senior.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage;
fetch("/user/seniorSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(senior.search),
}).then((response) => response.json()).then((data) => {
data.search = senior.search;
console.log("돌봄 대상자(시니어) 목록 조회 : ", data);
setSenior(data);
}).catch((error) => {
console.log('seniorSelectList() /user/seniorSelectList.json error : ', error);
});
}
//현재 선택한 대상자 정보
const [targetSenior, setTargetSenior] = React.useState({'senior_id': null});
//문진표
const [modalOpen, setModalOpen] = React.useState(false);
const openModal = (senior) => {
targetSenior['senior_id'] = senior['senior_id'];
setTargetSenior(senior);
questionnaireSelectList();
setModalOpen(true);
};
const closeModal = () => {
setTargetSenior({'senior_id': null});
setModalOpen(false);
};
//내원기록
const [modalOpen2, setModalOpen2] = React.useState(false);
const openModal2 = (senior) => {
targetSenior['senior_id'] = senior['senior_id'];
setTargetSenior(senior);
hospitalMedicalRecordSelectList();
setModalOpen2(true);
};
const closeModal2 = () => {
setTargetSenior({'senior_id': null});
setModalOpen2(false);
};
//심전도
const [modalOpen3, setModalOpen3] = React.useState(false);
const openModal3 = (senior) => {
targetSenior['senior_id'] = senior['senior_id'];
setTargetSenior(senior);
ecgSelectList();
setModalOpen3(true);
};
const closeModal3 = () => {
setTargetSenior({'senior_id': null});
setModalOpen3(false);
};
//혈압
const [modalOpen4, setModalOpen4] = React.useState(false);
const openModal4 = (senior) => {
targetSenior['senior_id'] = senior['senior_id'];
setTargetSenior(senior);
bloodPressureSelectList();
setModalOpen4(true);
};
const closeModal4 = () => {
setTargetSenior({'senior_id': null});
setModalOpen4(false);
};
/****************** 문진표 (시작) ******************/
const questionnaireInit = {
'senior_id': null,
'questionnaire_record_idx': null,
'weight_change_amount': 0,
'past_history': null,
'agent_id': defaultUserId,
'medical_record_idx': null,
'smoke_type': false,
'no_smoke_period': null,
'smoke_period': null,
'daily_smoke_amount': null,
'drink_type': false,
'weekly_drink_amount': null,
'onetime_alcoholic_kind': null,
'onetime_bottle_amount': null,
'onetime_cup_amount': null,
'exercise_type': false,
'weekly_exercise_amount': null,
'onetime_exercise_hour': null,
'onetime_exercise_minute': null,
'onetime_exercise_kind': null,
'medication_pill': '',
'medication_pill_etc': '',
};
//문진표 정보
const [questionnaire, setQuestionnaire] = React.useState({...questionnaireInit});
const questionnaireRef = React.useRef({...questionnaireInit});
//문진표 등록
const questionnaireInsert = () => {
questionnaire['senior_id'] = targetSenior['senior_id'];
questionnaire['agent_id'] = defaultUserId;
setQuestionnaire({...questionnaire});
fetch("/hospital/questionnaireInsert.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(questionnaire),
}).then((response) => response.json()).then((data) => {
console.log("문진표 등록 결과(건수) : ", data);
if (data > 0) {
alert("저장완료");
closeModal();
} else {
alert("저장에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('questionnaireInsert() /hospital/questionnaireInsert.json error : ', error);
});
}
//문진표 수정
const questionnaireUpdate = () => {
questionnaire['senior_id'] = targetSenior['senior_id'];
questionnaire['agent_id'] = defaultUserId;
setQuestionnaire({...questionnaire});
fetch("/hospital/questionnaireUpdate.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(questionnaire),
}).then((response) => response.json()).then((data) => {
console.log("문진표 수정 결과(건수) : ", data);
if (data > 0) {
alert("저장완료");
closeModal();
} else {
alert("저장에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('questionnaireUpdate() /hospital/questionnaireUpdate.json error : ', error);
});
}
//문진표 목록 조회
const questionnaireSelectList = () => {
fetch("/hospital/questionnaireSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(targetSenior),
}).then((response) => response.json()).then((data) => {
console.log("문진표 목록 조회 결과(건수) : ", data);
if (CommonUtil.isEmpty(data.questionnaireList) == false) {
let item = data.questionnaireList[0];//최신순으로 가지고오기 때문에, 0 인텍스가 젤 최신
if (CommonUtil.isEmpty(item['medication_pill'])) {
item['medication_pill'] = '';
} else {
const startCheckWord = '기타「';
const endCheckWord = '」';
if (item['medication_pill'].indexOf(startCheckWord) > -1) {
let startIndex = item['medication_pill'].indexOf(startCheckWord);
let lastIndex = item['medication_pill'].lastIndexOf(endCheckWord);
console.log("000000 item['medication_pill_etc'] : ", item['medication_pill_etc'], "item['medication_pill'] : ", item['medication_pill']);
item['medication_pill_etc'] = item['medication_pill'].substring(startIndex + startCheckWord.length, lastIndex);
let medicationPillFront = item['medication_pill'].substring(0, startIndex + startCheckWord.length);
let medicationPillBack = item['medication_pill'].substring(lastIndex);
item['medication_pill'] = medicationPillFront + medicationPillBack;
console.log("333333 item['medication_pill_etc'] : ", item['medication_pill_etc'], "item['medication_pill'] : ", item['medication_pill']);
} else {
item['medication_pill_etc'] = '';
}
}
setQuestionnaire(item);
}
}).catch((error) => {
console.log('questionnaireSelectList() /hospital/questionnaireSelectList.json error : ', error);
});
}
/****************** 문진표 (종료) ******************/
/****************** 병원 진료 기록 (시작) ******************/
const hospitalMedicalRecordInit = {
'senior_id': null,
'medical_record_idx': null,
'medical_date': CommonUtil.getDate(),
'medical_reason': '',
'medical_content': '',
'agent_id': defaultUserId
};
//병원 진료 기록 정보
const [hospitalMedicalRecord, setHospitalMedicalRecord] = React.useState({...hospitalMedicalRecordInit});
const hospitalMedicalRecordRef = React.useRef({...hospitalMedicalRecordInit});
//병원 진료 기록 유효성 검사
const hospitalMedicalRecordValidation = () => {
const target = hospitalMedicalRecord;
const targetRef = hospitalMedicalRecordRef;
if (CommonUtil.isEmpty(target['medical_date']) == true) {
targetRef.current['medical_date'].focus();
alert("진료 일자를 선택해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['medical_reason']) == true) {
targetRef.current['medical_reason'].focus();
alert("진료 사유를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['medical_content']) == true) {
targetRef.current['medical_content'].focus();
alert("진료 내용을 입력해 주세요.");
return false;
}
return true;
}
//병원 진료 기록 등록
const hospitalMedicalRecordInsert = () => {
if (hospitalMedicalRecordValidation() == false) {
return;
}
hospitalMedicalRecord['senior_id'] = targetSenior['senior_id'];
hospitalMedicalRecord['agent_id'] = defaultUserId;
setHospitalMedicalRecord({...hospitalMedicalRecord});
fetch("/hospital/hospitalMedicalRecordInsert.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(hospitalMedicalRecord),
}).then((response) => response.json()).then((data) => {
console.log("병원 진료 기록 등록 결과(건수) : ", data);
if (data > 0) {
setHospitalMedicalRecordInit();
hospitalMedicalRecordSelectList();
//closeModal2();
alert("등록완료");
} else {
alert("등록에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('hospitalMedicalRecordInsert() /hospital/hospitalMedicalRecordInsert.json error : ', error);
});
}
//병원 진료 기록 수정
const hospitalMedicalRecordUpdate = () => {
if (hospitalMedicalRecordValidation() == false) {
return;
}
hospitalMedicalRecord['senior_id'] = targetSenior['senior_id'];
hospitalMedicalRecord['agent_id'] = defaultUserId;
setHospitalMedicalRecord({...hospitalMedicalRecord});
fetch("/hospital/hospitalMedicalRecordUpdate.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(hospitalMedicalRecord),
}).then((response) => response.json()).then((data) => {
console.log("병원 진료 기록 수정 결과(건수) : ", data);
if (data > 0) {
setHospitalMedicalRecordInit();
hospitalMedicalRecordSelectList();
//closeModal2();
alert("수정완료");
} else {
alert("수정에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('hospitalMedicalRecordUpdate() /hospital/hospitalMedicalRecordUpdate.json error : ', error);
});
}
//병원 진료 기록 삭제
const hospitalMedicalRecordDelete = () => {
if (confirm('진료 기록을 삭제하시겠습니까?') == false) {
return;
}
fetch("/hospital/hospitalMedicalRecordDelete.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(hospitalMedicalRecord),
}).then((response) => response.json()).then((data) => {
console.log("병원 진료 기록 삭제 결과(건수) : ", data);
if (data > 0) {
setHospitalMedicalRecordInit();
hospitalMedicalRecordSelectList();
//closeModal2();
alert("삭제완료");
} else {
alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('hospitalMedicalRecordDelete() /hospital/hospitalMedicalRecordDelete.json error : ', error);
});
}
//초기화 취소
const setHospitalMedicalRecordInit = () => {
setHospitalMedicalRecord({...hospitalMedicalRecordInit});
}
//병원 진료 기록 정보
const [hospitalMedicalRecordList, setHospitalMedicalRecordList] = React.useState({hospitalMedicalRecordList: [], hospitalMedicalRecordListCount:0, search: {currentPage: 1, perPage: 5}});
//병원 진료 기록 목록 조회
const hospitalMedicalRecordSelectList = (currentPage) => {
hospitalMedicalRecordList.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage;
hospitalMedicalRecordList.search['senior_id'] = targetSenior['senior_id'];
setHospitalMedicalRecordList({...hospitalMedicalRecordList});
fetch("/hospital/hospitalMedicalRecordSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(hospitalMedicalRecordList.search),
}).then((response) => response.json()).then((data) => {
console.log("병원 진료 기록 목록 조회 결과(건수) : ", data);
data.search = hospitalMedicalRecordList.search;
setHospitalMedicalRecordList(data);
}).catch((error) => {
console.log('hospitalMedicalRecordSelectList() /hospital/hospitalMedicalRecordSelectList.json error : ', error);
});
}
/****************** 병원 진료 기록 (종료) ******************/
/****************** 심전도 (시작) ******************/
const ecgInit = {
'senior_id': null,
'ecg_record_idx': null,
'bradycardia_count': 0,
'tachycardia_count': 0,
'max_heart_rate': 0.0,
'min_heart_rate': 0.0,
'abnormal_heart_rate': 0.0,
'average_heart_rate': 0.0,
'max_rr': 0.0,
'min_rr': 0.0,
'average_rr': 0.0,
'ecg_reading_date': CommonUtil.getDate(),
'ecg_finding_type': '',
'ecg_finding_content': '',
'agent_id': defaultUserId,
'medical_record_idx': null,
'common_group_file_idx': null,
commonFileList: [],//심전도 업로드 파일 목록
isEdit: false,//직접 수정일 때, true (수정 or 등록 상관없음);
};
//심전도 정보
const [ecg, setEcg] = React.useState({...ecgInit});
const ecgRef = React.useRef({...ecgInit});
//심전도 유효성 검사
const ecgValidation = () => {
const target = ecg;
const targetRef = ecgRef;
if (CommonUtil.isEmpty(target['ecg_reading_date']) == true) {
targetRef.current['ecg_reading_date'].focus();
alert("판독 소견 측정 일자를 선택해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['ecg_finding_type']) == true) {
targetRef.current['ecg_finding_type'].focus();
alert("판독 소견 측정 종류를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['ecg_finding_content']) == true) {
targetRef.current['ecg_finding_content'].focus();
alert("판독 소견 측정 내용을 입력해 주세요.");
return false;
}
//파일 입력
if (target.isEdit == false) {
if (CommonUtil.isEmpty(target['commonFileList']) == true) {
//targetRef.current['commonFileList'].focus();
alert("심전도 측정 파일을 올려주세요.");
return false;
}
} else {//직접 입력
if (CommonUtil.isEmpty(target['bradycardia_count']) == true) {
targetRef.current['bradycardia_count'].focus();
alert("서맥 횟수를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['tachycardia_count']) == true) {
targetRef.current['tachycardia_count'].focus();
alert("빈맥 횟수 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['max_heart_rate']) == true) {
targetRef.current['average_heart_rate'].focus();
alert("최대 심박수를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['min_heart_rate']) == true) {
targetRef.current['average_heart_rate'].focus();
alert("최소 심박수를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['average_heart_rate']) == true) {
targetRef.current['average_heart_rate'].focus();
alert("평균 심박수를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['abnormal_heart_rate']) == true) {
targetRef.current['abnormal_heart_rate'].focus();
alert("이상 심박수를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['max_rr']) == true) {
targetRef.current['max_rr'].focus();
alert("최대 R-R를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['min_rr']) == true) {
targetRef.current['min_rr'].focus();
alert("최소 R-R를 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['average_rr']) == true) {
targetRef.current['average_rr'].focus();
alert("평균 R-R를 입력해 주세요.");
return false;
}
}
return true;
}
//심전도 등록
const ecgInsert = () => {
if (ecgValidation() == false) {
return;
}
ecg['senior_id'] = targetSenior['senior_id'];
ecg['agent_id'] = defaultUserId;
setEcg({...ecg});
fetch("/hospital/ecgInsert.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(ecg),
}).then((response) => response.json()).then((data) => {
console.log("심전도 등록 결과(건수) : ", data);
if (data > 0) {
setEcgInit();
ecgSelectList();
//closeModal3();
alert("등록완료");
} else {
alert("등록에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('ecgInsert() /hospital/ecgInsert.json error : ', error);
});
}
//심전도 수정
const ecgUpdate = () => {
if (ecgValidation() == false) {
return;
}
ecg['senior_id'] = targetSenior['senior_id'];
ecg['agent_id'] = defaultUserId;
setEcg({...ecg});
fetch("/hospital/ecgUpdate.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(ecg),
}).then((response) => response.json()).then((data) => {
console.log("심전도 수정 결과(건수) : ", data);
if (data > 0) {
setEcgInit();
ecg.commonFileList = [];
setEcg({...ecg});
ecgSelectList();
//closeModal3();
alert("수정완료");
} else {
alert("수정에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('ecgUpdate() /hospital/ecgUpdate.json error : ', error);
});
}
//심전도 삭제
const ecgDelete = () => {
if (confirm('심전도 판독 소견을 삭제하시겠습니까?') == false) {
return;
}
fetch("/hospital/ecgDelete.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(ecg),
}).then((response) => response.json()).then((data) => {
console.log("심전도 삭제 결과(건수) : ", data);
if (data > 0) {
setEcgInit();
ecg.commonFileList = [];
setEcg({...ecg});
ecgSelectList();
//closeModal3();
alert("삭제완료");
} else {
alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('ecgDelete() /hospital/ecgDelete.json error : ', error);
});
}
//초기화 취소
const setEcgInit = () => {
setEcg({...ecgInit});
}
//심전도 정보
const [ecgList, setEcgList] = React.useState({ecgList: [], ecgListCount:0, search: {currentPage: 1, perPage: 5}});
//심전도 목록 조회
const ecgSelectList = (currentPage) => {
ecgList.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage;
ecgList.search['senior_id'] = targetSenior['senior_id'];
setEcgList({...ecgList});
fetch("/hospital/ecgSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(ecgList.search),
}).then((response) => response.json()).then((data) => {
console.log("심전도 목록 조회 결과(건수) : ", data);
data.search = ecgList.search;
setEcgList(data);
}).catch((error) => {
console.log('ecgSelectList() /hospital/ecgSelectList.json error : ', error);
});
}
/****************** 심전도 기록 (종료) ******************/
/****************** 혈압 (시작) ******************/
const bloodPressureInit = {
'senior_id': null,
'blood_pressure_record_idx': null,
'max_blood_pressure': null,
'min_blood_pressure': null,
'pulse_rate': null,
'medical_record_idx': null,
'blood_pressure_record_date': CommonUtil.getDate(),
'agent_id': defaultUserId
};
//혈압 정보
const [bloodPressure, setBloodPressure] = React.useState({...bloodPressureInit});
const bloodPressureRef = React.useRef({...bloodPressureInit});
//혈압 유효성 검사
const bloodPressureValidation = () => {
const target = bloodPressure;
const targetRef = bloodPressureRef;
if (CommonUtil.isEmpty(target['blood_pressure_record_date']) == true) {
targetRef.current['blood_pressure_record_date'].focus();
alert("진료 일자를 선택해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['max_blood_pressure']) == true) {
targetRef.current['max_blood_pressure'].focus();
alert("최고 혈압을 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['min_blood_pressure']) == true) {
targetRef.current['min_blood_pressure'].focus();
alert("최저 혈압을 입력해 주세요.");
return false;
}
if (CommonUtil.isEmpty(target['pulse_rate']) == true) {
targetRef.current['pulse_rate'].focus();
alert("맥박수를 입력해 주세요.");
return false;
}
return true;
}
//혈압 등록
const bloodPressureInsert = () => {
if (bloodPressureValidation() == false) {
return;
}
bloodPressure['senior_id'] = targetSenior['senior_id'];
bloodPressure['agent_id'] = defaultUserId;
setBloodPressure({...bloodPressure});
fetch("/hospital/bloodPressureInsert.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(bloodPressure),
}).then((response) => response.json()).then((data) => {
console.log("혈압 등록 결과(건수) : ", data);
if (data > 0) {
setBloodPressureInit();
bloodPressureSelectList();
//closeModal2();
alert("등록완료");
} else {
alert("등록에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('bloodPressureInsert() /hospital/bloodPressureInsert.json error : ', error);
});
}
//혈압 수정
const bloodPressureUpdate = () => {
if (bloodPressureValidation() == false) {
return;
}
bloodPressure['senior_id'] = targetSenior['senior_id'];
bloodPressure['agent_id'] = defaultUserId;
setBloodPressure({...bloodPressure});
fetch("/hospital/bloodPressureUpdate.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(bloodPressure),
}).then((response) => response.json()).then((data) => {
console.log("혈압 수정 결과(건수) : ", data);
if (data > 0) {
setBloodPressureInit();
bloodPressureSelectList();
//closeModal2();
alert("수정완료");
} else {
alert("수정에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('bloodPressureUpdate() /hospital/bloodPressureUpdate.json error : ', error);
});
}
//혈압 삭제
const bloodPressureDelete = () => {
if (confirm('혈압 측정 정보를 삭제하시겠습니까?') == false) {
return;
}
fetch("/hospital/bloodPressureDelete.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(bloodPressure),
}).then((response) => response.json()).then((data) => {
console.log("혈압 삭제 결과(건수) : ", data);
if (data > 0) {
setBloodPressureInit();
bloodPressureSelectList();
//closeModal2();
alert("삭제완료");
} else {
alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다.");
}
}).catch((error) => {
console.log('bloodPressureDelete() /hospital/bloodPressureDelete.json error : ', error);
});
}
//초기화 취소
const setBloodPressureInit = () => {
setBloodPressure({...bloodPressureInit});
}
//혈압 정보
const [bloodPressureList, setBloodPressureList] = React.useState({bloodPressureList: [], bloodPressureListCount:0, search: {currentPage: 1, perPage: 5}});
//혈압 목록 조회
const bloodPressureSelectList = (currentPage) => {
bloodPressureList.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage;
bloodPressureList.search['senior_id'] = targetSenior['senior_id'];
setBloodPressureList({...bloodPressureList});
fetch("/hospital/bloodPressureSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(bloodPressureList.search),
}).then((response) => response.json()).then((data) => {
console.log("혈압 목록 조회 결과(건수) : ", data);
data.search = bloodPressureList.search;
setBloodPressureList(data);
}).catch((error) => {
console.log('bloodPressureSelectList() /hospital/bloodPressureSelectList.json error : ', error);
});
}
/****************** 혈압 (종료) ******************/
React.useEffect(() => {
searching();
}, []);
//현재 탭 Index
const [tabIndex, setTabIndex] = React.useState(0);
//탭 초기화
const tab = [{
title: `문진표관리`,
content: (
<div>
<div className="flex equip-tab">
<SubTitle explanation={"돌봄 대상자의 병원 내원 기록 및 문진표를 관리할 수 있습니다."} />
</div>
<table className={"protector-user"}>
<thead>
<tr>
<th>No</th>
<th>소속기관명</th>
<th>이름</th>
<th>생년월일</th>
<th>성별</th>
<th>연락처</th>
<th>문진표관리</th>
<th>내원기록관리</th>
</tr>
</thead>
<tbody>
{senior.seniorList.map((item, idx) => { return (
<tr key={idx}>
<td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td>
<td data-label="소속기관명">{item['agency_name']}</td>
<td data-label="이름">{item['user_name']}</td>
<td data-label="생년월일">{item['user_birth']}</td>
<td data-label="성별">{item['user_gender']}</td>
<td data-label="연락처">{item['user_phonenumber']}</td>
<td data-label="문진표관리">
<button className="btn-small gray-btn" onClick={() => openModal(item)}>작성하기</button>
</td>
<td data-label="내원기록관리">
<button className="btn-small gray-btn" onClick={() => openModal2(item)}>작성하기</button>
</td>
</tr>
)})}
{CommonUtil.isEmpty(senior.seniorList) ?
<tr>
<td colSpan={8}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={senior.search.currentPage}
perPage={senior.search.perPage}
totalCount={senior.seniorListCount}
maxRange={5}
click={seniorSelectList}
/>
</div>
)
}, {
title: `심전도관리`,
content: (
<div>
<div className="flex equip-tab">
<SubTitle explanation={"돌봄 대상자의 심전도 정보를 관리할 수 있습니다."} />
</div>
<table className={"protector-user"}>
<thead>
<tr>
<th>No</th>
<th>소속기관명</th>
<th>이름</th>
<th>생년월일</th>
<th>성별</th>
<th>연락처</th>
<th>심전도관리</th>
</tr>
</thead>
<tbody>
{senior.seniorList.map((item, idx) => { return (
<tr key={idx}>
<td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td>
<td data-label="소속기관명">{item['agency_name']}</td>
<td data-label="이름">{item['user_name']}</td>
<td data-label="생년월일">{item['user_birth']}</td>
<td data-label="성별">{item['user_gender']}</td>
<td data-label="연락처">{item['user_phonenumber']}</td>
<td data-label="심전도관리">
<button className="btn-small gray-btn" onClick={() => openModal3(item)}>심전도관리</button>
</td>
</tr>
)})}
{CommonUtil.isEmpty(senior.seniorList) ?
<tr>
<td colSpan={7}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={senior.search.currentPage}
perPage={senior.search.perPage}
totalCount={senior.seniorListCount}
maxRange={5}
click={seniorSelectList}
/>
</div>
)
}, {
title: `혈압관리`,
content: (
<div>
<div className="flex equip-tab">
<SubTitle explanation={"돌봄 대상자의 혈압 정보를 관리할 수 있습니다."} />
</div>
<table className={"protector-user"}>
<thead>
<tr>
<th>No</th>
<th>소속기관명</th>
<th>이름</th>
<th>생년월일</th>
<th>성별</th>
<th>연락처</th>
<th>혈압관리</th>
</tr>
</thead>
<tbody>
{senior.seniorList.map((item, idx) => { return (
<tr key={idx}>
<td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td>
<td data-label="소속기관명">{item['agency_name']}</td>
<td data-label="이름">{item['user_name']}</td>
<td data-label="생년월일">{item['user_birth']}</td>
<td data-label="성별">{item['user_gender']}</td>
<td data-label="연락처">{item['user_phonenumber']}</td>
<td data-label="혈압관리">
<button className="btn-small gray-btn" onClick={() => openModal4(item)}>혈압관리</button>
</td>
</tr>
)})}
{CommonUtil.isEmpty(senior.seniorList) ?
<tr>
<td colSpan={7}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={senior.search.currentPage}
perPage={senior.search.perPage}
totalCount={senior.seniorListCount}
maxRange={5}
click={seniorSelectList}
/>
</div>
)
}];
return (
<main>
{/* <Modal_Questionnaire open={modalOpen} close={closeModal} /> */}
{/* <Modal_MedicalHistory open={modalOpen2} close={closeModal2} /> */}
{/* <Modal_ECG open={modalOpen3} close={closeModal3} /> */}
{/* <Modal_Blood open={modalOpen4} close={closeModal4} /> */}
<div className="search-management flex-start margin-bottom2">
<select style={{maxWidth: '150px'}}
onChange={(e) => {senior.search.searchType = e.target.value; setSenior({...senior});}}>
<option value="" selected={CommonUtil.isEmpty(senior.search.searchType)}>전체</option>
<option value="user_name" selected={senior.search.searchType == 'user_name'}>이름</option>
<option value="user_id" selected={senior.search.searchType == 'user_id'}>아이디</option>
<option value="user_phonenumber" selected={senior.search.searchType == 'user_phonenumber'}>연락처</option>
</select>
<input type="text" className="senior-search" value={senior.search.searchText}
onChange={(e) => {senior.search.searchText = e.target.value; setSenior({...senior});}}
onKeyUp={(e) => searchingEnter(e)}/>
<button className="btn-small gray-btn" onClick={searching}>검색</button>
</div>
<div className="content-wrap">
<DetailTitle contentTitle={"대상자의 문진표 / 심전도 / 혈압 관리를 할 수 있습니다."} />
<div style={{ height: "calc(100% - 61px)" }}>
<div className="right" style={{ height: "100%", }}>
<div style={{ height: "100%" }}>
<div className="tab-container" style={{ marginTop: "5rem"}}>
{CommonUtil.isEmpty(state.loginUser) == false && state.loginUser['authority'] == 'ROLE_AGENCY' ?
<div className="flex-end margin-bottom">
<div className="flex searchselect" style={{width: 'auto'}}>
<input type="radio" id="my_senior" name="senior" checked={isMySenior}
onChange={(e) => {e.target.checked ? setIsMySenior(true) : null}}/>
<label for="my_senior" style={{marginRight: '3rem'}}>나의 대상자 보기</label>
<input type="radio" id="all_senior" name="senior" checked={!isMySenior}
onChange={(e) => {e.target.checked ? setIsMySenior(false) : null}}/>
<label for="all_senior" style={{marginRight: '0'}}>전체 대상자 보기</label>
</div>
</div>
:null}
<ul className="tab-menu flex-end">
{tab.map((item, idx) => { return (
<li onClick={() => setTabIndex(idx)} className={idx == tabIndex ? 'active' : null}>
{item.title}
</li>
)})}
</ul>
<div className="content-wrap userlist">
<ul className="tab-content">
<li>
{tab[tabIndex].content}
</li>
</ul>
</div>
</div>
</div>
</div>
</div>
</div>
<Modal open={modalOpen} close={closeModal} header="문진표 작성">
<div className="board-wrap">
<div>
<table className="margin-bottom2 questionnaire-table">
<tr>
<th>흡연을 하십니까?</th>
<td className="flex-start">
<input type="radio" name="smoke" id="smoke-true"
checked={questionnaire['smoke_type'] == true}
onChange={(e) => {
if (e.target.checked) {
questionnaire['smoke_type'] = true;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="smoke-true">예</label>
<input type="radio" name="smoke" id="smoke-false"
checked={questionnaire['smoke_type'] == false}
onChange={(e) => {
if (e.target.checked) {
questionnaire['smoke_type'] = false;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="smoke-false">아니요</label>
</td>
</tr>
<tr>
<th>음주를 하십니까?</th>
<td className="flex-start">
<input type="radio" name="drink" id="drink-true"
checked={questionnaire['drink_type'] == true}
onChange={(e) => {
if (e.target.checked) {
questionnaire['drink_type'] = true;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="drink-true">예</label>
<input type="radio" name="drink" id="drink-false"
checked={questionnaire['drink_type'] == false}
onChange={(e) => {
if (e.target.checked) {
questionnaire['drink_type'] = false;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="drink-false">아니요</label>
</td>
</tr>
<tr>
<th>일주일에 운동을 몇회 하십니까?</th>
<td className="flex-start">
<input type="radio" name="exercise" id="exercise-false"
checked={questionnaire['exercise_type'] == false}
onChange={(e) => {
if (e.target.checked) {
questionnaire['exercise_type'] = false;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="exercise-false">안함</label>
<input type="radio" name="exercise" id="exercise-1"
checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 1}
onChange={(e) => {
if (e.target.checked) {
questionnaire['exercise_type'] = true;
questionnaire['weekly_exercise_amount'] = 1;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="exercise-1">1회</label>
<input type="radio" name="exercise" id="exercise-2"
checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 2}
onChange={(e) => {
if (e.target.checked) {
questionnaire['exercise_type'] = true;
questionnaire['weekly_exercise_amount'] = 2;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="exercise-2">2회</label>
<input type="radio" name="exercise" id="exercise-3"
checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 3}
onChange={(e) => {
if (e.target.checked) {
questionnaire['exercise_type'] = true;
questionnaire['weekly_exercise_amount'] = 3;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="exercise-3">3회</label>
<input type="radio" name="exercise" id="exercise-4"
checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 4}
onChange={(e) => {
if (e.target.checked) {
questionnaire['exercise_type'] = true;
questionnaire['weekly_exercise_amount'] = 4;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="exercise-4">4회</label>
<input type="radio" name="exercise" id="exercise-5"
checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 5}
onChange={(e) => {
if (e.target.checked) {
questionnaire['exercise_type'] = true;
questionnaire['weekly_exercise_amount'] = 5;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="exercise-5">5회</label>
<input type="radio" name="exercise" id="exercise-6"
checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 6}
onChange={(e) => {
if (e.target.checked) {
questionnaire['exercise_type'] = true;
questionnaire['weekly_exercise_amount'] = 6;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="exercise-6">6회</label>
<input type="radio" name="exercise" id="exercise-7"
checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 7}
onChange={(e) => {
if (e.target.checked) {
questionnaire['exercise_type'] = true;
questionnaire['weekly_exercise_amount'] = 7;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="exercise-7">매일</label>
</td>
</tr>
<tr>
<th>최근 3개월 동안 갑작스런 체중 변화가 있었습니까?</th>
<td className="flex-start">
<input type="radio" name="weight" id="weight-up"
checked={questionnaire['weight_change_amount'] == 1}
onChange={(e) => {
if (e.target.checked) {
questionnaire['weight_change_amount'] = 1;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="weight-up">예 - 증가</label>
<input type="radio" name="weight" id="weight-down"
checked={questionnaire['weight_change_amount'] == -1}
onChange={(e) => {
if (e.target.checked) {
questionnaire['weight_change_amount'] = -1;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="weight-down">예 - 감소</label>
<input type="radio" name="weight" id="weight-false"
checked={questionnaire['weight_change_amount'] == 0}
onChange={(e) => {
if (e.target.checked) {
questionnaire['weight_change_amount'] = 0;
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="weight-false">아니요</label>
</td>
</tr>
<tr>
<th>현재 복용중인 약이 있으면 체크를 해주세요.</th>
<td className="flex-start">
<input type="checkbox" name="pills" id="pills-1"
checked={questionnaire['medication_pill'].indexOf('아스피린(항혈소판제)') > -1}
onChange={(e) => {
if (e.target.checked) {
questionnaire['medication_pill'] += '아스피린(항혈소판제),';
setQuestionnaire({...questionnaire});
} else {
questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('아스피린(항혈소판제),', '');
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="pills-1">아스피린(항혈소판제)</label>
<input type="checkbox" name="pills" id="pills-2"
checked={questionnaire['medication_pill'].indexOf('당뇨약') > -1}
onChange={(e) => {
if (e.target.checked) {
questionnaire['medication_pill'] += '당뇨약,';
setQuestionnaire({...questionnaire});
} else {
questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('당뇨약,', '');
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="pills-2">당뇨약</label>
<input type="checkbox" name="pills" id="pills-3"
checked={questionnaire['medication_pill'].indexOf('고혈압약') > -1}
onChange={(e) => {
if (e.target.checked) {
questionnaire['medication_pill'] += '고혈압약,';
setQuestionnaire({...questionnaire});
} else {
questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('고혈압약,', '');
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="pills-3">고혈압약</label>
<input type="checkbox" name="pills" id="pills-etc"
checked={questionnaire['medication_pill'].indexOf('기타「」,') > -1}
onChange={(e) => {
if (e.target.checked) {
questionnaire['medication_pill'] += '기타「」,';
setQuestionnaire({...questionnaire});
} else {
questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('기타「」,', '');
questionnaire['medication_pill_etc'] = '';
setQuestionnaire({...questionnaire});
}}
}
/>
<label for="pills-etc">기타</label>
<input type="text" style={{width: 'max-content', marginLeft: '1rem'}}
disabled={questionnaire['medication_pill'].indexOf('기타「」,') == -1}
value={questionnaire['medication_pill_etc']}
onChange={(e) => {
questionnaire['medication_pill_etc'] = e.target.value;
setQuestionnaire({...questionnaire});
}}
/>
</td>
</tr>
</table>
</div>
<div className="flex-center">
{CommonUtil.isEmpty(questionnaire['questionnaire_record_idx'])
? <button className="btn-small red-btn" onClick={questionnaireInsert}>저장</button>
: <button className="btn-small red-btn" onClick={questionnaireUpdate}>저장</button>
}
</div>
</div>
</Modal>
<Modal open={modalOpen2} close={closeModal2} header="내원기록 관리 및 조회">
<div className="board-wrap">
<table className="margin-bottom2 senior-insert ">
<tr>
<th>진료 일자</th>
<td>
<input type="date"
value={hospitalMedicalRecord['medical_date']}
onChange={(e) => {
hospitalMedicalRecord['medical_date'] = e.target.value;
setHospitalMedicalRecord({...hospitalMedicalRecord});
}}
ref={el => hospitalMedicalRecordRef.current['medical_date'] = el}
/>
</td>
</tr>
<tr>
<th>진료 사유</th>
<td className="flex-start ">
<input type="text"
value={hospitalMedicalRecord['medical_reason']}
onChange={(e) => {
hospitalMedicalRecord['medical_reason'] = e.target.value;
setHospitalMedicalRecord({...hospitalMedicalRecord});
}}
ref={el => hospitalMedicalRecordRef.current['medical_reason'] = el}
/>
</td>
</tr>
<tr>
<th>진료 내용</th>
<td colSpan={3}>
<textarea className="medicine" cols="30" rows="2"
value={hospitalMedicalRecord['medical_content']}
onChange={(e) => {
hospitalMedicalRecord['medical_content'] = e.target.value;
setHospitalMedicalRecord({...hospitalMedicalRecord});
}}
ref={el => hospitalMedicalRecordRef.current['medical_content'] = el}
></textarea>
</td>
</tr>
</table>
<div className="btn-wrap flex-center margin-bottom5">
{CommonUtil.isEmpty(hospitalMedicalRecord['medical_record_idx'])
? <button className="btn-small red-btn" onClick={hospitalMedicalRecordInsert}>등록</button>
: <>
<button className="btn-small gray-btn" onClick={setHospitalMedicalRecordInit}>수정취소</button>
<button className="btn-small red-btn" onClick={hospitalMedicalRecordUpdate}>수정</button>
<button className="btn-small red-btn" onClick={hospitalMedicalRecordDelete}>삭제</button>
</>
}
</div>
<div>
<table className="caregiver-user senior-insert senior-table">
<thead>
<tr>
<th>No</th>
<th>진료 일자</th>
<th>진료 사유</th>
<th>진료 내용</th>
<th>기록 작성자</th>
</tr>
</thead>
<tbody>
{hospitalMedicalRecordList.hospitalMedicalRecordList.map((item, idx) => { return (
<tr key={idx} onClick={() => {setHospitalMedicalRecord(item)}}>
<td data-label="No">{hospitalMedicalRecordList.hospitalMedicalRecordListCount - idx - (hospitalMedicalRecordList.search.currentPage - 1) * hospitalMedicalRecordList.search.perPage}</td>
<td data-label="진료 일자">{item['medical_date']}</td>
<td data-label="진료 내용">{item['medical_reason']}</td>
<td data-label="진료 사유">{item['medical_content']}</td>
<td data-label="기록 작성자">{item['insert_user_name']}</td>
</tr>
)})}
{CommonUtil.isEmpty(hospitalMedicalRecordList.hospitalMedicalRecordList) ?
<tr>
<td colSpan={5}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={hospitalMedicalRecordList.search.currentPage}
perPage={hospitalMedicalRecordList.search.perPage}
totalCount={hospitalMedicalRecordList.hospitalMedicalRecordListCount}
maxRange={5}
click={hospitalMedicalRecordSelectList}
/>
</div>
</div>
</Modal>
<Modal open={modalOpen3} close={closeModal3} header="심전도판독소견 관리 및 조회">
<div className="board-wrap">
<table className="margin-bottom2 senior-insert ">
<tr>
<th>측정 일자</th>
<td colSpan={5}>
<input type="date" value={ecg['ecg_reading_date']}
onChange={(e) => {
ecg['ecg_reading_date'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['ecg_reading_date'] = el}
/>
</td>
</tr>
<tr>
<th>판독 소견 종류</th>
<td colSpan={5}>
<input type="text" value={ecg['ecg_finding_type']}
onChange={(e) => {
ecg['ecg_finding_type'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['ecg_finding_type'] = el}
/>
</td>
</tr>
<tr>
<th>판독 소견 내용</th>
<td colSpan={5}>
<textarea className="medicine" cols="30" rows="2"
value={ecg['ecg_finding_content']}
onChange={(e) => {
ecg['ecg_finding_content'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['ecg_finding_content'] = el}
></textarea>
</td>
</tr>
{ecg.isEdit == false
? <tr>
<th>측정 파일</th>
<td colSpan={5}>
<CommonFile commonFileList={ecg['commonFileList']} multiple={false} accept={'.dat, .ecg'}/>
</td>
</tr>
: <>
<tr>
<th>서맥 횟수</th>
<td>
<input type="number" value={ecg['bradycardia_count']}
onChange={(e) => {
ecg['bradycardia_count'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['bradycardia_count'] = el}
/>
</td>
<th>빈맥 횟수</th>
<td>
<input type="number" value={ecg['tachycardia_count']}
onChange={(e) => {
ecg['tachycardia_count'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['tachycardia_count'] = el}
/>
</td>
<th>이상 심박수</th>
<td>
<input type="number" value={ecg['abnormal_heart_rate']}
onChange={(e) => {
ecg['abnormal_heart_rate'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['abnormal_heart_rate'] = el}
/>
</td>
</tr>
<tr>
<th>최대 심박수</th>
<td>
<input type="number" value={ecg['max_heart_rate']}
onChange={(e) => {
ecg['max_heart_rate'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['max_heart_rate'] = el}
/>
</td>
<th>최소 심박수</th>
<td>
<input type="number" value={ecg['min_heart_rate']}
onChange={(e) => {
ecg['min_heart_rate'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['min_heart_rate'] = el}
/>
</td>
<th>평균 심박수</th>
<td>
<input type="number" value={ecg['average_heart_rate']}
onChange={(e) => {
ecg['average_heart_rate'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['average_heart_rate'] = el}
/>
</td>
</tr>
<tr>
<th>최대 R-R</th>
<td>
<input type="number" value={ecg['max_rr']}
onChange={(e) => {
ecg['max_rr'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['max_rr'] = el}
/>
</td>
<th>최소 R-R</th>
<td>
<input type="number" value={ecg['min_rr']}
onChange={(e) => {
ecg['min_rr'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['min_rr'] = el}
/>
</td>
<th>평균 R-R</th>
<td>
<input type="number" value={ecg['average_rr']}
onChange={(e) => {
ecg['average_rr'] = e.target.value;
setEcg({...ecg});
}}
ref={el => ecgRef.current['average_rr'] = el}
/>
</td>
</tr>
</>}
</table>
<div className="btn-wrap flex-center margin-bottom5">
{CommonUtil.isEmpty(ecg['ecg_record_idx'])
? <button className="btn-small red-btn" onClick={ecgInsert}>등록</button>
: <>
<button className="btn-small gray-btn" onClick={setEcgInit}>수정취소</button>
<button className="btn-small red-btn" onClick={ecgUpdate}>수정</button>
<button className="btn-small red-btn" onClick={ecgDelete}>삭제</button>
</>
}
</div>
<div>
<table className="caregiver-user senior-table">
<thead>
<tr>
<th>No</th>
<th>소견 작성 일자</th>
<th>판독 소견 종류</th>
<th>소견 작성자</th>
</tr>
</thead>
<tbody>
{ecgList.ecgList.map((item, idx) => { return (
<tr key={idx} onClick={() => {item.isEdit = false; setEcg(item);}}>
<td data-label="No">{ecgList.ecgListCount - idx - (ecgList.search.currentPage - 1) * ecgList.search.perPage}</td>
<td data-label="소견 작성 일자">{item['ecg_reading_date']}</td>
<td data-label="판독 소견 종류">{item['ecg_finding_type']}</td>
<td data-label="소견 작성자">{item['insert_user_name']}</td>
</tr>
)})}
{CommonUtil.isEmpty(ecgList.ecgList) ?
<tr>
<td colSpan={4}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={ecgList.search.currentPage}
perPage={ecgList.search.perPage}
totalCount={ecgList.ecgListCount}
maxRange={5}
click={ecgSelectList}
/>
</div>
</div>
</Modal>
<Modal open={modalOpen4} close={closeModal4} header="혈압측정결과 관리 및 조회">
<div className="board-wrap">
<table className="margin-bottom2 senior-insert ">
<tr>
<th>측정일자</th>
<td>
<input type="date" value={bloodPressure['blood_pressure_record_date']}
onChange={(e) => {
bloodPressure['blood_pressure_record_date'] = e.target.value;
setBloodPressure({...bloodPressure});
}}
ref={el => bloodPressureRef.current['blood_pressure_record_date'] = el}
/>
</td>
</tr>
<tr>
<th>최고혈압</th>
<td>
<input type="number" value={bloodPressure['max_blood_pressure']}
onChange={(e) => {
bloodPressure['max_blood_pressure'] = e.target.value;
setBloodPressure({...bloodPressure});
}}
ref={el => bloodPressureRef.current['max_blood_pressure'] = el}
/>
</td>
</tr>
<tr>
<th>최저혈압</th>
<td>
<input type="number" value={bloodPressure['min_blood_pressure']}
onChange={(e) => {
bloodPressure['min_blood_pressure'] = e.target.value;
setBloodPressure({...bloodPressure});
}}
ref={el => bloodPressureRef.current['min_blood_pressure'] = el}
/>
</td>
</tr>
<tr>
<th>맥박수</th>
<td>
<input type="number" value={bloodPressure['pulse_rate']}
onChange={(e) => {
bloodPressure['pulse_rate'] = e.target.value;
setBloodPressure({...bloodPressure});
}}
ref={el => bloodPressureRef.current['pulse_rate'] = el}
/>
</td>
</tr>
</table>
<div className="btn-wrap flex-center margin-bottom5">
{CommonUtil.isEmpty(bloodPressure['blood_pressure_record_idx'])
? <button className="btn-small red-btn" onClick={bloodPressureInsert}>등록</button>
: <>
<button className="btn-small gray-btn" onClick={setBloodPressureInit}>수정취소</button>
<button className="btn-small red-btn" onClick={bloodPressureUpdate}>수정</button>
<button className="btn-small red-btn" onClick={bloodPressureDelete}>삭제</button>
</>
}
</div>
<div>
<table className="caregiver-user senior-insert senior-table">
<thead>
<tr>
<th>No</th>
<th>기록 작성일</th>
<th>최고 혈압</th>
<th>최저 혈압</th>
<th>맥박수</th>
<th>기록 작성자</th>
</tr>
</thead>
<tbody>
{bloodPressureList.bloodPressureList.map((item, idx) => { return (
<tr key={idx} onClick={() => {setBloodPressure(item);}}>
<td data-label="No">{bloodPressureList.bloodPressureListCount - idx - (bloodPressureList.search.currentPage - 1) * bloodPressureList.search.perPage}</td>
<td data-label="기록 작성일">{item['blood_pressure_record_date']}</td>
<td data-label="최고 혈압">{item['max_blood_pressure']}</td>
<td data-label="최저 혈압">{item['min_blood_pressure']}</td>
<td data-label="맥박수">{item['pulse_rate']}</td>
<td data-label="기록 작성자">{item['insert_user_name']}</td>
</tr>
)})}
{CommonUtil.isEmpty(bloodPressureList.bloodPressureList) ?
<tr>
<td colSpan={6}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={bloodPressureList.search.currentPage}
perPage={bloodPressureList.search.perPage}
totalCount={bloodPressureList.bloodPressureListCount}
maxRange={5}
click={bloodPressureSelectList}
/>
</div>
</div>
</Modal>
</main>
);
}