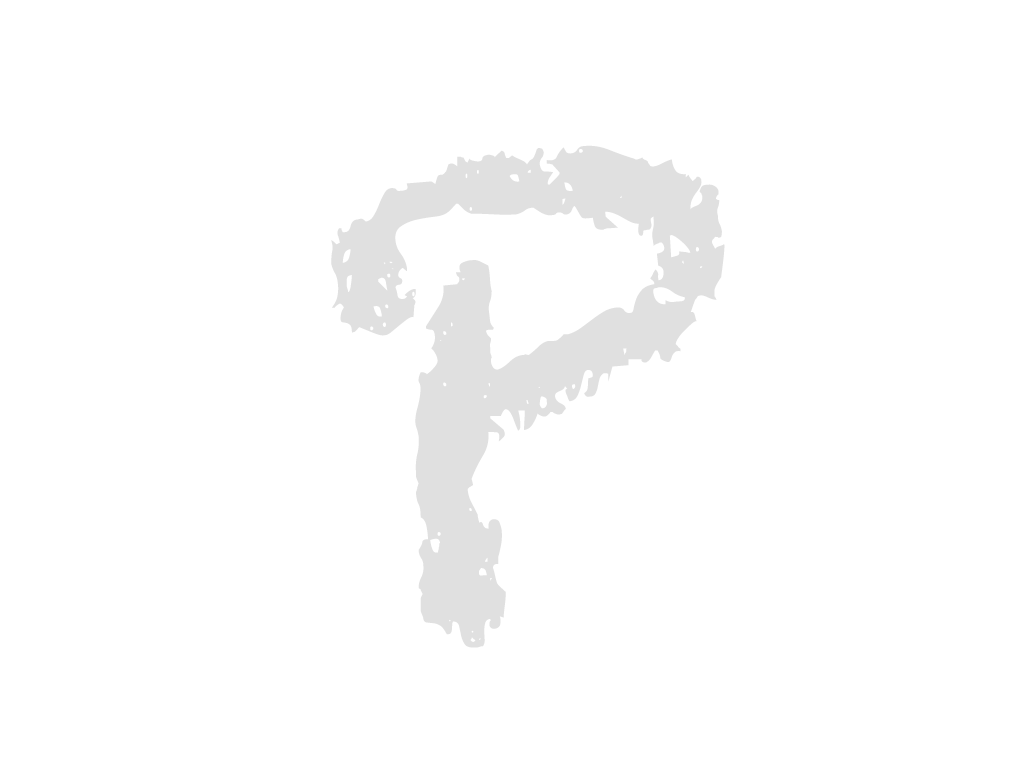
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
<template>
<div class="title-box flex justify-between mb40">
<p class="title">게시판</p>
<select v-model="sclsId" @change="handleClassId()">
<option
v-for="(item, index) in classList"
:key="index"
:value="item.sclsId"
>
{{ item.sclsNm }}
</option>
</select>
</div>
<div class="search-wrap flex justify-end mb20">
<select v-model="selectedSearchOption" class="mr10 data-wrap">
<option value="bbsTtl">제목</option>
<option value="bbsCnt">내용</option>
<option value="userNm">작성자</option>
<option value="bbsCls">카테고리</option>
</select>
<input
v-model="searchKeyword"
type="text"
placeholder="검색하세요."
@keyup.enter="boardDataSearch"
/>
<button type="button" @click="boardDataSearch()" title="게시글 검색">
<img src="../../../resources/img/look_t.png" alt="" />
</button>
</div>
<div class="table-wrap">
<table>
<thead>
<td>No.</td>
<td>제목</td>
<td>카테고리</td>
<td>작성자</td>
<td>등록일</td>
</thead>
<tbody>
<tr
v-for="(item, index) in dataList"
:key="item.id"
:class="{ 'selected-row': selectedRow == item.dataList }"
@click="[goToPage('noticeDetail'), selectBoardList(item)]"
>
<td>{{ createNo(index) }}</td>
<td>{{ item.bbsTtl }}</td>
<td>{{ item.bbsCls }}</td>
<td>{{ userNm }}</td>
<td>{{ item.bbsTm.substr(0, 16) }}</td>
</tr>
</tbody>
</table>
<article
class="table-pagination flex justify-center align-center mb20 mt30"
style="gap: 10px"
>
<button @click="goToPagination(currentPage - 1)">
<img src="../../../resources/img/btn27_90t_normal.png" alt="" />
</button>
<button
v-for="page in paginationButtons"
:key="page"
@click="goToPagination(page - 1)"
:class="{ 'selected-btn': currentPage === page - 1 }"
>
{{ page }}
</button>
<button @click="goToPagination(currentPage + 1)">
<img src="../../../resources/img/btn28_90t_normal.png" alt="" />
</button>
</article>
<div class="flex justify-end">
<button
type="button"
title="글쓰기"
class="new-btn"
@click="goToPage('noticeInsert')"
>
글쓰기
</button>
</div>
</div>
</template>
<script>
import SvgIcon from "@jamescoyle/vue-icon";
import { mdiMagnify } from "@mdi/js";
import axios from "axios";
export default {
data() {
return {
mdiMagnify: mdiMagnify,
// 게시글 정보
dataList: [],
totalPosts: 0,
selectedRow: "",
bbsTm: "",
// 페이징
currentPage: 0,
itemsPerPage: 8,
// 반 아이디 (추후 세션에서 받는걸로 수정)
sclsId: "",
classList: [],
userId: "",
// 검색어
searchKeyword: "",
selectedSearchOption: "bbsTtl",
selected: null,
};
},
methods: {
goToPage(page) {
this.$router.push({ name: page });
},
showConfirm(type) {
let message = "";
if (type === "cancel") {
message = "삭제하시겠습니까?";
} else if (type === "reset") {
message = "초기화하시겠습니까?";
} else if (type === "save") {
message = "등록하시겠습니까?";
}
if (confirm(message)) {
this.goBack();
}
},
// 게시글 전체 조회
boardList() {
const vm = this;
vm.sclsId = JSON.parse(sessionStorage.getItem("sclsId"));
axios({
url: "/board/findAll.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
page: vm.currentPage + 1,
pageSize: vm.itemsPerPage,
sclsId: vm.sclsId,
},
})
.then(function (res) {
console.log("dataList - response : ", res.data);
if (res.data.result.length !== 0) {
vm.dataList = res.data.result[0].boardClass[0].board;
vm.userNm = res.data.result[0].userNm;
vm.userId = res.data.result[0].userId;
vm.totalPosts = res.data.totalBoard;
vm.selectClass();
sessionStorage.removeItem("bbsId");
sessionStorage.removeItem("file");
} else {
vm.selectClass();
}
})
.catch(function (error) {
console.log("boardListError - error : ", error);
});
},
// 게시글 정보 세션에 저장
selectBoardList(item) {
sessionStorage.setItem("bbsId", JSON.stringify(item.bbsId));
},
// 반 아이디 세션에 저장
// 게시글 검색
boardDataSearch() {
const vm = this;
let searchPayload = {
keyword: vm.searchKeyword,
option: vm.selectedSearchOption,
page: vm.currentPage,
pageSize: vm.itemsPerPage,
sclsId: vm.sclsId,
};
axios({
url: "/board/search.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: searchPayload,
})
.then(function (res) {
console.log("boardDataSearch - response : ", res.data);
vm.dataList = res.data.result[0].boardClass[0].board;
vm.userNm = res.data.result[0].userNm;
vm.userId = res.data.result[0].userId;
vm.totalPosts = res.data.totalBoard;
})
.catch(function (error) {
console.log("dataSearch - error : ", error);
alert("게시글이 존재하지 않습니다.");
});
},
// 반 조회
selectClass() {
const vm = this;
axios({
url: "/classes/selectClass.json",
method: "post",
headers: {
"Content-Type": "application/json; charset=UTF-8",
},
data: {
userId: vm.userId,
},
})
.then(function (res) {
console.log("classData - response : ", res.data);
vm.classList = res.data.data;
vm.selected = res.data.data.sclsId;
})
.catch(function (error) {
console.log("classData - error : ", error);
});
},
handleClassId() {
sessionStorage.setItem("sclsId", JSON.stringify(this.sclsId));
this.boardList();
},
createNo(index) {
return this.totalPosts - (this.currentPage * this.itemsPerPage + index);
},
goToPagination(page) {
if (page < 0 || page >= this.totalPages) {
return;
}
this.currentPage = page;
this.boardList();
},
},
watch: {},
computed: {
totalPages() {
return Math.ceil(this.totalPosts / this.itemsPerPage);
},
paginationButtons() {
let start = Math.max(0, this.currentPage - 2);
let end = Math.min(start + 5, this.totalPages);
if (end - start < 5) {
start = Math.max(0, end - 5);
}
return Array.from({ length: end - start }, (_, i) => start + i + 1);
},
startIndex() {
return this.currentPage * this.itemsPerPage;
},
},
components: {
SvgIcon,
},
mounted() {
console.log("Main2 mounted");
this.boardList();
},
};
</script>