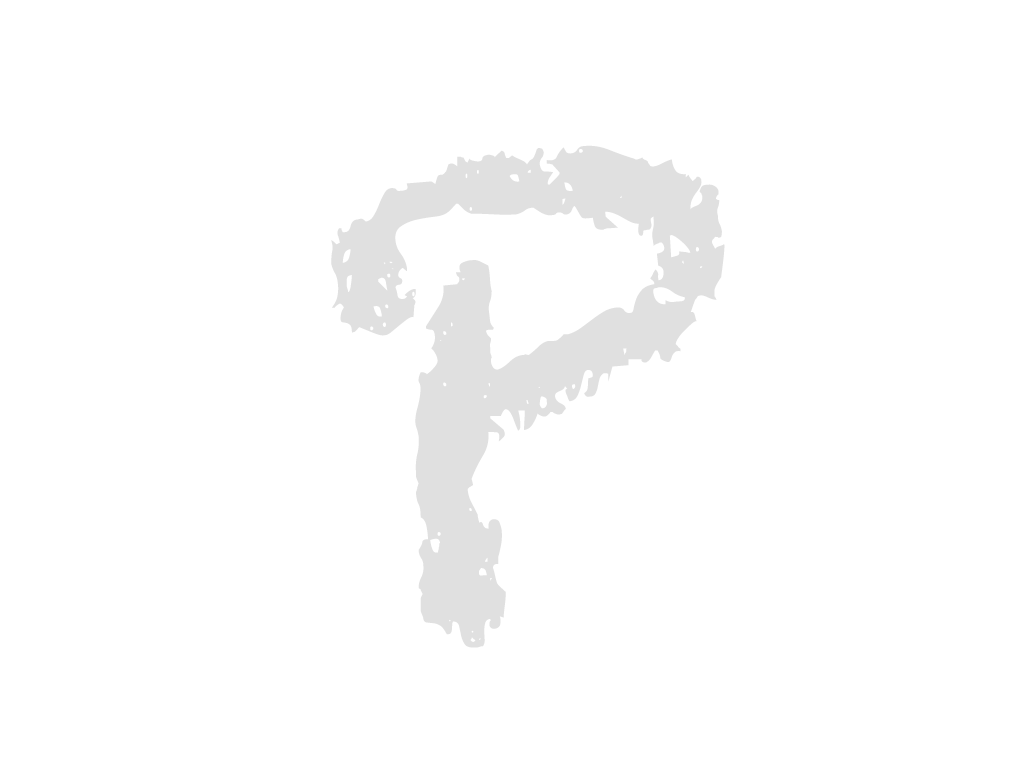
File name
Commit message
Commit date
File name
Commit message
Commit date
from flask_restx import Resource, Api, Namespace, fields,reqparse
from fog_model.fog_predict import fog_classfication
from fog_model.fog_model import darkchannel
from flask import request
from flask import Flask, render_template, request
from werkzeug.utils import secure_filename
import os
from haversine import haversine
from database.database import DB
import torch
from PIL import Image
from pothole_model.pothole import pothole
paths = os.getcwd()
Action = Namespace(
name="Action",
description="노드 분석을 위해 사용하는 api.",
)
def find_node(gps_address_y,gps_address_x):
db=DB()
nn_end = None
end_delta = float("inf")
value=0.0001
near_nodes=[]
while near_nodes==[]:
value=value*10
near_nodes=db.db_get_near_node(gps_address_y,gps_address_x,value)
for n in near_nodes:
e_dist = haversine((gps_address_x,gps_address_y), n)
if e_dist < end_delta :
nn_end = n
end_delta = e_dist
return nn_end
@Action.route('/image_summit')
class fileUpload(Resource):
@Action.doc(responses={200: 'Success'})
@Action.doc(responses={500: 'Register Failed'})
def post(self):
if request.method == 'POST':
f = request.files['file']
f.save(secure_filename(f.filename))
return {
'save': 'done' # str으로 반환하여 return
}, 200
@Action.route('/image_anal')
class fileUpload(Resource):
@Action.doc(responses={200: 'Success'})
@Action.doc(responses={500: 'Register Failed'})
def post(self):
if request.method == 'POST':
fc = fog_classfication()
dir = os.getcwd()
filename = request.json['filename']
file_type = request.json['file_type']
gps_address_x = float(request.json['gps_x'])
gps_address_y = float(request.json['gps_y'])
total_path = dir+ "\\"+ filename + file_type
model_fc= fc.predict(total_path)
model_yolo= torch.hub.load(paths +'/yolov5/', 'custom', path=paths+'/yolov5/best.pt', source='local')
im = Image.open(total_path)
results = model_yolo(im)
li_detect=list(results.pandas().xyxy[0]['name'])
if model_fc == "normal":
if 'vest' in li_detect and 'cone' in li_detect:
nn_end = find_node(gps_address_y,gps_address_x)
return {
'node': nn_end,
'fog' : 'normal',
'construction' : 'construction'
}, 200
else:
return {
'node': None,
'fog' : 'normal',
'construction' : 'normal'
}, 200
else:
if 'vest' in li_detect and 'cone' in li_detect:
nn_end = find_node(gps_address_y,gps_address_x)
return {
'node': nn_end,
'fog' : 'fog',
'construction' : 'construction'
}, 200
else:
nn_end = find_node(gps_address_y,gps_address_x)
return {
'node': nn_end,
'fog' : 'fog',
'construction' : 'normal'
}, 200
@Action.route('/pothole_report')
class fileUpload(Resource):
@Action.doc(responses={200: 'Success'})
@Action.doc(responses={500: 'Register Failed'})
def post(self):
if request.method == 'POST':
pc = pothole()
dir = os.getcwd()
report_id = request.json['report_id']
pothole_id = request.json['pothole_id']
pothole_x = float(request.json['pothole_x'])
pothole_y = float(request.json['pothole_y'])
z = float(request.json['z'])
pc.report(report_id,pothole_id,pothole_x,pothole_y,z)
return {
'report': 'done' # str으로 반환하여 return
}, 200
@Action.route('/pothole_display')
class fileUpload(Resource):
@Action.doc(responses={200: 'Success'})
@Action.doc(responses={500: 'Register Failed'})
def post(self):
if request.method == 'POST':
pc = pothole()
dir = os.getcwd()
timestamp = request.json['timestamp']
value=pc.display(timestamp)
return {
'pothole': list(value) # str으로 반환하여 return
}, 200