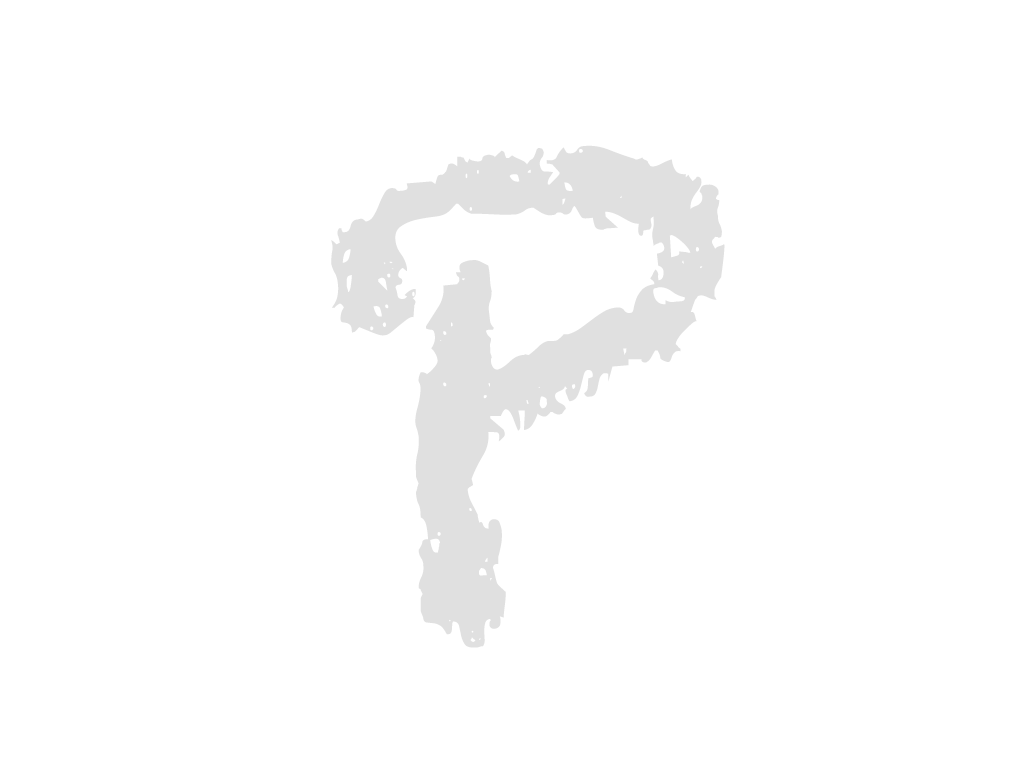
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React, { useState } from "react";
function AutoSearch(props) {
const [inputValue, setInputValue] = useState("");
const [accountResults, setAccountResults] = useState([]);
const accounts = props.accounts;
const list = document.querySelector(".auto-list");
const handleInputChange = (e) => {
const value = e.target.value;
setInputValue(value);
const filtered = accounts.filter((account) =>
account.toLowerCase().startsWith(value.toLowerCase())
);
setAccountResults(filtered);
if (value === "") {
list.style.display = "none"
list.style.padding = "0";
list.style.backgroundColor = "transparent";
list.style.border = "0";
}else{
list.style.display = "block"
list.style.padding = "10px";
list.style.backgroundColor = "#ffffff";
list.style.border = "1px solid #eee";
}
};
const handleItemClick = (account) => {
setInputValue(account);
setAccountResults([]);
};
return (
<>
<label htmlFor="" className="item-title mb5">
{props.titleName}
</label>
<input
type="text"
value={inputValue}
onChange={handleInputChange}
placeholder="거래처를 입력하세요"
className="full-input"
/>
<ul className="auto-list">
{accountResults.map((account, index) => (
<li key={index} onClick={() => handleItemClick(account)}>
{account}
</li>
))}
</ul>
</>
);
}
export default AutoSearch;