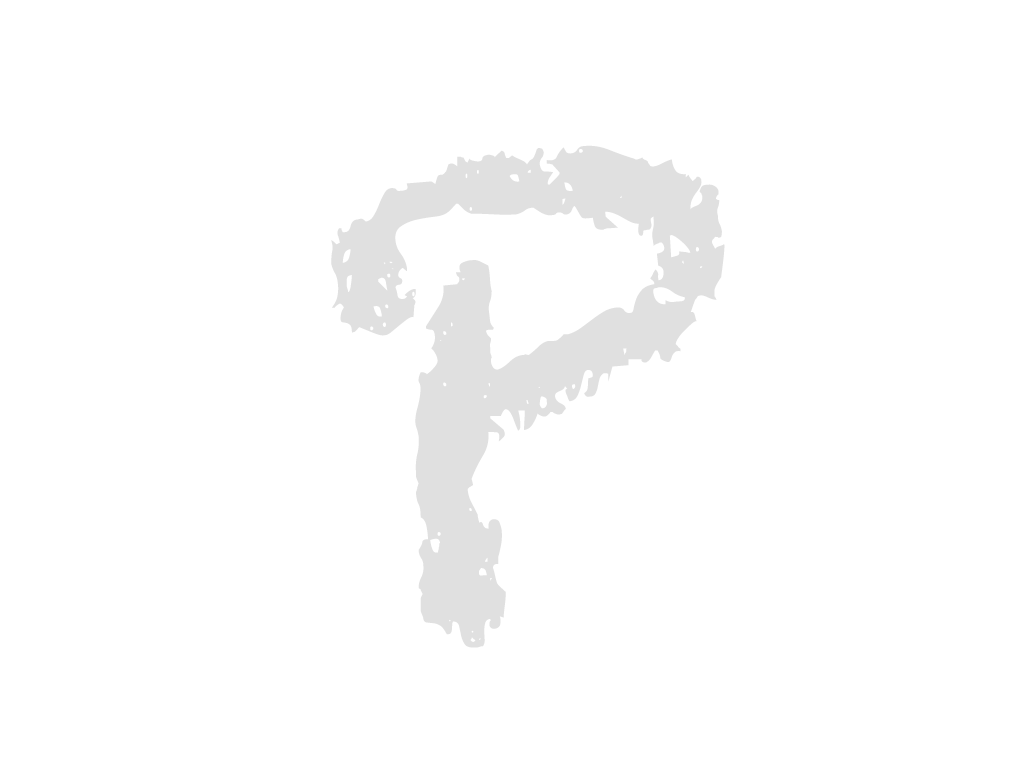
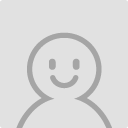
[FIX] driving문제 수정
@10fed03f31cf73eca8b8ad8b6781130d31b9e7d5
--- src/component/Map.js
+++ src/component/Map.js
... | ... | @@ -45,9 +45,6 @@ |
45 | 45 |
|
46 | 46 |
return ( |
47 | 47 |
<View style={styles.mapStyle}> |
48 |
- <View style={styles.searchContainer}> |
|
49 |
- <Search placeholder={'도착지를 입력하세요'} /> |
|
50 |
- </View> |
|
51 | 48 |
<MapView |
52 | 49 |
style={styles.mapStyle} |
53 | 50 |
provider={PROVIDER_GOOGLE} |
... | ... | @@ -70,7 +67,7 @@ |
70 | 67 |
/> |
71 | 68 |
))} |
72 | 69 |
</MapView> |
73 |
- <View style={styles.buttonstyle}> |
|
70 |
+ {/* <View style={styles.buttonstyle}> |
|
74 | 71 |
<View style={styles.markerButtonStyle}> |
75 | 72 |
<Button |
76 | 73 |
title={'마커 표시'} |
... | ... | @@ -82,7 +79,7 @@ |
82 | 79 |
backgroundColor={PRIMERY} |
83 | 80 |
/> |
84 | 81 |
</View> |
85 |
- </View> |
|
82 |
+ </View> */} |
|
86 | 83 |
</View> |
87 | 84 |
); |
88 | 85 |
} |
... | ... | @@ -97,14 +94,7 @@ |
97 | 94 |
mapStyle: { |
98 | 95 |
flex: 1, |
99 | 96 |
}, |
100 |
- searchContainer: { |
|
101 |
- paddingTop: 10, |
|
102 |
- flexDirection: 'row', |
|
103 |
- width: '100%', |
|
104 |
- justifyContent: 'center', |
|
105 |
- alignItems: 'center', |
|
106 |
- paddingHorizontal: 5, |
|
107 |
- }, |
|
97 |
+ |
|
108 | 98 |
buttonstyle: { |
109 | 99 |
marginHorizontal: 15, |
110 | 100 |
}, |
--- src/component/Photo.js
+++ src/component/Photo.js
... | ... | @@ -10,6 +10,7 @@ |
10 | 10 |
import RNFS from 'react-native-fs'; |
11 | 11 |
import {url} from '../url'; |
12 | 12 |
import ModalComponent from './ModalComponent'; |
13 |
+import {MapContext} from '../context/MapContext'; |
|
13 | 14 |
|
14 | 15 |
export default function Photo({onUploadFile}) { |
15 | 16 |
const {onQuery, location} = useContext(MapContext); |
--- src/context/MapContext.js
+++ src/context/MapContext.js
... | ... | @@ -1,6 +1,14 @@ |
1 | 1 |
import React, {createContext, useState, useEffect} from 'react'; |
2 | 2 |
import Geolocation from 'react-native-geolocation-service'; |
3 |
-import {Platform, PermissionsAndroid} from 'react-native'; |
|
3 |
+import {Platform, PermissionsAndroid, Alert} from 'react-native'; |
|
4 |
+import {url} from '../url'; |
|
5 |
+// import {accelerometer} from 'react-native-sensors'; |
|
6 |
+import { |
|
7 |
+ setUpdateIntervalForType, |
|
8 |
+ SensorTypes, |
|
9 |
+ accelerometer, |
|
10 |
+} from 'react-native-sensors'; |
|
11 |
+import {latLng} from 'leaflet'; |
|
4 | 12 |
|
5 | 13 |
export const MapContext = createContext(); |
6 | 14 |
|
... | ... | @@ -24,6 +32,7 @@ |
24 | 32 |
//현위치, marker 위치 검색내용 state |
25 | 33 |
const [location, setLocation] = useState(); |
26 | 34 |
const [searchQuery, setSearchQuery] = useState(null); |
35 |
+ const [isAccelerometerActive, setIsAccelerometerActive] = useState(false); // 상태 추가 |
|
27 | 36 |
|
28 | 37 |
//backedn 받아온 node |
29 | 38 |
const [startPoint, setStartPoint] = useState(); |
... | ... | @@ -55,10 +64,84 @@ |
55 | 64 |
}, []); |
56 | 65 |
|
57 | 66 |
//두좌표 거리 구하는 함수 |
67 |
+ function getDistance(lat1, lon1, lat2, lon2) { |
|
68 |
+ if (lat1 == lat2 && lon1 == lon2) return 0; |
|
69 |
+ |
|
70 |
+ var radLat1 = (Math.PI * lat1) / 180; |
|
71 |
+ var radLat2 = (Math.PI * lat2) / 180; |
|
72 |
+ var theta = lon1 - lon2; |
|
73 |
+ var radTheta = (Math.PI * theta) / 180; |
|
74 |
+ var dist = |
|
75 |
+ Math.sin(radLat1) * Math.sin(radLat2) + |
|
76 |
+ Math.cos(radLat1) * Math.cos(radLat2) * Math.cos(radTheta); |
|
77 |
+ if (dist > 1) dist = 1; |
|
78 |
+ |
|
79 |
+ dist = Math.acos(dist); |
|
80 |
+ dist = (dist * 180) / Math.PI; |
|
81 |
+ dist = dist * 60 * 1.1515 * 1.609344 * 1000; |
|
82 |
+ if (dist < 100) dist = Math.round(dist / 10) * 10; |
|
83 |
+ else dist = Math.round(dist / 100) * 100; |
|
84 |
+ |
|
85 |
+ return dist; |
|
86 |
+ } |
|
58 | 87 |
|
59 | 88 |
//목적지 send |
60 | 89 |
const onQuery = query => { |
90 |
+ setSearchQuery(query); |
|
61 | 91 |
const {latitude, longitude} = location; |
92 |
+ return fetch(`${url}/trip/trip2`, { |
|
93 |
+ method: 'POST', |
|
94 |
+ headers: { |
|
95 |
+ 'Content-Type': 'application/json; charset=UTF-8', |
|
96 |
+ }, |
|
97 |
+ body: JSON.stringify({ |
|
98 |
+ dest1_x: latitude, |
|
99 |
+ dest1_y: longitude, |
|
100 |
+ path_end: query, |
|
101 |
+ }), |
|
102 |
+ }) |
|
103 |
+ .then(response => response.json()) |
|
104 |
+ .then(data => { |
|
105 |
+ const nodes = data.nodes; |
|
106 |
+ const start_point = data.start_point; |
|
107 |
+ const end_point = data.end_point; |
|
108 |
+ |
|
109 |
+ //end_point obj 변환 |
|
110 |
+ let endPointObj = {}; |
|
111 |
+ endPointObj.latitude = end_point[0]; |
|
112 |
+ endPointObj.longitude = end_point[1]; |
|
113 |
+ setEndPoint(endPointObj); |
|
114 |
+ //nodes obj 변환 |
|
115 |
+ let nodesArr = nodes; |
|
116 |
+ let resultNodes = nodesArr.map(i => { |
|
117 |
+ let nodesObj = {}; |
|
118 |
+ nodesObj.latitude = i[0]; |
|
119 |
+ nodesObj.longitude = i[1]; |
|
120 |
+ return nodesObj; |
|
121 |
+ }); |
|
122 |
+ setNodes(resultNodes); |
|
123 |
+ //start_point obj 변환 |
|
124 |
+ let startPointObj = {}; |
|
125 |
+ startPointObj.latitude = start_point[0]; |
|
126 |
+ startPointObj.longitude = start_point[1]; |
|
127 |
+ setStartPoint(startPointObj); |
|
128 |
+ // console.log('resultNodes', resultNodes); |
|
129 |
+ //nodes total km |
|
130 |
+ let total = []; |
|
131 |
+ for (let i = 0; i < resultNodes.length - 1; i++) { |
|
132 |
+ let km = getDistance( |
|
133 |
+ resultNodes[i].latitude, |
|
134 |
+ resultNodes[i].longitude, |
|
135 |
+ resultNodes[i + 1].latitude, |
|
136 |
+ resultNodes[i + 1].longitude, |
|
137 |
+ ); |
|
138 |
+ total.push(km); |
|
139 |
+ } |
|
140 |
+ const totalKm = total.reduce((acc, curr) => acc + curr, 0); |
|
141 |
+ const resultKm = totalKm / 1000; |
|
142 |
+ setKm(resultKm); |
|
143 |
+ return resultNodes; |
|
144 |
+ }); |
|
62 | 145 |
}; |
63 | 146 |
|
64 | 147 |
const value = React.useMemo(() => ({ |
--- src/screens/DestinationList.js
+++ src/screens/DestinationList.js
... | ... | @@ -40,7 +40,7 @@ |
40 | 40 |
<Polyline coordinates={nodes} strokeWidth={10} strokeColor={PRIMERY} /> |
41 | 41 |
</Map> |
42 | 42 |
<BottomDrop> |
43 |
- <View style={directionAlign}> |
|
43 |
+ <View style={[directionAlign, {flexDirection: 'row'}]}> |
|
44 | 44 |
<Text style={{flex: 9}}>{searchQuery}</Text> |
45 | 45 |
<Button |
46 | 46 |
title={'운행 시작'} |
--- src/screens/Main.js
+++ src/screens/Main.js
... | ... | @@ -14,6 +14,7 @@ |
14 | 14 |
import {url} from '../url'; |
15 | 15 |
import {PRIMERY, RED, WHITE} from '../color'; |
16 | 16 |
import ModalComponent from '../component/ModalComponent'; |
17 |
+import Search from '../component/Search'; |
|
17 | 18 |
|
18 | 19 |
export default function Main({navigation}) { |
19 | 20 |
const {onQuery, searchQuery, location, nodes} = useContext(MapContext); |
... | ... | @@ -161,6 +162,9 @@ |
161 | 162 |
|
162 | 163 |
return ( |
163 | 164 |
<View style={{flex: 1}}> |
165 |
+ <View style={styles.searchContainer}> |
|
166 |
+ <Search placeholder={'도착지를 입력하세요'} /> |
|
167 |
+ </View> |
|
164 | 168 |
<Map |
165 | 169 |
lat={location.latitude} |
166 | 170 |
long={location.longitude} |
... | ... | @@ -208,7 +212,7 @@ |
208 | 212 |
|
209 | 213 |
const styles = StyleSheet.create({ |
210 | 214 |
container: { |
211 |
- width: '45%', |
|
215 |
+ width: '90%', |
|
212 | 216 |
// marginLeft: 5, |
213 | 217 |
// justifyContent: 'center', |
214 | 218 |
// alignItems: 'center', |
... | ... | @@ -216,6 +220,14 @@ |
216 | 220 |
bottom: 7, |
217 | 221 |
right: 15, |
218 | 222 |
}, |
223 |
+ searchContainer: { |
|
224 |
+ paddingTop: 10, |
|
225 |
+ flexDirection: 'row', |
|
226 |
+ width: '100%', |
|
227 |
+ justifyContent: 'center', |
|
228 |
+ alignItems: 'center', |
|
229 |
+ paddingHorizontal: 5, |
|
230 |
+ }, |
|
219 | 231 |
infoContainer: { |
220 | 232 |
marginTop: 16, |
221 | 233 |
justifyContent: 'center', |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?