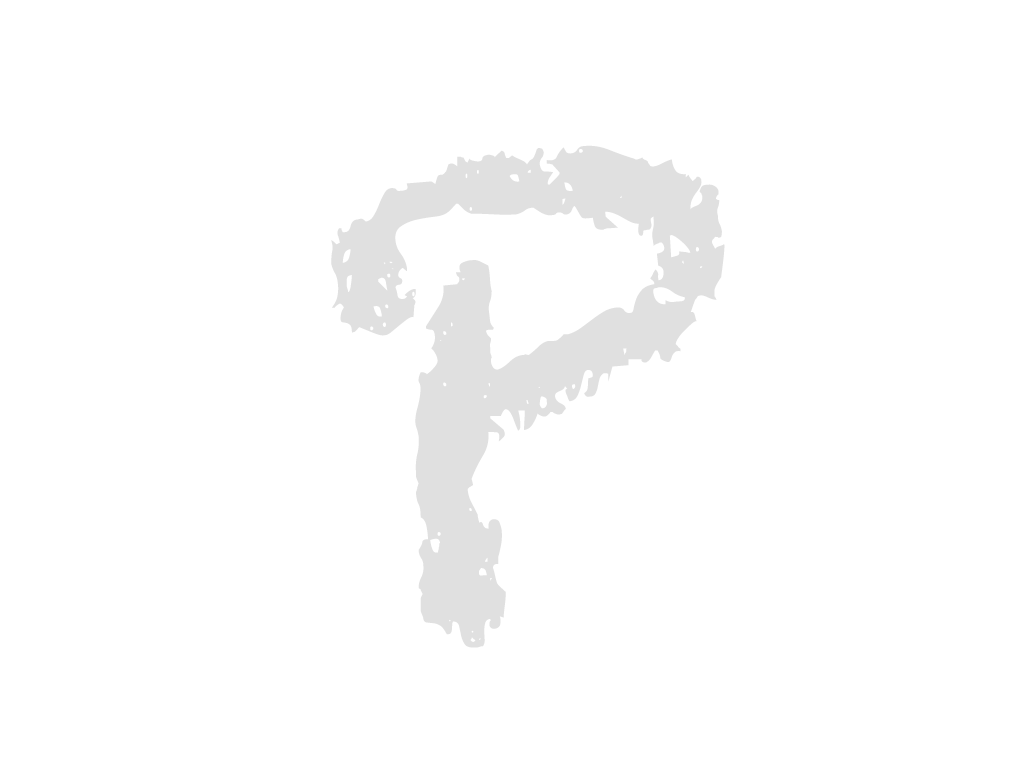
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<nav role="navigation" id="mainNav" aria-label="Main Menu">
<ul class="main-menu flex justify-start align-center" role="menu" >
<li v-for="(mainMenu, index) in menuList" :key="index" role="menuitem" :class="{
'pd10 cursor relative': true,
active: checkActiveMainMenu[index]
}" @mouseenter="showAllSubMenus(index)" @mouseleave="hideAllSubMenus" @click="clearQuery(mainMenu.childList[0])">
<router-link :to="mainMenu.routerUrl ? mainMenu.routerUrl : mainMenu.childList[0].routerUrl" class="text-ct"
@focusin="showAllSubMenus(index)" @focusout="scheduleHideAllSubMenus">{{
mainMenu.menuNm }}</router-link>
<ul v-if="mainMenu.childList.length > 0" class="sub-menu" :class="{
show: showAllMenus
}">
<!-- 이벤트 버블링 현상을 막기 위해 click.stop으로 사용 -->
<li v-for="(subMenu, subIndex) in mainMenu.childList" :key="subIndex" @click.stop="clearQuery(subMenu)">
<router-link :to="subMenu.routerUrl" active-class="sub-active" @focusin="showAllSubMenus(index)"
@focusout="scheduleHideAllSubMenus">{{ subMenu.menuNm }}</router-link>
</li>
</ul>
</li>
</ul>
<div class="nav-bg" :style="showAllMenus ? `height: ${maxSubMenuHeight}px` : `height: 0px`"></div>
</nav>
</template>
<script>
import store from "../../views/pages/AppStore";
import { findBySysMenu } from "../../resources/api/menu";
import queryParams from "../../resources/js/queryParams";
import { defaultSearchParams } from "../../resources/js/defaultSearchParams";
import cntnStatsSave from "../../resources/js/cntnStatsSave";
import { mdiMenu } from '@mdi/js';
export default {
mixins: [queryParams, cntnStatsSave],
props: {},
data() {
return {
showAllMenus: false,
hideTimeout: null,
currentActiveIndex: null,
roles: store.state.roles || [],
menuList: [],
currentOpenIndex: null,
currentActiveIndex: null,
currentSubActiveIndex: null,
currentSubSubActiveIndex: null,
menuPath: mdiMenu,
resetSearch: { ...defaultSearchParams },
};
},
created() {
this.findAll();
},
methods: {
// 메뉴 처리 이벤트
showAllSubMenus() {
this.$emit('hover', true);
if (this.hideTimeout) {
clearTimeout(this.hideTimeout);
this.hideTimeout = null;
}
this.showAllMenus = true;
},
hideAllSubMenus() {
this.$emit('hover', false);
this.showAllMenus = false;
},
scheduleHideAllSubMenus() {
this.hideTimeout = setTimeout(() => {
this.hideAllSubMenus();
}, 100);
},
// 목록 조회
async findAll() {
const vm = this;
if (this.roles.length > 0) {
this.roles = this.roles.map((auth) => auth.authority);
}
try {
const params = {
roles: this.roles,
menuType: store.state.userType,
};
const res = await findBySysMenu(params);
if (res.status == 200) {
this.menuList = res.data.data.menuList;
this.$store.commit('setMenuList', this.menuList);
}
} catch (error) {
// alert("에러가 발생했습니다.\n관리자에게 문의하세요.!!!");
alert(vm.$getCmmnMessage('err005'));
}
},
async selectMenu(index, menu) {
// this.saveQueryParams("queryParams", this.resetSearch); // 검색조건 초기화
// this.currentActiveIndex = index;
this.currentSubActiveIndex = null;
this.currentSubSubActiveIndex = null;
//await this.cntnStatsSave(menu.menuId);
this.currentOpenIndex = null;
if (menu.linkTypeNm === "0") {
// 현재창
// this.$router.push({
// path: menu.routerUrl,
// });
} else if (menu.linkTypeNm === "1") {
// 새창
window.open(menu.routerUrl, "_blank");
}
},
async selectSubMenu(mainIndex, subIndex, subMenu, mainMenu) {
this.saveQueryParams("queryParams", this.resetSearch); // 검색조건 초기화
this.currentActiveIndex = mainIndex;
this.currentSubActiveIndex = subIndex;
this.currentSubSubActiveIndex = null;
await this.cntnStatsSave(subMenu.menuId);
// 메뉴 정보 스토리지 생성
this.$store.commit('`setMenu`', subMenu);
// this.$store.commit('setMenuInfo', { 'menuNm': subMenu.menuNm, 'menuCn': subMenu.menuCn });
// this.$store.commit('setPageNaviInfo', { 'main': mainMenu.menuNm, 'sub': subMenu.menuNm });
this.$store.commit('setCurrentMenu', subMenu.menuId);
this.currentOpenIndex = null;
if (subMenu.linkTypeNm === "0") {
// 현재창
this.$router.push({
path: subMenu.routerUrl,
});
} else if (subMenu.linkTypeNm === "1") {
// 새창
window.open(subMenu.routerUrl, "_blank");
}
},
async clearQuery(menu) {
this.saveQueryParams("queryParams", this.resetSearch); // 검색조건 초기화
await this.cntnStatsSave(menu.menuId);
this.$store.commit('`setMenu`', menu);
this.$store.commit('setCurrentMenu', menu.menuId);
},
async selectSubSubMenu(mainIndex, subIndex, subSubIndex, menu) {
this.saveQueryParams("queryParams", this.resetSearch); // 검색조건 초기화
this.currentActiveIndex = mainIndex;
this.currentSubActiveIndex = subIndex;
this.currentSubSubActiveIndex = subSubIndex;
await this.cntnStatsSave(menu.menuId);
if (menu.linkTypeNm === "0") {
// 현재창
this.$router.push({
path: menu.routerUrl,
});
} else if (menu.linkTypeNm === "1") {
// 새창
window.open(menu.routerUrl, "_blank");
}
},
},
watch: {
currentActiveIndex: function (newV, oldV) {
},
},
computed: {
maxSubMenuHeight: function () {
let maxSubItems = Math.max(...this.menuList.map(menu => menu.childList.length));
return (maxSubItems * 55) + 90;
},
//하위메뉴 활성화 되어있는지 확인
checkActiveMainMenu() {
return this.menuList.map(mainMenu => {
return mainMenu.childList.some(subMenu => this.$route.path.includes(subMenu.routerUrl));
});
}
},
mounted() {
},
};
</script>