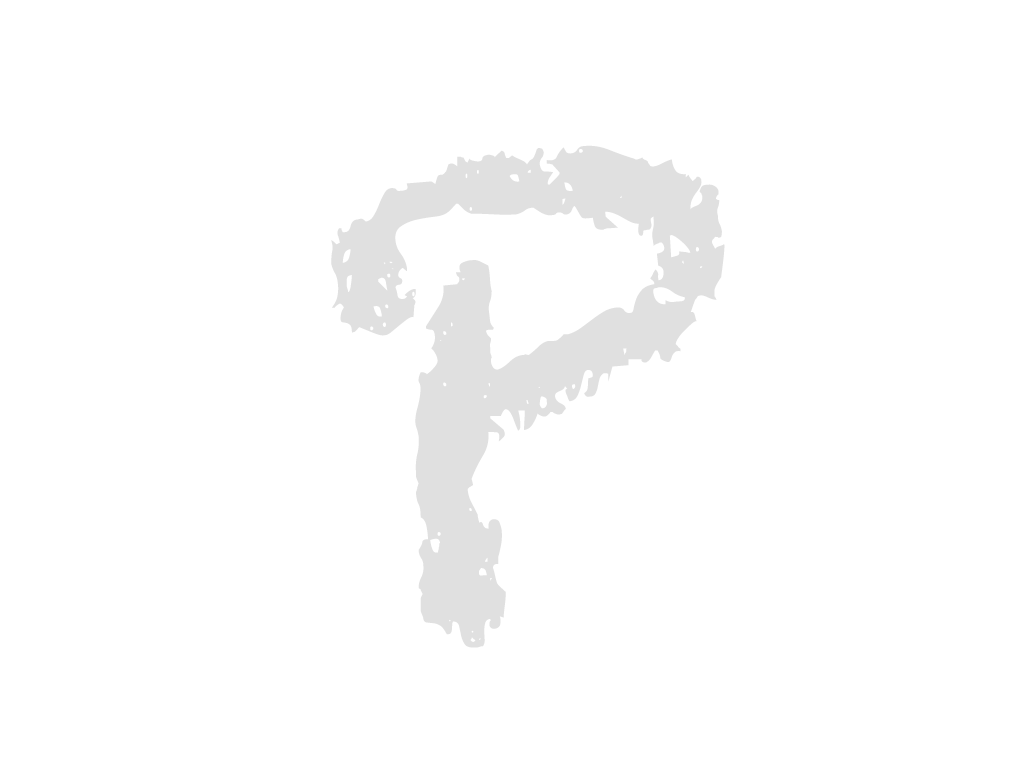
--- tools/algo/ANOVA.py
+++ tools/algo/ANOVA.py
... | ... | @@ -6,6 +6,7 @@ |
6 | 6 |
from tools.algo.humidity import absolute_humidity |
7 | 7 |
from tools.algo.interpolation import interpolate_value |
8 | 8 |
from datetime import datetime |
9 |
+import plotly.express as px |
|
9 | 10 |
|
10 | 11 |
if __name__ == "__main__": |
11 | 12 |
df_weather = pd.read_csv("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308310000_f.csv") |
... | ... | @@ -30,5 +31,8 @@ |
30 | 31 |
|
31 | 32 |
F_statistic, pVal = stats.f_oneway(df_failure[df_failure["불량 여부"] == 0].loc[:,['상대습도', '기온', '절대습도']], |
32 | 33 |
df_failure[df_failure["불량 여부"] == 1].loc[:,['상대습도', '기온', '절대습도']]) |
33 |
- |
|
34 |
- print(F_statistic, pVal)(파일 끝에 줄바꿈 문자 없음) |
|
34 |
+ fig_df = pd.DataFrame(np.transpose([F_statistic, pVal]), index=['상대습도', '기온', '절대습도'], columns=["F_", "pVal"]) |
|
35 |
+ fig = px.scatter(fig_df) |
|
36 |
+ fig.add_hline(y=0.05, line_dash="dash", line_color="red") |
|
37 |
+ fig.show() |
|
38 |
+ print(fig_df)(파일 끝에 줄바꿈 문자 없음) |
--- tools/algo/SARIMA.py
+++ tools/algo/SARIMA.py
... | ... | @@ -1,9 +1,12 @@ |
1 | 1 |
import pandas as pd |
2 |
+import numpy as np |
|
2 | 3 |
from statsmodels.tsa.statespace.sarimax import SARIMAX |
4 |
+from statsmodels.graphics.tsaplots import plot_acf, plot_pacf |
|
3 | 5 |
import matplotlib.pyplot as plt |
4 | 6 |
from datetime import datetime, timedelta |
5 | 7 |
import plotly.express as px |
6 | 8 |
from tools.algo.humidity import absolute_humidity |
9 |
+import pickle |
|
7 | 10 |
|
8 | 11 |
|
9 | 12 |
def sarima(file, col_key='상대습도', future_hours=24): |
... | ... | @@ -34,6 +37,30 @@ |
34 | 37 |
|
35 | 38 |
forecast_df.to_csv(f"{file.split('.')[0]}_forecast.csv", index=False) |
36 | 39 |
|
40 |
+ with open('sarima_model.pkl', 'wb') as pkl_file: |
|
41 |
+ pickle.dump(model_fit, pkl_file) |
|
42 |
+ |
|
43 |
+def forecast_from_saved_model(file, future_hours=24): |
|
44 |
+ # Load the saved model |
|
45 |
+ with open('sarima_model.pkl', 'rb') as pkl_file: |
|
46 |
+ loaded_model = pickle.load(pkl_file) |
|
47 |
+ |
|
48 |
+ df = pd.read_csv(file) |
|
49 |
+ |
|
50 |
+ df['관측시각'] = df['관측시각'].apply(lambda x: datetime.strptime(f"{x}", '%Y%m%d%H%M')) |
|
51 |
+ |
|
52 |
+ # Forecast the next 'future_hours' using the loaded model |
|
53 |
+ forecast_values = loaded_model.forecast(steps=future_hours) |
|
54 |
+ forecast_index = [df['관측시각'].iloc[-1] + timedelta(hours=i) for i in range(1, future_hours + 1)] |
|
55 |
+ forecast_df = pd.DataFrame({ |
|
56 |
+ '관측시각': forecast_index, |
|
57 |
+ 'forecast': forecast_values |
|
58 |
+ }) |
|
59 |
+ |
|
60 |
+ forecast_df.to_csv(f"{file.split('.')[0]}_forecast.csv", index=False) |
|
61 |
+ |
|
62 |
+ return forecast_df |
|
63 |
+ |
|
37 | 64 |
if __name__ == "__main__": |
38 | 65 |
df = pd.read_csv("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308310000_f.csv") |
39 | 66 |
ah = absolute_humidity(df["상대습도"], df["기온"]) |
... | ... | @@ -49,13 +76,13 @@ |
49 | 76 |
# df["절대습도"],72,3) |
50 | 77 |
# )) |
51 | 78 |
# fig.show() |
52 |
- # log_df = np.log(df["절대습도"]) |
|
53 |
- diff_1 = (df["절대습도"].diff(periods=1).iloc[1:]) |
|
79 |
+ log_df = np.log(df["상대습도"]) |
|
80 |
+ diff_1 = (log_df.diff(periods=1).iloc[1:]) |
|
54 | 81 |
diff_2 = diff_1.diff(periods=1).iloc[1:] |
55 |
- # plot_acf(diff_2) |
|
56 |
- # plot_pacf(diff_2) |
|
82 |
+ plot_acf(diff_2) |
|
83 |
+ plot_pacf(diff_2) |
|
57 | 84 |
plt.show() |
58 |
- model = SARIMAX(df["절대습도"], order=(1,0,2), seasonal_order=(0,1,2,24)) |
|
85 |
+ model = SARIMAX(df["상대습도"], order=(2,0,2), seasonal_order=(1,1,2,24)) |
|
59 | 86 |
model_fit = model.fit() |
60 | 87 |
# ARIMA_model = pm.auto_arima(df['절대습도'], |
61 | 88 |
# start_p=1, |
... | ... | @@ -73,5 +100,5 @@ |
73 | 100 |
print(model_fit.summary()) |
74 | 101 |
df['forecast'] = model_fit.predict(start=-100, end=-1, dynamic=True) |
75 | 102 |
# df[['절대습도', 'forecast']].plot(figsize=(12, 8)) |
76 |
- fig = px.line(df[['절대습도', 'forecast']]) |
|
103 |
+ fig = px.line(df[['상대습도', 'forecast']]) |
|
77 | 104 |
fig.show()(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?