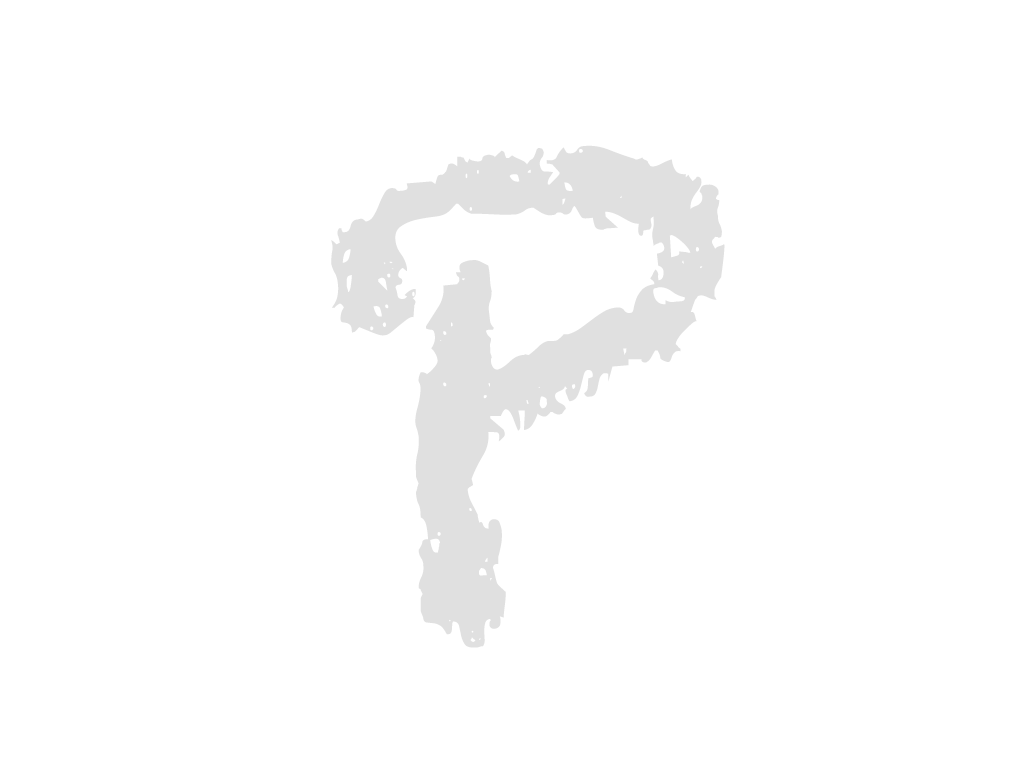
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="chart-wrap">
<div id="chartdiv" ref="chartdiv"></div>
</div>
</template>
<script>
import * as am5 from '@amcharts/amcharts5';
import * as am5xy from '@amcharts/amcharts5/xy';
import am5themes_Animated from '@amcharts/amcharts5/themes/Animated';
export default {
props: {
setData: {
type: Object,
default: {},
}, color: {
type: Array,
default: [],
},
},
data() {
return {
};
},
methods: {
chartCreate: function (data, color) {
let chartWarp = this.$refs["chartdiv"]; // 차트 상위 div ref 매칭
chartWarp.innerHTML = ""; // 차트 상위 div 내용 초기화 (기존 차트 삭제)
let div = document.createElement("div"); // 차트를 담을 빈 div 생성 (차트 하위 div)
div.style.width = "100%"; // 차트를 담을 div의 넓이
div.style.height = "100%"; // 차트를 담을 div의 높이
chartWarp.appendChild(div); // 차트 상위 div 안에 차트 하위 div를 추가
let root = am5.Root.new(div); // 차트 하위 div에 차트(root) 담기
this.charts = root; // 차트 정보 전역에 담기
root._logo.dispose();
// Set themes
// https://www.amcharts.com/docs/v5/concepts/themes/
root.setThemes([
am5themes_Animated.new(root)
]);
// Create chart
// https://www.amcharts.com/docs/v5/charts/xy-chart/
let chart = root.container.children.push(
am5xy.XYChart.new(root, {
panX: false,
panY: false,
wheelX: "none",
wheelY: "none",
paddingLeft: 0,
layout: root.verticalLayout
})
);
// Add cursor
// https://www.amcharts.com/docs/v5/charts/xy-chart/cursor/
let cursor = chart.set("cursor", am5xy.XYCursor.new(root, {}));
cursor.lineY.set("visible", false);
// Create axes
// https://www.amcharts.com/docs/v5/charts/xy-chart/axes/
let xRenderer = am5xy.AxisRendererX.new(root, {
minGridDistance: 30,
minorGridEnabled: true
});
xRenderer.labels.template.setAll({ text: "" });
let xAxis = chart.xAxes.push(
am5xy.CategoryAxis.new(root, {
maxDeviation: 0,
categoryField: "category",
renderer: xRenderer,
tooltip: am5.Tooltip.new(root, {
labelText: "{realName}"
})
})
);
// let yAxis = chart.yAxes.push(
// am5xy.ValueAxis.new(root, {
// maxDeviation: 0.3,
// renderer: am5xy.AxisRendererY.new(root, {})
// })
// );
let yAxis = chart.yAxes.push(
am5xy.ValueAxis.new(root, {
maxDeviation: 0.3,
renderer: am5xy.AxisRendererY.new(root, { opposite: false })
})
);
// Create series
// https://www.amcharts.com/docs/v5/charts/xy-chart/series/
let series = chart.series.push(
am5xy.ColumnSeries.new(root, {
name: "Series 1",
xAxis: xAxis,
yAxis: yAxis,
valueYField: "value",
sequencedInterpolation: true,
categoryXField: "category",
tooltip: am5.Tooltip.new(root, {
labelText: "{provider} {realName}: {valueY}"
})
})
);
series.columns.template.setAll({
fillOpacity: 0.9,
strokeOpacity: 0
});
series.columns.template.adapters.add("fill", function (fill, target) {
const dataIndex = series.dataItems.indexOf(target.dataItem);
// Check if the data index is valid
if (dataIndex !== -1) {
// Calculate the reverse index to apply colors from the end of the array
const reverseIndex = series.dataItems.length - 1 - dataIndex;
// Use the provided colors array
return color[reverseIndex % color.length];
} else {
// If data index is invalid, use the default chart colors
return chart.get("colors").getIndex(series.dataItems.indexOf(target.dataItem));
}
});
// series.columns.template.adapters.add("stroke", (stroke, target) => {
// return chart.get("colors").getIndex(series.columns.indexOf(target));
// });
let lineSeries = chart.series.push(
am5xy.LineSeries.new(root, {
name: "평균",
xAxis: xAxis,
yAxis: yAxis,
valueYField: "quantity",
sequencedInterpolation: true,
stroke: chart.get("colors").getIndex(13),
fill: chart.get("colors").getIndex(13),
categoryXField: "category",
tooltip: am5.Tooltip.new(root, {
labelText: "{name}{valueY}"
})
})
);
lineSeries.strokes.template.set("strokeWidth", 2);
lineSeries.bullets.push(function () {
return am5.Bullet.new(root, {
locationY: 1,
locationX: undefined,
sprite: am5.Circle.new(root, {
radius: 5,
fill: lineSeries.get("fill")
})
});
});
// when data validated, adjust location of data item based on count
lineSeries.events.on("datavalidated", function () {
am5.array.each(lineSeries.dataItems, function (dataItem) {
// if count divides by two, location is 0 (on the grid)
if (
dataItem.dataContext.count / 2 ==
Math.round(dataItem.dataContext.count / 2)
) {
dataItem.set("locationX", 0);
}
// otherwise location is 0.5 (middle)
else {
dataItem.set("locationX", 0.5);
}
});
});
let chartData = [];
// process data ant prepare it for the chart
for (var providerName in data) {
//첫번째 데이터
let providerData = data[providerName];
// add data of one provider to temp array
let tempArray = [];
let count = 0;
// add items
for (var itemName in providerData) {
if (itemName != "quantity") {
count++;
// we generate unique category for each column (providerName + "_" + itemName) and store realName
tempArray.push({
name: itemName + count,
category: providerName + "_" + itemName,
realName: itemName,
value: providerData[itemName],
provider: providerName
});
}
}
// sort temp array
tempArray.sort(function (a, b) {
if (a.value > b.value) {
return 1;
} else if (a.value < b.value) {
return -1;
} else {
return 0;
}
});
// add quantity and count to middle data item (line series uses it)
let lineSeriesDataIndex = Math.floor(count / 2);
tempArray[lineSeriesDataIndex].quantity = providerData.quantity;
tempArray[lineSeriesDataIndex].count = count;
// push to the final data
am5.array.each(tempArray, function (item) {
chartData.push(item);
});
// create range (the additional label at the bottom)
let range = xAxis.makeDataItem({});
xAxis.createAxisRange(range);
range.set("category", tempArray[0].category);
range.set("endCategory", tempArray[tempArray.length - 1].category);
let label = range.get("label");
label.setAll({
text: tempArray[0].provider,
dy: 0,
fontWeight: "bold",
tooltipText: tempArray[0].provider
});
// let tick = range.get("tick");
// tick.setAll({ visible: true, strokeOpacity: 1, length: 50, location: 0 });
let grid = range.get("grid");
grid.setAll({ strokeOpacity: 1 });
}
// add range for the last grid
let range = xAxis.makeDataItem({});
xAxis.createAxisRange(range);
range.set("category", chartData[chartData.length - 1].category);
// let tick = range.get("tick");
// tick.setAll({ visible: true, strokeOpacity: 1, length: 50, location: 1 });
let grid = range.get("grid");
grid.setAll({ strokeOpacity: 1, location: 1 });
xAxis.data.setAll(chartData);
series.data.setAll(chartData);
lineSeries.data.setAll(chartData);
console.log("::::",series.data._values)
let legend = chart.children.unshift(am5.Legend.new(root, {
x: am5.percent(100), // 범례 중심 위치 (50: 중앙)
centerX: am5.percent(80), // 범례 중심 위치 (50: 중앙)
y:am5.percent(0),
centerY: am5.percent(0),
marginBottom: 10,
nameField: "value",
}));
legend.data.setAll(chart.series.values);
// Make stuff animate on load
// https://www.amcharts.com/docs/v5/concepts/animations/
series.appear(1000);
chart.appear(1000, 100);
}
},
watch: {
'setData': function (newData) {
this.chartCreate(newData, this.color)
}
},
computed: {
},
components: {
},
mounted() {
}
}
</script>