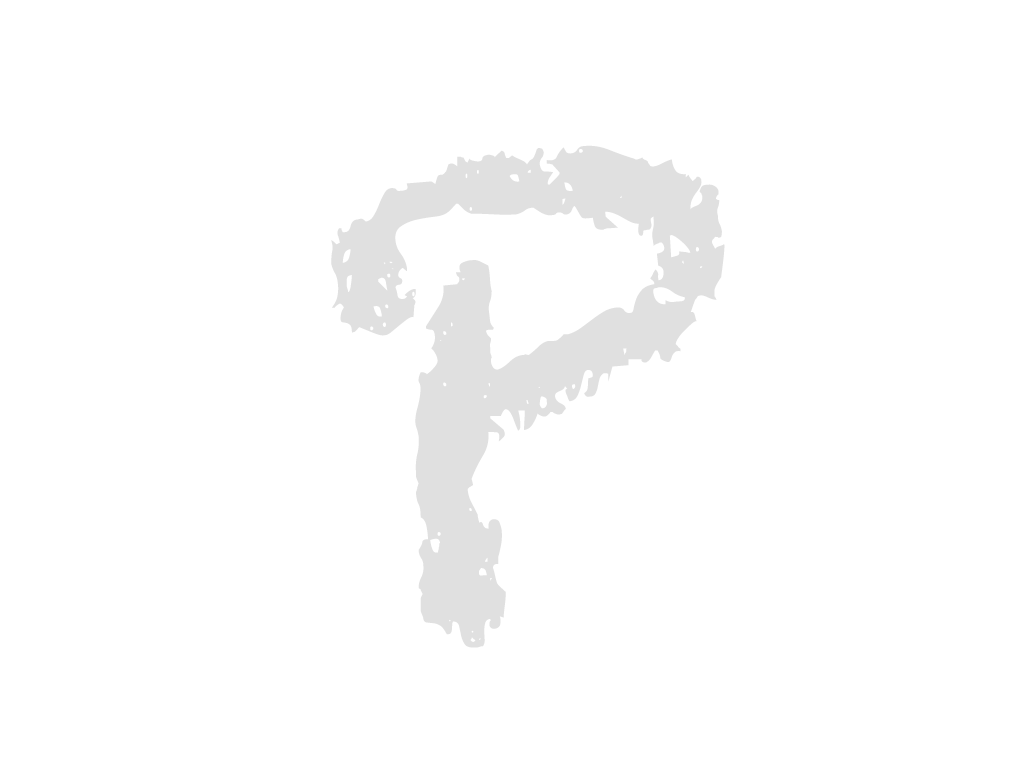
File name
Commit message
Commit date
2023-04-18
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React from "react";
import { useNavigate } from "react-router";
import { useSelector } from "react-redux";
import { useStateWithCallbackLazy } from 'use-state-with-callback';
import DetailTitle from "../../component/DetailTitle.jsx";
import SubTitle from "../../component/SubTitle.jsx";
import Modal from "../../component/Modal.jsx";
import House from "../../../resources/files/icon/house.png";
import Arrow from "../../../resources/files/icon/arrow.png";
import Pagination from "../../component/Pagination.jsx";
import CommonUtil from "../../../resources/js/CommonUtil.js";
export default function GovernmentEquipmentSelect() {
const navigate = useNavigate();
//전역 변수 저장 객체
const state = useSelector((state) => { return state });
const defaultGovernmentId = CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['government_id'];
const defaultAgencyId = CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['agency_id'];
// 시스템 코드 - 장비 상태
const [equipmentStates, setEquipmentStates] = React.useState({});
// 시스템 코드 - 장비 상태 조회
const equipmentStatesSelect = () => {
console.log('equipmentStatesSelect Function Run');
//fetch post
fetch("/common/systemCode/equipmentStatesSelect.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify({})
}).then((response) => response.json()).then((data) => {
console.log('equipmentStatesSelect response : ', data);
setEquipmentStates(data);
}).catch((error) => {
console.log('equipmentStatesSelect error : ', error);
});
}
//공통 검색 정보
const [equipmentSearch, setEquipmentSearch] = React.useState({
'equipment_state': null,
'searchType': null,
'searchText': null,
});
//장비 정보 변경
const equipmentSearchChange = (targetKey, value) => {
equipmentSearch[targetKey] = value;
setEquipmentSearch({ ...equipmentSearch });
}
//장비 검색
const equipmentSearching = () => {
for (const key in equipmentSearch) {
rentalEquipmentSearch[key] = equipmentSearch[key];
}
setRentalEquipmentSearch({ ...rentalEquipmentSearch });
rentalEquipmentSelectList(1);
}
const equipmentSearchingEnter = (key) => {
if (key == 'Enter') {
equipmentSearching();
} else {
return;
}
}
//입고 및 미대여 장비 검색 정보
const [rentalEquipmentSearch, setRentalEquipmentSearch] = React.useState({
'government_id': defaultGovernmentId,
'agency_id': defaultAgencyId,
'currentPage': 1,
'perPage': 10,
});
//입고 및 미대여 목록
const [rentalEquipment, setRentalEquipment] = React.useState({ equipmentList: [], equipmentListCount: 0 });
console.log("rentalEquipmentSearch:", rentalEquipmentSearch)
//입고 및 미대여 목록 조회
const rentalEquipmentSelectList = (currentPage) => {
rentalEquipmentSearch.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage;
setRentalEquipmentSearch({ ...rentalEquipmentSearch });
fetch("/equipment/equipmentSelectList.json", {
method: "POST",
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
body: JSON.stringify(rentalEquipmentSearch)
}).then((response) => response.json()).then((data) => {
console.log('rentalEquipmentSelectList response : ', data);
setRentalEquipment(data);
}).catch((error) => {
console.log('rentalEquipmentSelectList error : ', error);
});
}
//Mounted
React.useEffect(() => {
equipmentStatesSelect();
equipmentSearching();
}, []);
//대여(사용) 중 장비 목록 내용
const rentalContent = (
<>
<table class="caregiver-user protector-user">
<thead>
<tr>
<th>No</th>
<th>모델명</th>
<th>시리얼넘버</th>
<th>입고일자</th>
<th>장비상태</th>
<th>기관</th>
<th>대여시행자</th>
<th>대여일</th>
<th>대여여부</th>
<th>장비사용대상자</th>
</tr>
</thead>
<tbody>
{rentalEquipment.equipmentList.map((item, idx) => {
return (
<tr>
<td data-label="No">{rentalEquipment.equipmentListCount - idx - (rentalEquipmentSearch.currentPage - 1) * rentalEquipmentSearch.perPage}</td>
<td data-label="모델명">{item['equipment_name']}</td>
<td data-label="시리얼넘버">{item['equipment_serial_number']}</td>
<td data-label="입고일자">{item['equipment_stock_date']}</td>
<td data-label="장비상태">{equipmentStates[item['equipment_state']]}</td>
<td>{item['agency_name']}</td>
<td data-label="대여시행자">
{CommonUtil.isEmpty(item['rental_detail_insert_user_name']) == false ?
item['rental_detail_insert_user_name'] + '(' + item['rental_detail_insert_user_id'] + ')'
: '-'}
</td>
<td data-label="대여일">{CommonUtil.isEmpty(item['rental_start_date']) == false ? item['rental_start_date'] : '-'}</td>
<td data-label="대여여부">{CommonUtil.isEmpty(item['user_name']) == false ? '대여중' : '미대여'}</td>
<td data-label="장비사용대상자">{CommonUtil.isEmpty(item['user_name']) == false ? item['user_name'] : '-'}</td>
</tr>
)
})}
{CommonUtil.isEmpty(rentalEquipment.equipmentList) ?
<tr>
<td colSpan={11}>조회된 데이터가 없습니다</td>
</tr>
: null}
</tbody>
</table>
<Pagination
currentPage={rentalEquipmentSearch.currentPage}
perPage={rentalEquipmentSearch.perPage}
totalCount={rentalEquipment.equipmentListCount}
maxRange={5}
click={rentalEquipmentSelectList}
/>
</>
);
return (
<main>
<div className="search-management flex-end margin-bottom2 margin-top gap">
<select style={{ maxWidth: '150px' }}
onChange={(e) => equipmentSearchChange('equipment_state', e.target.value)}>
<option value="">상태</option>
{Object.keys(equipmentStates).map((key, idx) => {
return (
<option key={key} value={key}>
{equipmentStates[key]}
</option>
)
})}
</select>
<select style={{ maxWidth: '150px' }}
onChange={(e) => equipmentSearchChange('searchType', e.target.value)}>
<option value="">전체</option>
<option value="equipment_name">모델명</option>
<option value="equipment_serial_number">시리얼넘버</option>
</select>
<input type="text"
value={equipmentSearch.searchText}
onChange={(e) => equipmentSearchChange('searchText', e.target.value)}
onKeyUp={(e) => equipmentSearchingEnter(e.key)}
/>
<button className={"btn-small gray-btn"} style={{ maxWidth: '150px' }} onClick={equipmentSearching}>검색</button>
</div>
<div className="content-wrap">
<DetailTitle contentTitle={"장비 사용 현황"} />
<div className="board-wrap" style={{ marginTop: "3rem" }} >
<div className="btn-wrap margin-bottom">
<div className="btn-wrap flex-end margin-bottom ">
<button className={"btn-small gray-btn"} onClick={() => navigate("/AgencySeniorSelect")}>대상자 관리</button>
</div>
{rentalContent}
</div>
</div>
</div>
</main >
);
}