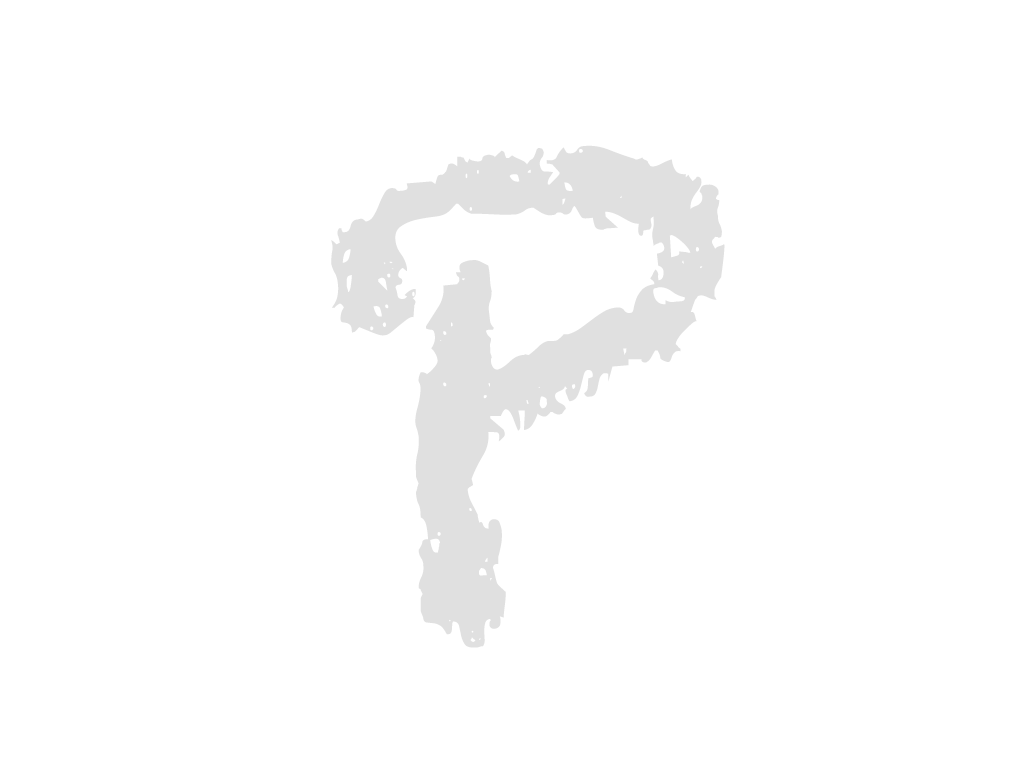
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div @dragover.prevent @dragenter.prevent="highlight" @dragleave.prevent="unhighlight" @drop.prevent="handleDrop"
:class="{ 'drag-drop pd30 mb20': true, 'dragging': isDragging }">
<div class="sample mb20" v-show="type === 'registration'">
<div class="flex justify-center align-center">
<div class="sample-proof pl5 pr5">
<p class="text-ct mb5">사업자등록증명원</p>
<img src="../../../resources/img/proof_sample.jpg" alt="사업자등록증명원 샘플이미지">
</div>
<div class="sample-registration pl5 pr5">
<p class="text-ct mb5">사업자등록증</p>
<img src="../../../resources/img/registration_sample.jpg" alt="사업자등록증 샘플이미지">
</div>
</div>
</div>
<p class="detail-text text-ct mb15">{{ text }}</p>
<div class="flex justify-center">
<label :for="type ? type : `file-upload-${id}`" class="small-btn upload-btn text-ct block relative" aria-controls="file-upload" aria-label="파일 업로드 버튼" :aria-required="type === 'registration' || type === 'applyForm' || type === 'certification'">
<img src="../../../resources/img/upload.png" alt="업로드 아이콘" class="inline-block mr10"
style="vertical-align:bottom" />
파일선택
</label>
<input type="file" :id="type ? type : `file-upload-${id}`" class="absolute opacity-0" @change="handleFileInput" aria-hidden="true" />
</div>
</div>
<div v-if="files.filelist.length > 0">
<ul>
<li v-for="(file, index) in files.filelist" :key="index"
class="file pd5 flex justify-between align-center mb5">
<div class="ellipsis gd-10">
<span class="filename">{{ file.name }}</span>
<span class="extension">[{{ formatFileSize(file.size) }}]</span>
</div>
<div class="gd-2 flex justify-end align-center">
<button type="button" class="icon-btn black" v-if="updateFile !== null"></button>
<button type="button" class="icon-btn black" aria-label="removeFile" title="이미지 취소" @click="removeFiles()" id="removeFile" v-else><svg-icon type="mdi" :path="closePath" role="img" aria-labelledby="removeFile"></svg-icon></button>
</div>
</li>
</ul>
</div>
<div v-if="newFiles.length > 0">
<ul>
<li v-for="(file, index) in newFiles" :key="index" class="file pd5 flex justify-between align-center mb5">
<div class="ellipsis gd-10">
<span class="filename">{{ file.name }}</span>
<span class="extension">[{{ formatFileSize(file.size) }}]</span>
</div>
<div class="gd-2 flex justify-end align-center">
<button type="button" class="icon-btn black" aria-label="removeFile" title="이미지 취소" @click="removeFile(file, index)" id="removeFile"><svg-icon type="mdi" :path="closePath" role="img" aria-labelledby="removeFile"></svg-icon></button>
</div>
</li>
</ul>
</div>
</template>
<script>
import { mdiWindowClose } from '@mdi/js';
import { takeWhile } from 'lodash';
export default {
props: {
text: {
type: String,
default: ''
},
type: {
type: String,
default: ''
},
updateFile: {
type: Object,
default: null
},
discussionFiles: {
type: Array,
default: null
},
options: {
type: Object,
default: () => ({
allowedExtensions: ['pdf', 'doc', 'docx', 'xls', 'xlsx', 'csv', 'txt', 'zip', 'jpg', 'jpeg', 'png', 'hwp', 'pptx', 'pptm', 'ppt']
})
},
id: {
type: String
}
},
data() {
return {
isDragging: false,
files: {
type: "",
filelist: []
},
newFiles: [],
closePath:mdiWindowClose,
};
},
methods: {
highlight() {
this.isDragging = true;
},
unhighlight() {
this.isDragging = false;
},
handleDrop(event) {
event.preventDefault();
this.isDragging = false;
const allowedExtensions = this.options.allowedExtensions;
const files = event.dataTransfer.files;
for (let i = 0; i < files.length; i++) {
const file = files[i];
const fileExtension = this.getExtension(file.name).toLowerCase();
if (allowedExtensions.includes(fileExtension)) {
// 허용되는 확장자인 경우 처리
if (this.type !== '') {
this.handleFiles(files, this.type);
} else {
this.handleFileList(files);
}
} else {
// 허용되지 않는 확장자인 경우 경고 메시지 표시 등의 처리
alert(`${file.name}은 허용되지 않는 파일 형식입니다.`);
}
}
},
// handleFileInput(event) {
// if (this.type !== '') {
// this.handleFiles(event.target.files, this.type);
// } else {
// this.handleFileList(event.target.files);
// }
// },
handleFileInput(event) {
const allowedExtensions = this.options.allowedExtensions;
const files = event.target.files;
for (let i = 0; i < files.length; i++) {
const file = files[i];
const fileExtension = this.getExtension(file.name).toLowerCase();
if (allowedExtensions.includes(fileExtension)) {
// 허용되는 확장자인 경우 처리
if (this.type !== '') {
this.handleFiles(files, this.type);
} else {
this.handleFileList(files);
}
} else {
// 허용되지 않는 확장자인 경우 경고 메시지 표시 등의 처리
alert(`${file.name}은 허용되지 않는 파일 형식입니다.`);
}
}
},
handleFileList(files) {
let result = true;
for (let i = 0; i < this.newFiles.length; i++) {
if (this.newFiles[i].name === files[0].name) {
result = false;
break;
}
}
if (result) {
this.newFiles.push(files[0]);
} else {
alert("동일한 이름을 가진 파일은 첨부할 수 없습니다.\n파일명 변경 후 다시 첨부 해주시기 바랍니다.")
}
},
handleFiles(files, type) {
this.files = {
type: type,
filelist: files
};
},
getName(filename) {
const lastIndex = filename.lastIndexOf('.');
return filename.substring(0, lastIndex);
},
getExtension(filename) {
const lastIndex = filename.lastIndexOf('.');
return filename.substring(lastIndex + 1);
},
formatFileSize(size) {
const units = ['B', 'KB', 'MB', 'GB', 'TB'];
let i;
// 파일 크기를 적절한 단위로 변환
for (i = 0; size >= 1024 && i < units.length - 1; i++) {
size /= 1024;
}
// 파일 크기와 단위를 문자열로 반환
if (i === units.length - 1) {
return `${size.toFixed(2)} ${units[i]}`;
} else {
return `${size.toFixed(2)} ${units[i]}`;
}
},
removeFile(file, index) {
this.newFiles.splice(index, 1);
this.$emit('fileDelet' , file)
},
//세현
removeFiles() {
this.$emit('file-deleted' , this.files.type)
this.files = {
type: "",
filelist: []
}
},
},
watch: {
'updateFile' : function(newV){
if (this.updateFile !== null) {
this.files.type = this.type;
this.files.filelist[0] =newV;
}
},
'files': function (newV, oldV) {
this.$emit('emitFiles', newV);
},
'newFiles.length': function (newV) {
this.$emit("emitFileList", this.newFiles)
},
'discussionFiles': function (newV, oldV) {
const fileList = newV;
const initFiles = [];
if(fileList.length > 0) {
fileList.forEach(file => {
const files = { 'fileId': null, 'name': null, 'size': null };
files.fileId = file.fileId
files.name = file.fileNm;
files.size = file.fileSz;
// this.newFiles.push(files)
initFiles.push(files)
})
}
this.newFiles = initFiles;
},
},
computed: {
},
components: {
},
mounted() {
if (this.updateFile !== null) {
this.files.type = this.type;
this.files.filelist[0] = this.updateFile
}
if (this.discussionFiles !== null) {
// console.log('discussionFiles', this.discussionFiles)
const fileList = this.discussionFiles;
const initFiles = [];
if(fileList.length > 0) {
fileList.forEach(file => {
const files = { 'fileId': null, 'name': null, 'size': null };
files.fileId = file.fileId
files.name = file.fileNm;
files.size = file.fileSz;
// this.newFiles.push(files)
initFiles.push(files)
})
}
this.newFiles = initFiles;
}
}
};
</script>