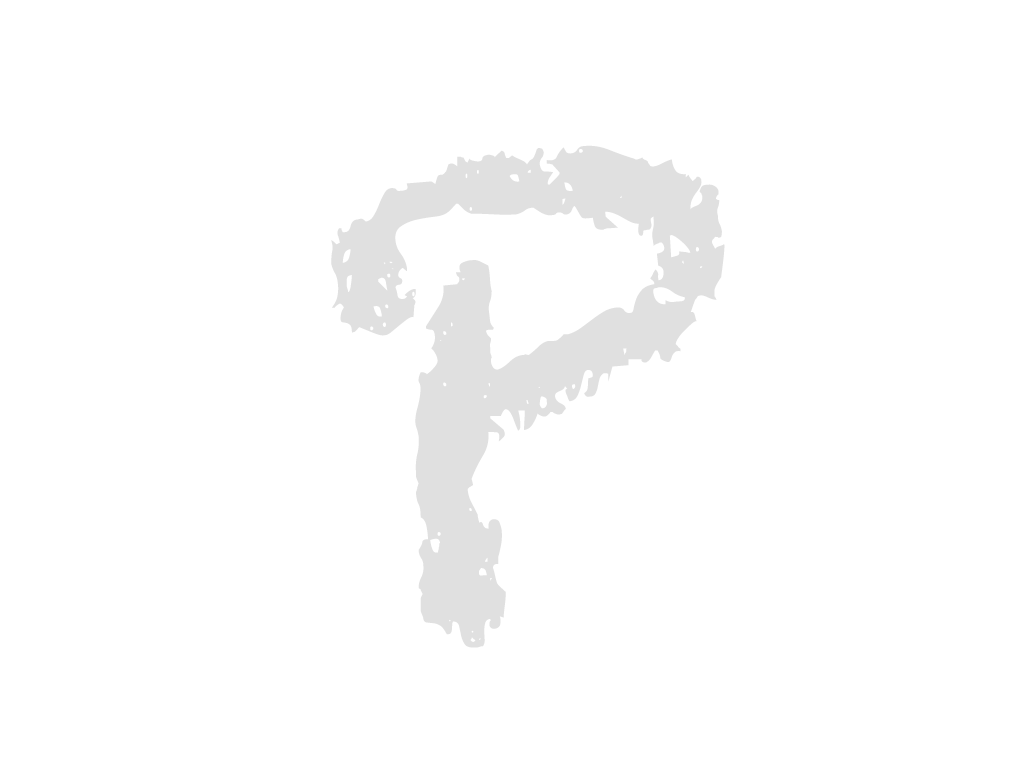
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="side">
<div class="profile mb30">
<div class="flex align-center" style="gap: 18px">
<img src="../../resources/img/img16_s.png" alt="" style="border-radius: 100%; width: 10rem" />
<div class="mt25">
<div>
<p class="name mb10">{{ studentInfo.studentName }}</p>
<p class="mb5">
<span><img src="../../resources/img/new_img/icon/school_icon.png" alt="" /></span>
{{ studentInfo.institutionName }} {{ studentInfo.grade }}학년
{{ studentInfo.className }}
</p>
</div>
<!-- <progress-bar :progress="progress"></progress-bar> -->
</div>
</div>
<div class="problem-box mt25">
<p style="font-weight: bold">
지금까지 푼 총 문제 수 :
<span style="color: #9528b7; font-size: 16px; font-weight: bold"
>{{ studentInfo.totalProblemsSolved }}개</span
>
</p>
</div>
</div>
<hr style="margin: 25px 20px" />
<div class="history-box">
<p class="title2 mb20">최근 학습 히스토리</p>
<ul class="flex-column" style="gap: 10px">
<!-- 더미 데이터
<li>· 에듀캐롯 3학년 1학기</li>
<li>· 에듀캐롯 3학년 2학기</li>
-->
<li v-for="historyItem in studentInfo.history" :key="historyItem.unitId">
· [{{ historyItem.bookName }}] {{ historyItem.unitName }}
</li>
</ul>
</div>
<!-- <div class="ask mb30">
<p class="title1 mb15">선생님께 질문 있어요~</p>
<div class="memo mb15">
<textarea
name=""
id=""
placeholder="궁금한 것을 적어보세요."
v-model="studentInfo.studentQuestion"
></textarea>
</div>
<div class="flex justify-end">
<button @click="updateQuestion">질문하기</button>
</div>
</div> -->
<div class="mt25">
<div class="button-box flex">
<button @click="handleClickEvent" style="width: 100%">
<img
class="look-btn"
src="../../resources/img/new_img/plan/wrong_note_btn.png"
alt=""
style="width: 100%"
/>
</button>
</div>
<div class="button-box flex">
<button @click="goPopupOpen" style="width: 100%">
<img src="../../resources/img/new_img/plan/question_btn.png" alt="" style="width: 100%" />
</button>
</div>
</div>
</div>
<!-- 질문하기 팝업 -->
<div class="popup-wrap" v-if="this.popupOpen === true" style="z-index: 100">
<div class="question-popup">
<div class="flex justify-between align-center">
<p class="question-text mt20">선생님께 질문하기</p>
<img class="look-btn" @click="goPopupClose" src="../../resources/img/btn25_93t_normal.png" alt="" />
</div>
<p style="color: #8c8c8c; font-size: 20px; margin: 40px 0px">선생님! 질문있어요!!</p>
<div class="memo mt25">
<p class="title1 memo-text">내용</p>
<textarea name="" id="" placeholder="궁금한 것을 적어보세요." v-model="question"></textarea>
</div>
<div class="questionBtn-box flex justify-end" style="gap: 15px">
<button style="border-color: #eaedf4; color: #8c8c8c" @click="goPopupClose">취소하기</button>
<button @click="updateQuestion" style="border-color: #6327b9; color: white; background-color: #6327b9">
질문하기
</button>
</div>
</div>
</div>
</template>
<script>
import ProgressBar from '../component/ProgressBar.vue';
import axios from 'axios';
export default {
data() {
return {
progress: 20,
studentInfo: {
studentName: '',
institutionName: '',
grade: '',
className: '',
studentQuestion: '',
history: [],
},
userId: '1', // userId 임시 설정
popupOpen: false,
question: null,
};
},
methods: {
pageSetting() {
this.userId = this.$store.getters.getUserInfo.userId;
console.log('유저 아이디: ', this.userId);
this.fetchStudentInfo();
},
// 학생 정보를 가져오는 메서드
fetchStudentInfo() {
axios
.post('/studentInfo/getInfo.json', { userId: this.userId })
.then((response) => {
console.log('학생 정보 조회 : ', response.data);
this.studentInfo = response.data;
})
.catch((error) => {
console.error('학생 정보 가져오기 실패:', error);
});
},
// 질문 업데이트 메서드
updateQuestion() {
if (this.question === null) {
alert('질문을 입력해주세요');
return;
}
axios
.post('/studentInfo/updateQuestion.json', {
userId: this.userId,
// userId: 'USID_000000000000002',
studentQuestion: this.question,
})
.then((response) => {
console.log('질문이 성공적으로 업데이트되었습니다.');
alert('질문이 성공적으로 업데이트되었습니다.');
})
.catch((error) => {
console.error('질문 업데이트 실패:', error);
alert('질문 업데이트에 실패했습니다.');
});
this.question = null;
},
handleClick() {
this.toggleText();
this.goToPage('PreviewNote');
},
handleClick() {
this.toggleTextAndNavigate();
},
toggleTextAndNavigate() {
if (this.buttonText === '오답노트') {
this.buttonText = '학습 코스';
this.goToPage('PreviewNote');
// 버튼 텍스트가 '학습 코스'일 때 이동할 경로
} else {
this.buttonText = '오답노트';
this.goToPage('Dashboard');
// 버튼 텍스트가 '오답노트'일 때 이동할 경로
}
},
handleClickEvent() {
this.goToPage('PreviewNote');
},
goToPage(page) {
this.$router.push({ name: page });
},
goPopupOpen() {
this.popupOpen = true;
},
goPopupClose() {
this.popupOpen = false;
this.question = null;
},
},
watch: {},
computed: {},
components: {
ProgressBar,
},
mounted() {
console.log('Side mounted');
this.pageSetting();
},
};
</script>
<style scoped>
.login-btn img {
width: 430px;
height: 85px;
}
.problem-box {
display: flex;
align-items: center;
justify-content: center;
border: 2px solid #6327b9;
background-color: #f0eaf8;
border-radius: 8px;
padding: 10px 10px;
}
.history-box {
background-color: white;
padding: 30px 20px;
border: 1px solid #c6c6c6;
border-radius: 10px;
margin: 25px 20px 0px;
}
.history-box li {
background-color: #eaedf4;
border-radius: 10px;
font-size: 18px;
list-style-type: none;
padding: 10px;
}
.button-box {
margin: 10px 20px;
}
.question-popup {
background-color: white;
border-radius: 10px;
padding: 30px 40px;
position: absolute;
top: 15%;
left: 25%;
width: 100rem;
}
.question-text {
font-family: 'ONEMobilePOPOTF';
font-size: 32px;
color: #6327b9;
}
.memo-text {
font-family: 'ONEMobilePOPOTF';
color: #6327b9;
}
.memo textarea {
border: 2px solid #cac0e3;
border-radius: 10px;
width: 100%;
padding: 20px;
height: 30rem;
margin: 20px 0px 40px;
}
.questionBtn-box button {
font-size: 18px;
padding: 8px 30px;
border: 2px solid;
border-radius: 8px;
font-weight: bold;
font-family: 'ONEMobileOTF-Regular';
}
</style>