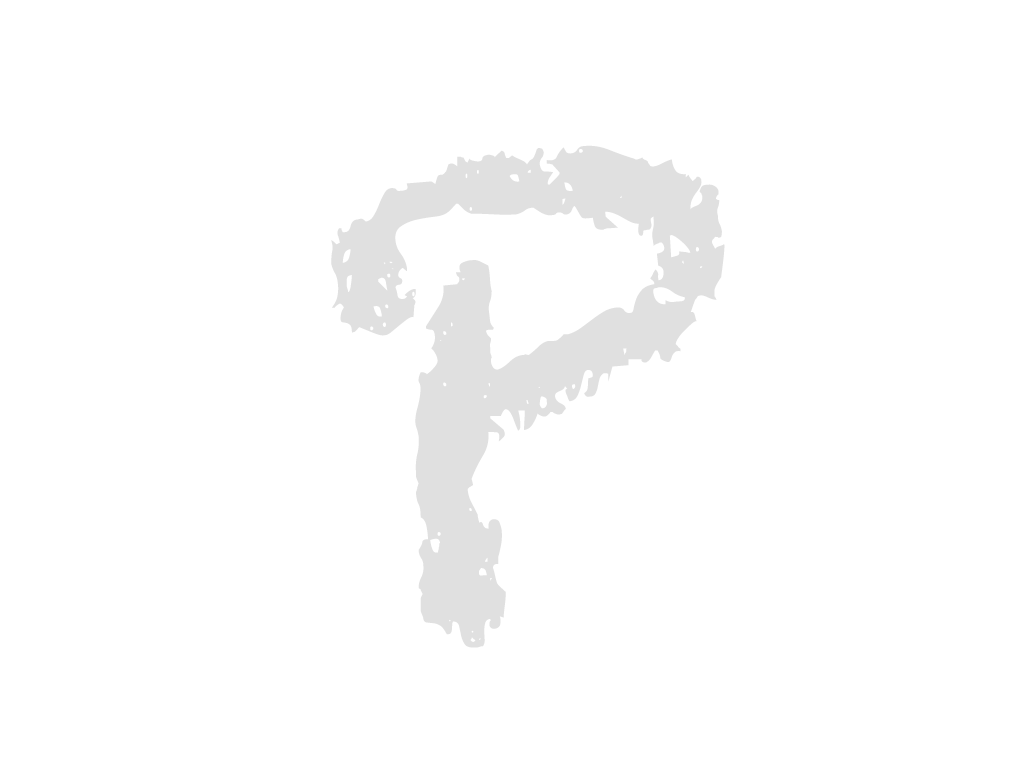
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React, {useCallback, useRef, useState} from 'react';
import {useCameraDevices, Camera} from 'react-native-vision-camera';
import RNFS from 'react-native-fs';
import {url} from '../url';
import ModalComponent from './ModalComponent';
export default function Action() {
const devices = useCameraDevices();
const device = devices.back;
const camera = useRef(null);
const [isModalVisible, setModalVisible] = useState(false);
const toggleModal = () => {
setModalVisible(!isModalVisible);
};
const requestCameraPermission = useCallback(async () => {
const permission = await Camera.requestCameraPermission();
if (permission === 'denied') await Linking.openSettings();
}, []);
const takePicture = async () => {
const {path} = await camera.current.takePhoto({
flash: 'off',
qualityPrioritization: 'speed', //'quality',
});
let pathSplit = path.split('/');
let pathSplitLength = pathSplit.length;
let fileName = pathSplit[pathSplitLength - 1]; //확장자까지 붙은 파일명
let splitFileName = fileName.split('.'); // 파일명을 다시 "." 로 나누면 파일이름과 확장자로 나뉜다
let sFileName = splitFileName[0]; // 파일이름
let files = [
{
name: 'file',
filename: fileName,
filepath: path,
filetype: 'image/jpg',
},
];
//이미지 서버 전송
let upload = response => {
let jobId = response.jobId;
console.log('UPLOAD HAS BEGUN! JobId: ' + jobId);
};
let uploadProgress = response => {
let percentage = Math.floor(
(response.totalBytesSent / response.totalBytesExpectedToSend) * 100,
);
console.log('UPLOAD IS ' + percentage + '% DONE!');
};
await RNFS.uploadFiles({
toUrl: `${url}/action/image_summit`,
files: files,
method: 'POST',
headers: {},
begin: upload,
progress: uploadProgress,
})
.promise.then(response => {
if (response.statusCode != 200) {
throw 'SERVER ERROR';
}
console.log('FILES UPLOADED!');
//이미지 분석 요청
return fetch(`${url}/action/image_anal`, {
method: 'POST',
headers: {
'Content-Type': 'application/json; charset=UTF-8',
},
body: JSON.stringify({
filename: sFileName,
file_type: '.jpg',
gps_x: location.latitude,
gps_y: location.longitude,
}),
});
})
.then(response => response.json())
.then(data => {
console.log('fileSend response data : ', data);
const {node, fog} = data;
if (node === null || fog !== 'fog') {
throw 'no data';
}
onUploadFile(node);
toggleModal();
// 분석 결과 node remove 요청
return fetch(`${url}/trip/remove`, {
method: 'POST',
headers: {
'Content-Type': 'application/json; charset=UTF-8',
},
body: JSON.stringify({
gps_x: node[0],
gps_y: node[1],
}),
});
})
.then(response => response.json())
.then(data => {
console.log('remove data', data);
if (data.done === 'done') {
return;
}
})
.catch(err => {
console.log(err);
});
};
useEffect(() => {
requestCameraPermission();
timerId = setInterval(() => {
takePicture();
}, 5000);
return () => clearInterval(timerId);
}, []);
if (device == null) return <Text>device == null</Text>;
return (
<View>
<Camera ref={camera} device={device} isActive={true} photo={true} />
<ModalComponent
isVisible={isModalVisible}
toggleModal={toggleModal}
alertTitle="안개 발생 알림"
alertMessage="전방에 안개가 발생하였습니다."
/>
</View>
);
}