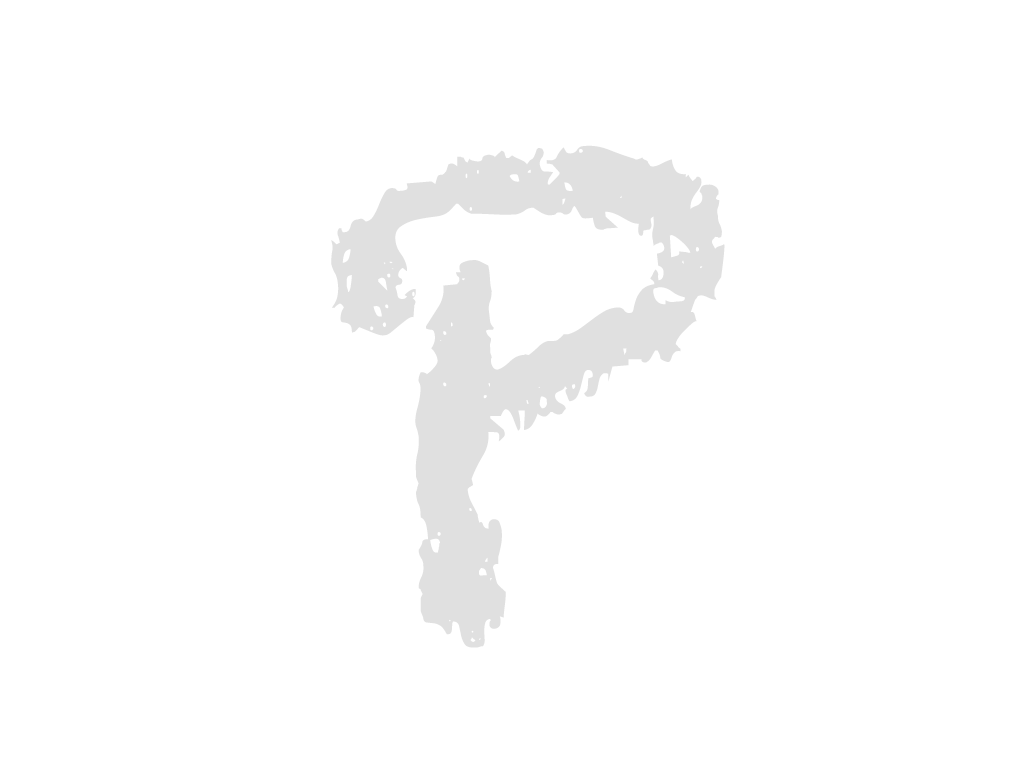
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
{"version":3,"names":["isClassDeclaration","isExportDefaultSpecifier","isExportNamespaceSpecifier","isImportDefaultSpecifier","isImportNamespaceSpecifier","isStatement","ImportSpecifier","node","importKind","word","space","print","imported","local","name","ImportDefaultSpecifier","ExportDefaultSpecifier","exported","ExportSpecifier","exportKind","ExportNamespaceSpecifier","token","ExportAllDeclaration","source","printAssertions","semicolon","ExportNamedDeclaration","format","decoratorsBeforeExport","declaration","printJoin","decorators","declar","specifiers","slice","hasSpecial","first","shift","length","printList","ExportDefaultDeclaration","ImportDeclaration","isTypeKind","hasSpecifiers","attributes","ImportAttribute","key","value","ImportNamespaceSpecifier"],"sources":["../../src/generators/modules.ts"],"sourcesContent":["import type Printer from \"../printer\";\nimport {\n isClassDeclaration,\n isExportDefaultSpecifier,\n isExportNamespaceSpecifier,\n isImportDefaultSpecifier,\n isImportNamespaceSpecifier,\n isStatement,\n} from \"@babel/types\";\nimport type * as t from \"@babel/types\";\n\nexport function ImportSpecifier(this: Printer, node: t.ImportSpecifier) {\n if (node.importKind === \"type\" || node.importKind === \"typeof\") {\n this.word(node.importKind);\n this.space();\n }\n\n this.print(node.imported, node);\n // @ts-expect-error todo(flow-ts) maybe check node type instead of relying on name to be undefined on t.StringLiteral\n if (node.local && node.local.name !== node.imported.name) {\n this.space();\n this.word(\"as\");\n this.space();\n this.print(node.local, node);\n }\n}\n\nexport function ImportDefaultSpecifier(\n this: Printer,\n node: t.ImportDefaultSpecifier,\n) {\n this.print(node.local, node);\n}\n\nexport function ExportDefaultSpecifier(\n this: Printer,\n node: t.ExportDefaultSpecifier,\n) {\n this.print(node.exported, node);\n}\n\nexport function ExportSpecifier(this: Printer, node: t.ExportSpecifier) {\n if (node.exportKind === \"type\") {\n this.word(\"type\");\n this.space();\n }\n\n this.print(node.local, node);\n // @ts-expect-error todo(flow-ts) maybe check node type instead of relying on name to be undefined on t.StringLiteral\n if (node.exported && node.local.name !== node.exported.name) {\n this.space();\n this.word(\"as\");\n this.space();\n this.print(node.exported, node);\n }\n}\n\nexport function ExportNamespaceSpecifier(\n this: Printer,\n node: t.ExportNamespaceSpecifier,\n) {\n this.token(\"*\");\n this.space();\n this.word(\"as\");\n this.space();\n this.print(node.exported, node);\n}\n\nexport function ExportAllDeclaration(\n this: Printer,\n node: t.ExportAllDeclaration | t.DeclareExportAllDeclaration,\n) {\n this.word(\"export\");\n this.space();\n if (node.exportKind === \"type\") {\n this.word(\"type\");\n this.space();\n }\n this.token(\"*\");\n this.space();\n this.word(\"from\");\n this.space();\n this.print(node.source, node);\n // @ts-expect-error Fixme: assertions is not defined in DeclareExportAllDeclaration\n this.printAssertions(node);\n this.semicolon();\n}\n\nexport function ExportNamedDeclaration(\n this: Printer,\n node: t.ExportNamedDeclaration,\n) {\n if (!process.env.BABEL_8_BREAKING) {\n if (\n this.format.decoratorsBeforeExport &&\n isClassDeclaration(node.declaration)\n ) {\n this.printJoin(node.declaration.decorators, node);\n }\n }\n\n this.word(\"export\");\n this.space();\n if (node.declaration) {\n const declar = node.declaration;\n this.print(declar, node);\n if (!isStatement(declar)) this.semicolon();\n } else {\n if (node.exportKind === \"type\") {\n this.word(\"type\");\n this.space();\n }\n\n const specifiers = node.specifiers.slice(0);\n\n // print \"special\" specifiers first\n let hasSpecial = false;\n for (;;) {\n const first = specifiers[0];\n if (\n isExportDefaultSpecifier(first) ||\n isExportNamespaceSpecifier(first)\n ) {\n hasSpecial = true;\n this.print(specifiers.shift(), node);\n if (specifiers.length) {\n this.token(\",\");\n this.space();\n }\n } else {\n break;\n }\n }\n\n if (specifiers.length || (!specifiers.length && !hasSpecial)) {\n this.token(\"{\");\n if (specifiers.length) {\n this.space();\n this.printList(specifiers, node);\n this.space();\n }\n this.token(\"}\");\n }\n\n if (node.source) {\n this.space();\n this.word(\"from\");\n this.space();\n this.print(node.source, node);\n this.printAssertions(node);\n }\n\n this.semicolon();\n }\n}\n\nexport function ExportDefaultDeclaration(\n this: Printer,\n node: t.ExportDefaultDeclaration,\n) {\n if (!process.env.BABEL_8_BREAKING) {\n if (\n this.format.decoratorsBeforeExport &&\n isClassDeclaration(node.declaration)\n ) {\n this.printJoin(node.declaration.decorators, node);\n }\n }\n\n this.word(\"export\");\n this.space();\n this.word(\"default\");\n this.space();\n const declar = node.declaration;\n this.print(declar, node);\n if (!isStatement(declar)) this.semicolon();\n}\n\nexport function ImportDeclaration(this: Printer, node: t.ImportDeclaration) {\n this.word(\"import\");\n this.space();\n\n const isTypeKind = node.importKind === \"type\" || node.importKind === \"typeof\";\n if (isTypeKind) {\n this.word(node.importKind);\n this.space();\n }\n\n const specifiers = node.specifiers.slice(0);\n const hasSpecifiers = !!specifiers.length;\n // print \"special\" specifiers first. The loop condition is constant,\n // but there is a \"break\" in the body.\n while (hasSpecifiers) {\n const first = specifiers[0];\n if (isImportDefaultSpecifier(first) || isImportNamespaceSpecifier(first)) {\n this.print(specifiers.shift(), node);\n if (specifiers.length) {\n this.token(\",\");\n this.space();\n }\n } else {\n break;\n }\n }\n\n if (specifiers.length) {\n this.token(\"{\");\n this.space();\n this.printList(specifiers, node);\n this.space();\n this.token(\"}\");\n } else if (isTypeKind && !hasSpecifiers) {\n this.token(\"{\");\n this.token(\"}\");\n }\n\n if (hasSpecifiers || isTypeKind) {\n this.space();\n this.word(\"from\");\n this.space();\n }\n\n this.print(node.source, node);\n\n this.printAssertions(node);\n if (!process.env.BABEL_8_BREAKING) {\n // @ts-ignore(Babel 7 vs Babel 8) Babel 7 supports module attributes\n if (node.attributes?.length) {\n this.space();\n this.word(\"with\");\n this.space();\n // @ts-ignore(Babel 7 vs Babel 8) Babel 7 supports module attributes\n this.printList(node.attributes, node);\n }\n }\n\n this.semicolon();\n}\n\nexport function ImportAttribute(this: Printer, node: t.ImportAttribute) {\n this.print(node.key);\n this.token(\":\");\n this.space();\n this.print(node.value);\n}\n\nexport function ImportNamespaceSpecifier(\n this: Printer,\n node: t.ImportNamespaceSpecifier,\n) {\n this.token(\"*\");\n this.space();\n this.word(\"as\");\n this.space();\n this.print(node.local, node);\n}\n"],"mappings":";;;;;;;;;;;;;;;;;AACA;;;EACEA,kB;EACAC,wB;EACAC,0B;EACAC,wB;EACAC,0B;EACAC;;;AAIK,SAASC,eAAT,CAAwCC,IAAxC,EAAiE;EACtE,IAAIA,IAAI,CAACC,UAAL,KAAoB,MAApB,IAA8BD,IAAI,CAACC,UAAL,KAAoB,QAAtD,EAAgE;IAC9D,KAAKC,IAAL,CAAUF,IAAI,CAACC,UAAf;IACA,KAAKE,KAAL;EACD;;EAED,KAAKC,KAAL,CAAWJ,IAAI,CAACK,QAAhB,EAA0BL,IAA1B;;EAEA,IAAIA,IAAI,CAACM,KAAL,IAAcN,IAAI,CAACM,KAAL,CAAWC,IAAX,KAAoBP,IAAI,CAACK,QAAL,CAAcE,IAApD,EAA0D;IACxD,KAAKJ,KAAL;IACA,KAAKD,IAAL,CAAU,IAAV;IACA,KAAKC,KAAL;IACA,KAAKC,KAAL,CAAWJ,IAAI,CAACM,KAAhB,EAAuBN,IAAvB;EACD;AACF;;AAEM,SAASQ,sBAAT,CAELR,IAFK,EAGL;EACA,KAAKI,KAAL,CAAWJ,IAAI,CAACM,KAAhB,EAAuBN,IAAvB;AACD;;AAEM,SAASS,sBAAT,CAELT,IAFK,EAGL;EACA,KAAKI,KAAL,CAAWJ,IAAI,CAACU,QAAhB,EAA0BV,IAA1B;AACD;;AAEM,SAASW,eAAT,CAAwCX,IAAxC,EAAiE;EACtE,IAAIA,IAAI,CAACY,UAAL,KAAoB,MAAxB,EAAgC;IAC9B,KAAKV,IAAL,CAAU,MAAV;IACA,KAAKC,KAAL;EACD;;EAED,KAAKC,KAAL,CAAWJ,IAAI,CAACM,KAAhB,EAAuBN,IAAvB;;EAEA,IAAIA,IAAI,CAACU,QAAL,IAAiBV,IAAI,CAACM,KAAL,CAAWC,IAAX,KAAoBP,IAAI,CAACU,QAAL,CAAcH,IAAvD,EAA6D;IAC3D,KAAKJ,KAAL;IACA,KAAKD,IAAL,CAAU,IAAV;IACA,KAAKC,KAAL;IACA,KAAKC,KAAL,CAAWJ,IAAI,CAACU,QAAhB,EAA0BV,IAA1B;EACD;AACF;;AAEM,SAASa,wBAAT,CAELb,IAFK,EAGL;EACA,KAAKc,SAAL;EACA,KAAKX,KAAL;EACA,KAAKD,IAAL,CAAU,IAAV;EACA,KAAKC,KAAL;EACA,KAAKC,KAAL,CAAWJ,IAAI,CAACU,QAAhB,EAA0BV,IAA1B;AACD;;AAEM,SAASe,oBAAT,CAELf,IAFK,EAGL;EACA,KAAKE,IAAL,CAAU,QAAV;EACA,KAAKC,KAAL;;EACA,IAAIH,IAAI,CAACY,UAAL,KAAoB,MAAxB,EAAgC;IAC9B,KAAKV,IAAL,CAAU,MAAV;IACA,KAAKC,KAAL;EACD;;EACD,KAAKW,SAAL;EACA,KAAKX,KAAL;EACA,KAAKD,IAAL,CAAU,MAAV;EACA,KAAKC,KAAL;EACA,KAAKC,KAAL,CAAWJ,IAAI,CAACgB,MAAhB,EAAwBhB,IAAxB;EAEA,KAAKiB,eAAL,CAAqBjB,IAArB;EACA,KAAKkB,SAAL;AACD;;AAEM,SAASC,sBAAT,CAELnB,IAFK,EAGL;EACmC;IACjC,IACE,KAAKoB,MAAL,CAAYC,sBAAZ,IACA5B,kBAAkB,CAACO,IAAI,CAACsB,WAAN,CAFpB,EAGE;MACA,KAAKC,SAAL,CAAevB,IAAI,CAACsB,WAAL,CAAiBE,UAAhC,EAA4CxB,IAA5C;IACD;EACF;EAED,KAAKE,IAAL,CAAU,QAAV;EACA,KAAKC,KAAL;;EACA,IAAIH,IAAI,CAACsB,WAAT,EAAsB;IACpB,MAAMG,MAAM,GAAGzB,IAAI,CAACsB,WAApB;IACA,KAAKlB,KAAL,CAAWqB,MAAX,EAAmBzB,IAAnB;IACA,IAAI,CAACF,WAAW,CAAC2B,MAAD,CAAhB,EAA0B,KAAKP,SAAL;EAC3B,CAJD,MAIO;IACL,IAAIlB,IAAI,CAACY,UAAL,KAAoB,MAAxB,EAAgC;MAC9B,KAAKV,IAAL,CAAU,MAAV;MACA,KAAKC,KAAL;IACD;;IAED,MAAMuB,UAAU,GAAG1B,IAAI,CAAC0B,UAAL,CAAgBC,KAAhB,CAAsB,CAAtB,CAAnB;IAGA,IAAIC,UAAU,GAAG,KAAjB;;IACA,SAAS;MACP,MAAMC,KAAK,GAAGH,UAAU,CAAC,CAAD,CAAxB;;MACA,IACEhC,wBAAwB,CAACmC,KAAD,CAAxB,IACAlC,0BAA0B,CAACkC,KAAD,CAF5B,EAGE;QACAD,UAAU,GAAG,IAAb;QACA,KAAKxB,KAAL,CAAWsB,UAAU,CAACI,KAAX,EAAX,EAA+B9B,IAA/B;;QACA,IAAI0B,UAAU,CAACK,MAAf,EAAuB;UACrB,KAAKjB,SAAL;UACA,KAAKX,KAAL;QACD;MACF,CAVD,MAUO;QACL;MACD;IACF;;IAED,IAAIuB,UAAU,CAACK,MAAX,IAAsB,CAACL,UAAU,CAACK,MAAZ,IAAsB,CAACH,UAAjD,EAA8D;MAC5D,KAAKd,SAAL;;MACA,IAAIY,UAAU,CAACK,MAAf,EAAuB;QACrB,KAAK5B,KAAL;QACA,KAAK6B,SAAL,CAAeN,UAAf,EAA2B1B,IAA3B;QACA,KAAKG,KAAL;MACD;;MACD,KAAKW,SAAL;IACD;;IAED,IAAId,IAAI,CAACgB,MAAT,EAAiB;MACf,KAAKb,KAAL;MACA,KAAKD,IAAL,CAAU,MAAV;MACA,KAAKC,KAAL;MACA,KAAKC,KAAL,CAAWJ,IAAI,CAACgB,MAAhB,EAAwBhB,IAAxB;MACA,KAAKiB,eAAL,CAAqBjB,IAArB;IACD;;IAED,KAAKkB,SAAL;EACD;AACF;;AAEM,SAASe,wBAAT,CAELjC,IAFK,EAGL;EACmC;IACjC,IACE,KAAKoB,MAAL,CAAYC,sBAAZ,IACA5B,kBAAkB,CAACO,IAAI,CAACsB,WAAN,CAFpB,EAGE;MACA,KAAKC,SAAL,CAAevB,IAAI,CAACsB,WAAL,CAAiBE,UAAhC,EAA4CxB,IAA5C;IACD;EACF;EAED,KAAKE,IAAL,CAAU,QAAV;EACA,KAAKC,KAAL;EACA,KAAKD,IAAL,CAAU,SAAV;EACA,KAAKC,KAAL;EACA,MAAMsB,MAAM,GAAGzB,IAAI,CAACsB,WAApB;EACA,KAAKlB,KAAL,CAAWqB,MAAX,EAAmBzB,IAAnB;EACA,IAAI,CAACF,WAAW,CAAC2B,MAAD,CAAhB,EAA0B,KAAKP,SAAL;AAC3B;;AAEM,SAASgB,iBAAT,CAA0ClC,IAA1C,EAAqE;EAC1E,KAAKE,IAAL,CAAU,QAAV;EACA,KAAKC,KAAL;EAEA,MAAMgC,UAAU,GAAGnC,IAAI,CAACC,UAAL,KAAoB,MAApB,IAA8BD,IAAI,CAACC,UAAL,KAAoB,QAArE;;EACA,IAAIkC,UAAJ,EAAgB;IACd,KAAKjC,IAAL,CAAUF,IAAI,CAACC,UAAf;IACA,KAAKE,KAAL;EACD;;EAED,MAAMuB,UAAU,GAAG1B,IAAI,CAAC0B,UAAL,CAAgBC,KAAhB,CAAsB,CAAtB,CAAnB;EACA,MAAMS,aAAa,GAAG,CAAC,CAACV,UAAU,CAACK,MAAnC;;EAGA,OAAOK,aAAP,EAAsB;IACpB,MAAMP,KAAK,GAAGH,UAAU,CAAC,CAAD,CAAxB;;IACA,IAAI9B,wBAAwB,CAACiC,KAAD,CAAxB,IAAmChC,0BAA0B,CAACgC,KAAD,CAAjE,EAA0E;MACxE,KAAKzB,KAAL,CAAWsB,UAAU,CAACI,KAAX,EAAX,EAA+B9B,IAA/B;;MACA,IAAI0B,UAAU,CAACK,MAAf,EAAuB;QACrB,KAAKjB,SAAL;QACA,KAAKX,KAAL;MACD;IACF,CAND,MAMO;MACL;IACD;EACF;;EAED,IAAIuB,UAAU,CAACK,MAAf,EAAuB;IACrB,KAAKjB,SAAL;IACA,KAAKX,KAAL;IACA,KAAK6B,SAAL,CAAeN,UAAf,EAA2B1B,IAA3B;IACA,KAAKG,KAAL;IACA,KAAKW,SAAL;EACD,CAND,MAMO,IAAIqB,UAAU,IAAI,CAACC,aAAnB,EAAkC;IACvC,KAAKtB,SAAL;IACA,KAAKA,SAAL;EACD;;EAED,IAAIsB,aAAa,IAAID,UAArB,EAAiC;IAC/B,KAAKhC,KAAL;IACA,KAAKD,IAAL,CAAU,MAAV;IACA,KAAKC,KAAL;EACD;;EAED,KAAKC,KAAL,CAAWJ,IAAI,CAACgB,MAAhB,EAAwBhB,IAAxB;EAEA,KAAKiB,eAAL,CAAqBjB,IAArB;EACmC;IAAA;;IAEjC,wBAAIA,IAAI,CAACqC,UAAT,aAAI,iBAAiBN,MAArB,EAA6B;MAC3B,KAAK5B,KAAL;MACA,KAAKD,IAAL,CAAU,MAAV;MACA,KAAKC,KAAL;MAEA,KAAK6B,SAAL,CAAehC,IAAI,CAACqC,UAApB,EAAgCrC,IAAhC;IACD;EACF;EAED,KAAKkB,SAAL;AACD;;AAEM,SAASoB,eAAT,CAAwCtC,IAAxC,EAAiE;EACtE,KAAKI,KAAL,CAAWJ,IAAI,CAACuC,GAAhB;EACA,KAAKzB,SAAL;EACA,KAAKX,KAAL;EACA,KAAKC,KAAL,CAAWJ,IAAI,CAACwC,KAAhB;AACD;;AAEM,SAASC,wBAAT,CAELzC,IAFK,EAGL;EACA,KAAKc,SAAL;EACA,KAAKX,KAAL;EACA,KAAKD,IAAL,CAAU,IAAV;EACA,KAAKC,KAAL;EACA,KAAKC,KAAL,CAAWJ,IAAI,CAACM,KAAhB,EAAuBN,IAAvB;AACD"}